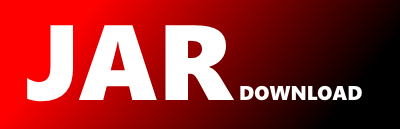
microsoft.dynamics.crm.entity.EntityNameAttributeMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.complex.AttributeRequiredLevelManagedProperty;
import microsoft.dynamics.crm.complex.AttributeTypeDisplayName;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.complex.Label;
import microsoft.dynamics.crm.enums.AttributeTypeCode;
@JsonPropertyOrder({
"@odata.type",
"IsEntityReferenceStored"})
@JsonInclude(Include.NON_NULL)
public class EntityNameAttributeMetadata extends EnumAttributeMetadata implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.EntityNameAttributeMetadata";
}
@JsonProperty("IsEntityReferenceStored")
protected Boolean isEntityReferenceStored;
protected EntityNameAttributeMetadata() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEntityNameAttributeMetadata() {
return new Builder();
}
public static final class Builder {
private String metadataId;
private Boolean hasChanged;
private String attributeOf;
private AttributeTypeCode attributeType;
private AttributeTypeDisplayName attributeTypeName;
private Integer columnNumber;
private Label description;
private Label displayName;
private String deprecatedVersion;
private String introducedVersion;
private String entityLogicalName;
private BooleanManagedProperty isAuditEnabled;
private Boolean isCustomAttribute;
private Boolean isPrimaryId;
private Boolean isValidODataAttribute;
private Boolean isPrimaryName;
private Boolean isValidForCreate;
private Boolean isValidForRead;
private Boolean isValidForUpdate;
private Boolean canBeSecuredForRead;
private Boolean canBeSecuredForCreate;
private Boolean canBeSecuredForUpdate;
private Boolean isSecured;
private Boolean isRetrievable;
private Boolean isFilterable;
private Boolean isSearchable;
private Boolean isManaged;
private BooleanManagedProperty isGlobalFilterEnabled;
private BooleanManagedProperty isSortableEnabled;
private String linkedAttributeId;
private String logicalName;
private BooleanManagedProperty isCustomizable;
private BooleanManagedProperty isRenameable;
private BooleanManagedProperty isValidForAdvancedFind;
private Boolean isValidForForm;
private Boolean isRequiredForForm;
private Boolean isValidForGrid;
private AttributeRequiredLevelManagedProperty requiredLevel;
private BooleanManagedProperty canModifyAdditionalSettings;
private String schemaName;
private String externalName;
private Boolean isLogical;
private Boolean isDataSourceSecret;
private String inheritsFrom;
private OffsetDateTime createdOn;
private OffsetDateTime modifiedOn;
private Integer sourceType;
private String autoNumberFormat;
private Integer defaultFormValue;
private Boolean isEntityReferenceStored;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder metadataId(String metadataId) {
this.metadataId = metadataId;
this.changedFields = changedFields.add("MetadataId");
return this;
}
public Builder hasChanged(Boolean hasChanged) {
this.hasChanged = hasChanged;
this.changedFields = changedFields.add("HasChanged");
return this;
}
public Builder attributeOf(String attributeOf) {
this.attributeOf = attributeOf;
this.changedFields = changedFields.add("AttributeOf");
return this;
}
public Builder attributeType(AttributeTypeCode attributeType) {
this.attributeType = attributeType;
this.changedFields = changedFields.add("AttributeType");
return this;
}
public Builder attributeTypeName(AttributeTypeDisplayName attributeTypeName) {
this.attributeTypeName = attributeTypeName;
this.changedFields = changedFields.add("AttributeTypeName");
return this;
}
public Builder columnNumber(Integer columnNumber) {
this.columnNumber = columnNumber;
this.changedFields = changedFields.add("ColumnNumber");
return this;
}
public Builder description(Label description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder displayName(Label displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder deprecatedVersion(String deprecatedVersion) {
this.deprecatedVersion = deprecatedVersion;
this.changedFields = changedFields.add("DeprecatedVersion");
return this;
}
public Builder introducedVersion(String introducedVersion) {
this.introducedVersion = introducedVersion;
this.changedFields = changedFields.add("IntroducedVersion");
return this;
}
public Builder entityLogicalName(String entityLogicalName) {
this.entityLogicalName = entityLogicalName;
this.changedFields = changedFields.add("EntityLogicalName");
return this;
}
public Builder isAuditEnabled(BooleanManagedProperty isAuditEnabled) {
this.isAuditEnabled = isAuditEnabled;
this.changedFields = changedFields.add("IsAuditEnabled");
return this;
}
public Builder isCustomAttribute(Boolean isCustomAttribute) {
this.isCustomAttribute = isCustomAttribute;
this.changedFields = changedFields.add("IsCustomAttribute");
return this;
}
public Builder isPrimaryId(Boolean isPrimaryId) {
this.isPrimaryId = isPrimaryId;
this.changedFields = changedFields.add("IsPrimaryId");
return this;
}
public Builder isValidODataAttribute(Boolean isValidODataAttribute) {
this.isValidODataAttribute = isValidODataAttribute;
this.changedFields = changedFields.add("IsValidODataAttribute");
return this;
}
public Builder isPrimaryName(Boolean isPrimaryName) {
this.isPrimaryName = isPrimaryName;
this.changedFields = changedFields.add("IsPrimaryName");
return this;
}
public Builder isValidForCreate(Boolean isValidForCreate) {
this.isValidForCreate = isValidForCreate;
this.changedFields = changedFields.add("IsValidForCreate");
return this;
}
public Builder isValidForRead(Boolean isValidForRead) {
this.isValidForRead = isValidForRead;
this.changedFields = changedFields.add("IsValidForRead");
return this;
}
public Builder isValidForUpdate(Boolean isValidForUpdate) {
this.isValidForUpdate = isValidForUpdate;
this.changedFields = changedFields.add("IsValidForUpdate");
return this;
}
public Builder canBeSecuredForRead(Boolean canBeSecuredForRead) {
this.canBeSecuredForRead = canBeSecuredForRead;
this.changedFields = changedFields.add("CanBeSecuredForRead");
return this;
}
public Builder canBeSecuredForCreate(Boolean canBeSecuredForCreate) {
this.canBeSecuredForCreate = canBeSecuredForCreate;
this.changedFields = changedFields.add("CanBeSecuredForCreate");
return this;
}
public Builder canBeSecuredForUpdate(Boolean canBeSecuredForUpdate) {
this.canBeSecuredForUpdate = canBeSecuredForUpdate;
this.changedFields = changedFields.add("CanBeSecuredForUpdate");
return this;
}
public Builder isSecured(Boolean isSecured) {
this.isSecured = isSecured;
this.changedFields = changedFields.add("IsSecured");
return this;
}
public Builder isRetrievable(Boolean isRetrievable) {
this.isRetrievable = isRetrievable;
this.changedFields = changedFields.add("IsRetrievable");
return this;
}
public Builder isFilterable(Boolean isFilterable) {
this.isFilterable = isFilterable;
this.changedFields = changedFields.add("IsFilterable");
return this;
}
public Builder isSearchable(Boolean isSearchable) {
this.isSearchable = isSearchable;
this.changedFields = changedFields.add("IsSearchable");
return this;
}
public Builder isManaged(Boolean isManaged) {
this.isManaged = isManaged;
this.changedFields = changedFields.add("IsManaged");
return this;
}
public Builder isGlobalFilterEnabled(BooleanManagedProperty isGlobalFilterEnabled) {
this.isGlobalFilterEnabled = isGlobalFilterEnabled;
this.changedFields = changedFields.add("IsGlobalFilterEnabled");
return this;
}
public Builder isSortableEnabled(BooleanManagedProperty isSortableEnabled) {
this.isSortableEnabled = isSortableEnabled;
this.changedFields = changedFields.add("IsSortableEnabled");
return this;
}
public Builder linkedAttributeId(String linkedAttributeId) {
this.linkedAttributeId = linkedAttributeId;
this.changedFields = changedFields.add("LinkedAttributeId");
return this;
}
public Builder logicalName(String logicalName) {
this.logicalName = logicalName;
this.changedFields = changedFields.add("LogicalName");
return this;
}
public Builder isCustomizable(BooleanManagedProperty isCustomizable) {
this.isCustomizable = isCustomizable;
this.changedFields = changedFields.add("IsCustomizable");
return this;
}
public Builder isRenameable(BooleanManagedProperty isRenameable) {
this.isRenameable = isRenameable;
this.changedFields = changedFields.add("IsRenameable");
return this;
}
public Builder isValidForAdvancedFind(BooleanManagedProperty isValidForAdvancedFind) {
this.isValidForAdvancedFind = isValidForAdvancedFind;
this.changedFields = changedFields.add("IsValidForAdvancedFind");
return this;
}
public Builder isValidForForm(Boolean isValidForForm) {
this.isValidForForm = isValidForForm;
this.changedFields = changedFields.add("IsValidForForm");
return this;
}
public Builder isRequiredForForm(Boolean isRequiredForForm) {
this.isRequiredForForm = isRequiredForForm;
this.changedFields = changedFields.add("IsRequiredForForm");
return this;
}
public Builder isValidForGrid(Boolean isValidForGrid) {
this.isValidForGrid = isValidForGrid;
this.changedFields = changedFields.add("IsValidForGrid");
return this;
}
public Builder requiredLevel(AttributeRequiredLevelManagedProperty requiredLevel) {
this.requiredLevel = requiredLevel;
this.changedFields = changedFields.add("RequiredLevel");
return this;
}
public Builder canModifyAdditionalSettings(BooleanManagedProperty canModifyAdditionalSettings) {
this.canModifyAdditionalSettings = canModifyAdditionalSettings;
this.changedFields = changedFields.add("CanModifyAdditionalSettings");
return this;
}
public Builder schemaName(String schemaName) {
this.schemaName = schemaName;
this.changedFields = changedFields.add("SchemaName");
return this;
}
public Builder externalName(String externalName) {
this.externalName = externalName;
this.changedFields = changedFields.add("ExternalName");
return this;
}
public Builder isLogical(Boolean isLogical) {
this.isLogical = isLogical;
this.changedFields = changedFields.add("IsLogical");
return this;
}
public Builder isDataSourceSecret(Boolean isDataSourceSecret) {
this.isDataSourceSecret = isDataSourceSecret;
this.changedFields = changedFields.add("IsDataSourceSecret");
return this;
}
public Builder inheritsFrom(String inheritsFrom) {
this.inheritsFrom = inheritsFrom;
this.changedFields = changedFields.add("InheritsFrom");
return this;
}
public Builder createdOn(OffsetDateTime createdOn) {
this.createdOn = createdOn;
this.changedFields = changedFields.add("CreatedOn");
return this;
}
public Builder modifiedOn(OffsetDateTime modifiedOn) {
this.modifiedOn = modifiedOn;
this.changedFields = changedFields.add("ModifiedOn");
return this;
}
public Builder sourceType(Integer sourceType) {
this.sourceType = sourceType;
this.changedFields = changedFields.add("SourceType");
return this;
}
public Builder autoNumberFormat(String autoNumberFormat) {
this.autoNumberFormat = autoNumberFormat;
this.changedFields = changedFields.add("AutoNumberFormat");
return this;
}
public Builder defaultFormValue(Integer defaultFormValue) {
this.defaultFormValue = defaultFormValue;
this.changedFields = changedFields.add("DefaultFormValue");
return this;
}
public Builder isEntityReferenceStored(Boolean isEntityReferenceStored) {
this.isEntityReferenceStored = isEntityReferenceStored;
this.changedFields = changedFields.add("IsEntityReferenceStored");
return this;
}
public EntityNameAttributeMetadata build() {
EntityNameAttributeMetadata _x = new EntityNameAttributeMetadata();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.EntityNameAttributeMetadata";
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
_x.attributeOf = attributeOf;
_x.attributeType = attributeType;
_x.attributeTypeName = attributeTypeName;
_x.columnNumber = columnNumber;
_x.description = description;
_x.displayName = displayName;
_x.deprecatedVersion = deprecatedVersion;
_x.introducedVersion = introducedVersion;
_x.entityLogicalName = entityLogicalName;
_x.isAuditEnabled = isAuditEnabled;
_x.isCustomAttribute = isCustomAttribute;
_x.isPrimaryId = isPrimaryId;
_x.isValidODataAttribute = isValidODataAttribute;
_x.isPrimaryName = isPrimaryName;
_x.isValidForCreate = isValidForCreate;
_x.isValidForRead = isValidForRead;
_x.isValidForUpdate = isValidForUpdate;
_x.canBeSecuredForRead = canBeSecuredForRead;
_x.canBeSecuredForCreate = canBeSecuredForCreate;
_x.canBeSecuredForUpdate = canBeSecuredForUpdate;
_x.isSecured = isSecured;
_x.isRetrievable = isRetrievable;
_x.isFilterable = isFilterable;
_x.isSearchable = isSearchable;
_x.isManaged = isManaged;
_x.isGlobalFilterEnabled = isGlobalFilterEnabled;
_x.isSortableEnabled = isSortableEnabled;
_x.linkedAttributeId = linkedAttributeId;
_x.logicalName = logicalName;
_x.isCustomizable = isCustomizable;
_x.isRenameable = isRenameable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.isValidForForm = isValidForForm;
_x.isRequiredForForm = isRequiredForForm;
_x.isValidForGrid = isValidForGrid;
_x.requiredLevel = requiredLevel;
_x.canModifyAdditionalSettings = canModifyAdditionalSettings;
_x.schemaName = schemaName;
_x.externalName = externalName;
_x.isLogical = isLogical;
_x.isDataSourceSecret = isDataSourceSecret;
_x.inheritsFrom = inheritsFrom;
_x.createdOn = createdOn;
_x.modifiedOn = modifiedOn;
_x.sourceType = sourceType;
_x.autoNumberFormat = autoNumberFormat;
_x.defaultFormValue = defaultFormValue;
_x.isEntityReferenceStored = isEntityReferenceStored;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && metadataId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(metadataId.toString()));
}
}
@Property(name="IsEntityReferenceStored")
@JsonIgnore
public Optional getIsEntityReferenceStored() {
return Optional.ofNullable(isEntityReferenceStored);
}
public EntityNameAttributeMetadata withIsEntityReferenceStored(Boolean isEntityReferenceStored) {
EntityNameAttributeMetadata _x = _copy();
_x.changedFields = changedFields.add("IsEntityReferenceStored");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityNameAttributeMetadata");
_x.isEntityReferenceStored = isEntityReferenceStored;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EntityNameAttributeMetadata patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
EntityNameAttributeMetadata _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EntityNameAttributeMetadata put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
EntityNameAttributeMetadata _x = _copy();
_x.changedFields = null;
return _x;
}
private EntityNameAttributeMetadata _copy() {
EntityNameAttributeMetadata _x = new EntityNameAttributeMetadata();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
_x.attributeOf = attributeOf;
_x.attributeType = attributeType;
_x.attributeTypeName = attributeTypeName;
_x.columnNumber = columnNumber;
_x.description = description;
_x.displayName = displayName;
_x.deprecatedVersion = deprecatedVersion;
_x.introducedVersion = introducedVersion;
_x.entityLogicalName = entityLogicalName;
_x.isAuditEnabled = isAuditEnabled;
_x.isCustomAttribute = isCustomAttribute;
_x.isPrimaryId = isPrimaryId;
_x.isValidODataAttribute = isValidODataAttribute;
_x.isPrimaryName = isPrimaryName;
_x.isValidForCreate = isValidForCreate;
_x.isValidForRead = isValidForRead;
_x.isValidForUpdate = isValidForUpdate;
_x.canBeSecuredForRead = canBeSecuredForRead;
_x.canBeSecuredForCreate = canBeSecuredForCreate;
_x.canBeSecuredForUpdate = canBeSecuredForUpdate;
_x.isSecured = isSecured;
_x.isRetrievable = isRetrievable;
_x.isFilterable = isFilterable;
_x.isSearchable = isSearchable;
_x.isManaged = isManaged;
_x.isGlobalFilterEnabled = isGlobalFilterEnabled;
_x.isSortableEnabled = isSortableEnabled;
_x.linkedAttributeId = linkedAttributeId;
_x.logicalName = logicalName;
_x.isCustomizable = isCustomizable;
_x.isRenameable = isRenameable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.isValidForForm = isValidForForm;
_x.isRequiredForForm = isRequiredForForm;
_x.isValidForGrid = isValidForGrid;
_x.requiredLevel = requiredLevel;
_x.canModifyAdditionalSettings = canModifyAdditionalSettings;
_x.schemaName = schemaName;
_x.externalName = externalName;
_x.isLogical = isLogical;
_x.isDataSourceSecret = isDataSourceSecret;
_x.inheritsFrom = inheritsFrom;
_x.createdOn = createdOn;
_x.modifiedOn = modifiedOn;
_x.sourceType = sourceType;
_x.autoNumberFormat = autoNumberFormat;
_x.defaultFormValue = defaultFormValue;
_x.isEntityReferenceStored = isEntityReferenceStored;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("EntityNameAttributeMetadata[");
b.append("MetadataId=");
b.append(this.metadataId);
b.append(", ");
b.append("HasChanged=");
b.append(this.hasChanged);
b.append(", ");
b.append("AttributeOf=");
b.append(this.attributeOf);
b.append(", ");
b.append("AttributeType=");
b.append(this.attributeType);
b.append(", ");
b.append("AttributeTypeName=");
b.append(this.attributeTypeName);
b.append(", ");
b.append("ColumnNumber=");
b.append(this.columnNumber);
b.append(", ");
b.append("Description=");
b.append(this.description);
b.append(", ");
b.append("DisplayName=");
b.append(this.displayName);
b.append(", ");
b.append("DeprecatedVersion=");
b.append(this.deprecatedVersion);
b.append(", ");
b.append("IntroducedVersion=");
b.append(this.introducedVersion);
b.append(", ");
b.append("EntityLogicalName=");
b.append(this.entityLogicalName);
b.append(", ");
b.append("IsAuditEnabled=");
b.append(this.isAuditEnabled);
b.append(", ");
b.append("IsCustomAttribute=");
b.append(this.isCustomAttribute);
b.append(", ");
b.append("IsPrimaryId=");
b.append(this.isPrimaryId);
b.append(", ");
b.append("IsValidODataAttribute=");
b.append(this.isValidODataAttribute);
b.append(", ");
b.append("IsPrimaryName=");
b.append(this.isPrimaryName);
b.append(", ");
b.append("IsValidForCreate=");
b.append(this.isValidForCreate);
b.append(", ");
b.append("IsValidForRead=");
b.append(this.isValidForRead);
b.append(", ");
b.append("IsValidForUpdate=");
b.append(this.isValidForUpdate);
b.append(", ");
b.append("CanBeSecuredForRead=");
b.append(this.canBeSecuredForRead);
b.append(", ");
b.append("CanBeSecuredForCreate=");
b.append(this.canBeSecuredForCreate);
b.append(", ");
b.append("CanBeSecuredForUpdate=");
b.append(this.canBeSecuredForUpdate);
b.append(", ");
b.append("IsSecured=");
b.append(this.isSecured);
b.append(", ");
b.append("IsRetrievable=");
b.append(this.isRetrievable);
b.append(", ");
b.append("IsFilterable=");
b.append(this.isFilterable);
b.append(", ");
b.append("IsSearchable=");
b.append(this.isSearchable);
b.append(", ");
b.append("IsManaged=");
b.append(this.isManaged);
b.append(", ");
b.append("IsGlobalFilterEnabled=");
b.append(this.isGlobalFilterEnabled);
b.append(", ");
b.append("IsSortableEnabled=");
b.append(this.isSortableEnabled);
b.append(", ");
b.append("LinkedAttributeId=");
b.append(this.linkedAttributeId);
b.append(", ");
b.append("LogicalName=");
b.append(this.logicalName);
b.append(", ");
b.append("IsCustomizable=");
b.append(this.isCustomizable);
b.append(", ");
b.append("IsRenameable=");
b.append(this.isRenameable);
b.append(", ");
b.append("IsValidForAdvancedFind=");
b.append(this.isValidForAdvancedFind);
b.append(", ");
b.append("IsValidForForm=");
b.append(this.isValidForForm);
b.append(", ");
b.append("IsRequiredForForm=");
b.append(this.isRequiredForForm);
b.append(", ");
b.append("IsValidForGrid=");
b.append(this.isValidForGrid);
b.append(", ");
b.append("RequiredLevel=");
b.append(this.requiredLevel);
b.append(", ");
b.append("CanModifyAdditionalSettings=");
b.append(this.canModifyAdditionalSettings);
b.append(", ");
b.append("SchemaName=");
b.append(this.schemaName);
b.append(", ");
b.append("ExternalName=");
b.append(this.externalName);
b.append(", ");
b.append("IsLogical=");
b.append(this.isLogical);
b.append(", ");
b.append("IsDataSourceSecret=");
b.append(this.isDataSourceSecret);
b.append(", ");
b.append("InheritsFrom=");
b.append(this.inheritsFrom);
b.append(", ");
b.append("CreatedOn=");
b.append(this.createdOn);
b.append(", ");
b.append("ModifiedOn=");
b.append(this.modifiedOn);
b.append(", ");
b.append("SourceType=");
b.append(this.sourceType);
b.append(", ");
b.append("AutoNumberFormat=");
b.append(this.autoNumberFormat);
b.append(", ");
b.append("DefaultFormValue=");
b.append(this.defaultFormValue);
b.append(", ");
b.append("IsEntityReferenceStored=");
b.append(this.isEntityReferenceStored);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy