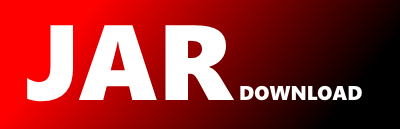
microsoft.dynamics.crm.entity.Sdkmessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.SdkmessagefilterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessageprocessingstepCollectionRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
@JsonPropertyOrder({
"@odata.type",
"_organizationid_value",
"isprivate",
"_modifiedby_value",
"introducedversion",
"workflowsdkstepenabled",
"expand",
"availability",
"modifiedon",
"template",
"isvalidforexecuteasync",
"categoryname",
"autotransact",
"createdon",
"overwritetime",
"isactive",
"_createdby_value",
"name",
"_modifiedonbehalfby_value",
"ismanaged",
"customizationlevel",
"sdkmessageid",
"sdkmessageidunique",
"throttlesettings",
"_createdonbehalfby_value",
"solutionid",
"componentstate",
"versionnumber",
"isreadonly"})
@JsonInclude(Include.NON_NULL)
public class Sdkmessage extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.sdkmessage";
}
@JsonProperty("_organizationid_value")
protected String _organizationid_value;
@JsonProperty("isprivate")
protected Boolean isprivate;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("introducedversion")
protected String introducedversion;
@JsonProperty("workflowsdkstepenabled")
protected Boolean workflowsdkstepenabled;
@JsonProperty("expand")
protected Boolean expand;
@JsonProperty("availability")
protected Integer availability;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("template")
protected Boolean template;
@JsonProperty("isvalidforexecuteasync")
protected Boolean isvalidforexecuteasync;
@JsonProperty("categoryname")
protected String categoryname;
@JsonProperty("autotransact")
protected Boolean autotransact;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("isactive")
protected Boolean isactive;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("name")
protected String name;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("customizationlevel")
protected Integer customizationlevel;
@JsonProperty("sdkmessageid")
protected String sdkmessageid;
@JsonProperty("sdkmessageidunique")
protected String sdkmessageidunique;
@JsonProperty("throttlesettings")
protected String throttlesettings;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("isreadonly")
protected Boolean isreadonly;
protected Sdkmessage() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSdkmessage() {
return new Builder();
}
public static final class Builder {
private String _organizationid_value;
private Boolean isprivate;
private String _modifiedby_value;
private String introducedversion;
private Boolean workflowsdkstepenabled;
private Boolean expand;
private Integer availability;
private OffsetDateTime modifiedon;
private Boolean template;
private Boolean isvalidforexecuteasync;
private String categoryname;
private Boolean autotransact;
private OffsetDateTime createdon;
private OffsetDateTime overwritetime;
private Boolean isactive;
private String _createdby_value;
private String name;
private String _modifiedonbehalfby_value;
private Boolean ismanaged;
private Integer customizationlevel;
private String sdkmessageid;
private String sdkmessageidunique;
private String throttlesettings;
private String _createdonbehalfby_value;
private String solutionid;
private Integer componentstate;
private Long versionnumber;
private Boolean isreadonly;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder _organizationid_value(String _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder isprivate(Boolean isprivate) {
this.isprivate = isprivate;
this.changedFields = changedFields.add("isprivate");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder introducedversion(String introducedversion) {
this.introducedversion = introducedversion;
this.changedFields = changedFields.add("introducedversion");
return this;
}
public Builder workflowsdkstepenabled(Boolean workflowsdkstepenabled) {
this.workflowsdkstepenabled = workflowsdkstepenabled;
this.changedFields = changedFields.add("workflowsdkstepenabled");
return this;
}
public Builder expand(Boolean expand) {
this.expand = expand;
this.changedFields = changedFields.add("expand");
return this;
}
public Builder availability(Integer availability) {
this.availability = availability;
this.changedFields = changedFields.add("availability");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder template(Boolean template) {
this.template = template;
this.changedFields = changedFields.add("template");
return this;
}
public Builder isvalidforexecuteasync(Boolean isvalidforexecuteasync) {
this.isvalidforexecuteasync = isvalidforexecuteasync;
this.changedFields = changedFields.add("isvalidforexecuteasync");
return this;
}
public Builder categoryname(String categoryname) {
this.categoryname = categoryname;
this.changedFields = changedFields.add("categoryname");
return this;
}
public Builder autotransact(Boolean autotransact) {
this.autotransact = autotransact;
this.changedFields = changedFields.add("autotransact");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder isactive(Boolean isactive) {
this.isactive = isactive;
this.changedFields = changedFields.add("isactive");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder customizationlevel(Integer customizationlevel) {
this.customizationlevel = customizationlevel;
this.changedFields = changedFields.add("customizationlevel");
return this;
}
public Builder sdkmessageid(String sdkmessageid) {
this.sdkmessageid = sdkmessageid;
this.changedFields = changedFields.add("sdkmessageid");
return this;
}
public Builder sdkmessageidunique(String sdkmessageidunique) {
this.sdkmessageidunique = sdkmessageidunique;
this.changedFields = changedFields.add("sdkmessageidunique");
return this;
}
public Builder throttlesettings(String throttlesettings) {
this.throttlesettings = throttlesettings;
this.changedFields = changedFields.add("throttlesettings");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder isreadonly(Boolean isreadonly) {
this.isreadonly = isreadonly;
this.changedFields = changedFields.add("isreadonly");
return this;
}
public Sdkmessage build() {
Sdkmessage _x = new Sdkmessage();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.sdkmessage";
_x._organizationid_value = _organizationid_value;
_x.isprivate = isprivate;
_x._modifiedby_value = _modifiedby_value;
_x.introducedversion = introducedversion;
_x.workflowsdkstepenabled = workflowsdkstepenabled;
_x.expand = expand;
_x.availability = availability;
_x.modifiedon = modifiedon;
_x.template = template;
_x.isvalidforexecuteasync = isvalidforexecuteasync;
_x.categoryname = categoryname;
_x.autotransact = autotransact;
_x.createdon = createdon;
_x.overwritetime = overwritetime;
_x.isactive = isactive;
_x._createdby_value = _createdby_value;
_x.name = name;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.ismanaged = ismanaged;
_x.customizationlevel = customizationlevel;
_x.sdkmessageid = sdkmessageid;
_x.sdkmessageidunique = sdkmessageidunique;
_x.throttlesettings = throttlesettings;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.solutionid = solutionid;
_x.componentstate = componentstate;
_x.versionnumber = versionnumber;
_x.isreadonly = isreadonly;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && sdkmessageid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(sdkmessageid.toString()));
}
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Sdkmessage with_organizationid_value(String _organizationid_value) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="isprivate")
@JsonIgnore
public Optional getIsprivate() {
return Optional.ofNullable(isprivate);
}
public Sdkmessage withIsprivate(Boolean isprivate) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("isprivate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.isprivate = isprivate;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Sdkmessage with_modifiedby_value(String _modifiedby_value) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="introducedversion")
@JsonIgnore
public Optional getIntroducedversion() {
return Optional.ofNullable(introducedversion);
}
public Sdkmessage withIntroducedversion(String introducedversion) {
Checks.checkIsAscii(introducedversion);
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("introducedversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.introducedversion = introducedversion;
return _x;
}
@Property(name="workflowsdkstepenabled")
@JsonIgnore
public Optional getWorkflowsdkstepenabled() {
return Optional.ofNullable(workflowsdkstepenabled);
}
public Sdkmessage withWorkflowsdkstepenabled(Boolean workflowsdkstepenabled) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("workflowsdkstepenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.workflowsdkstepenabled = workflowsdkstepenabled;
return _x;
}
@Property(name="expand")
@JsonIgnore
public Optional getExpand() {
return Optional.ofNullable(expand);
}
public Sdkmessage withExpand(Boolean expand) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("expand");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.expand = expand;
return _x;
}
@Property(name="availability")
@JsonIgnore
public Optional getAvailability() {
return Optional.ofNullable(availability);
}
public Sdkmessage withAvailability(Integer availability) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("availability");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.availability = availability;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Sdkmessage withModifiedon(OffsetDateTime modifiedon) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="template")
@JsonIgnore
public Optional getTemplate() {
return Optional.ofNullable(template);
}
public Sdkmessage withTemplate(Boolean template) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("template");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.template = template;
return _x;
}
@Property(name="isvalidforexecuteasync")
@JsonIgnore
public Optional getIsvalidforexecuteasync() {
return Optional.ofNullable(isvalidforexecuteasync);
}
public Sdkmessage withIsvalidforexecuteasync(Boolean isvalidforexecuteasync) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("isvalidforexecuteasync");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.isvalidforexecuteasync = isvalidforexecuteasync;
return _x;
}
@Property(name="categoryname")
@JsonIgnore
public Optional getCategoryname() {
return Optional.ofNullable(categoryname);
}
public Sdkmessage withCategoryname(String categoryname) {
Checks.checkIsAscii(categoryname);
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("categoryname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.categoryname = categoryname;
return _x;
}
@Property(name="autotransact")
@JsonIgnore
public Optional getAutotransact() {
return Optional.ofNullable(autotransact);
}
public Sdkmessage withAutotransact(Boolean autotransact) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("autotransact");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.autotransact = autotransact;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Sdkmessage withCreatedon(OffsetDateTime createdon) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.createdon = createdon;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Sdkmessage withOverwritetime(OffsetDateTime overwritetime) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="isactive")
@JsonIgnore
public Optional getIsactive() {
return Optional.ofNullable(isactive);
}
public Sdkmessage withIsactive(Boolean isactive) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("isactive");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.isactive = isactive;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Sdkmessage with_createdby_value(String _createdby_value) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Sdkmessage withName(String name) {
Checks.checkIsAscii(name);
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.name = name;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Sdkmessage with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Sdkmessage withIsmanaged(Boolean ismanaged) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="customizationlevel")
@JsonIgnore
public Optional getCustomizationlevel() {
return Optional.ofNullable(customizationlevel);
}
public Sdkmessage withCustomizationlevel(Integer customizationlevel) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("customizationlevel");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.customizationlevel = customizationlevel;
return _x;
}
@Property(name="sdkmessageid")
@JsonIgnore
public Optional getSdkmessageid() {
return Optional.ofNullable(sdkmessageid);
}
public Sdkmessage withSdkmessageid(String sdkmessageid) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("sdkmessageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.sdkmessageid = sdkmessageid;
return _x;
}
@Property(name="sdkmessageidunique")
@JsonIgnore
public Optional getSdkmessageidunique() {
return Optional.ofNullable(sdkmessageidunique);
}
public Sdkmessage withSdkmessageidunique(String sdkmessageidunique) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("sdkmessageidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.sdkmessageidunique = sdkmessageidunique;
return _x;
}
@Property(name="throttlesettings")
@JsonIgnore
public Optional getThrottlesettings() {
return Optional.ofNullable(throttlesettings);
}
public Sdkmessage withThrottlesettings(String throttlesettings) {
Checks.checkIsAscii(throttlesettings);
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("throttlesettings");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.throttlesettings = throttlesettings;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Sdkmessage with_createdonbehalfby_value(String _createdonbehalfby_value) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Sdkmessage withSolutionid(String solutionid) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.solutionid = solutionid;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Sdkmessage withComponentstate(Integer componentstate) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.componentstate = componentstate;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Sdkmessage withVersionnumber(Long versionnumber) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="isreadonly")
@JsonIgnore
public Optional getIsreadonly() {
return Optional.ofNullable(isreadonly);
}
public Sdkmessage withIsreadonly(Boolean isreadonly) {
Sdkmessage _x = _copy();
_x.changedFields = changedFields.add("isreadonly");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.sdkmessage");
_x.isreadonly = isreadonly;
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="sdkmessageid_sdkmessageprocessingstep")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getSdkmessageid_sdkmessageprocessingstep() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("sdkmessageid_sdkmessageprocessingstep"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"));
}
@NavigationProperty(name="sdkmessageid_sdkmessagefilter")
@JsonIgnore
public SdkmessagefilterCollectionRequest getSdkmessageid_sdkmessagefilter() {
return new SdkmessagefilterCollectionRequest(
contextPath.addSegment("sdkmessageid_sdkmessagefilter"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Sdkmessage patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Sdkmessage _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Sdkmessage put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Sdkmessage _x = _copy();
_x.changedFields = null;
return _x;
}
private Sdkmessage _copy() {
Sdkmessage _x = new Sdkmessage();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x._organizationid_value = _organizationid_value;
_x.isprivate = isprivate;
_x._modifiedby_value = _modifiedby_value;
_x.introducedversion = introducedversion;
_x.workflowsdkstepenabled = workflowsdkstepenabled;
_x.expand = expand;
_x.availability = availability;
_x.modifiedon = modifiedon;
_x.template = template;
_x.isvalidforexecuteasync = isvalidforexecuteasync;
_x.categoryname = categoryname;
_x.autotransact = autotransact;
_x.createdon = createdon;
_x.overwritetime = overwritetime;
_x.isactive = isactive;
_x._createdby_value = _createdby_value;
_x.name = name;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.ismanaged = ismanaged;
_x.customizationlevel = customizationlevel;
_x.sdkmessageid = sdkmessageid;
_x.sdkmessageidunique = sdkmessageidunique;
_x.throttlesettings = throttlesettings;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.solutionid = solutionid;
_x.componentstate = componentstate;
_x.versionnumber = versionnumber;
_x.isreadonly = isreadonly;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Sdkmessage[");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("isprivate=");
b.append(this.isprivate);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("introducedversion=");
b.append(this.introducedversion);
b.append(", ");
b.append("workflowsdkstepenabled=");
b.append(this.workflowsdkstepenabled);
b.append(", ");
b.append("expand=");
b.append(this.expand);
b.append(", ");
b.append("availability=");
b.append(this.availability);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("template=");
b.append(this.template);
b.append(", ");
b.append("isvalidforexecuteasync=");
b.append(this.isvalidforexecuteasync);
b.append(", ");
b.append("categoryname=");
b.append(this.categoryname);
b.append(", ");
b.append("autotransact=");
b.append(this.autotransact);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("isactive=");
b.append(this.isactive);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("customizationlevel=");
b.append(this.customizationlevel);
b.append(", ");
b.append("sdkmessageid=");
b.append(this.sdkmessageid);
b.append(", ");
b.append("sdkmessageidunique=");
b.append(this.sdkmessageidunique);
b.append(", ");
b.append("throttlesettings=");
b.append(this.throttlesettings);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("isreadonly=");
b.append(this.isreadonly);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy