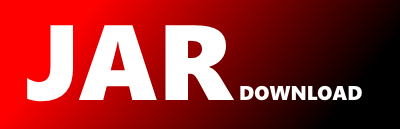
microsoft.dynamics.crm.entity.Solutionhistorydata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"ismicrosoftpublisher",
"status",
"isoverwritecustomizations",
"solutionid",
"solutionversion",
"correlationid",
"solutionhistorydataid",
"starttime",
"operation",
"exceptionstack",
"ismanaged",
"result",
"errorcode",
"solutionname",
"endtime",
"packagename",
"publishername",
"suboperation",
"packageversion",
"activityid",
"ispatch",
"exceptionmessage"})
@JsonInclude(Include.NON_NULL)
public class Solutionhistorydata extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.solutionhistorydata";
}
@JsonProperty("ismicrosoftpublisher")
protected Boolean ismicrosoftpublisher;
@JsonProperty("status")
protected Integer status;
@JsonProperty("isoverwritecustomizations")
protected Boolean isoverwritecustomizations;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("solutionversion")
protected String solutionversion;
@JsonProperty("correlationid")
protected String correlationid;
@JsonProperty("solutionhistorydataid")
protected String solutionhistorydataid;
@JsonProperty("starttime")
protected OffsetDateTime starttime;
@JsonProperty("operation")
protected Integer operation;
@JsonProperty("exceptionstack")
protected String exceptionstack;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("result")
protected Integer result;
@JsonProperty("errorcode")
protected Integer errorcode;
@JsonProperty("solutionname")
protected String solutionname;
@JsonProperty("endtime")
protected OffsetDateTime endtime;
@JsonProperty("packagename")
protected String packagename;
@JsonProperty("publishername")
protected String publishername;
@JsonProperty("suboperation")
protected Integer suboperation;
@JsonProperty("packageversion")
protected String packageversion;
@JsonProperty("activityid")
protected String activityid;
@JsonProperty("ispatch")
protected Boolean ispatch;
@JsonProperty("exceptionmessage")
protected String exceptionmessage;
protected Solutionhistorydata() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSolutionhistorydata() {
return new Builder();
}
public static final class Builder {
private Boolean ismicrosoftpublisher;
private Integer status;
private Boolean isoverwritecustomizations;
private String solutionid;
private String solutionversion;
private String correlationid;
private String solutionhistorydataid;
private OffsetDateTime starttime;
private Integer operation;
private String exceptionstack;
private Boolean ismanaged;
private Integer result;
private Integer errorcode;
private String solutionname;
private OffsetDateTime endtime;
private String packagename;
private String publishername;
private Integer suboperation;
private String packageversion;
private String activityid;
private Boolean ispatch;
private String exceptionmessage;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder ismicrosoftpublisher(Boolean ismicrosoftpublisher) {
this.ismicrosoftpublisher = ismicrosoftpublisher;
this.changedFields = changedFields.add("ismicrosoftpublisher");
return this;
}
public Builder status(Integer status) {
this.status = status;
this.changedFields = changedFields.add("status");
return this;
}
public Builder isoverwritecustomizations(Boolean isoverwritecustomizations) {
this.isoverwritecustomizations = isoverwritecustomizations;
this.changedFields = changedFields.add("isoverwritecustomizations");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder solutionversion(String solutionversion) {
this.solutionversion = solutionversion;
this.changedFields = changedFields.add("solutionversion");
return this;
}
public Builder correlationid(String correlationid) {
this.correlationid = correlationid;
this.changedFields = changedFields.add("correlationid");
return this;
}
public Builder solutionhistorydataid(String solutionhistorydataid) {
this.solutionhistorydataid = solutionhistorydataid;
this.changedFields = changedFields.add("solutionhistorydataid");
return this;
}
public Builder starttime(OffsetDateTime starttime) {
this.starttime = starttime;
this.changedFields = changedFields.add("starttime");
return this;
}
public Builder operation(Integer operation) {
this.operation = operation;
this.changedFields = changedFields.add("operation");
return this;
}
public Builder exceptionstack(String exceptionstack) {
this.exceptionstack = exceptionstack;
this.changedFields = changedFields.add("exceptionstack");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder result(Integer result) {
this.result = result;
this.changedFields = changedFields.add("result");
return this;
}
public Builder errorcode(Integer errorcode) {
this.errorcode = errorcode;
this.changedFields = changedFields.add("errorcode");
return this;
}
public Builder solutionname(String solutionname) {
this.solutionname = solutionname;
this.changedFields = changedFields.add("solutionname");
return this;
}
public Builder endtime(OffsetDateTime endtime) {
this.endtime = endtime;
this.changedFields = changedFields.add("endtime");
return this;
}
public Builder packagename(String packagename) {
this.packagename = packagename;
this.changedFields = changedFields.add("packagename");
return this;
}
public Builder publishername(String publishername) {
this.publishername = publishername;
this.changedFields = changedFields.add("publishername");
return this;
}
public Builder suboperation(Integer suboperation) {
this.suboperation = suboperation;
this.changedFields = changedFields.add("suboperation");
return this;
}
public Builder packageversion(String packageversion) {
this.packageversion = packageversion;
this.changedFields = changedFields.add("packageversion");
return this;
}
public Builder activityid(String activityid) {
this.activityid = activityid;
this.changedFields = changedFields.add("activityid");
return this;
}
public Builder ispatch(Boolean ispatch) {
this.ispatch = ispatch;
this.changedFields = changedFields.add("ispatch");
return this;
}
public Builder exceptionmessage(String exceptionmessage) {
this.exceptionmessage = exceptionmessage;
this.changedFields = changedFields.add("exceptionmessage");
return this;
}
public Solutionhistorydata build() {
Solutionhistorydata _x = new Solutionhistorydata();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.solutionhistorydata";
_x.ismicrosoftpublisher = ismicrosoftpublisher;
_x.status = status;
_x.isoverwritecustomizations = isoverwritecustomizations;
_x.solutionid = solutionid;
_x.solutionversion = solutionversion;
_x.correlationid = correlationid;
_x.solutionhistorydataid = solutionhistorydataid;
_x.starttime = starttime;
_x.operation = operation;
_x.exceptionstack = exceptionstack;
_x.ismanaged = ismanaged;
_x.result = result;
_x.errorcode = errorcode;
_x.solutionname = solutionname;
_x.endtime = endtime;
_x.packagename = packagename;
_x.publishername = publishername;
_x.suboperation = suboperation;
_x.packageversion = packageversion;
_x.activityid = activityid;
_x.ispatch = ispatch;
_x.exceptionmessage = exceptionmessage;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && solutionhistorydataid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(solutionhistorydataid.toString()));
}
}
@Property(name="ismicrosoftpublisher")
@JsonIgnore
public Optional getIsmicrosoftpublisher() {
return Optional.ofNullable(ismicrosoftpublisher);
}
public Solutionhistorydata withIsmicrosoftpublisher(Boolean ismicrosoftpublisher) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("ismicrosoftpublisher");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.ismicrosoftpublisher = ismicrosoftpublisher;
return _x;
}
@Property(name="status")
@JsonIgnore
public Optional getStatus() {
return Optional.ofNullable(status);
}
public Solutionhistorydata withStatus(Integer status) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("status");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.status = status;
return _x;
}
@Property(name="isoverwritecustomizations")
@JsonIgnore
public Optional getIsoverwritecustomizations() {
return Optional.ofNullable(isoverwritecustomizations);
}
public Solutionhistorydata withIsoverwritecustomizations(Boolean isoverwritecustomizations) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("isoverwritecustomizations");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.isoverwritecustomizations = isoverwritecustomizations;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Solutionhistorydata withSolutionid(String solutionid) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.solutionid = solutionid;
return _x;
}
@Property(name="solutionversion")
@JsonIgnore
public Optional getSolutionversion() {
return Optional.ofNullable(solutionversion);
}
public Solutionhistorydata withSolutionversion(String solutionversion) {
Checks.checkIsAscii(solutionversion);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("solutionversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.solutionversion = solutionversion;
return _x;
}
@Property(name="correlationid")
@JsonIgnore
public Optional getCorrelationid() {
return Optional.ofNullable(correlationid);
}
public Solutionhistorydata withCorrelationid(String correlationid) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("correlationid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.correlationid = correlationid;
return _x;
}
@Property(name="solutionhistorydataid")
@JsonIgnore
public Optional getSolutionhistorydataid() {
return Optional.ofNullable(solutionhistorydataid);
}
public Solutionhistorydata withSolutionhistorydataid(String solutionhistorydataid) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("solutionhistorydataid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.solutionhistorydataid = solutionhistorydataid;
return _x;
}
@Property(name="starttime")
@JsonIgnore
public Optional getStarttime() {
return Optional.ofNullable(starttime);
}
public Solutionhistorydata withStarttime(OffsetDateTime starttime) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("starttime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.starttime = starttime;
return _x;
}
@Property(name="operation")
@JsonIgnore
public Optional getOperation() {
return Optional.ofNullable(operation);
}
public Solutionhistorydata withOperation(Integer operation) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("operation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.operation = operation;
return _x;
}
@Property(name="exceptionstack")
@JsonIgnore
public Optional getExceptionstack() {
return Optional.ofNullable(exceptionstack);
}
public Solutionhistorydata withExceptionstack(String exceptionstack) {
Checks.checkIsAscii(exceptionstack);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("exceptionstack");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.exceptionstack = exceptionstack;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Solutionhistorydata withIsmanaged(Boolean ismanaged) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="result")
@JsonIgnore
public Optional getResult() {
return Optional.ofNullable(result);
}
public Solutionhistorydata withResult(Integer result) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("result");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.result = result;
return _x;
}
@Property(name="errorcode")
@JsonIgnore
public Optional getErrorcode() {
return Optional.ofNullable(errorcode);
}
public Solutionhistorydata withErrorcode(Integer errorcode) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("errorcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.errorcode = errorcode;
return _x;
}
@Property(name="solutionname")
@JsonIgnore
public Optional getSolutionname() {
return Optional.ofNullable(solutionname);
}
public Solutionhistorydata withSolutionname(String solutionname) {
Checks.checkIsAscii(solutionname);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("solutionname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.solutionname = solutionname;
return _x;
}
@Property(name="endtime")
@JsonIgnore
public Optional getEndtime() {
return Optional.ofNullable(endtime);
}
public Solutionhistorydata withEndtime(OffsetDateTime endtime) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("endtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.endtime = endtime;
return _x;
}
@Property(name="packagename")
@JsonIgnore
public Optional getPackagename() {
return Optional.ofNullable(packagename);
}
public Solutionhistorydata withPackagename(String packagename) {
Checks.checkIsAscii(packagename);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("packagename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.packagename = packagename;
return _x;
}
@Property(name="publishername")
@JsonIgnore
public Optional getPublishername() {
return Optional.ofNullable(publishername);
}
public Solutionhistorydata withPublishername(String publishername) {
Checks.checkIsAscii(publishername);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("publishername");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.publishername = publishername;
return _x;
}
@Property(name="suboperation")
@JsonIgnore
public Optional getSuboperation() {
return Optional.ofNullable(suboperation);
}
public Solutionhistorydata withSuboperation(Integer suboperation) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("suboperation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.suboperation = suboperation;
return _x;
}
@Property(name="packageversion")
@JsonIgnore
public Optional getPackageversion() {
return Optional.ofNullable(packageversion);
}
public Solutionhistorydata withPackageversion(String packageversion) {
Checks.checkIsAscii(packageversion);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("packageversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.packageversion = packageversion;
return _x;
}
@Property(name="activityid")
@JsonIgnore
public Optional getActivityid() {
return Optional.ofNullable(activityid);
}
public Solutionhistorydata withActivityid(String activityid) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("activityid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.activityid = activityid;
return _x;
}
@Property(name="ispatch")
@JsonIgnore
public Optional getIspatch() {
return Optional.ofNullable(ispatch);
}
public Solutionhistorydata withIspatch(Boolean ispatch) {
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("ispatch");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.ispatch = ispatch;
return _x;
}
@Property(name="exceptionmessage")
@JsonIgnore
public Optional getExceptionmessage() {
return Optional.ofNullable(exceptionmessage);
}
public Solutionhistorydata withExceptionmessage(String exceptionmessage) {
Checks.checkIsAscii(exceptionmessage);
Solutionhistorydata _x = _copy();
_x.changedFields = changedFields.add("exceptionmessage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.solutionhistorydata");
_x.exceptionmessage = exceptionmessage;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Solutionhistorydata patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Solutionhistorydata _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Solutionhistorydata put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Solutionhistorydata _x = _copy();
_x.changedFields = null;
return _x;
}
private Solutionhistorydata _copy() {
Solutionhistorydata _x = new Solutionhistorydata();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.ismicrosoftpublisher = ismicrosoftpublisher;
_x.status = status;
_x.isoverwritecustomizations = isoverwritecustomizations;
_x.solutionid = solutionid;
_x.solutionversion = solutionversion;
_x.correlationid = correlationid;
_x.solutionhistorydataid = solutionhistorydataid;
_x.starttime = starttime;
_x.operation = operation;
_x.exceptionstack = exceptionstack;
_x.ismanaged = ismanaged;
_x.result = result;
_x.errorcode = errorcode;
_x.solutionname = solutionname;
_x.endtime = endtime;
_x.packagename = packagename;
_x.publishername = publishername;
_x.suboperation = suboperation;
_x.packageversion = packageversion;
_x.activityid = activityid;
_x.ispatch = ispatch;
_x.exceptionmessage = exceptionmessage;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Solutionhistorydata[");
b.append("ismicrosoftpublisher=");
b.append(this.ismicrosoftpublisher);
b.append(", ");
b.append("status=");
b.append(this.status);
b.append(", ");
b.append("isoverwritecustomizations=");
b.append(this.isoverwritecustomizations);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("solutionversion=");
b.append(this.solutionversion);
b.append(", ");
b.append("correlationid=");
b.append(this.correlationid);
b.append(", ");
b.append("solutionhistorydataid=");
b.append(this.solutionhistorydataid);
b.append(", ");
b.append("starttime=");
b.append(this.starttime);
b.append(", ");
b.append("operation=");
b.append(this.operation);
b.append(", ");
b.append("exceptionstack=");
b.append(this.exceptionstack);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("result=");
b.append(this.result);
b.append(", ");
b.append("errorcode=");
b.append(this.errorcode);
b.append(", ");
b.append("solutionname=");
b.append(this.solutionname);
b.append(", ");
b.append("endtime=");
b.append(this.endtime);
b.append(", ");
b.append("packagename=");
b.append(this.packagename);
b.append(", ");
b.append("publishername=");
b.append(this.publishername);
b.append(", ");
b.append("suboperation=");
b.append(this.suboperation);
b.append(", ");
b.append("packageversion=");
b.append(this.packageversion);
b.append(", ");
b.append("activityid=");
b.append(this.activityid);
b.append(", ");
b.append("ispatch=");
b.append(this.ispatch);
b.append(", ");
b.append("exceptionmessage=");
b.append(this.exceptionmessage);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy