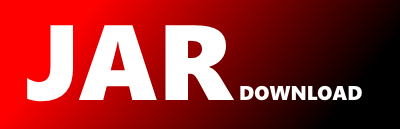
microsoft.dynamics.crm.entity.Publisheraddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.request.PublisherRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
@JsonPropertyOrder({
"@odata.type",
"postofficebox",
"line3",
"publisheraddressid",
"telephone1",
"importsequencenumber",
"latitude",
"line1",
"longitude",
"primarycontactname",
"versionnumber",
"shippingmethodcode",
"utcconversiontimezonecode",
"country",
"addresstypecode",
"_createdonbehalfby_value",
"utcoffset",
"telephone3",
"name",
"county",
"line2",
"postalcode",
"_parentid_value",
"stateorprovince",
"_modifiedonbehalfby_value",
"freighttermscode",
"timezoneruleversionnumber",
"fax",
"_modifiedby_value",
"city",
"createdon",
"telephone2",
"modifiedon",
"_createdby_value",
"upszone",
"addressnumber"})
@JsonInclude(Include.NON_NULL)
public class Publisheraddress extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.publisheraddress";
}
@JsonProperty("postofficebox")
protected String postofficebox;
@JsonProperty("line3")
protected String line3;
@JsonProperty("publisheraddressid")
protected String publisheraddressid;
@JsonProperty("telephone1")
protected String telephone1;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("latitude")
protected Double latitude;
@JsonProperty("line1")
protected String line1;
@JsonProperty("longitude")
protected Double longitude;
@JsonProperty("primarycontactname")
protected String primarycontactname;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("shippingmethodcode")
protected Integer shippingmethodcode;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("country")
protected String country;
@JsonProperty("addresstypecode")
protected Integer addresstypecode;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("utcoffset")
protected Integer utcoffset;
@JsonProperty("telephone3")
protected String telephone3;
@JsonProperty("name")
protected String name;
@JsonProperty("county")
protected String county;
@JsonProperty("line2")
protected String line2;
@JsonProperty("postalcode")
protected String postalcode;
@JsonProperty("_parentid_value")
protected String _parentid_value;
@JsonProperty("stateorprovince")
protected String stateorprovince;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("freighttermscode")
protected Integer freighttermscode;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("fax")
protected String fax;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("city")
protected String city;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("telephone2")
protected String telephone2;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("upszone")
protected String upszone;
@JsonProperty("addressnumber")
protected Integer addressnumber;
protected Publisheraddress() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderPublisheraddress() {
return new Builder();
}
public static final class Builder {
private String postofficebox;
private String line3;
private String publisheraddressid;
private String telephone1;
private Integer importsequencenumber;
private Double latitude;
private String line1;
private Double longitude;
private String primarycontactname;
private Long versionnumber;
private Integer shippingmethodcode;
private Integer utcconversiontimezonecode;
private String country;
private Integer addresstypecode;
private String _createdonbehalfby_value;
private Integer utcoffset;
private String telephone3;
private String name;
private String county;
private String line2;
private String postalcode;
private String _parentid_value;
private String stateorprovince;
private String _modifiedonbehalfby_value;
private Integer freighttermscode;
private Integer timezoneruleversionnumber;
private String fax;
private String _modifiedby_value;
private String city;
private OffsetDateTime createdon;
private String telephone2;
private OffsetDateTime modifiedon;
private String _createdby_value;
private String upszone;
private Integer addressnumber;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder postofficebox(String postofficebox) {
this.postofficebox = postofficebox;
this.changedFields = changedFields.add("postofficebox");
return this;
}
public Builder line3(String line3) {
this.line3 = line3;
this.changedFields = changedFields.add("line3");
return this;
}
public Builder publisheraddressid(String publisheraddressid) {
this.publisheraddressid = publisheraddressid;
this.changedFields = changedFields.add("publisheraddressid");
return this;
}
public Builder telephone1(String telephone1) {
this.telephone1 = telephone1;
this.changedFields = changedFields.add("telephone1");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder latitude(Double latitude) {
this.latitude = latitude;
this.changedFields = changedFields.add("latitude");
return this;
}
public Builder line1(String line1) {
this.line1 = line1;
this.changedFields = changedFields.add("line1");
return this;
}
public Builder longitude(Double longitude) {
this.longitude = longitude;
this.changedFields = changedFields.add("longitude");
return this;
}
public Builder primarycontactname(String primarycontactname) {
this.primarycontactname = primarycontactname;
this.changedFields = changedFields.add("primarycontactname");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder shippingmethodcode(Integer shippingmethodcode) {
this.shippingmethodcode = shippingmethodcode;
this.changedFields = changedFields.add("shippingmethodcode");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder country(String country) {
this.country = country;
this.changedFields = changedFields.add("country");
return this;
}
public Builder addresstypecode(Integer addresstypecode) {
this.addresstypecode = addresstypecode;
this.changedFields = changedFields.add("addresstypecode");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder utcoffset(Integer utcoffset) {
this.utcoffset = utcoffset;
this.changedFields = changedFields.add("utcoffset");
return this;
}
public Builder telephone3(String telephone3) {
this.telephone3 = telephone3;
this.changedFields = changedFields.add("telephone3");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder county(String county) {
this.county = county;
this.changedFields = changedFields.add("county");
return this;
}
public Builder line2(String line2) {
this.line2 = line2;
this.changedFields = changedFields.add("line2");
return this;
}
public Builder postalcode(String postalcode) {
this.postalcode = postalcode;
this.changedFields = changedFields.add("postalcode");
return this;
}
public Builder _parentid_value(String _parentid_value) {
this._parentid_value = _parentid_value;
this.changedFields = changedFields.add("_parentid_value");
return this;
}
public Builder stateorprovince(String stateorprovince) {
this.stateorprovince = stateorprovince;
this.changedFields = changedFields.add("stateorprovince");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder freighttermscode(Integer freighttermscode) {
this.freighttermscode = freighttermscode;
this.changedFields = changedFields.add("freighttermscode");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder fax(String fax) {
this.fax = fax;
this.changedFields = changedFields.add("fax");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder city(String city) {
this.city = city;
this.changedFields = changedFields.add("city");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder telephone2(String telephone2) {
this.telephone2 = telephone2;
this.changedFields = changedFields.add("telephone2");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder upszone(String upszone) {
this.upszone = upszone;
this.changedFields = changedFields.add("upszone");
return this;
}
public Builder addressnumber(Integer addressnumber) {
this.addressnumber = addressnumber;
this.changedFields = changedFields.add("addressnumber");
return this;
}
public Publisheraddress build() {
Publisheraddress _x = new Publisheraddress();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.publisheraddress";
_x.postofficebox = postofficebox;
_x.line3 = line3;
_x.publisheraddressid = publisheraddressid;
_x.telephone1 = telephone1;
_x.importsequencenumber = importsequencenumber;
_x.latitude = latitude;
_x.line1 = line1;
_x.longitude = longitude;
_x.primarycontactname = primarycontactname;
_x.versionnumber = versionnumber;
_x.shippingmethodcode = shippingmethodcode;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.country = country;
_x.addresstypecode = addresstypecode;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.utcoffset = utcoffset;
_x.telephone3 = telephone3;
_x.name = name;
_x.county = county;
_x.line2 = line2;
_x.postalcode = postalcode;
_x._parentid_value = _parentid_value;
_x.stateorprovince = stateorprovince;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.freighttermscode = freighttermscode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.fax = fax;
_x._modifiedby_value = _modifiedby_value;
_x.city = city;
_x.createdon = createdon;
_x.telephone2 = telephone2;
_x.modifiedon = modifiedon;
_x._createdby_value = _createdby_value;
_x.upszone = upszone;
_x.addressnumber = addressnumber;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && publisheraddressid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(publisheraddressid.toString()));
}
}
@Property(name="postofficebox")
@JsonIgnore
public Optional getPostofficebox() {
return Optional.ofNullable(postofficebox);
}
public Publisheraddress withPostofficebox(String postofficebox) {
Checks.checkIsAscii(postofficebox);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.postofficebox = postofficebox;
return _x;
}
@Property(name="line3")
@JsonIgnore
public Optional getLine3() {
return Optional.ofNullable(line3);
}
public Publisheraddress withLine3(String line3) {
Checks.checkIsAscii(line3);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.line3 = line3;
return _x;
}
@Property(name="publisheraddressid")
@JsonIgnore
public Optional getPublisheraddressid() {
return Optional.ofNullable(publisheraddressid);
}
public Publisheraddress withPublisheraddressid(String publisheraddressid) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("publisheraddressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.publisheraddressid = publisheraddressid;
return _x;
}
@Property(name="telephone1")
@JsonIgnore
public Optional getTelephone1() {
return Optional.ofNullable(telephone1);
}
public Publisheraddress withTelephone1(String telephone1) {
Checks.checkIsAscii(telephone1);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.telephone1 = telephone1;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Publisheraddress withImportsequencenumber(Integer importsequencenumber) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="latitude")
@JsonIgnore
public Optional getLatitude() {
return Optional.ofNullable(latitude);
}
public Publisheraddress withLatitude(Double latitude) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.latitude = latitude;
return _x;
}
@Property(name="line1")
@JsonIgnore
public Optional getLine1() {
return Optional.ofNullable(line1);
}
public Publisheraddress withLine1(String line1) {
Checks.checkIsAscii(line1);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.line1 = line1;
return _x;
}
@Property(name="longitude")
@JsonIgnore
public Optional getLongitude() {
return Optional.ofNullable(longitude);
}
public Publisheraddress withLongitude(Double longitude) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.longitude = longitude;
return _x;
}
@Property(name="primarycontactname")
@JsonIgnore
public Optional getPrimarycontactname() {
return Optional.ofNullable(primarycontactname);
}
public Publisheraddress withPrimarycontactname(String primarycontactname) {
Checks.checkIsAscii(primarycontactname);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.primarycontactname = primarycontactname;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Publisheraddress withVersionnumber(Long versionnumber) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="shippingmethodcode")
@JsonIgnore
public Optional getShippingmethodcode() {
return Optional.ofNullable(shippingmethodcode);
}
public Publisheraddress withShippingmethodcode(Integer shippingmethodcode) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.shippingmethodcode = shippingmethodcode;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Publisheraddress withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="country")
@JsonIgnore
public Optional getCountry() {
return Optional.ofNullable(country);
}
public Publisheraddress withCountry(String country) {
Checks.checkIsAscii(country);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.country = country;
return _x;
}
@Property(name="addresstypecode")
@JsonIgnore
public Optional getAddresstypecode() {
return Optional.ofNullable(addresstypecode);
}
public Publisheraddress withAddresstypecode(Integer addresstypecode) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.addresstypecode = addresstypecode;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Publisheraddress with_createdonbehalfby_value(String _createdonbehalfby_value) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="utcoffset")
@JsonIgnore
public Optional getUtcoffset() {
return Optional.ofNullable(utcoffset);
}
public Publisheraddress withUtcoffset(Integer utcoffset) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.utcoffset = utcoffset;
return _x;
}
@Property(name="telephone3")
@JsonIgnore
public Optional getTelephone3() {
return Optional.ofNullable(telephone3);
}
public Publisheraddress withTelephone3(String telephone3) {
Checks.checkIsAscii(telephone3);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.telephone3 = telephone3;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Publisheraddress withName(String name) {
Checks.checkIsAscii(name);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.name = name;
return _x;
}
@Property(name="county")
@JsonIgnore
public Optional getCounty() {
return Optional.ofNullable(county);
}
public Publisheraddress withCounty(String county) {
Checks.checkIsAscii(county);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.county = county;
return _x;
}
@Property(name="line2")
@JsonIgnore
public Optional getLine2() {
return Optional.ofNullable(line2);
}
public Publisheraddress withLine2(String line2) {
Checks.checkIsAscii(line2);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.line2 = line2;
return _x;
}
@Property(name="postalcode")
@JsonIgnore
public Optional getPostalcode() {
return Optional.ofNullable(postalcode);
}
public Publisheraddress withPostalcode(String postalcode) {
Checks.checkIsAscii(postalcode);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.postalcode = postalcode;
return _x;
}
@Property(name="_parentid_value")
@JsonIgnore
public Optional get_parentid_value() {
return Optional.ofNullable(_parentid_value);
}
public Publisheraddress with_parentid_value(String _parentid_value) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("_parentid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x._parentid_value = _parentid_value;
return _x;
}
@Property(name="stateorprovince")
@JsonIgnore
public Optional getStateorprovince() {
return Optional.ofNullable(stateorprovince);
}
public Publisheraddress withStateorprovince(String stateorprovince) {
Checks.checkIsAscii(stateorprovince);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.stateorprovince = stateorprovince;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Publisheraddress with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="freighttermscode")
@JsonIgnore
public Optional getFreighttermscode() {
return Optional.ofNullable(freighttermscode);
}
public Publisheraddress withFreighttermscode(Integer freighttermscode) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.freighttermscode = freighttermscode;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Publisheraddress withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="fax")
@JsonIgnore
public Optional getFax() {
return Optional.ofNullable(fax);
}
public Publisheraddress withFax(String fax) {
Checks.checkIsAscii(fax);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.fax = fax;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Publisheraddress with_modifiedby_value(String _modifiedby_value) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="city")
@JsonIgnore
public Optional getCity() {
return Optional.ofNullable(city);
}
public Publisheraddress withCity(String city) {
Checks.checkIsAscii(city);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.city = city;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Publisheraddress withCreatedon(OffsetDateTime createdon) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.createdon = createdon;
return _x;
}
@Property(name="telephone2")
@JsonIgnore
public Optional getTelephone2() {
return Optional.ofNullable(telephone2);
}
public Publisheraddress withTelephone2(String telephone2) {
Checks.checkIsAscii(telephone2);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.telephone2 = telephone2;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Publisheraddress withModifiedon(OffsetDateTime modifiedon) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Publisheraddress with_createdby_value(String _createdby_value) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="upszone")
@JsonIgnore
public Optional getUpszone() {
return Optional.ofNullable(upszone);
}
public Publisheraddress withUpszone(String upszone) {
Checks.checkIsAscii(upszone);
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.upszone = upszone;
return _x;
}
@Property(name="addressnumber")
@JsonIgnore
public Optional getAddressnumber() {
return Optional.ofNullable(addressnumber);
}
public Publisheraddress withAddressnumber(Integer addressnumber) {
Publisheraddress _x = _copy();
_x.changedFields = changedFields.add("addressnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.publisheraddress");
_x.addressnumber = addressnumber;
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="parentid")
@JsonIgnore
public PublisherRequest getParentid() {
return new PublisherRequest(contextPath.addSegment("parentid"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Publisheraddress patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Publisheraddress _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Publisheraddress put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Publisheraddress _x = _copy();
_x.changedFields = null;
return _x;
}
private Publisheraddress _copy() {
Publisheraddress _x = new Publisheraddress();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.postofficebox = postofficebox;
_x.line3 = line3;
_x.publisheraddressid = publisheraddressid;
_x.telephone1 = telephone1;
_x.importsequencenumber = importsequencenumber;
_x.latitude = latitude;
_x.line1 = line1;
_x.longitude = longitude;
_x.primarycontactname = primarycontactname;
_x.versionnumber = versionnumber;
_x.shippingmethodcode = shippingmethodcode;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.country = country;
_x.addresstypecode = addresstypecode;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.utcoffset = utcoffset;
_x.telephone3 = telephone3;
_x.name = name;
_x.county = county;
_x.line2 = line2;
_x.postalcode = postalcode;
_x._parentid_value = _parentid_value;
_x.stateorprovince = stateorprovince;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.freighttermscode = freighttermscode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.fax = fax;
_x._modifiedby_value = _modifiedby_value;
_x.city = city;
_x.createdon = createdon;
_x.telephone2 = telephone2;
_x.modifiedon = modifiedon;
_x._createdby_value = _createdby_value;
_x.upszone = upszone;
_x.addressnumber = addressnumber;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Publisheraddress[");
b.append("postofficebox=");
b.append(this.postofficebox);
b.append(", ");
b.append("line3=");
b.append(this.line3);
b.append(", ");
b.append("publisheraddressid=");
b.append(this.publisheraddressid);
b.append(", ");
b.append("telephone1=");
b.append(this.telephone1);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("latitude=");
b.append(this.latitude);
b.append(", ");
b.append("line1=");
b.append(this.line1);
b.append(", ");
b.append("longitude=");
b.append(this.longitude);
b.append(", ");
b.append("primarycontactname=");
b.append(this.primarycontactname);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("shippingmethodcode=");
b.append(this.shippingmethodcode);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("country=");
b.append(this.country);
b.append(", ");
b.append("addresstypecode=");
b.append(this.addresstypecode);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("utcoffset=");
b.append(this.utcoffset);
b.append(", ");
b.append("telephone3=");
b.append(this.telephone3);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("county=");
b.append(this.county);
b.append(", ");
b.append("line2=");
b.append(this.line2);
b.append(", ");
b.append("postalcode=");
b.append(this.postalcode);
b.append(", ");
b.append("_parentid_value=");
b.append(this._parentid_value);
b.append(", ");
b.append("stateorprovince=");
b.append(this.stateorprovince);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("freighttermscode=");
b.append(this.freighttermscode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("fax=");
b.append(this.fax);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("city=");
b.append(this.city);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("telephone2=");
b.append(this.telephone2);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("upszone=");
b.append(this.upszone);
b.append(", ");
b.append("addressnumber=");
b.append(this.addressnumber);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy