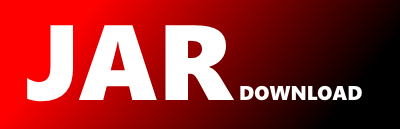
microsoft.dynamics.crm.entity.set.Knowledgearticles Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import microsoft.dynamics.crm.entity.collection.request.KnowledgearticleCollectionRequest;
public final class Knowledgearticles extends KnowledgearticleCollectionRequest {
public Knowledgearticles(ContextPath contextPath) {
super(contextPath);
}
public Systemusers createdby() {
return new Systemusers(contextPath.addSegment("createdby"));
}
public Systemusers createdonbehalfby() {
return new Systemusers(contextPath.addSegment("createdonbehalfby"));
}
public Activityparties knowledgearticle_activity_parties() {
return new Activityparties(contextPath.addSegment("knowledgearticle_activity_parties"));
}
public Activitypointers knowledgeArticle_ActivityPointers() {
return new Activitypointers(contextPath.addSegment("KnowledgeArticle_ActivityPointers"));
}
public Annotations knowledgearticle_Annotations() {
return new Annotations(contextPath.addSegment("knowledgearticle_Annotations"));
}
public Appointments knowledgeArticle_Appointments() {
return new Appointments(contextPath.addSegment("KnowledgeArticle_Appointments"));
}
public Asyncoperations knowledgearticle_AsyncOperations() {
return new Asyncoperations(contextPath.addSegment("knowledgearticle_AsyncOperations"));
}
public Bulkdeletefailures knowledgearticle_BulkDeleteFailures() {
return new Bulkdeletefailures(contextPath.addSegment("knowledgearticle_BulkDeleteFailures"));
}
public Categories knowledgearticle_category() {
return new Categories(contextPath.addSegment("knowledgearticle_category"));
}
public Connections knowledgearticle_connections1() {
return new Connections(contextPath.addSegment("knowledgearticle_connections1"));
}
public Connections knowledgearticle_connections2() {
return new Connections(contextPath.addSegment("knowledgearticle_connections2"));
}
public Duplicaterecords knowledgearticle_DuplicateBaseRecord() {
return new Duplicaterecords(contextPath.addSegment("knowledgearticle_DuplicateBaseRecord"));
}
public Duplicaterecords knowledgearticle_DuplicateMatchingRecord() {
return new Duplicaterecords(contextPath.addSegment("knowledgearticle_DuplicateMatchingRecord"));
}
public Emails knowledgeArticle_Emails() {
return new Emails(contextPath.addSegment("KnowledgeArticle_Emails"));
}
public Expiredprocesses knowledgearticle_expiredprocess() {
return new Expiredprocesses(contextPath.addSegment("knowledgearticle_expiredprocess"));
}
public Faxes knowledgeArticle_Faxes() {
return new Faxes(contextPath.addSegment("KnowledgeArticle_Faxes"));
}
public Feedback knowledgeArticle_Feedback() {
return new Feedback(contextPath.addSegment("KnowledgeArticle_Feedback"));
}
public Letters knowledgeArticle_Letters() {
return new Letters(contextPath.addSegment("KnowledgeArticle_Letters"));
}
public Newprocesses knowledgearticle_newprocess() {
return new Newprocesses(contextPath.addSegment("knowledgearticle_newprocess"));
}
public Knowledgearticles knowledgearticle_parentarticle_contentid() {
return new Knowledgearticles(contextPath.addSegment("knowledgearticle_parentarticle_contentid"));
}
public Phonecalls knowledgeArticle_Phonecalls() {
return new Phonecalls(contextPath.addSegment("KnowledgeArticle_Phonecalls"));
}
public Postfollows knowledgearticle_PostFollows() {
return new Postfollows(contextPath.addSegment("knowledgearticle_PostFollows"));
}
public Postregardings knowledgearticle_PostRegardings() {
return new Postregardings(contextPath.addSegment("knowledgearticle_PostRegardings"));
}
public Knowledgearticles knowledgearticle_previousarticle_contentid() {
return new Knowledgearticles(contextPath.addSegment("knowledgearticle_previousarticle_contentid"));
}
public Principalobjectattributeaccessset knowledgearticle_PrincipalObjectAttributeAccess() {
return new Principalobjectattributeaccessset(contextPath.addSegment("knowledgearticle_PrincipalObjectAttributeAccess"));
}
public Processsessions knowledgearticle_ProcessSession() {
return new Processsessions(contextPath.addSegment("knowledgearticle_ProcessSession"));
}
public Queueitems knowledgearticle_QueueItems() {
return new Queueitems(contextPath.addSegment("knowledgearticle_QueueItems"));
}
public Recurringappointmentmasters knowledgeArticle_RecurringAppointmentMasters() {
return new Recurringappointmentmasters(contextPath.addSegment("KnowledgeArticle_RecurringAppointmentMasters"));
}
public Knowledgearticles knowledgearticle_rootarticle_id() {
return new Knowledgearticles(contextPath.addSegment("knowledgearticle_rootarticle_id"));
}
public Sharepointdocumentlocations knowledgearticle_SharePointDocumentLocations() {
return new Sharepointdocumentlocations(contextPath.addSegment("knowledgearticle_SharePointDocumentLocations"));
}
public Socialactivities knowledgeArticle_SocialActivities() {
return new Socialactivities(contextPath.addSegment("KnowledgeArticle_SocialActivities"));
}
public Syncerrors knowledgeArticle_SyncErrors() {
return new Syncerrors(contextPath.addSegment("KnowledgeArticle_SyncErrors"));
}
public Tasks knowledgeArticle_Tasks() {
return new Tasks(contextPath.addSegment("KnowledgeArticle_Tasks"));
}
public Teams knowledgearticle_Teams() {
return new Teams(contextPath.addSegment("knowledgearticle_Teams"));
}
public Translationprocesses knowledgearticle_translationprocess() {
return new Translationprocesses(contextPath.addSegment("knowledgearticle_translationprocess"));
}
public Knowledgearticleviews knowledgearticle_views() {
return new Knowledgearticleviews(contextPath.addSegment("knowledgearticle_views"));
}
public Languagelocale languagelocaleid() {
return new Languagelocale(contextPath.addSegment("languagelocaleid"));
}
public Systemusers modifiedby() {
return new Systemusers(contextPath.addSegment("modifiedby"));
}
public Systemusers modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("modifiedonbehalfby"));
}
public Owners ownerid() {
return new Owners(contextPath.addSegment("ownerid"));
}
public Businessunits owningbusinessunit() {
return new Businessunits(contextPath.addSegment("owningbusinessunit"));
}
public Teams owningteam() {
return new Teams(contextPath.addSegment("owningteam"));
}
public Systemusers owninguser() {
return new Systemusers(contextPath.addSegment("owninguser"));
}
public Knowledgearticles parentArticleContentId() {
return new Knowledgearticles(contextPath.addSegment("ParentArticleContentId"));
}
public Knowledgearticles previousArticleContentId() {
return new Knowledgearticles(contextPath.addSegment("PreviousArticleContentId"));
}
public Systemusers primaryauthorid() {
return new Systemusers(contextPath.addSegment("primaryauthorid"));
}
public Knowledgearticles rootArticleId() {
return new Knowledgearticles(contextPath.addSegment("RootArticleId"));
}
public Processstages stageid_processstage() {
return new Processstages(contextPath.addSegment("stageid_processstage"));
}
public Subjects subjectid() {
return new Subjects(contextPath.addSegment("subjectid"));
}
public Transactioncurrencies transactioncurrencyid() {
return new Transactioncurrencies(contextPath.addSegment("transactioncurrencyid"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy