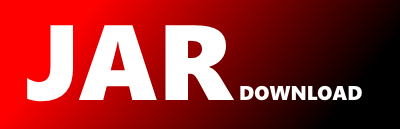
microsoft.dynamics.crm.entity.set.Principalobjectattributeaccessset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
public final class Principalobjectattributeaccessset extends PrincipalobjectattributeaccessCollectionRequest {
public Principalobjectattributeaccessset(ContextPath contextPath) {
super(contextPath);
}
public Accounts objectid_account() {
return new Accounts(contextPath.addSegment("objectid_account"));
}
public Applicationusers objectid_applicationuser() {
return new Applicationusers(contextPath.addSegment("objectid_applicationuser"));
}
public Appointments objectid_appointment() {
return new Appointments(contextPath.addSegment("objectid_appointment"));
}
public Attributeimageconfigs objectid_attributeimageconfig() {
return new Attributeimageconfigs(contextPath.addSegment("objectid_attributeimageconfig"));
}
public Businessunits objectid_businessunit() {
return new Businessunits(contextPath.addSegment("objectid_businessunit"));
}
public Cascadegrantrevokeaccessrecordstrackers objectid_cascadegrantrevokeaccessrecordstracker() {
return new Cascadegrantrevokeaccessrecordstrackers(contextPath.addSegment("objectid_cascadegrantrevokeaccessrecordstracker"));
}
public Cascadegrantrevokeaccessversiontrackers objectid_cascadegrantrevokeaccessversiontracker() {
return new Cascadegrantrevokeaccessversiontrackers(contextPath.addSegment("objectid_cascadegrantrevokeaccessversiontracker"));
}
public Connections objectid_connection() {
return new Connections(contextPath.addSegment("objectid_connection"));
}
public Connectionreferences objectid_connectionreference() {
return new Connectionreferences(contextPath.addSegment("objectid_connectionreference"));
}
public Connectors objectid_connector() {
return new Connectors(contextPath.addSegment("objectid_connector"));
}
public Contacts objectid_contact() {
return new Contacts(contextPath.addSegment("objectid_contact"));
}
public Customeraddresses objectid_customeraddress() {
return new Customeraddresses(contextPath.addSegment("objectid_customeraddress"));
}
public Datalakeworkspaces objectid_datalakeworkspace() {
return new Datalakeworkspaces(contextPath.addSegment("objectid_datalakeworkspace"));
}
public Datalakeworkspacepermissions objectid_datalakeworkspacepermission() {
return new Datalakeworkspacepermissions(contextPath.addSegment("objectid_datalakeworkspacepermission"));
}
public Emails objectid_email() {
return new Emails(contextPath.addSegment("objectid_email"));
}
public Entityanalyticsconfigs objectid_entityanalyticsconfig() {
return new Entityanalyticsconfigs(contextPath.addSegment("objectid_entityanalyticsconfig"));
}
public Entityimageconfigs objectid_entityimageconfig() {
return new Entityimageconfigs(contextPath.addSegment("objectid_entityimageconfig"));
}
public Environmentvariabledefinitions objectid_environmentvariabledefinition() {
return new Environmentvariabledefinitions(contextPath.addSegment("objectid_environmentvariabledefinition"));
}
public Environmentvariablevalues objectid_environmentvariablevalue() {
return new Environmentvariablevalues(contextPath.addSegment("objectid_environmentvariablevalue"));
}
public Exportsolutionuploads objectid_exportsolutionupload() {
return new Exportsolutionuploads(contextPath.addSegment("objectid_exportsolutionupload"));
}
public Faxes objectid_fax() {
return new Faxes(contextPath.addSegment("objectid_fax"));
}
public Feedback objectid_feedback() {
return new Feedback(contextPath.addSegment("objectid_feedback"));
}
public Flowsessions objectid_flowsession() {
return new Flowsessions(contextPath.addSegment("objectid_flowsession"));
}
public Ggw_crews objectid_ggw_crew() {
return new Ggw_crews(contextPath.addSegment("objectid_ggw_crew"));
}
public Ggw_events objectid_ggw_event() {
return new Ggw_events(contextPath.addSegment("objectid_ggw_event"));
}
public Ggw_teams objectid_ggw_team() {
return new Ggw_teams(contextPath.addSegment("objectid_ggw_team"));
}
public Ggw_team_applications objectid_ggw_team_application() {
return new Ggw_team_applications(contextPath.addSegment("objectid_ggw_team_application"));
}
public Goals objectid_goal() {
return new Goals(contextPath.addSegment("objectid_goal"));
}
public Kbarticles objectid_kbarticle() {
return new Kbarticles(contextPath.addSegment("objectid_kbarticle"));
}
public Knowledgearticles objectid_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("objectid_knowledgearticle"));
}
public Knowledgearticleviews objectid_knowledgearticleviews() {
return new Knowledgearticleviews(contextPath.addSegment("objectid_knowledgearticleviews"));
}
public Knowledgebaserecords objectid_knowledgebaserecord() {
return new Knowledgebaserecords(contextPath.addSegment("objectid_knowledgebaserecord"));
}
public Letters objectid_letter() {
return new Letters(contextPath.addSegment("objectid_letter"));
}
public Mailmergetemplates objectid_mailmergetemplate() {
return new Mailmergetemplates(contextPath.addSegment("objectid_mailmergetemplate"));
}
public Msdyn_aibdatasets objectid_msdyn_aibdataset() {
return new Msdyn_aibdatasets(contextPath.addSegment("objectid_msdyn_aibdataset"));
}
public Msdyn_aibdatasetfiles objectid_msdyn_aibdatasetfile() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("objectid_msdyn_aibdatasetfile"));
}
public Msdyn_aibdatasetrecords objectid_msdyn_aibdatasetrecord() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("objectid_msdyn_aibdatasetrecord"));
}
public Msdyn_aibdatasetscontainers objectid_msdyn_aibdatasetscontainer() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("objectid_msdyn_aibdatasetscontainer"));
}
public Msdyn_aibfiles objectid_msdyn_aibfile() {
return new Msdyn_aibfiles(contextPath.addSegment("objectid_msdyn_aibfile"));
}
public Msdyn_aibfileattacheddatas objectid_msdyn_aibfileattacheddata() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("objectid_msdyn_aibfileattacheddata"));
}
public Msdyn_aiconfigurations objectid_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurations(contextPath.addSegment("objectid_msdyn_aiconfiguration"));
}
public Msdyn_aifptrainingdocuments objectid_msdyn_aifptrainingdocument() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("objectid_msdyn_aifptrainingdocument"));
}
public Msdyn_aimodels objectid_msdyn_aimodel() {
return new Msdyn_aimodels(contextPath.addSegment("objectid_msdyn_aimodel"));
}
public Msdyn_aiodimages objectid_msdyn_aiodimage() {
return new Msdyn_aiodimages(contextPath.addSegment("objectid_msdyn_aiodimage"));
}
public Msdyn_aiodlabels objectid_msdyn_aiodlabel() {
return new Msdyn_aiodlabels(contextPath.addSegment("objectid_msdyn_aiodlabel"));
}
public Msdyn_aiodtrainingboundingboxes objectid_msdyn_aiodtrainingboundingbox() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("objectid_msdyn_aiodtrainingboundingbox"));
}
public Msdyn_aiodtrainingimages objectid_msdyn_aiodtrainingimage() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("objectid_msdyn_aiodtrainingimage"));
}
public Msdyn_aitemplates objectid_msdyn_aitemplate() {
return new Msdyn_aitemplates(contextPath.addSegment("objectid_msdyn_aitemplate"));
}
public Msdyn_analysiscomponents objectid_msdyn_analysiscomponent() {
return new Msdyn_analysiscomponents(contextPath.addSegment("objectid_msdyn_analysiscomponent"));
}
public Msdyn_analysisjobs objectid_msdyn_analysisjob() {
return new Msdyn_analysisjobs(contextPath.addSegment("objectid_msdyn_analysisjob"));
}
public Msdyn_analysisresults objectid_msdyn_analysisresult() {
return new Msdyn_analysisresults(contextPath.addSegment("objectid_msdyn_analysisresult"));
}
public Msdyn_analysisresultdetails objectid_msdyn_analysisresultdetail() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("objectid_msdyn_analysisresultdetail"));
}
public Msdyn_dataflows objectid_msdyn_dataflow() {
return new Msdyn_dataflows(contextPath.addSegment("objectid_msdyn_dataflow"));
}
public Msdyn_helppages objectid_msdyn_helppage() {
return new Msdyn_helppages(contextPath.addSegment("objectid_msdyn_helppage"));
}
public Msdyn_knowledgearticleimages objectid_msdyn_knowledgearticleimage() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("objectid_msdyn_knowledgearticleimage"));
}
public Msdyn_knowledgearticletemplates objectid_msdyn_knowledgearticletemplate() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("objectid_msdyn_knowledgearticletemplate"));
}
public Msdyn_richtextfiles objectid_msdyn_richtextfile() {
return new Msdyn_richtextfiles(contextPath.addSegment("objectid_msdyn_richtextfile"));
}
public Msdyn_serviceconfigurations objectid_msdyn_serviceconfiguration() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("objectid_msdyn_serviceconfiguration"));
}
public Msdyn_slakpis objectid_msdyn_slakpi() {
return new Msdyn_slakpis(contextPath.addSegment("objectid_msdyn_slakpi"));
}
public Msdyn_solutionhealthrules objectid_msdyn_solutionhealthrule() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("objectid_msdyn_solutionhealthrule"));
}
public Msdyn_solutionhealthrulearguments objectid_msdyn_solutionhealthruleargument() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("objectid_msdyn_solutionhealthruleargument"));
}
public Msdyn_solutionhealthrulesets objectid_msdyn_solutionhealthruleset() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("objectid_msdyn_solutionhealthruleset"));
}
public Phonecalls objectid_phonecall() {
return new Phonecalls(contextPath.addSegment("objectid_phonecall"));
}
public Positions objectid_position() {
return new Positions(contextPath.addSegment("objectid_position"));
}
public Processstageparameters objectid_processstageparameter() {
return new Processstageparameters(contextPath.addSegment("objectid_processstageparameter"));
}
public Queues objectid_queue() {
return new Queues(contextPath.addSegment("objectid_queue"));
}
public Queueitems objectid_queueitem() {
return new Queueitems(contextPath.addSegment("objectid_queueitem"));
}
public Recurringappointmentmasters objectid_recurringappointmentmaster() {
return new Recurringappointmentmasters(contextPath.addSegment("objectid_recurringappointmentmaster"));
}
public Relationshipattributes objectid_relationshipattribute() {
return new Relationshipattributes(contextPath.addSegment("objectid_relationshipattribute"));
}
public Reportcategories objectid_reportcategory() {
return new Reportcategories(contextPath.addSegment("objectid_reportcategory"));
}
public Serviceplans objectid_serviceplan() {
return new Serviceplans(contextPath.addSegment("objectid_serviceplan"));
}
public Sharepointdocumentlocations objectid_sharepointdocumentlocation() {
return new Sharepointdocumentlocations(contextPath.addSegment("objectid_sharepointdocumentlocation"));
}
public Sharepointsites objectid_sharepointsite() {
return new Sharepointsites(contextPath.addSegment("objectid_sharepointsite"));
}
public Socialactivities objectid_socialactivity() {
return new Socialactivities(contextPath.addSegment("objectid_socialactivity"));
}
public Socialprofiles objectid_socialprofile() {
return new Socialprofiles(contextPath.addSegment("objectid_socialprofile"));
}
public Solutioncomponentattributeconfigurations objectid_solutioncomponentattributeconfiguration() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("objectid_solutioncomponentattributeconfiguration"));
}
public Solutioncomponentconfigurations objectid_solutioncomponentconfiguration() {
return new Solutioncomponentconfigurations(contextPath.addSegment("objectid_solutioncomponentconfiguration"));
}
public Solutioncomponentrelationshipconfigurations objectid_solutioncomponentrelationshipconfiguration() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("objectid_solutioncomponentrelationshipconfiguration"));
}
public Stagesolutionuploads objectid_stagesolutionupload() {
return new Stagesolutionuploads(contextPath.addSegment("objectid_stagesolutionupload"));
}
public Systemusers objectid_systemuser() {
return new Systemusers(contextPath.addSegment("objectid_systemuser"));
}
public Tasks objectid_task() {
return new Tasks(contextPath.addSegment("objectid_task"));
}
public Teams objectid_team() {
return new Teams(contextPath.addSegment("objectid_team"));
}
public Territories objectid_territory() {
return new Territories(contextPath.addSegment("objectid_territory"));
}
public Workflowbinaries objectid_workflowbinary() {
return new Workflowbinaries(contextPath.addSegment("objectid_workflowbinary"));
}
public Organizations organizationid() {
return new Organizations(contextPath.addSegment("organizationid"));
}
public Systemusers principalid_systemuser() {
return new Systemusers(contextPath.addSegment("principalid_systemuser"));
}
public Teams principalid_team() {
return new Teams(contextPath.addSegment("principalid_team"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy