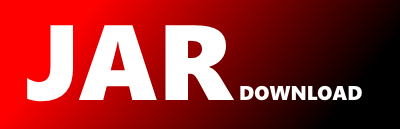
microsoft.dynamics.crm.entity.Report Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.FunctionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.Optional;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.complex.DownloadReportDefinitionResponse;
import microsoft.dynamics.crm.complex.GetReportHistoryLimitResponse;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ReportCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ReportcategoryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ReportRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
@JsonPropertyOrder({
"@odata.type",
"isscheduledreport",
"solutionid",
"description",
"_ownerid_value",
"overwritetime",
"reportnameonsrs",
"signaturelcid",
"queryinfo",
"createdon",
"modifiedon",
"bodytext",
"_createdonbehalfby_value",
"signaturedate",
"iscustomizable",
"customreportxml",
"iscustomreport",
"schedulexml",
"reportid",
"_owningteam_value",
"mimetype",
"signatureminorversion",
"filename",
"languagecode",
"_createdby_value",
"signatureid",
"bodyurl",
"defaultfilter",
"_owninguser_value",
"ispersonal",
"_parentreportid_value",
"componentstate",
"timezoneruleversionnumber",
"rdlhash",
"_modifiedonbehalfby_value",
"originalbodytext",
"_modifiedby_value",
"introducedversion",
"reportidunique",
"versionnumber",
"filesize",
"bodybinary",
"bodybinary_binary",
"reporttypecode",
"_owningbusinessunit_value",
"ismanaged",
"signaturemajorversion",
"utcconversiontimezonecode",
"createdinmajorversion",
"name"})
@JsonInclude(Include.NON_NULL)
public class Report extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.report";
}
@JsonProperty("isscheduledreport")
protected Boolean isscheduledreport;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("description")
protected String description;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("reportnameonsrs")
protected String reportnameonsrs;
@JsonProperty("signaturelcid")
protected Integer signaturelcid;
@JsonProperty("queryinfo")
protected String queryinfo;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("bodytext")
protected String bodytext;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("signaturedate")
protected OffsetDateTime signaturedate;
@JsonProperty("iscustomizable")
protected BooleanManagedProperty iscustomizable;
@JsonProperty("customreportxml")
protected String customreportxml;
@JsonProperty("iscustomreport")
protected Boolean iscustomreport;
@JsonProperty("schedulexml")
protected String schedulexml;
@JsonProperty("reportid")
protected String reportid;
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("mimetype")
protected String mimetype;
@JsonProperty("signatureminorversion")
protected Integer signatureminorversion;
@JsonProperty("filename")
protected String filename;
@JsonProperty("languagecode")
protected Integer languagecode;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("signatureid")
protected String signatureid;
@JsonProperty("bodyurl")
protected String bodyurl;
@JsonProperty("defaultfilter")
protected String defaultfilter;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("ispersonal")
protected Boolean ispersonal;
@JsonProperty("_parentreportid_value")
protected String _parentreportid_value;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("rdlhash")
protected Integer rdlhash;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("originalbodytext")
protected String originalbodytext;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("introducedversion")
protected String introducedversion;
@JsonProperty("reportidunique")
protected String reportidunique;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("filesize")
protected Integer filesize;
@JsonProperty("bodybinary")
protected String bodybinary;
@JsonProperty("bodybinary_binary")
protected byte[] bodybinary_binary;
@JsonProperty("reporttypecode")
protected Integer reporttypecode;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("signaturemajorversion")
protected Integer signaturemajorversion;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("createdinmajorversion")
protected Integer createdinmajorversion;
@JsonProperty("name")
protected String name;
protected Report() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderReport() {
return new Builder();
}
public static final class Builder {
private Boolean isscheduledreport;
private String solutionid;
private String description;
private String _ownerid_value;
private OffsetDateTime overwritetime;
private String reportnameonsrs;
private Integer signaturelcid;
private String queryinfo;
private OffsetDateTime createdon;
private OffsetDateTime modifiedon;
private String bodytext;
private String _createdonbehalfby_value;
private OffsetDateTime signaturedate;
private BooleanManagedProperty iscustomizable;
private String customreportxml;
private Boolean iscustomreport;
private String schedulexml;
private String reportid;
private String _owningteam_value;
private String mimetype;
private Integer signatureminorversion;
private String filename;
private Integer languagecode;
private String _createdby_value;
private String signatureid;
private String bodyurl;
private String defaultfilter;
private String _owninguser_value;
private Boolean ispersonal;
private String _parentreportid_value;
private Integer componentstate;
private Integer timezoneruleversionnumber;
private Integer rdlhash;
private String _modifiedonbehalfby_value;
private String originalbodytext;
private String _modifiedby_value;
private String introducedversion;
private String reportidunique;
private Long versionnumber;
private Integer filesize;
private String bodybinary;
private byte[] bodybinary_binary;
private Integer reporttypecode;
private String _owningbusinessunit_value;
private Boolean ismanaged;
private Integer signaturemajorversion;
private Integer utcconversiontimezonecode;
private Integer createdinmajorversion;
private String name;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder isscheduledreport(Boolean isscheduledreport) {
this.isscheduledreport = isscheduledreport;
this.changedFields = changedFields.add("isscheduledreport");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder reportnameonsrs(String reportnameonsrs) {
this.reportnameonsrs = reportnameonsrs;
this.changedFields = changedFields.add("reportnameonsrs");
return this;
}
public Builder signaturelcid(Integer signaturelcid) {
this.signaturelcid = signaturelcid;
this.changedFields = changedFields.add("signaturelcid");
return this;
}
public Builder queryinfo(String queryinfo) {
this.queryinfo = queryinfo;
this.changedFields = changedFields.add("queryinfo");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder bodytext(String bodytext) {
this.bodytext = bodytext;
this.changedFields = changedFields.add("bodytext");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder signaturedate(OffsetDateTime signaturedate) {
this.signaturedate = signaturedate;
this.changedFields = changedFields.add("signaturedate");
return this;
}
public Builder iscustomizable(BooleanManagedProperty iscustomizable) {
this.iscustomizable = iscustomizable;
this.changedFields = changedFields.add("iscustomizable");
return this;
}
public Builder customreportxml(String customreportxml) {
this.customreportxml = customreportxml;
this.changedFields = changedFields.add("customreportxml");
return this;
}
public Builder iscustomreport(Boolean iscustomreport) {
this.iscustomreport = iscustomreport;
this.changedFields = changedFields.add("iscustomreport");
return this;
}
public Builder schedulexml(String schedulexml) {
this.schedulexml = schedulexml;
this.changedFields = changedFields.add("schedulexml");
return this;
}
public Builder reportid(String reportid) {
this.reportid = reportid;
this.changedFields = changedFields.add("reportid");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder mimetype(String mimetype) {
this.mimetype = mimetype;
this.changedFields = changedFields.add("mimetype");
return this;
}
public Builder signatureminorversion(Integer signatureminorversion) {
this.signatureminorversion = signatureminorversion;
this.changedFields = changedFields.add("signatureminorversion");
return this;
}
public Builder filename(String filename) {
this.filename = filename;
this.changedFields = changedFields.add("filename");
return this;
}
public Builder languagecode(Integer languagecode) {
this.languagecode = languagecode;
this.changedFields = changedFields.add("languagecode");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder signatureid(String signatureid) {
this.signatureid = signatureid;
this.changedFields = changedFields.add("signatureid");
return this;
}
public Builder bodyurl(String bodyurl) {
this.bodyurl = bodyurl;
this.changedFields = changedFields.add("bodyurl");
return this;
}
public Builder defaultfilter(String defaultfilter) {
this.defaultfilter = defaultfilter;
this.changedFields = changedFields.add("defaultfilter");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder ispersonal(Boolean ispersonal) {
this.ispersonal = ispersonal;
this.changedFields = changedFields.add("ispersonal");
return this;
}
public Builder _parentreportid_value(String _parentreportid_value) {
this._parentreportid_value = _parentreportid_value;
this.changedFields = changedFields.add("_parentreportid_value");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder rdlhash(Integer rdlhash) {
this.rdlhash = rdlhash;
this.changedFields = changedFields.add("rdlhash");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder originalbodytext(String originalbodytext) {
this.originalbodytext = originalbodytext;
this.changedFields = changedFields.add("originalbodytext");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder introducedversion(String introducedversion) {
this.introducedversion = introducedversion;
this.changedFields = changedFields.add("introducedversion");
return this;
}
public Builder reportidunique(String reportidunique) {
this.reportidunique = reportidunique;
this.changedFields = changedFields.add("reportidunique");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder filesize(Integer filesize) {
this.filesize = filesize;
this.changedFields = changedFields.add("filesize");
return this;
}
public Builder bodybinary(String bodybinary) {
this.bodybinary = bodybinary;
this.changedFields = changedFields.add("bodybinary");
return this;
}
public Builder bodybinary_binary(byte[] bodybinary_binary) {
this.bodybinary_binary = bodybinary_binary;
this.changedFields = changedFields.add("bodybinary_binary");
return this;
}
public Builder reporttypecode(Integer reporttypecode) {
this.reporttypecode = reporttypecode;
this.changedFields = changedFields.add("reporttypecode");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder signaturemajorversion(Integer signaturemajorversion) {
this.signaturemajorversion = signaturemajorversion;
this.changedFields = changedFields.add("signaturemajorversion");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder createdinmajorversion(Integer createdinmajorversion) {
this.createdinmajorversion = createdinmajorversion;
this.changedFields = changedFields.add("createdinmajorversion");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Report build() {
Report _x = new Report();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.report";
_x.isscheduledreport = isscheduledreport;
_x.solutionid = solutionid;
_x.description = description;
_x._ownerid_value = _ownerid_value;
_x.overwritetime = overwritetime;
_x.reportnameonsrs = reportnameonsrs;
_x.signaturelcid = signaturelcid;
_x.queryinfo = queryinfo;
_x.createdon = createdon;
_x.modifiedon = modifiedon;
_x.bodytext = bodytext;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.signaturedate = signaturedate;
_x.iscustomizable = iscustomizable;
_x.customreportxml = customreportxml;
_x.iscustomreport = iscustomreport;
_x.schedulexml = schedulexml;
_x.reportid = reportid;
_x._owningteam_value = _owningteam_value;
_x.mimetype = mimetype;
_x.signatureminorversion = signatureminorversion;
_x.filename = filename;
_x.languagecode = languagecode;
_x._createdby_value = _createdby_value;
_x.signatureid = signatureid;
_x.bodyurl = bodyurl;
_x.defaultfilter = defaultfilter;
_x._owninguser_value = _owninguser_value;
_x.ispersonal = ispersonal;
_x._parentreportid_value = _parentreportid_value;
_x.componentstate = componentstate;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.rdlhash = rdlhash;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.originalbodytext = originalbodytext;
_x._modifiedby_value = _modifiedby_value;
_x.introducedversion = introducedversion;
_x.reportidunique = reportidunique;
_x.versionnumber = versionnumber;
_x.filesize = filesize;
_x.bodybinary = bodybinary;
_x.bodybinary_binary = bodybinary_binary;
_x.reporttypecode = reporttypecode;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.ismanaged = ismanaged;
_x.signaturemajorversion = signaturemajorversion;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.createdinmajorversion = createdinmajorversion;
_x.name = name;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && reportid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(reportid.toString()));
}
}
@Property(name="isscheduledreport")
@JsonIgnore
public Optional getIsscheduledreport() {
return Optional.ofNullable(isscheduledreport);
}
public Report withIsscheduledreport(Boolean isscheduledreport) {
Report _x = _copy();
_x.changedFields = changedFields.add("isscheduledreport");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.isscheduledreport = isscheduledreport;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Report withSolutionid(String solutionid) {
Report _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.solutionid = solutionid;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Report withDescription(String description) {
Checks.checkIsAscii(description);
Report _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.description = description;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Report with_ownerid_value(String _ownerid_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Report withOverwritetime(OffsetDateTime overwritetime) {
Report _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="reportnameonsrs")
@JsonIgnore
public Optional getReportnameonsrs() {
return Optional.ofNullable(reportnameonsrs);
}
public Report withReportnameonsrs(String reportnameonsrs) {
Checks.checkIsAscii(reportnameonsrs);
Report _x = _copy();
_x.changedFields = changedFields.add("reportnameonsrs");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.reportnameonsrs = reportnameonsrs;
return _x;
}
@Property(name="signaturelcid")
@JsonIgnore
public Optional getSignaturelcid() {
return Optional.ofNullable(signaturelcid);
}
public Report withSignaturelcid(Integer signaturelcid) {
Report _x = _copy();
_x.changedFields = changedFields.add("signaturelcid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.signaturelcid = signaturelcid;
return _x;
}
@Property(name="queryinfo")
@JsonIgnore
public Optional getQueryinfo() {
return Optional.ofNullable(queryinfo);
}
public Report withQueryinfo(String queryinfo) {
Checks.checkIsAscii(queryinfo);
Report _x = _copy();
_x.changedFields = changedFields.add("queryinfo");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.queryinfo = queryinfo;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Report withCreatedon(OffsetDateTime createdon) {
Report _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.createdon = createdon;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Report withModifiedon(OffsetDateTime modifiedon) {
Report _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="bodytext")
@JsonIgnore
public Optional getBodytext() {
return Optional.ofNullable(bodytext);
}
public Report withBodytext(String bodytext) {
Checks.checkIsAscii(bodytext);
Report _x = _copy();
_x.changedFields = changedFields.add("bodytext");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.bodytext = bodytext;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Report with_createdonbehalfby_value(String _createdonbehalfby_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="signaturedate")
@JsonIgnore
public Optional getSignaturedate() {
return Optional.ofNullable(signaturedate);
}
public Report withSignaturedate(OffsetDateTime signaturedate) {
Report _x = _copy();
_x.changedFields = changedFields.add("signaturedate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.signaturedate = signaturedate;
return _x;
}
@Property(name="iscustomizable")
@JsonIgnore
public Optional getIscustomizable() {
return Optional.ofNullable(iscustomizable);
}
public Report withIscustomizable(BooleanManagedProperty iscustomizable) {
Report _x = _copy();
_x.changedFields = changedFields.add("iscustomizable");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.iscustomizable = iscustomizable;
return _x;
}
@Property(name="customreportxml")
@JsonIgnore
public Optional getCustomreportxml() {
return Optional.ofNullable(customreportxml);
}
public Report withCustomreportxml(String customreportxml) {
Checks.checkIsAscii(customreportxml);
Report _x = _copy();
_x.changedFields = changedFields.add("customreportxml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.customreportxml = customreportxml;
return _x;
}
@Property(name="iscustomreport")
@JsonIgnore
public Optional getIscustomreport() {
return Optional.ofNullable(iscustomreport);
}
public Report withIscustomreport(Boolean iscustomreport) {
Report _x = _copy();
_x.changedFields = changedFields.add("iscustomreport");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.iscustomreport = iscustomreport;
return _x;
}
@Property(name="schedulexml")
@JsonIgnore
public Optional getSchedulexml() {
return Optional.ofNullable(schedulexml);
}
public Report withSchedulexml(String schedulexml) {
Checks.checkIsAscii(schedulexml);
Report _x = _copy();
_x.changedFields = changedFields.add("schedulexml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.schedulexml = schedulexml;
return _x;
}
@Property(name="reportid")
@JsonIgnore
public Optional getReportid() {
return Optional.ofNullable(reportid);
}
public Report withReportid(String reportid) {
Report _x = _copy();
_x.changedFields = changedFields.add("reportid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.reportid = reportid;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Report with_owningteam_value(String _owningteam_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="mimetype")
@JsonIgnore
public Optional getMimetype() {
return Optional.ofNullable(mimetype);
}
public Report withMimetype(String mimetype) {
Checks.checkIsAscii(mimetype);
Report _x = _copy();
_x.changedFields = changedFields.add("mimetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.mimetype = mimetype;
return _x;
}
@Property(name="signatureminorversion")
@JsonIgnore
public Optional getSignatureminorversion() {
return Optional.ofNullable(signatureminorversion);
}
public Report withSignatureminorversion(Integer signatureminorversion) {
Report _x = _copy();
_x.changedFields = changedFields.add("signatureminorversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.signatureminorversion = signatureminorversion;
return _x;
}
@Property(name="filename")
@JsonIgnore
public Optional getFilename() {
return Optional.ofNullable(filename);
}
public Report withFilename(String filename) {
Checks.checkIsAscii(filename);
Report _x = _copy();
_x.changedFields = changedFields.add("filename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.filename = filename;
return _x;
}
@Property(name="languagecode")
@JsonIgnore
public Optional getLanguagecode() {
return Optional.ofNullable(languagecode);
}
public Report withLanguagecode(Integer languagecode) {
Report _x = _copy();
_x.changedFields = changedFields.add("languagecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.languagecode = languagecode;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Report with_createdby_value(String _createdby_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="signatureid")
@JsonIgnore
public Optional getSignatureid() {
return Optional.ofNullable(signatureid);
}
public Report withSignatureid(String signatureid) {
Report _x = _copy();
_x.changedFields = changedFields.add("signatureid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.signatureid = signatureid;
return _x;
}
@Property(name="bodyurl")
@JsonIgnore
public Optional getBodyurl() {
return Optional.ofNullable(bodyurl);
}
public Report withBodyurl(String bodyurl) {
Checks.checkIsAscii(bodyurl);
Report _x = _copy();
_x.changedFields = changedFields.add("bodyurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.bodyurl = bodyurl;
return _x;
}
@Property(name="defaultfilter")
@JsonIgnore
public Optional getDefaultfilter() {
return Optional.ofNullable(defaultfilter);
}
public Report withDefaultfilter(String defaultfilter) {
Checks.checkIsAscii(defaultfilter);
Report _x = _copy();
_x.changedFields = changedFields.add("defaultfilter");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.defaultfilter = defaultfilter;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Report with_owninguser_value(String _owninguser_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="ispersonal")
@JsonIgnore
public Optional getIspersonal() {
return Optional.ofNullable(ispersonal);
}
public Report withIspersonal(Boolean ispersonal) {
Report _x = _copy();
_x.changedFields = changedFields.add("ispersonal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.ispersonal = ispersonal;
return _x;
}
@Property(name="_parentreportid_value")
@JsonIgnore
public Optional get_parentreportid_value() {
return Optional.ofNullable(_parentreportid_value);
}
public Report with_parentreportid_value(String _parentreportid_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_parentreportid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._parentreportid_value = _parentreportid_value;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Report withComponentstate(Integer componentstate) {
Report _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.componentstate = componentstate;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Report withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Report _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="rdlhash")
@JsonIgnore
public Optional getRdlhash() {
return Optional.ofNullable(rdlhash);
}
public Report withRdlhash(Integer rdlhash) {
Report _x = _copy();
_x.changedFields = changedFields.add("rdlhash");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.rdlhash = rdlhash;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Report with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="originalbodytext")
@JsonIgnore
public Optional getOriginalbodytext() {
return Optional.ofNullable(originalbodytext);
}
public Report withOriginalbodytext(String originalbodytext) {
Checks.checkIsAscii(originalbodytext);
Report _x = _copy();
_x.changedFields = changedFields.add("originalbodytext");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.originalbodytext = originalbodytext;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Report with_modifiedby_value(String _modifiedby_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="introducedversion")
@JsonIgnore
public Optional getIntroducedversion() {
return Optional.ofNullable(introducedversion);
}
public Report withIntroducedversion(String introducedversion) {
Checks.checkIsAscii(introducedversion);
Report _x = _copy();
_x.changedFields = changedFields.add("introducedversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.introducedversion = introducedversion;
return _x;
}
@Property(name="reportidunique")
@JsonIgnore
public Optional getReportidunique() {
return Optional.ofNullable(reportidunique);
}
public Report withReportidunique(String reportidunique) {
Report _x = _copy();
_x.changedFields = changedFields.add("reportidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.reportidunique = reportidunique;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Report withVersionnumber(Long versionnumber) {
Report _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="filesize")
@JsonIgnore
public Optional getFilesize() {
return Optional.ofNullable(filesize);
}
public Report withFilesize(Integer filesize) {
Report _x = _copy();
_x.changedFields = changedFields.add("filesize");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.filesize = filesize;
return _x;
}
@Property(name="bodybinary")
@JsonIgnore
public Optional getBodybinary() {
return Optional.ofNullable(bodybinary);
}
public Report withBodybinary(String bodybinary) {
Checks.checkIsAscii(bodybinary);
Report _x = _copy();
_x.changedFields = changedFields.add("bodybinary");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.bodybinary = bodybinary;
return _x;
}
@Property(name="bodybinary_binary")
@JsonIgnore
public Optional getBodybinary_binary() {
return Optional.ofNullable(bodybinary_binary);
}
public Report withBodybinary_binary(byte[] bodybinary_binary) {
Report _x = _copy();
_x.changedFields = changedFields.add("bodybinary_binary");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.bodybinary_binary = bodybinary_binary;
return _x;
}
@Property(name="reporttypecode")
@JsonIgnore
public Optional getReporttypecode() {
return Optional.ofNullable(reporttypecode);
}
public Report withReporttypecode(Integer reporttypecode) {
Report _x = _copy();
_x.changedFields = changedFields.add("reporttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.reporttypecode = reporttypecode;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Report with_owningbusinessunit_value(String _owningbusinessunit_value) {
Report _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Report withIsmanaged(Boolean ismanaged) {
Report _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="signaturemajorversion")
@JsonIgnore
public Optional getSignaturemajorversion() {
return Optional.ofNullable(signaturemajorversion);
}
public Report withSignaturemajorversion(Integer signaturemajorversion) {
Report _x = _copy();
_x.changedFields = changedFields.add("signaturemajorversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.signaturemajorversion = signaturemajorversion;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Report withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Report _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="createdinmajorversion")
@JsonIgnore
public Optional getCreatedinmajorversion() {
return Optional.ofNullable(createdinmajorversion);
}
public Report withCreatedinmajorversion(Integer createdinmajorversion) {
Report _x = _copy();
_x.changedFields = changedFields.add("createdinmajorversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.createdinmajorversion = createdinmajorversion;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Report withName(String name) {
Checks.checkIsAscii(name);
Report _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.report");
_x.name = name;
return _x;
}
@NavigationProperty(name="Report_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getReport_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Report_AsyncOperations"));
}
@NavigationProperty(name="report_reportcategories")
@JsonIgnore
public ReportcategoryCollectionRequest getReport_reportcategories() {
return new ReportcategoryCollectionRequest(
contextPath.addSegment("report_reportcategories"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="Report_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getReport_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Report_ProcessSessions"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="Report_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getReport_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Report_SyncErrors"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="parentreportid")
@JsonIgnore
public ReportRequest getParentreportid() {
return new ReportRequest(contextPath.addSegment("parentreportid"));
}
@NavigationProperty(name="report_parent_report")
@JsonIgnore
public ReportCollectionRequest getReport_parent_report() {
return new ReportCollectionRequest(
contextPath.addSegment("report_parent_report"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Report patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Report _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Report put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Report _x = _copy();
_x.changedFields = null;
return _x;
}
private Report _copy() {
Report _x = new Report();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.isscheduledreport = isscheduledreport;
_x.solutionid = solutionid;
_x.description = description;
_x._ownerid_value = _ownerid_value;
_x.overwritetime = overwritetime;
_x.reportnameonsrs = reportnameonsrs;
_x.signaturelcid = signaturelcid;
_x.queryinfo = queryinfo;
_x.createdon = createdon;
_x.modifiedon = modifiedon;
_x.bodytext = bodytext;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.signaturedate = signaturedate;
_x.iscustomizable = iscustomizable;
_x.customreportxml = customreportxml;
_x.iscustomreport = iscustomreport;
_x.schedulexml = schedulexml;
_x.reportid = reportid;
_x._owningteam_value = _owningteam_value;
_x.mimetype = mimetype;
_x.signatureminorversion = signatureminorversion;
_x.filename = filename;
_x.languagecode = languagecode;
_x._createdby_value = _createdby_value;
_x.signatureid = signatureid;
_x.bodyurl = bodyurl;
_x.defaultfilter = defaultfilter;
_x._owninguser_value = _owninguser_value;
_x.ispersonal = ispersonal;
_x._parentreportid_value = _parentreportid_value;
_x.componentstate = componentstate;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.rdlhash = rdlhash;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.originalbodytext = originalbodytext;
_x._modifiedby_value = _modifiedby_value;
_x.introducedversion = introducedversion;
_x.reportidunique = reportidunique;
_x.versionnumber = versionnumber;
_x.filesize = filesize;
_x.bodybinary = bodybinary;
_x.bodybinary_binary = bodybinary_binary;
_x.reporttypecode = reporttypecode;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.ismanaged = ismanaged;
_x.signaturemajorversion = signaturemajorversion;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.createdinmajorversion = createdinmajorversion;
_x.name = name;
return _x;
}
@Function(name = "DownloadReportDefinition")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped downloadReportDefinition() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.DownloadReportDefinition"), DownloadReportDefinitionResponse.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Function(name = "GetReportHistoryLimit")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped getReportHistoryLimit() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.GetReportHistoryLimit"), GetReportHistoryLimitResponse.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Report[");
b.append("isscheduledreport=");
b.append(this.isscheduledreport);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("reportnameonsrs=");
b.append(this.reportnameonsrs);
b.append(", ");
b.append("signaturelcid=");
b.append(this.signaturelcid);
b.append(", ");
b.append("queryinfo=");
b.append(this.queryinfo);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("bodytext=");
b.append(this.bodytext);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("signaturedate=");
b.append(this.signaturedate);
b.append(", ");
b.append("iscustomizable=");
b.append(this.iscustomizable);
b.append(", ");
b.append("customreportxml=");
b.append(this.customreportxml);
b.append(", ");
b.append("iscustomreport=");
b.append(this.iscustomreport);
b.append(", ");
b.append("schedulexml=");
b.append(this.schedulexml);
b.append(", ");
b.append("reportid=");
b.append(this.reportid);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("mimetype=");
b.append(this.mimetype);
b.append(", ");
b.append("signatureminorversion=");
b.append(this.signatureminorversion);
b.append(", ");
b.append("filename=");
b.append(this.filename);
b.append(", ");
b.append("languagecode=");
b.append(this.languagecode);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("signatureid=");
b.append(this.signatureid);
b.append(", ");
b.append("bodyurl=");
b.append(this.bodyurl);
b.append(", ");
b.append("defaultfilter=");
b.append(this.defaultfilter);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("ispersonal=");
b.append(this.ispersonal);
b.append(", ");
b.append("_parentreportid_value=");
b.append(this._parentreportid_value);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("rdlhash=");
b.append(this.rdlhash);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("originalbodytext=");
b.append(this.originalbodytext);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("introducedversion=");
b.append(this.introducedversion);
b.append(", ");
b.append("reportidunique=");
b.append(this.reportidunique);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("filesize=");
b.append(this.filesize);
b.append(", ");
b.append("bodybinary=");
b.append(this.bodybinary);
b.append(", ");
b.append("bodybinary_binary=");
b.append(this.bodybinary_binary);
b.append(", ");
b.append("reporttypecode=");
b.append(this.reporttypecode);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("signaturemajorversion=");
b.append(this.signaturemajorversion);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("createdinmajorversion=");
b.append(this.createdinmajorversion);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy