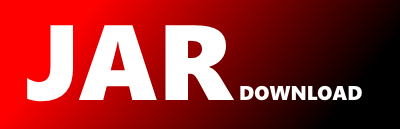
microsoft.dynamics.crm.entity.Msdyn_aiodlabel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiodtrainingboundingboxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
@JsonPropertyOrder({
"@odata.type",
"_owninguser_value",
"createdon",
"timezoneruleversionnumber",
"overriddencreatedon",
"msdyn_name",
"statecode",
"msdyn_sourcerecordid",
"msdyn_sourceentitysetname",
"_modifiedonbehalfby_value",
"msdyn_labelstring",
"_createdonbehalfby_value",
"versionnumber",
"_createdby_value",
"statuscode",
"_modifiedby_value",
"modifiedon",
"utcconversiontimezonecode",
"importsequencenumber",
"msdyn_aiodlabelid",
"_ownerid_value",
"_owningteam_value",
"_owningbusinessunit_value",
"msdyn_sourceattributelogicalname"})
@JsonInclude(Include.NON_NULL)
public class Msdyn_aiodlabel extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.msdyn_aiodlabel";
}
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("msdyn_name")
protected String msdyn_name;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("msdyn_sourcerecordid")
protected String msdyn_sourcerecordid;
@JsonProperty("msdyn_sourceentitysetname")
protected String msdyn_sourceentitysetname;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("msdyn_labelstring")
protected String msdyn_labelstring;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("msdyn_aiodlabelid")
protected String msdyn_aiodlabelid;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("msdyn_sourceattributelogicalname")
protected String msdyn_sourceattributelogicalname;
protected Msdyn_aiodlabel() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMsdyn_aiodlabel() {
return new Builder();
}
public static final class Builder {
private String _owninguser_value;
private OffsetDateTime createdon;
private Integer timezoneruleversionnumber;
private OffsetDateTime overriddencreatedon;
private String msdyn_name;
private Integer statecode;
private String msdyn_sourcerecordid;
private String msdyn_sourceentitysetname;
private String _modifiedonbehalfby_value;
private String msdyn_labelstring;
private String _createdonbehalfby_value;
private Long versionnumber;
private String _createdby_value;
private Integer statuscode;
private String _modifiedby_value;
private OffsetDateTime modifiedon;
private Integer utcconversiontimezonecode;
private Integer importsequencenumber;
private String msdyn_aiodlabelid;
private String _ownerid_value;
private String _owningteam_value;
private String _owningbusinessunit_value;
private String msdyn_sourceattributelogicalname;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder msdyn_name(String msdyn_name) {
this.msdyn_name = msdyn_name;
this.changedFields = changedFields.add("msdyn_name");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder msdyn_sourcerecordid(String msdyn_sourcerecordid) {
this.msdyn_sourcerecordid = msdyn_sourcerecordid;
this.changedFields = changedFields.add("msdyn_sourcerecordid");
return this;
}
public Builder msdyn_sourceentitysetname(String msdyn_sourceentitysetname) {
this.msdyn_sourceentitysetname = msdyn_sourceentitysetname;
this.changedFields = changedFields.add("msdyn_sourceentitysetname");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder msdyn_labelstring(String msdyn_labelstring) {
this.msdyn_labelstring = msdyn_labelstring;
this.changedFields = changedFields.add("msdyn_labelstring");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder msdyn_aiodlabelid(String msdyn_aiodlabelid) {
this.msdyn_aiodlabelid = msdyn_aiodlabelid;
this.changedFields = changedFields.add("msdyn_aiodlabelid");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder msdyn_sourceattributelogicalname(String msdyn_sourceattributelogicalname) {
this.msdyn_sourceattributelogicalname = msdyn_sourceattributelogicalname;
this.changedFields = changedFields.add("msdyn_sourceattributelogicalname");
return this;
}
public Msdyn_aiodlabel build() {
Msdyn_aiodlabel _x = new Msdyn_aiodlabel();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.msdyn_aiodlabel";
_x._owninguser_value = _owninguser_value;
_x.createdon = createdon;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.overriddencreatedon = overriddencreatedon;
_x.msdyn_name = msdyn_name;
_x.statecode = statecode;
_x.msdyn_sourcerecordid = msdyn_sourcerecordid;
_x.msdyn_sourceentitysetname = msdyn_sourceentitysetname;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.msdyn_labelstring = msdyn_labelstring;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x._createdby_value = _createdby_value;
_x.statuscode = statuscode;
_x._modifiedby_value = _modifiedby_value;
_x.modifiedon = modifiedon;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.importsequencenumber = importsequencenumber;
_x.msdyn_aiodlabelid = msdyn_aiodlabelid;
_x._ownerid_value = _ownerid_value;
_x._owningteam_value = _owningteam_value;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.msdyn_sourceattributelogicalname = msdyn_sourceattributelogicalname;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && msdyn_aiodlabelid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(msdyn_aiodlabelid.toString()));
}
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Msdyn_aiodlabel with_owninguser_value(String _owninguser_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Msdyn_aiodlabel withCreatedon(OffsetDateTime createdon) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.createdon = createdon;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Msdyn_aiodlabel withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Msdyn_aiodlabel withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="msdyn_name")
@JsonIgnore
public Optional getMsdyn_name() {
return Optional.ofNullable(msdyn_name);
}
public Msdyn_aiodlabel withMsdyn_name(String msdyn_name) {
Checks.checkIsAscii(msdyn_name);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_name = msdyn_name;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Msdyn_aiodlabel withStatecode(Integer statecode) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.statecode = statecode;
return _x;
}
@Property(name="msdyn_sourcerecordid")
@JsonIgnore
public Optional getMsdyn_sourcerecordid() {
return Optional.ofNullable(msdyn_sourcerecordid);
}
public Msdyn_aiodlabel withMsdyn_sourcerecordid(String msdyn_sourcerecordid) {
Checks.checkIsAscii(msdyn_sourcerecordid);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_sourcerecordid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_sourcerecordid = msdyn_sourcerecordid;
return _x;
}
@Property(name="msdyn_sourceentitysetname")
@JsonIgnore
public Optional getMsdyn_sourceentitysetname() {
return Optional.ofNullable(msdyn_sourceentitysetname);
}
public Msdyn_aiodlabel withMsdyn_sourceentitysetname(String msdyn_sourceentitysetname) {
Checks.checkIsAscii(msdyn_sourceentitysetname);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_sourceentitysetname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_sourceentitysetname = msdyn_sourceentitysetname;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Msdyn_aiodlabel with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="msdyn_labelstring")
@JsonIgnore
public Optional getMsdyn_labelstring() {
return Optional.ofNullable(msdyn_labelstring);
}
public Msdyn_aiodlabel withMsdyn_labelstring(String msdyn_labelstring) {
Checks.checkIsAscii(msdyn_labelstring);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_labelstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_labelstring = msdyn_labelstring;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Msdyn_aiodlabel with_createdonbehalfby_value(String _createdonbehalfby_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Msdyn_aiodlabel withVersionnumber(Long versionnumber) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Msdyn_aiodlabel with_createdby_value(String _createdby_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Msdyn_aiodlabel withStatuscode(Integer statuscode) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.statuscode = statuscode;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Msdyn_aiodlabel with_modifiedby_value(String _modifiedby_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Msdyn_aiodlabel withModifiedon(OffsetDateTime modifiedon) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Msdyn_aiodlabel withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Msdyn_aiodlabel withImportsequencenumber(Integer importsequencenumber) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="msdyn_aiodlabelid")
@JsonIgnore
public Optional getMsdyn_aiodlabelid() {
return Optional.ofNullable(msdyn_aiodlabelid);
}
public Msdyn_aiodlabel withMsdyn_aiodlabelid(String msdyn_aiodlabelid) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_aiodlabelid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_aiodlabelid = msdyn_aiodlabelid;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Msdyn_aiodlabel with_ownerid_value(String _ownerid_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Msdyn_aiodlabel with_owningteam_value(String _owningteam_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Msdyn_aiodlabel with_owningbusinessunit_value(String _owningbusinessunit_value) {
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="msdyn_sourceattributelogicalname")
@JsonIgnore
public Optional getMsdyn_sourceattributelogicalname() {
return Optional.ofNullable(msdyn_sourceattributelogicalname);
}
public Msdyn_aiodlabel withMsdyn_sourceattributelogicalname(String msdyn_sourceattributelogicalname) {
Checks.checkIsAscii(msdyn_sourceattributelogicalname);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = changedFields.add("msdyn_sourceattributelogicalname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.msdyn_aiodlabel");
_x.msdyn_sourceattributelogicalname = msdyn_sourceattributelogicalname;
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="msdyn_aiodlabel_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getMsdyn_aiodlabel_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_SyncErrors"));
}
@NavigationProperty(name="msdyn_aiodlabel_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getMsdyn_aiodlabel_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_DuplicateMatchingRecord"));
}
@NavigationProperty(name="msdyn_aiodlabel_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getMsdyn_aiodlabel_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_DuplicateBaseRecord"));
}
@NavigationProperty(name="msdyn_aiodlabel_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getMsdyn_aiodlabel_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_AsyncOperations"));
}
@NavigationProperty(name="msdyn_aiodlabel_MailboxTrackingFolders")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getMsdyn_aiodlabel_MailboxTrackingFolders() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_MailboxTrackingFolders"));
}
@NavigationProperty(name="msdyn_aiodlabel_ProcessSession")
@JsonIgnore
public ProcesssessionCollectionRequest getMsdyn_aiodlabel_ProcessSession() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_ProcessSession"));
}
@NavigationProperty(name="msdyn_aiodlabel_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getMsdyn_aiodlabel_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_BulkDeleteFailures"));
}
@NavigationProperty(name="msdyn_aiodlabel_PrincipalObjectAttributeAccesses")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getMsdyn_aiodlabel_PrincipalObjectAttributeAccesses() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_PrincipalObjectAttributeAccesses"));
}
@NavigationProperty(name="msdyn_aiodlabel_msdyn_aiconfiguration")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getMsdyn_aiodlabel_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_msdyn_aiconfiguration"));
}
@NavigationProperty(name="msdyn_aiodlabel_msdyn_aiodtrainingboundingbox")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getMsdyn_aiodlabel_msdyn_aiodtrainingboundingbox() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("msdyn_aiodlabel_msdyn_aiodtrainingboundingbox"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Msdyn_aiodlabel patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Msdyn_aiodlabel put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Msdyn_aiodlabel _x = _copy();
_x.changedFields = null;
return _x;
}
private Msdyn_aiodlabel _copy() {
Msdyn_aiodlabel _x = new Msdyn_aiodlabel();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x._owninguser_value = _owninguser_value;
_x.createdon = createdon;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.overriddencreatedon = overriddencreatedon;
_x.msdyn_name = msdyn_name;
_x.statecode = statecode;
_x.msdyn_sourcerecordid = msdyn_sourcerecordid;
_x.msdyn_sourceentitysetname = msdyn_sourceentitysetname;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.msdyn_labelstring = msdyn_labelstring;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x._createdby_value = _createdby_value;
_x.statuscode = statuscode;
_x._modifiedby_value = _modifiedby_value;
_x.modifiedon = modifiedon;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.importsequencenumber = importsequencenumber;
_x.msdyn_aiodlabelid = msdyn_aiodlabelid;
_x._ownerid_value = _ownerid_value;
_x._owningteam_value = _owningteam_value;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.msdyn_sourceattributelogicalname = msdyn_sourceattributelogicalname;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Msdyn_aiodlabel[");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("msdyn_name=");
b.append(this.msdyn_name);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("msdyn_sourcerecordid=");
b.append(this.msdyn_sourcerecordid);
b.append(", ");
b.append("msdyn_sourceentitysetname=");
b.append(this.msdyn_sourceentitysetname);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("msdyn_labelstring=");
b.append(this.msdyn_labelstring);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("msdyn_aiodlabelid=");
b.append(this.msdyn_aiodlabelid);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("msdyn_sourceattributelogicalname=");
b.append(this.msdyn_sourceattributelogicalname);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy