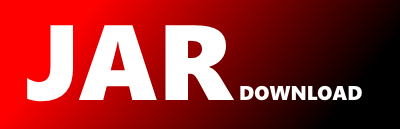
microsoft.dynamics.crm.entity.Postfollow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.AppointmentRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.KnowledgearticleRequest;
import microsoft.dynamics.crm.entity.request.PhonecallRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcesssessionRequest;
import microsoft.dynamics.crm.entity.request.QueueRequest;
import microsoft.dynamics.crm.entity.request.RecurringappointmentmasterRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TaskRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
@JsonPropertyOrder({
"@odata.type",
"_owningteam_value",
"_ownerid_value",
"_createdby_value",
"_owninguser_value",
"_regardingobjectid_value",
"utcconversiontimezonecode",
"timezoneruleversionnumber",
"_owningbusinessunit_value",
"createdon",
"yammerpoststate",
"_createdonbehalfby_value",
"versionnumber",
"postfollowid"})
@JsonInclude(Include.NON_NULL)
public class Postfollow extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.postfollow";
}
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("_regardingobjectid_value")
protected String _regardingobjectid_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("yammerpoststate")
protected Integer yammerpoststate;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("postfollowid")
protected String postfollowid;
protected Postfollow() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderPostfollow() {
return new Builder();
}
public static final class Builder {
private String _owningteam_value;
private String _ownerid_value;
private String _createdby_value;
private String _owninguser_value;
private String _regardingobjectid_value;
private Integer utcconversiontimezonecode;
private Integer timezoneruleversionnumber;
private String _owningbusinessunit_value;
private OffsetDateTime createdon;
private Integer yammerpoststate;
private String _createdonbehalfby_value;
private Long versionnumber;
private String postfollowid;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder _regardingobjectid_value(String _regardingobjectid_value) {
this._regardingobjectid_value = _regardingobjectid_value;
this.changedFields = changedFields.add("_regardingobjectid_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder yammerpoststate(Integer yammerpoststate) {
this.yammerpoststate = yammerpoststate;
this.changedFields = changedFields.add("yammerpoststate");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder postfollowid(String postfollowid) {
this.postfollowid = postfollowid;
this.changedFields = changedFields.add("postfollowid");
return this;
}
public Postfollow build() {
Postfollow _x = new Postfollow();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.postfollow";
_x._owningteam_value = _owningteam_value;
_x._ownerid_value = _ownerid_value;
_x._createdby_value = _createdby_value;
_x._owninguser_value = _owninguser_value;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.createdon = createdon;
_x.yammerpoststate = yammerpoststate;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x.postfollowid = postfollowid;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && postfollowid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(postfollowid.toString()));
}
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Postfollow with_owningteam_value(String _owningteam_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Postfollow with_ownerid_value(String _ownerid_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Postfollow with_createdby_value(String _createdby_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Postfollow with_owninguser_value(String _owninguser_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="_regardingobjectid_value")
@JsonIgnore
public Optional get_regardingobjectid_value() {
return Optional.ofNullable(_regardingobjectid_value);
}
public Postfollow with_regardingobjectid_value(String _regardingobjectid_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_regardingobjectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._regardingobjectid_value = _regardingobjectid_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Postfollow withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Postfollow withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Postfollow with_owningbusinessunit_value(String _owningbusinessunit_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Postfollow withCreatedon(OffsetDateTime createdon) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.createdon = createdon;
return _x;
}
@Property(name="yammerpoststate")
@JsonIgnore
public Optional getYammerpoststate() {
return Optional.ofNullable(yammerpoststate);
}
public Postfollow withYammerpoststate(Integer yammerpoststate) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("yammerpoststate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.yammerpoststate = yammerpoststate;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Postfollow with_createdonbehalfby_value(String _createdonbehalfby_value) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Postfollow withVersionnumber(Long versionnumber) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="postfollowid")
@JsonIgnore
public Optional getPostfollowid() {
return Optional.ofNullable(postfollowid);
}
public Postfollow withPostfollowid(String postfollowid) {
Postfollow _x = _copy();
_x.changedFields = changedFields.add("postfollowid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.postfollow");
_x.postfollowid = postfollowid;
return _x;
}
@NavigationProperty(name="regardingobjectid_task")
@JsonIgnore
public TaskRequest getRegardingobjectid_task() {
return new TaskRequest(contextPath.addSegment("regardingobjectid_task"));
}
@NavigationProperty(name="regardingobjectid_appointment")
@JsonIgnore
public AppointmentRequest getRegardingobjectid_appointment() {
return new AppointmentRequest(contextPath.addSegment("regardingobjectid_appointment"));
}
@NavigationProperty(name="regardingobjectid_phonecall")
@JsonIgnore
public PhonecallRequest getRegardingobjectid_phonecall() {
return new PhonecallRequest(contextPath.addSegment("regardingobjectid_phonecall"));
}
@NavigationProperty(name="regardingobjectid_recurringappointmentmaster")
@JsonIgnore
public RecurringappointmentmasterRequest getRegardingobjectid_recurringappointmentmaster() {
return new RecurringappointmentmasterRequest(contextPath.addSegment("regardingobjectid_recurringappointmentmaster"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="regardingobjectid_account")
@JsonIgnore
public AccountRequest getRegardingobjectid_account() {
return new AccountRequest(contextPath.addSegment("regardingobjectid_account"));
}
@NavigationProperty(name="regardingobjectid_contact")
@JsonIgnore
public ContactRequest getRegardingobjectid_contact() {
return new ContactRequest(contextPath.addSegment("regardingobjectid_contact"));
}
@NavigationProperty(name="regardingobjectid_systemuser")
@JsonIgnore
public SystemuserRequest getRegardingobjectid_systemuser() {
return new SystemuserRequest(contextPath.addSegment("regardingobjectid_systemuser"));
}
@NavigationProperty(name="PostFollow_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getPostFollow_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("PostFollow_AsyncOperations"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="regardingobjectid_queue")
@JsonIgnore
public QueueRequest getRegardingobjectid_queue() {
return new QueueRequest(contextPath.addSegment("regardingobjectid_queue"));
}
@NavigationProperty(name="PostFollow_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getPostFollow_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("PostFollow_SyncErrors"));
}
@NavigationProperty(name="regardingobjectid_processsession")
@JsonIgnore
public ProcesssessionRequest getRegardingobjectid_processsession() {
return new ProcesssessionRequest(contextPath.addSegment("regardingobjectid_processsession"));
}
@NavigationProperty(name="regardingobjectid_knowledgearticle")
@JsonIgnore
public KnowledgearticleRequest getRegardingobjectid_knowledgearticle() {
return new KnowledgearticleRequest(contextPath.addSegment("regardingobjectid_knowledgearticle"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Postfollow patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Postfollow _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Postfollow put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Postfollow _x = _copy();
_x.changedFields = null;
return _x;
}
private Postfollow _copy() {
Postfollow _x = new Postfollow();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x._owningteam_value = _owningteam_value;
_x._ownerid_value = _ownerid_value;
_x._createdby_value = _createdby_value;
_x._owninguser_value = _owninguser_value;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.createdon = createdon;
_x.yammerpoststate = yammerpoststate;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x.postfollowid = postfollowid;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Postfollow[");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("_regardingobjectid_value=");
b.append(this._regardingobjectid_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("yammerpoststate=");
b.append(this.yammerpoststate);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("postfollowid=");
b.append(this.postfollowid);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy