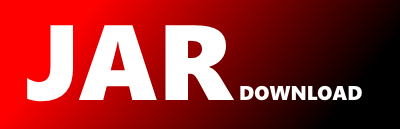
microsoft.dynamics.crm.entity.ManagedPropertyMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
import microsoft.dynamics.crm.complex.Label;
import microsoft.dynamics.crm.enums.ManagedPropertyEvaluationPriority;
import microsoft.dynamics.crm.enums.ManagedPropertyOperation;
import microsoft.dynamics.crm.enums.ManagedPropertyType;
@JsonPropertyOrder({
"@odata.type",
"LogicalName",
"DisplayName",
"ManagedPropertyType",
"Operation",
"IsGlobalForOperation",
"EvaluationPriority",
"IsPrivate",
"ErrorCode",
"EnablesEntityName",
"EnablesAttributeName",
"Description",
"IntroducedVersion"})
@JsonInclude(Include.NON_NULL)
public class ManagedPropertyMetadata extends MetadataBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.ManagedPropertyMetadata";
}
@JsonProperty("LogicalName")
protected String logicalName;
@JsonProperty("DisplayName")
protected Label displayName;
@JsonProperty("ManagedPropertyType")
protected ManagedPropertyType managedPropertyType;
@JsonProperty("Operation")
protected ManagedPropertyOperation operation;
@JsonProperty("IsGlobalForOperation")
protected Boolean isGlobalForOperation;
@JsonProperty("EvaluationPriority")
protected ManagedPropertyEvaluationPriority evaluationPriority;
@JsonProperty("IsPrivate")
protected Boolean isPrivate;
@JsonProperty("ErrorCode")
protected Integer errorCode;
@JsonProperty("EnablesEntityName")
protected String enablesEntityName;
@JsonProperty("EnablesAttributeName")
protected String enablesAttributeName;
@JsonProperty("Description")
protected Label description;
@JsonProperty("IntroducedVersion")
protected String introducedVersion;
protected ManagedPropertyMetadata() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderManagedPropertyMetadata() {
return new Builder();
}
public static final class Builder {
private String metadataId;
private Boolean hasChanged;
private String logicalName;
private Label displayName;
private ManagedPropertyType managedPropertyType;
private ManagedPropertyOperation operation;
private Boolean isGlobalForOperation;
private ManagedPropertyEvaluationPriority evaluationPriority;
private Boolean isPrivate;
private Integer errorCode;
private String enablesEntityName;
private String enablesAttributeName;
private Label description;
private String introducedVersion;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder metadataId(String metadataId) {
this.metadataId = metadataId;
this.changedFields = changedFields.add("MetadataId");
return this;
}
public Builder hasChanged(Boolean hasChanged) {
this.hasChanged = hasChanged;
this.changedFields = changedFields.add("HasChanged");
return this;
}
public Builder logicalName(String logicalName) {
this.logicalName = logicalName;
this.changedFields = changedFields.add("LogicalName");
return this;
}
public Builder displayName(Label displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder managedPropertyType(ManagedPropertyType managedPropertyType) {
this.managedPropertyType = managedPropertyType;
this.changedFields = changedFields.add("ManagedPropertyType");
return this;
}
public Builder operation(ManagedPropertyOperation operation) {
this.operation = operation;
this.changedFields = changedFields.add("Operation");
return this;
}
public Builder isGlobalForOperation(Boolean isGlobalForOperation) {
this.isGlobalForOperation = isGlobalForOperation;
this.changedFields = changedFields.add("IsGlobalForOperation");
return this;
}
public Builder evaluationPriority(ManagedPropertyEvaluationPriority evaluationPriority) {
this.evaluationPriority = evaluationPriority;
this.changedFields = changedFields.add("EvaluationPriority");
return this;
}
public Builder isPrivate(Boolean isPrivate) {
this.isPrivate = isPrivate;
this.changedFields = changedFields.add("IsPrivate");
return this;
}
public Builder errorCode(Integer errorCode) {
this.errorCode = errorCode;
this.changedFields = changedFields.add("ErrorCode");
return this;
}
public Builder enablesEntityName(String enablesEntityName) {
this.enablesEntityName = enablesEntityName;
this.changedFields = changedFields.add("EnablesEntityName");
return this;
}
public Builder enablesAttributeName(String enablesAttributeName) {
this.enablesAttributeName = enablesAttributeName;
this.changedFields = changedFields.add("EnablesAttributeName");
return this;
}
public Builder description(Label description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder introducedVersion(String introducedVersion) {
this.introducedVersion = introducedVersion;
this.changedFields = changedFields.add("IntroducedVersion");
return this;
}
public ManagedPropertyMetadata build() {
ManagedPropertyMetadata _x = new ManagedPropertyMetadata();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.ManagedPropertyMetadata";
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
_x.logicalName = logicalName;
_x.displayName = displayName;
_x.managedPropertyType = managedPropertyType;
_x.operation = operation;
_x.isGlobalForOperation = isGlobalForOperation;
_x.evaluationPriority = evaluationPriority;
_x.isPrivate = isPrivate;
_x.errorCode = errorCode;
_x.enablesEntityName = enablesEntityName;
_x.enablesAttributeName = enablesAttributeName;
_x.description = description;
_x.introducedVersion = introducedVersion;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && metadataId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(metadataId.toString()));
}
}
@Property(name="LogicalName")
@JsonIgnore
public Optional getLogicalName() {
return Optional.ofNullable(logicalName);
}
public ManagedPropertyMetadata withLogicalName(String logicalName) {
Checks.checkIsAscii(logicalName);
ManagedPropertyMetadata _x = _copy();
_x.changedFields = changedFields.add("LogicalName");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.ManagedPropertyMetadata");
_x.logicalName = logicalName;
return _x;
}
@Property(name="DisplayName")
@JsonIgnore
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy