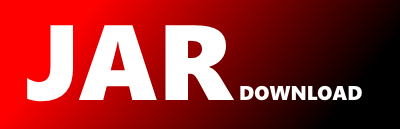
microsoft.dynamics.crm.entity.Fileattachment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.AsyncoperationRequest;
import microsoft.dynamics.crm.entity.request.CascadegrantrevokeaccessrecordstrackerRequest;
import microsoft.dynamics.crm.entity.request.ExportsolutionuploadRequest;
import microsoft.dynamics.crm.entity.request.FlowsessionRequest;
import microsoft.dynamics.crm.entity.request.Msdyn_aibfileRequest;
import microsoft.dynamics.crm.entity.request.Msdyn_aiconfigurationRequest;
import microsoft.dynamics.crm.entity.request.Msdyn_knowledgearticleimageRequest;
import microsoft.dynamics.crm.entity.request.SolutionRequest;
import microsoft.dynamics.crm.entity.request.StagesolutionuploadRequest;
import microsoft.dynamics.crm.entity.request.WorkflowbinaryRequest;
import microsoft.dynamics.crm.entity.request.WorkflowlogRequest;
@JsonPropertyOrder({
"@odata.type",
"regardingfieldname",
"filename",
"_objectid_value",
"fileattachmentid",
"createdon",
"mimetype",
"versionnumber",
"filesizeinbytes",
"objecttypecode"})
@JsonInclude(Include.NON_NULL)
public class Fileattachment extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.fileattachment";
}
@JsonProperty("regardingfieldname")
protected String regardingfieldname;
@JsonProperty("filename")
protected String filename;
@JsonProperty("_objectid_value")
protected String _objectid_value;
@JsonProperty("fileattachmentid")
protected String fileattachmentid;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("mimetype")
protected String mimetype;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("filesizeinbytes")
protected Long filesizeinbytes;
@JsonProperty("objecttypecode")
protected String objecttypecode;
protected Fileattachment() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderFileattachment() {
return new Builder();
}
public static final class Builder {
private String regardingfieldname;
private String filename;
private String _objectid_value;
private String fileattachmentid;
private OffsetDateTime createdon;
private String mimetype;
private Long versionnumber;
private Long filesizeinbytes;
private String objecttypecode;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder regardingfieldname(String regardingfieldname) {
this.regardingfieldname = regardingfieldname;
this.changedFields = changedFields.add("regardingfieldname");
return this;
}
public Builder filename(String filename) {
this.filename = filename;
this.changedFields = changedFields.add("filename");
return this;
}
public Builder _objectid_value(String _objectid_value) {
this._objectid_value = _objectid_value;
this.changedFields = changedFields.add("_objectid_value");
return this;
}
public Builder fileattachmentid(String fileattachmentid) {
this.fileattachmentid = fileattachmentid;
this.changedFields = changedFields.add("fileattachmentid");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder mimetype(String mimetype) {
this.mimetype = mimetype;
this.changedFields = changedFields.add("mimetype");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder filesizeinbytes(Long filesizeinbytes) {
this.filesizeinbytes = filesizeinbytes;
this.changedFields = changedFields.add("filesizeinbytes");
return this;
}
public Builder objecttypecode(String objecttypecode) {
this.objecttypecode = objecttypecode;
this.changedFields = changedFields.add("objecttypecode");
return this;
}
public Fileattachment build() {
Fileattachment _x = new Fileattachment();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.fileattachment";
_x.regardingfieldname = regardingfieldname;
_x.filename = filename;
_x._objectid_value = _objectid_value;
_x.fileattachmentid = fileattachmentid;
_x.createdon = createdon;
_x.mimetype = mimetype;
_x.versionnumber = versionnumber;
_x.filesizeinbytes = filesizeinbytes;
_x.objecttypecode = objecttypecode;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && fileattachmentid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(fileattachmentid.toString()));
}
}
@Property(name="regardingfieldname")
@JsonIgnore
public Optional getRegardingfieldname() {
return Optional.ofNullable(regardingfieldname);
}
public Fileattachment withRegardingfieldname(String regardingfieldname) {
Checks.checkIsAscii(regardingfieldname);
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("regardingfieldname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.regardingfieldname = regardingfieldname;
return _x;
}
@Property(name="filename")
@JsonIgnore
public Optional getFilename() {
return Optional.ofNullable(filename);
}
public Fileattachment withFilename(String filename) {
Checks.checkIsAscii(filename);
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("filename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.filename = filename;
return _x;
}
@Property(name="_objectid_value")
@JsonIgnore
public Optional get_objectid_value() {
return Optional.ofNullable(_objectid_value);
}
public Fileattachment with_objectid_value(String _objectid_value) {
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("_objectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x._objectid_value = _objectid_value;
return _x;
}
@Property(name="fileattachmentid")
@JsonIgnore
public Optional getFileattachmentid() {
return Optional.ofNullable(fileattachmentid);
}
public Fileattachment withFileattachmentid(String fileattachmentid) {
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("fileattachmentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.fileattachmentid = fileattachmentid;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Fileattachment withCreatedon(OffsetDateTime createdon) {
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.createdon = createdon;
return _x;
}
@Property(name="mimetype")
@JsonIgnore
public Optional getMimetype() {
return Optional.ofNullable(mimetype);
}
public Fileattachment withMimetype(String mimetype) {
Checks.checkIsAscii(mimetype);
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("mimetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.mimetype = mimetype;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Fileattachment withVersionnumber(Long versionnumber) {
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="filesizeinbytes")
@JsonIgnore
public Optional getFilesizeinbytes() {
return Optional.ofNullable(filesizeinbytes);
}
public Fileattachment withFilesizeinbytes(Long filesizeinbytes) {
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("filesizeinbytes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.filesizeinbytes = filesizeinbytes;
return _x;
}
@Property(name="objecttypecode")
@JsonIgnore
public Optional getObjecttypecode() {
return Optional.ofNullable(objecttypecode);
}
public Fileattachment withObjecttypecode(String objecttypecode) {
Checks.checkIsAscii(objecttypecode);
Fileattachment _x = _copy();
_x.changedFields = changedFields.add("objecttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.fileattachment");
_x.objecttypecode = objecttypecode;
return _x;
}
@NavigationProperty(name="FileAttachment_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getFileAttachment_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("FileAttachment_SyncErrors"));
}
@NavigationProperty(name="FileAttachment_Solution")
@JsonIgnore
public SolutionRequest getFileAttachment_Solution() {
return new SolutionRequest(contextPath.addSegment("FileAttachment_Solution"));
}
@NavigationProperty(name="solution_fileid")
@JsonIgnore
public SolutionCollectionRequest getSolution_fileid() {
return new SolutionCollectionRequest(
contextPath.addSegment("solution_fileid"));
}
@NavigationProperty(name="objectid_stagesolutionupload")
@JsonIgnore
public StagesolutionuploadRequest getObjectid_stagesolutionupload() {
return new StagesolutionuploadRequest(contextPath.addSegment("objectid_stagesolutionupload"));
}
@NavigationProperty(name="objectid_exportsolutionupload")
@JsonIgnore
public ExportsolutionuploadRequest getObjectid_exportsolutionupload() {
return new ExportsolutionuploadRequest(contextPath.addSegment("objectid_exportsolutionupload"));
}
@NavigationProperty(name="objectid_asyncoperation")
@JsonIgnore
public AsyncoperationRequest getObjectid_asyncoperation() {
return new AsyncoperationRequest(contextPath.addSegment("objectid_asyncoperation"));
}
@NavigationProperty(name="FileAttachment_AsyncOperation_DataBlobId")
@JsonIgnore
public AsyncoperationCollectionRequest getFileAttachment_AsyncOperation_DataBlobId() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("FileAttachment_AsyncOperation_DataBlobId"));
}
@NavigationProperty(name="objectid_workflowlog")
@JsonIgnore
public WorkflowlogRequest getObjectid_workflowlog() {
return new WorkflowlogRequest(contextPath.addSegment("objectid_workflowlog"));
}
@NavigationProperty(name="objectid_flowsession")
@JsonIgnore
public FlowsessionRequest getObjectid_flowsession() {
return new FlowsessionRequest(contextPath.addSegment("objectid_flowsession"));
}
@NavigationProperty(name="objectid_workflowbinary")
@JsonIgnore
public WorkflowbinaryRequest getObjectid_workflowbinary() {
return new WorkflowbinaryRequest(contextPath.addSegment("objectid_workflowbinary"));
}
@NavigationProperty(name="objectid_msdyn_knowledgearticleimage")
@JsonIgnore
public Msdyn_knowledgearticleimageRequest getObjectid_msdyn_knowledgearticleimage() {
return new Msdyn_knowledgearticleimageRequest(contextPath.addSegment("objectid_msdyn_knowledgearticleimage"));
}
@NavigationProperty(name="objectid_msdyn_aiconfiguration")
@JsonIgnore
public Msdyn_aiconfigurationRequest getObjectid_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurationRequest(contextPath.addSegment("objectid_msdyn_aiconfiguration"));
}
@NavigationProperty(name="objectid_msdyn_aibfile")
@JsonIgnore
public Msdyn_aibfileRequest getObjectid_msdyn_aibfile() {
return new Msdyn_aibfileRequest(contextPath.addSegment("objectid_msdyn_aibfile"));
}
@NavigationProperty(name="objectid_cascadegrantrevokeaccessrecordstracker")
@JsonIgnore
public CascadegrantrevokeaccessrecordstrackerRequest getObjectid_cascadegrantrevokeaccessrecordstracker() {
return new CascadegrantrevokeaccessrecordstrackerRequest(contextPath.addSegment("objectid_cascadegrantrevokeaccessrecordstracker"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fileattachment patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Fileattachment _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Fileattachment put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Fileattachment _x = _copy();
_x.changedFields = null;
return _x;
}
private Fileattachment _copy() {
Fileattachment _x = new Fileattachment();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.regardingfieldname = regardingfieldname;
_x.filename = filename;
_x._objectid_value = _objectid_value;
_x.fileattachmentid = fileattachmentid;
_x.createdon = createdon;
_x.mimetype = mimetype;
_x.versionnumber = versionnumber;
_x.filesizeinbytes = filesizeinbytes;
_x.objecttypecode = objecttypecode;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Fileattachment[");
b.append("regardingfieldname=");
b.append(this.regardingfieldname);
b.append(", ");
b.append("filename=");
b.append(this.filename);
b.append(", ");
b.append("_objectid_value=");
b.append(this._objectid_value);
b.append(", ");
b.append("fileattachmentid=");
b.append(this.fileattachmentid);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("mimetype=");
b.append(this.mimetype);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("filesizeinbytes=");
b.append(this.filesizeinbytes);
b.append(", ");
b.append("objecttypecode=");
b.append(this.objecttypecode);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy