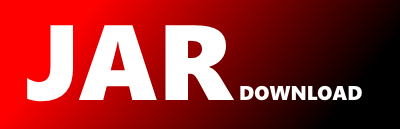
microsoft.dynamics.crm.entity.Fieldsecurityprofile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.FieldpermissionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SystemuserCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TeamCollectionRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.SolutionRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
@JsonPropertyOrder({
"@odata.type",
"_modifiedby_value",
"versionnumber",
"name",
"_modifiedonbehalfby_value",
"overwritetime",
"createdon",
"modifiedon",
"componentstate",
"description",
"_organizationid_value",
"ismanaged",
"solutionid",
"fieldsecurityprofileidunique",
"fieldsecurityprofileid",
"_createdby_value",
"_createdonbehalfby_value"})
@JsonInclude(Include.NON_NULL)
public class Fieldsecurityprofile extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.fieldsecurityprofile";
}
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("name")
protected String name;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("description")
protected String description;
@JsonProperty("_organizationid_value")
protected String _organizationid_value;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("fieldsecurityprofileidunique")
protected String fieldsecurityprofileidunique;
@JsonProperty("fieldsecurityprofileid")
protected String fieldsecurityprofileid;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
protected Fieldsecurityprofile() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderFieldsecurityprofile() {
return new Builder();
}
public static final class Builder {
private String _modifiedby_value;
private Long versionnumber;
private String name;
private String _modifiedonbehalfby_value;
private OffsetDateTime overwritetime;
private OffsetDateTime createdon;
private OffsetDateTime modifiedon;
private Integer componentstate;
private String description;
private String _organizationid_value;
private Boolean ismanaged;
private String solutionid;
private String fieldsecurityprofileidunique;
private String fieldsecurityprofileid;
private String _createdby_value;
private String _createdonbehalfby_value;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder _organizationid_value(String _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder fieldsecurityprofileidunique(String fieldsecurityprofileidunique) {
this.fieldsecurityprofileidunique = fieldsecurityprofileidunique;
this.changedFields = changedFields.add("fieldsecurityprofileidunique");
return this;
}
public Builder fieldsecurityprofileid(String fieldsecurityprofileid) {
this.fieldsecurityprofileid = fieldsecurityprofileid;
this.changedFields = changedFields.add("fieldsecurityprofileid");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Fieldsecurityprofile build() {
Fieldsecurityprofile _x = new Fieldsecurityprofile();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.fieldsecurityprofile";
_x._modifiedby_value = _modifiedby_value;
_x.versionnumber = versionnumber;
_x.name = name;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.overwritetime = overwritetime;
_x.createdon = createdon;
_x.modifiedon = modifiedon;
_x.componentstate = componentstate;
_x.description = description;
_x._organizationid_value = _organizationid_value;
_x.ismanaged = ismanaged;
_x.solutionid = solutionid;
_x.fieldsecurityprofileidunique = fieldsecurityprofileidunique;
_x.fieldsecurityprofileid = fieldsecurityprofileid;
_x._createdby_value = _createdby_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && fieldsecurityprofileid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(fieldsecurityprofileid.toString()));
}
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Fieldsecurityprofile with_modifiedby_value(String _modifiedby_value) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Fieldsecurityprofile withVersionnumber(Long versionnumber) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Fieldsecurityprofile withName(String name) {
Checks.checkIsAscii(name);
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.name = name;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Fieldsecurityprofile with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Fieldsecurityprofile withOverwritetime(OffsetDateTime overwritetime) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Fieldsecurityprofile withCreatedon(OffsetDateTime createdon) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.createdon = createdon;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Fieldsecurityprofile withModifiedon(OffsetDateTime modifiedon) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Fieldsecurityprofile withComponentstate(Integer componentstate) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.componentstate = componentstate;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Fieldsecurityprofile withDescription(String description) {
Checks.checkIsAscii(description);
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.description = description;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Fieldsecurityprofile with_organizationid_value(String _organizationid_value) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Fieldsecurityprofile withIsmanaged(Boolean ismanaged) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Fieldsecurityprofile withSolutionid(String solutionid) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.solutionid = solutionid;
return _x;
}
@Property(name="fieldsecurityprofileidunique")
@JsonIgnore
public Optional getFieldsecurityprofileidunique() {
return Optional.ofNullable(fieldsecurityprofileidunique);
}
public Fieldsecurityprofile withFieldsecurityprofileidunique(String fieldsecurityprofileidunique) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("fieldsecurityprofileidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.fieldsecurityprofileidunique = fieldsecurityprofileidunique;
return _x;
}
@Property(name="fieldsecurityprofileid")
@JsonIgnore
public Optional getFieldsecurityprofileid() {
return Optional.ofNullable(fieldsecurityprofileid);
}
public Fieldsecurityprofile withFieldsecurityprofileid(String fieldsecurityprofileid) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("fieldsecurityprofileid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x.fieldsecurityprofileid = fieldsecurityprofileid;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Fieldsecurityprofile with_createdby_value(String _createdby_value) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Fieldsecurityprofile with_createdonbehalfby_value(String _createdonbehalfby_value) {
Fieldsecurityprofile _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fieldsecurityprofile");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="FieldSecurityProfile_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getFieldSecurityProfile_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("FieldSecurityProfile_SyncErrors"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"));
}
@NavigationProperty(name="systemuserprofiles_association")
@JsonIgnore
public SystemuserCollectionRequest getSystemuserprofiles_association() {
return new SystemuserCollectionRequest(
contextPath.addSegment("systemuserprofiles_association"));
}
@NavigationProperty(name="lk_fieldpermission_fieldsecurityprofileid")
@JsonIgnore
public FieldpermissionCollectionRequest getLk_fieldpermission_fieldsecurityprofileid() {
return new FieldpermissionCollectionRequest(
contextPath.addSegment("lk_fieldpermission_fieldsecurityprofileid"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="teamprofiles_association")
@JsonIgnore
public TeamCollectionRequest getTeamprofiles_association() {
return new TeamCollectionRequest(
contextPath.addSegment("teamprofiles_association"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="solution_fieldsecurityprofile")
@JsonIgnore
public SolutionRequest getSolution_fieldsecurityprofile() {
return new SolutionRequest(contextPath.addSegment("solution_fieldsecurityprofile"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fieldsecurityprofile patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Fieldsecurityprofile _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fieldsecurityprofile put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Fieldsecurityprofile _x = _copy();
_x.changedFields = null;
return _x;
}
private Fieldsecurityprofile _copy() {
Fieldsecurityprofile _x = new Fieldsecurityprofile();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x._modifiedby_value = _modifiedby_value;
_x.versionnumber = versionnumber;
_x.name = name;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.overwritetime = overwritetime;
_x.createdon = createdon;
_x.modifiedon = modifiedon;
_x.componentstate = componentstate;
_x.description = description;
_x._organizationid_value = _organizationid_value;
_x.ismanaged = ismanaged;
_x.solutionid = solutionid;
_x.fieldsecurityprofileidunique = fieldsecurityprofileidunique;
_x.fieldsecurityprofileid = fieldsecurityprofileid;
_x._createdby_value = _createdby_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Fieldsecurityprofile[");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("fieldsecurityprofileidunique=");
b.append(this.fieldsecurityprofileidunique);
b.append(", ");
b.append("fieldsecurityprofileid=");
b.append(this.fieldsecurityprofileid);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy