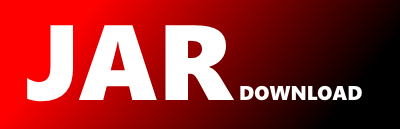
microsoft.dynamics.crm.entity.Workflow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.Optional;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.complex.InputArgumentCollection;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExpiredprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FlowsessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aimodelCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_solutionhealthruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.NewprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcessstageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesstriggerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TranslationprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowbinaryCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.WorkflowRequest;
@JsonPropertyOrder({
"@odata.type",
"deletestage",
"iscustomprocessingstepallowedforotherpublishers",
"iscustomizable",
"processtriggerscope",
"processtriggerformid",
"name",
"_createdonbehalfby_value",
"versionnumber",
"entityimage",
"scope",
"inputparameters",
"_modifiedby_value",
"createstage",
"runas",
"ondemand",
"uiflowtype",
"rendererobjecttypecode",
"workflowid",
"modifiedon",
"entityimage_timestamp",
"syncworkflowlogonfailure",
"createdon",
"iscrmuiworkflow",
"triggeroncreate",
"processroleassignment",
"businessprocesstype",
"formid",
"updatestage",
"rank",
"type",
"_owningbusinessunit_value",
"uidata",
"_plugintypeid_value",
"category",
"statecode",
"_activeworkflowid_value",
"_owningteam_value",
"asyncautodelete",
"triggeronupdateattributelist",
"description",
"uniquename",
"statuscode",
"ismanaged",
"workflowidunique",
"overwritetime",
"processorder",
"_modifiedonbehalfby_value",
"_createdby_value",
"subprocess",
"entityimage_url",
"solutionid",
"_parentworkflowid_value",
"_owninguser_value",
"languagecode",
"introducedversion",
"xaml",
"istransacted",
"componentstate",
"clientdata",
"mode",
"triggerondelete",
"entityimageid",
"primaryentity",
"_ownerid_value",
"_sdkmessageid_value"})
@JsonInclude(Include.NON_NULL)
public class Workflow extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.workflow";
}
@JsonProperty("deletestage")
protected Integer deletestage;
@JsonProperty("iscustomprocessingstepallowedforotherpublishers")
protected BooleanManagedProperty iscustomprocessingstepallowedforotherpublishers;
@JsonProperty("iscustomizable")
protected BooleanManagedProperty iscustomizable;
@JsonProperty("processtriggerscope")
protected Integer processtriggerscope;
@JsonProperty("processtriggerformid")
protected String processtriggerformid;
@JsonProperty("name")
protected String name;
@JsonProperty("_createdonbehalfby_value")
protected String _createdonbehalfby_value;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("scope")
protected Integer scope;
@JsonProperty("inputparameters")
protected String inputparameters;
@JsonProperty("_modifiedby_value")
protected String _modifiedby_value;
@JsonProperty("createstage")
protected Integer createstage;
@JsonProperty("runas")
protected Integer runas;
@JsonProperty("ondemand")
protected Boolean ondemand;
@JsonProperty("uiflowtype")
protected Integer uiflowtype;
@JsonProperty("rendererobjecttypecode")
protected String rendererobjecttypecode;
@JsonProperty("workflowid")
protected String workflowid;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("syncworkflowlogonfailure")
protected Boolean syncworkflowlogonfailure;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("iscrmuiworkflow")
protected Boolean iscrmuiworkflow;
@JsonProperty("triggeroncreate")
protected Boolean triggeroncreate;
@JsonProperty("processroleassignment")
protected String processroleassignment;
@JsonProperty("businessprocesstype")
protected Integer businessprocesstype;
@JsonProperty("formid")
protected String formid;
@JsonProperty("updatestage")
protected Integer updatestage;
@JsonProperty("rank")
protected Integer rank;
@JsonProperty("type")
protected Integer type;
@JsonProperty("_owningbusinessunit_value")
protected String _owningbusinessunit_value;
@JsonProperty("uidata")
protected String uidata;
@JsonProperty("_plugintypeid_value")
protected String _plugintypeid_value;
@JsonProperty("category")
protected Integer category;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("_activeworkflowid_value")
protected String _activeworkflowid_value;
@JsonProperty("_owningteam_value")
protected String _owningteam_value;
@JsonProperty("asyncautodelete")
protected Boolean asyncautodelete;
@JsonProperty("triggeronupdateattributelist")
protected String triggeronupdateattributelist;
@JsonProperty("description")
protected String description;
@JsonProperty("uniquename")
protected String uniquename;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("workflowidunique")
protected String workflowidunique;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("processorder")
protected Integer processorder;
@JsonProperty("_modifiedonbehalfby_value")
protected String _modifiedonbehalfby_value;
@JsonProperty("_createdby_value")
protected String _createdby_value;
@JsonProperty("subprocess")
protected Boolean subprocess;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("solutionid")
protected String solutionid;
@JsonProperty("_parentworkflowid_value")
protected String _parentworkflowid_value;
@JsonProperty("_owninguser_value")
protected String _owninguser_value;
@JsonProperty("languagecode")
protected Integer languagecode;
@JsonProperty("introducedversion")
protected String introducedversion;
@JsonProperty("xaml")
protected String xaml;
@JsonProperty("istransacted")
protected Boolean istransacted;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("clientdata")
protected String clientdata;
@JsonProperty("mode")
protected Integer mode;
@JsonProperty("triggerondelete")
protected Boolean triggerondelete;
@JsonProperty("entityimageid")
protected String entityimageid;
@JsonProperty("primaryentity")
protected String primaryentity;
@JsonProperty("_ownerid_value")
protected String _ownerid_value;
@JsonProperty("_sdkmessageid_value")
protected String _sdkmessageid_value;
protected Workflow() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWorkflow() {
return new Builder();
}
public static final class Builder {
private Integer deletestage;
private BooleanManagedProperty iscustomprocessingstepallowedforotherpublishers;
private BooleanManagedProperty iscustomizable;
private Integer processtriggerscope;
private String processtriggerformid;
private String name;
private String _createdonbehalfby_value;
private Long versionnumber;
private byte[] entityimage;
private Integer scope;
private String inputparameters;
private String _modifiedby_value;
private Integer createstage;
private Integer runas;
private Boolean ondemand;
private Integer uiflowtype;
private String rendererobjecttypecode;
private String workflowid;
private OffsetDateTime modifiedon;
private Long entityimage_timestamp;
private Boolean syncworkflowlogonfailure;
private OffsetDateTime createdon;
private Boolean iscrmuiworkflow;
private Boolean triggeroncreate;
private String processroleassignment;
private Integer businessprocesstype;
private String formid;
private Integer updatestage;
private Integer rank;
private Integer type;
private String _owningbusinessunit_value;
private String uidata;
private String _plugintypeid_value;
private Integer category;
private Integer statecode;
private String _activeworkflowid_value;
private String _owningteam_value;
private Boolean asyncautodelete;
private String triggeronupdateattributelist;
private String description;
private String uniquename;
private Integer statuscode;
private Boolean ismanaged;
private String workflowidunique;
private OffsetDateTime overwritetime;
private Integer processorder;
private String _modifiedonbehalfby_value;
private String _createdby_value;
private Boolean subprocess;
private String entityimage_url;
private String solutionid;
private String _parentworkflowid_value;
private String _owninguser_value;
private Integer languagecode;
private String introducedversion;
private String xaml;
private Boolean istransacted;
private Integer componentstate;
private String clientdata;
private Integer mode;
private Boolean triggerondelete;
private String entityimageid;
private String primaryentity;
private String _ownerid_value;
private String _sdkmessageid_value;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder deletestage(Integer deletestage) {
this.deletestage = deletestage;
this.changedFields = changedFields.add("deletestage");
return this;
}
public Builder iscustomprocessingstepallowedforotherpublishers(BooleanManagedProperty iscustomprocessingstepallowedforotherpublishers) {
this.iscustomprocessingstepallowedforotherpublishers = iscustomprocessingstepallowedforotherpublishers;
this.changedFields = changedFields.add("iscustomprocessingstepallowedforotherpublishers");
return this;
}
public Builder iscustomizable(BooleanManagedProperty iscustomizable) {
this.iscustomizable = iscustomizable;
this.changedFields = changedFields.add("iscustomizable");
return this;
}
public Builder processtriggerscope(Integer processtriggerscope) {
this.processtriggerscope = processtriggerscope;
this.changedFields = changedFields.add("processtriggerscope");
return this;
}
public Builder processtriggerformid(String processtriggerformid) {
this.processtriggerformid = processtriggerformid;
this.changedFields = changedFields.add("processtriggerformid");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder _createdonbehalfby_value(String _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder scope(Integer scope) {
this.scope = scope;
this.changedFields = changedFields.add("scope");
return this;
}
public Builder inputparameters(String inputparameters) {
this.inputparameters = inputparameters;
this.changedFields = changedFields.add("inputparameters");
return this;
}
public Builder _modifiedby_value(String _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder createstage(Integer createstage) {
this.createstage = createstage;
this.changedFields = changedFields.add("createstage");
return this;
}
public Builder runas(Integer runas) {
this.runas = runas;
this.changedFields = changedFields.add("runas");
return this;
}
public Builder ondemand(Boolean ondemand) {
this.ondemand = ondemand;
this.changedFields = changedFields.add("ondemand");
return this;
}
public Builder uiflowtype(Integer uiflowtype) {
this.uiflowtype = uiflowtype;
this.changedFields = changedFields.add("uiflowtype");
return this;
}
public Builder rendererobjecttypecode(String rendererobjecttypecode) {
this.rendererobjecttypecode = rendererobjecttypecode;
this.changedFields = changedFields.add("rendererobjecttypecode");
return this;
}
public Builder workflowid(String workflowid) {
this.workflowid = workflowid;
this.changedFields = changedFields.add("workflowid");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder syncworkflowlogonfailure(Boolean syncworkflowlogonfailure) {
this.syncworkflowlogonfailure = syncworkflowlogonfailure;
this.changedFields = changedFields.add("syncworkflowlogonfailure");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder iscrmuiworkflow(Boolean iscrmuiworkflow) {
this.iscrmuiworkflow = iscrmuiworkflow;
this.changedFields = changedFields.add("iscrmuiworkflow");
return this;
}
public Builder triggeroncreate(Boolean triggeroncreate) {
this.triggeroncreate = triggeroncreate;
this.changedFields = changedFields.add("triggeroncreate");
return this;
}
public Builder processroleassignment(String processroleassignment) {
this.processroleassignment = processroleassignment;
this.changedFields = changedFields.add("processroleassignment");
return this;
}
public Builder businessprocesstype(Integer businessprocesstype) {
this.businessprocesstype = businessprocesstype;
this.changedFields = changedFields.add("businessprocesstype");
return this;
}
public Builder formid(String formid) {
this.formid = formid;
this.changedFields = changedFields.add("formid");
return this;
}
public Builder updatestage(Integer updatestage) {
this.updatestage = updatestage;
this.changedFields = changedFields.add("updatestage");
return this;
}
public Builder rank(Integer rank) {
this.rank = rank;
this.changedFields = changedFields.add("rank");
return this;
}
public Builder type(Integer type) {
this.type = type;
this.changedFields = changedFields.add("type");
return this;
}
public Builder _owningbusinessunit_value(String _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder uidata(String uidata) {
this.uidata = uidata;
this.changedFields = changedFields.add("uidata");
return this;
}
public Builder _plugintypeid_value(String _plugintypeid_value) {
this._plugintypeid_value = _plugintypeid_value;
this.changedFields = changedFields.add("_plugintypeid_value");
return this;
}
public Builder category(Integer category) {
this.category = category;
this.changedFields = changedFields.add("category");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder _activeworkflowid_value(String _activeworkflowid_value) {
this._activeworkflowid_value = _activeworkflowid_value;
this.changedFields = changedFields.add("_activeworkflowid_value");
return this;
}
public Builder _owningteam_value(String _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder asyncautodelete(Boolean asyncautodelete) {
this.asyncautodelete = asyncautodelete;
this.changedFields = changedFields.add("asyncautodelete");
return this;
}
public Builder triggeronupdateattributelist(String triggeronupdateattributelist) {
this.triggeronupdateattributelist = triggeronupdateattributelist;
this.changedFields = changedFields.add("triggeronupdateattributelist");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder uniquename(String uniquename) {
this.uniquename = uniquename;
this.changedFields = changedFields.add("uniquename");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder workflowidunique(String workflowidunique) {
this.workflowidunique = workflowidunique;
this.changedFields = changedFields.add("workflowidunique");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder processorder(Integer processorder) {
this.processorder = processorder;
this.changedFields = changedFields.add("processorder");
return this;
}
public Builder _modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder _createdby_value(String _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder subprocess(Boolean subprocess) {
this.subprocess = subprocess;
this.changedFields = changedFields.add("subprocess");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder solutionid(String solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder _parentworkflowid_value(String _parentworkflowid_value) {
this._parentworkflowid_value = _parentworkflowid_value;
this.changedFields = changedFields.add("_parentworkflowid_value");
return this;
}
public Builder _owninguser_value(String _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder languagecode(Integer languagecode) {
this.languagecode = languagecode;
this.changedFields = changedFields.add("languagecode");
return this;
}
public Builder introducedversion(String introducedversion) {
this.introducedversion = introducedversion;
this.changedFields = changedFields.add("introducedversion");
return this;
}
public Builder xaml(String xaml) {
this.xaml = xaml;
this.changedFields = changedFields.add("xaml");
return this;
}
public Builder istransacted(Boolean istransacted) {
this.istransacted = istransacted;
this.changedFields = changedFields.add("istransacted");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder clientdata(String clientdata) {
this.clientdata = clientdata;
this.changedFields = changedFields.add("clientdata");
return this;
}
public Builder mode(Integer mode) {
this.mode = mode;
this.changedFields = changedFields.add("mode");
return this;
}
public Builder triggerondelete(Boolean triggerondelete) {
this.triggerondelete = triggerondelete;
this.changedFields = changedFields.add("triggerondelete");
return this;
}
public Builder entityimageid(String entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder primaryentity(String primaryentity) {
this.primaryentity = primaryentity;
this.changedFields = changedFields.add("primaryentity");
return this;
}
public Builder _ownerid_value(String _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _sdkmessageid_value(String _sdkmessageid_value) {
this._sdkmessageid_value = _sdkmessageid_value;
this.changedFields = changedFields.add("_sdkmessageid_value");
return this;
}
public Workflow build() {
Workflow _x = new Workflow();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "Microsoft.Dynamics.CRM.workflow";
_x.deletestage = deletestage;
_x.iscustomprocessingstepallowedforotherpublishers = iscustomprocessingstepallowedforotherpublishers;
_x.iscustomizable = iscustomizable;
_x.processtriggerscope = processtriggerscope;
_x.processtriggerformid = processtriggerformid;
_x.name = name;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x.entityimage = entityimage;
_x.scope = scope;
_x.inputparameters = inputparameters;
_x._modifiedby_value = _modifiedby_value;
_x.createstage = createstage;
_x.runas = runas;
_x.ondemand = ondemand;
_x.uiflowtype = uiflowtype;
_x.rendererobjecttypecode = rendererobjecttypecode;
_x.workflowid = workflowid;
_x.modifiedon = modifiedon;
_x.entityimage_timestamp = entityimage_timestamp;
_x.syncworkflowlogonfailure = syncworkflowlogonfailure;
_x.createdon = createdon;
_x.iscrmuiworkflow = iscrmuiworkflow;
_x.triggeroncreate = triggeroncreate;
_x.processroleassignment = processroleassignment;
_x.businessprocesstype = businessprocesstype;
_x.formid = formid;
_x.updatestage = updatestage;
_x.rank = rank;
_x.type = type;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.uidata = uidata;
_x._plugintypeid_value = _plugintypeid_value;
_x.category = category;
_x.statecode = statecode;
_x._activeworkflowid_value = _activeworkflowid_value;
_x._owningteam_value = _owningteam_value;
_x.asyncautodelete = asyncautodelete;
_x.triggeronupdateattributelist = triggeronupdateattributelist;
_x.description = description;
_x.uniquename = uniquename;
_x.statuscode = statuscode;
_x.ismanaged = ismanaged;
_x.workflowidunique = workflowidunique;
_x.overwritetime = overwritetime;
_x.processorder = processorder;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x._createdby_value = _createdby_value;
_x.subprocess = subprocess;
_x.entityimage_url = entityimage_url;
_x.solutionid = solutionid;
_x._parentworkflowid_value = _parentworkflowid_value;
_x._owninguser_value = _owninguser_value;
_x.languagecode = languagecode;
_x.introducedversion = introducedversion;
_x.xaml = xaml;
_x.istransacted = istransacted;
_x.componentstate = componentstate;
_x.clientdata = clientdata;
_x.mode = mode;
_x.triggerondelete = triggerondelete;
_x.entityimageid = entityimageid;
_x.primaryentity = primaryentity;
_x._ownerid_value = _ownerid_value;
_x._sdkmessageid_value = _sdkmessageid_value;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && workflowid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(workflowid.toString()));
}
}
@Property(name="deletestage")
@JsonIgnore
public Optional getDeletestage() {
return Optional.ofNullable(deletestage);
}
public Workflow withDeletestage(Integer deletestage) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("deletestage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.deletestage = deletestage;
return _x;
}
@Property(name="iscustomprocessingstepallowedforotherpublishers")
@JsonIgnore
public Optional getIscustomprocessingstepallowedforotherpublishers() {
return Optional.ofNullable(iscustomprocessingstepallowedforotherpublishers);
}
public Workflow withIscustomprocessingstepallowedforotherpublishers(BooleanManagedProperty iscustomprocessingstepallowedforotherpublishers) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("iscustomprocessingstepallowedforotherpublishers");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.iscustomprocessingstepallowedforotherpublishers = iscustomprocessingstepallowedforotherpublishers;
return _x;
}
@Property(name="iscustomizable")
@JsonIgnore
public Optional getIscustomizable() {
return Optional.ofNullable(iscustomizable);
}
public Workflow withIscustomizable(BooleanManagedProperty iscustomizable) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("iscustomizable");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.iscustomizable = iscustomizable;
return _x;
}
@Property(name="processtriggerscope")
@JsonIgnore
public Optional getProcesstriggerscope() {
return Optional.ofNullable(processtriggerscope);
}
public Workflow withProcesstriggerscope(Integer processtriggerscope) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("processtriggerscope");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.processtriggerscope = processtriggerscope;
return _x;
}
@Property(name="processtriggerformid")
@JsonIgnore
public Optional getProcesstriggerformid() {
return Optional.ofNullable(processtriggerformid);
}
public Workflow withProcesstriggerformid(String processtriggerformid) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("processtriggerformid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.processtriggerformid = processtriggerformid;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Workflow withName(String name) {
Checks.checkIsAscii(name);
Workflow _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.name = name;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Workflow with_createdonbehalfby_value(String _createdonbehalfby_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Workflow withVersionnumber(Long versionnumber) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Workflow withEntityimage(byte[] entityimage) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.entityimage = entityimage;
return _x;
}
@Property(name="scope")
@JsonIgnore
public Optional getScope() {
return Optional.ofNullable(scope);
}
public Workflow withScope(Integer scope) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("scope");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.scope = scope;
return _x;
}
@Property(name="inputparameters")
@JsonIgnore
public Optional getInputparameters() {
return Optional.ofNullable(inputparameters);
}
public Workflow withInputparameters(String inputparameters) {
Checks.checkIsAscii(inputparameters);
Workflow _x = _copy();
_x.changedFields = changedFields.add("inputparameters");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.inputparameters = inputparameters;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Workflow with_modifiedby_value(String _modifiedby_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="createstage")
@JsonIgnore
public Optional getCreatestage() {
return Optional.ofNullable(createstage);
}
public Workflow withCreatestage(Integer createstage) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("createstage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.createstage = createstage;
return _x;
}
@Property(name="runas")
@JsonIgnore
public Optional getRunas() {
return Optional.ofNullable(runas);
}
public Workflow withRunas(Integer runas) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("runas");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.runas = runas;
return _x;
}
@Property(name="ondemand")
@JsonIgnore
public Optional getOndemand() {
return Optional.ofNullable(ondemand);
}
public Workflow withOndemand(Boolean ondemand) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("ondemand");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.ondemand = ondemand;
return _x;
}
@Property(name="uiflowtype")
@JsonIgnore
public Optional getUiflowtype() {
return Optional.ofNullable(uiflowtype);
}
public Workflow withUiflowtype(Integer uiflowtype) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("uiflowtype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.uiflowtype = uiflowtype;
return _x;
}
@Property(name="rendererobjecttypecode")
@JsonIgnore
public Optional getRendererobjecttypecode() {
return Optional.ofNullable(rendererobjecttypecode);
}
public Workflow withRendererobjecttypecode(String rendererobjecttypecode) {
Checks.checkIsAscii(rendererobjecttypecode);
Workflow _x = _copy();
_x.changedFields = changedFields.add("rendererobjecttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.rendererobjecttypecode = rendererobjecttypecode;
return _x;
}
@Property(name="workflowid")
@JsonIgnore
public Optional getWorkflowid() {
return Optional.ofNullable(workflowid);
}
public Workflow withWorkflowid(String workflowid) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("workflowid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.workflowid = workflowid;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Workflow withModifiedon(OffsetDateTime modifiedon) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Workflow withEntityimage_timestamp(Long entityimage_timestamp) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="syncworkflowlogonfailure")
@JsonIgnore
public Optional getSyncworkflowlogonfailure() {
return Optional.ofNullable(syncworkflowlogonfailure);
}
public Workflow withSyncworkflowlogonfailure(Boolean syncworkflowlogonfailure) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("syncworkflowlogonfailure");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.syncworkflowlogonfailure = syncworkflowlogonfailure;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Workflow withCreatedon(OffsetDateTime createdon) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.createdon = createdon;
return _x;
}
@Property(name="iscrmuiworkflow")
@JsonIgnore
public Optional getIscrmuiworkflow() {
return Optional.ofNullable(iscrmuiworkflow);
}
public Workflow withIscrmuiworkflow(Boolean iscrmuiworkflow) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("iscrmuiworkflow");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.iscrmuiworkflow = iscrmuiworkflow;
return _x;
}
@Property(name="triggeroncreate")
@JsonIgnore
public Optional getTriggeroncreate() {
return Optional.ofNullable(triggeroncreate);
}
public Workflow withTriggeroncreate(Boolean triggeroncreate) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("triggeroncreate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.triggeroncreate = triggeroncreate;
return _x;
}
@Property(name="processroleassignment")
@JsonIgnore
public Optional getProcessroleassignment() {
return Optional.ofNullable(processroleassignment);
}
public Workflow withProcessroleassignment(String processroleassignment) {
Checks.checkIsAscii(processroleassignment);
Workflow _x = _copy();
_x.changedFields = changedFields.add("processroleassignment");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.processroleassignment = processroleassignment;
return _x;
}
@Property(name="businessprocesstype")
@JsonIgnore
public Optional getBusinessprocesstype() {
return Optional.ofNullable(businessprocesstype);
}
public Workflow withBusinessprocesstype(Integer businessprocesstype) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("businessprocesstype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.businessprocesstype = businessprocesstype;
return _x;
}
@Property(name="formid")
@JsonIgnore
public Optional getFormid() {
return Optional.ofNullable(formid);
}
public Workflow withFormid(String formid) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("formid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.formid = formid;
return _x;
}
@Property(name="updatestage")
@JsonIgnore
public Optional getUpdatestage() {
return Optional.ofNullable(updatestage);
}
public Workflow withUpdatestage(Integer updatestage) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("updatestage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.updatestage = updatestage;
return _x;
}
@Property(name="rank")
@JsonIgnore
public Optional getRank() {
return Optional.ofNullable(rank);
}
public Workflow withRank(Integer rank) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("rank");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.rank = rank;
return _x;
}
@Property(name="type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public Workflow withType(Integer type) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("type");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.type = type;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Workflow with_owningbusinessunit_value(String _owningbusinessunit_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="uidata")
@JsonIgnore
public Optional getUidata() {
return Optional.ofNullable(uidata);
}
public Workflow withUidata(String uidata) {
Checks.checkIsAscii(uidata);
Workflow _x = _copy();
_x.changedFields = changedFields.add("uidata");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.uidata = uidata;
return _x;
}
@Property(name="_plugintypeid_value")
@JsonIgnore
public Optional get_plugintypeid_value() {
return Optional.ofNullable(_plugintypeid_value);
}
public Workflow with_plugintypeid_value(String _plugintypeid_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_plugintypeid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._plugintypeid_value = _plugintypeid_value;
return _x;
}
@Property(name="category")
@JsonIgnore
public Optional getCategory() {
return Optional.ofNullable(category);
}
public Workflow withCategory(Integer category) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("category");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.category = category;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Workflow withStatecode(Integer statecode) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.statecode = statecode;
return _x;
}
@Property(name="_activeworkflowid_value")
@JsonIgnore
public Optional get_activeworkflowid_value() {
return Optional.ofNullable(_activeworkflowid_value);
}
public Workflow with_activeworkflowid_value(String _activeworkflowid_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_activeworkflowid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._activeworkflowid_value = _activeworkflowid_value;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Workflow with_owningteam_value(String _owningteam_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="asyncautodelete")
@JsonIgnore
public Optional getAsyncautodelete() {
return Optional.ofNullable(asyncautodelete);
}
public Workflow withAsyncautodelete(Boolean asyncautodelete) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("asyncautodelete");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.asyncautodelete = asyncautodelete;
return _x;
}
@Property(name="triggeronupdateattributelist")
@JsonIgnore
public Optional getTriggeronupdateattributelist() {
return Optional.ofNullable(triggeronupdateattributelist);
}
public Workflow withTriggeronupdateattributelist(String triggeronupdateattributelist) {
Checks.checkIsAscii(triggeronupdateattributelist);
Workflow _x = _copy();
_x.changedFields = changedFields.add("triggeronupdateattributelist");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.triggeronupdateattributelist = triggeronupdateattributelist;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Workflow withDescription(String description) {
Checks.checkIsAscii(description);
Workflow _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.description = description;
return _x;
}
@Property(name="uniquename")
@JsonIgnore
public Optional getUniquename() {
return Optional.ofNullable(uniquename);
}
public Workflow withUniquename(String uniquename) {
Checks.checkIsAscii(uniquename);
Workflow _x = _copy();
_x.changedFields = changedFields.add("uniquename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.uniquename = uniquename;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Workflow withStatuscode(Integer statuscode) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.statuscode = statuscode;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Workflow withIsmanaged(Boolean ismanaged) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="workflowidunique")
@JsonIgnore
public Optional getWorkflowidunique() {
return Optional.ofNullable(workflowidunique);
}
public Workflow withWorkflowidunique(String workflowidunique) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("workflowidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.workflowidunique = workflowidunique;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Workflow withOverwritetime(OffsetDateTime overwritetime) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="processorder")
@JsonIgnore
public Optional getProcessorder() {
return Optional.ofNullable(processorder);
}
public Workflow withProcessorder(Integer processorder) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("processorder");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.processorder = processorder;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Workflow with_modifiedonbehalfby_value(String _modifiedonbehalfby_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Workflow with_createdby_value(String _createdby_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="subprocess")
@JsonIgnore
public Optional getSubprocess() {
return Optional.ofNullable(subprocess);
}
public Workflow withSubprocess(Boolean subprocess) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("subprocess");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.subprocess = subprocess;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Workflow withEntityimage_url(String entityimage_url) {
Checks.checkIsAscii(entityimage_url);
Workflow _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Workflow withSolutionid(String solutionid) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.solutionid = solutionid;
return _x;
}
@Property(name="_parentworkflowid_value")
@JsonIgnore
public Optional get_parentworkflowid_value() {
return Optional.ofNullable(_parentworkflowid_value);
}
public Workflow with_parentworkflowid_value(String _parentworkflowid_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_parentworkflowid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._parentworkflowid_value = _parentworkflowid_value;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Workflow with_owninguser_value(String _owninguser_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="languagecode")
@JsonIgnore
public Optional getLanguagecode() {
return Optional.ofNullable(languagecode);
}
public Workflow withLanguagecode(Integer languagecode) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("languagecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.languagecode = languagecode;
return _x;
}
@Property(name="introducedversion")
@JsonIgnore
public Optional getIntroducedversion() {
return Optional.ofNullable(introducedversion);
}
public Workflow withIntroducedversion(String introducedversion) {
Checks.checkIsAscii(introducedversion);
Workflow _x = _copy();
_x.changedFields = changedFields.add("introducedversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.introducedversion = introducedversion;
return _x;
}
@Property(name="xaml")
@JsonIgnore
public Optional getXaml() {
return Optional.ofNullable(xaml);
}
public Workflow withXaml(String xaml) {
Checks.checkIsAscii(xaml);
Workflow _x = _copy();
_x.changedFields = changedFields.add("xaml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.xaml = xaml;
return _x;
}
@Property(name="istransacted")
@JsonIgnore
public Optional getIstransacted() {
return Optional.ofNullable(istransacted);
}
public Workflow withIstransacted(Boolean istransacted) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("istransacted");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.istransacted = istransacted;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Workflow withComponentstate(Integer componentstate) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.componentstate = componentstate;
return _x;
}
@Property(name="clientdata")
@JsonIgnore
public Optional getClientdata() {
return Optional.ofNullable(clientdata);
}
public Workflow withClientdata(String clientdata) {
Checks.checkIsAscii(clientdata);
Workflow _x = _copy();
_x.changedFields = changedFields.add("clientdata");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.clientdata = clientdata;
return _x;
}
@Property(name="mode")
@JsonIgnore
public Optional getMode() {
return Optional.ofNullable(mode);
}
public Workflow withMode(Integer mode) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("mode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.mode = mode;
return _x;
}
@Property(name="triggerondelete")
@JsonIgnore
public Optional getTriggerondelete() {
return Optional.ofNullable(triggerondelete);
}
public Workflow withTriggerondelete(Boolean triggerondelete) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("triggerondelete");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.triggerondelete = triggerondelete;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Workflow withEntityimageid(String entityimageid) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="primaryentity")
@JsonIgnore
public Optional getPrimaryentity() {
return Optional.ofNullable(primaryentity);
}
public Workflow withPrimaryentity(String primaryentity) {
Checks.checkIsAscii(primaryentity);
Workflow _x = _copy();
_x.changedFields = changedFields.add("primaryentity");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x.primaryentity = primaryentity;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Workflow with_ownerid_value(String _ownerid_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_sdkmessageid_value")
@JsonIgnore
public Optional get_sdkmessageid_value() {
return Optional.ofNullable(_sdkmessageid_value);
}
public Workflow with_sdkmessageid_value(String _sdkmessageid_value) {
Workflow _x = _copy();
_x.changedFields = changedFields.add("_sdkmessageid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.workflow");
_x._sdkmessageid_value = _sdkmessageid_value;
return _x;
}
@NavigationProperty(name="slabase_workflowid")
@JsonIgnore
public SlaCollectionRequest getSlabase_workflowid() {
return new SlaCollectionRequest(
contextPath.addSegment("slabase_workflowid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
@NavigationProperty(name="lk_asyncoperation_workflowactivationid")
@JsonIgnore
public AsyncoperationCollectionRequest getLk_asyncoperation_workflowactivationid() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_workflowactivationid"));
}
@NavigationProperty(name="parentworkflowid")
@JsonIgnore
public WorkflowRequest getParentworkflowid() {
return new WorkflowRequest(contextPath.addSegment("parentworkflowid"));
}
@NavigationProperty(name="workflow_parent_workflow")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_parent_workflow() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_parent_workflow"));
}
@NavigationProperty(name="workflow_expiredprocess")
@JsonIgnore
public ExpiredprocessCollectionRequest getWorkflow_expiredprocess() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("workflow_expiredprocess"));
}
@NavigationProperty(name="slaitembase_workflowid")
@JsonIgnore
public SlaitemCollectionRequest getSlaitembase_workflowid() {
return new SlaitemCollectionRequest(
contextPath.addSegment("slaitembase_workflowid"));
}
@NavigationProperty(name="workflow_translationprocess")
@JsonIgnore
public TranslationprocessCollectionRequest getWorkflow_translationprocess() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("workflow_translationprocess"));
}
@NavigationProperty(name="process_processtrigger")
@JsonIgnore
public ProcesstriggerCollectionRequest getProcess_processtrigger() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("process_processtrigger"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
@NavigationProperty(name="Workflow_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getWorkflow_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Workflow_Annotation"));
}
@NavigationProperty(name="lk_processsession_processid")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_processid() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_processid"));
}
@NavigationProperty(name="process_processstage")
@JsonIgnore
public ProcessstageCollectionRequest getProcess_processstage() {
return new ProcessstageCollectionRequest(
contextPath.addSegment("process_processstage"));
}
@NavigationProperty(name="Workflow_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getWorkflow_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Workflow_SyncErrors"));
}
@NavigationProperty(name="workflow_newprocess")
@JsonIgnore
public NewprocessCollectionRequest getWorkflow_newprocess() {
return new NewprocessCollectionRequest(
contextPath.addSegment("workflow_newprocess"));
}
@NavigationProperty(name="activeworkflowid")
@JsonIgnore
public WorkflowRequest getActiveworkflowid() {
return new WorkflowRequest(contextPath.addSegment("activeworkflowid"));
}
@NavigationProperty(name="workflow_active_workflow")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_active_workflow() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_active_workflow"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
@NavigationProperty(name="regardingobjectid_process")
@JsonIgnore
public FlowsessionCollectionRequest getRegardingobjectid_process() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("regardingobjectid_process"));
}
@NavigationProperty(name="workflow_workflowbinary_Process")
@JsonIgnore
public WorkflowbinaryCollectionRequest getWorkflow_workflowbinary_Process() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("workflow_workflowbinary_Process"));
}
@NavigationProperty(name="msdyn_retrainworkflow_msdyn_toaimodel")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getMsdyn_retrainworkflow_msdyn_toaimodel() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("msdyn_retrainworkflow_msdyn_toaimodel"));
}
@NavigationProperty(name="msdyn_workflow_msdyn_solutionhealthrule_Workflow")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getMsdyn_workflow_msdyn_solutionhealthrule_Workflow() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_Workflow"));
}
@NavigationProperty(name="msdyn_workflow_msdyn_solutionhealthrule_resolutionaction")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getMsdyn_workflow_msdyn_solutionhealthrule_resolutionaction() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_resolutionaction"));
}
@NavigationProperty(name="msdyn_scheduleinferenceworkflow_msdyn_toaimodel")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getMsdyn_scheduleinferenceworkflow_msdyn_toaimodel() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("msdyn_scheduleinferenceworkflow_msdyn_toaimodel"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Workflow patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Workflow _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Workflow put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Workflow _x = _copy();
_x.changedFields = null;
return _x;
}
private Workflow _copy() {
Workflow _x = new Workflow();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.deletestage = deletestage;
_x.iscustomprocessingstepallowedforotherpublishers = iscustomprocessingstepallowedforotherpublishers;
_x.iscustomizable = iscustomizable;
_x.processtriggerscope = processtriggerscope;
_x.processtriggerformid = processtriggerformid;
_x.name = name;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.versionnumber = versionnumber;
_x.entityimage = entityimage;
_x.scope = scope;
_x.inputparameters = inputparameters;
_x._modifiedby_value = _modifiedby_value;
_x.createstage = createstage;
_x.runas = runas;
_x.ondemand = ondemand;
_x.uiflowtype = uiflowtype;
_x.rendererobjecttypecode = rendererobjecttypecode;
_x.workflowid = workflowid;
_x.modifiedon = modifiedon;
_x.entityimage_timestamp = entityimage_timestamp;
_x.syncworkflowlogonfailure = syncworkflowlogonfailure;
_x.createdon = createdon;
_x.iscrmuiworkflow = iscrmuiworkflow;
_x.triggeroncreate = triggeroncreate;
_x.processroleassignment = processroleassignment;
_x.businessprocesstype = businessprocesstype;
_x.formid = formid;
_x.updatestage = updatestage;
_x.rank = rank;
_x.type = type;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.uidata = uidata;
_x._plugintypeid_value = _plugintypeid_value;
_x.category = category;
_x.statecode = statecode;
_x._activeworkflowid_value = _activeworkflowid_value;
_x._owningteam_value = _owningteam_value;
_x.asyncautodelete = asyncautodelete;
_x.triggeronupdateattributelist = triggeronupdateattributelist;
_x.description = description;
_x.uniquename = uniquename;
_x.statuscode = statuscode;
_x.ismanaged = ismanaged;
_x.workflowidunique = workflowidunique;
_x.overwritetime = overwritetime;
_x.processorder = processorder;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x._createdby_value = _createdby_value;
_x.subprocess = subprocess;
_x.entityimage_url = entityimage_url;
_x.solutionid = solutionid;
_x._parentworkflowid_value = _parentworkflowid_value;
_x._owninguser_value = _owninguser_value;
_x.languagecode = languagecode;
_x.introducedversion = introducedversion;
_x.xaml = xaml;
_x.istransacted = istransacted;
_x.componentstate = componentstate;
_x.clientdata = clientdata;
_x.mode = mode;
_x.triggerondelete = triggerondelete;
_x.entityimageid = entityimageid;
_x.primaryentity = primaryentity;
_x._ownerid_value = _ownerid_value;
_x._sdkmessageid_value = _sdkmessageid_value;
return _x;
}
@Action(name = "CreateWorkflowFromTemplate")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped createWorkflowFromTemplate(String workflowName) {
Preconditions.checkNotNull(workflowName, "workflowName cannot be null");
Map _parameters = ParameterMap
.put("WorkflowName", "Edm.String", Checks.checkIsAscii(workflowName))
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.CreateWorkflowFromTemplate"), Workflow.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "ExecuteWorkflow")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped executeWorkflow(String entityId, InputArgumentCollection inputArguments) {
Preconditions.checkNotNull(entityId, "entityId cannot be null");
Map _parameters = ParameterMap
.put("EntityId", "Edm.Guid", entityId)
.put("InputArguments", "Microsoft.Dynamics.CRM.InputArgumentCollection", inputArguments)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.ExecuteWorkflow"), Asyncoperation.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "InitializeModernFlowFromAsyncWorkflow")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped initializeModernFlowFromAsyncWorkflow() {
Map _parameters = ParameterMap.empty();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.InitializeModernFlowFromAsyncWorkflow"), Workflow.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Workflow[");
b.append("deletestage=");
b.append(this.deletestage);
b.append(", ");
b.append("iscustomprocessingstepallowedforotherpublishers=");
b.append(this.iscustomprocessingstepallowedforotherpublishers);
b.append(", ");
b.append("iscustomizable=");
b.append(this.iscustomizable);
b.append(", ");
b.append("processtriggerscope=");
b.append(this.processtriggerscope);
b.append(", ");
b.append("processtriggerformid=");
b.append(this.processtriggerformid);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("scope=");
b.append(this.scope);
b.append(", ");
b.append("inputparameters=");
b.append(this.inputparameters);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("createstage=");
b.append(this.createstage);
b.append(", ");
b.append("runas=");
b.append(this.runas);
b.append(", ");
b.append("ondemand=");
b.append(this.ondemand);
b.append(", ");
b.append("uiflowtype=");
b.append(this.uiflowtype);
b.append(", ");
b.append("rendererobjecttypecode=");
b.append(this.rendererobjecttypecode);
b.append(", ");
b.append("workflowid=");
b.append(this.workflowid);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("syncworkflowlogonfailure=");
b.append(this.syncworkflowlogonfailure);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("iscrmuiworkflow=");
b.append(this.iscrmuiworkflow);
b.append(", ");
b.append("triggeroncreate=");
b.append(this.triggeroncreate);
b.append(", ");
b.append("processroleassignment=");
b.append(this.processroleassignment);
b.append(", ");
b.append("businessprocesstype=");
b.append(this.businessprocesstype);
b.append(", ");
b.append("formid=");
b.append(this.formid);
b.append(", ");
b.append("updatestage=");
b.append(this.updatestage);
b.append(", ");
b.append("rank=");
b.append(this.rank);
b.append(", ");
b.append("type=");
b.append(this.type);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("uidata=");
b.append(this.uidata);
b.append(", ");
b.append("_plugintypeid_value=");
b.append(this._plugintypeid_value);
b.append(", ");
b.append("category=");
b.append(this.category);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("_activeworkflowid_value=");
b.append(this._activeworkflowid_value);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("asyncautodelete=");
b.append(this.asyncautodelete);
b.append(", ");
b.append("triggeronupdateattributelist=");
b.append(this.triggeronupdateattributelist);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("uniquename=");
b.append(this.uniquename);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("workflowidunique=");
b.append(this.workflowidunique);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("processorder=");
b.append(this.processorder);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("subprocess=");
b.append(this.subprocess);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("_parentworkflowid_value=");
b.append(this._parentworkflowid_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("languagecode=");
b.append(this.languagecode);
b.append(", ");
b.append("introducedversion=");
b.append(this.introducedversion);
b.append(", ");
b.append("xaml=");
b.append(this.xaml);
b.append(", ");
b.append("istransacted=");
b.append(this.istransacted);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("clientdata=");
b.append(this.clientdata);
b.append(", ");
b.append("mode=");
b.append(this.mode);
b.append(", ");
b.append("triggerondelete=");
b.append(this.triggerondelete);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("primaryentity=");
b.append(this.primaryentity);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_sdkmessageid_value=");
b.append(this._sdkmessageid_value);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy