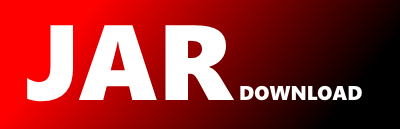
microsoft.dynamics.crm.entity.collection.request.SystemformCollectionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.collection.request;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.CollectionPageEntityRequest;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.TypedObject;
import java.util.Map;
import microsoft.dynamics.crm.entity.Systemform;
import microsoft.dynamics.crm.entity.Systemuser;
import microsoft.dynamics.crm.entity.request.AsyncoperationRequest;
import microsoft.dynamics.crm.entity.request.BulkdeletefailureRequest;
import microsoft.dynamics.crm.entity.request.ProcesstriggerRequest;
import microsoft.dynamics.crm.entity.request.SystemformRequest;
import microsoft.dynamics.crm.schema.SchemaInfo;
public class SystemformCollectionRequest extends CollectionPageEntityRequest{
protected ContextPath contextPath;
public SystemformCollectionRequest(ContextPath contextPath) {
super(contextPath, Systemform.class, cp -> new SystemformRequest(cp), SchemaInfo.INSTANCE);
this.contextPath = contextPath;
}
public AsyncoperationCollectionRequest systemForm_AsyncOperations() {
return new AsyncoperationCollectionRequest(contextPath.addSegment("SystemForm_AsyncOperations"));
}
public AsyncoperationRequest systemForm_AsyncOperations(String asyncoperationid) {
return new AsyncoperationRequest(contextPath.addSegment("SystemForm_AsyncOperations").addKeys(new NameValue(asyncoperationid.toString())));
}
public ProcesstriggerCollectionRequest processtrigger_systemform() {
return new ProcesstriggerCollectionRequest(contextPath.addSegment("processtrigger_systemform"));
}
public ProcesstriggerRequest processtrigger_systemform(String processtriggerid) {
return new ProcesstriggerRequest(contextPath.addSegment("processtrigger_systemform").addKeys(new NameValue(processtriggerid.toString())));
}
public BulkdeletefailureCollectionRequest systemForm_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(contextPath.addSegment("SystemForm_BulkDeleteFailures"));
}
public BulkdeletefailureRequest systemForm_BulkDeleteFailures(String bulkdeletefailureid) {
return new BulkdeletefailureRequest(contextPath.addSegment("SystemForm_BulkDeleteFailures").addKeys(new NameValue(bulkdeletefailureid.toString())));
}
public SystemformCollectionRequest form_ancestor_form() {
return new SystemformCollectionRequest(contextPath.addSegment("form_ancestor_form"));
}
public SystemformRequest form_ancestor_form(String formid) {
return new SystemformRequest(contextPath.addSegment("form_ancestor_form").addKeys(new NameValue(formid.toString())));
}
@Function(name = "RetrieveFilteredForms")
@JsonIgnore
public CollectionPageNonEntityRequest retrieveFilteredForms(String entityLogicalName, Integer formType, Systemuser user) {
Preconditions.checkNotNull(entityLogicalName, "entityLogicalName cannot be null");
Preconditions.checkNotNull(formType, "formType cannot be null");
Preconditions.checkNotNull(user, "user cannot be null");
Map _parameters = ParameterMap
.put("EntityLogicalName", "Edm.String", Checks.checkIsAscii(entityLogicalName))
.put("FormType", "Edm.Int32", formType)
.put("User", "Microsoft.Dynamics.CRM.systemuser", user)
.build();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveFilteredForms"), Systemform.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Function(name = "RetrieveUnpublishedMultiple")
@JsonIgnore
public CollectionPageNonEntityRequest retrieveUnpublishedMultiple() {
Map _parameters = ParameterMap.empty();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUnpublishedMultiple"), Systemform.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy