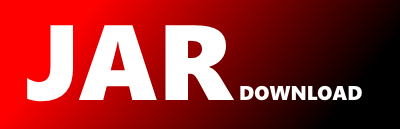
microsoft.dynamics.crm.entity.Mailbox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.ActivitypointerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SystemuserCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TracelogCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.EmailserverprofileRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.QueueRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
@JsonPropertyOrder({
"@odata.type",
"testemailconfigurationretrycount",
"noactcount",
"isactsyncorgflagset",
"exchangesyncstatexml",
"isforwardmailbox",
"_emailserverprofile_value",
"isemailaddressapprovedbyo365admin",
"actdeliverymethod",
"mailboxprocessingcontext",
"folderhierarchy",
"_modifiedby_value",
"outgoingemaildeliverymethod",
"exchangecontactsimportstatus",
"entityimageid",
"officeappsdeploymentscheduled",
"mailboxid",
"timezoneruleversionnumber",
"incomingemaildeliverymethod",
"forcedunlockcount",
"lastsuccessfulsynccompletedon",
"averagetotalduration",
"oauthtokenexpireson",
"_owninguser_value",
"modifiedon",
"lastsyncerror",
"postponeofficeappsdeploymentuntil",
"createdon",
"versionnumber",
"oauthrefreshtoken",
"_organizationid_value",
"lastduration",
"testemailconfigurationscheduled",
"officeappsdeploymenterror",
"enabledforact",
"outgoingemailstatus",
"lastsyncerrorcount",
"emailaddress",
"utcconversiontimezonecode",
"lastsyncerroroccurredon",
"processanddeleteemails",
"entityimage",
"allowemailconnectortousecredentials",
"itemsprocessedforlastsync",
"entityimage_timestamp",
"officeappsdeploymentcompleteon",
"processinglastattemptedon",
"username",
"_modifiedonbehalfby_value",
"officeappsdeploymentstatus",
"postponesendinguntil",
"postponetestemailconfigurationuntil",
"actstatus",
"statuscode",
"lastsyncstartedon",
"receivingpostponeduntilforact",
"processedtimes",
"exchangecontactsimportcompletedon",
"ispasswordset",
"_owningteam_value",
"_ownerid_value",
"lastsyncerrormachinename",
"_regardingobjectid_value",
"ewsurl",
"processingstatecode",
"transientfailurecount",
"lastsyncerrorcode",
"password",
"_createdonbehalfby_value",
"isoauthrefreshtokenset",
"postponemailboxprocessinguntil",
"testmailboxaccesscompletedon",
"processemailreceivedafter",
"emailrouteraccessapproval",
"mailboxstatus",
"noemailcount",
"incomingemailstatus",
"enabledforoutgoingemail",
"_createdby_value",
"isoauthaccesstokenset",
"oauthaccesstoken",
"enabledforincomingemail",
"isexchangecontactsimportscheduled",
"name",
"_owningbusinessunit_value",
"isserviceaccount",
"lastautodiscoveredon",
"orgmarkedasprimaryforexchangesync",
"entityimage_url",
"itemsfailedforlastsync",
"undeliverablefolder",
"lastmailboxforcedunlockoccurredon",
"verboseloggingenabled",
"statecode",
"hostid",
"receivingpostponeduntil"})
@JsonInclude(Include.NON_NULL)
public class Mailbox extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.mailbox";
}
@JsonProperty("testemailconfigurationretrycount")
protected Integer testemailconfigurationretrycount;
@JsonProperty("noactcount")
protected Integer noactcount;
@JsonProperty("isactsyncorgflagset")
protected Boolean isactsyncorgflagset;
@JsonProperty("exchangesyncstatexml")
protected String exchangesyncstatexml;
@JsonProperty("isforwardmailbox")
protected Boolean isforwardmailbox;
@JsonProperty("_emailserverprofile_value")
protected String _emailserverprofile_value;
@JsonProperty("isemailaddressapprovedbyo365admin")
protected Boolean isemailaddressapprovedbyo365admin;
@JsonProperty("actdeliverymethod")
protected Integer actdeliverymethod;
@JsonProperty("mailboxprocessingcontext")
protected Integer mailboxprocessingcontext;
@JsonProperty("folderhierarchy")
protected String folderhierarchy;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("outgoingemaildeliverymethod")
protected Integer outgoingemaildeliverymethod;
@JsonProperty("exchangecontactsimportstatus")
protected Integer exchangecontactsimportstatus;
@JsonProperty("entityimageid")
protected String entityimageid;
@JsonProperty("officeappsdeploymentscheduled")
protected Boolean officeappsdeploymentscheduled;
@JsonProperty("mailboxid")
protected String mailboxid;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("incomingemaildeliverymethod")
protected Integer incomingemaildeliverymethod;
@JsonProperty("forcedunlockcount")
protected Integer forcedunlockcount;
@JsonProperty("lastsuccessfulsynccompletedon")
protected OffsetDateTime lastsuccessfulsynccompletedon;
@JsonProperty("averagetotalduration")
protected Integer averagetotalduration;
@JsonProperty("oauthtokenexpireson")
protected OffsetDateTime oauthtokenexpireson;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("lastsyncerror")
protected String lastsyncerror;
@JsonProperty("postponeofficeappsdeploymentuntil")
protected OffsetDateTime postponeofficeappsdeploymentuntil;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("oauthrefreshtoken")
protected String oauthrefreshtoken;
@JsonProperty("_organizationid_value")
protected String _organizationid_value;
@JsonProperty("lastduration")
protected Integer lastduration;
@JsonProperty("testemailconfigurationscheduled")
protected Boolean testemailconfigurationscheduled;
@JsonProperty("officeappsdeploymenterror")
protected String officeappsdeploymenterror;
@JsonProperty("enabledforact")
protected Boolean enabledforact;
@JsonProperty("outgoingemailstatus")
protected Integer outgoingemailstatus;
@JsonProperty("lastsyncerrorcount")
protected Integer lastsyncerrorcount;
@JsonProperty("emailaddress")
protected String emailaddress;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("lastsyncerroroccurredon")
protected OffsetDateTime lastsyncerroroccurredon;
@JsonProperty("processanddeleteemails")
protected Boolean processanddeleteemails;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("allowemailconnectortousecredentials")
protected Boolean allowemailconnectortousecredentials;
@JsonProperty("itemsprocessedforlastsync")
protected Integer itemsprocessedforlastsync;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("officeappsdeploymentcompleteon")
protected OffsetDateTime officeappsdeploymentcompleteon;
@JsonProperty("processinglastattemptedon")
protected OffsetDateTime processinglastattemptedon;
@JsonProperty("username")
protected String username;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("officeappsdeploymentstatus")
protected Integer officeappsdeploymentstatus;
@JsonProperty("postponesendinguntil")
protected OffsetDateTime postponesendinguntil;
@JsonProperty("postponetestemailconfigurationuntil")
protected OffsetDateTime postponetestemailconfigurationuntil;
@JsonProperty("actstatus")
protected Integer actstatus;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("lastsyncstartedon")
protected OffsetDateTime lastsyncstartedon;
@JsonProperty("receivingpostponeduntilforact")
protected OffsetDateTime receivingpostponeduntilforact;
@JsonProperty("processedtimes")
protected Integer processedtimes;
@JsonProperty("exchangecontactsimportcompletedon")
protected OffsetDateTime exchangecontactsimportcompletedon;
@JsonProperty("ispasswordset")
protected Boolean ispasswordset;
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("lastsyncerrormachinename")
protected String lastsyncerrormachinename;
@JsonProperty("_regardingobjectid_value")
protected String _regardingobjectid_value;
@JsonProperty("ewsurl")
protected String ewsurl;
@JsonProperty("processingstatecode")
protected Integer processingstatecode;
@JsonProperty("transientfailurecount")
protected Integer transientfailurecount;
@JsonProperty("lastsyncerrorcode")
protected Integer lastsyncerrorcode;
@JsonProperty("password")
protected String password;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("isoauthrefreshtokenset")
protected Boolean isoauthrefreshtokenset;
@JsonProperty("postponemailboxprocessinguntil")
protected OffsetDateTime postponemailboxprocessinguntil;
@JsonProperty("testmailboxaccesscompletedon")
protected OffsetDateTime testmailboxaccesscompletedon;
@JsonProperty("processemailreceivedafter")
protected OffsetDateTime processemailreceivedafter;
@JsonProperty("emailrouteraccessapproval")
protected Integer emailrouteraccessapproval;
@JsonProperty("mailboxstatus")
protected Integer mailboxstatus;
@JsonProperty("noemailcount")
protected Integer noemailcount;
@JsonProperty("incomingemailstatus")
protected Integer incomingemailstatus;
@JsonProperty("enabledforoutgoingemail")
protected Boolean enabledforoutgoingemail;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("isoauthaccesstokenset")
protected Boolean isoauthaccesstokenset;
@JsonProperty("oauthaccesstoken")
protected String oauthaccesstoken;
@JsonProperty("enabledforincomingemail")
protected Boolean enabledforincomingemail;
@JsonProperty("isexchangecontactsimportscheduled")
protected Boolean isexchangecontactsimportscheduled;
@JsonProperty("name")
protected String name;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("isserviceaccount")
protected Boolean isserviceaccount;
@JsonProperty("lastautodiscoveredon")
protected OffsetDateTime lastautodiscoveredon;
@JsonProperty("orgmarkedasprimaryforexchangesync")
protected Boolean orgmarkedasprimaryforexchangesync;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("itemsfailedforlastsync")
protected Integer itemsfailedforlastsync;
@JsonProperty("undeliverablefolder")
protected String undeliverablefolder;
@JsonProperty("lastmailboxforcedunlockoccurredon")
protected OffsetDateTime lastmailboxforcedunlockoccurredon;
@JsonProperty("verboseloggingenabled")
protected Integer verboseloggingenabled;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("hostid")
protected String hostid;
@JsonProperty("receivingpostponeduntil")
protected OffsetDateTime receivingpostponeduntil;
protected Mailbox() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMailbox() {
return new Builder();
}
public static final class Builder {
private Integer testemailconfigurationretrycount;
private Integer noactcount;
private Boolean isactsyncorgflagset;
private String exchangesyncstatexml;
private Boolean isforwardmailbox;
private String _emailserverprofile_value;
private Boolean isemailaddressapprovedbyo365admin;
private Integer actdeliverymethod;
private Integer mailboxprocessingcontext;
private String folderhierarchy;
private String _modifiedby_value;
private Integer outgoingemaildeliverymethod;
private Integer exchangecontactsimportstatus;
private String entityimageid;
private Boolean officeappsdeploymentscheduled;
private String mailboxid;
private Integer timezoneruleversionnumber;
private Integer incomingemaildeliverymethod;
private Integer forcedunlockcount;
private OffsetDateTime lastsuccessfulsynccompletedon;
private Integer averagetotalduration;
private OffsetDateTime oauthtokenexpireson;
private String _owninguser_value;
private OffsetDateTime modifiedon;
private String lastsyncerror;
private OffsetDateTime postponeofficeappsdeploymentuntil;
private OffsetDateTime createdon;
private Long versionnumber;
private String oauthrefreshtoken;
private String _organizationid_value;
private Integer lastduration;
private Boolean testemailconfigurationscheduled;
private String officeappsdeploymenterror;
private Boolean enabledforact;
private Integer outgoingemailstatus;
private Integer lastsyncerrorcount;
private String emailaddress;
private Integer utcconversiontimezonecode;
private OffsetDateTime lastsyncerroroccurredon;
private Boolean processanddeleteemails;
private byte[] entityimage;
private Boolean allowemailconnectortousecredentials;
private Integer itemsprocessedforlastsync;
private Long entityimage_timestamp;
private OffsetDateTime officeappsdeploymentcompleteon;
private OffsetDateTime processinglastattemptedon;
private String username;
private String _modifiedonbehalfby_value;
private Integer officeappsdeploymentstatus;
private OffsetDateTime postponesendinguntil;
private OffsetDateTime postponetestemailconfigurationuntil;
private Integer actstatus;
private Integer statuscode;
private OffsetDateTime lastsyncstartedon;
private OffsetDateTime receivingpostponeduntilforact;
private Integer processedtimes;
private OffsetDateTime exchangecontactsimportcompletedon;
private Boolean ispasswordset;
private String _owningteam_value;
private String _ownerid_value;
private String lastsyncerrormachinename;
private String _regardingobjectid_value;
private String ewsurl;
private Integer processingstatecode;
private Integer transientfailurecount;
private Integer lastsyncerrorcode;
private String password;
private String _createdonbehalfby_value;
private Boolean isoauthrefreshtokenset;
private OffsetDateTime postponemailboxprocessinguntil;
private OffsetDateTime testmailboxaccesscompletedon;
private OffsetDateTime processemailreceivedafter;
private Integer emailrouteraccessapproval;
private Integer mailboxstatus;
private Integer noemailcount;
private Integer incomingemailstatus;
private Boolean enabledforoutgoingemail;
private String _createdby_value;
private Boolean isoauthaccesstokenset;
private String oauthaccesstoken;
private Boolean enabledforincomingemail;
private Boolean isexchangecontactsimportscheduled;
private String name;
private String _owningbusinessunit_value;
private Boolean isserviceaccount;
private OffsetDateTime lastautodiscoveredon;
private Boolean orgmarkedasprimaryforexchangesync;
private String entityimage_url;
private Integer itemsfailedforlastsync;
private String undeliverablefolder;
private OffsetDateTime lastmailboxforcedunlockoccurredon;
private Integer verboseloggingenabled;
private Integer statecode;
private String hostid;
private OffsetDateTime receivingpostponeduntil;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder testemailconfigurationretrycount(Integer testemailconfigurationretrycount) {
this.testemailconfigurationretrycount = testemailconfigurationretrycount;
this.changedFields = changedFields.add("testemailconfigurationretrycount");
return this;
}
public Builder noactcount(Integer noactcount) {
this.noactcount = noactcount;
this.changedFields = changedFields.add("noactcount");
return this;
}
public Builder isactsyncorgflagset(Boolean isactsyncorgflagset) {
this.isactsyncorgflagset = isactsyncorgflagset;
this.changedFields = changedFields.add("isactsyncorgflagset");
return this;
}
public Builder exchangesyncstatexml(String exchangesyncstatexml) {
this.exchangesyncstatexml = exchangesyncstatexml;
this.changedFields = changedFields.add("exchangesyncstatexml");
return this;
}
public Builder isforwardmailbox(Boolean isforwardmailbox) {
this.isforwardmailbox = isforwardmailbox;
this.changedFields = changedFields.add("isforwardmailbox");
return this;
}
public Builder _emailserverprofile_value(String _emailserverprofile_value) {
this._emailserverprofile_value = _emailserverprofile_value;
this.changedFields = changedFields.add("_emailserverprofile_value");
return this;
}
public Builder isemailaddressapprovedbyo365admin(Boolean isemailaddressapprovedbyo365admin) {
this.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
this.changedFields = changedFields.add("isemailaddressapprovedbyo365admin");
return this;
}
public Builder actdeliverymethod(Integer actdeliverymethod) {
this.actdeliverymethod = actdeliverymethod;
this.changedFields = changedFields.add("actdeliverymethod");
return this;
}
public Builder mailboxprocessingcontext(Integer mailboxprocessingcontext) {
this.mailboxprocessingcontext = mailboxprocessingcontext;
this.changedFields = changedFields.add("mailboxprocessingcontext");
return this;
}
public Builder folderhierarchy(String folderhierarchy) {
this.folderhierarchy = folderhierarchy;
this.changedFields = changedFields.add("folderhierarchy");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder outgoingemaildeliverymethod(Integer outgoingemaildeliverymethod) {
this.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
this.changedFields = changedFields.add("outgoingemaildeliverymethod");
return this;
}
public Builder exchangecontactsimportstatus(Integer exchangecontactsimportstatus) {
this.exchangecontactsimportstatus = exchangecontactsimportstatus;
this.changedFields = changedFields.add("exchangecontactsimportstatus");
return this;
}
public Builder entityimageid(String entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder officeappsdeploymentscheduled(Boolean officeappsdeploymentscheduled) {
this.officeappsdeploymentscheduled = officeappsdeploymentscheduled;
this.changedFields = changedFields.add("officeappsdeploymentscheduled");
return this;
}
public Builder mailboxid(String mailboxid) {
this.mailboxid = mailboxid;
this.changedFields = changedFields.add("mailboxid");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder incomingemaildeliverymethod(Integer incomingemaildeliverymethod) {
this.incomingemaildeliverymethod = incomingemaildeliverymethod;
this.changedFields = changedFields.add("incomingemaildeliverymethod");
return this;
}
public Builder forcedunlockcount(Integer forcedunlockcount) {
this.forcedunlockcount = forcedunlockcount;
this.changedFields = changedFields.add("forcedunlockcount");
return this;
}
public Builder lastsuccessfulsynccompletedon(OffsetDateTime lastsuccessfulsynccompletedon) {
this.lastsuccessfulsynccompletedon = lastsuccessfulsynccompletedon;
this.changedFields = changedFields.add("lastsuccessfulsynccompletedon");
return this;
}
public Builder averagetotalduration(Integer averagetotalduration) {
this.averagetotalduration = averagetotalduration;
this.changedFields = changedFields.add("averagetotalduration");
return this;
}
public Builder oauthtokenexpireson(OffsetDateTime oauthtokenexpireson) {
this.oauthtokenexpireson = oauthtokenexpireson;
this.changedFields = changedFields.add("oauthtokenexpireson");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder lastsyncerror(String lastsyncerror) {
this.lastsyncerror = lastsyncerror;
this.changedFields = changedFields.add("lastsyncerror");
return this;
}
public Builder postponeofficeappsdeploymentuntil(OffsetDateTime postponeofficeappsdeploymentuntil) {
this.postponeofficeappsdeploymentuntil = postponeofficeappsdeploymentuntil;
this.changedFields = changedFields.add("postponeofficeappsdeploymentuntil");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder oauthrefreshtoken(String oauthrefreshtoken) {
this.oauthrefreshtoken = oauthrefreshtoken;
this.changedFields = changedFields.add("oauthrefreshtoken");
return this;
}
public Builder _organizationid_value(String _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder lastduration(Integer lastduration) {
this.lastduration = lastduration;
this.changedFields = changedFields.add("lastduration");
return this;
}
public Builder testemailconfigurationscheduled(Boolean testemailconfigurationscheduled) {
this.testemailconfigurationscheduled = testemailconfigurationscheduled;
this.changedFields = changedFields.add("testemailconfigurationscheduled");
return this;
}
public Builder officeappsdeploymenterror(String officeappsdeploymenterror) {
this.officeappsdeploymenterror = officeappsdeploymenterror;
this.changedFields = changedFields.add("officeappsdeploymenterror");
return this;
}
public Builder enabledforact(Boolean enabledforact) {
this.enabledforact = enabledforact;
this.changedFields = changedFields.add("enabledforact");
return this;
}
public Builder outgoingemailstatus(Integer outgoingemailstatus) {
this.outgoingemailstatus = outgoingemailstatus;
this.changedFields = changedFields.add("outgoingemailstatus");
return this;
}
public Builder lastsyncerrorcount(Integer lastsyncerrorcount) {
this.lastsyncerrorcount = lastsyncerrorcount;
this.changedFields = changedFields.add("lastsyncerrorcount");
return this;
}
public Builder emailaddress(String emailaddress) {
this.emailaddress = emailaddress;
this.changedFields = changedFields.add("emailaddress");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder lastsyncerroroccurredon(OffsetDateTime lastsyncerroroccurredon) {
this.lastsyncerroroccurredon = lastsyncerroroccurredon;
this.changedFields = changedFields.add("lastsyncerroroccurredon");
return this;
}
public Builder processanddeleteemails(Boolean processanddeleteemails) {
this.processanddeleteemails = processanddeleteemails;
this.changedFields = changedFields.add("processanddeleteemails");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder allowemailconnectortousecredentials(Boolean allowemailconnectortousecredentials) {
this.allowemailconnectortousecredentials = allowemailconnectortousecredentials;
this.changedFields = changedFields.add("allowemailconnectortousecredentials");
return this;
}
public Builder itemsprocessedforlastsync(Integer itemsprocessedforlastsync) {
this.itemsprocessedforlastsync = itemsprocessedforlastsync;
this.changedFields = changedFields.add("itemsprocessedforlastsync");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder officeappsdeploymentcompleteon(OffsetDateTime officeappsdeploymentcompleteon) {
this.officeappsdeploymentcompleteon = officeappsdeploymentcompleteon;
this.changedFields = changedFields.add("officeappsdeploymentcompleteon");
return this;
}
public Builder processinglastattemptedon(OffsetDateTime processinglastattemptedon) {
this.processinglastattemptedon = processinglastattemptedon;
this.changedFields = changedFields.add("processinglastattemptedon");
return this;
}
public Builder username(String username) {
this.username = username;
this.changedFields = changedFields.add("username");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder officeappsdeploymentstatus(Integer officeappsdeploymentstatus) {
this.officeappsdeploymentstatus = officeappsdeploymentstatus;
this.changedFields = changedFields.add("officeappsdeploymentstatus");
return this;
}
public Builder postponesendinguntil(OffsetDateTime postponesendinguntil) {
this.postponesendinguntil = postponesendinguntil;
this.changedFields = changedFields.add("postponesendinguntil");
return this;
}
public Builder postponetestemailconfigurationuntil(OffsetDateTime postponetestemailconfigurationuntil) {
this.postponetestemailconfigurationuntil = postponetestemailconfigurationuntil;
this.changedFields = changedFields.add("postponetestemailconfigurationuntil");
return this;
}
public Builder actstatus(Integer actstatus) {
this.actstatus = actstatus;
this.changedFields = changedFields.add("actstatus");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder lastsyncstartedon(OffsetDateTime lastsyncstartedon) {
this.lastsyncstartedon = lastsyncstartedon;
this.changedFields = changedFields.add("lastsyncstartedon");
return this;
}
public Builder receivingpostponeduntilforact(OffsetDateTime receivingpostponeduntilforact) {
this.receivingpostponeduntilforact = receivingpostponeduntilforact;
this.changedFields = changedFields.add("receivingpostponeduntilforact");
return this;
}
public Builder processedtimes(Integer processedtimes) {
this.processedtimes = processedtimes;
this.changedFields = changedFields.add("processedtimes");
return this;
}
public Builder exchangecontactsimportcompletedon(OffsetDateTime exchangecontactsimportcompletedon) {
this.exchangecontactsimportcompletedon = exchangecontactsimportcompletedon;
this.changedFields = changedFields.add("exchangecontactsimportcompletedon");
return this;
}
public Builder ispasswordset(Boolean ispasswordset) {
this.ispasswordset = ispasswordset;
this.changedFields = changedFields.add("ispasswordset");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder lastsyncerrormachinename(String lastsyncerrormachinename) {
this.lastsyncerrormachinename = lastsyncerrormachinename;
this.changedFields = changedFields.add("lastsyncerrormachinename");
return this;
}
public Builder _regardingobjectid_value(String _regardingobjectid_value) {
this._regardingobjectid_value = _regardingobjectid_value;
this.changedFields = changedFields.add("_regardingobjectid_value");
return this;
}
public Builder ewsurl(String ewsurl) {
this.ewsurl = ewsurl;
this.changedFields = changedFields.add("ewsurl");
return this;
}
public Builder processingstatecode(Integer processingstatecode) {
this.processingstatecode = processingstatecode;
this.changedFields = changedFields.add("processingstatecode");
return this;
}
public Builder transientfailurecount(Integer transientfailurecount) {
this.transientfailurecount = transientfailurecount;
this.changedFields = changedFields.add("transientfailurecount");
return this;
}
public Builder lastsyncerrorcode(Integer lastsyncerrorcode) {
this.lastsyncerrorcode = lastsyncerrorcode;
this.changedFields = changedFields.add("lastsyncerrorcode");
return this;
}
public Builder password(String password) {
this.password = password;
this.changedFields = changedFields.add("password");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder isoauthrefreshtokenset(Boolean isoauthrefreshtokenset) {
this.isoauthrefreshtokenset = isoauthrefreshtokenset;
this.changedFields = changedFields.add("isoauthrefreshtokenset");
return this;
}
public Builder postponemailboxprocessinguntil(OffsetDateTime postponemailboxprocessinguntil) {
this.postponemailboxprocessinguntil = postponemailboxprocessinguntil;
this.changedFields = changedFields.add("postponemailboxprocessinguntil");
return this;
}
public Builder testmailboxaccesscompletedon(OffsetDateTime testmailboxaccesscompletedon) {
this.testmailboxaccesscompletedon = testmailboxaccesscompletedon;
this.changedFields = changedFields.add("testmailboxaccesscompletedon");
return this;
}
public Builder processemailreceivedafter(OffsetDateTime processemailreceivedafter) {
this.processemailreceivedafter = processemailreceivedafter;
this.changedFields = changedFields.add("processemailreceivedafter");
return this;
}
public Builder emailrouteraccessapproval(Integer emailrouteraccessapproval) {
this.emailrouteraccessapproval = emailrouteraccessapproval;
this.changedFields = changedFields.add("emailrouteraccessapproval");
return this;
}
public Builder mailboxstatus(Integer mailboxstatus) {
this.mailboxstatus = mailboxstatus;
this.changedFields = changedFields.add("mailboxstatus");
return this;
}
public Builder noemailcount(Integer noemailcount) {
this.noemailcount = noemailcount;
this.changedFields = changedFields.add("noemailcount");
return this;
}
public Builder incomingemailstatus(Integer incomingemailstatus) {
this.incomingemailstatus = incomingemailstatus;
this.changedFields = changedFields.add("incomingemailstatus");
return this;
}
public Builder enabledforoutgoingemail(Boolean enabledforoutgoingemail) {
this.enabledforoutgoingemail = enabledforoutgoingemail;
this.changedFields = changedFields.add("enabledforoutgoingemail");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder isoauthaccesstokenset(Boolean isoauthaccesstokenset) {
this.isoauthaccesstokenset = isoauthaccesstokenset;
this.changedFields = changedFields.add("isoauthaccesstokenset");
return this;
}
public Builder oauthaccesstoken(String oauthaccesstoken) {
this.oauthaccesstoken = oauthaccesstoken;
this.changedFields = changedFields.add("oauthaccesstoken");
return this;
}
public Builder enabledforincomingemail(Boolean enabledforincomingemail) {
this.enabledforincomingemail = enabledforincomingemail;
this.changedFields = changedFields.add("enabledforincomingemail");
return this;
}
public Builder isexchangecontactsimportscheduled(Boolean isexchangecontactsimportscheduled) {
this.isexchangecontactsimportscheduled = isexchangecontactsimportscheduled;
this.changedFields = changedFields.add("isexchangecontactsimportscheduled");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder isserviceaccount(Boolean isserviceaccount) {
this.isserviceaccount = isserviceaccount;
this.changedFields = changedFields.add("isserviceaccount");
return this;
}
public Builder lastautodiscoveredon(OffsetDateTime lastautodiscoveredon) {
this.lastautodiscoveredon = lastautodiscoveredon;
this.changedFields = changedFields.add("lastautodiscoveredon");
return this;
}
public Builder orgmarkedasprimaryforexchangesync(Boolean orgmarkedasprimaryforexchangesync) {
this.orgmarkedasprimaryforexchangesync = orgmarkedasprimaryforexchangesync;
this.changedFields = changedFields.add("orgmarkedasprimaryforexchangesync");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder itemsfailedforlastsync(Integer itemsfailedforlastsync) {
this.itemsfailedforlastsync = itemsfailedforlastsync;
this.changedFields = changedFields.add("itemsfailedforlastsync");
return this;
}
public Builder undeliverablefolder(String undeliverablefolder) {
this.undeliverablefolder = undeliverablefolder;
this.changedFields = changedFields.add("undeliverablefolder");
return this;
}
public Builder lastmailboxforcedunlockoccurredon(OffsetDateTime lastmailboxforcedunlockoccurredon) {
this.lastmailboxforcedunlockoccurredon = lastmailboxforcedunlockoccurredon;
this.changedFields = changedFields.add("lastmailboxforcedunlockoccurredon");
return this;
}
public Builder verboseloggingenabled(Integer verboseloggingenabled) {
this.verboseloggingenabled = verboseloggingenabled;
this.changedFields = changedFields.add("verboseloggingenabled");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder hostid(String hostid) {
this.hostid = hostid;
this.changedFields = changedFields.add("hostid");
return this;
}
public Builder receivingpostponeduntil(OffsetDateTime receivingpostponeduntil) {
this.receivingpostponeduntil = receivingpostponeduntil;
this.changedFields = changedFields.add("receivingpostponeduntil");
return this;
}
public Mailbox build() {
Mailbox _x = new Mailbox();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.mailbox";
_x.testemailconfigurationretrycount = testemailconfigurationretrycount;
_x.noactcount = noactcount;
_x.isactsyncorgflagset = isactsyncorgflagset;
_x.exchangesyncstatexml = exchangesyncstatexml;
_x.isforwardmailbox = isforwardmailbox;
_x._emailserverprofile_value = _emailserverprofile_value;
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
_x.actdeliverymethod = actdeliverymethod;
_x.mailboxprocessingcontext = mailboxprocessingcontext;
_x.folderhierarchy = folderhierarchy;
_x._modifiedby_value = _modifiedby_value;
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
_x.exchangecontactsimportstatus = exchangecontactsimportstatus;
_x.entityimageid = entityimageid;
_x.officeappsdeploymentscheduled = officeappsdeploymentscheduled;
_x.mailboxid = mailboxid;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
_x.forcedunlockcount = forcedunlockcount;
_x.lastsuccessfulsynccompletedon = lastsuccessfulsynccompletedon;
_x.averagetotalduration = averagetotalduration;
_x.oauthtokenexpireson = oauthtokenexpireson;
_x._owninguser_value = _owninguser_value;
_x.modifiedon = modifiedon;
_x.lastsyncerror = lastsyncerror;
_x.postponeofficeappsdeploymentuntil = postponeofficeappsdeploymentuntil;
_x.createdon = createdon;
_x.versionnumber = versionnumber;
_x.oauthrefreshtoken = oauthrefreshtoken;
_x._organizationid_value = _organizationid_value;
_x.lastduration = lastduration;
_x.testemailconfigurationscheduled = testemailconfigurationscheduled;
_x.officeappsdeploymenterror = officeappsdeploymenterror;
_x.enabledforact = enabledforact;
_x.outgoingemailstatus = outgoingemailstatus;
_x.lastsyncerrorcount = lastsyncerrorcount;
_x.emailaddress = emailaddress;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.lastsyncerroroccurredon = lastsyncerroroccurredon;
_x.processanddeleteemails = processanddeleteemails;
_x.entityimage = entityimage;
_x.allowemailconnectortousecredentials = allowemailconnectortousecredentials;
_x.itemsprocessedforlastsync = itemsprocessedforlastsync;
_x.entityimage_timestamp = entityimage_timestamp;
_x.officeappsdeploymentcompleteon = officeappsdeploymentcompleteon;
_x.processinglastattemptedon = processinglastattemptedon;
_x.username = username;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.officeappsdeploymentstatus = officeappsdeploymentstatus;
_x.postponesendinguntil = postponesendinguntil;
_x.postponetestemailconfigurationuntil = postponetestemailconfigurationuntil;
_x.actstatus = actstatus;
_x.statuscode = statuscode;
_x.lastsyncstartedon = lastsyncstartedon;
_x.receivingpostponeduntilforact = receivingpostponeduntilforact;
_x.processedtimes = processedtimes;
_x.exchangecontactsimportcompletedon = exchangecontactsimportcompletedon;
_x.ispasswordset = ispasswordset;
_x._owningteam_value = _owningteam_value;
_x._ownerid_value = _ownerid_value;
_x.lastsyncerrormachinename = lastsyncerrormachinename;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.ewsurl = ewsurl;
_x.processingstatecode = processingstatecode;
_x.transientfailurecount = transientfailurecount;
_x.lastsyncerrorcode = lastsyncerrorcode;
_x.password = password;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.isoauthrefreshtokenset = isoauthrefreshtokenset;
_x.postponemailboxprocessinguntil = postponemailboxprocessinguntil;
_x.testmailboxaccesscompletedon = testmailboxaccesscompletedon;
_x.processemailreceivedafter = processemailreceivedafter;
_x.emailrouteraccessapproval = emailrouteraccessapproval;
_x.mailboxstatus = mailboxstatus;
_x.noemailcount = noemailcount;
_x.incomingemailstatus = incomingemailstatus;
_x.enabledforoutgoingemail = enabledforoutgoingemail;
_x._createdby_value = _createdby_value;
_x.isoauthaccesstokenset = isoauthaccesstokenset;
_x.oauthaccesstoken = oauthaccesstoken;
_x.enabledforincomingemail = enabledforincomingemail;
_x.isexchangecontactsimportscheduled = isexchangecontactsimportscheduled;
_x.name = name;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.isserviceaccount = isserviceaccount;
_x.lastautodiscoveredon = lastautodiscoveredon;
_x.orgmarkedasprimaryforexchangesync = orgmarkedasprimaryforexchangesync;
_x.entityimage_url = entityimage_url;
_x.itemsfailedforlastsync = itemsfailedforlastsync;
_x.undeliverablefolder = undeliverablefolder;
_x.lastmailboxforcedunlockoccurredon = lastmailboxforcedunlockoccurredon;
_x.verboseloggingenabled = verboseloggingenabled;
_x.statecode = statecode;
_x.hostid = hostid;
_x.receivingpostponeduntil = receivingpostponeduntil;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && mailboxid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(mailboxid.toString()));
}
}
@Property(name="testemailconfigurationretrycount")
@JsonIgnore
public Optional getTestemailconfigurationretrycount() {
return Optional.ofNullable(testemailconfigurationretrycount);
}
public Mailbox withTestemailconfigurationretrycount(Integer testemailconfigurationretrycount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("testemailconfigurationretrycount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.testemailconfigurationretrycount = testemailconfigurationretrycount;
return _x;
}
@Property(name="noactcount")
@JsonIgnore
public Optional getNoactcount() {
return Optional.ofNullable(noactcount);
}
public Mailbox withNoactcount(Integer noactcount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("noactcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.noactcount = noactcount;
return _x;
}
@Property(name="isactsyncorgflagset")
@JsonIgnore
public Optional getIsactsyncorgflagset() {
return Optional.ofNullable(isactsyncorgflagset);
}
public Mailbox withIsactsyncorgflagset(Boolean isactsyncorgflagset) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isactsyncorgflagset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isactsyncorgflagset = isactsyncorgflagset;
return _x;
}
@Property(name="exchangesyncstatexml")
@JsonIgnore
public Optional getExchangesyncstatexml() {
return Optional.ofNullable(exchangesyncstatexml);
}
public Mailbox withExchangesyncstatexml(String exchangesyncstatexml) {
Checks.checkIsAscii(exchangesyncstatexml);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("exchangesyncstatexml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.exchangesyncstatexml = exchangesyncstatexml;
return _x;
}
@Property(name="isforwardmailbox")
@JsonIgnore
public Optional getIsforwardmailbox() {
return Optional.ofNullable(isforwardmailbox);
}
public Mailbox withIsforwardmailbox(Boolean isforwardmailbox) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isforwardmailbox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isforwardmailbox = isforwardmailbox;
return _x;
}
@Property(name="_emailserverprofile_value")
@JsonIgnore
public Optional get_emailserverprofile_value() {
return Optional.ofNullable(_emailserverprofile_value);
}
public Mailbox with_emailserverprofile_value(String _emailserverprofile_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_emailserverprofile_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._emailserverprofile_value = _emailserverprofile_value;
return _x;
}
@Property(name="isemailaddressapprovedbyo365admin")
@JsonIgnore
public Optional getIsemailaddressapprovedbyo365admin() {
return Optional.ofNullable(isemailaddressapprovedbyo365admin);
}
public Mailbox withIsemailaddressapprovedbyo365admin(Boolean isemailaddressapprovedbyo365admin) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isemailaddressapprovedbyo365admin");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
return _x;
}
@Property(name="actdeliverymethod")
@JsonIgnore
public Optional getActdeliverymethod() {
return Optional.ofNullable(actdeliverymethod);
}
public Mailbox withActdeliverymethod(Integer actdeliverymethod) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("actdeliverymethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.actdeliverymethod = actdeliverymethod;
return _x;
}
@Property(name="mailboxprocessingcontext")
@JsonIgnore
public Optional getMailboxprocessingcontext() {
return Optional.ofNullable(mailboxprocessingcontext);
}
public Mailbox withMailboxprocessingcontext(Integer mailboxprocessingcontext) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("mailboxprocessingcontext");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.mailboxprocessingcontext = mailboxprocessingcontext;
return _x;
}
@Property(name="folderhierarchy")
@JsonIgnore
public Optional getFolderhierarchy() {
return Optional.ofNullable(folderhierarchy);
}
public Mailbox withFolderhierarchy(String folderhierarchy) {
Checks.checkIsAscii(folderhierarchy);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("folderhierarchy");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.folderhierarchy = folderhierarchy;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Mailbox with_modifiedby_value(String _modifiedby_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="outgoingemaildeliverymethod")
@JsonIgnore
public Optional getOutgoingemaildeliverymethod() {
return Optional.ofNullable(outgoingemaildeliverymethod);
}
public Mailbox withOutgoingemaildeliverymethod(Integer outgoingemaildeliverymethod) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("outgoingemaildeliverymethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
return _x;
}
@Property(name="exchangecontactsimportstatus")
@JsonIgnore
public Optional getExchangecontactsimportstatus() {
return Optional.ofNullable(exchangecontactsimportstatus);
}
public Mailbox withExchangecontactsimportstatus(Integer exchangecontactsimportstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("exchangecontactsimportstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.exchangecontactsimportstatus = exchangecontactsimportstatus;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Mailbox withEntityimageid(String entityimageid) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="officeappsdeploymentscheduled")
@JsonIgnore
public Optional getOfficeappsdeploymentscheduled() {
return Optional.ofNullable(officeappsdeploymentscheduled);
}
public Mailbox withOfficeappsdeploymentscheduled(Boolean officeappsdeploymentscheduled) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("officeappsdeploymentscheduled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.officeappsdeploymentscheduled = officeappsdeploymentscheduled;
return _x;
}
@Property(name="mailboxid")
@JsonIgnore
public Optional getMailboxid() {
return Optional.ofNullable(mailboxid);
}
public Mailbox withMailboxid(String mailboxid) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("mailboxid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.mailboxid = mailboxid;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Mailbox withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="incomingemaildeliverymethod")
@JsonIgnore
public Optional getIncomingemaildeliverymethod() {
return Optional.ofNullable(incomingemaildeliverymethod);
}
public Mailbox withIncomingemaildeliverymethod(Integer incomingemaildeliverymethod) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("incomingemaildeliverymethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
return _x;
}
@Property(name="forcedunlockcount")
@JsonIgnore
public Optional getForcedunlockcount() {
return Optional.ofNullable(forcedunlockcount);
}
public Mailbox withForcedunlockcount(Integer forcedunlockcount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("forcedunlockcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.forcedunlockcount = forcedunlockcount;
return _x;
}
@Property(name="lastsuccessfulsynccompletedon")
@JsonIgnore
public Optional getLastsuccessfulsynccompletedon() {
return Optional.ofNullable(lastsuccessfulsynccompletedon);
}
public Mailbox withLastsuccessfulsynccompletedon(OffsetDateTime lastsuccessfulsynccompletedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsuccessfulsynccompletedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsuccessfulsynccompletedon = lastsuccessfulsynccompletedon;
return _x;
}
@Property(name="averagetotalduration")
@JsonIgnore
public Optional getAveragetotalduration() {
return Optional.ofNullable(averagetotalduration);
}
public Mailbox withAveragetotalduration(Integer averagetotalduration) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("averagetotalduration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.averagetotalduration = averagetotalduration;
return _x;
}
@Property(name="oauthtokenexpireson")
@JsonIgnore
public Optional getOauthtokenexpireson() {
return Optional.ofNullable(oauthtokenexpireson);
}
public Mailbox withOauthtokenexpireson(OffsetDateTime oauthtokenexpireson) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("oauthtokenexpireson");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.oauthtokenexpireson = oauthtokenexpireson;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Mailbox with_owninguser_value(String _owninguser_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Mailbox withModifiedon(OffsetDateTime modifiedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="lastsyncerror")
@JsonIgnore
public Optional getLastsyncerror() {
return Optional.ofNullable(lastsyncerror);
}
public Mailbox withLastsyncerror(String lastsyncerror) {
Checks.checkIsAscii(lastsyncerror);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncerror");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncerror = lastsyncerror;
return _x;
}
@Property(name="postponeofficeappsdeploymentuntil")
@JsonIgnore
public Optional getPostponeofficeappsdeploymentuntil() {
return Optional.ofNullable(postponeofficeappsdeploymentuntil);
}
public Mailbox withPostponeofficeappsdeploymentuntil(OffsetDateTime postponeofficeappsdeploymentuntil) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("postponeofficeappsdeploymentuntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.postponeofficeappsdeploymentuntil = postponeofficeappsdeploymentuntil;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Mailbox withCreatedon(OffsetDateTime createdon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.createdon = createdon;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Mailbox withVersionnumber(Long versionnumber) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="oauthrefreshtoken")
@JsonIgnore
public Optional getOauthrefreshtoken() {
return Optional.ofNullable(oauthrefreshtoken);
}
public Mailbox withOauthrefreshtoken(String oauthrefreshtoken) {
Checks.checkIsAscii(oauthrefreshtoken);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("oauthrefreshtoken");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.oauthrefreshtoken = oauthrefreshtoken;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Mailbox with_organizationid_value(String _organizationid_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="lastduration")
@JsonIgnore
public Optional getLastduration() {
return Optional.ofNullable(lastduration);
}
public Mailbox withLastduration(Integer lastduration) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastduration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastduration = lastduration;
return _x;
}
@Property(name="testemailconfigurationscheduled")
@JsonIgnore
public Optional getTestemailconfigurationscheduled() {
return Optional.ofNullable(testemailconfigurationscheduled);
}
public Mailbox withTestemailconfigurationscheduled(Boolean testemailconfigurationscheduled) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("testemailconfigurationscheduled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.testemailconfigurationscheduled = testemailconfigurationscheduled;
return _x;
}
@Property(name="officeappsdeploymenterror")
@JsonIgnore
public Optional getOfficeappsdeploymenterror() {
return Optional.ofNullable(officeappsdeploymenterror);
}
public Mailbox withOfficeappsdeploymenterror(String officeappsdeploymenterror) {
Checks.checkIsAscii(officeappsdeploymenterror);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("officeappsdeploymenterror");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.officeappsdeploymenterror = officeappsdeploymenterror;
return _x;
}
@Property(name="enabledforact")
@JsonIgnore
public Optional getEnabledforact() {
return Optional.ofNullable(enabledforact);
}
public Mailbox withEnabledforact(Boolean enabledforact) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("enabledforact");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.enabledforact = enabledforact;
return _x;
}
@Property(name="outgoingemailstatus")
@JsonIgnore
public Optional getOutgoingemailstatus() {
return Optional.ofNullable(outgoingemailstatus);
}
public Mailbox withOutgoingemailstatus(Integer outgoingemailstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("outgoingemailstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.outgoingemailstatus = outgoingemailstatus;
return _x;
}
@Property(name="lastsyncerrorcount")
@JsonIgnore
public Optional getLastsyncerrorcount() {
return Optional.ofNullable(lastsyncerrorcount);
}
public Mailbox withLastsyncerrorcount(Integer lastsyncerrorcount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncerrorcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncerrorcount = lastsyncerrorcount;
return _x;
}
@Property(name="emailaddress")
@JsonIgnore
public Optional getEmailaddress() {
return Optional.ofNullable(emailaddress);
}
public Mailbox withEmailaddress(String emailaddress) {
Checks.checkIsAscii(emailaddress);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("emailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.emailaddress = emailaddress;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Mailbox withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="lastsyncerroroccurredon")
@JsonIgnore
public Optional getLastsyncerroroccurredon() {
return Optional.ofNullable(lastsyncerroroccurredon);
}
public Mailbox withLastsyncerroroccurredon(OffsetDateTime lastsyncerroroccurredon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncerroroccurredon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncerroroccurredon = lastsyncerroroccurredon;
return _x;
}
@Property(name="processanddeleteemails")
@JsonIgnore
public Optional getProcessanddeleteemails() {
return Optional.ofNullable(processanddeleteemails);
}
public Mailbox withProcessanddeleteemails(Boolean processanddeleteemails) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("processanddeleteemails");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.processanddeleteemails = processanddeleteemails;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Mailbox withEntityimage(byte[] entityimage) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.entityimage = entityimage;
return _x;
}
@Property(name="allowemailconnectortousecredentials")
@JsonIgnore
public Optional getAllowemailconnectortousecredentials() {
return Optional.ofNullable(allowemailconnectortousecredentials);
}
public Mailbox withAllowemailconnectortousecredentials(Boolean allowemailconnectortousecredentials) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("allowemailconnectortousecredentials");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.allowemailconnectortousecredentials = allowemailconnectortousecredentials;
return _x;
}
@Property(name="itemsprocessedforlastsync")
@JsonIgnore
public Optional getItemsprocessedforlastsync() {
return Optional.ofNullable(itemsprocessedforlastsync);
}
public Mailbox withItemsprocessedforlastsync(Integer itemsprocessedforlastsync) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("itemsprocessedforlastsync");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.itemsprocessedforlastsync = itemsprocessedforlastsync;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Mailbox withEntityimage_timestamp(Long entityimage_timestamp) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="officeappsdeploymentcompleteon")
@JsonIgnore
public Optional getOfficeappsdeploymentcompleteon() {
return Optional.ofNullable(officeappsdeploymentcompleteon);
}
public Mailbox withOfficeappsdeploymentcompleteon(OffsetDateTime officeappsdeploymentcompleteon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("officeappsdeploymentcompleteon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.officeappsdeploymentcompleteon = officeappsdeploymentcompleteon;
return _x;
}
@Property(name="processinglastattemptedon")
@JsonIgnore
public Optional getProcessinglastattemptedon() {
return Optional.ofNullable(processinglastattemptedon);
}
public Mailbox withProcessinglastattemptedon(OffsetDateTime processinglastattemptedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("processinglastattemptedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.processinglastattemptedon = processinglastattemptedon;
return _x;
}
@Property(name="username")
@JsonIgnore
public Optional getUsername() {
return Optional.ofNullable(username);
}
public Mailbox withUsername(String username) {
Checks.checkIsAscii(username);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("username");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.username = username;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Mailbox with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="officeappsdeploymentstatus")
@JsonIgnore
public Optional getOfficeappsdeploymentstatus() {
return Optional.ofNullable(officeappsdeploymentstatus);
}
public Mailbox withOfficeappsdeploymentstatus(Integer officeappsdeploymentstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("officeappsdeploymentstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.officeappsdeploymentstatus = officeappsdeploymentstatus;
return _x;
}
@Property(name="postponesendinguntil")
@JsonIgnore
public Optional getPostponesendinguntil() {
return Optional.ofNullable(postponesendinguntil);
}
public Mailbox withPostponesendinguntil(OffsetDateTime postponesendinguntil) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("postponesendinguntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.postponesendinguntil = postponesendinguntil;
return _x;
}
@Property(name="postponetestemailconfigurationuntil")
@JsonIgnore
public Optional getPostponetestemailconfigurationuntil() {
return Optional.ofNullable(postponetestemailconfigurationuntil);
}
public Mailbox withPostponetestemailconfigurationuntil(OffsetDateTime postponetestemailconfigurationuntil) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("postponetestemailconfigurationuntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.postponetestemailconfigurationuntil = postponetestemailconfigurationuntil;
return _x;
}
@Property(name="actstatus")
@JsonIgnore
public Optional getActstatus() {
return Optional.ofNullable(actstatus);
}
public Mailbox withActstatus(Integer actstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("actstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.actstatus = actstatus;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Mailbox withStatuscode(Integer statuscode) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.statuscode = statuscode;
return _x;
}
@Property(name="lastsyncstartedon")
@JsonIgnore
public Optional getLastsyncstartedon() {
return Optional.ofNullable(lastsyncstartedon);
}
public Mailbox withLastsyncstartedon(OffsetDateTime lastsyncstartedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncstartedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncstartedon = lastsyncstartedon;
return _x;
}
@Property(name="receivingpostponeduntilforact")
@JsonIgnore
public Optional getReceivingpostponeduntilforact() {
return Optional.ofNullable(receivingpostponeduntilforact);
}
public Mailbox withReceivingpostponeduntilforact(OffsetDateTime receivingpostponeduntilforact) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("receivingpostponeduntilforact");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.receivingpostponeduntilforact = receivingpostponeduntilforact;
return _x;
}
@Property(name="processedtimes")
@JsonIgnore
public Optional getProcessedtimes() {
return Optional.ofNullable(processedtimes);
}
public Mailbox withProcessedtimes(Integer processedtimes) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("processedtimes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.processedtimes = processedtimes;
return _x;
}
@Property(name="exchangecontactsimportcompletedon")
@JsonIgnore
public Optional getExchangecontactsimportcompletedon() {
return Optional.ofNullable(exchangecontactsimportcompletedon);
}
public Mailbox withExchangecontactsimportcompletedon(OffsetDateTime exchangecontactsimportcompletedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("exchangecontactsimportcompletedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.exchangecontactsimportcompletedon = exchangecontactsimportcompletedon;
return _x;
}
@Property(name="ispasswordset")
@JsonIgnore
public Optional getIspasswordset() {
return Optional.ofNullable(ispasswordset);
}
public Mailbox withIspasswordset(Boolean ispasswordset) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("ispasswordset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.ispasswordset = ispasswordset;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Mailbox with_owningteam_value(String _owningteam_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Mailbox with_ownerid_value(String _ownerid_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="lastsyncerrormachinename")
@JsonIgnore
public Optional getLastsyncerrormachinename() {
return Optional.ofNullable(lastsyncerrormachinename);
}
public Mailbox withLastsyncerrormachinename(String lastsyncerrormachinename) {
Checks.checkIsAscii(lastsyncerrormachinename);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncerrormachinename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncerrormachinename = lastsyncerrormachinename;
return _x;
}
@Property(name="_regardingobjectid_value")
@JsonIgnore
public Optional get_regardingobjectid_value() {
return Optional.ofNullable(_regardingobjectid_value);
}
public Mailbox with_regardingobjectid_value(String _regardingobjectid_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_regardingobjectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._regardingobjectid_value = _regardingobjectid_value;
return _x;
}
@Property(name="ewsurl")
@JsonIgnore
public Optional getEwsurl() {
return Optional.ofNullable(ewsurl);
}
public Mailbox withEwsurl(String ewsurl) {
Checks.checkIsAscii(ewsurl);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("ewsurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.ewsurl = ewsurl;
return _x;
}
@Property(name="processingstatecode")
@JsonIgnore
public Optional getProcessingstatecode() {
return Optional.ofNullable(processingstatecode);
}
public Mailbox withProcessingstatecode(Integer processingstatecode) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("processingstatecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.processingstatecode = processingstatecode;
return _x;
}
@Property(name="transientfailurecount")
@JsonIgnore
public Optional getTransientfailurecount() {
return Optional.ofNullable(transientfailurecount);
}
public Mailbox withTransientfailurecount(Integer transientfailurecount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("transientfailurecount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.transientfailurecount = transientfailurecount;
return _x;
}
@Property(name="lastsyncerrorcode")
@JsonIgnore
public Optional getLastsyncerrorcode() {
return Optional.ofNullable(lastsyncerrorcode);
}
public Mailbox withLastsyncerrorcode(Integer lastsyncerrorcode) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastsyncerrorcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastsyncerrorcode = lastsyncerrorcode;
return _x;
}
@Property(name="password")
@JsonIgnore
public Optional getPassword() {
return Optional.ofNullable(password);
}
public Mailbox withPassword(String password) {
Checks.checkIsAscii(password);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("password");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.password = password;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Mailbox with_createdonbehalfby_value(String _createdonbehalfby_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="isoauthrefreshtokenset")
@JsonIgnore
public Optional getIsoauthrefreshtokenset() {
return Optional.ofNullable(isoauthrefreshtokenset);
}
public Mailbox withIsoauthrefreshtokenset(Boolean isoauthrefreshtokenset) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isoauthrefreshtokenset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isoauthrefreshtokenset = isoauthrefreshtokenset;
return _x;
}
@Property(name="postponemailboxprocessinguntil")
@JsonIgnore
public Optional getPostponemailboxprocessinguntil() {
return Optional.ofNullable(postponemailboxprocessinguntil);
}
public Mailbox withPostponemailboxprocessinguntil(OffsetDateTime postponemailboxprocessinguntil) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("postponemailboxprocessinguntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.postponemailboxprocessinguntil = postponemailboxprocessinguntil;
return _x;
}
@Property(name="testmailboxaccesscompletedon")
@JsonIgnore
public Optional getTestmailboxaccesscompletedon() {
return Optional.ofNullable(testmailboxaccesscompletedon);
}
public Mailbox withTestmailboxaccesscompletedon(OffsetDateTime testmailboxaccesscompletedon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("testmailboxaccesscompletedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.testmailboxaccesscompletedon = testmailboxaccesscompletedon;
return _x;
}
@Property(name="processemailreceivedafter")
@JsonIgnore
public Optional getProcessemailreceivedafter() {
return Optional.ofNullable(processemailreceivedafter);
}
public Mailbox withProcessemailreceivedafter(OffsetDateTime processemailreceivedafter) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("processemailreceivedafter");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.processemailreceivedafter = processemailreceivedafter;
return _x;
}
@Property(name="emailrouteraccessapproval")
@JsonIgnore
public Optional getEmailrouteraccessapproval() {
return Optional.ofNullable(emailrouteraccessapproval);
}
public Mailbox withEmailrouteraccessapproval(Integer emailrouteraccessapproval) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("emailrouteraccessapproval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.emailrouteraccessapproval = emailrouteraccessapproval;
return _x;
}
@Property(name="mailboxstatus")
@JsonIgnore
public Optional getMailboxstatus() {
return Optional.ofNullable(mailboxstatus);
}
public Mailbox withMailboxstatus(Integer mailboxstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("mailboxstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.mailboxstatus = mailboxstatus;
return _x;
}
@Property(name="noemailcount")
@JsonIgnore
public Optional getNoemailcount() {
return Optional.ofNullable(noemailcount);
}
public Mailbox withNoemailcount(Integer noemailcount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("noemailcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.noemailcount = noemailcount;
return _x;
}
@Property(name="incomingemailstatus")
@JsonIgnore
public Optional getIncomingemailstatus() {
return Optional.ofNullable(incomingemailstatus);
}
public Mailbox withIncomingemailstatus(Integer incomingemailstatus) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("incomingemailstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.incomingemailstatus = incomingemailstatus;
return _x;
}
@Property(name="enabledforoutgoingemail")
@JsonIgnore
public Optional getEnabledforoutgoingemail() {
return Optional.ofNullable(enabledforoutgoingemail);
}
public Mailbox withEnabledforoutgoingemail(Boolean enabledforoutgoingemail) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("enabledforoutgoingemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.enabledforoutgoingemail = enabledforoutgoingemail;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Mailbox with_createdby_value(String _createdby_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="isoauthaccesstokenset")
@JsonIgnore
public Optional getIsoauthaccesstokenset() {
return Optional.ofNullable(isoauthaccesstokenset);
}
public Mailbox withIsoauthaccesstokenset(Boolean isoauthaccesstokenset) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isoauthaccesstokenset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isoauthaccesstokenset = isoauthaccesstokenset;
return _x;
}
@Property(name="oauthaccesstoken")
@JsonIgnore
public Optional getOauthaccesstoken() {
return Optional.ofNullable(oauthaccesstoken);
}
public Mailbox withOauthaccesstoken(String oauthaccesstoken) {
Checks.checkIsAscii(oauthaccesstoken);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("oauthaccesstoken");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.oauthaccesstoken = oauthaccesstoken;
return _x;
}
@Property(name="enabledforincomingemail")
@JsonIgnore
public Optional getEnabledforincomingemail() {
return Optional.ofNullable(enabledforincomingemail);
}
public Mailbox withEnabledforincomingemail(Boolean enabledforincomingemail) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("enabledforincomingemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.enabledforincomingemail = enabledforincomingemail;
return _x;
}
@Property(name="isexchangecontactsimportscheduled")
@JsonIgnore
public Optional getIsexchangecontactsimportscheduled() {
return Optional.ofNullable(isexchangecontactsimportscheduled);
}
public Mailbox withIsexchangecontactsimportscheduled(Boolean isexchangecontactsimportscheduled) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isexchangecontactsimportscheduled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isexchangecontactsimportscheduled = isexchangecontactsimportscheduled;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Mailbox withName(String name) {
Checks.checkIsAscii(name);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.name = name;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Mailbox with_owningbusinessunit_value(String _owningbusinessunit_value) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="isserviceaccount")
@JsonIgnore
public Optional getIsserviceaccount() {
return Optional.ofNullable(isserviceaccount);
}
public Mailbox withIsserviceaccount(Boolean isserviceaccount) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("isserviceaccount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.isserviceaccount = isserviceaccount;
return _x;
}
@Property(name="lastautodiscoveredon")
@JsonIgnore
public Optional getLastautodiscoveredon() {
return Optional.ofNullable(lastautodiscoveredon);
}
public Mailbox withLastautodiscoveredon(OffsetDateTime lastautodiscoveredon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastautodiscoveredon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastautodiscoveredon = lastautodiscoveredon;
return _x;
}
@Property(name="orgmarkedasprimaryforexchangesync")
@JsonIgnore
public Optional getOrgmarkedasprimaryforexchangesync() {
return Optional.ofNullable(orgmarkedasprimaryforexchangesync);
}
public Mailbox withOrgmarkedasprimaryforexchangesync(Boolean orgmarkedasprimaryforexchangesync) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("orgmarkedasprimaryforexchangesync");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.orgmarkedasprimaryforexchangesync = orgmarkedasprimaryforexchangesync;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Mailbox withEntityimage_url(String entityimage_url) {
Checks.checkIsAscii(entityimage_url);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="itemsfailedforlastsync")
@JsonIgnore
public Optional getItemsfailedforlastsync() {
return Optional.ofNullable(itemsfailedforlastsync);
}
public Mailbox withItemsfailedforlastsync(Integer itemsfailedforlastsync) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("itemsfailedforlastsync");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.itemsfailedforlastsync = itemsfailedforlastsync;
return _x;
}
@Property(name="undeliverablefolder")
@JsonIgnore
public Optional getUndeliverablefolder() {
return Optional.ofNullable(undeliverablefolder);
}
public Mailbox withUndeliverablefolder(String undeliverablefolder) {
Checks.checkIsAscii(undeliverablefolder);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("undeliverablefolder");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.undeliverablefolder = undeliverablefolder;
return _x;
}
@Property(name="lastmailboxforcedunlockoccurredon")
@JsonIgnore
public Optional getLastmailboxforcedunlockoccurredon() {
return Optional.ofNullable(lastmailboxforcedunlockoccurredon);
}
public Mailbox withLastmailboxforcedunlockoccurredon(OffsetDateTime lastmailboxforcedunlockoccurredon) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("lastmailboxforcedunlockoccurredon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.lastmailboxforcedunlockoccurredon = lastmailboxforcedunlockoccurredon;
return _x;
}
@Property(name="verboseloggingenabled")
@JsonIgnore
public Optional getVerboseloggingenabled() {
return Optional.ofNullable(verboseloggingenabled);
}
public Mailbox withVerboseloggingenabled(Integer verboseloggingenabled) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("verboseloggingenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.verboseloggingenabled = verboseloggingenabled;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Mailbox withStatecode(Integer statecode) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.statecode = statecode;
return _x;
}
@Property(name="hostid")
@JsonIgnore
public Optional getHostid() {
return Optional.ofNullable(hostid);
}
public Mailbox withHostid(String hostid) {
Checks.checkIsAscii(hostid);
Mailbox _x = _copy();
_x.changedFields = changedFields.add("hostid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.hostid = hostid;
return _x;
}
@Property(name="receivingpostponeduntil")
@JsonIgnore
public Optional getReceivingpostponeduntil() {
return Optional.ofNullable(receivingpostponeduntil);
}
public Mailbox withReceivingpostponeduntil(OffsetDateTime receivingpostponeduntil) {
Mailbox _x = _copy();
_x.changedFields = changedFields.add("receivingpostponeduntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.mailbox");
_x.receivingpostponeduntil = receivingpostponeduntil;
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="regardingobjectid")
@JsonIgnore
public SystemuserRequest getRegardingobjectid() {
return new SystemuserRequest(contextPath.addSegment("regardingobjectid"));
}
@NavigationProperty(name="emailserverprofile")
@JsonIgnore
public EmailserverprofileRequest getEmailserverprofile() {
return new EmailserverprofileRequest(contextPath.addSegment("emailserverprofile"));
}
@NavigationProperty(name="systemuser_defaultmailbox_mailbox")
@JsonIgnore
public SystemuserCollectionRequest getSystemuser_defaultmailbox_mailbox() {
return new SystemuserCollectionRequest(
contextPath.addSegment("systemuser_defaultmailbox_mailbox"));
}
@NavigationProperty(name="queue_defaultmailbox_mailbox")
@JsonIgnore
public QueueCollectionRequest getQueue_defaultmailbox_mailbox() {
return new QueueCollectionRequest(
contextPath.addSegment("queue_defaultmailbox_mailbox"));
}
@NavigationProperty(name="tracelog_Mailbox")
@JsonIgnore
public TracelogCollectionRequest getTracelog_Mailbox() {
return new TracelogCollectionRequest(
contextPath.addSegment("tracelog_Mailbox"));
}
@NavigationProperty(name="activitypointer_sendermailboxid_mailbox")
@JsonIgnore
public ActivitypointerCollectionRequest getActivitypointer_sendermailboxid_mailbox() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("activitypointer_sendermailboxid_mailbox"));
}
@NavigationProperty(name="mailbox_asyncoperations")
@JsonIgnore
public AsyncoperationCollectionRequest getMailbox_asyncoperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("mailbox_asyncoperations"));
}
@NavigationProperty(name="regardingobjectid_queue")
@JsonIgnore
public QueueRequest getRegardingobjectid_queue() {
return new QueueRequest(contextPath.addSegment("regardingobjectid_queue"));
}
@NavigationProperty(name="email_sendermailboxid_mailbox")
@JsonIgnore
public EmailCollectionRequest getEmail_sendermailboxid_mailbox() {
return new EmailCollectionRequest(
contextPath.addSegment("email_sendermailboxid_mailbox"));
}
@NavigationProperty(name="Mailbox_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getMailbox_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Mailbox_SyncErrors"));
}
@NavigationProperty(name="Mailbox_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getMailbox_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Mailbox_Annotation"));
}
@NavigationProperty(name="Mailbox_MailboxTrackingFolder")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getMailbox_MailboxTrackingFolder() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("Mailbox_MailboxTrackingFolder"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"));
}
@NavigationProperty(name="mailbox_processsessions")
@JsonIgnore
public ProcesssessionCollectionRequest getMailbox_processsessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("mailbox_processsessions"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Mailbox patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Mailbox _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Mailbox put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Mailbox _x = _copy();
_x.changedFields = null;
return _x;
}
private Mailbox _copy() {
Mailbox _x = new Mailbox();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.testemailconfigurationretrycount = testemailconfigurationretrycount;
_x.noactcount = noactcount;
_x.isactsyncorgflagset = isactsyncorgflagset;
_x.exchangesyncstatexml = exchangesyncstatexml;
_x.isforwardmailbox = isforwardmailbox;
_x._emailserverprofile_value = _emailserverprofile_value;
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
_x.actdeliverymethod = actdeliverymethod;
_x.mailboxprocessingcontext = mailboxprocessingcontext;
_x.folderhierarchy = folderhierarchy;
_x._modifiedby_value = _modifiedby_value;
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
_x.exchangecontactsimportstatus = exchangecontactsimportstatus;
_x.entityimageid = entityimageid;
_x.officeappsdeploymentscheduled = officeappsdeploymentscheduled;
_x.mailboxid = mailboxid;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
_x.forcedunlockcount = forcedunlockcount;
_x.lastsuccessfulsynccompletedon = lastsuccessfulsynccompletedon;
_x.averagetotalduration = averagetotalduration;
_x.oauthtokenexpireson = oauthtokenexpireson;
_x._owninguser_value = _owninguser_value;
_x.modifiedon = modifiedon;
_x.lastsyncerror = lastsyncerror;
_x.postponeofficeappsdeploymentuntil = postponeofficeappsdeploymentuntil;
_x.createdon = createdon;
_x.versionnumber = versionnumber;
_x.oauthrefreshtoken = oauthrefreshtoken;
_x._organizationid_value = _organizationid_value;
_x.lastduration = lastduration;
_x.testemailconfigurationscheduled = testemailconfigurationscheduled;
_x.officeappsdeploymenterror = officeappsdeploymenterror;
_x.enabledforact = enabledforact;
_x.outgoingemailstatus = outgoingemailstatus;
_x.lastsyncerrorcount = lastsyncerrorcount;
_x.emailaddress = emailaddress;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.lastsyncerroroccurredon = lastsyncerroroccurredon;
_x.processanddeleteemails = processanddeleteemails;
_x.entityimage = entityimage;
_x.allowemailconnectortousecredentials = allowemailconnectortousecredentials;
_x.itemsprocessedforlastsync = itemsprocessedforlastsync;
_x.entityimage_timestamp = entityimage_timestamp;
_x.officeappsdeploymentcompleteon = officeappsdeploymentcompleteon;
_x.processinglastattemptedon = processinglastattemptedon;
_x.username = username;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.officeappsdeploymentstatus = officeappsdeploymentstatus;
_x.postponesendinguntil = postponesendinguntil;
_x.postponetestemailconfigurationuntil = postponetestemailconfigurationuntil;
_x.actstatus = actstatus;
_x.statuscode = statuscode;
_x.lastsyncstartedon = lastsyncstartedon;
_x.receivingpostponeduntilforact = receivingpostponeduntilforact;
_x.processedtimes = processedtimes;
_x.exchangecontactsimportcompletedon = exchangecontactsimportcompletedon;
_x.ispasswordset = ispasswordset;
_x._owningteam_value = _owningteam_value;
_x._ownerid_value = _ownerid_value;
_x.lastsyncerrormachinename = lastsyncerrormachinename;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.ewsurl = ewsurl;
_x.processingstatecode = processingstatecode;
_x.transientfailurecount = transientfailurecount;
_x.lastsyncerrorcode = lastsyncerrorcode;
_x.password = password;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.isoauthrefreshtokenset = isoauthrefreshtokenset;
_x.postponemailboxprocessinguntil = postponemailboxprocessinguntil;
_x.testmailboxaccesscompletedon = testmailboxaccesscompletedon;
_x.processemailreceivedafter = processemailreceivedafter;
_x.emailrouteraccessapproval = emailrouteraccessapproval;
_x.mailboxstatus = mailboxstatus;
_x.noemailcount = noemailcount;
_x.incomingemailstatus = incomingemailstatus;
_x.enabledforoutgoingemail = enabledforoutgoingemail;
_x._createdby_value = _createdby_value;
_x.isoauthaccesstokenset = isoauthaccesstokenset;
_x.oauthaccesstoken = oauthaccesstoken;
_x.enabledforincomingemail = enabledforincomingemail;
_x.isexchangecontactsimportscheduled = isexchangecontactsimportscheduled;
_x.name = name;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.isserviceaccount = isserviceaccount;
_x.lastautodiscoveredon = lastautodiscoveredon;
_x.orgmarkedasprimaryforexchangesync = orgmarkedasprimaryforexchangesync;
_x.entityimage_url = entityimage_url;
_x.itemsfailedforlastsync = itemsfailedforlastsync;
_x.undeliverablefolder = undeliverablefolder;
_x.lastmailboxforcedunlockoccurredon = lastmailboxforcedunlockoccurredon;
_x.verboseloggingenabled = verboseloggingenabled;
_x.statecode = statecode;
_x.hostid = hostid;
_x.receivingpostponeduntil = receivingpostponeduntil;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Mailbox[");
b.append("testemailconfigurationretrycount=");
b.append(this.testemailconfigurationretrycount);
b.append(", ");
b.append("noactcount=");
b.append(this.noactcount);
b.append(", ");
b.append("isactsyncorgflagset=");
b.append(this.isactsyncorgflagset);
b.append(", ");
b.append("exchangesyncstatexml=");
b.append(this.exchangesyncstatexml);
b.append(", ");
b.append("isforwardmailbox=");
b.append(this.isforwardmailbox);
b.append(", ");
b.append("_emailserverprofile_value=");
b.append(this._emailserverprofile_value);
b.append(", ");
b.append("isemailaddressapprovedbyo365admin=");
b.append(this.isemailaddressapprovedbyo365admin);
b.append(", ");
b.append("actdeliverymethod=");
b.append(this.actdeliverymethod);
b.append(", ");
b.append("mailboxprocessingcontext=");
b.append(this.mailboxprocessingcontext);
b.append(", ");
b.append("folderhierarchy=");
b.append(this.folderhierarchy);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("outgoingemaildeliverymethod=");
b.append(this.outgoingemaildeliverymethod);
b.append(", ");
b.append("exchangecontactsimportstatus=");
b.append(this.exchangecontactsimportstatus);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("officeappsdeploymentscheduled=");
b.append(this.officeappsdeploymentscheduled);
b.append(", ");
b.append("mailboxid=");
b.append(this.mailboxid);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("incomingemaildeliverymethod=");
b.append(this.incomingemaildeliverymethod);
b.append(", ");
b.append("forcedunlockcount=");
b.append(this.forcedunlockcount);
b.append(", ");
b.append("lastsuccessfulsynccompletedon=");
b.append(this.lastsuccessfulsynccompletedon);
b.append(", ");
b.append("averagetotalduration=");
b.append(this.averagetotalduration);
b.append(", ");
b.append("oauthtokenexpireson=");
b.append(this.oauthtokenexpireson);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("lastsyncerror=");
b.append(this.lastsyncerror);
b.append(", ");
b.append("postponeofficeappsdeploymentuntil=");
b.append(this.postponeofficeappsdeploymentuntil);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("oauthrefreshtoken=");
b.append(this.oauthrefreshtoken);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("lastduration=");
b.append(this.lastduration);
b.append(", ");
b.append("testemailconfigurationscheduled=");
b.append(this.testemailconfigurationscheduled);
b.append(", ");
b.append("officeappsdeploymenterror=");
b.append(this.officeappsdeploymenterror);
b.append(", ");
b.append("enabledforact=");
b.append(this.enabledforact);
b.append(", ");
b.append("outgoingemailstatus=");
b.append(this.outgoingemailstatus);
b.append(", ");
b.append("lastsyncerrorcount=");
b.append(this.lastsyncerrorcount);
b.append(", ");
b.append("emailaddress=");
b.append(this.emailaddress);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("lastsyncerroroccurredon=");
b.append(this.lastsyncerroroccurredon);
b.append(", ");
b.append("processanddeleteemails=");
b.append(this.processanddeleteemails);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("allowemailconnectortousecredentials=");
b.append(this.allowemailconnectortousecredentials);
b.append(", ");
b.append("itemsprocessedforlastsync=");
b.append(this.itemsprocessedforlastsync);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("officeappsdeploymentcompleteon=");
b.append(this.officeappsdeploymentcompleteon);
b.append(", ");
b.append("processinglastattemptedon=");
b.append(this.processinglastattemptedon);
b.append(", ");
b.append("username=");
b.append(this.username);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("officeappsdeploymentstatus=");
b.append(this.officeappsdeploymentstatus);
b.append(", ");
b.append("postponesendinguntil=");
b.append(this.postponesendinguntil);
b.append(", ");
b.append("postponetestemailconfigurationuntil=");
b.append(this.postponetestemailconfigurationuntil);
b.append(", ");
b.append("actstatus=");
b.append(this.actstatus);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("lastsyncstartedon=");
b.append(this.lastsyncstartedon);
b.append(", ");
b.append("receivingpostponeduntilforact=");
b.append(this.receivingpostponeduntilforact);
b.append(", ");
b.append("processedtimes=");
b.append(this.processedtimes);
b.append(", ");
b.append("exchangecontactsimportcompletedon=");
b.append(this.exchangecontactsimportcompletedon);
b.append(", ");
b.append("ispasswordset=");
b.append(this.ispasswordset);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("lastsyncerrormachinename=");
b.append(this.lastsyncerrormachinename);
b.append(", ");
b.append("_regardingobjectid_value=");
b.append(this._regardingobjectid_value);
b.append(", ");
b.append("ewsurl=");
b.append(this.ewsurl);
b.append(", ");
b.append("processingstatecode=");
b.append(this.processingstatecode);
b.append(", ");
b.append("transientfailurecount=");
b.append(this.transientfailurecount);
b.append(", ");
b.append("lastsyncerrorcode=");
b.append(this.lastsyncerrorcode);
b.append(", ");
b.append("password=");
b.append(this.password);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("isoauthrefreshtokenset=");
b.append(this.isoauthrefreshtokenset);
b.append(", ");
b.append("postponemailboxprocessinguntil=");
b.append(this.postponemailboxprocessinguntil);
b.append(", ");
b.append("testmailboxaccesscompletedon=");
b.append(this.testmailboxaccesscompletedon);
b.append(", ");
b.append("processemailreceivedafter=");
b.append(this.processemailreceivedafter);
b.append(", ");
b.append("emailrouteraccessapproval=");
b.append(this.emailrouteraccessapproval);
b.append(", ");
b.append("mailboxstatus=");
b.append(this.mailboxstatus);
b.append(", ");
b.append("noemailcount=");
b.append(this.noemailcount);
b.append(", ");
b.append("incomingemailstatus=");
b.append(this.incomingemailstatus);
b.append(", ");
b.append("enabledforoutgoingemail=");
b.append(this.enabledforoutgoingemail);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("isoauthaccesstokenset=");
b.append(this.isoauthaccesstokenset);
b.append(", ");
b.append("oauthaccesstoken=");
b.append(this.oauthaccesstoken);
b.append(", ");
b.append("enabledforincomingemail=");
b.append(this.enabledforincomingemail);
b.append(", ");
b.append("isexchangecontactsimportscheduled=");
b.append(this.isexchangecontactsimportscheduled);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("isserviceaccount=");
b.append(this.isserviceaccount);
b.append(", ");
b.append("lastautodiscoveredon=");
b.append(this.lastautodiscoveredon);
b.append(", ");
b.append("orgmarkedasprimaryforexchangesync=");
b.append(this.orgmarkedasprimaryforexchangesync);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("itemsfailedforlastsync=");
b.append(this.itemsfailedforlastsync);
b.append(", ");
b.append("undeliverablefolder=");
b.append(this.undeliverablefolder);
b.append(", ");
b.append("lastmailboxforcedunlockoccurredon=");
b.append(this.lastmailboxforcedunlockoccurredon);
b.append(", ");
b.append("verboseloggingenabled=");
b.append(this.verboseloggingenabled);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("hostid=");
b.append(this.hostid);
b.append(", ");
b.append("receivingpostponeduntil=");
b.append(this.receivingpostponeduntil);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy