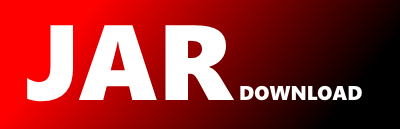
microsoft.dynamics.crm.entity.request.Ggw_teamRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.request;
import com.fasterxml.jackson.annotation.JsonIgnoreType;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.EntityRequest;
import com.github.davidmoten.odata.client.NameValue;
import microsoft.dynamics.crm.entity.Ggw_team;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_crewCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_team_applicationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonIgnoreType
public class Ggw_teamRequest extends EntityRequest {
public Ggw_teamRequest(ContextPath contextPath) {
super(Ggw_team.class, contextPath, SchemaInfo.INSTANCE);
}
public SystemuserRequest createdby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
public SystemuserRequest createdonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
public SystemuserRequest modifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
public SystemuserRequest modifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
public SystemuserRequest owninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
public TeamRequest owningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
public PrincipalRequest ownerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
public BusinessunitRequest owningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
public SyncerrorCollectionRequest ggw_team_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("ggw_team_SyncErrors"));
}
public SyncerrorRequest ggw_team_SyncErrors(String syncerrorid) {
return new SyncerrorRequest(contextPath.addSegment("ggw_team_SyncErrors").addKeys(new NameValue(syncerrorid.toString())));
}
public DuplicaterecordCollectionRequest ggw_team_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("ggw_team_DuplicateMatchingRecord"));
}
public DuplicaterecordRequest ggw_team_DuplicateMatchingRecord(String duplicateid) {
return new DuplicaterecordRequest(contextPath.addSegment("ggw_team_DuplicateMatchingRecord").addKeys(new NameValue(duplicateid.toString())));
}
public DuplicaterecordCollectionRequest ggw_team_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("ggw_team_DuplicateBaseRecord"));
}
public DuplicaterecordRequest ggw_team_DuplicateBaseRecord(String duplicateid) {
return new DuplicaterecordRequest(contextPath.addSegment("ggw_team_DuplicateBaseRecord").addKeys(new NameValue(duplicateid.toString())));
}
public AsyncoperationCollectionRequest ggw_team_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("ggw_team_AsyncOperations"));
}
public AsyncoperationRequest ggw_team_AsyncOperations(String asyncoperationid) {
return new AsyncoperationRequest(contextPath.addSegment("ggw_team_AsyncOperations").addKeys(new NameValue(asyncoperationid.toString())));
}
public MailboxtrackingfolderCollectionRequest ggw_team_MailboxTrackingFolders() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("ggw_team_MailboxTrackingFolders"));
}
public MailboxtrackingfolderRequest ggw_team_MailboxTrackingFolders(String mailboxtrackingfolderid) {
return new MailboxtrackingfolderRequest(contextPath.addSegment("ggw_team_MailboxTrackingFolders").addKeys(new NameValue(mailboxtrackingfolderid.toString())));
}
public ProcesssessionCollectionRequest ggw_team_ProcessSession() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("ggw_team_ProcessSession"));
}
public ProcesssessionRequest ggw_team_ProcessSession(String processsessionid) {
return new ProcesssessionRequest(contextPath.addSegment("ggw_team_ProcessSession").addKeys(new NameValue(processsessionid.toString())));
}
public BulkdeletefailureCollectionRequest ggw_team_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("ggw_team_BulkDeleteFailures"));
}
public BulkdeletefailureRequest ggw_team_BulkDeleteFailures(String bulkdeletefailureid) {
return new BulkdeletefailureRequest(contextPath.addSegment("ggw_team_BulkDeleteFailures").addKeys(new NameValue(bulkdeletefailureid.toString())));
}
public PrincipalobjectattributeaccessCollectionRequest ggw_team_PrincipalObjectAttributeAccesses() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("ggw_team_PrincipalObjectAttributeAccesses"));
}
public PrincipalobjectattributeaccessRequest ggw_team_PrincipalObjectAttributeAccesses(String principalobjectattributeaccessid) {
return new PrincipalobjectattributeaccessRequest(contextPath.addSegment("ggw_team_PrincipalObjectAttributeAccesses").addKeys(new NameValue(principalobjectattributeaccessid.toString())));
}
public Ggw_eventRequest ggw_EventId() {
return new Ggw_eventRequest(contextPath.addSegment("ggw_EventId"));
}
public Ggw_crewCollectionRequest ggw_ggw_crew_ggw_team() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("ggw_ggw_crew_ggw_team"));
}
public Ggw_crewRequest ggw_ggw_crew_ggw_team(String ggw_crewid) {
return new Ggw_crewRequest(contextPath.addSegment("ggw_ggw_crew_ggw_team").addKeys(new NameValue(ggw_crewid.toString())));
}
public Ggw_team_applicationCollectionRequest ggw_ggw_team_ggw_team_application() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("ggw_ggw_team_ggw_team_application"));
}
public Ggw_team_applicationRequest ggw_ggw_team_ggw_team_application(String ggw_team_applicationid) {
return new Ggw_team_applicationRequest(contextPath.addSegment("ggw_ggw_team_ggw_team_application").addKeys(new NameValue(ggw_team_applicationid.toString())));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy