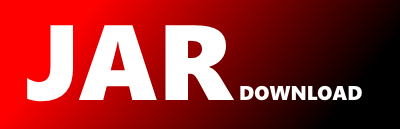
microsoft.dynamics.crm.entity.Plugintracelog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
@JsonPropertyOrder({
"@odata.type",
"secureconfiguration",
"organizationid",
"_createdby_value",
"performanceexecutionduration",
"plugintracelogid",
"createdon",
"primaryentity",
"performanceconstructorstarttime",
"persistencekey",
"_createdonbehalfby_value",
"pluginstepid",
"mode",
"operationtype",
"messagename",
"performanceconstructorduration",
"performanceexecutionstarttime",
"configuration",
"profile",
"depth",
"typename",
"issystemcreated",
"correlationid",
"requestid",
"exceptiondetails",
"messageblock"})
@JsonInclude(Include.NON_NULL)
public class Plugintracelog extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.plugintracelog";
}
@JsonProperty("secureconfiguration")
protected String secureconfiguration;
@JsonProperty("organizationid")
protected String organizationid;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("performanceexecutionduration")
protected Integer performanceexecutionduration;
@JsonProperty("plugintracelogid")
protected String plugintracelogid;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("primaryentity")
protected String primaryentity;
@JsonProperty("performanceconstructorstarttime")
protected OffsetDateTime performanceconstructorstarttime;
@JsonProperty("persistencekey")
protected String persistencekey;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("pluginstepid")
protected String pluginstepid;
@JsonProperty("mode")
protected Integer mode;
@JsonProperty("operationtype")
protected Integer operationtype;
@JsonProperty("messagename")
protected String messagename;
@JsonProperty("performanceconstructorduration")
protected Integer performanceconstructorduration;
@JsonProperty("performanceexecutionstarttime")
protected OffsetDateTime performanceexecutionstarttime;
@JsonProperty("configuration")
protected String configuration;
@JsonProperty("profile")
protected String profile;
@JsonProperty("depth")
protected Integer depth;
@JsonProperty("typename")
protected String typename;
@JsonProperty("issystemcreated")
protected Boolean issystemcreated;
@JsonProperty("correlationid")
protected String correlationid;
@JsonProperty("requestid")
protected String requestid;
@JsonProperty("exceptiondetails")
protected String exceptiondetails;
@JsonProperty("messageblock")
protected String messageblock;
protected Plugintracelog() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderPlugintracelog() {
return new Builder();
}
public static final class Builder {
private String secureconfiguration;
private String organizationid;
private String _createdby_value;
private Integer performanceexecutionduration;
private String plugintracelogid;
private OffsetDateTime createdon;
private String primaryentity;
private OffsetDateTime performanceconstructorstarttime;
private String persistencekey;
private String _createdonbehalfby_value;
private String pluginstepid;
private Integer mode;
private Integer operationtype;
private String messagename;
private Integer performanceconstructorduration;
private OffsetDateTime performanceexecutionstarttime;
private String configuration;
private String profile;
private Integer depth;
private String typename;
private Boolean issystemcreated;
private String correlationid;
private String requestid;
private String exceptiondetails;
private String messageblock;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder secureconfiguration(String secureconfiguration) {
this.secureconfiguration = secureconfiguration;
this.changedFields = changedFields.add("secureconfiguration");
return this;
}
public Builder organizationid(String organizationid) {
this.organizationid = organizationid;
this.changedFields = changedFields.add("organizationid");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder performanceexecutionduration(Integer performanceexecutionduration) {
this.performanceexecutionduration = performanceexecutionduration;
this.changedFields = changedFields.add("performanceexecutionduration");
return this;
}
public Builder plugintracelogid(String plugintracelogid) {
this.plugintracelogid = plugintracelogid;
this.changedFields = changedFields.add("plugintracelogid");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder primaryentity(String primaryentity) {
this.primaryentity = primaryentity;
this.changedFields = changedFields.add("primaryentity");
return this;
}
public Builder performanceconstructorstarttime(OffsetDateTime performanceconstructorstarttime) {
this.performanceconstructorstarttime = performanceconstructorstarttime;
this.changedFields = changedFields.add("performanceconstructorstarttime");
return this;
}
public Builder persistencekey(String persistencekey) {
this.persistencekey = persistencekey;
this.changedFields = changedFields.add("persistencekey");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder pluginstepid(String pluginstepid) {
this.pluginstepid = pluginstepid;
this.changedFields = changedFields.add("pluginstepid");
return this;
}
public Builder mode(Integer mode) {
this.mode = mode;
this.changedFields = changedFields.add("mode");
return this;
}
public Builder operationtype(Integer operationtype) {
this.operationtype = operationtype;
this.changedFields = changedFields.add("operationtype");
return this;
}
public Builder messagename(String messagename) {
this.messagename = messagename;
this.changedFields = changedFields.add("messagename");
return this;
}
public Builder performanceconstructorduration(Integer performanceconstructorduration) {
this.performanceconstructorduration = performanceconstructorduration;
this.changedFields = changedFields.add("performanceconstructorduration");
return this;
}
public Builder performanceexecutionstarttime(OffsetDateTime performanceexecutionstarttime) {
this.performanceexecutionstarttime = performanceexecutionstarttime;
this.changedFields = changedFields.add("performanceexecutionstarttime");
return this;
}
public Builder configuration(String configuration) {
this.configuration = configuration;
this.changedFields = changedFields.add("configuration");
return this;
}
public Builder profile(String profile) {
this.profile = profile;
this.changedFields = changedFields.add("profile");
return this;
}
public Builder depth(Integer depth) {
this.depth = depth;
this.changedFields = changedFields.add("depth");
return this;
}
public Builder typename(String typename) {
this.typename = typename;
this.changedFields = changedFields.add("typename");
return this;
}
public Builder issystemcreated(Boolean issystemcreated) {
this.issystemcreated = issystemcreated;
this.changedFields = changedFields.add("issystemcreated");
return this;
}
public Builder correlationid(String correlationid) {
this.correlationid = correlationid;
this.changedFields = changedFields.add("correlationid");
return this;
}
public Builder requestid(String requestid) {
this.requestid = requestid;
this.changedFields = changedFields.add("requestid");
return this;
}
public Builder exceptiondetails(String exceptiondetails) {
this.exceptiondetails = exceptiondetails;
this.changedFields = changedFields.add("exceptiondetails");
return this;
}
public Builder messageblock(String messageblock) {
this.messageblock = messageblock;
this.changedFields = changedFields.add("messageblock");
return this;
}
public Plugintracelog build() {
Plugintracelog _x = new Plugintracelog();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.plugintracelog";
_x.secureconfiguration = secureconfiguration;
_x.organizationid = organizationid;
_x._createdby_value = _createdby_value;
_x.performanceexecutionduration = performanceexecutionduration;
_x.plugintracelogid = plugintracelogid;
_x.createdon = createdon;
_x.primaryentity = primaryentity;
_x.performanceconstructorstarttime = performanceconstructorstarttime;
_x.persistencekey = persistencekey;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.pluginstepid = pluginstepid;
_x.mode = mode;
_x.operationtype = operationtype;
_x.messagename = messagename;
_x.performanceconstructorduration = performanceconstructorduration;
_x.performanceexecutionstarttime = performanceexecutionstarttime;
_x.configuration = configuration;
_x.profile = profile;
_x.depth = depth;
_x.typename = typename;
_x.issystemcreated = issystemcreated;
_x.correlationid = correlationid;
_x.requestid = requestid;
_x.exceptiondetails = exceptiondetails;
_x.messageblock = messageblock;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && plugintracelogid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(plugintracelogid.toString()));
}
}
@Property(name="secureconfiguration")
@JsonIgnore
public Optional getSecureconfiguration() {
return Optional.ofNullable(secureconfiguration);
}
public Plugintracelog withSecureconfiguration(String secureconfiguration) {
Checks.checkIsAscii(secureconfiguration);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("secureconfiguration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.secureconfiguration = secureconfiguration;
return _x;
}
@Property(name="organizationid")
@JsonIgnore
public Optional getOrganizationid() {
return Optional.ofNullable(organizationid);
}
public Plugintracelog withOrganizationid(String organizationid) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("organizationid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.organizationid = organizationid;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Plugintracelog with_createdby_value(String _createdby_value) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="performanceexecutionduration")
@JsonIgnore
public Optional getPerformanceexecutionduration() {
return Optional.ofNullable(performanceexecutionduration);
}
public Plugintracelog withPerformanceexecutionduration(Integer performanceexecutionduration) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("performanceexecutionduration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.performanceexecutionduration = performanceexecutionduration;
return _x;
}
@Property(name="plugintracelogid")
@JsonIgnore
public Optional getPlugintracelogid() {
return Optional.ofNullable(plugintracelogid);
}
public Plugintracelog withPlugintracelogid(String plugintracelogid) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("plugintracelogid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.plugintracelogid = plugintracelogid;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Plugintracelog withCreatedon(OffsetDateTime createdon) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.createdon = createdon;
return _x;
}
@Property(name="primaryentity")
@JsonIgnore
public Optional getPrimaryentity() {
return Optional.ofNullable(primaryentity);
}
public Plugintracelog withPrimaryentity(String primaryentity) {
Checks.checkIsAscii(primaryentity);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("primaryentity");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.primaryentity = primaryentity;
return _x;
}
@Property(name="performanceconstructorstarttime")
@JsonIgnore
public Optional getPerformanceconstructorstarttime() {
return Optional.ofNullable(performanceconstructorstarttime);
}
public Plugintracelog withPerformanceconstructorstarttime(OffsetDateTime performanceconstructorstarttime) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("performanceconstructorstarttime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.performanceconstructorstarttime = performanceconstructorstarttime;
return _x;
}
@Property(name="persistencekey")
@JsonIgnore
public Optional getPersistencekey() {
return Optional.ofNullable(persistencekey);
}
public Plugintracelog withPersistencekey(String persistencekey) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("persistencekey");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.persistencekey = persistencekey;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Plugintracelog with_createdonbehalfby_value(String _createdonbehalfby_value) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="pluginstepid")
@JsonIgnore
public Optional getPluginstepid() {
return Optional.ofNullable(pluginstepid);
}
public Plugintracelog withPluginstepid(String pluginstepid) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("pluginstepid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.pluginstepid = pluginstepid;
return _x;
}
@Property(name="mode")
@JsonIgnore
public Optional getMode() {
return Optional.ofNullable(mode);
}
public Plugintracelog withMode(Integer mode) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("mode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.mode = mode;
return _x;
}
@Property(name="operationtype")
@JsonIgnore
public Optional getOperationtype() {
return Optional.ofNullable(operationtype);
}
public Plugintracelog withOperationtype(Integer operationtype) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("operationtype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.operationtype = operationtype;
return _x;
}
@Property(name="messagename")
@JsonIgnore
public Optional getMessagename() {
return Optional.ofNullable(messagename);
}
public Plugintracelog withMessagename(String messagename) {
Checks.checkIsAscii(messagename);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("messagename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.messagename = messagename;
return _x;
}
@Property(name="performanceconstructorduration")
@JsonIgnore
public Optional getPerformanceconstructorduration() {
return Optional.ofNullable(performanceconstructorduration);
}
public Plugintracelog withPerformanceconstructorduration(Integer performanceconstructorduration) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("performanceconstructorduration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.performanceconstructorduration = performanceconstructorduration;
return _x;
}
@Property(name="performanceexecutionstarttime")
@JsonIgnore
public Optional getPerformanceexecutionstarttime() {
return Optional.ofNullable(performanceexecutionstarttime);
}
public Plugintracelog withPerformanceexecutionstarttime(OffsetDateTime performanceexecutionstarttime) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("performanceexecutionstarttime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.performanceexecutionstarttime = performanceexecutionstarttime;
return _x;
}
@Property(name="configuration")
@JsonIgnore
public Optional getConfiguration() {
return Optional.ofNullable(configuration);
}
public Plugintracelog withConfiguration(String configuration) {
Checks.checkIsAscii(configuration);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("configuration");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.configuration = configuration;
return _x;
}
@Property(name="profile")
@JsonIgnore
public Optional getProfile() {
return Optional.ofNullable(profile);
}
public Plugintracelog withProfile(String profile) {
Checks.checkIsAscii(profile);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("profile");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.profile = profile;
return _x;
}
@Property(name="depth")
@JsonIgnore
public Optional getDepth() {
return Optional.ofNullable(depth);
}
public Plugintracelog withDepth(Integer depth) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("depth");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.depth = depth;
return _x;
}
@Property(name="typename")
@JsonIgnore
public Optional getTypename() {
return Optional.ofNullable(typename);
}
public Plugintracelog withTypename(String typename) {
Checks.checkIsAscii(typename);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("typename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.typename = typename;
return _x;
}
@Property(name="issystemcreated")
@JsonIgnore
public Optional getIssystemcreated() {
return Optional.ofNullable(issystemcreated);
}
public Plugintracelog withIssystemcreated(Boolean issystemcreated) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("issystemcreated");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.issystemcreated = issystemcreated;
return _x;
}
@Property(name="correlationid")
@JsonIgnore
public Optional getCorrelationid() {
return Optional.ofNullable(correlationid);
}
public Plugintracelog withCorrelationid(String correlationid) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("correlationid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.correlationid = correlationid;
return _x;
}
@Property(name="requestid")
@JsonIgnore
public Optional getRequestid() {
return Optional.ofNullable(requestid);
}
public Plugintracelog withRequestid(String requestid) {
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("requestid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.requestid = requestid;
return _x;
}
@Property(name="exceptiondetails")
@JsonIgnore
public Optional getExceptiondetails() {
return Optional.ofNullable(exceptiondetails);
}
public Plugintracelog withExceptiondetails(String exceptiondetails) {
Checks.checkIsAscii(exceptiondetails);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("exceptiondetails");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.exceptiondetails = exceptiondetails;
return _x;
}
@Property(name="messageblock")
@JsonIgnore
public Optional getMessageblock() {
return Optional.ofNullable(messageblock);
}
public Plugintracelog withMessageblock(String messageblock) {
Checks.checkIsAscii(messageblock);
Plugintracelog _x = _copy();
_x.changedFields = changedFields.add("messageblock");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.plugintracelog");
_x.messageblock = messageblock;
return _x;
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Plugintracelog patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Plugintracelog _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Plugintracelog put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Plugintracelog _x = _copy();
_x.changedFields = null;
return _x;
}
private Plugintracelog _copy() {
Plugintracelog _x = new Plugintracelog();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.secureconfiguration = secureconfiguration;
_x.organizationid = organizationid;
_x._createdby_value = _createdby_value;
_x.performanceexecutionduration = performanceexecutionduration;
_x.plugintracelogid = plugintracelogid;
_x.createdon = createdon;
_x.primaryentity = primaryentity;
_x.performanceconstructorstarttime = performanceconstructorstarttime;
_x.persistencekey = persistencekey;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.pluginstepid = pluginstepid;
_x.mode = mode;
_x.operationtype = operationtype;
_x.messagename = messagename;
_x.performanceconstructorduration = performanceconstructorduration;
_x.performanceexecutionstarttime = performanceexecutionstarttime;
_x.configuration = configuration;
_x.profile = profile;
_x.depth = depth;
_x.typename = typename;
_x.issystemcreated = issystemcreated;
_x.correlationid = correlationid;
_x.requestid = requestid;
_x.exceptiondetails = exceptiondetails;
_x.messageblock = messageblock;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Plugintracelog[");
b.append("secureconfiguration=");
b.append(this.secureconfiguration);
b.append(", ");
b.append("organizationid=");
b.append(this.organizationid);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("performanceexecutionduration=");
b.append(this.performanceexecutionduration);
b.append(", ");
b.append("plugintracelogid=");
b.append(this.plugintracelogid);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("primaryentity=");
b.append(this.primaryentity);
b.append(", ");
b.append("performanceconstructorstarttime=");
b.append(this.performanceconstructorstarttime);
b.append(", ");
b.append("persistencekey=");
b.append(this.persistencekey);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("pluginstepid=");
b.append(this.pluginstepid);
b.append(", ");
b.append("mode=");
b.append(this.mode);
b.append(", ");
b.append("operationtype=");
b.append(this.operationtype);
b.append(", ");
b.append("messagename=");
b.append(this.messagename);
b.append(", ");
b.append("performanceconstructorduration=");
b.append(this.performanceconstructorduration);
b.append(", ");
b.append("performanceexecutionstarttime=");
b.append(this.performanceexecutionstarttime);
b.append(", ");
b.append("configuration=");
b.append(this.configuration);
b.append(", ");
b.append("profile=");
b.append(this.profile);
b.append(", ");
b.append("depth=");
b.append(this.depth);
b.append(", ");
b.append("typename=");
b.append(this.typename);
b.append(", ");
b.append("issystemcreated=");
b.append(this.issystemcreated);
b.append(", ");
b.append("correlationid=");
b.append(this.correlationid);
b.append(", ");
b.append("requestid=");
b.append(this.requestid);
b.append(", ");
b.append("exceptiondetails=");
b.append(this.exceptiondetails);
b.append(", ");
b.append("messageblock=");
b.append(this.messageblock);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy