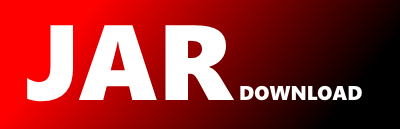
microsoft.dynamics.crm.entity.Usersettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"timezonedaylightsecond",
"numberseparator",
"timezonedaylightbias",
"homepagelayout",
"negativecurrencyformatcode",
"defaultsearchexperience",
"timeseparator",
"longdateformatcode",
"calendartype",
"timezonecode",
"usecrmformforcontact",
"timezonedaylighthour",
"isemailconversationviewenabled",
"versionnumber",
"getstartedpanecontentenabled",
"dateseparator",
"uilanguageid",
"usecrmformfortask",
"autocreatecontactonpromote",
"amdesignator",
"timezonestandarddayofweek",
"timezonebias",
"defaultcalendarview",
"advancedfindstartupmode",
"visualizationpanelayout",
"defaultcountrycode",
"negativeformatcode",
"isresourcebookingexchangesyncenabled",
"localeid",
"autocaptureuserstatus",
"numbergroupformat",
"_modifiedby_value",
"userprofile",
"splitviewstate",
"homepagesubarea",
"helplanguageid",
"homepagearea",
"selectedglobalfilterid",
"_createdonbehalfby_value",
"personalizationsettings",
"dateformatstring",
"trackingtokenid",
"issendasallowed",
"isdefaultcountrycodecheckenabled",
"timezonedaylightdayofweek",
"fullnameconventioncode",
"timezonestandardday",
"usecrmformforappointment",
"dateformatcode",
"timezonestandardhour",
"timezonestandardmonth",
"timezonestandardsecond",
"timezonestandardbias",
"isduplicatedetectionenabledwhengoingonline",
"businessunitid",
"paginglimit",
"outlooksyncinterval",
"createdon",
"offlinesyncinterval",
"systemuserid",
"timezonestandardminute",
"timezonedaylightminute",
"_createdby_value",
"currencysymbol",
"reportscripterrors",
"currencyformatcode",
"timezonedaylightday",
"nexttrackingnumber",
"synccontactcompany",
"entityformmode",
"datavalidationmodeforexporttoexcel",
"decimalsymbol",
"isguidedhelpenabled",
"resourcebookingexchangesyncversion",
"timeformatstring",
"showweeknumber",
"timezonestandardyear",
"lastalertsviewedtime",
"pmdesignator",
"ignoreunsolicitedemail",
"timezonedaylightmonth",
"timeformatcode",
"_modifiedonbehalfby_value",
"workdaystarttime",
"_transactioncurrencyid_value",
"defaultdashboardid",
"isappsforcrmalertdismissed",
"addressbooksyncinterval",
"workdaystoptime",
"timezonedaylightyear",
"incomingemailfilteringmethod",
"modifiedon",
"isautodatacaptureenabled",
"usecrmformforemail",
"useimagestrips"})
@JsonInclude(Include.NON_NULL)
public class Usersettings extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.usersettings";
}
@JsonProperty("timezonedaylightsecond")
protected Integer timezonedaylightsecond;
@JsonProperty("numberseparator")
protected String numberseparator;
@JsonProperty("timezonedaylightbias")
protected Integer timezonedaylightbias;
@JsonProperty("homepagelayout")
protected String homepagelayout;
@JsonProperty("negativecurrencyformatcode")
protected Integer negativecurrencyformatcode;
@JsonProperty("defaultsearchexperience")
protected Integer defaultsearchexperience;
@JsonProperty("timeseparator")
protected String timeseparator;
@JsonProperty("longdateformatcode")
protected Integer longdateformatcode;
@JsonProperty("calendartype")
protected Integer calendartype;
@JsonProperty("timezonecode")
protected Integer timezonecode;
@JsonProperty("usecrmformforcontact")
protected Boolean usecrmformforcontact;
@JsonProperty("timezonedaylighthour")
protected Integer timezonedaylighthour;
@JsonProperty("isemailconversationviewenabled")
protected Boolean isemailconversationviewenabled;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("getstartedpanecontentenabled")
protected Boolean getstartedpanecontentenabled;
@JsonProperty("dateseparator")
protected String dateseparator;
@JsonProperty("uilanguageid")
protected Integer uilanguageid;
@JsonProperty("usecrmformfortask")
protected Boolean usecrmformfortask;
@JsonProperty("autocreatecontactonpromote")
protected Integer autocreatecontactonpromote;
@JsonProperty("amdesignator")
protected String amdesignator;
@JsonProperty("timezonestandarddayofweek")
protected Integer timezonestandarddayofweek;
@JsonProperty("timezonebias")
protected Integer timezonebias;
@JsonProperty("defaultcalendarview")
protected Integer defaultcalendarview;
@JsonProperty("advancedfindstartupmode")
protected Integer advancedfindstartupmode;
@JsonProperty("visualizationpanelayout")
protected Integer visualizationpanelayout;
@JsonProperty("defaultcountrycode")
protected String defaultcountrycode;
@JsonProperty("negativeformatcode")
protected Integer negativeformatcode;
@JsonProperty("isresourcebookingexchangesyncenabled")
protected Boolean isresourcebookingexchangesyncenabled;
@JsonProperty("localeid")
protected Integer localeid;
@JsonProperty("autocaptureuserstatus")
protected Integer autocaptureuserstatus;
@JsonProperty("numbergroupformat")
protected String numbergroupformat;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("userprofile")
protected String userprofile;
@JsonProperty("splitviewstate")
protected Boolean splitviewstate;
@JsonProperty("homepagesubarea")
protected String homepagesubarea;
@JsonProperty("helplanguageid")
protected Integer helplanguageid;
@JsonProperty("homepagearea")
protected String homepagearea;
@JsonProperty("selectedglobalfilterid")
protected String selectedglobalfilterid;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("personalizationsettings")
protected String personalizationsettings;
@JsonProperty("dateformatstring")
protected String dateformatstring;
@JsonProperty("trackingtokenid")
protected Integer trackingtokenid;
@JsonProperty("issendasallowed")
protected Boolean issendasallowed;
@JsonProperty("isdefaultcountrycodecheckenabled")
protected Boolean isdefaultcountrycodecheckenabled;
@JsonProperty("timezonedaylightdayofweek")
protected Integer timezonedaylightdayofweek;
@JsonProperty("fullnameconventioncode")
protected Integer fullnameconventioncode;
@JsonProperty("timezonestandardday")
protected Integer timezonestandardday;
@JsonProperty("usecrmformforappointment")
protected Boolean usecrmformforappointment;
@JsonProperty("dateformatcode")
protected Integer dateformatcode;
@JsonProperty("timezonestandardhour")
protected Integer timezonestandardhour;
@JsonProperty("timezonestandardmonth")
protected Integer timezonestandardmonth;
@JsonProperty("timezonestandardsecond")
protected Integer timezonestandardsecond;
@JsonProperty("timezonestandardbias")
protected Integer timezonestandardbias;
@JsonProperty("isduplicatedetectionenabledwhengoingonline")
protected Boolean isduplicatedetectionenabledwhengoingonline;
@JsonProperty("businessunitid")
protected String businessunitid;
@JsonProperty("paginglimit")
protected Integer paginglimit;
@JsonProperty("outlooksyncinterval")
protected Integer outlooksyncinterval;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("offlinesyncinterval")
protected Integer offlinesyncinterval;
@JsonProperty("systemuserid")
protected String systemuserid;
@JsonProperty("timezonestandardminute")
protected Integer timezonestandardminute;
@JsonProperty("timezonedaylightminute")
protected Integer timezonedaylightminute;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("currencysymbol")
protected String currencysymbol;
@JsonProperty("reportscripterrors")
protected Integer reportscripterrors;
@JsonProperty("currencyformatcode")
protected Integer currencyformatcode;
@JsonProperty("timezonedaylightday")
protected Integer timezonedaylightday;
@JsonProperty("nexttrackingnumber")
protected Integer nexttrackingnumber;
@JsonProperty("synccontactcompany")
protected Boolean synccontactcompany;
@JsonProperty("entityformmode")
protected Integer entityformmode;
@JsonProperty("datavalidationmodeforexporttoexcel")
protected Integer datavalidationmodeforexporttoexcel;
@JsonProperty("decimalsymbol")
protected String decimalsymbol;
@JsonProperty("isguidedhelpenabled")
protected Boolean isguidedhelpenabled;
@JsonProperty("resourcebookingexchangesyncversion")
protected Long resourcebookingexchangesyncversion;
@JsonProperty("timeformatstring")
protected String timeformatstring;
@JsonProperty("showweeknumber")
protected Boolean showweeknumber;
@JsonProperty("timezonestandardyear")
protected Integer timezonestandardyear;
@JsonProperty("lastalertsviewedtime")
protected OffsetDateTime lastalertsviewedtime;
@JsonProperty("pmdesignator")
protected String pmdesignator;
@JsonProperty("ignoreunsolicitedemail")
protected Boolean ignoreunsolicitedemail;
@JsonProperty("timezonedaylightmonth")
protected Integer timezonedaylightmonth;
@JsonProperty("timeformatcode")
protected Integer timeformatcode;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("workdaystarttime")
protected String workdaystarttime;
@JsonProperty("_transactioncurrencyid_value")
protected String _transactioncurrencyid_value;
@JsonProperty("defaultdashboardid")
protected String defaultdashboardid;
@JsonProperty("isappsforcrmalertdismissed")
protected Boolean isappsforcrmalertdismissed;
@JsonProperty("addressbooksyncinterval")
protected Integer addressbooksyncinterval;
@JsonProperty("workdaystoptime")
protected String workdaystoptime;
@JsonProperty("timezonedaylightyear")
protected Integer timezonedaylightyear;
@JsonProperty("incomingemailfilteringmethod")
protected Integer incomingemailfilteringmethod;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("isautodatacaptureenabled")
protected Boolean isautodatacaptureenabled;
@JsonProperty("usecrmformforemail")
protected Boolean usecrmformforemail;
@JsonProperty("useimagestrips")
protected Boolean useimagestrips;
protected Usersettings() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderUsersettings() {
return new Builder();
}
public static final class Builder {
private Integer timezonedaylightsecond;
private String numberseparator;
private Integer timezonedaylightbias;
private String homepagelayout;
private Integer negativecurrencyformatcode;
private Integer defaultsearchexperience;
private String timeseparator;
private Integer longdateformatcode;
private Integer calendartype;
private Integer timezonecode;
private Boolean usecrmformforcontact;
private Integer timezonedaylighthour;
private Boolean isemailconversationviewenabled;
private Long versionnumber;
private Boolean getstartedpanecontentenabled;
private String dateseparator;
private Integer uilanguageid;
private Boolean usecrmformfortask;
private Integer autocreatecontactonpromote;
private String amdesignator;
private Integer timezonestandarddayofweek;
private Integer timezonebias;
private Integer defaultcalendarview;
private Integer advancedfindstartupmode;
private Integer visualizationpanelayout;
private String defaultcountrycode;
private Integer negativeformatcode;
private Boolean isresourcebookingexchangesyncenabled;
private Integer localeid;
private Integer autocaptureuserstatus;
private String numbergroupformat;
private String _modifiedby_value;
private String userprofile;
private Boolean splitviewstate;
private String homepagesubarea;
private Integer helplanguageid;
private String homepagearea;
private String selectedglobalfilterid;
private String _createdonbehalfby_value;
private String personalizationsettings;
private String dateformatstring;
private Integer trackingtokenid;
private Boolean issendasallowed;
private Boolean isdefaultcountrycodecheckenabled;
private Integer timezonedaylightdayofweek;
private Integer fullnameconventioncode;
private Integer timezonestandardday;
private Boolean usecrmformforappointment;
private Integer dateformatcode;
private Integer timezonestandardhour;
private Integer timezonestandardmonth;
private Integer timezonestandardsecond;
private Integer timezonestandardbias;
private Boolean isduplicatedetectionenabledwhengoingonline;
private String businessunitid;
private Integer paginglimit;
private Integer outlooksyncinterval;
private OffsetDateTime createdon;
private Integer offlinesyncinterval;
private String systemuserid;
private Integer timezonestandardminute;
private Integer timezonedaylightminute;
private String _createdby_value;
private String currencysymbol;
private Integer reportscripterrors;
private Integer currencyformatcode;
private Integer timezonedaylightday;
private Integer nexttrackingnumber;
private Boolean synccontactcompany;
private Integer entityformmode;
private Integer datavalidationmodeforexporttoexcel;
private String decimalsymbol;
private Boolean isguidedhelpenabled;
private Long resourcebookingexchangesyncversion;
private String timeformatstring;
private Boolean showweeknumber;
private Integer timezonestandardyear;
private OffsetDateTime lastalertsviewedtime;
private String pmdesignator;
private Boolean ignoreunsolicitedemail;
private Integer timezonedaylightmonth;
private Integer timeformatcode;
private String _modifiedonbehalfby_value;
private String workdaystarttime;
private String _transactioncurrencyid_value;
private String defaultdashboardid;
private Boolean isappsforcrmalertdismissed;
private Integer addressbooksyncinterval;
private String workdaystoptime;
private Integer timezonedaylightyear;
private Integer incomingemailfilteringmethod;
private OffsetDateTime modifiedon;
private Boolean isautodatacaptureenabled;
private Boolean usecrmformforemail;
private Boolean useimagestrips;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder timezonedaylightsecond(Integer timezonedaylightsecond) {
this.timezonedaylightsecond = timezonedaylightsecond;
this.changedFields = changedFields.add("timezonedaylightsecond");
return this;
}
public Builder numberseparator(String numberseparator) {
this.numberseparator = numberseparator;
this.changedFields = changedFields.add("numberseparator");
return this;
}
public Builder timezonedaylightbias(Integer timezonedaylightbias) {
this.timezonedaylightbias = timezonedaylightbias;
this.changedFields = changedFields.add("timezonedaylightbias");
return this;
}
public Builder homepagelayout(String homepagelayout) {
this.homepagelayout = homepagelayout;
this.changedFields = changedFields.add("homepagelayout");
return this;
}
public Builder negativecurrencyformatcode(Integer negativecurrencyformatcode) {
this.negativecurrencyformatcode = negativecurrencyformatcode;
this.changedFields = changedFields.add("negativecurrencyformatcode");
return this;
}
public Builder defaultsearchexperience(Integer defaultsearchexperience) {
this.defaultsearchexperience = defaultsearchexperience;
this.changedFields = changedFields.add("defaultsearchexperience");
return this;
}
public Builder timeseparator(String timeseparator) {
this.timeseparator = timeseparator;
this.changedFields = changedFields.add("timeseparator");
return this;
}
public Builder longdateformatcode(Integer longdateformatcode) {
this.longdateformatcode = longdateformatcode;
this.changedFields = changedFields.add("longdateformatcode");
return this;
}
public Builder calendartype(Integer calendartype) {
this.calendartype = calendartype;
this.changedFields = changedFields.add("calendartype");
return this;
}
public Builder timezonecode(Integer timezonecode) {
this.timezonecode = timezonecode;
this.changedFields = changedFields.add("timezonecode");
return this;
}
public Builder usecrmformforcontact(Boolean usecrmformforcontact) {
this.usecrmformforcontact = usecrmformforcontact;
this.changedFields = changedFields.add("usecrmformforcontact");
return this;
}
public Builder timezonedaylighthour(Integer timezonedaylighthour) {
this.timezonedaylighthour = timezonedaylighthour;
this.changedFields = changedFields.add("timezonedaylighthour");
return this;
}
public Builder isemailconversationviewenabled(Boolean isemailconversationviewenabled) {
this.isemailconversationviewenabled = isemailconversationviewenabled;
this.changedFields = changedFields.add("isemailconversationviewenabled");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder getstartedpanecontentenabled(Boolean getstartedpanecontentenabled) {
this.getstartedpanecontentenabled = getstartedpanecontentenabled;
this.changedFields = changedFields.add("getstartedpanecontentenabled");
return this;
}
public Builder dateseparator(String dateseparator) {
this.dateseparator = dateseparator;
this.changedFields = changedFields.add("dateseparator");
return this;
}
public Builder uilanguageid(Integer uilanguageid) {
this.uilanguageid = uilanguageid;
this.changedFields = changedFields.add("uilanguageid");
return this;
}
public Builder usecrmformfortask(Boolean usecrmformfortask) {
this.usecrmformfortask = usecrmformfortask;
this.changedFields = changedFields.add("usecrmformfortask");
return this;
}
public Builder autocreatecontactonpromote(Integer autocreatecontactonpromote) {
this.autocreatecontactonpromote = autocreatecontactonpromote;
this.changedFields = changedFields.add("autocreatecontactonpromote");
return this;
}
public Builder amdesignator(String amdesignator) {
this.amdesignator = amdesignator;
this.changedFields = changedFields.add("amdesignator");
return this;
}
public Builder timezonestandarddayofweek(Integer timezonestandarddayofweek) {
this.timezonestandarddayofweek = timezonestandarddayofweek;
this.changedFields = changedFields.add("timezonestandarddayofweek");
return this;
}
public Builder timezonebias(Integer timezonebias) {
this.timezonebias = timezonebias;
this.changedFields = changedFields.add("timezonebias");
return this;
}
public Builder defaultcalendarview(Integer defaultcalendarview) {
this.defaultcalendarview = defaultcalendarview;
this.changedFields = changedFields.add("defaultcalendarview");
return this;
}
public Builder advancedfindstartupmode(Integer advancedfindstartupmode) {
this.advancedfindstartupmode = advancedfindstartupmode;
this.changedFields = changedFields.add("advancedfindstartupmode");
return this;
}
public Builder visualizationpanelayout(Integer visualizationpanelayout) {
this.visualizationpanelayout = visualizationpanelayout;
this.changedFields = changedFields.add("visualizationpanelayout");
return this;
}
public Builder defaultcountrycode(String defaultcountrycode) {
this.defaultcountrycode = defaultcountrycode;
this.changedFields = changedFields.add("defaultcountrycode");
return this;
}
public Builder negativeformatcode(Integer negativeformatcode) {
this.negativeformatcode = negativeformatcode;
this.changedFields = changedFields.add("negativeformatcode");
return this;
}
public Builder isresourcebookingexchangesyncenabled(Boolean isresourcebookingexchangesyncenabled) {
this.isresourcebookingexchangesyncenabled = isresourcebookingexchangesyncenabled;
this.changedFields = changedFields.add("isresourcebookingexchangesyncenabled");
return this;
}
public Builder localeid(Integer localeid) {
this.localeid = localeid;
this.changedFields = changedFields.add("localeid");
return this;
}
public Builder autocaptureuserstatus(Integer autocaptureuserstatus) {
this.autocaptureuserstatus = autocaptureuserstatus;
this.changedFields = changedFields.add("autocaptureuserstatus");
return this;
}
public Builder numbergroupformat(String numbergroupformat) {
this.numbergroupformat = numbergroupformat;
this.changedFields = changedFields.add("numbergroupformat");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder userprofile(String userprofile) {
this.userprofile = userprofile;
this.changedFields = changedFields.add("userprofile");
return this;
}
public Builder splitviewstate(Boolean splitviewstate) {
this.splitviewstate = splitviewstate;
this.changedFields = changedFields.add("splitviewstate");
return this;
}
public Builder homepagesubarea(String homepagesubarea) {
this.homepagesubarea = homepagesubarea;
this.changedFields = changedFields.add("homepagesubarea");
return this;
}
public Builder helplanguageid(Integer helplanguageid) {
this.helplanguageid = helplanguageid;
this.changedFields = changedFields.add("helplanguageid");
return this;
}
public Builder homepagearea(String homepagearea) {
this.homepagearea = homepagearea;
this.changedFields = changedFields.add("homepagearea");
return this;
}
public Builder selectedglobalfilterid(String selectedglobalfilterid) {
this.selectedglobalfilterid = selectedglobalfilterid;
this.changedFields = changedFields.add("selectedglobalfilterid");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder personalizationsettings(String personalizationsettings) {
this.personalizationsettings = personalizationsettings;
this.changedFields = changedFields.add("personalizationsettings");
return this;
}
public Builder dateformatstring(String dateformatstring) {
this.dateformatstring = dateformatstring;
this.changedFields = changedFields.add("dateformatstring");
return this;
}
public Builder trackingtokenid(Integer trackingtokenid) {
this.trackingtokenid = trackingtokenid;
this.changedFields = changedFields.add("trackingtokenid");
return this;
}
public Builder issendasallowed(Boolean issendasallowed) {
this.issendasallowed = issendasallowed;
this.changedFields = changedFields.add("issendasallowed");
return this;
}
public Builder isdefaultcountrycodecheckenabled(Boolean isdefaultcountrycodecheckenabled) {
this.isdefaultcountrycodecheckenabled = isdefaultcountrycodecheckenabled;
this.changedFields = changedFields.add("isdefaultcountrycodecheckenabled");
return this;
}
public Builder timezonedaylightdayofweek(Integer timezonedaylightdayofweek) {
this.timezonedaylightdayofweek = timezonedaylightdayofweek;
this.changedFields = changedFields.add("timezonedaylightdayofweek");
return this;
}
public Builder fullnameconventioncode(Integer fullnameconventioncode) {
this.fullnameconventioncode = fullnameconventioncode;
this.changedFields = changedFields.add("fullnameconventioncode");
return this;
}
public Builder timezonestandardday(Integer timezonestandardday) {
this.timezonestandardday = timezonestandardday;
this.changedFields = changedFields.add("timezonestandardday");
return this;
}
public Builder usecrmformforappointment(Boolean usecrmformforappointment) {
this.usecrmformforappointment = usecrmformforappointment;
this.changedFields = changedFields.add("usecrmformforappointment");
return this;
}
public Builder dateformatcode(Integer dateformatcode) {
this.dateformatcode = dateformatcode;
this.changedFields = changedFields.add("dateformatcode");
return this;
}
public Builder timezonestandardhour(Integer timezonestandardhour) {
this.timezonestandardhour = timezonestandardhour;
this.changedFields = changedFields.add("timezonestandardhour");
return this;
}
public Builder timezonestandardmonth(Integer timezonestandardmonth) {
this.timezonestandardmonth = timezonestandardmonth;
this.changedFields = changedFields.add("timezonestandardmonth");
return this;
}
public Builder timezonestandardsecond(Integer timezonestandardsecond) {
this.timezonestandardsecond = timezonestandardsecond;
this.changedFields = changedFields.add("timezonestandardsecond");
return this;
}
public Builder timezonestandardbias(Integer timezonestandardbias) {
this.timezonestandardbias = timezonestandardbias;
this.changedFields = changedFields.add("timezonestandardbias");
return this;
}
public Builder isduplicatedetectionenabledwhengoingonline(Boolean isduplicatedetectionenabledwhengoingonline) {
this.isduplicatedetectionenabledwhengoingonline = isduplicatedetectionenabledwhengoingonline;
this.changedFields = changedFields.add("isduplicatedetectionenabledwhengoingonline");
return this;
}
public Builder businessunitid(String businessunitid) {
this.businessunitid = businessunitid;
this.changedFields = changedFields.add("businessunitid");
return this;
}
public Builder paginglimit(Integer paginglimit) {
this.paginglimit = paginglimit;
this.changedFields = changedFields.add("paginglimit");
return this;
}
public Builder outlooksyncinterval(Integer outlooksyncinterval) {
this.outlooksyncinterval = outlooksyncinterval;
this.changedFields = changedFields.add("outlooksyncinterval");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder offlinesyncinterval(Integer offlinesyncinterval) {
this.offlinesyncinterval = offlinesyncinterval;
this.changedFields = changedFields.add("offlinesyncinterval");
return this;
}
public Builder systemuserid(String systemuserid) {
this.systemuserid = systemuserid;
this.changedFields = changedFields.add("systemuserid");
return this;
}
public Builder timezonestandardminute(Integer timezonestandardminute) {
this.timezonestandardminute = timezonestandardminute;
this.changedFields = changedFields.add("timezonestandardminute");
return this;
}
public Builder timezonedaylightminute(Integer timezonedaylightminute) {
this.timezonedaylightminute = timezonedaylightminute;
this.changedFields = changedFields.add("timezonedaylightminute");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder currencysymbol(String currencysymbol) {
this.currencysymbol = currencysymbol;
this.changedFields = changedFields.add("currencysymbol");
return this;
}
public Builder reportscripterrors(Integer reportscripterrors) {
this.reportscripterrors = reportscripterrors;
this.changedFields = changedFields.add("reportscripterrors");
return this;
}
public Builder currencyformatcode(Integer currencyformatcode) {
this.currencyformatcode = currencyformatcode;
this.changedFields = changedFields.add("currencyformatcode");
return this;
}
public Builder timezonedaylightday(Integer timezonedaylightday) {
this.timezonedaylightday = timezonedaylightday;
this.changedFields = changedFields.add("timezonedaylightday");
return this;
}
public Builder nexttrackingnumber(Integer nexttrackingnumber) {
this.nexttrackingnumber = nexttrackingnumber;
this.changedFields = changedFields.add("nexttrackingnumber");
return this;
}
public Builder synccontactcompany(Boolean synccontactcompany) {
this.synccontactcompany = synccontactcompany;
this.changedFields = changedFields.add("synccontactcompany");
return this;
}
public Builder entityformmode(Integer entityformmode) {
this.entityformmode = entityformmode;
this.changedFields = changedFields.add("entityformmode");
return this;
}
public Builder datavalidationmodeforexporttoexcel(Integer datavalidationmodeforexporttoexcel) {
this.datavalidationmodeforexporttoexcel = datavalidationmodeforexporttoexcel;
this.changedFields = changedFields.add("datavalidationmodeforexporttoexcel");
return this;
}
public Builder decimalsymbol(String decimalsymbol) {
this.decimalsymbol = decimalsymbol;
this.changedFields = changedFields.add("decimalsymbol");
return this;
}
public Builder isguidedhelpenabled(Boolean isguidedhelpenabled) {
this.isguidedhelpenabled = isguidedhelpenabled;
this.changedFields = changedFields.add("isguidedhelpenabled");
return this;
}
public Builder resourcebookingexchangesyncversion(Long resourcebookingexchangesyncversion) {
this.resourcebookingexchangesyncversion = resourcebookingexchangesyncversion;
this.changedFields = changedFields.add("resourcebookingexchangesyncversion");
return this;
}
public Builder timeformatstring(String timeformatstring) {
this.timeformatstring = timeformatstring;
this.changedFields = changedFields.add("timeformatstring");
return this;
}
public Builder showweeknumber(Boolean showweeknumber) {
this.showweeknumber = showweeknumber;
this.changedFields = changedFields.add("showweeknumber");
return this;
}
public Builder timezonestandardyear(Integer timezonestandardyear) {
this.timezonestandardyear = timezonestandardyear;
this.changedFields = changedFields.add("timezonestandardyear");
return this;
}
public Builder lastalertsviewedtime(OffsetDateTime lastalertsviewedtime) {
this.lastalertsviewedtime = lastalertsviewedtime;
this.changedFields = changedFields.add("lastalertsviewedtime");
return this;
}
public Builder pmdesignator(String pmdesignator) {
this.pmdesignator = pmdesignator;
this.changedFields = changedFields.add("pmdesignator");
return this;
}
public Builder ignoreunsolicitedemail(Boolean ignoreunsolicitedemail) {
this.ignoreunsolicitedemail = ignoreunsolicitedemail;
this.changedFields = changedFields.add("ignoreunsolicitedemail");
return this;
}
public Builder timezonedaylightmonth(Integer timezonedaylightmonth) {
this.timezonedaylightmonth = timezonedaylightmonth;
this.changedFields = changedFields.add("timezonedaylightmonth");
return this;
}
public Builder timeformatcode(Integer timeformatcode) {
this.timeformatcode = timeformatcode;
this.changedFields = changedFields.add("timeformatcode");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder workdaystarttime(String workdaystarttime) {
this.workdaystarttime = workdaystarttime;
this.changedFields = changedFields.add("workdaystarttime");
return this;
}
public Builder _transactioncurrencyid_value(String _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder defaultdashboardid(String defaultdashboardid) {
this.defaultdashboardid = defaultdashboardid;
this.changedFields = changedFields.add("defaultdashboardid");
return this;
}
public Builder isappsforcrmalertdismissed(Boolean isappsforcrmalertdismissed) {
this.isappsforcrmalertdismissed = isappsforcrmalertdismissed;
this.changedFields = changedFields.add("isappsforcrmalertdismissed");
return this;
}
public Builder addressbooksyncinterval(Integer addressbooksyncinterval) {
this.addressbooksyncinterval = addressbooksyncinterval;
this.changedFields = changedFields.add("addressbooksyncinterval");
return this;
}
public Builder workdaystoptime(String workdaystoptime) {
this.workdaystoptime = workdaystoptime;
this.changedFields = changedFields.add("workdaystoptime");
return this;
}
public Builder timezonedaylightyear(Integer timezonedaylightyear) {
this.timezonedaylightyear = timezonedaylightyear;
this.changedFields = changedFields.add("timezonedaylightyear");
return this;
}
public Builder incomingemailfilteringmethod(Integer incomingemailfilteringmethod) {
this.incomingemailfilteringmethod = incomingemailfilteringmethod;
this.changedFields = changedFields.add("incomingemailfilteringmethod");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder isautodatacaptureenabled(Boolean isautodatacaptureenabled) {
this.isautodatacaptureenabled = isautodatacaptureenabled;
this.changedFields = changedFields.add("isautodatacaptureenabled");
return this;
}
public Builder usecrmformforemail(Boolean usecrmformforemail) {
this.usecrmformforemail = usecrmformforemail;
this.changedFields = changedFields.add("usecrmformforemail");
return this;
}
public Builder useimagestrips(Boolean useimagestrips) {
this.useimagestrips = useimagestrips;
this.changedFields = changedFields.add("useimagestrips");
return this;
}
public Usersettings build() {
Usersettings _x = new Usersettings();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.usersettings";
_x.timezonedaylightsecond = timezonedaylightsecond;
_x.numberseparator = numberseparator;
_x.timezonedaylightbias = timezonedaylightbias;
_x.homepagelayout = homepagelayout;
_x.negativecurrencyformatcode = negativecurrencyformatcode;
_x.defaultsearchexperience = defaultsearchexperience;
_x.timeseparator = timeseparator;
_x.longdateformatcode = longdateformatcode;
_x.calendartype = calendartype;
_x.timezonecode = timezonecode;
_x.usecrmformforcontact = usecrmformforcontact;
_x.timezonedaylighthour = timezonedaylighthour;
_x.isemailconversationviewenabled = isemailconversationviewenabled;
_x.versionnumber = versionnumber;
_x.getstartedpanecontentenabled = getstartedpanecontentenabled;
_x.dateseparator = dateseparator;
_x.uilanguageid = uilanguageid;
_x.usecrmformfortask = usecrmformfortask;
_x.autocreatecontactonpromote = autocreatecontactonpromote;
_x.amdesignator = amdesignator;
_x.timezonestandarddayofweek = timezonestandarddayofweek;
_x.timezonebias = timezonebias;
_x.defaultcalendarview = defaultcalendarview;
_x.advancedfindstartupmode = advancedfindstartupmode;
_x.visualizationpanelayout = visualizationpanelayout;
_x.defaultcountrycode = defaultcountrycode;
_x.negativeformatcode = negativeformatcode;
_x.isresourcebookingexchangesyncenabled = isresourcebookingexchangesyncenabled;
_x.localeid = localeid;
_x.autocaptureuserstatus = autocaptureuserstatus;
_x.numbergroupformat = numbergroupformat;
_x._modifiedby_value = _modifiedby_value;
_x.userprofile = userprofile;
_x.splitviewstate = splitviewstate;
_x.homepagesubarea = homepagesubarea;
_x.helplanguageid = helplanguageid;
_x.homepagearea = homepagearea;
_x.selectedglobalfilterid = selectedglobalfilterid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.personalizationsettings = personalizationsettings;
_x.dateformatstring = dateformatstring;
_x.trackingtokenid = trackingtokenid;
_x.issendasallowed = issendasallowed;
_x.isdefaultcountrycodecheckenabled = isdefaultcountrycodecheckenabled;
_x.timezonedaylightdayofweek = timezonedaylightdayofweek;
_x.fullnameconventioncode = fullnameconventioncode;
_x.timezonestandardday = timezonestandardday;
_x.usecrmformforappointment = usecrmformforappointment;
_x.dateformatcode = dateformatcode;
_x.timezonestandardhour = timezonestandardhour;
_x.timezonestandardmonth = timezonestandardmonth;
_x.timezonestandardsecond = timezonestandardsecond;
_x.timezonestandardbias = timezonestandardbias;
_x.isduplicatedetectionenabledwhengoingonline = isduplicatedetectionenabledwhengoingonline;
_x.businessunitid = businessunitid;
_x.paginglimit = paginglimit;
_x.outlooksyncinterval = outlooksyncinterval;
_x.createdon = createdon;
_x.offlinesyncinterval = offlinesyncinterval;
_x.systemuserid = systemuserid;
_x.timezonestandardminute = timezonestandardminute;
_x.timezonedaylightminute = timezonedaylightminute;
_x._createdby_value = _createdby_value;
_x.currencysymbol = currencysymbol;
_x.reportscripterrors = reportscripterrors;
_x.currencyformatcode = currencyformatcode;
_x.timezonedaylightday = timezonedaylightday;
_x.nexttrackingnumber = nexttrackingnumber;
_x.synccontactcompany = synccontactcompany;
_x.entityformmode = entityformmode;
_x.datavalidationmodeforexporttoexcel = datavalidationmodeforexporttoexcel;
_x.decimalsymbol = decimalsymbol;
_x.isguidedhelpenabled = isguidedhelpenabled;
_x.resourcebookingexchangesyncversion = resourcebookingexchangesyncversion;
_x.timeformatstring = timeformatstring;
_x.showweeknumber = showweeknumber;
_x.timezonestandardyear = timezonestandardyear;
_x.lastalertsviewedtime = lastalertsviewedtime;
_x.pmdesignator = pmdesignator;
_x.ignoreunsolicitedemail = ignoreunsolicitedemail;
_x.timezonedaylightmonth = timezonedaylightmonth;
_x.timeformatcode = timeformatcode;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.workdaystarttime = workdaystarttime;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.defaultdashboardid = defaultdashboardid;
_x.isappsforcrmalertdismissed = isappsforcrmalertdismissed;
_x.addressbooksyncinterval = addressbooksyncinterval;
_x.workdaystoptime = workdaystoptime;
_x.timezonedaylightyear = timezonedaylightyear;
_x.incomingemailfilteringmethod = incomingemailfilteringmethod;
_x.modifiedon = modifiedon;
_x.isautodatacaptureenabled = isautodatacaptureenabled;
_x.usecrmformforemail = usecrmformforemail;
_x.useimagestrips = useimagestrips;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && systemuserid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(systemuserid.toString()));
}
}
@Property(name="timezonedaylightsecond")
@JsonIgnore
public Optional getTimezonedaylightsecond() {
return Optional.ofNullable(timezonedaylightsecond);
}
public Usersettings withTimezonedaylightsecond(Integer timezonedaylightsecond) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightsecond");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightsecond = timezonedaylightsecond;
return _x;
}
@Property(name="numberseparator")
@JsonIgnore
public Optional getNumberseparator() {
return Optional.ofNullable(numberseparator);
}
public Usersettings withNumberseparator(String numberseparator) {
Checks.checkIsAscii(numberseparator);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("numberseparator");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.numberseparator = numberseparator;
return _x;
}
@Property(name="timezonedaylightbias")
@JsonIgnore
public Optional getTimezonedaylightbias() {
return Optional.ofNullable(timezonedaylightbias);
}
public Usersettings withTimezonedaylightbias(Integer timezonedaylightbias) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightbias");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightbias = timezonedaylightbias;
return _x;
}
@Property(name="homepagelayout")
@JsonIgnore
public Optional getHomepagelayout() {
return Optional.ofNullable(homepagelayout);
}
public Usersettings withHomepagelayout(String homepagelayout) {
Checks.checkIsAscii(homepagelayout);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("homepagelayout");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.homepagelayout = homepagelayout;
return _x;
}
@Property(name="negativecurrencyformatcode")
@JsonIgnore
public Optional getNegativecurrencyformatcode() {
return Optional.ofNullable(negativecurrencyformatcode);
}
public Usersettings withNegativecurrencyformatcode(Integer negativecurrencyformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("negativecurrencyformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.negativecurrencyformatcode = negativecurrencyformatcode;
return _x;
}
@Property(name="defaultsearchexperience")
@JsonIgnore
public Optional getDefaultsearchexperience() {
return Optional.ofNullable(defaultsearchexperience);
}
public Usersettings withDefaultsearchexperience(Integer defaultsearchexperience) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("defaultsearchexperience");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.defaultsearchexperience = defaultsearchexperience;
return _x;
}
@Property(name="timeseparator")
@JsonIgnore
public Optional getTimeseparator() {
return Optional.ofNullable(timeseparator);
}
public Usersettings withTimeseparator(String timeseparator) {
Checks.checkIsAscii(timeseparator);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timeseparator");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timeseparator = timeseparator;
return _x;
}
@Property(name="longdateformatcode")
@JsonIgnore
public Optional getLongdateformatcode() {
return Optional.ofNullable(longdateformatcode);
}
public Usersettings withLongdateformatcode(Integer longdateformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("longdateformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.longdateformatcode = longdateformatcode;
return _x;
}
@Property(name="calendartype")
@JsonIgnore
public Optional getCalendartype() {
return Optional.ofNullable(calendartype);
}
public Usersettings withCalendartype(Integer calendartype) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("calendartype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.calendartype = calendartype;
return _x;
}
@Property(name="timezonecode")
@JsonIgnore
public Optional getTimezonecode() {
return Optional.ofNullable(timezonecode);
}
public Usersettings withTimezonecode(Integer timezonecode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonecode = timezonecode;
return _x;
}
@Property(name="usecrmformforcontact")
@JsonIgnore
public Optional getUsecrmformforcontact() {
return Optional.ofNullable(usecrmformforcontact);
}
public Usersettings withUsecrmformforcontact(Boolean usecrmformforcontact) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("usecrmformforcontact");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.usecrmformforcontact = usecrmformforcontact;
return _x;
}
@Property(name="timezonedaylighthour")
@JsonIgnore
public Optional getTimezonedaylighthour() {
return Optional.ofNullable(timezonedaylighthour);
}
public Usersettings withTimezonedaylighthour(Integer timezonedaylighthour) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylighthour");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylighthour = timezonedaylighthour;
return _x;
}
@Property(name="isemailconversationviewenabled")
@JsonIgnore
public Optional getIsemailconversationviewenabled() {
return Optional.ofNullable(isemailconversationviewenabled);
}
public Usersettings withIsemailconversationviewenabled(Boolean isemailconversationviewenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isemailconversationviewenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isemailconversationviewenabled = isemailconversationviewenabled;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Usersettings withVersionnumber(Long versionnumber) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="getstartedpanecontentenabled")
@JsonIgnore
public Optional getGetstartedpanecontentenabled() {
return Optional.ofNullable(getstartedpanecontentenabled);
}
public Usersettings withGetstartedpanecontentenabled(Boolean getstartedpanecontentenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("getstartedpanecontentenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.getstartedpanecontentenabled = getstartedpanecontentenabled;
return _x;
}
@Property(name="dateseparator")
@JsonIgnore
public Optional getDateseparator() {
return Optional.ofNullable(dateseparator);
}
public Usersettings withDateseparator(String dateseparator) {
Checks.checkIsAscii(dateseparator);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("dateseparator");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.dateseparator = dateseparator;
return _x;
}
@Property(name="uilanguageid")
@JsonIgnore
public Optional getUilanguageid() {
return Optional.ofNullable(uilanguageid);
}
public Usersettings withUilanguageid(Integer uilanguageid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("uilanguageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.uilanguageid = uilanguageid;
return _x;
}
@Property(name="usecrmformfortask")
@JsonIgnore
public Optional getUsecrmformfortask() {
return Optional.ofNullable(usecrmformfortask);
}
public Usersettings withUsecrmformfortask(Boolean usecrmformfortask) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("usecrmformfortask");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.usecrmformfortask = usecrmformfortask;
return _x;
}
@Property(name="autocreatecontactonpromote")
@JsonIgnore
public Optional getAutocreatecontactonpromote() {
return Optional.ofNullable(autocreatecontactonpromote);
}
public Usersettings withAutocreatecontactonpromote(Integer autocreatecontactonpromote) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("autocreatecontactonpromote");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.autocreatecontactonpromote = autocreatecontactonpromote;
return _x;
}
@Property(name="amdesignator")
@JsonIgnore
public Optional getAmdesignator() {
return Optional.ofNullable(amdesignator);
}
public Usersettings withAmdesignator(String amdesignator) {
Checks.checkIsAscii(amdesignator);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("amdesignator");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.amdesignator = amdesignator;
return _x;
}
@Property(name="timezonestandarddayofweek")
@JsonIgnore
public Optional getTimezonestandarddayofweek() {
return Optional.ofNullable(timezonestandarddayofweek);
}
public Usersettings withTimezonestandarddayofweek(Integer timezonestandarddayofweek) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandarddayofweek");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandarddayofweek = timezonestandarddayofweek;
return _x;
}
@Property(name="timezonebias")
@JsonIgnore
public Optional getTimezonebias() {
return Optional.ofNullable(timezonebias);
}
public Usersettings withTimezonebias(Integer timezonebias) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonebias");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonebias = timezonebias;
return _x;
}
@Property(name="defaultcalendarview")
@JsonIgnore
public Optional getDefaultcalendarview() {
return Optional.ofNullable(defaultcalendarview);
}
public Usersettings withDefaultcalendarview(Integer defaultcalendarview) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("defaultcalendarview");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.defaultcalendarview = defaultcalendarview;
return _x;
}
@Property(name="advancedfindstartupmode")
@JsonIgnore
public Optional getAdvancedfindstartupmode() {
return Optional.ofNullable(advancedfindstartupmode);
}
public Usersettings withAdvancedfindstartupmode(Integer advancedfindstartupmode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("advancedfindstartupmode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.advancedfindstartupmode = advancedfindstartupmode;
return _x;
}
@Property(name="visualizationpanelayout")
@JsonIgnore
public Optional getVisualizationpanelayout() {
return Optional.ofNullable(visualizationpanelayout);
}
public Usersettings withVisualizationpanelayout(Integer visualizationpanelayout) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("visualizationpanelayout");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.visualizationpanelayout = visualizationpanelayout;
return _x;
}
@Property(name="defaultcountrycode")
@JsonIgnore
public Optional getDefaultcountrycode() {
return Optional.ofNullable(defaultcountrycode);
}
public Usersettings withDefaultcountrycode(String defaultcountrycode) {
Checks.checkIsAscii(defaultcountrycode);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("defaultcountrycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.defaultcountrycode = defaultcountrycode;
return _x;
}
@Property(name="negativeformatcode")
@JsonIgnore
public Optional getNegativeformatcode() {
return Optional.ofNullable(negativeformatcode);
}
public Usersettings withNegativeformatcode(Integer negativeformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("negativeformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.negativeformatcode = negativeformatcode;
return _x;
}
@Property(name="isresourcebookingexchangesyncenabled")
@JsonIgnore
public Optional getIsresourcebookingexchangesyncenabled() {
return Optional.ofNullable(isresourcebookingexchangesyncenabled);
}
public Usersettings withIsresourcebookingexchangesyncenabled(Boolean isresourcebookingexchangesyncenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isresourcebookingexchangesyncenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isresourcebookingexchangesyncenabled = isresourcebookingexchangesyncenabled;
return _x;
}
@Property(name="localeid")
@JsonIgnore
public Optional getLocaleid() {
return Optional.ofNullable(localeid);
}
public Usersettings withLocaleid(Integer localeid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("localeid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.localeid = localeid;
return _x;
}
@Property(name="autocaptureuserstatus")
@JsonIgnore
public Optional getAutocaptureuserstatus() {
return Optional.ofNullable(autocaptureuserstatus);
}
public Usersettings withAutocaptureuserstatus(Integer autocaptureuserstatus) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("autocaptureuserstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.autocaptureuserstatus = autocaptureuserstatus;
return _x;
}
@Property(name="numbergroupformat")
@JsonIgnore
public Optional getNumbergroupformat() {
return Optional.ofNullable(numbergroupformat);
}
public Usersettings withNumbergroupformat(String numbergroupformat) {
Checks.checkIsAscii(numbergroupformat);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("numbergroupformat");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.numbergroupformat = numbergroupformat;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Usersettings with_modifiedby_value(String _modifiedby_value) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="userprofile")
@JsonIgnore
public Optional getUserprofile() {
return Optional.ofNullable(userprofile);
}
public Usersettings withUserprofile(String userprofile) {
Checks.checkIsAscii(userprofile);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("userprofile");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.userprofile = userprofile;
return _x;
}
@Property(name="splitviewstate")
@JsonIgnore
public Optional getSplitviewstate() {
return Optional.ofNullable(splitviewstate);
}
public Usersettings withSplitviewstate(Boolean splitviewstate) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("splitviewstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.splitviewstate = splitviewstate;
return _x;
}
@Property(name="homepagesubarea")
@JsonIgnore
public Optional getHomepagesubarea() {
return Optional.ofNullable(homepagesubarea);
}
public Usersettings withHomepagesubarea(String homepagesubarea) {
Checks.checkIsAscii(homepagesubarea);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("homepagesubarea");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.homepagesubarea = homepagesubarea;
return _x;
}
@Property(name="helplanguageid")
@JsonIgnore
public Optional getHelplanguageid() {
return Optional.ofNullable(helplanguageid);
}
public Usersettings withHelplanguageid(Integer helplanguageid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("helplanguageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.helplanguageid = helplanguageid;
return _x;
}
@Property(name="homepagearea")
@JsonIgnore
public Optional getHomepagearea() {
return Optional.ofNullable(homepagearea);
}
public Usersettings withHomepagearea(String homepagearea) {
Checks.checkIsAscii(homepagearea);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("homepagearea");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.homepagearea = homepagearea;
return _x;
}
@Property(name="selectedglobalfilterid")
@JsonIgnore
public Optional getSelectedglobalfilterid() {
return Optional.ofNullable(selectedglobalfilterid);
}
public Usersettings withSelectedglobalfilterid(String selectedglobalfilterid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("selectedglobalfilterid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.selectedglobalfilterid = selectedglobalfilterid;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Usersettings with_createdonbehalfby_value(String _createdonbehalfby_value) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="personalizationsettings")
@JsonIgnore
public Optional getPersonalizationsettings() {
return Optional.ofNullable(personalizationsettings);
}
public Usersettings withPersonalizationsettings(String personalizationsettings) {
Checks.checkIsAscii(personalizationsettings);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("personalizationsettings");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.personalizationsettings = personalizationsettings;
return _x;
}
@Property(name="dateformatstring")
@JsonIgnore
public Optional getDateformatstring() {
return Optional.ofNullable(dateformatstring);
}
public Usersettings withDateformatstring(String dateformatstring) {
Checks.checkIsAscii(dateformatstring);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("dateformatstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.dateformatstring = dateformatstring;
return _x;
}
@Property(name="trackingtokenid")
@JsonIgnore
public Optional getTrackingtokenid() {
return Optional.ofNullable(trackingtokenid);
}
public Usersettings withTrackingtokenid(Integer trackingtokenid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("trackingtokenid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.trackingtokenid = trackingtokenid;
return _x;
}
@Property(name="issendasallowed")
@JsonIgnore
public Optional getIssendasallowed() {
return Optional.ofNullable(issendasallowed);
}
public Usersettings withIssendasallowed(Boolean issendasallowed) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("issendasallowed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.issendasallowed = issendasallowed;
return _x;
}
@Property(name="isdefaultcountrycodecheckenabled")
@JsonIgnore
public Optional getIsdefaultcountrycodecheckenabled() {
return Optional.ofNullable(isdefaultcountrycodecheckenabled);
}
public Usersettings withIsdefaultcountrycodecheckenabled(Boolean isdefaultcountrycodecheckenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isdefaultcountrycodecheckenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isdefaultcountrycodecheckenabled = isdefaultcountrycodecheckenabled;
return _x;
}
@Property(name="timezonedaylightdayofweek")
@JsonIgnore
public Optional getTimezonedaylightdayofweek() {
return Optional.ofNullable(timezonedaylightdayofweek);
}
public Usersettings withTimezonedaylightdayofweek(Integer timezonedaylightdayofweek) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightdayofweek");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightdayofweek = timezonedaylightdayofweek;
return _x;
}
@Property(name="fullnameconventioncode")
@JsonIgnore
public Optional getFullnameconventioncode() {
return Optional.ofNullable(fullnameconventioncode);
}
public Usersettings withFullnameconventioncode(Integer fullnameconventioncode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("fullnameconventioncode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.fullnameconventioncode = fullnameconventioncode;
return _x;
}
@Property(name="timezonestandardday")
@JsonIgnore
public Optional getTimezonestandardday() {
return Optional.ofNullable(timezonestandardday);
}
public Usersettings withTimezonestandardday(Integer timezonestandardday) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardday");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardday = timezonestandardday;
return _x;
}
@Property(name="usecrmformforappointment")
@JsonIgnore
public Optional getUsecrmformforappointment() {
return Optional.ofNullable(usecrmformforappointment);
}
public Usersettings withUsecrmformforappointment(Boolean usecrmformforappointment) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("usecrmformforappointment");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.usecrmformforappointment = usecrmformforappointment;
return _x;
}
@Property(name="dateformatcode")
@JsonIgnore
public Optional getDateformatcode() {
return Optional.ofNullable(dateformatcode);
}
public Usersettings withDateformatcode(Integer dateformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("dateformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.dateformatcode = dateformatcode;
return _x;
}
@Property(name="timezonestandardhour")
@JsonIgnore
public Optional getTimezonestandardhour() {
return Optional.ofNullable(timezonestandardhour);
}
public Usersettings withTimezonestandardhour(Integer timezonestandardhour) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardhour");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardhour = timezonestandardhour;
return _x;
}
@Property(name="timezonestandardmonth")
@JsonIgnore
public Optional getTimezonestandardmonth() {
return Optional.ofNullable(timezonestandardmonth);
}
public Usersettings withTimezonestandardmonth(Integer timezonestandardmonth) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardmonth");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardmonth = timezonestandardmonth;
return _x;
}
@Property(name="timezonestandardsecond")
@JsonIgnore
public Optional getTimezonestandardsecond() {
return Optional.ofNullable(timezonestandardsecond);
}
public Usersettings withTimezonestandardsecond(Integer timezonestandardsecond) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardsecond");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardsecond = timezonestandardsecond;
return _x;
}
@Property(name="timezonestandardbias")
@JsonIgnore
public Optional getTimezonestandardbias() {
return Optional.ofNullable(timezonestandardbias);
}
public Usersettings withTimezonestandardbias(Integer timezonestandardbias) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardbias");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardbias = timezonestandardbias;
return _x;
}
@Property(name="isduplicatedetectionenabledwhengoingonline")
@JsonIgnore
public Optional getIsduplicatedetectionenabledwhengoingonline() {
return Optional.ofNullable(isduplicatedetectionenabledwhengoingonline);
}
public Usersettings withIsduplicatedetectionenabledwhengoingonline(Boolean isduplicatedetectionenabledwhengoingonline) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isduplicatedetectionenabledwhengoingonline");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isduplicatedetectionenabledwhengoingonline = isduplicatedetectionenabledwhengoingonline;
return _x;
}
@Property(name="businessunitid")
@JsonIgnore
public Optional getBusinessunitid() {
return Optional.ofNullable(businessunitid);
}
public Usersettings withBusinessunitid(String businessunitid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("businessunitid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.businessunitid = businessunitid;
return _x;
}
@Property(name="paginglimit")
@JsonIgnore
public Optional getPaginglimit() {
return Optional.ofNullable(paginglimit);
}
public Usersettings withPaginglimit(Integer paginglimit) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("paginglimit");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.paginglimit = paginglimit;
return _x;
}
@Property(name="outlooksyncinterval")
@JsonIgnore
public Optional getOutlooksyncinterval() {
return Optional.ofNullable(outlooksyncinterval);
}
public Usersettings withOutlooksyncinterval(Integer outlooksyncinterval) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("outlooksyncinterval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.outlooksyncinterval = outlooksyncinterval;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Usersettings withCreatedon(OffsetDateTime createdon) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.createdon = createdon;
return _x;
}
@Property(name="offlinesyncinterval")
@JsonIgnore
public Optional getOfflinesyncinterval() {
return Optional.ofNullable(offlinesyncinterval);
}
public Usersettings withOfflinesyncinterval(Integer offlinesyncinterval) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("offlinesyncinterval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.offlinesyncinterval = offlinesyncinterval;
return _x;
}
@Property(name="systemuserid")
@JsonIgnore
public Optional getSystemuserid() {
return Optional.ofNullable(systemuserid);
}
public Usersettings withSystemuserid(String systemuserid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("systemuserid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.systemuserid = systemuserid;
return _x;
}
@Property(name="timezonestandardminute")
@JsonIgnore
public Optional getTimezonestandardminute() {
return Optional.ofNullable(timezonestandardminute);
}
public Usersettings withTimezonestandardminute(Integer timezonestandardminute) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardminute");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardminute = timezonestandardminute;
return _x;
}
@Property(name="timezonedaylightminute")
@JsonIgnore
public Optional getTimezonedaylightminute() {
return Optional.ofNullable(timezonedaylightminute);
}
public Usersettings withTimezonedaylightminute(Integer timezonedaylightminute) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightminute");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightminute = timezonedaylightminute;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Usersettings with_createdby_value(String _createdby_value) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="currencysymbol")
@JsonIgnore
public Optional getCurrencysymbol() {
return Optional.ofNullable(currencysymbol);
}
public Usersettings withCurrencysymbol(String currencysymbol) {
Checks.checkIsAscii(currencysymbol);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("currencysymbol");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.currencysymbol = currencysymbol;
return _x;
}
@Property(name="reportscripterrors")
@JsonIgnore
public Optional getReportscripterrors() {
return Optional.ofNullable(reportscripterrors);
}
public Usersettings withReportscripterrors(Integer reportscripterrors) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("reportscripterrors");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.reportscripterrors = reportscripterrors;
return _x;
}
@Property(name="currencyformatcode")
@JsonIgnore
public Optional getCurrencyformatcode() {
return Optional.ofNullable(currencyformatcode);
}
public Usersettings withCurrencyformatcode(Integer currencyformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("currencyformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.currencyformatcode = currencyformatcode;
return _x;
}
@Property(name="timezonedaylightday")
@JsonIgnore
public Optional getTimezonedaylightday() {
return Optional.ofNullable(timezonedaylightday);
}
public Usersettings withTimezonedaylightday(Integer timezonedaylightday) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightday");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightday = timezonedaylightday;
return _x;
}
@Property(name="nexttrackingnumber")
@JsonIgnore
public Optional getNexttrackingnumber() {
return Optional.ofNullable(nexttrackingnumber);
}
public Usersettings withNexttrackingnumber(Integer nexttrackingnumber) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("nexttrackingnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.nexttrackingnumber = nexttrackingnumber;
return _x;
}
@Property(name="synccontactcompany")
@JsonIgnore
public Optional getSynccontactcompany() {
return Optional.ofNullable(synccontactcompany);
}
public Usersettings withSynccontactcompany(Boolean synccontactcompany) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("synccontactcompany");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.synccontactcompany = synccontactcompany;
return _x;
}
@Property(name="entityformmode")
@JsonIgnore
public Optional getEntityformmode() {
return Optional.ofNullable(entityformmode);
}
public Usersettings withEntityformmode(Integer entityformmode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("entityformmode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.entityformmode = entityformmode;
return _x;
}
@Property(name="datavalidationmodeforexporttoexcel")
@JsonIgnore
public Optional getDatavalidationmodeforexporttoexcel() {
return Optional.ofNullable(datavalidationmodeforexporttoexcel);
}
public Usersettings withDatavalidationmodeforexporttoexcel(Integer datavalidationmodeforexporttoexcel) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("datavalidationmodeforexporttoexcel");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.datavalidationmodeforexporttoexcel = datavalidationmodeforexporttoexcel;
return _x;
}
@Property(name="decimalsymbol")
@JsonIgnore
public Optional getDecimalsymbol() {
return Optional.ofNullable(decimalsymbol);
}
public Usersettings withDecimalsymbol(String decimalsymbol) {
Checks.checkIsAscii(decimalsymbol);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("decimalsymbol");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.decimalsymbol = decimalsymbol;
return _x;
}
@Property(name="isguidedhelpenabled")
@JsonIgnore
public Optional getIsguidedhelpenabled() {
return Optional.ofNullable(isguidedhelpenabled);
}
public Usersettings withIsguidedhelpenabled(Boolean isguidedhelpenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isguidedhelpenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isguidedhelpenabled = isguidedhelpenabled;
return _x;
}
@Property(name="resourcebookingexchangesyncversion")
@JsonIgnore
public Optional getResourcebookingexchangesyncversion() {
return Optional.ofNullable(resourcebookingexchangesyncversion);
}
public Usersettings withResourcebookingexchangesyncversion(Long resourcebookingexchangesyncversion) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("resourcebookingexchangesyncversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.resourcebookingexchangesyncversion = resourcebookingexchangesyncversion;
return _x;
}
@Property(name="timeformatstring")
@JsonIgnore
public Optional getTimeformatstring() {
return Optional.ofNullable(timeformatstring);
}
public Usersettings withTimeformatstring(String timeformatstring) {
Checks.checkIsAscii(timeformatstring);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timeformatstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timeformatstring = timeformatstring;
return _x;
}
@Property(name="showweeknumber")
@JsonIgnore
public Optional getShowweeknumber() {
return Optional.ofNullable(showweeknumber);
}
public Usersettings withShowweeknumber(Boolean showweeknumber) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("showweeknumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.showweeknumber = showweeknumber;
return _x;
}
@Property(name="timezonestandardyear")
@JsonIgnore
public Optional getTimezonestandardyear() {
return Optional.ofNullable(timezonestandardyear);
}
public Usersettings withTimezonestandardyear(Integer timezonestandardyear) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonestandardyear");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonestandardyear = timezonestandardyear;
return _x;
}
@Property(name="lastalertsviewedtime")
@JsonIgnore
public Optional getLastalertsviewedtime() {
return Optional.ofNullable(lastalertsviewedtime);
}
public Usersettings withLastalertsviewedtime(OffsetDateTime lastalertsviewedtime) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("lastalertsviewedtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.lastalertsviewedtime = lastalertsviewedtime;
return _x;
}
@Property(name="pmdesignator")
@JsonIgnore
public Optional getPmdesignator() {
return Optional.ofNullable(pmdesignator);
}
public Usersettings withPmdesignator(String pmdesignator) {
Checks.checkIsAscii(pmdesignator);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("pmdesignator");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.pmdesignator = pmdesignator;
return _x;
}
@Property(name="ignoreunsolicitedemail")
@JsonIgnore
public Optional getIgnoreunsolicitedemail() {
return Optional.ofNullable(ignoreunsolicitedemail);
}
public Usersettings withIgnoreunsolicitedemail(Boolean ignoreunsolicitedemail) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("ignoreunsolicitedemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.ignoreunsolicitedemail = ignoreunsolicitedemail;
return _x;
}
@Property(name="timezonedaylightmonth")
@JsonIgnore
public Optional getTimezonedaylightmonth() {
return Optional.ofNullable(timezonedaylightmonth);
}
public Usersettings withTimezonedaylightmonth(Integer timezonedaylightmonth) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightmonth");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightmonth = timezonedaylightmonth;
return _x;
}
@Property(name="timeformatcode")
@JsonIgnore
public Optional getTimeformatcode() {
return Optional.ofNullable(timeformatcode);
}
public Usersettings withTimeformatcode(Integer timeformatcode) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timeformatcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timeformatcode = timeformatcode;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Usersettings with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="workdaystarttime")
@JsonIgnore
public Optional getWorkdaystarttime() {
return Optional.ofNullable(workdaystarttime);
}
public Usersettings withWorkdaystarttime(String workdaystarttime) {
Checks.checkIsAscii(workdaystarttime);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("workdaystarttime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.workdaystarttime = workdaystarttime;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Usersettings with_transactioncurrencyid_value(String _transactioncurrencyid_value) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="defaultdashboardid")
@JsonIgnore
public Optional getDefaultdashboardid() {
return Optional.ofNullable(defaultdashboardid);
}
public Usersettings withDefaultdashboardid(String defaultdashboardid) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("defaultdashboardid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.defaultdashboardid = defaultdashboardid;
return _x;
}
@Property(name="isappsforcrmalertdismissed")
@JsonIgnore
public Optional getIsappsforcrmalertdismissed() {
return Optional.ofNullable(isappsforcrmalertdismissed);
}
public Usersettings withIsappsforcrmalertdismissed(Boolean isappsforcrmalertdismissed) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isappsforcrmalertdismissed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isappsforcrmalertdismissed = isappsforcrmalertdismissed;
return _x;
}
@Property(name="addressbooksyncinterval")
@JsonIgnore
public Optional getAddressbooksyncinterval() {
return Optional.ofNullable(addressbooksyncinterval);
}
public Usersettings withAddressbooksyncinterval(Integer addressbooksyncinterval) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("addressbooksyncinterval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.addressbooksyncinterval = addressbooksyncinterval;
return _x;
}
@Property(name="workdaystoptime")
@JsonIgnore
public Optional getWorkdaystoptime() {
return Optional.ofNullable(workdaystoptime);
}
public Usersettings withWorkdaystoptime(String workdaystoptime) {
Checks.checkIsAscii(workdaystoptime);
Usersettings _x = _copy();
_x.changedFields = changedFields.add("workdaystoptime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.workdaystoptime = workdaystoptime;
return _x;
}
@Property(name="timezonedaylightyear")
@JsonIgnore
public Optional getTimezonedaylightyear() {
return Optional.ofNullable(timezonedaylightyear);
}
public Usersettings withTimezonedaylightyear(Integer timezonedaylightyear) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("timezonedaylightyear");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.timezonedaylightyear = timezonedaylightyear;
return _x;
}
@Property(name="incomingemailfilteringmethod")
@JsonIgnore
public Optional getIncomingemailfilteringmethod() {
return Optional.ofNullable(incomingemailfilteringmethod);
}
public Usersettings withIncomingemailfilteringmethod(Integer incomingemailfilteringmethod) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("incomingemailfilteringmethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.incomingemailfilteringmethod = incomingemailfilteringmethod;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Usersettings withModifiedon(OffsetDateTime modifiedon) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="isautodatacaptureenabled")
@JsonIgnore
public Optional getIsautodatacaptureenabled() {
return Optional.ofNullable(isautodatacaptureenabled);
}
public Usersettings withIsautodatacaptureenabled(Boolean isautodatacaptureenabled) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("isautodatacaptureenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.isautodatacaptureenabled = isautodatacaptureenabled;
return _x;
}
@Property(name="usecrmformforemail")
@JsonIgnore
public Optional getUsecrmformforemail() {
return Optional.ofNullable(usecrmformforemail);
}
public Usersettings withUsecrmformforemail(Boolean usecrmformforemail) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("usecrmformforemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.usecrmformforemail = usecrmformforemail;
return _x;
}
@Property(name="useimagestrips")
@JsonIgnore
public Optional getUseimagestrips() {
return Optional.ofNullable(useimagestrips);
}
public Usersettings withUseimagestrips(Boolean useimagestrips) {
Usersettings _x = _copy();
_x.changedFields = changedFields.add("useimagestrips");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.usersettings");
_x.useimagestrips = useimagestrips;
return _x;
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="systemuserid_systemuser")
@JsonIgnore
public SystemuserRequest getSystemuserid_systemuser() {
return new SystemuserRequest(contextPath.addSegment("systemuserid_systemuser"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="businessunitid_businessunit")
@JsonIgnore
public BusinessunitRequest getBusinessunitid_businessunit() {
return new BusinessunitRequest(contextPath.addSegment("businessunitid_businessunit"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Usersettings patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Usersettings _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Usersettings put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Usersettings _x = _copy();
_x.changedFields = null;
return _x;
}
private Usersettings _copy() {
Usersettings _x = new Usersettings();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.timezonedaylightsecond = timezonedaylightsecond;
_x.numberseparator = numberseparator;
_x.timezonedaylightbias = timezonedaylightbias;
_x.homepagelayout = homepagelayout;
_x.negativecurrencyformatcode = negativecurrencyformatcode;
_x.defaultsearchexperience = defaultsearchexperience;
_x.timeseparator = timeseparator;
_x.longdateformatcode = longdateformatcode;
_x.calendartype = calendartype;
_x.timezonecode = timezonecode;
_x.usecrmformforcontact = usecrmformforcontact;
_x.timezonedaylighthour = timezonedaylighthour;
_x.isemailconversationviewenabled = isemailconversationviewenabled;
_x.versionnumber = versionnumber;
_x.getstartedpanecontentenabled = getstartedpanecontentenabled;
_x.dateseparator = dateseparator;
_x.uilanguageid = uilanguageid;
_x.usecrmformfortask = usecrmformfortask;
_x.autocreatecontactonpromote = autocreatecontactonpromote;
_x.amdesignator = amdesignator;
_x.timezonestandarddayofweek = timezonestandarddayofweek;
_x.timezonebias = timezonebias;
_x.defaultcalendarview = defaultcalendarview;
_x.advancedfindstartupmode = advancedfindstartupmode;
_x.visualizationpanelayout = visualizationpanelayout;
_x.defaultcountrycode = defaultcountrycode;
_x.negativeformatcode = negativeformatcode;
_x.isresourcebookingexchangesyncenabled = isresourcebookingexchangesyncenabled;
_x.localeid = localeid;
_x.autocaptureuserstatus = autocaptureuserstatus;
_x.numbergroupformat = numbergroupformat;
_x._modifiedby_value = _modifiedby_value;
_x.userprofile = userprofile;
_x.splitviewstate = splitviewstate;
_x.homepagesubarea = homepagesubarea;
_x.helplanguageid = helplanguageid;
_x.homepagearea = homepagearea;
_x.selectedglobalfilterid = selectedglobalfilterid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.personalizationsettings = personalizationsettings;
_x.dateformatstring = dateformatstring;
_x.trackingtokenid = trackingtokenid;
_x.issendasallowed = issendasallowed;
_x.isdefaultcountrycodecheckenabled = isdefaultcountrycodecheckenabled;
_x.timezonedaylightdayofweek = timezonedaylightdayofweek;
_x.fullnameconventioncode = fullnameconventioncode;
_x.timezonestandardday = timezonestandardday;
_x.usecrmformforappointment = usecrmformforappointment;
_x.dateformatcode = dateformatcode;
_x.timezonestandardhour = timezonestandardhour;
_x.timezonestandardmonth = timezonestandardmonth;
_x.timezonestandardsecond = timezonestandardsecond;
_x.timezonestandardbias = timezonestandardbias;
_x.isduplicatedetectionenabledwhengoingonline = isduplicatedetectionenabledwhengoingonline;
_x.businessunitid = businessunitid;
_x.paginglimit = paginglimit;
_x.outlooksyncinterval = outlooksyncinterval;
_x.createdon = createdon;
_x.offlinesyncinterval = offlinesyncinterval;
_x.systemuserid = systemuserid;
_x.timezonestandardminute = timezonestandardminute;
_x.timezonedaylightminute = timezonedaylightminute;
_x._createdby_value = _createdby_value;
_x.currencysymbol = currencysymbol;
_x.reportscripterrors = reportscripterrors;
_x.currencyformatcode = currencyformatcode;
_x.timezonedaylightday = timezonedaylightday;
_x.nexttrackingnumber = nexttrackingnumber;
_x.synccontactcompany = synccontactcompany;
_x.entityformmode = entityformmode;
_x.datavalidationmodeforexporttoexcel = datavalidationmodeforexporttoexcel;
_x.decimalsymbol = decimalsymbol;
_x.isguidedhelpenabled = isguidedhelpenabled;
_x.resourcebookingexchangesyncversion = resourcebookingexchangesyncversion;
_x.timeformatstring = timeformatstring;
_x.showweeknumber = showweeknumber;
_x.timezonestandardyear = timezonestandardyear;
_x.lastalertsviewedtime = lastalertsviewedtime;
_x.pmdesignator = pmdesignator;
_x.ignoreunsolicitedemail = ignoreunsolicitedemail;
_x.timezonedaylightmonth = timezonedaylightmonth;
_x.timeformatcode = timeformatcode;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.workdaystarttime = workdaystarttime;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.defaultdashboardid = defaultdashboardid;
_x.isappsforcrmalertdismissed = isappsforcrmalertdismissed;
_x.addressbooksyncinterval = addressbooksyncinterval;
_x.workdaystoptime = workdaystoptime;
_x.timezonedaylightyear = timezonedaylightyear;
_x.incomingemailfilteringmethod = incomingemailfilteringmethod;
_x.modifiedon = modifiedon;
_x.isautodatacaptureenabled = isautodatacaptureenabled;
_x.usecrmformforemail = usecrmformforemail;
_x.useimagestrips = useimagestrips;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Usersettings[");
b.append("timezonedaylightsecond=");
b.append(this.timezonedaylightsecond);
b.append(", ");
b.append("numberseparator=");
b.append(this.numberseparator);
b.append(", ");
b.append("timezonedaylightbias=");
b.append(this.timezonedaylightbias);
b.append(", ");
b.append("homepagelayout=");
b.append(this.homepagelayout);
b.append(", ");
b.append("negativecurrencyformatcode=");
b.append(this.negativecurrencyformatcode);
b.append(", ");
b.append("defaultsearchexperience=");
b.append(this.defaultsearchexperience);
b.append(", ");
b.append("timeseparator=");
b.append(this.timeseparator);
b.append(", ");
b.append("longdateformatcode=");
b.append(this.longdateformatcode);
b.append(", ");
b.append("calendartype=");
b.append(this.calendartype);
b.append(", ");
b.append("timezonecode=");
b.append(this.timezonecode);
b.append(", ");
b.append("usecrmformforcontact=");
b.append(this.usecrmformforcontact);
b.append(", ");
b.append("timezonedaylighthour=");
b.append(this.timezonedaylighthour);
b.append(", ");
b.append("isemailconversationviewenabled=");
b.append(this.isemailconversationviewenabled);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("getstartedpanecontentenabled=");
b.append(this.getstartedpanecontentenabled);
b.append(", ");
b.append("dateseparator=");
b.append(this.dateseparator);
b.append(", ");
b.append("uilanguageid=");
b.append(this.uilanguageid);
b.append(", ");
b.append("usecrmformfortask=");
b.append(this.usecrmformfortask);
b.append(", ");
b.append("autocreatecontactonpromote=");
b.append(this.autocreatecontactonpromote);
b.append(", ");
b.append("amdesignator=");
b.append(this.amdesignator);
b.append(", ");
b.append("timezonestandarddayofweek=");
b.append(this.timezonestandarddayofweek);
b.append(", ");
b.append("timezonebias=");
b.append(this.timezonebias);
b.append(", ");
b.append("defaultcalendarview=");
b.append(this.defaultcalendarview);
b.append(", ");
b.append("advancedfindstartupmode=");
b.append(this.advancedfindstartupmode);
b.append(", ");
b.append("visualizationpanelayout=");
b.append(this.visualizationpanelayout);
b.append(", ");
b.append("defaultcountrycode=");
b.append(this.defaultcountrycode);
b.append(", ");
b.append("negativeformatcode=");
b.append(this.negativeformatcode);
b.append(", ");
b.append("isresourcebookingexchangesyncenabled=");
b.append(this.isresourcebookingexchangesyncenabled);
b.append(", ");
b.append("localeid=");
b.append(this.localeid);
b.append(", ");
b.append("autocaptureuserstatus=");
b.append(this.autocaptureuserstatus);
b.append(", ");
b.append("numbergroupformat=");
b.append(this.numbergroupformat);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("userprofile=");
b.append(this.userprofile);
b.append(", ");
b.append("splitviewstate=");
b.append(this.splitviewstate);
b.append(", ");
b.append("homepagesubarea=");
b.append(this.homepagesubarea);
b.append(", ");
b.append("helplanguageid=");
b.append(this.helplanguageid);
b.append(", ");
b.append("homepagearea=");
b.append(this.homepagearea);
b.append(", ");
b.append("selectedglobalfilterid=");
b.append(this.selectedglobalfilterid);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("personalizationsettings=");
b.append(this.personalizationsettings);
b.append(", ");
b.append("dateformatstring=");
b.append(this.dateformatstring);
b.append(", ");
b.append("trackingtokenid=");
b.append(this.trackingtokenid);
b.append(", ");
b.append("issendasallowed=");
b.append(this.issendasallowed);
b.append(", ");
b.append("isdefaultcountrycodecheckenabled=");
b.append(this.isdefaultcountrycodecheckenabled);
b.append(", ");
b.append("timezonedaylightdayofweek=");
b.append(this.timezonedaylightdayofweek);
b.append(", ");
b.append("fullnameconventioncode=");
b.append(this.fullnameconventioncode);
b.append(", ");
b.append("timezonestandardday=");
b.append(this.timezonestandardday);
b.append(", ");
b.append("usecrmformforappointment=");
b.append(this.usecrmformforappointment);
b.append(", ");
b.append("dateformatcode=");
b.append(this.dateformatcode);
b.append(", ");
b.append("timezonestandardhour=");
b.append(this.timezonestandardhour);
b.append(", ");
b.append("timezonestandardmonth=");
b.append(this.timezonestandardmonth);
b.append(", ");
b.append("timezonestandardsecond=");
b.append(this.timezonestandardsecond);
b.append(", ");
b.append("timezonestandardbias=");
b.append(this.timezonestandardbias);
b.append(", ");
b.append("isduplicatedetectionenabledwhengoingonline=");
b.append(this.isduplicatedetectionenabledwhengoingonline);
b.append(", ");
b.append("businessunitid=");
b.append(this.businessunitid);
b.append(", ");
b.append("paginglimit=");
b.append(this.paginglimit);
b.append(", ");
b.append("outlooksyncinterval=");
b.append(this.outlooksyncinterval);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("offlinesyncinterval=");
b.append(this.offlinesyncinterval);
b.append(", ");
b.append("systemuserid=");
b.append(this.systemuserid);
b.append(", ");
b.append("timezonestandardminute=");
b.append(this.timezonestandardminute);
b.append(", ");
b.append("timezonedaylightminute=");
b.append(this.timezonedaylightminute);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("currencysymbol=");
b.append(this.currencysymbol);
b.append(", ");
b.append("reportscripterrors=");
b.append(this.reportscripterrors);
b.append(", ");
b.append("currencyformatcode=");
b.append(this.currencyformatcode);
b.append(", ");
b.append("timezonedaylightday=");
b.append(this.timezonedaylightday);
b.append(", ");
b.append("nexttrackingnumber=");
b.append(this.nexttrackingnumber);
b.append(", ");
b.append("synccontactcompany=");
b.append(this.synccontactcompany);
b.append(", ");
b.append("entityformmode=");
b.append(this.entityformmode);
b.append(", ");
b.append("datavalidationmodeforexporttoexcel=");
b.append(this.datavalidationmodeforexporttoexcel);
b.append(", ");
b.append("decimalsymbol=");
b.append(this.decimalsymbol);
b.append(", ");
b.append("isguidedhelpenabled=");
b.append(this.isguidedhelpenabled);
b.append(", ");
b.append("resourcebookingexchangesyncversion=");
b.append(this.resourcebookingexchangesyncversion);
b.append(", ");
b.append("timeformatstring=");
b.append(this.timeformatstring);
b.append(", ");
b.append("showweeknumber=");
b.append(this.showweeknumber);
b.append(", ");
b.append("timezonestandardyear=");
b.append(this.timezonestandardyear);
b.append(", ");
b.append("lastalertsviewedtime=");
b.append(this.lastalertsviewedtime);
b.append(", ");
b.append("pmdesignator=");
b.append(this.pmdesignator);
b.append(", ");
b.append("ignoreunsolicitedemail=");
b.append(this.ignoreunsolicitedemail);
b.append(", ");
b.append("timezonedaylightmonth=");
b.append(this.timezonedaylightmonth);
b.append(", ");
b.append("timeformatcode=");
b.append(this.timeformatcode);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("workdaystarttime=");
b.append(this.workdaystarttime);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("defaultdashboardid=");
b.append(this.defaultdashboardid);
b.append(", ");
b.append("isappsforcrmalertdismissed=");
b.append(this.isappsforcrmalertdismissed);
b.append(", ");
b.append("addressbooksyncinterval=");
b.append(this.addressbooksyncinterval);
b.append(", ");
b.append("workdaystoptime=");
b.append(this.workdaystoptime);
b.append(", ");
b.append("timezonedaylightyear=");
b.append(this.timezonedaylightyear);
b.append(", ");
b.append("incomingemailfilteringmethod=");
b.append(this.incomingemailfilteringmethod);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("isautodatacaptureenabled=");
b.append(this.isautodatacaptureenabled);
b.append(", ");
b.append("usecrmformforemail=");
b.append(this.usecrmformforemail);
b.append(", ");
b.append("useimagestrips=");
b.append(this.useimagestrips);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy