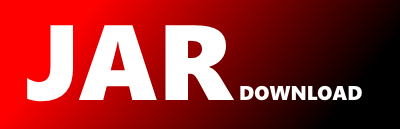
microsoft.dynamics.crm.entity.set.Teams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import microsoft.dynamics.crm.entity.collection.request.TeamCollectionRequest;
public final class Teams extends TeamCollectionRequest {
public Teams(ContextPath contextPath) {
super(contextPath);
}
public Systemusers administratorid() {
return new Systemusers(contextPath.addSegment("administratorid"));
}
public Teamtemplates associatedteamtemplateid() {
return new Teamtemplates(contextPath.addSegment("associatedteamtemplateid"));
}
public Businessunits businessunitid() {
return new Businessunits(contextPath.addSegment("businessunitid"));
}
public Systemusers createdby() {
return new Systemusers(contextPath.addSegment("createdby"));
}
public Systemusers createdonbehalfby() {
return new Systemusers(contextPath.addSegment("createdonbehalfby"));
}
public Importfiles importFile_Team() {
return new Importfiles(contextPath.addSegment("ImportFile_Team"));
}
public Systemusers modifiedby() {
return new Systemusers(contextPath.addSegment("modifiedby"));
}
public Systemusers modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("modifiedonbehalfby"));
}
public Organizations organizationid_organization() {
return new Organizations(contextPath.addSegment("organizationid_organization"));
}
public Postfollows owningTeam_postfollows() {
return new Postfollows(contextPath.addSegment("OwningTeam_postfollows"));
}
public Queues queueid() {
return new Queues(contextPath.addSegment("queueid"));
}
public Knowledgearticles regardingobjectid_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("regardingobjectid_knowledgearticle"));
}
public Processstages stageid_processstage() {
return new Processstages(contextPath.addSegment("stageid_processstage"));
}
public Accounts team_accounts() {
return new Accounts(contextPath.addSegment("team_accounts"));
}
public Activitypointers team_activity() {
return new Activitypointers(contextPath.addSegment("team_activity"));
}
public Annotations team_annotations() {
return new Annotations(contextPath.addSegment("team_annotations"));
}
public Appointments team_appointment() {
return new Appointments(contextPath.addSegment("team_appointment"));
}
public Asyncoperations team_asyncoperation() {
return new Asyncoperations(contextPath.addSegment("team_asyncoperation"));
}
public Asyncoperations team_AsyncOperations() {
return new Asyncoperations(contextPath.addSegment("Team_AsyncOperations"));
}
public Bulkdeletefailures team_BulkDeleteFailures() {
return new Bulkdeletefailures(contextPath.addSegment("Team_BulkDeleteFailures"));
}
public Connectionreferences team_connectionreference() {
return new Connectionreferences(contextPath.addSegment("team_connectionreference"));
}
public Connections team_connections1() {
return new Connections(contextPath.addSegment("team_connections1"));
}
public Connections team_connections2() {
return new Connections(contextPath.addSegment("team_connections2"));
}
public Connectors team_connector() {
return new Connectors(contextPath.addSegment("team_connector"));
}
public Contacts team_contacts() {
return new Contacts(contextPath.addSegment("team_contacts"));
}
public Duplicaterecords team_DuplicateBaseRecord() {
return new Duplicaterecords(contextPath.addSegment("Team_DuplicateBaseRecord"));
}
public Duplicaterecords team_DuplicateMatchingRecord() {
return new Duplicaterecords(contextPath.addSegment("Team_DuplicateMatchingRecord"));
}
public Duplicaterules team_DuplicateRules() {
return new Duplicaterules(contextPath.addSegment("team_DuplicateRules"));
}
public Emails team_email() {
return new Emails(contextPath.addSegment("team_email"));
}
public Templates team_email_templates() {
return new Templates(contextPath.addSegment("team_email_templates"));
}
public Emailserverprofiles team_emailserverprofile() {
return new Emailserverprofiles(contextPath.addSegment("team_emailserverprofile"));
}
public Environmentvariabledefinitions team_environmentvariabledefinition() {
return new Environmentvariabledefinitions(contextPath.addSegment("team_environmentvariabledefinition"));
}
public Environmentvariablevalues team_environmentvariablevalue() {
return new Environmentvariablevalues(contextPath.addSegment("team_environmentvariablevalue"));
}
public Exchangesyncidmappings team_exchangesyncidmapping() {
return new Exchangesyncidmappings(contextPath.addSegment("team_exchangesyncidmapping"));
}
public Exportsolutionuploads team_exportsolutionupload() {
return new Exportsolutionuploads(contextPath.addSegment("team_exportsolutionupload"));
}
public Faxes team_fax() {
return new Faxes(contextPath.addSegment("team_fax"));
}
public Flowsessions team_flowsession() {
return new Flowsessions(contextPath.addSegment("team_flowsession"));
}
public Ggw_crews team_ggw_crew() {
return new Ggw_crews(contextPath.addSegment("team_ggw_crew"));
}
public Ggw_events team_ggw_event() {
return new Ggw_events(contextPath.addSegment("team_ggw_event"));
}
public Ggw_teams team_ggw_team() {
return new Ggw_teams(contextPath.addSegment("team_ggw_team"));
}
public Ggw_team_applications team_ggw_team_application() {
return new Ggw_team_applications(contextPath.addSegment("team_ggw_team_application"));
}
public Goals team_goal() {
return new Goals(contextPath.addSegment("team_goal"));
}
public Goals team_goal_goalowner() {
return new Goals(contextPath.addSegment("team_goal_goalowner"));
}
public Goalrollupqueries team_goalrollupquery() {
return new Goalrollupqueries(contextPath.addSegment("team_goalrollupquery"));
}
public Importdataset team_ImportData() {
return new Importdataset(contextPath.addSegment("team_ImportData"));
}
public Importfiles team_ImportFiles() {
return new Importfiles(contextPath.addSegment("team_ImportFiles"));
}
public Importlogs team_ImportLogs() {
return new Importlogs(contextPath.addSegment("team_ImportLogs"));
}
public Importmaps team_ImportMaps() {
return new Importmaps(contextPath.addSegment("team_ImportMaps"));
}
public Imports team_Imports() {
return new Imports(contextPath.addSegment("team_Imports"));
}
public Knowledgearticles team_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("team_knowledgearticle"));
}
public Letters team_letter() {
return new Letters(contextPath.addSegment("team_letter"));
}
public Mailboxes team_mailbox() {
return new Mailboxes(contextPath.addSegment("team_mailbox"));
}
public Mailboxtrackingfolders team_mailboxtrackingfolder() {
return new Mailboxtrackingfolders(contextPath.addSegment("team_mailboxtrackingfolder"));
}
public Msdyn_aibdatasets team_msdyn_aibdataset() {
return new Msdyn_aibdatasets(contextPath.addSegment("team_msdyn_aibdataset"));
}
public Msdyn_aibdatasetfiles team_msdyn_aibdatasetfile() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("team_msdyn_aibdatasetfile"));
}
public Msdyn_aibdatasetrecords team_msdyn_aibdatasetrecord() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("team_msdyn_aibdatasetrecord"));
}
public Msdyn_aibdatasetscontainers team_msdyn_aibdatasetscontainer() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("team_msdyn_aibdatasetscontainer"));
}
public Msdyn_aibfiles team_msdyn_aibfile() {
return new Msdyn_aibfiles(contextPath.addSegment("team_msdyn_aibfile"));
}
public Msdyn_aibfileattacheddatas team_msdyn_aibfileattacheddata() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("team_msdyn_aibfileattacheddata"));
}
public Msdyn_aiconfigurations team_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurations(contextPath.addSegment("team_msdyn_aiconfiguration"));
}
public Msdyn_aifptrainingdocuments team_msdyn_aifptrainingdocument() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("team_msdyn_aifptrainingdocument"));
}
public Msdyn_aimodels team_msdyn_aimodel() {
return new Msdyn_aimodels(contextPath.addSegment("team_msdyn_aimodel"));
}
public Msdyn_aiodimages team_msdyn_aiodimage() {
return new Msdyn_aiodimages(contextPath.addSegment("team_msdyn_aiodimage"));
}
public Msdyn_aiodlabels team_msdyn_aiodlabel() {
return new Msdyn_aiodlabels(contextPath.addSegment("team_msdyn_aiodlabel"));
}
public Msdyn_aiodtrainingboundingboxes team_msdyn_aiodtrainingboundingbox() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("team_msdyn_aiodtrainingboundingbox"));
}
public Msdyn_aiodtrainingimages team_msdyn_aiodtrainingimage() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("team_msdyn_aiodtrainingimage"));
}
public Msdyn_aitemplates team_msdyn_aitemplate() {
return new Msdyn_aitemplates(contextPath.addSegment("team_msdyn_aitemplate"));
}
public Msdyn_analysiscomponents team_msdyn_analysiscomponent() {
return new Msdyn_analysiscomponents(contextPath.addSegment("team_msdyn_analysiscomponent"));
}
public Msdyn_analysisjobs team_msdyn_analysisjob() {
return new Msdyn_analysisjobs(contextPath.addSegment("team_msdyn_analysisjob"));
}
public Msdyn_analysisresults team_msdyn_analysisresult() {
return new Msdyn_analysisresults(contextPath.addSegment("team_msdyn_analysisresult"));
}
public Msdyn_analysisresultdetails team_msdyn_analysisresultdetail() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("team_msdyn_analysisresultdetail"));
}
public Msdyn_dataflows team_msdyn_dataflow() {
return new Msdyn_dataflows(contextPath.addSegment("team_msdyn_dataflow"));
}
public Msdyn_knowledgearticleimages team_msdyn_knowledgearticleimage() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("team_msdyn_knowledgearticleimage"));
}
public Msdyn_knowledgearticletemplates team_msdyn_knowledgearticletemplate() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("team_msdyn_knowledgearticletemplate"));
}
public Msdyn_richtextfiles team_msdyn_richtextfile() {
return new Msdyn_richtextfiles(contextPath.addSegment("team_msdyn_richtextfile"));
}
public Msdyn_serviceconfigurations team_msdyn_serviceconfiguration() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("team_msdyn_serviceconfiguration"));
}
public Msdyn_slakpis team_msdyn_slakpi() {
return new Msdyn_slakpis(contextPath.addSegment("team_msdyn_slakpi"));
}
public Msdyn_solutionhealthrules team_msdyn_solutionhealthrule() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("team_msdyn_solutionhealthrule"));
}
public Msdyn_solutionhealthrulearguments team_msdyn_solutionhealthruleargument() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("team_msdyn_solutionhealthruleargument"));
}
public Interactionforemails team_new_interactionforemail() {
return new Interactionforemails(contextPath.addSegment("team_new_interactionforemail"));
}
public Phonecalls team_phonecall() {
return new Phonecalls(contextPath.addSegment("team_phonecall"));
}
public Postregardings team_PostRegardings() {
return new Postregardings(contextPath.addSegment("team_PostRegardings"));
}
public Principalobjectattributeaccessset team_principalobjectattributeaccess() {
return new Principalobjectattributeaccessset(contextPath.addSegment("team_principalobjectattributeaccess"));
}
public Principalobjectattributeaccessset team_principalobjectattributeaccess_principalid() {
return new Principalobjectattributeaccessset(contextPath.addSegment("team_principalobjectattributeaccess_principalid"));
}
public Processsessions team_processsession() {
return new Processsessions(contextPath.addSegment("team_processsession"));
}
public Processsessions team_ProcessSessions() {
return new Processsessions(contextPath.addSegment("Team_ProcessSessions"));
}
public Processstageparameters team_processstageparameter() {
return new Processstageparameters(contextPath.addSegment("team_processstageparameter"));
}
public Queueitems team_queueitembase_workerid() {
return new Queueitems(contextPath.addSegment("team_queueitembase_workerid"));
}
public Recurringappointmentmasters team_recurringappointmentmaster() {
return new Recurringappointmentmasters(contextPath.addSegment("team_recurringappointmentmaster"));
}
public Sharepointdocumentlocations team_sharepointdocumentlocation() {
return new Sharepointdocumentlocations(contextPath.addSegment("team_sharepointdocumentlocation"));
}
public Sharepointsites team_sharepointsite() {
return new Sharepointsites(contextPath.addSegment("team_sharepointsite"));
}
public Slas team_slaBase() {
return new Slas(contextPath.addSegment("team_slaBase"));
}
public Socialactivities team_socialactivity() {
return new Socialactivities(contextPath.addSegment("team_socialactivity"));
}
public Stagesolutionuploads team_stagesolutionupload() {
return new Stagesolutionuploads(contextPath.addSegment("team_stagesolutionupload"));
}
public Syncerrors team_SyncError() {
return new Syncerrors(contextPath.addSegment("team_SyncError"));
}
public Syncerrors team_SyncErrors() {
return new Syncerrors(contextPath.addSegment("Team_SyncErrors"));
}
public Tasks team_task() {
return new Tasks(contextPath.addSegment("team_task"));
}
public Userforms team_userform() {
return new Userforms(contextPath.addSegment("team_userform"));
}
public Userqueries team_userquery() {
return new Userqueries(contextPath.addSegment("team_userquery"));
}
public Userqueryvisualizations team_userqueryvisualizations() {
return new Userqueryvisualizations(contextPath.addSegment("team_userqueryvisualizations"));
}
public Workflows team_workflow() {
return new Workflows(contextPath.addSegment("team_workflow"));
}
public Workflowbinaries team_workflowbinary() {
return new Workflowbinaries(contextPath.addSegment("team_workflowbinary"));
}
public Workflowlogs team_workflowlog() {
return new Workflowlogs(contextPath.addSegment("team_workflowlog"));
}
public Systemusers teammembership_association() {
return new Systemusers(contextPath.addSegment("teammembership_association"));
}
public Fieldsecurityprofiles teamprofiles_association() {
return new Fieldsecurityprofiles(contextPath.addSegment("teamprofiles_association"));
}
public Roles teamroles_association() {
return new Roles(contextPath.addSegment("teamroles_association"));
}
public Transactioncurrencies transactioncurrencyid() {
return new Transactioncurrencies(contextPath.addSegment("transactioncurrencyid"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy