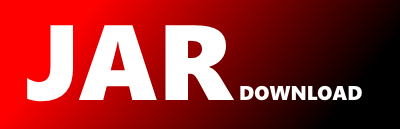
microsoft.dynamics.crm.entity.Theme Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
import microsoft.dynamics.crm.entity.request.WebresourceRequest;
@JsonPropertyOrder({
"@odata.type",
"headercolor",
"name",
"controlborder",
"globallinkcolor",
"navbarbackgroundcolor",
"versionnumber",
"_modifiedby_value",
"backgroundcolor",
"_logoid_value",
"_organizationid_value",
"isdefaulttheme",
"exchangerate",
"importsequencenumber",
"pageheaderbackgroundcolor",
"overriddencreatedon",
"processcontrolcolor",
"panelheaderbackgroundcolor",
"_transactioncurrencyid_value",
"statuscode",
"type",
"utcconversiontimezonecode",
"hoverlinkeffect",
"accentcolor",
"timezoneruleversionnumber",
"_createdonbehalfby_value",
"themeid",
"defaultcustomentitycolor",
"createdon",
"defaultentitycolor",
"selectedlinkeffect",
"navbarshelfcolor",
"statecode",
"maincolor",
"logotooltip",
"_createdby_value",
"controlshade",
"_modifiedonbehalfby_value",
"modifiedon"})
@JsonInclude(Include.NON_NULL)
public class Theme extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.theme";
}
@JsonProperty("headercolor")
protected String headercolor;
@JsonProperty("name")
protected String name;
@JsonProperty("controlborder")
protected String controlborder;
@JsonProperty("globallinkcolor")
protected String globallinkcolor;
@JsonProperty("navbarbackgroundcolor")
protected String navbarbackgroundcolor;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("backgroundcolor")
protected String backgroundcolor;
@JsonProperty("_logoid_value")
protected String _logoid_value;
@JsonProperty("_organizationid_value")
protected String _organizationid_value;
@JsonProperty("isdefaulttheme")
protected Boolean isdefaulttheme;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("pageheaderbackgroundcolor")
protected String pageheaderbackgroundcolor;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("processcontrolcolor")
protected String processcontrolcolor;
@JsonProperty("panelheaderbackgroundcolor")
protected String panelheaderbackgroundcolor;
@JsonProperty("_transactioncurrencyid_value")
protected String _transactioncurrencyid_value;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("type")
protected Boolean type;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("hoverlinkeffect")
protected String hoverlinkeffect;
@JsonProperty("accentcolor")
protected String accentcolor;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("themeid")
protected String themeid;
@JsonProperty("defaultcustomentitycolor")
protected String defaultcustomentitycolor;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("defaultentitycolor")
protected String defaultentitycolor;
@JsonProperty("selectedlinkeffect")
protected String selectedlinkeffect;
@JsonProperty("navbarshelfcolor")
protected String navbarshelfcolor;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("maincolor")
protected String maincolor;
@JsonProperty("logotooltip")
protected String logotooltip;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("controlshade")
protected String controlshade;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
protected Theme() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderTheme() {
return new Builder();
}
public static final class Builder {
private String headercolor;
private String name;
private String controlborder;
private String globallinkcolor;
private String navbarbackgroundcolor;
private Long versionnumber;
private String _modifiedby_value;
private String backgroundcolor;
private String _logoid_value;
private String _organizationid_value;
private Boolean isdefaulttheme;
private BigDecimal exchangerate;
private Integer importsequencenumber;
private String pageheaderbackgroundcolor;
private OffsetDateTime overriddencreatedon;
private String processcontrolcolor;
private String panelheaderbackgroundcolor;
private String _transactioncurrencyid_value;
private Integer statuscode;
private Boolean type;
private Integer utcconversiontimezonecode;
private String hoverlinkeffect;
private String accentcolor;
private Integer timezoneruleversionnumber;
private String _createdonbehalfby_value;
private String themeid;
private String defaultcustomentitycolor;
private OffsetDateTime createdon;
private String defaultentitycolor;
private String selectedlinkeffect;
private String navbarshelfcolor;
private Integer statecode;
private String maincolor;
private String logotooltip;
private String _createdby_value;
private String controlshade;
private String _modifiedonbehalfby_value;
private OffsetDateTime modifiedon;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder headercolor(String headercolor) {
this.headercolor = headercolor;
this.changedFields = changedFields.add("headercolor");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder controlborder(String controlborder) {
this.controlborder = controlborder;
this.changedFields = changedFields.add("controlborder");
return this;
}
public Builder globallinkcolor(String globallinkcolor) {
this.globallinkcolor = globallinkcolor;
this.changedFields = changedFields.add("globallinkcolor");
return this;
}
public Builder navbarbackgroundcolor(String navbarbackgroundcolor) {
this.navbarbackgroundcolor = navbarbackgroundcolor;
this.changedFields = changedFields.add("navbarbackgroundcolor");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder backgroundcolor(String backgroundcolor) {
this.backgroundcolor = backgroundcolor;
this.changedFields = changedFields.add("backgroundcolor");
return this;
}
public Builder _logoid_value(String _logoid_value) {
this._logoid_value = _logoid_value;
this.changedFields = changedFields.add("_logoid_value");
return this;
}
public Builder _organizationid_value(String _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder isdefaulttheme(Boolean isdefaulttheme) {
this.isdefaulttheme = isdefaulttheme;
this.changedFields = changedFields.add("isdefaulttheme");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder pageheaderbackgroundcolor(String pageheaderbackgroundcolor) {
this.pageheaderbackgroundcolor = pageheaderbackgroundcolor;
this.changedFields = changedFields.add("pageheaderbackgroundcolor");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder processcontrolcolor(String processcontrolcolor) {
this.processcontrolcolor = processcontrolcolor;
this.changedFields = changedFields.add("processcontrolcolor");
return this;
}
public Builder panelheaderbackgroundcolor(String panelheaderbackgroundcolor) {
this.panelheaderbackgroundcolor = panelheaderbackgroundcolor;
this.changedFields = changedFields.add("panelheaderbackgroundcolor");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder type(Boolean type) {
this.type = type;
this.changedFields = changedFields.add("type");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder hoverlinkeffect(String hoverlinkeffect) {
this.hoverlinkeffect = hoverlinkeffect;
this.changedFields = changedFields.add("hoverlinkeffect");
return this;
}
public Builder accentcolor(String accentcolor) {
this.accentcolor = accentcolor;
this.changedFields = changedFields.add("accentcolor");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder themeid(String themeid) {
this.themeid = themeid;
this.changedFields = changedFields.add("themeid");
return this;
}
public Builder defaultcustomentitycolor(String defaultcustomentitycolor) {
this.defaultcustomentitycolor = defaultcustomentitycolor;
this.changedFields = changedFields.add("defaultcustomentitycolor");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder defaultentitycolor(String defaultentitycolor) {
this.defaultentitycolor = defaultentitycolor;
this.changedFields = changedFields.add("defaultentitycolor");
return this;
}
public Builder selectedlinkeffect(String selectedlinkeffect) {
this.selectedlinkeffect = selectedlinkeffect;
this.changedFields = changedFields.add("selectedlinkeffect");
return this;
}
public Builder navbarshelfcolor(String navbarshelfcolor) {
this.navbarshelfcolor = navbarshelfcolor;
this.changedFields = changedFields.add("navbarshelfcolor");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder maincolor(String maincolor) {
this.maincolor = maincolor;
this.changedFields = changedFields.add("maincolor");
return this;
}
public Builder logotooltip(String logotooltip) {
this.logotooltip = logotooltip;
this.changedFields = changedFields.add("logotooltip");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder controlshade(String controlshade) {
this.controlshade = controlshade;
this.changedFields = changedFields.add("controlshade");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Theme build() {
Theme _x = new Theme();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.theme";
_x.headercolor = headercolor;
_x.name = name;
_x.controlborder = controlborder;
_x.globallinkcolor = globallinkcolor;
_x.navbarbackgroundcolor = navbarbackgroundcolor;
_x.versionnumber = versionnumber;
_x._modifiedby_value = _modifiedby_value;
_x.backgroundcolor = backgroundcolor;
_x._logoid_value = _logoid_value;
_x._organizationid_value = _organizationid_value;
_x.isdefaulttheme = isdefaulttheme;
_x.exchangerate = exchangerate;
_x.importsequencenumber = importsequencenumber;
_x.pageheaderbackgroundcolor = pageheaderbackgroundcolor;
_x.overriddencreatedon = overriddencreatedon;
_x.processcontrolcolor = processcontrolcolor;
_x.panelheaderbackgroundcolor = panelheaderbackgroundcolor;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.statuscode = statuscode;
_x.type = type;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.hoverlinkeffect = hoverlinkeffect;
_x.accentcolor = accentcolor;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.themeid = themeid;
_x.defaultcustomentitycolor = defaultcustomentitycolor;
_x.createdon = createdon;
_x.defaultentitycolor = defaultentitycolor;
_x.selectedlinkeffect = selectedlinkeffect;
_x.navbarshelfcolor = navbarshelfcolor;
_x.statecode = statecode;
_x.maincolor = maincolor;
_x.logotooltip = logotooltip;
_x._createdby_value = _createdby_value;
_x.controlshade = controlshade;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.modifiedon = modifiedon;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && themeid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(themeid.toString()));
}
}
@Property(name="headercolor")
@JsonIgnore
public Optional getHeadercolor() {
return Optional.ofNullable(headercolor);
}
public Theme withHeadercolor(String headercolor) {
Checks.checkIsAscii(headercolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("headercolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.headercolor = headercolor;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Theme withName(String name) {
Checks.checkIsAscii(name);
Theme _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.name = name;
return _x;
}
@Property(name="controlborder")
@JsonIgnore
public Optional getControlborder() {
return Optional.ofNullable(controlborder);
}
public Theme withControlborder(String controlborder) {
Checks.checkIsAscii(controlborder);
Theme _x = _copy();
_x.changedFields = changedFields.add("controlborder");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.controlborder = controlborder;
return _x;
}
@Property(name="globallinkcolor")
@JsonIgnore
public Optional getGloballinkcolor() {
return Optional.ofNullable(globallinkcolor);
}
public Theme withGloballinkcolor(String globallinkcolor) {
Checks.checkIsAscii(globallinkcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("globallinkcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.globallinkcolor = globallinkcolor;
return _x;
}
@Property(name="navbarbackgroundcolor")
@JsonIgnore
public Optional getNavbarbackgroundcolor() {
return Optional.ofNullable(navbarbackgroundcolor);
}
public Theme withNavbarbackgroundcolor(String navbarbackgroundcolor) {
Checks.checkIsAscii(navbarbackgroundcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("navbarbackgroundcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.navbarbackgroundcolor = navbarbackgroundcolor;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Theme withVersionnumber(Long versionnumber) {
Theme _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Theme with_modifiedby_value(String _modifiedby_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="backgroundcolor")
@JsonIgnore
public Optional getBackgroundcolor() {
return Optional.ofNullable(backgroundcolor);
}
public Theme withBackgroundcolor(String backgroundcolor) {
Checks.checkIsAscii(backgroundcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("backgroundcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.backgroundcolor = backgroundcolor;
return _x;
}
@Property(name="_logoid_value")
@JsonIgnore
public Optional get_logoid_value() {
return Optional.ofNullable(_logoid_value);
}
public Theme with_logoid_value(String _logoid_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_logoid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._logoid_value = _logoid_value;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Theme with_organizationid_value(String _organizationid_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="isdefaulttheme")
@JsonIgnore
public Optional getIsdefaulttheme() {
return Optional.ofNullable(isdefaulttheme);
}
public Theme withIsdefaulttheme(Boolean isdefaulttheme) {
Theme _x = _copy();
_x.changedFields = changedFields.add("isdefaulttheme");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.isdefaulttheme = isdefaulttheme;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Theme withExchangerate(BigDecimal exchangerate) {
Theme _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Theme withImportsequencenumber(Integer importsequencenumber) {
Theme _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="pageheaderbackgroundcolor")
@JsonIgnore
public Optional getPageheaderbackgroundcolor() {
return Optional.ofNullable(pageheaderbackgroundcolor);
}
public Theme withPageheaderbackgroundcolor(String pageheaderbackgroundcolor) {
Checks.checkIsAscii(pageheaderbackgroundcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("pageheaderbackgroundcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.pageheaderbackgroundcolor = pageheaderbackgroundcolor;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Theme withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Theme _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="processcontrolcolor")
@JsonIgnore
public Optional getProcesscontrolcolor() {
return Optional.ofNullable(processcontrolcolor);
}
public Theme withProcesscontrolcolor(String processcontrolcolor) {
Checks.checkIsAscii(processcontrolcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("processcontrolcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.processcontrolcolor = processcontrolcolor;
return _x;
}
@Property(name="panelheaderbackgroundcolor")
@JsonIgnore
public Optional getPanelheaderbackgroundcolor() {
return Optional.ofNullable(panelheaderbackgroundcolor);
}
public Theme withPanelheaderbackgroundcolor(String panelheaderbackgroundcolor) {
Checks.checkIsAscii(panelheaderbackgroundcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("panelheaderbackgroundcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.panelheaderbackgroundcolor = panelheaderbackgroundcolor;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Theme with_transactioncurrencyid_value(String _transactioncurrencyid_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Theme withStatuscode(Integer statuscode) {
Theme _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.statuscode = statuscode;
return _x;
}
@Property(name="type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public Theme withType(Boolean type) {
Theme _x = _copy();
_x.changedFields = changedFields.add("type");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.type = type;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Theme withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Theme _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="hoverlinkeffect")
@JsonIgnore
public Optional getHoverlinkeffect() {
return Optional.ofNullable(hoverlinkeffect);
}
public Theme withHoverlinkeffect(String hoverlinkeffect) {
Checks.checkIsAscii(hoverlinkeffect);
Theme _x = _copy();
_x.changedFields = changedFields.add("hoverlinkeffect");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.hoverlinkeffect = hoverlinkeffect;
return _x;
}
@Property(name="accentcolor")
@JsonIgnore
public Optional getAccentcolor() {
return Optional.ofNullable(accentcolor);
}
public Theme withAccentcolor(String accentcolor) {
Checks.checkIsAscii(accentcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("accentcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.accentcolor = accentcolor;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Theme withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Theme _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Theme with_createdonbehalfby_value(String _createdonbehalfby_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="themeid")
@JsonIgnore
public Optional getThemeid() {
return Optional.ofNullable(themeid);
}
public Theme withThemeid(String themeid) {
Theme _x = _copy();
_x.changedFields = changedFields.add("themeid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.themeid = themeid;
return _x;
}
@Property(name="defaultcustomentitycolor")
@JsonIgnore
public Optional getDefaultcustomentitycolor() {
return Optional.ofNullable(defaultcustomentitycolor);
}
public Theme withDefaultcustomentitycolor(String defaultcustomentitycolor) {
Checks.checkIsAscii(defaultcustomentitycolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("defaultcustomentitycolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.defaultcustomentitycolor = defaultcustomentitycolor;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Theme withCreatedon(OffsetDateTime createdon) {
Theme _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.createdon = createdon;
return _x;
}
@Property(name="defaultentitycolor")
@JsonIgnore
public Optional getDefaultentitycolor() {
return Optional.ofNullable(defaultentitycolor);
}
public Theme withDefaultentitycolor(String defaultentitycolor) {
Checks.checkIsAscii(defaultentitycolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("defaultentitycolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.defaultentitycolor = defaultentitycolor;
return _x;
}
@Property(name="selectedlinkeffect")
@JsonIgnore
public Optional getSelectedlinkeffect() {
return Optional.ofNullable(selectedlinkeffect);
}
public Theme withSelectedlinkeffect(String selectedlinkeffect) {
Checks.checkIsAscii(selectedlinkeffect);
Theme _x = _copy();
_x.changedFields = changedFields.add("selectedlinkeffect");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.selectedlinkeffect = selectedlinkeffect;
return _x;
}
@Property(name="navbarshelfcolor")
@JsonIgnore
public Optional getNavbarshelfcolor() {
return Optional.ofNullable(navbarshelfcolor);
}
public Theme withNavbarshelfcolor(String navbarshelfcolor) {
Checks.checkIsAscii(navbarshelfcolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("navbarshelfcolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.navbarshelfcolor = navbarshelfcolor;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Theme withStatecode(Integer statecode) {
Theme _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.statecode = statecode;
return _x;
}
@Property(name="maincolor")
@JsonIgnore
public Optional getMaincolor() {
return Optional.ofNullable(maincolor);
}
public Theme withMaincolor(String maincolor) {
Checks.checkIsAscii(maincolor);
Theme _x = _copy();
_x.changedFields = changedFields.add("maincolor");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.maincolor = maincolor;
return _x;
}
@Property(name="logotooltip")
@JsonIgnore
public Optional getLogotooltip() {
return Optional.ofNullable(logotooltip);
}
public Theme withLogotooltip(String logotooltip) {
Checks.checkIsAscii(logotooltip);
Theme _x = _copy();
_x.changedFields = changedFields.add("logotooltip");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.logotooltip = logotooltip;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Theme with_createdby_value(String _createdby_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="controlshade")
@JsonIgnore
public Optional getControlshade() {
return Optional.ofNullable(controlshade);
}
public Theme withControlshade(String controlshade) {
Checks.checkIsAscii(controlshade);
Theme _x = _copy();
_x.changedFields = changedFields.add("controlshade");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.controlshade = controlshade;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Theme with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Theme _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Theme withModifiedon(OffsetDateTime modifiedon) {
Theme _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.theme");
_x.modifiedon = modifiedon;
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"));
}
@NavigationProperty(name="theme_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getTheme_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("theme_AsyncOperations"));
}
@NavigationProperty(name="theme_ProcessSession")
@JsonIgnore
public ProcesssessionCollectionRequest getTheme_ProcessSession() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("theme_ProcessSession"));
}
@NavigationProperty(name="theme_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getTheme_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("theme_BulkDeleteFailures"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"));
}
@NavigationProperty(name="logoimage")
@JsonIgnore
public WebresourceRequest getLogoimage() {
return new WebresourceRequest(contextPath.addSegment("logoimage"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Theme patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Theme _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Theme put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Theme _x = _copy();
_x.changedFields = null;
return _x;
}
private Theme _copy() {
Theme _x = new Theme();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.headercolor = headercolor;
_x.name = name;
_x.controlborder = controlborder;
_x.globallinkcolor = globallinkcolor;
_x.navbarbackgroundcolor = navbarbackgroundcolor;
_x.versionnumber = versionnumber;
_x._modifiedby_value = _modifiedby_value;
_x.backgroundcolor = backgroundcolor;
_x._logoid_value = _logoid_value;
_x._organizationid_value = _organizationid_value;
_x.isdefaulttheme = isdefaulttheme;
_x.exchangerate = exchangerate;
_x.importsequencenumber = importsequencenumber;
_x.pageheaderbackgroundcolor = pageheaderbackgroundcolor;
_x.overriddencreatedon = overriddencreatedon;
_x.processcontrolcolor = processcontrolcolor;
_x.panelheaderbackgroundcolor = panelheaderbackgroundcolor;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.statuscode = statuscode;
_x.type = type;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.hoverlinkeffect = hoverlinkeffect;
_x.accentcolor = accentcolor;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.themeid = themeid;
_x.defaultcustomentitycolor = defaultcustomentitycolor;
_x.createdon = createdon;
_x.defaultentitycolor = defaultentitycolor;
_x.selectedlinkeffect = selectedlinkeffect;
_x.navbarshelfcolor = navbarshelfcolor;
_x.statecode = statecode;
_x.maincolor = maincolor;
_x.logotooltip = logotooltip;
_x._createdby_value = _createdby_value;
_x.controlshade = controlshade;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.modifiedon = modifiedon;
return _x;
}
@Action(name = "PublishTheme")
@JsonIgnore
public ActionRequestNoReturn publishTheme() {
Map _parameters = ParameterMap.empty();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.PublishTheme"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Theme[");
b.append("headercolor=");
b.append(this.headercolor);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("controlborder=");
b.append(this.controlborder);
b.append(", ");
b.append("globallinkcolor=");
b.append(this.globallinkcolor);
b.append(", ");
b.append("navbarbackgroundcolor=");
b.append(this.navbarbackgroundcolor);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("backgroundcolor=");
b.append(this.backgroundcolor);
b.append(", ");
b.append("_logoid_value=");
b.append(this._logoid_value);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("isdefaulttheme=");
b.append(this.isdefaulttheme);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("pageheaderbackgroundcolor=");
b.append(this.pageheaderbackgroundcolor);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("processcontrolcolor=");
b.append(this.processcontrolcolor);
b.append(", ");
b.append("panelheaderbackgroundcolor=");
b.append(this.panelheaderbackgroundcolor);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("type=");
b.append(this.type);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("hoverlinkeffect=");
b.append(this.hoverlinkeffect);
b.append(", ");
b.append("accentcolor=");
b.append(this.accentcolor);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("themeid=");
b.append(this.themeid);
b.append(", ");
b.append("defaultcustomentitycolor=");
b.append(this.defaultcustomentitycolor);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("defaultentitycolor=");
b.append(this.defaultentitycolor);
b.append(", ");
b.append("selectedlinkeffect=");
b.append(this.selectedlinkeffect);
b.append(", ");
b.append("navbarshelfcolor=");
b.append(this.navbarshelfcolor);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("maincolor=");
b.append(this.maincolor);
b.append(", ");
b.append("logotooltip=");
b.append(this.logotooltip);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("controlshade=");
b.append(this.controlshade);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy