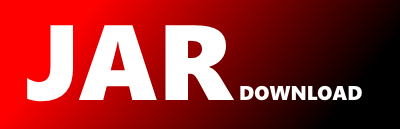
microsoft.dynamics.crm.entity.request.AnnotationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.request;
import com.fasterxml.jackson.annotation.JsonIgnoreType;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.EntityRequest;
import com.github.davidmoten.odata.client.NameValue;
import microsoft.dynamics.crm.entity.Annotation;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonIgnoreType
public class AnnotationRequest extends EntityRequest {
public AnnotationRequest(ContextPath contextPath) {
super(Annotation.class, contextPath, SchemaInfo.INSTANCE);
}
public KnowledgearticleRequest objectid_knowledgearticle() {
return new KnowledgearticleRequest(contextPath.addSegment("objectid_knowledgearticle"));
}
public KnowledgebaserecordRequest objectid_knowledgebaserecord() {
return new KnowledgebaserecordRequest(contextPath.addSegment("objectid_knowledgebaserecord"));
}
public AccountRequest objectid_account() {
return new AccountRequest(contextPath.addSegment("objectid_account"));
}
public SystemuserRequest modifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
public KbarticleRequest objectid_kbarticle() {
return new KbarticleRequest(contextPath.addSegment("objectid_kbarticle"));
}
public AppointmentRequest objectid_appointment() {
return new AppointmentRequest(contextPath.addSegment("objectid_appointment"));
}
public BusinessunitRequest owningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
public BulkdeletefailureCollectionRequest annotation_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Annotation_BulkDeleteFailures"));
}
public BulkdeletefailureRequest annotation_BulkDeleteFailures(String bulkdeletefailureid) {
return new BulkdeletefailureRequest(contextPath.addSegment("Annotation_BulkDeleteFailures").addKeys(new NameValue(bulkdeletefailureid.toString())));
}
public SlaRequest objectid_sla() {
return new SlaRequest(contextPath.addSegment("objectid_sla"));
}
public CalendarRequest objectid_calendar() {
return new CalendarRequest(contextPath.addSegment("objectid_calendar"));
}
public SystemuserRequest createdby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
public FaxRequest objectid_fax() {
return new FaxRequest(contextPath.addSegment("objectid_fax"));
}
public ContactRequest objectid_contact() {
return new ContactRequest(contextPath.addSegment("objectid_contact"));
}
public SystemuserRequest owninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
public LetterRequest objectid_letter() {
return new LetterRequest(contextPath.addSegment("objectid_letter"));
}
public PrincipalRequest ownerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
public GoalRequest objectid_goal() {
return new GoalRequest(contextPath.addSegment("objectid_goal"));
}
public TaskRequest objectid_task() {
return new TaskRequest(contextPath.addSegment("objectid_task"));
}
public EmailRequest objectid_email() {
return new EmailRequest(contextPath.addSegment("objectid_email"));
}
public WorkflowRequest objectid_workflow() {
return new WorkflowRequest(contextPath.addSegment("objectid_workflow"));
}
public SystemuserRequest createdonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
public SystemuserRequest modifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
public AsyncoperationCollectionRequest annotation_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Annotation_AsyncOperations"));
}
public AsyncoperationRequest annotation_AsyncOperations(String asyncoperationid) {
return new AsyncoperationRequest(contextPath.addSegment("Annotation_AsyncOperations").addKeys(new NameValue(asyncoperationid.toString())));
}
public ProcesssessionCollectionRequest annotation_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Annotation_ProcessSessions"));
}
public ProcesssessionRequest annotation_ProcessSessions(String processsessionid) {
return new ProcesssessionRequest(contextPath.addSegment("Annotation_ProcessSessions").addKeys(new NameValue(processsessionid.toString())));
}
public MailboxRequest objectid_mailbox() {
return new MailboxRequest(contextPath.addSegment("objectid_mailbox"));
}
public SocialactivityRequest objectid_socialactivity() {
return new SocialactivityRequest(contextPath.addSegment("objectid_socialactivity"));
}
public TeamRequest owningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
public DuplicateruleRequest objectid_duplicaterule() {
return new DuplicateruleRequest(contextPath.addSegment("objectid_duplicaterule"));
}
public SyncerrorCollectionRequest annotation_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Annotation_SyncErrors"));
}
public SyncerrorRequest annotation_SyncErrors(String syncerrorid) {
return new SyncerrorRequest(contextPath.addSegment("Annotation_SyncErrors").addKeys(new NameValue(syncerrorid.toString())));
}
public PhonecallRequest objectid_phonecall() {
return new PhonecallRequest(contextPath.addSegment("objectid_phonecall"));
}
public EmailserverprofileRequest objectid_emailserverprofile() {
return new EmailserverprofileRequest(contextPath.addSegment("objectid_emailserverprofile"));
}
public RecurringappointmentmasterRequest objectid_recurringappointmentmaster() {
return new RecurringappointmentmasterRequest(contextPath.addSegment("objectid_recurringappointmentmaster"));
}
public Msdyn_aimodelRequest objectid_msdyn_aimodel() {
return new Msdyn_aimodelRequest(contextPath.addSegment("objectid_msdyn_aimodel"));
}
public Msdyn_aifptrainingdocumentRequest objectid_msdyn_aifptrainingdocument() {
return new Msdyn_aifptrainingdocumentRequest(contextPath.addSegment("objectid_msdyn_aifptrainingdocument"));
}
public Msdyn_aiodimageRequest objectid_msdyn_aiodimage() {
return new Msdyn_aiodimageRequest(contextPath.addSegment("objectid_msdyn_aiodimage"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy