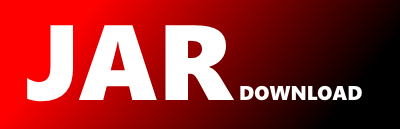
microsoft.dynamics.crm.entity.set.Connections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
public final class Connections extends ConnectionCollectionRequest {
public Connections(ContextPath contextPath) {
super(contextPath);
}
public Asyncoperations connection_AsyncOperations() {
return new Asyncoperations(contextPath.addSegment("Connection_AsyncOperations"));
}
public Principalobjectattributeaccessset connection_principalobjectattributeaccess() {
return new Principalobjectattributeaccessset(contextPath.addSegment("connection_principalobjectattributeaccess"));
}
public Processsessions connection_ProcessSessions() {
return new Processsessions(contextPath.addSegment("Connection_ProcessSessions"));
}
public Connections connection_related_connection() {
return new Connections(contextPath.addSegment("connection_related_connection"));
}
public Syncerrors connection_SyncErrors() {
return new Syncerrors(contextPath.addSegment("Connection_SyncErrors"));
}
public Systemusers createdby() {
return new Systemusers(contextPath.addSegment("createdby"));
}
public Systemusers createdonbehalfby() {
return new Systemusers(contextPath.addSegment("createdonbehalfby"));
}
public Systemusers modifiedby() {
return new Systemusers(contextPath.addSegment("modifiedby"));
}
public Systemusers modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("modifiedonbehalfby"));
}
public Owners ownerid() {
return new Owners(contextPath.addSegment("ownerid"));
}
public Businessunits owningbusinessunit() {
return new Businessunits(contextPath.addSegment("owningbusinessunit"));
}
public Accounts record1id_account() {
return new Accounts(contextPath.addSegment("record1id_account"));
}
public Activitypointers record1id_activitypointer() {
return new Activitypointers(contextPath.addSegment("record1id_activitypointer"));
}
public Appointments record1id_appointment() {
return new Appointments(contextPath.addSegment("record1id_appointment"));
}
public Contacts record1id_contact() {
return new Contacts(contextPath.addSegment("record1id_contact"));
}
public Emails record1id_email() {
return new Emails(contextPath.addSegment("record1id_email"));
}
public Faxes record1id_fax() {
return new Faxes(contextPath.addSegment("record1id_fax"));
}
public Goals record1id_goal() {
return new Goals(contextPath.addSegment("record1id_goal"));
}
public Knowledgearticles record1id_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("record1id_knowledgearticle"));
}
public Knowledgebaserecords record1id_knowledgebaserecord() {
return new Knowledgebaserecords(contextPath.addSegment("record1id_knowledgebaserecord"));
}
public Letters record1id_letter() {
return new Letters(contextPath.addSegment("record1id_letter"));
}
public Phonecalls record1id_phonecall() {
return new Phonecalls(contextPath.addSegment("record1id_phonecall"));
}
public Positions record1id_position() {
return new Positions(contextPath.addSegment("record1id_position"));
}
public Processsessions record1id_processsession() {
return new Processsessions(contextPath.addSegment("record1id_processsession"));
}
public Recurringappointmentmasters record1id_recurringappointmentmaster() {
return new Recurringappointmentmasters(contextPath.addSegment("record1id_recurringappointmentmaster"));
}
public Socialactivities record1id_socialactivity() {
return new Socialactivities(contextPath.addSegment("record1id_socialactivity"));
}
public Socialprofiles record1id_socialprofile() {
return new Socialprofiles(contextPath.addSegment("record1id_socialprofile"));
}
public Systemusers record1id_systemuser() {
return new Systemusers(contextPath.addSegment("record1id_systemuser"));
}
public Tasks record1id_task() {
return new Tasks(contextPath.addSegment("record1id_task"));
}
public Teams record1id_team() {
return new Teams(contextPath.addSegment("record1id_team"));
}
public Territories record1id_territory() {
return new Territories(contextPath.addSegment("record1id_territory"));
}
public Connectionroles record1roleid() {
return new Connectionroles(contextPath.addSegment("record1roleid"));
}
public Accounts record2id_account() {
return new Accounts(contextPath.addSegment("record2id_account"));
}
public Activitypointers record2id_activitypointer() {
return new Activitypointers(contextPath.addSegment("record2id_activitypointer"));
}
public Appointments record2id_appointment() {
return new Appointments(contextPath.addSegment("record2id_appointment"));
}
public Contacts record2id_contact() {
return new Contacts(contextPath.addSegment("record2id_contact"));
}
public Emails record2id_email() {
return new Emails(contextPath.addSegment("record2id_email"));
}
public Faxes record2id_fax() {
return new Faxes(contextPath.addSegment("record2id_fax"));
}
public Goals record2id_goal() {
return new Goals(contextPath.addSegment("record2id_goal"));
}
public Knowledgearticles record2id_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("record2id_knowledgearticle"));
}
public Knowledgebaserecords record2id_knowledgebaserecord() {
return new Knowledgebaserecords(contextPath.addSegment("record2id_knowledgebaserecord"));
}
public Letters record2id_letter() {
return new Letters(contextPath.addSegment("record2id_letter"));
}
public Phonecalls record2id_phonecall() {
return new Phonecalls(contextPath.addSegment("record2id_phonecall"));
}
public Positions record2id_position() {
return new Positions(contextPath.addSegment("record2id_position"));
}
public Processsessions record2id_processsession() {
return new Processsessions(contextPath.addSegment("record2id_processsession"));
}
public Recurringappointmentmasters record2id_recurringappointmentmaster() {
return new Recurringappointmentmasters(contextPath.addSegment("record2id_recurringappointmentmaster"));
}
public Socialactivities record2id_socialactivity() {
return new Socialactivities(contextPath.addSegment("record2id_socialactivity"));
}
public Socialprofiles record2id_socialprofile() {
return new Socialprofiles(contextPath.addSegment("record2id_socialprofile"));
}
public Systemusers record2id_systemuser() {
return new Systemusers(contextPath.addSegment("record2id_systemuser"));
}
public Tasks record2id_task() {
return new Tasks(contextPath.addSegment("record2id_task"));
}
public Teams record2id_team() {
return new Teams(contextPath.addSegment("record2id_team"));
}
public Territories record2id_territory() {
return new Territories(contextPath.addSegment("record2id_territory"));
}
public Connectionroles record2roleid() {
return new Connectionroles(contextPath.addSegment("record2roleid"));
}
public Connections relatedconnectionid() {
return new Connections(contextPath.addSegment("relatedconnectionid"));
}
public Transactioncurrencies transactioncurrencyid() {
return new Transactioncurrencies(contextPath.addSegment("transactioncurrencyid"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy