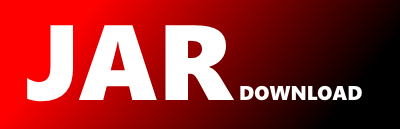
microsoft.dynamics.crm.entity.Fax Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.ActioncardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.ActivitypointerRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.KnowledgearticleRequest;
import microsoft.dynamics.crm.entity.request.KnowledgebaserecordRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.SlaRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"tsid",
"numberofpages",
"overriddencreatedon",
"subcategory",
"subscriptionid",
"importsequencenumber",
"directioncode",
"faxnumber",
"coverpagename",
"billingcode",
"category"})
@JsonInclude(Include.NON_NULL)
public class Fax extends Activitypointer implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.fax";
}
@JsonProperty("tsid")
protected String tsid;
@JsonProperty("numberofpages")
protected Integer numberofpages;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("subcategory")
protected String subcategory;
@JsonProperty("subscriptionid")
protected String subscriptionid;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("directioncode")
protected Boolean directioncode;
@JsonProperty("faxnumber")
protected String faxnumber;
@JsonProperty("coverpagename")
protected String coverpagename;
@JsonProperty("billingcode")
protected String billingcode;
@JsonProperty("category")
protected String category;
protected Fax() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderFax() {
return new Builder();
}
public static final class Builder {
private OffsetDateTime lastonholdtime;
private Integer actualdurationminutes;
private String _owningteam_value;
private String exchangeitemid;
private Boolean ismapiprivate;
private OffsetDateTime createdon;
private String seriesid;
private Boolean leftvoicemail;
private OffsetDateTime deliverylastattemptedon;
private Boolean isbilled;
private Boolean isworkflowcreated;
private String _sendermailboxid_value;
private String description;
private String _regardingobjectid_value;
private Integer onholdtime;
private String _modifiedby_value;
private Integer community;
private String activityid;
private OffsetDateTime sortdate;
private Integer instancetypecode;
private Integer timezoneruleversionnumber;
private String _createdonbehalfby_value;
private String _transactioncurrencyid_value;
private Long versionnumber;
private String processid;
private OffsetDateTime scheduledend;
private Integer prioritycode;
private String _slaid_value;
private String stageid;
private OffsetDateTime actualstart;
private String _owningbusinessunit_value;
private String _owninguser_value;
private Integer utcconversiontimezonecode;
private String exchangeweblink;
private Integer scheduleddurationminutes;
private OffsetDateTime senton;
private OffsetDateTime scheduledstart;
private Integer statecode;
private String subject;
private OffsetDateTime postponeactivityprocessinguntil;
private String _modifiedonbehalfby_value;
private BigDecimal exchangerate;
private Boolean isregularactivity;
private Integer deliveryprioritycode;
private String activityadditionalparams;
private String traversedpath;
private String _createdby_value;
private String activitytypecode;
private String _ownerid_value;
private OffsetDateTime modifiedon;
private String _slainvokedid_value;
private Integer statuscode;
private OffsetDateTime actualend;
private String tsid;
private Integer numberofpages;
private OffsetDateTime overriddencreatedon;
private String subcategory;
private String subscriptionid;
private Integer importsequencenumber;
private Boolean directioncode;
private String faxnumber;
private String coverpagename;
private String billingcode;
private String category;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder lastonholdtime(OffsetDateTime lastonholdtime) {
this.lastonholdtime = lastonholdtime;
this.changedFields = changedFields.add("lastonholdtime");
return this;
}
public Builder actualdurationminutes(Integer actualdurationminutes) {
this.actualdurationminutes = actualdurationminutes;
this.changedFields = changedFields.add("actualdurationminutes");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder exchangeitemid(String exchangeitemid) {
this.exchangeitemid = exchangeitemid;
this.changedFields = changedFields.add("exchangeitemid");
return this;
}
public Builder ismapiprivate(Boolean ismapiprivate) {
this.ismapiprivate = ismapiprivate;
this.changedFields = changedFields.add("ismapiprivate");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder seriesid(String seriesid) {
this.seriesid = seriesid;
this.changedFields = changedFields.add("seriesid");
return this;
}
public Builder leftvoicemail(Boolean leftvoicemail) {
this.leftvoicemail = leftvoicemail;
this.changedFields = changedFields.add("leftvoicemail");
return this;
}
public Builder deliverylastattemptedon(OffsetDateTime deliverylastattemptedon) {
this.deliverylastattemptedon = deliverylastattemptedon;
this.changedFields = changedFields.add("deliverylastattemptedon");
return this;
}
public Builder isbilled(Boolean isbilled) {
this.isbilled = isbilled;
this.changedFields = changedFields.add("isbilled");
return this;
}
public Builder isworkflowcreated(Boolean isworkflowcreated) {
this.isworkflowcreated = isworkflowcreated;
this.changedFields = changedFields.add("isworkflowcreated");
return this;
}
public Builder _sendermailboxid_value(String _sendermailboxid_value) {
this._sendermailboxid_value = _sendermailboxid_value;
this.changedFields = changedFields.add("_sendermailboxid_value");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder _regardingobjectid_value(String _regardingobjectid_value) {
this._regardingobjectid_value = _regardingobjectid_value;
this.changedFields = changedFields.add("_regardingobjectid_value");
return this;
}
public Builder onholdtime(Integer onholdtime) {
this.onholdtime = onholdtime;
this.changedFields = changedFields.add("onholdtime");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder community(Integer community) {
this.community = community;
this.changedFields = changedFields.add("community");
return this;
}
public Builder activityid(String activityid) {
this.activityid = activityid;
this.changedFields = changedFields.add("activityid");
return this;
}
public Builder sortdate(OffsetDateTime sortdate) {
this.sortdate = sortdate;
this.changedFields = changedFields.add("sortdate");
return this;
}
public Builder instancetypecode(Integer instancetypecode) {
this.instancetypecode = instancetypecode;
this.changedFields = changedFields.add("instancetypecode");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder processid(String processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder scheduledend(OffsetDateTime scheduledend) {
this.scheduledend = scheduledend;
this.changedFields = changedFields.add("scheduledend");
return this;
}
public Builder prioritycode(Integer prioritycode) {
this.prioritycode = prioritycode;
this.changedFields = changedFields.add("prioritycode");
return this;
}
public Builder _slaid_value(String _slaid_value) {
this._slaid_value = _slaid_value;
this.changedFields = changedFields.add("_slaid_value");
return this;
}
public Builder stageid(String stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder actualstart(OffsetDateTime actualstart) {
this.actualstart = actualstart;
this.changedFields = changedFields.add("actualstart");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder exchangeweblink(String exchangeweblink) {
this.exchangeweblink = exchangeweblink;
this.changedFields = changedFields.add("exchangeweblink");
return this;
}
public Builder scheduleddurationminutes(Integer scheduleddurationminutes) {
this.scheduleddurationminutes = scheduleddurationminutes;
this.changedFields = changedFields.add("scheduleddurationminutes");
return this;
}
public Builder senton(OffsetDateTime senton) {
this.senton = senton;
this.changedFields = changedFields.add("senton");
return this;
}
public Builder scheduledstart(OffsetDateTime scheduledstart) {
this.scheduledstart = scheduledstart;
this.changedFields = changedFields.add("scheduledstart");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder subject(String subject) {
this.subject = subject;
this.changedFields = changedFields.add("subject");
return this;
}
public Builder postponeactivityprocessinguntil(OffsetDateTime postponeactivityprocessinguntil) {
this.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
this.changedFields = changedFields.add("postponeactivityprocessinguntil");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder isregularactivity(Boolean isregularactivity) {
this.isregularactivity = isregularactivity;
this.changedFields = changedFields.add("isregularactivity");
return this;
}
public Builder deliveryprioritycode(Integer deliveryprioritycode) {
this.deliveryprioritycode = deliveryprioritycode;
this.changedFields = changedFields.add("deliveryprioritycode");
return this;
}
public Builder activityadditionalparams(String activityadditionalparams) {
this.activityadditionalparams = activityadditionalparams;
this.changedFields = changedFields.add("activityadditionalparams");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder activitytypecode(String activitytypecode) {
this.activitytypecode = activitytypecode;
this.changedFields = changedFields.add("activitytypecode");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder _slainvokedid_value(String _slainvokedid_value) {
this._slainvokedid_value = _slainvokedid_value;
this.changedFields = changedFields.add("_slainvokedid_value");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder actualend(OffsetDateTime actualend) {
this.actualend = actualend;
this.changedFields = changedFields.add("actualend");
return this;
}
public Builder tsid(String tsid) {
this.tsid = tsid;
this.changedFields = changedFields.add("tsid");
return this;
}
public Builder numberofpages(Integer numberofpages) {
this.numberofpages = numberofpages;
this.changedFields = changedFields.add("numberofpages");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder subcategory(String subcategory) {
this.subcategory = subcategory;
this.changedFields = changedFields.add("subcategory");
return this;
}
public Builder subscriptionid(String subscriptionid) {
this.subscriptionid = subscriptionid;
this.changedFields = changedFields.add("subscriptionid");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder directioncode(Boolean directioncode) {
this.directioncode = directioncode;
this.changedFields = changedFields.add("directioncode");
return this;
}
public Builder faxnumber(String faxnumber) {
this.faxnumber = faxnumber;
this.changedFields = changedFields.add("faxnumber");
return this;
}
public Builder coverpagename(String coverpagename) {
this.coverpagename = coverpagename;
this.changedFields = changedFields.add("coverpagename");
return this;
}
public Builder billingcode(String billingcode) {
this.billingcode = billingcode;
this.changedFields = changedFields.add("billingcode");
return this;
}
public Builder category(String category) {
this.category = category;
this.changedFields = changedFields.add("category");
return this;
}
public Fax build() {
Fax _x = new Fax();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.fax";
_x.lastonholdtime = lastonholdtime;
_x.actualdurationminutes = actualdurationminutes;
_x._owningteam_value = _owningteam_value;
_x.exchangeitemid = exchangeitemid;
_x.ismapiprivate = ismapiprivate;
_x.createdon = createdon;
_x.seriesid = seriesid;
_x.leftvoicemail = leftvoicemail;
_x.deliverylastattemptedon = deliverylastattemptedon;
_x.isbilled = isbilled;
_x.isworkflowcreated = isworkflowcreated;
_x._sendermailboxid_value = _sendermailboxid_value;
_x.description = description;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.onholdtime = onholdtime;
_x._modifiedby_value = _modifiedby_value;
_x.community = community;
_x.activityid = activityid;
_x.sortdate = sortdate;
_x.instancetypecode = instancetypecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.versionnumber = versionnumber;
_x.processid = processid;
_x.scheduledend = scheduledend;
_x.prioritycode = prioritycode;
_x._slaid_value = _slaid_value;
_x.stageid = stageid;
_x.actualstart = actualstart;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._owninguser_value = _owninguser_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.exchangeweblink = exchangeweblink;
_x.scheduleddurationminutes = scheduleddurationminutes;
_x.senton = senton;
_x.scheduledstart = scheduledstart;
_x.statecode = statecode;
_x.subject = subject;
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.isregularactivity = isregularactivity;
_x.deliveryprioritycode = deliveryprioritycode;
_x.activityadditionalparams = activityadditionalparams;
_x.traversedpath = traversedpath;
_x._createdby_value = _createdby_value;
_x.activitytypecode = activitytypecode;
_x._ownerid_value = _ownerid_value;
_x.modifiedon = modifiedon;
_x._slainvokedid_value = _slainvokedid_value;
_x.statuscode = statuscode;
_x.actualend = actualend;
_x.tsid = tsid;
_x.numberofpages = numberofpages;
_x.overriddencreatedon = overriddencreatedon;
_x.subcategory = subcategory;
_x.subscriptionid = subscriptionid;
_x.importsequencenumber = importsequencenumber;
_x.directioncode = directioncode;
_x.faxnumber = faxnumber;
_x.coverpagename = coverpagename;
_x.billingcode = billingcode;
_x.category = category;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && activityid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(activityid.toString()));
}
}
@Property(name="tsid")
@JsonIgnore
public Optional getTsid() {
return Optional.ofNullable(tsid);
}
public Fax withTsid(String tsid) {
Checks.checkIsAscii(tsid);
Fax _x = _copy();
_x.changedFields = changedFields.add("tsid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.tsid = tsid;
return _x;
}
@Property(name="numberofpages")
@JsonIgnore
public Optional getNumberofpages() {
return Optional.ofNullable(numberofpages);
}
public Fax withNumberofpages(Integer numberofpages) {
Fax _x = _copy();
_x.changedFields = changedFields.add("numberofpages");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.numberofpages = numberofpages;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Fax withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Fax _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="subcategory")
@JsonIgnore
public Optional getSubcategory() {
return Optional.ofNullable(subcategory);
}
public Fax withSubcategory(String subcategory) {
Checks.checkIsAscii(subcategory);
Fax _x = _copy();
_x.changedFields = changedFields.add("subcategory");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.subcategory = subcategory;
return _x;
}
@Property(name="subscriptionid")
@JsonIgnore
public Optional getSubscriptionid() {
return Optional.ofNullable(subscriptionid);
}
public Fax withSubscriptionid(String subscriptionid) {
Fax _x = _copy();
_x.changedFields = changedFields.add("subscriptionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.subscriptionid = subscriptionid;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Fax withImportsequencenumber(Integer importsequencenumber) {
Fax _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="directioncode")
@JsonIgnore
public Optional getDirectioncode() {
return Optional.ofNullable(directioncode);
}
public Fax withDirectioncode(Boolean directioncode) {
Fax _x = _copy();
_x.changedFields = changedFields.add("directioncode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.directioncode = directioncode;
return _x;
}
@Property(name="faxnumber")
@JsonIgnore
public Optional getFaxnumber() {
return Optional.ofNullable(faxnumber);
}
public Fax withFaxnumber(String faxnumber) {
Checks.checkIsAscii(faxnumber);
Fax _x = _copy();
_x.changedFields = changedFields.add("faxnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.faxnumber = faxnumber;
return _x;
}
@Property(name="coverpagename")
@JsonIgnore
public Optional getCoverpagename() {
return Optional.ofNullable(coverpagename);
}
public Fax withCoverpagename(String coverpagename) {
Checks.checkIsAscii(coverpagename);
Fax _x = _copy();
_x.changedFields = changedFields.add("coverpagename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.coverpagename = coverpagename;
return _x;
}
@Property(name="billingcode")
@JsonIgnore
public Optional getBillingcode() {
return Optional.ofNullable(billingcode);
}
public Fax withBillingcode(String billingcode) {
Checks.checkIsAscii(billingcode);
Fax _x = _copy();
_x.changedFields = changedFields.add("billingcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.billingcode = billingcode;
return _x;
}
@Property(name="category")
@JsonIgnore
public Optional getCategory() {
return Optional.ofNullable(category);
}
public Fax withCategory(String category) {
Checks.checkIsAscii(category);
Fax _x = _copy();
_x.changedFields = changedFields.add("category");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fax");
_x.category = category;
return _x;
}
@NavigationProperty(name="regardingobjectid_knowledgebaserecord_fax")
@JsonIgnore
public KnowledgebaserecordRequest getRegardingobjectid_knowledgebaserecord_fax() {
return new KnowledgebaserecordRequest(contextPath.addSegment("regardingobjectid_knowledgebaserecord_fax"));
}
@NavigationProperty(name="Fax_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getFax_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Fax_BulkDeleteFailures"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"));
}
@NavigationProperty(name="owningbusinessunit_fax")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit_fax() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit_fax"));
}
@NavigationProperty(name="regardingobjectid_account_fax")
@JsonIgnore
public AccountRequest getRegardingobjectid_account_fax() {
return new AccountRequest(contextPath.addSegment("regardingobjectid_account_fax"));
}
@NavigationProperty(name="Fax_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getFax_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Fax_Annotation"));
}
@NavigationProperty(name="fax_actioncard")
@JsonIgnore
public ActioncardCollectionRequest getFax_actioncard() {
return new ActioncardCollectionRequest(
contextPath.addSegment("fax_actioncard"));
}
@NavigationProperty(name="createdby_fax")
@JsonIgnore
public SystemuserRequest getCreatedby_fax() {
return new SystemuserRequest(contextPath.addSegment("createdby_fax"));
}
@NavigationProperty(name="createdonbehalfby_fax")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby_fax() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby_fax"));
}
@NavigationProperty(name="transactioncurrencyid_fax")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid_fax() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid_fax"));
}
@NavigationProperty(name="owningteam_fax")
@JsonIgnore
public TeamRequest getOwningteam_fax() {
return new TeamRequest(contextPath.addSegment("owningteam_fax"));
}
@NavigationProperty(name="Fax_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getFax_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Fax_DuplicateMatchingRecord"));
}
@NavigationProperty(name="regardingobjectid_knowledgearticle_fax")
@JsonIgnore
public KnowledgearticleRequest getRegardingobjectid_knowledgearticle_fax() {
return new KnowledgearticleRequest(contextPath.addSegment("regardingobjectid_knowledgearticle_fax"));
}
@NavigationProperty(name="owninguser_fax")
@JsonIgnore
public SystemuserRequest getOwninguser_fax() {
return new SystemuserRequest(contextPath.addSegment("owninguser_fax"));
}
@NavigationProperty(name="sla_fax_sla")
@JsonIgnore
public SlaRequest getSla_fax_sla() {
return new SlaRequest(contextPath.addSegment("sla_fax_sla"));
}
@NavigationProperty(name="Fax_QueueItem")
@JsonIgnore
public QueueitemCollectionRequest getFax_QueueItem() {
return new QueueitemCollectionRequest(
contextPath.addSegment("Fax_QueueItem"));
}
@NavigationProperty(name="fax_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getFax_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("fax_activity_parties"));
}
@NavigationProperty(name="fax_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getFax_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("fax_principalobjectattributeaccess"));
}
@NavigationProperty(name="modifiedby_fax")
@JsonIgnore
public SystemuserRequest getModifiedby_fax() {
return new SystemuserRequest(contextPath.addSegment("modifiedby_fax"));
}
@NavigationProperty(name="modifiedonbehalfby_fax")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby_fax() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby_fax"));
}
@NavigationProperty(name="activityid_activitypointer")
@JsonIgnore
public ActivitypointerRequest getActivityid_activitypointer() {
return new ActivitypointerRequest(contextPath.addSegment("activityid_activitypointer"));
}
@NavigationProperty(name="fax_connections2")
@JsonIgnore
public ConnectionCollectionRequest getFax_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("fax_connections2"));
}
@NavigationProperty(name="slainvokedid_fax_sla")
@JsonIgnore
public SlaRequest getSlainvokedid_fax_sla() {
return new SlaRequest(contextPath.addSegment("slainvokedid_fax_sla"));
}
@NavigationProperty(name="Fax_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getFax_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Fax_ProcessSessions"));
}
@NavigationProperty(name="regardingobjectid_contact_fax")
@JsonIgnore
public ContactRequest getRegardingobjectid_contact_fax() {
return new ContactRequest(contextPath.addSegment("regardingobjectid_contact_fax"));
}
@NavigationProperty(name="slakpiinstance_fax")
@JsonIgnore
public SlakpiinstanceCollectionRequest getSlakpiinstance_fax() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("slakpiinstance_fax"));
}
@NavigationProperty(name="fax_connections1")
@JsonIgnore
public ConnectionCollectionRequest getFax_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("fax_connections1"));
}
@NavigationProperty(name="Fax_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getFax_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Fax_SyncErrors"));
}
@NavigationProperty(name="Fax_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getFax_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Fax_DuplicateBaseRecord"));
}
@NavigationProperty(name="Fax_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getFax_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Fax_AsyncOperations"));
}
@NavigationProperty(name="ownerid_fax")
@JsonIgnore
public PrincipalRequest getOwnerid_fax() {
return new PrincipalRequest(contextPath.addSegment("ownerid_fax"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fax patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Fax _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fax put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Fax _x = _copy();
_x.changedFields = null;
return _x;
}
private Fax _copy() {
Fax _x = new Fax();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.lastonholdtime = lastonholdtime;
_x.actualdurationminutes = actualdurationminutes;
_x._owningteam_value = _owningteam_value;
_x.exchangeitemid = exchangeitemid;
_x.ismapiprivate = ismapiprivate;
_x.createdon = createdon;
_x.seriesid = seriesid;
_x.leftvoicemail = leftvoicemail;
_x.deliverylastattemptedon = deliverylastattemptedon;
_x.isbilled = isbilled;
_x.isworkflowcreated = isworkflowcreated;
_x._sendermailboxid_value = _sendermailboxid_value;
_x.description = description;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.onholdtime = onholdtime;
_x._modifiedby_value = _modifiedby_value;
_x.community = community;
_x.activityid = activityid;
_x.sortdate = sortdate;
_x.instancetypecode = instancetypecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.versionnumber = versionnumber;
_x.processid = processid;
_x.scheduledend = scheduledend;
_x.prioritycode = prioritycode;
_x._slaid_value = _slaid_value;
_x.stageid = stageid;
_x.actualstart = actualstart;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._owninguser_value = _owninguser_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.exchangeweblink = exchangeweblink;
_x.scheduleddurationminutes = scheduleddurationminutes;
_x.senton = senton;
_x.scheduledstart = scheduledstart;
_x.statecode = statecode;
_x.subject = subject;
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.isregularactivity = isregularactivity;
_x.deliveryprioritycode = deliveryprioritycode;
_x.activityadditionalparams = activityadditionalparams;
_x.traversedpath = traversedpath;
_x._createdby_value = _createdby_value;
_x.activitytypecode = activitytypecode;
_x._ownerid_value = _ownerid_value;
_x.modifiedon = modifiedon;
_x._slainvokedid_value = _slainvokedid_value;
_x.statuscode = statuscode;
_x.actualend = actualend;
_x.tsid = tsid;
_x.numberofpages = numberofpages;
_x.overriddencreatedon = overriddencreatedon;
_x.subcategory = subcategory;
_x.subscriptionid = subscriptionid;
_x.importsequencenumber = importsequencenumber;
_x.directioncode = directioncode;
_x.faxnumber = faxnumber;
_x.coverpagename = coverpagename;
_x.billingcode = billingcode;
_x.category = category;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Fax[");
b.append("lastonholdtime=");
b.append(this.lastonholdtime);
b.append(", ");
b.append("actualdurationminutes=");
b.append(this.actualdurationminutes);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("exchangeitemid=");
b.append(this.exchangeitemid);
b.append(", ");
b.append("ismapiprivate=");
b.append(this.ismapiprivate);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("seriesid=");
b.append(this.seriesid);
b.append(", ");
b.append("leftvoicemail=");
b.append(this.leftvoicemail);
b.append(", ");
b.append("deliverylastattemptedon=");
b.append(this.deliverylastattemptedon);
b.append(", ");
b.append("isbilled=");
b.append(this.isbilled);
b.append(", ");
b.append("isworkflowcreated=");
b.append(this.isworkflowcreated);
b.append(", ");
b.append("_sendermailboxid_value=");
b.append(this._sendermailboxid_value);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("_regardingobjectid_value=");
b.append(this._regardingobjectid_value);
b.append(", ");
b.append("onholdtime=");
b.append(this.onholdtime);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("community=");
b.append(this.community);
b.append(", ");
b.append("activityid=");
b.append(this.activityid);
b.append(", ");
b.append("sortdate=");
b.append(this.sortdate);
b.append(", ");
b.append("instancetypecode=");
b.append(this.instancetypecode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("scheduledend=");
b.append(this.scheduledend);
b.append(", ");
b.append("prioritycode=");
b.append(this.prioritycode);
b.append(", ");
b.append("_slaid_value=");
b.append(this._slaid_value);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("actualstart=");
b.append(this.actualstart);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("exchangeweblink=");
b.append(this.exchangeweblink);
b.append(", ");
b.append("scheduleddurationminutes=");
b.append(this.scheduleddurationminutes);
b.append(", ");
b.append("senton=");
b.append(this.senton);
b.append(", ");
b.append("scheduledstart=");
b.append(this.scheduledstart);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("subject=");
b.append(this.subject);
b.append(", ");
b.append("postponeactivityprocessinguntil=");
b.append(this.postponeactivityprocessinguntil);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("isregularactivity=");
b.append(this.isregularactivity);
b.append(", ");
b.append("deliveryprioritycode=");
b.append(this.deliveryprioritycode);
b.append(", ");
b.append("activityadditionalparams=");
b.append(this.activityadditionalparams);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("activitytypecode=");
b.append(this.activitytypecode);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("_slainvokedid_value=");
b.append(this._slainvokedid_value);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("actualend=");
b.append(this.actualend);
b.append(", ");
b.append("tsid=");
b.append(this.tsid);
b.append(", ");
b.append("numberofpages=");
b.append(this.numberofpages);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("subcategory=");
b.append(this.subcategory);
b.append(", ");
b.append("subscriptionid=");
b.append(this.subscriptionid);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("directioncode=");
b.append(this.directioncode);
b.append(", ");
b.append("faxnumber=");
b.append(this.faxnumber);
b.append(", ");
b.append("coverpagename=");
b.append(this.coverpagename);
b.append(", ");
b.append("billingcode=");
b.append(this.billingcode);
b.append(", ");
b.append("category=");
b.append(this.category);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy