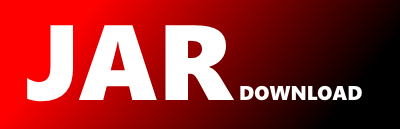
microsoft.dynamics.crm.entity.collection.request.PostCollectionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.collection.request;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.CollectionPageEntityRequest;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.TypedObject;
import java.time.OffsetDateTime;
import java.util.Map;
import microsoft.dynamics.crm.entity.Crmbaseentity;
import microsoft.dynamics.crm.entity.Post;
import microsoft.dynamics.crm.entity.request.AsyncoperationRequest;
import microsoft.dynamics.crm.entity.request.BulkdeletefailureRequest;
import microsoft.dynamics.crm.entity.request.PostRequest;
import microsoft.dynamics.crm.entity.request.PostcommentRequest;
import microsoft.dynamics.crm.entity.request.PostlikeRequest;
import microsoft.dynamics.crm.schema.SchemaInfo;
public class PostCollectionRequest extends CollectionPageEntityRequest{
protected ContextPath contextPath;
public PostCollectionRequest(ContextPath contextPath) {
super(contextPath, Post.class, cp -> new PostRequest(cp), SchemaInfo.INSTANCE);
this.contextPath = contextPath;
}
public AsyncoperationCollectionRequest post_AsyncOperations() {
return new AsyncoperationCollectionRequest(contextPath.addSegment("post_AsyncOperations"));
}
public AsyncoperationRequest post_AsyncOperations(String asyncoperationid) {
return new AsyncoperationRequest(contextPath.addSegment("post_AsyncOperations").addKeys(new NameValue(asyncoperationid.toString())));
}
public BulkdeletefailureCollectionRequest post_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(contextPath.addSegment("post_BulkDeleteFailures"));
}
public BulkdeletefailureRequest post_BulkDeleteFailures(String bulkdeletefailureid) {
return new BulkdeletefailureRequest(contextPath.addSegment("post_BulkDeleteFailures").addKeys(new NameValue(bulkdeletefailureid.toString())));
}
public PostcommentCollectionRequest post_Comments() {
return new PostcommentCollectionRequest(contextPath.addSegment("Post_Comments"));
}
public PostcommentRequest post_Comments(String postcommentid) {
return new PostcommentRequest(contextPath.addSegment("Post_Comments").addKeys(new NameValue(postcommentid.toString())));
}
public PostlikeCollectionRequest post_Likes() {
return new PostlikeCollectionRequest(contextPath.addSegment("Post_Likes"));
}
public PostlikeRequest post_Likes(String postlikeid) {
return new PostlikeRequest(contextPath.addSegment("Post_Likes").addKeys(new NameValue(postlikeid.toString())));
}
@Function(name = "RetrievePersonalWall")
@JsonIgnore
public CollectionPageNonEntityRequest retrievePersonalWall(Integer pageNumber, Integer pageSize, Integer commentsPerPost, OffsetDateTime startDate, OffsetDateTime endDate, Integer type, Integer source, Boolean sortDirection, String keyword) {
Preconditions.checkNotNull(pageNumber, "pageNumber cannot be null");
Preconditions.checkNotNull(pageSize, "pageSize cannot be null");
Preconditions.checkNotNull(commentsPerPost, "commentsPerPost cannot be null");
Preconditions.checkNotNull(startDate, "startDate cannot be null");
Preconditions.checkNotNull(endDate, "endDate cannot be null");
Preconditions.checkNotNull(type, "type cannot be null");
Preconditions.checkNotNull(source, "source cannot be null");
Preconditions.checkNotNull(sortDirection, "sortDirection cannot be null");
Preconditions.checkNotNull(keyword, "keyword cannot be null");
Map _parameters = ParameterMap
.put("PageNumber", "Edm.Int32", pageNumber)
.put("PageSize", "Edm.Int32", pageSize)
.put("CommentsPerPost", "Edm.Int32", commentsPerPost)
.put("StartDate", "Edm.DateTimeOffset", startDate)
.put("EndDate", "Edm.DateTimeOffset", endDate)
.put("Type", "Edm.Int32", type)
.put("Source", "Edm.Int32", source)
.put("SortDirection", "Edm.Boolean", sortDirection)
.put("Keyword", "Edm.String", Checks.checkIsAscii(keyword))
.build();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrievePersonalWall"), Post.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Function(name = "RetrieveRecordWall")
@JsonIgnore
public CollectionPageNonEntityRequest retrieveRecordWall(Crmbaseentity entity, Integer pageNumber, Integer pageSize, Integer commentsPerPost, OffsetDateTime startDate, OffsetDateTime endDate, Integer type, Integer source, Boolean sortDirection, String keyword) {
Preconditions.checkNotNull(entity, "entity cannot be null");
Preconditions.checkNotNull(pageNumber, "pageNumber cannot be null");
Preconditions.checkNotNull(pageSize, "pageSize cannot be null");
Preconditions.checkNotNull(commentsPerPost, "commentsPerPost cannot be null");
Preconditions.checkNotNull(startDate, "startDate cannot be null");
Preconditions.checkNotNull(endDate, "endDate cannot be null");
Preconditions.checkNotNull(type, "type cannot be null");
Preconditions.checkNotNull(source, "source cannot be null");
Preconditions.checkNotNull(sortDirection, "sortDirection cannot be null");
Preconditions.checkNotNull(keyword, "keyword cannot be null");
Map _parameters = ParameterMap
.put("Entity", "Microsoft.Dynamics.CRM.crmbaseentity", entity)
.put("PageNumber", "Edm.Int32", pageNumber)
.put("PageSize", "Edm.Int32", pageSize)
.put("CommentsPerPost", "Edm.Int32", commentsPerPost)
.put("StartDate", "Edm.DateTimeOffset", startDate)
.put("EndDate", "Edm.DateTimeOffset", endDate)
.put("Type", "Edm.Int32", type)
.put("Source", "Edm.Int32", source)
.put("SortDirection", "Edm.Boolean", sortDirection)
.put("Keyword", "Edm.String", Checks.checkIsAscii(keyword))
.build();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveRecordWall"), Post.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy