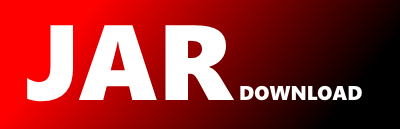
microsoft.dynamics.crm.entity.request.WorkflowRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.request;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonIgnoreType;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.EntityRequest;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.TypedObject;
import java.util.Map;
import microsoft.dynamics.crm.complex.InputArgumentCollection;
import microsoft.dynamics.crm.entity.Asyncoperation;
import microsoft.dynamics.crm.entity.Workflow;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExpiredprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FlowsessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aimodelCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_solutionhealthruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.NewprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcessstageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesstriggerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TranslationprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowbinaryCollectionRequest;
import microsoft.dynamics.crm.schema.SchemaInfo;
@JsonIgnoreType
public class WorkflowRequest extends EntityRequest {
public WorkflowRequest(ContextPath contextPath) {
super(Workflow.class, contextPath, SchemaInfo.INSTANCE);
}
public SlaCollectionRequest slabase_workflowid() {
return new SlaCollectionRequest(
contextPath.addSegment("slabase_workflowid"));
}
public SlaRequest slabase_workflowid(String slaid) {
return new SlaRequest(contextPath.addSegment("slabase_workflowid").addKeys(new NameValue(slaid.toString())));
}
public BusinessunitRequest owningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"));
}
public SystemuserRequest createdonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"));
}
public PrincipalRequest ownerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"));
}
public AsyncoperationCollectionRequest lk_asyncoperation_workflowactivationid() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_workflowactivationid"));
}
public AsyncoperationRequest lk_asyncoperation_workflowactivationid(String asyncoperationid) {
return new AsyncoperationRequest(contextPath.addSegment("lk_asyncoperation_workflowactivationid").addKeys(new NameValue(asyncoperationid.toString())));
}
public WorkflowRequest parentworkflowid() {
return new WorkflowRequest(contextPath.addSegment("parentworkflowid"));
}
public WorkflowCollectionRequest workflow_parent_workflow() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_parent_workflow"));
}
public WorkflowRequest workflow_parent_workflow(String workflowid) {
return new WorkflowRequest(contextPath.addSegment("workflow_parent_workflow").addKeys(new NameValue(workflowid.toString())));
}
public ExpiredprocessCollectionRequest workflow_expiredprocess() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("workflow_expiredprocess"));
}
public ExpiredprocessRequest workflow_expiredprocess(String businessprocessflowinstanceid) {
return new ExpiredprocessRequest(contextPath.addSegment("workflow_expiredprocess").addKeys(new NameValue(businessprocessflowinstanceid.toString())));
}
public SlaitemCollectionRequest slaitembase_workflowid() {
return new SlaitemCollectionRequest(
contextPath.addSegment("slaitembase_workflowid"));
}
public SlaitemRequest slaitembase_workflowid(String slaitemid) {
return new SlaitemRequest(contextPath.addSegment("slaitembase_workflowid").addKeys(new NameValue(slaitemid.toString())));
}
public TranslationprocessCollectionRequest workflow_translationprocess() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("workflow_translationprocess"));
}
public TranslationprocessRequest workflow_translationprocess(String businessprocessflowinstanceid) {
return new TranslationprocessRequest(contextPath.addSegment("workflow_translationprocess").addKeys(new NameValue(businessprocessflowinstanceid.toString())));
}
public ProcesstriggerCollectionRequest process_processtrigger() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("process_processtrigger"));
}
public ProcesstriggerRequest process_processtrigger(String processtriggerid) {
return new ProcesstriggerRequest(contextPath.addSegment("process_processtrigger").addKeys(new NameValue(processtriggerid.toString())));
}
public TeamRequest owningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"));
}
public AnnotationCollectionRequest workflow_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Workflow_Annotation"));
}
public AnnotationRequest workflow_Annotation(String annotationid) {
return new AnnotationRequest(contextPath.addSegment("Workflow_Annotation").addKeys(new NameValue(annotationid.toString())));
}
public ProcesssessionCollectionRequest lk_processsession_processid() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_processid"));
}
public ProcesssessionRequest lk_processsession_processid(String processsessionid) {
return new ProcesssessionRequest(contextPath.addSegment("lk_processsession_processid").addKeys(new NameValue(processsessionid.toString())));
}
public ProcessstageCollectionRequest process_processstage() {
return new ProcessstageCollectionRequest(
contextPath.addSegment("process_processstage"));
}
public ProcessstageRequest process_processstage(String processstageid) {
return new ProcessstageRequest(contextPath.addSegment("process_processstage").addKeys(new NameValue(processstageid.toString())));
}
public SyncerrorCollectionRequest workflow_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Workflow_SyncErrors"));
}
public SyncerrorRequest workflow_SyncErrors(String syncerrorid) {
return new SyncerrorRequest(contextPath.addSegment("Workflow_SyncErrors").addKeys(new NameValue(syncerrorid.toString())));
}
public NewprocessCollectionRequest workflow_newprocess() {
return new NewprocessCollectionRequest(
contextPath.addSegment("workflow_newprocess"));
}
public NewprocessRequest workflow_newprocess(String businessprocessflowinstanceid) {
return new NewprocessRequest(contextPath.addSegment("workflow_newprocess").addKeys(new NameValue(businessprocessflowinstanceid.toString())));
}
public WorkflowRequest activeworkflowid() {
return new WorkflowRequest(contextPath.addSegment("activeworkflowid"));
}
public WorkflowCollectionRequest workflow_active_workflow() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_active_workflow"));
}
public WorkflowRequest workflow_active_workflow(String workflowid) {
return new WorkflowRequest(contextPath.addSegment("workflow_active_workflow").addKeys(new NameValue(workflowid.toString())));
}
public SystemuserRequest modifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"));
}
public SystemuserRequest createdby() {
return new SystemuserRequest(contextPath.addSegment("createdby"));
}
public SystemuserRequest modifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"));
}
public SystemuserRequest owninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"));
}
public FlowsessionCollectionRequest regardingobjectid_process() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("regardingobjectid_process"));
}
public FlowsessionRequest regardingobjectid_process(String flowsessionid) {
return new FlowsessionRequest(contextPath.addSegment("regardingobjectid_process").addKeys(new NameValue(flowsessionid.toString())));
}
public WorkflowbinaryCollectionRequest workflow_workflowbinary_Process() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("workflow_workflowbinary_Process"));
}
public WorkflowbinaryRequest workflow_workflowbinary_Process(String workflowbinaryid) {
return new WorkflowbinaryRequest(contextPath.addSegment("workflow_workflowbinary_Process").addKeys(new NameValue(workflowbinaryid.toString())));
}
public Msdyn_aimodelCollectionRequest msdyn_retrainworkflow_msdyn_toaimodel() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("msdyn_retrainworkflow_msdyn_toaimodel"));
}
public Msdyn_aimodelRequest msdyn_retrainworkflow_msdyn_toaimodel(String msdyn_aimodelid) {
return new Msdyn_aimodelRequest(contextPath.addSegment("msdyn_retrainworkflow_msdyn_toaimodel").addKeys(new NameValue(msdyn_aimodelid.toString())));
}
public Msdyn_solutionhealthruleCollectionRequest msdyn_workflow_msdyn_solutionhealthrule_Workflow() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_Workflow"));
}
public Msdyn_solutionhealthruleRequest msdyn_workflow_msdyn_solutionhealthrule_Workflow(String msdyn_solutionhealthruleid) {
return new Msdyn_solutionhealthruleRequest(contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_Workflow").addKeys(new NameValue(msdyn_solutionhealthruleid.toString())));
}
public Msdyn_solutionhealthruleCollectionRequest msdyn_workflow_msdyn_solutionhealthrule_resolutionaction() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_resolutionaction"));
}
public Msdyn_solutionhealthruleRequest msdyn_workflow_msdyn_solutionhealthrule_resolutionaction(String msdyn_solutionhealthruleid) {
return new Msdyn_solutionhealthruleRequest(contextPath.addSegment("msdyn_workflow_msdyn_solutionhealthrule_resolutionaction").addKeys(new NameValue(msdyn_solutionhealthruleid.toString())));
}
public Msdyn_aimodelCollectionRequest msdyn_scheduleinferenceworkflow_msdyn_toaimodel() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("msdyn_scheduleinferenceworkflow_msdyn_toaimodel"));
}
public Msdyn_aimodelRequest msdyn_scheduleinferenceworkflow_msdyn_toaimodel(String msdyn_aimodelid) {
return new Msdyn_aimodelRequest(contextPath.addSegment("msdyn_scheduleinferenceworkflow_msdyn_toaimodel").addKeys(new NameValue(msdyn_aimodelid.toString())));
}
@Action(name = "CreateWorkflowFromTemplate")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped createWorkflowFromTemplate(String workflowName) {
Preconditions.checkNotNull(workflowName, "workflowName cannot be null");
Map _parameters = ParameterMap
.put("WorkflowName", "Edm.String", Checks.checkIsAscii(workflowName))
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.CreateWorkflowFromTemplate"), Workflow.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "ExecuteWorkflow")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped executeWorkflow(String entityId, InputArgumentCollection inputArguments) {
Preconditions.checkNotNull(entityId, "entityId cannot be null");
Map _parameters = ParameterMap
.put("EntityId", "Edm.Guid", entityId)
.put("InputArguments", "Microsoft.Dynamics.CRM.InputArgumentCollection", inputArguments)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.ExecuteWorkflow"), Asyncoperation.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
@Action(name = "InitializeModernFlowFromAsyncWorkflow")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped initializeModernFlowFromAsyncWorkflow() {
Map _parameters = ParameterMap.empty();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.InitializeModernFlowFromAsyncWorkflow"), Workflow.class, _parameters, microsoft.dynamics.crm.schema.SchemaInfo.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy