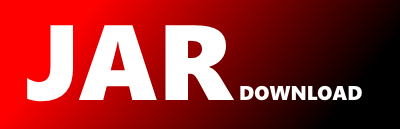
microsoft.dynamics.crm.entity.Account Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.collection.request.AccountCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActioncardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypointerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppointmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ContactCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomeraddressCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FaxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LetterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PhonecallCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostfollowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostregardingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurringappointmentmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SharepointdocumentlocationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialactivityCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TaskCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.SlaRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"overriddencreatedon",
"territorycode",
"accountid",
"address1_telephone1",
"address1_postofficebox",
"address1_line2",
"emailaddress3",
"address1_telephone3",
"address2_county",
"createdon",
"numberofemployees",
"_owningbusinessunit_value",
"timespentbymeonemailandmeetings",
"address2_longitude",
"ownershipcode",
"timezoneruleversionnumber",
"primarysatoriid",
"_masterid_value",
"address2_latitude",
"address2_fax",
"_slaid_value",
"aging60_base",
"telephone1",
"entityimage",
"revenue",
"industrycode",
"aging30_base",
"emailaddress1",
"address1_latitude",
"_transactioncurrencyid_value",
"tickersymbol",
"address2_shippingmethodcode",
"customersizecode",
"address2_name",
"address1_stateorprovince",
"address1_line3",
"accountratingcode",
"address1_line1",
"importsequencenumber",
"paymenttermscode",
"donotfax",
"aging90_base",
"businesstypecode",
"address2_line3",
"address2_utcoffset",
"donotphone",
"exchangerate",
"_ownerid_value",
"_modifiedonbehalfby_value",
"utcconversiontimezonecode",
"ftpsiteurl",
"shippingmethodcode",
"stockexchange",
"address2_telephone3",
"address2_line1",
"_owningteam_value",
"aging60",
"participatesinworkflow",
"address1_postalcode",
"address2_addressid",
"marketcap",
"address2_addresstypecode",
"aging90",
"donotbulkemail",
"aging30",
"entityimage_url",
"sic",
"sharesoutstanding",
"accountcategorycode",
"_owninguser_value",
"stageid",
"versionnumber",
"preferredappointmenttimecode",
"donotsendmm",
"address1_name",
"fax",
"address1_fax",
"merged",
"address1_city",
"description",
"address2_stateorprovince",
"yominame",
"donotpostalmail",
"address1_country",
"donotemail",
"address1_composite",
"_slainvokedid_value",
"address1_primarycontactname",
"onholdtime",
"_createdbyexternalparty_value",
"marketingonly",
"statuscode",
"traversedpath",
"processid",
"_createdonbehalfby_value",
"_modifiedbyexternalparty_value",
"address2_postalcode",
"_modifiedby_value",
"address1_shippingmethodcode",
"_createdby_value",
"entityimage_timestamp",
"address1_upszone",
"address2_primarycontactname",
"primarytwitterid",
"customertypecode",
"address2_postofficebox",
"statecode",
"address2_city",
"_primarycontactid_value",
"address1_freighttermscode",
"address1_longitude",
"telephone2",
"address1_addresstypecode",
"donotbulkpostalmail",
"lastusedincampaign",
"_preferredsystemuserid_value",
"creditlimit_base",
"telephone3",
"address1_addressid",
"preferredappointmentdaycode",
"websiteurl",
"accountclassificationcode",
"address2_composite",
"name",
"address2_telephone1",
"creditlimit",
"lastonholdtime",
"address2_line2",
"creditonhold",
"entityimageid",
"accountnumber",
"followemail",
"modifiedon",
"address1_county",
"marketcap_base",
"address2_upszone",
"address2_country",
"address1_telephone2",
"address2_freighttermscode",
"address1_utcoffset",
"emailaddress2",
"_parentaccountid_value",
"preferredcontactmethodcode",
"revenue_base",
"address2_telephone2"})
@JsonInclude(Include.NON_NULL)
public class Account extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.account";
}
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("territorycode")
protected Integer territorycode;
@JsonProperty("accountid")
protected UUID accountid;
@JsonProperty("address1_telephone1")
protected String address1_telephone1;
@JsonProperty("address1_postofficebox")
protected String address1_postofficebox;
@JsonProperty("address1_line2")
protected String address1_line2;
@JsonProperty("emailaddress3")
protected String emailaddress3;
@JsonProperty("address1_telephone3")
protected String address1_telephone3;
@JsonProperty("address2_county")
protected String address2_county;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("numberofemployees")
protected Integer numberofemployees;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("timespentbymeonemailandmeetings")
protected String timespentbymeonemailandmeetings;
@JsonProperty("address2_longitude")
protected Double address2_longitude;
@JsonProperty("ownershipcode")
protected Integer ownershipcode;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("primarysatoriid")
protected String primarysatoriid;
@JsonProperty("_masterid_value")
protected UUID _masterid_value;
@JsonProperty("address2_latitude")
protected Double address2_latitude;
@JsonProperty("address2_fax")
protected String address2_fax;
@JsonProperty("_slaid_value")
protected UUID _slaid_value;
@JsonProperty("aging60_base")
protected BigDecimal aging60_base;
@JsonProperty("telephone1")
protected String telephone1;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("revenue")
protected BigDecimal revenue;
@JsonProperty("industrycode")
protected Integer industrycode;
@JsonProperty("aging30_base")
protected BigDecimal aging30_base;
@JsonProperty("emailaddress1")
protected String emailaddress1;
@JsonProperty("address1_latitude")
protected Double address1_latitude;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("tickersymbol")
protected String tickersymbol;
@JsonProperty("address2_shippingmethodcode")
protected Integer address2_shippingmethodcode;
@JsonProperty("customersizecode")
protected Integer customersizecode;
@JsonProperty("address2_name")
protected String address2_name;
@JsonProperty("address1_stateorprovince")
protected String address1_stateorprovince;
@JsonProperty("address1_line3")
protected String address1_line3;
@JsonProperty("accountratingcode")
protected Integer accountratingcode;
@JsonProperty("address1_line1")
protected String address1_line1;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("paymenttermscode")
protected Integer paymenttermscode;
@JsonProperty("donotfax")
protected Boolean donotfax;
@JsonProperty("aging90_base")
protected BigDecimal aging90_base;
@JsonProperty("businesstypecode")
protected Integer businesstypecode;
@JsonProperty("address2_line3")
protected String address2_line3;
@JsonProperty("address2_utcoffset")
protected Integer address2_utcoffset;
@JsonProperty("donotphone")
protected Boolean donotphone;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("ftpsiteurl")
protected String ftpsiteurl;
@JsonProperty("shippingmethodcode")
protected Integer shippingmethodcode;
@JsonProperty("stockexchange")
protected String stockexchange;
@JsonProperty("address2_telephone3")
protected String address2_telephone3;
@JsonProperty("address2_line1")
protected String address2_line1;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("aging60")
protected BigDecimal aging60;
@JsonProperty("participatesinworkflow")
protected Boolean participatesinworkflow;
@JsonProperty("address1_postalcode")
protected String address1_postalcode;
@JsonProperty("address2_addressid")
protected UUID address2_addressid;
@JsonProperty("marketcap")
protected BigDecimal marketcap;
@JsonProperty("address2_addresstypecode")
protected Integer address2_addresstypecode;
@JsonProperty("aging90")
protected BigDecimal aging90;
@JsonProperty("donotbulkemail")
protected Boolean donotbulkemail;
@JsonProperty("aging30")
protected BigDecimal aging30;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("sic")
protected String sic;
@JsonProperty("sharesoutstanding")
protected Integer sharesoutstanding;
@JsonProperty("accountcategorycode")
protected Integer accountcategorycode;
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("stageid")
protected UUID stageid;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("preferredappointmenttimecode")
protected Integer preferredappointmenttimecode;
@JsonProperty("donotsendmm")
protected Boolean donotsendmm;
@JsonProperty("address1_name")
protected String address1_name;
@JsonProperty("fax")
protected String fax;
@JsonProperty("address1_fax")
protected String address1_fax;
@JsonProperty("merged")
protected Boolean merged;
@JsonProperty("address1_city")
protected String address1_city;
@JsonProperty("description")
protected String description;
@JsonProperty("address2_stateorprovince")
protected String address2_stateorprovince;
@JsonProperty("yominame")
protected String yominame;
@JsonProperty("donotpostalmail")
protected Boolean donotpostalmail;
@JsonProperty("address1_country")
protected String address1_country;
@JsonProperty("donotemail")
protected Boolean donotemail;
@JsonProperty("address1_composite")
protected String address1_composite;
@JsonProperty("_slainvokedid_value")
protected UUID _slainvokedid_value;
@JsonProperty("address1_primarycontactname")
protected String address1_primarycontactname;
@JsonProperty("onholdtime")
protected Integer onholdtime;
@JsonProperty("_createdbyexternalparty_value")
protected UUID _createdbyexternalparty_value;
@JsonProperty("marketingonly")
protected Boolean marketingonly;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("traversedpath")
protected String traversedpath;
@JsonProperty("processid")
protected UUID processid;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("_modifiedbyexternalparty_value")
protected UUID _modifiedbyexternalparty_value;
@JsonProperty("address2_postalcode")
protected String address2_postalcode;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("address1_shippingmethodcode")
protected Integer address1_shippingmethodcode;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("address1_upszone")
protected String address1_upszone;
@JsonProperty("address2_primarycontactname")
protected String address2_primarycontactname;
@JsonProperty("primarytwitterid")
protected String primarytwitterid;
@JsonProperty("customertypecode")
protected Integer customertypecode;
@JsonProperty("address2_postofficebox")
protected String address2_postofficebox;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("address2_city")
protected String address2_city;
@JsonProperty("_primarycontactid_value")
protected UUID _primarycontactid_value;
@JsonProperty("address1_freighttermscode")
protected Integer address1_freighttermscode;
@JsonProperty("address1_longitude")
protected Double address1_longitude;
@JsonProperty("telephone2")
protected String telephone2;
@JsonProperty("address1_addresstypecode")
protected Integer address1_addresstypecode;
@JsonProperty("donotbulkpostalmail")
protected Boolean donotbulkpostalmail;
@JsonProperty("lastusedincampaign")
protected OffsetDateTime lastusedincampaign;
@JsonProperty("_preferredsystemuserid_value")
protected UUID _preferredsystemuserid_value;
@JsonProperty("creditlimit_base")
protected BigDecimal creditlimit_base;
@JsonProperty("telephone3")
protected String telephone3;
@JsonProperty("address1_addressid")
protected UUID address1_addressid;
@JsonProperty("preferredappointmentdaycode")
protected Integer preferredappointmentdaycode;
@JsonProperty("websiteurl")
protected String websiteurl;
@JsonProperty("accountclassificationcode")
protected Integer accountclassificationcode;
@JsonProperty("address2_composite")
protected String address2_composite;
@JsonProperty("name")
protected String name;
@JsonProperty("address2_telephone1")
protected String address2_telephone1;
@JsonProperty("creditlimit")
protected BigDecimal creditlimit;
@JsonProperty("lastonholdtime")
protected OffsetDateTime lastonholdtime;
@JsonProperty("address2_line2")
protected String address2_line2;
@JsonProperty("creditonhold")
protected Boolean creditonhold;
@JsonProperty("entityimageid")
protected UUID entityimageid;
@JsonProperty("accountnumber")
protected String accountnumber;
@JsonProperty("followemail")
protected Boolean followemail;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("address1_county")
protected String address1_county;
@JsonProperty("marketcap_base")
protected BigDecimal marketcap_base;
@JsonProperty("address2_upszone")
protected String address2_upszone;
@JsonProperty("address2_country")
protected String address2_country;
@JsonProperty("address1_telephone2")
protected String address1_telephone2;
@JsonProperty("address2_freighttermscode")
protected Integer address2_freighttermscode;
@JsonProperty("address1_utcoffset")
protected Integer address1_utcoffset;
@JsonProperty("emailaddress2")
protected String emailaddress2;
@JsonProperty("_parentaccountid_value")
protected UUID _parentaccountid_value;
@JsonProperty("preferredcontactmethodcode")
protected Integer preferredcontactmethodcode;
@JsonProperty("revenue_base")
protected BigDecimal revenue_base;
@JsonProperty("address2_telephone2")
protected String address2_telephone2;
protected Account() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAccount() {
return new Builder();
}
public static final class Builder {
private OffsetDateTime overriddencreatedon;
private Integer territorycode;
private UUID accountid;
private String address1_telephone1;
private String address1_postofficebox;
private String address1_line2;
private String emailaddress3;
private String address1_telephone3;
private String address2_county;
private OffsetDateTime createdon;
private Integer numberofemployees;
private UUID _owningbusinessunit_value;
private String timespentbymeonemailandmeetings;
private Double address2_longitude;
private Integer ownershipcode;
private Integer timezoneruleversionnumber;
private String primarysatoriid;
private UUID _masterid_value;
private Double address2_latitude;
private String address2_fax;
private UUID _slaid_value;
private BigDecimal aging60_base;
private String telephone1;
private byte[] entityimage;
private BigDecimal revenue;
private Integer industrycode;
private BigDecimal aging30_base;
private String emailaddress1;
private Double address1_latitude;
private UUID _transactioncurrencyid_value;
private String tickersymbol;
private Integer address2_shippingmethodcode;
private Integer customersizecode;
private String address2_name;
private String address1_stateorprovince;
private String address1_line3;
private Integer accountratingcode;
private String address1_line1;
private Integer importsequencenumber;
private Integer paymenttermscode;
private Boolean donotfax;
private BigDecimal aging90_base;
private Integer businesstypecode;
private String address2_line3;
private Integer address2_utcoffset;
private Boolean donotphone;
private BigDecimal exchangerate;
private UUID _ownerid_value;
private UUID _modifiedonbehalfby_value;
private Integer utcconversiontimezonecode;
private String ftpsiteurl;
private Integer shippingmethodcode;
private String stockexchange;
private String address2_telephone3;
private String address2_line1;
private UUID _owningteam_value;
private BigDecimal aging60;
private Boolean participatesinworkflow;
private String address1_postalcode;
private UUID address2_addressid;
private BigDecimal marketcap;
private Integer address2_addresstypecode;
private BigDecimal aging90;
private Boolean donotbulkemail;
private BigDecimal aging30;
private String entityimage_url;
private String sic;
private Integer sharesoutstanding;
private Integer accountcategorycode;
private UUID _owninguser_value;
private UUID stageid;
private Long versionnumber;
private Integer preferredappointmenttimecode;
private Boolean donotsendmm;
private String address1_name;
private String fax;
private String address1_fax;
private Boolean merged;
private String address1_city;
private String description;
private String address2_stateorprovince;
private String yominame;
private Boolean donotpostalmail;
private String address1_country;
private Boolean donotemail;
private String address1_composite;
private UUID _slainvokedid_value;
private String address1_primarycontactname;
private Integer onholdtime;
private UUID _createdbyexternalparty_value;
private Boolean marketingonly;
private Integer statuscode;
private String traversedpath;
private UUID processid;
private UUID _createdonbehalfby_value;
private UUID _modifiedbyexternalparty_value;
private String address2_postalcode;
private UUID _modifiedby_value;
private Integer address1_shippingmethodcode;
private UUID _createdby_value;
private Long entityimage_timestamp;
private String address1_upszone;
private String address2_primarycontactname;
private String primarytwitterid;
private Integer customertypecode;
private String address2_postofficebox;
private Integer statecode;
private String address2_city;
private UUID _primarycontactid_value;
private Integer address1_freighttermscode;
private Double address1_longitude;
private String telephone2;
private Integer address1_addresstypecode;
private Boolean donotbulkpostalmail;
private OffsetDateTime lastusedincampaign;
private UUID _preferredsystemuserid_value;
private BigDecimal creditlimit_base;
private String telephone3;
private UUID address1_addressid;
private Integer preferredappointmentdaycode;
private String websiteurl;
private Integer accountclassificationcode;
private String address2_composite;
private String name;
private String address2_telephone1;
private BigDecimal creditlimit;
private OffsetDateTime lastonholdtime;
private String address2_line2;
private Boolean creditonhold;
private UUID entityimageid;
private String accountnumber;
private Boolean followemail;
private OffsetDateTime modifiedon;
private String address1_county;
private BigDecimal marketcap_base;
private String address2_upszone;
private String address2_country;
private String address1_telephone2;
private Integer address2_freighttermscode;
private Integer address1_utcoffset;
private String emailaddress2;
private UUID _parentaccountid_value;
private Integer preferredcontactmethodcode;
private BigDecimal revenue_base;
private String address2_telephone2;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder territorycode(Integer territorycode) {
this.territorycode = territorycode;
this.changedFields = changedFields.add("territorycode");
return this;
}
public Builder accountid(UUID accountid) {
this.accountid = accountid;
this.changedFields = changedFields.add("accountid");
return this;
}
public Builder address1_telephone1(String address1_telephone1) {
this.address1_telephone1 = address1_telephone1;
this.changedFields = changedFields.add("address1_telephone1");
return this;
}
public Builder address1_postofficebox(String address1_postofficebox) {
this.address1_postofficebox = address1_postofficebox;
this.changedFields = changedFields.add("address1_postofficebox");
return this;
}
public Builder address1_line2(String address1_line2) {
this.address1_line2 = address1_line2;
this.changedFields = changedFields.add("address1_line2");
return this;
}
public Builder emailaddress3(String emailaddress3) {
this.emailaddress3 = emailaddress3;
this.changedFields = changedFields.add("emailaddress3");
return this;
}
public Builder address1_telephone3(String address1_telephone3) {
this.address1_telephone3 = address1_telephone3;
this.changedFields = changedFields.add("address1_telephone3");
return this;
}
public Builder address2_county(String address2_county) {
this.address2_county = address2_county;
this.changedFields = changedFields.add("address2_county");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder numberofemployees(Integer numberofemployees) {
this.numberofemployees = numberofemployees;
this.changedFields = changedFields.add("numberofemployees");
return this;
}
public Builder _owningbusinessunit_value(UUID _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder timespentbymeonemailandmeetings(String timespentbymeonemailandmeetings) {
this.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
this.changedFields = changedFields.add("timespentbymeonemailandmeetings");
return this;
}
public Builder address2_longitude(Double address2_longitude) {
this.address2_longitude = address2_longitude;
this.changedFields = changedFields.add("address2_longitude");
return this;
}
public Builder ownershipcode(Integer ownershipcode) {
this.ownershipcode = ownershipcode;
this.changedFields = changedFields.add("ownershipcode");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder primarysatoriid(String primarysatoriid) {
this.primarysatoriid = primarysatoriid;
this.changedFields = changedFields.add("primarysatoriid");
return this;
}
public Builder _masterid_value(UUID _masterid_value) {
this._masterid_value = _masterid_value;
this.changedFields = changedFields.add("_masterid_value");
return this;
}
public Builder address2_latitude(Double address2_latitude) {
this.address2_latitude = address2_latitude;
this.changedFields = changedFields.add("address2_latitude");
return this;
}
public Builder address2_fax(String address2_fax) {
this.address2_fax = address2_fax;
this.changedFields = changedFields.add("address2_fax");
return this;
}
public Builder _slaid_value(UUID _slaid_value) {
this._slaid_value = _slaid_value;
this.changedFields = changedFields.add("_slaid_value");
return this;
}
public Builder aging60_base(BigDecimal aging60_base) {
this.aging60_base = aging60_base;
this.changedFields = changedFields.add("aging60_base");
return this;
}
public Builder telephone1(String telephone1) {
this.telephone1 = telephone1;
this.changedFields = changedFields.add("telephone1");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder revenue(BigDecimal revenue) {
this.revenue = revenue;
this.changedFields = changedFields.add("revenue");
return this;
}
public Builder industrycode(Integer industrycode) {
this.industrycode = industrycode;
this.changedFields = changedFields.add("industrycode");
return this;
}
public Builder aging30_base(BigDecimal aging30_base) {
this.aging30_base = aging30_base;
this.changedFields = changedFields.add("aging30_base");
return this;
}
public Builder emailaddress1(String emailaddress1) {
this.emailaddress1 = emailaddress1;
this.changedFields = changedFields.add("emailaddress1");
return this;
}
public Builder address1_latitude(Double address1_latitude) {
this.address1_latitude = address1_latitude;
this.changedFields = changedFields.add("address1_latitude");
return this;
}
public Builder _transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder tickersymbol(String tickersymbol) {
this.tickersymbol = tickersymbol;
this.changedFields = changedFields.add("tickersymbol");
return this;
}
public Builder address2_shippingmethodcode(Integer address2_shippingmethodcode) {
this.address2_shippingmethodcode = address2_shippingmethodcode;
this.changedFields = changedFields.add("address2_shippingmethodcode");
return this;
}
public Builder customersizecode(Integer customersizecode) {
this.customersizecode = customersizecode;
this.changedFields = changedFields.add("customersizecode");
return this;
}
public Builder address2_name(String address2_name) {
this.address2_name = address2_name;
this.changedFields = changedFields.add("address2_name");
return this;
}
public Builder address1_stateorprovince(String address1_stateorprovince) {
this.address1_stateorprovince = address1_stateorprovince;
this.changedFields = changedFields.add("address1_stateorprovince");
return this;
}
public Builder address1_line3(String address1_line3) {
this.address1_line3 = address1_line3;
this.changedFields = changedFields.add("address1_line3");
return this;
}
public Builder accountratingcode(Integer accountratingcode) {
this.accountratingcode = accountratingcode;
this.changedFields = changedFields.add("accountratingcode");
return this;
}
public Builder address1_line1(String address1_line1) {
this.address1_line1 = address1_line1;
this.changedFields = changedFields.add("address1_line1");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder paymenttermscode(Integer paymenttermscode) {
this.paymenttermscode = paymenttermscode;
this.changedFields = changedFields.add("paymenttermscode");
return this;
}
public Builder donotfax(Boolean donotfax) {
this.donotfax = donotfax;
this.changedFields = changedFields.add("donotfax");
return this;
}
public Builder aging90_base(BigDecimal aging90_base) {
this.aging90_base = aging90_base;
this.changedFields = changedFields.add("aging90_base");
return this;
}
public Builder businesstypecode(Integer businesstypecode) {
this.businesstypecode = businesstypecode;
this.changedFields = changedFields.add("businesstypecode");
return this;
}
public Builder address2_line3(String address2_line3) {
this.address2_line3 = address2_line3;
this.changedFields = changedFields.add("address2_line3");
return this;
}
public Builder address2_utcoffset(Integer address2_utcoffset) {
this.address2_utcoffset = address2_utcoffset;
this.changedFields = changedFields.add("address2_utcoffset");
return this;
}
public Builder donotphone(Boolean donotphone) {
this.donotphone = donotphone;
this.changedFields = changedFields.add("donotphone");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder _ownerid_value(UUID _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder ftpsiteurl(String ftpsiteurl) {
this.ftpsiteurl = ftpsiteurl;
this.changedFields = changedFields.add("ftpsiteurl");
return this;
}
public Builder shippingmethodcode(Integer shippingmethodcode) {
this.shippingmethodcode = shippingmethodcode;
this.changedFields = changedFields.add("shippingmethodcode");
return this;
}
public Builder stockexchange(String stockexchange) {
this.stockexchange = stockexchange;
this.changedFields = changedFields.add("stockexchange");
return this;
}
public Builder address2_telephone3(String address2_telephone3) {
this.address2_telephone3 = address2_telephone3;
this.changedFields = changedFields.add("address2_telephone3");
return this;
}
public Builder address2_line1(String address2_line1) {
this.address2_line1 = address2_line1;
this.changedFields = changedFields.add("address2_line1");
return this;
}
public Builder _owningteam_value(UUID _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder aging60(BigDecimal aging60) {
this.aging60 = aging60;
this.changedFields = changedFields.add("aging60");
return this;
}
public Builder participatesinworkflow(Boolean participatesinworkflow) {
this.participatesinworkflow = participatesinworkflow;
this.changedFields = changedFields.add("participatesinworkflow");
return this;
}
public Builder address1_postalcode(String address1_postalcode) {
this.address1_postalcode = address1_postalcode;
this.changedFields = changedFields.add("address1_postalcode");
return this;
}
public Builder address2_addressid(UUID address2_addressid) {
this.address2_addressid = address2_addressid;
this.changedFields = changedFields.add("address2_addressid");
return this;
}
public Builder marketcap(BigDecimal marketcap) {
this.marketcap = marketcap;
this.changedFields = changedFields.add("marketcap");
return this;
}
public Builder address2_addresstypecode(Integer address2_addresstypecode) {
this.address2_addresstypecode = address2_addresstypecode;
this.changedFields = changedFields.add("address2_addresstypecode");
return this;
}
public Builder aging90(BigDecimal aging90) {
this.aging90 = aging90;
this.changedFields = changedFields.add("aging90");
return this;
}
public Builder donotbulkemail(Boolean donotbulkemail) {
this.donotbulkemail = donotbulkemail;
this.changedFields = changedFields.add("donotbulkemail");
return this;
}
public Builder aging30(BigDecimal aging30) {
this.aging30 = aging30;
this.changedFields = changedFields.add("aging30");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder sic(String sic) {
this.sic = sic;
this.changedFields = changedFields.add("sic");
return this;
}
public Builder sharesoutstanding(Integer sharesoutstanding) {
this.sharesoutstanding = sharesoutstanding;
this.changedFields = changedFields.add("sharesoutstanding");
return this;
}
public Builder accountcategorycode(Integer accountcategorycode) {
this.accountcategorycode = accountcategorycode;
this.changedFields = changedFields.add("accountcategorycode");
return this;
}
public Builder _owninguser_value(UUID _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder stageid(UUID stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder preferredappointmenttimecode(Integer preferredappointmenttimecode) {
this.preferredappointmenttimecode = preferredappointmenttimecode;
this.changedFields = changedFields.add("preferredappointmenttimecode");
return this;
}
public Builder donotsendmm(Boolean donotsendmm) {
this.donotsendmm = donotsendmm;
this.changedFields = changedFields.add("donotsendmm");
return this;
}
public Builder address1_name(String address1_name) {
this.address1_name = address1_name;
this.changedFields = changedFields.add("address1_name");
return this;
}
public Builder fax(String fax) {
this.fax = fax;
this.changedFields = changedFields.add("fax");
return this;
}
public Builder address1_fax(String address1_fax) {
this.address1_fax = address1_fax;
this.changedFields = changedFields.add("address1_fax");
return this;
}
public Builder merged(Boolean merged) {
this.merged = merged;
this.changedFields = changedFields.add("merged");
return this;
}
public Builder address1_city(String address1_city) {
this.address1_city = address1_city;
this.changedFields = changedFields.add("address1_city");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder address2_stateorprovince(String address2_stateorprovince) {
this.address2_stateorprovince = address2_stateorprovince;
this.changedFields = changedFields.add("address2_stateorprovince");
return this;
}
public Builder yominame(String yominame) {
this.yominame = yominame;
this.changedFields = changedFields.add("yominame");
return this;
}
public Builder donotpostalmail(Boolean donotpostalmail) {
this.donotpostalmail = donotpostalmail;
this.changedFields = changedFields.add("donotpostalmail");
return this;
}
public Builder address1_country(String address1_country) {
this.address1_country = address1_country;
this.changedFields = changedFields.add("address1_country");
return this;
}
public Builder donotemail(Boolean donotemail) {
this.donotemail = donotemail;
this.changedFields = changedFields.add("donotemail");
return this;
}
public Builder address1_composite(String address1_composite) {
this.address1_composite = address1_composite;
this.changedFields = changedFields.add("address1_composite");
return this;
}
public Builder _slainvokedid_value(UUID _slainvokedid_value) {
this._slainvokedid_value = _slainvokedid_value;
this.changedFields = changedFields.add("_slainvokedid_value");
return this;
}
public Builder address1_primarycontactname(String address1_primarycontactname) {
this.address1_primarycontactname = address1_primarycontactname;
this.changedFields = changedFields.add("address1_primarycontactname");
return this;
}
public Builder onholdtime(Integer onholdtime) {
this.onholdtime = onholdtime;
this.changedFields = changedFields.add("onholdtime");
return this;
}
public Builder _createdbyexternalparty_value(UUID _createdbyexternalparty_value) {
this._createdbyexternalparty_value = _createdbyexternalparty_value;
this.changedFields = changedFields.add("_createdbyexternalparty_value");
return this;
}
public Builder marketingonly(Boolean marketingonly) {
this.marketingonly = marketingonly;
this.changedFields = changedFields.add("marketingonly");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder processid(UUID processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder _modifiedbyexternalparty_value(UUID _modifiedbyexternalparty_value) {
this._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
this.changedFields = changedFields.add("_modifiedbyexternalparty_value");
return this;
}
public Builder address2_postalcode(String address2_postalcode) {
this.address2_postalcode = address2_postalcode;
this.changedFields = changedFields.add("address2_postalcode");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder address1_shippingmethodcode(Integer address1_shippingmethodcode) {
this.address1_shippingmethodcode = address1_shippingmethodcode;
this.changedFields = changedFields.add("address1_shippingmethodcode");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder address1_upszone(String address1_upszone) {
this.address1_upszone = address1_upszone;
this.changedFields = changedFields.add("address1_upszone");
return this;
}
public Builder address2_primarycontactname(String address2_primarycontactname) {
this.address2_primarycontactname = address2_primarycontactname;
this.changedFields = changedFields.add("address2_primarycontactname");
return this;
}
public Builder primarytwitterid(String primarytwitterid) {
this.primarytwitterid = primarytwitterid;
this.changedFields = changedFields.add("primarytwitterid");
return this;
}
public Builder customertypecode(Integer customertypecode) {
this.customertypecode = customertypecode;
this.changedFields = changedFields.add("customertypecode");
return this;
}
public Builder address2_postofficebox(String address2_postofficebox) {
this.address2_postofficebox = address2_postofficebox;
this.changedFields = changedFields.add("address2_postofficebox");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder address2_city(String address2_city) {
this.address2_city = address2_city;
this.changedFields = changedFields.add("address2_city");
return this;
}
public Builder _primarycontactid_value(UUID _primarycontactid_value) {
this._primarycontactid_value = _primarycontactid_value;
this.changedFields = changedFields.add("_primarycontactid_value");
return this;
}
public Builder address1_freighttermscode(Integer address1_freighttermscode) {
this.address1_freighttermscode = address1_freighttermscode;
this.changedFields = changedFields.add("address1_freighttermscode");
return this;
}
public Builder address1_longitude(Double address1_longitude) {
this.address1_longitude = address1_longitude;
this.changedFields = changedFields.add("address1_longitude");
return this;
}
public Builder telephone2(String telephone2) {
this.telephone2 = telephone2;
this.changedFields = changedFields.add("telephone2");
return this;
}
public Builder address1_addresstypecode(Integer address1_addresstypecode) {
this.address1_addresstypecode = address1_addresstypecode;
this.changedFields = changedFields.add("address1_addresstypecode");
return this;
}
public Builder donotbulkpostalmail(Boolean donotbulkpostalmail) {
this.donotbulkpostalmail = donotbulkpostalmail;
this.changedFields = changedFields.add("donotbulkpostalmail");
return this;
}
public Builder lastusedincampaign(OffsetDateTime lastusedincampaign) {
this.lastusedincampaign = lastusedincampaign;
this.changedFields = changedFields.add("lastusedincampaign");
return this;
}
public Builder _preferredsystemuserid_value(UUID _preferredsystemuserid_value) {
this._preferredsystemuserid_value = _preferredsystemuserid_value;
this.changedFields = changedFields.add("_preferredsystemuserid_value");
return this;
}
public Builder creditlimit_base(BigDecimal creditlimit_base) {
this.creditlimit_base = creditlimit_base;
this.changedFields = changedFields.add("creditlimit_base");
return this;
}
public Builder telephone3(String telephone3) {
this.telephone3 = telephone3;
this.changedFields = changedFields.add("telephone3");
return this;
}
public Builder address1_addressid(UUID address1_addressid) {
this.address1_addressid = address1_addressid;
this.changedFields = changedFields.add("address1_addressid");
return this;
}
public Builder preferredappointmentdaycode(Integer preferredappointmentdaycode) {
this.preferredappointmentdaycode = preferredappointmentdaycode;
this.changedFields = changedFields.add("preferredappointmentdaycode");
return this;
}
public Builder websiteurl(String websiteurl) {
this.websiteurl = websiteurl;
this.changedFields = changedFields.add("websiteurl");
return this;
}
public Builder accountclassificationcode(Integer accountclassificationcode) {
this.accountclassificationcode = accountclassificationcode;
this.changedFields = changedFields.add("accountclassificationcode");
return this;
}
public Builder address2_composite(String address2_composite) {
this.address2_composite = address2_composite;
this.changedFields = changedFields.add("address2_composite");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder address2_telephone1(String address2_telephone1) {
this.address2_telephone1 = address2_telephone1;
this.changedFields = changedFields.add("address2_telephone1");
return this;
}
public Builder creditlimit(BigDecimal creditlimit) {
this.creditlimit = creditlimit;
this.changedFields = changedFields.add("creditlimit");
return this;
}
public Builder lastonholdtime(OffsetDateTime lastonholdtime) {
this.lastonholdtime = lastonholdtime;
this.changedFields = changedFields.add("lastonholdtime");
return this;
}
public Builder address2_line2(String address2_line2) {
this.address2_line2 = address2_line2;
this.changedFields = changedFields.add("address2_line2");
return this;
}
public Builder creditonhold(Boolean creditonhold) {
this.creditonhold = creditonhold;
this.changedFields = changedFields.add("creditonhold");
return this;
}
public Builder entityimageid(UUID entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder accountnumber(String accountnumber) {
this.accountnumber = accountnumber;
this.changedFields = changedFields.add("accountnumber");
return this;
}
public Builder followemail(Boolean followemail) {
this.followemail = followemail;
this.changedFields = changedFields.add("followemail");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder address1_county(String address1_county) {
this.address1_county = address1_county;
this.changedFields = changedFields.add("address1_county");
return this;
}
public Builder marketcap_base(BigDecimal marketcap_base) {
this.marketcap_base = marketcap_base;
this.changedFields = changedFields.add("marketcap_base");
return this;
}
public Builder address2_upszone(String address2_upszone) {
this.address2_upszone = address2_upszone;
this.changedFields = changedFields.add("address2_upszone");
return this;
}
public Builder address2_country(String address2_country) {
this.address2_country = address2_country;
this.changedFields = changedFields.add("address2_country");
return this;
}
public Builder address1_telephone2(String address1_telephone2) {
this.address1_telephone2 = address1_telephone2;
this.changedFields = changedFields.add("address1_telephone2");
return this;
}
public Builder address2_freighttermscode(Integer address2_freighttermscode) {
this.address2_freighttermscode = address2_freighttermscode;
this.changedFields = changedFields.add("address2_freighttermscode");
return this;
}
public Builder address1_utcoffset(Integer address1_utcoffset) {
this.address1_utcoffset = address1_utcoffset;
this.changedFields = changedFields.add("address1_utcoffset");
return this;
}
public Builder emailaddress2(String emailaddress2) {
this.emailaddress2 = emailaddress2;
this.changedFields = changedFields.add("emailaddress2");
return this;
}
public Builder _parentaccountid_value(UUID _parentaccountid_value) {
this._parentaccountid_value = _parentaccountid_value;
this.changedFields = changedFields.add("_parentaccountid_value");
return this;
}
public Builder preferredcontactmethodcode(Integer preferredcontactmethodcode) {
this.preferredcontactmethodcode = preferredcontactmethodcode;
this.changedFields = changedFields.add("preferredcontactmethodcode");
return this;
}
public Builder revenue_base(BigDecimal revenue_base) {
this.revenue_base = revenue_base;
this.changedFields = changedFields.add("revenue_base");
return this;
}
public Builder address2_telephone2(String address2_telephone2) {
this.address2_telephone2 = address2_telephone2;
this.changedFields = changedFields.add("address2_telephone2");
return this;
}
public Account build() {
Account _x = new Account();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.account";
_x.overriddencreatedon = overriddencreatedon;
_x.territorycode = territorycode;
_x.accountid = accountid;
_x.address1_telephone1 = address1_telephone1;
_x.address1_postofficebox = address1_postofficebox;
_x.address1_line2 = address1_line2;
_x.emailaddress3 = emailaddress3;
_x.address1_telephone3 = address1_telephone3;
_x.address2_county = address2_county;
_x.createdon = createdon;
_x.numberofemployees = numberofemployees;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
_x.address2_longitude = address2_longitude;
_x.ownershipcode = ownershipcode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.primarysatoriid = primarysatoriid;
_x._masterid_value = _masterid_value;
_x.address2_latitude = address2_latitude;
_x.address2_fax = address2_fax;
_x._slaid_value = _slaid_value;
_x.aging60_base = aging60_base;
_x.telephone1 = telephone1;
_x.entityimage = entityimage;
_x.revenue = revenue;
_x.industrycode = industrycode;
_x.aging30_base = aging30_base;
_x.emailaddress1 = emailaddress1;
_x.address1_latitude = address1_latitude;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.tickersymbol = tickersymbol;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.customersizecode = customersizecode;
_x.address2_name = address2_name;
_x.address1_stateorprovince = address1_stateorprovince;
_x.address1_line3 = address1_line3;
_x.accountratingcode = accountratingcode;
_x.address1_line1 = address1_line1;
_x.importsequencenumber = importsequencenumber;
_x.paymenttermscode = paymenttermscode;
_x.donotfax = donotfax;
_x.aging90_base = aging90_base;
_x.businesstypecode = businesstypecode;
_x.address2_line3 = address2_line3;
_x.address2_utcoffset = address2_utcoffset;
_x.donotphone = donotphone;
_x.exchangerate = exchangerate;
_x._ownerid_value = _ownerid_value;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.ftpsiteurl = ftpsiteurl;
_x.shippingmethodcode = shippingmethodcode;
_x.stockexchange = stockexchange;
_x.address2_telephone3 = address2_telephone3;
_x.address2_line1 = address2_line1;
_x._owningteam_value = _owningteam_value;
_x.aging60 = aging60;
_x.participatesinworkflow = participatesinworkflow;
_x.address1_postalcode = address1_postalcode;
_x.address2_addressid = address2_addressid;
_x.marketcap = marketcap;
_x.address2_addresstypecode = address2_addresstypecode;
_x.aging90 = aging90;
_x.donotbulkemail = donotbulkemail;
_x.aging30 = aging30;
_x.entityimage_url = entityimage_url;
_x.sic = sic;
_x.sharesoutstanding = sharesoutstanding;
_x.accountcategorycode = accountcategorycode;
_x._owninguser_value = _owninguser_value;
_x.stageid = stageid;
_x.versionnumber = versionnumber;
_x.preferredappointmenttimecode = preferredappointmenttimecode;
_x.donotsendmm = donotsendmm;
_x.address1_name = address1_name;
_x.fax = fax;
_x.address1_fax = address1_fax;
_x.merged = merged;
_x.address1_city = address1_city;
_x.description = description;
_x.address2_stateorprovince = address2_stateorprovince;
_x.yominame = yominame;
_x.donotpostalmail = donotpostalmail;
_x.address1_country = address1_country;
_x.donotemail = donotemail;
_x.address1_composite = address1_composite;
_x._slainvokedid_value = _slainvokedid_value;
_x.address1_primarycontactname = address1_primarycontactname;
_x.onholdtime = onholdtime;
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
_x.marketingonly = marketingonly;
_x.statuscode = statuscode;
_x.traversedpath = traversedpath;
_x.processid = processid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
_x.address2_postalcode = address2_postalcode;
_x._modifiedby_value = _modifiedby_value;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x._createdby_value = _createdby_value;
_x.entityimage_timestamp = entityimage_timestamp;
_x.address1_upszone = address1_upszone;
_x.address2_primarycontactname = address2_primarycontactname;
_x.primarytwitterid = primarytwitterid;
_x.customertypecode = customertypecode;
_x.address2_postofficebox = address2_postofficebox;
_x.statecode = statecode;
_x.address2_city = address2_city;
_x._primarycontactid_value = _primarycontactid_value;
_x.address1_freighttermscode = address1_freighttermscode;
_x.address1_longitude = address1_longitude;
_x.telephone2 = telephone2;
_x.address1_addresstypecode = address1_addresstypecode;
_x.donotbulkpostalmail = donotbulkpostalmail;
_x.lastusedincampaign = lastusedincampaign;
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
_x.creditlimit_base = creditlimit_base;
_x.telephone3 = telephone3;
_x.address1_addressid = address1_addressid;
_x.preferredappointmentdaycode = preferredappointmentdaycode;
_x.websiteurl = websiteurl;
_x.accountclassificationcode = accountclassificationcode;
_x.address2_composite = address2_composite;
_x.name = name;
_x.address2_telephone1 = address2_telephone1;
_x.creditlimit = creditlimit;
_x.lastonholdtime = lastonholdtime;
_x.address2_line2 = address2_line2;
_x.creditonhold = creditonhold;
_x.entityimageid = entityimageid;
_x.accountnumber = accountnumber;
_x.followemail = followemail;
_x.modifiedon = modifiedon;
_x.address1_county = address1_county;
_x.marketcap_base = marketcap_base;
_x.address2_upszone = address2_upszone;
_x.address2_country = address2_country;
_x.address1_telephone2 = address1_telephone2;
_x.address2_freighttermscode = address2_freighttermscode;
_x.address1_utcoffset = address1_utcoffset;
_x.emailaddress2 = emailaddress2;
_x._parentaccountid_value = _parentaccountid_value;
_x.preferredcontactmethodcode = preferredcontactmethodcode;
_x.revenue_base = revenue_base;
_x.address2_telephone2 = address2_telephone2;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && accountid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(accountid, UUID.class));
}
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Account withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Account _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="territorycode")
@JsonIgnore
public Optional getTerritorycode() {
return Optional.ofNullable(territorycode);
}
public Account withTerritorycode(Integer territorycode) {
Account _x = _copy();
_x.changedFields = changedFields.add("territorycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.territorycode = territorycode;
return _x;
}
@Property(name="accountid")
@JsonIgnore
public Optional getAccountid() {
return Optional.ofNullable(accountid);
}
public Account withAccountid(UUID accountid) {
Account _x = _copy();
_x.changedFields = changedFields.add("accountid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.accountid = accountid;
return _x;
}
@Property(name="address1_telephone1")
@JsonIgnore
public Optional getAddress1_telephone1() {
return Optional.ofNullable(address1_telephone1);
}
public Account withAddress1_telephone1(String address1_telephone1) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_telephone1 = address1_telephone1;
return _x;
}
@Property(name="address1_postofficebox")
@JsonIgnore
public Optional getAddress1_postofficebox() {
return Optional.ofNullable(address1_postofficebox);
}
public Account withAddress1_postofficebox(String address1_postofficebox) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_postofficebox = address1_postofficebox;
return _x;
}
@Property(name="address1_line2")
@JsonIgnore
public Optional getAddress1_line2() {
return Optional.ofNullable(address1_line2);
}
public Account withAddress1_line2(String address1_line2) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_line2 = address1_line2;
return _x;
}
@Property(name="emailaddress3")
@JsonIgnore
public Optional getEmailaddress3() {
return Optional.ofNullable(emailaddress3);
}
public Account withEmailaddress3(String emailaddress3) {
Account _x = _copy();
_x.changedFields = changedFields.add("emailaddress3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.emailaddress3 = emailaddress3;
return _x;
}
@Property(name="address1_telephone3")
@JsonIgnore
public Optional getAddress1_telephone3() {
return Optional.ofNullable(address1_telephone3);
}
public Account withAddress1_telephone3(String address1_telephone3) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_telephone3 = address1_telephone3;
return _x;
}
@Property(name="address2_county")
@JsonIgnore
public Optional getAddress2_county() {
return Optional.ofNullable(address2_county);
}
public Account withAddress2_county(String address2_county) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_county = address2_county;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Account withCreatedon(OffsetDateTime createdon) {
Account _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.createdon = createdon;
return _x;
}
@Property(name="numberofemployees")
@JsonIgnore
public Optional getNumberofemployees() {
return Optional.ofNullable(numberofemployees);
}
public Account withNumberofemployees(Integer numberofemployees) {
Account _x = _copy();
_x.changedFields = changedFields.add("numberofemployees");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.numberofemployees = numberofemployees;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Account with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="timespentbymeonemailandmeetings")
@JsonIgnore
public Optional getTimespentbymeonemailandmeetings() {
return Optional.ofNullable(timespentbymeonemailandmeetings);
}
public Account withTimespentbymeonemailandmeetings(String timespentbymeonemailandmeetings) {
Account _x = _copy();
_x.changedFields = changedFields.add("timespentbymeonemailandmeetings");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
return _x;
}
@Property(name="address2_longitude")
@JsonIgnore
public Optional getAddress2_longitude() {
return Optional.ofNullable(address2_longitude);
}
public Account withAddress2_longitude(Double address2_longitude) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_longitude = address2_longitude;
return _x;
}
@Property(name="ownershipcode")
@JsonIgnore
public Optional getOwnershipcode() {
return Optional.ofNullable(ownershipcode);
}
public Account withOwnershipcode(Integer ownershipcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("ownershipcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.ownershipcode = ownershipcode;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Account withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Account _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="primarysatoriid")
@JsonIgnore
public Optional getPrimarysatoriid() {
return Optional.ofNullable(primarysatoriid);
}
public Account withPrimarysatoriid(String primarysatoriid) {
Account _x = _copy();
_x.changedFields = changedFields.add("primarysatoriid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.primarysatoriid = primarysatoriid;
return _x;
}
@Property(name="_masterid_value")
@JsonIgnore
public Optional get_masterid_value() {
return Optional.ofNullable(_masterid_value);
}
public Account with_masterid_value(UUID _masterid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_masterid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._masterid_value = _masterid_value;
return _x;
}
@Property(name="address2_latitude")
@JsonIgnore
public Optional getAddress2_latitude() {
return Optional.ofNullable(address2_latitude);
}
public Account withAddress2_latitude(Double address2_latitude) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_latitude = address2_latitude;
return _x;
}
@Property(name="address2_fax")
@JsonIgnore
public Optional getAddress2_fax() {
return Optional.ofNullable(address2_fax);
}
public Account withAddress2_fax(String address2_fax) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_fax = address2_fax;
return _x;
}
@Property(name="_slaid_value")
@JsonIgnore
public Optional get_slaid_value() {
return Optional.ofNullable(_slaid_value);
}
public Account with_slaid_value(UUID _slaid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_slaid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._slaid_value = _slaid_value;
return _x;
}
@Property(name="aging60_base")
@JsonIgnore
public Optional getAging60_base() {
return Optional.ofNullable(aging60_base);
}
public Account withAging60_base(BigDecimal aging60_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging60_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging60_base = aging60_base;
return _x;
}
@Property(name="telephone1")
@JsonIgnore
public Optional getTelephone1() {
return Optional.ofNullable(telephone1);
}
public Account withTelephone1(String telephone1) {
Account _x = _copy();
_x.changedFields = changedFields.add("telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.telephone1 = telephone1;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Account withEntityimage(byte[] entityimage) {
Account _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.entityimage = entityimage;
return _x;
}
@Property(name="revenue")
@JsonIgnore
public Optional getRevenue() {
return Optional.ofNullable(revenue);
}
public Account withRevenue(BigDecimal revenue) {
Account _x = _copy();
_x.changedFields = changedFields.add("revenue");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.revenue = revenue;
return _x;
}
@Property(name="industrycode")
@JsonIgnore
public Optional getIndustrycode() {
return Optional.ofNullable(industrycode);
}
public Account withIndustrycode(Integer industrycode) {
Account _x = _copy();
_x.changedFields = changedFields.add("industrycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.industrycode = industrycode;
return _x;
}
@Property(name="aging30_base")
@JsonIgnore
public Optional getAging30_base() {
return Optional.ofNullable(aging30_base);
}
public Account withAging30_base(BigDecimal aging30_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging30_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging30_base = aging30_base;
return _x;
}
@Property(name="emailaddress1")
@JsonIgnore
public Optional getEmailaddress1() {
return Optional.ofNullable(emailaddress1);
}
public Account withEmailaddress1(String emailaddress1) {
Account _x = _copy();
_x.changedFields = changedFields.add("emailaddress1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.emailaddress1 = emailaddress1;
return _x;
}
@Property(name="address1_latitude")
@JsonIgnore
public Optional getAddress1_latitude() {
return Optional.ofNullable(address1_latitude);
}
public Account withAddress1_latitude(Double address1_latitude) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_latitude = address1_latitude;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Account with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="tickersymbol")
@JsonIgnore
public Optional getTickersymbol() {
return Optional.ofNullable(tickersymbol);
}
public Account withTickersymbol(String tickersymbol) {
Account _x = _copy();
_x.changedFields = changedFields.add("tickersymbol");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.tickersymbol = tickersymbol;
return _x;
}
@Property(name="address2_shippingmethodcode")
@JsonIgnore
public Optional getAddress2_shippingmethodcode() {
return Optional.ofNullable(address2_shippingmethodcode);
}
public Account withAddress2_shippingmethodcode(Integer address2_shippingmethodcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_shippingmethodcode = address2_shippingmethodcode;
return _x;
}
@Property(name="customersizecode")
@JsonIgnore
public Optional getCustomersizecode() {
return Optional.ofNullable(customersizecode);
}
public Account withCustomersizecode(Integer customersizecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("customersizecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.customersizecode = customersizecode;
return _x;
}
@Property(name="address2_name")
@JsonIgnore
public Optional getAddress2_name() {
return Optional.ofNullable(address2_name);
}
public Account withAddress2_name(String address2_name) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_name = address2_name;
return _x;
}
@Property(name="address1_stateorprovince")
@JsonIgnore
public Optional getAddress1_stateorprovince() {
return Optional.ofNullable(address1_stateorprovince);
}
public Account withAddress1_stateorprovince(String address1_stateorprovince) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_stateorprovince = address1_stateorprovince;
return _x;
}
@Property(name="address1_line3")
@JsonIgnore
public Optional getAddress1_line3() {
return Optional.ofNullable(address1_line3);
}
public Account withAddress1_line3(String address1_line3) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_line3 = address1_line3;
return _x;
}
@Property(name="accountratingcode")
@JsonIgnore
public Optional getAccountratingcode() {
return Optional.ofNullable(accountratingcode);
}
public Account withAccountratingcode(Integer accountratingcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("accountratingcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.accountratingcode = accountratingcode;
return _x;
}
@Property(name="address1_line1")
@JsonIgnore
public Optional getAddress1_line1() {
return Optional.ofNullable(address1_line1);
}
public Account withAddress1_line1(String address1_line1) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_line1 = address1_line1;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Account withImportsequencenumber(Integer importsequencenumber) {
Account _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="paymenttermscode")
@JsonIgnore
public Optional getPaymenttermscode() {
return Optional.ofNullable(paymenttermscode);
}
public Account withPaymenttermscode(Integer paymenttermscode) {
Account _x = _copy();
_x.changedFields = changedFields.add("paymenttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.paymenttermscode = paymenttermscode;
return _x;
}
@Property(name="donotfax")
@JsonIgnore
public Optional getDonotfax() {
return Optional.ofNullable(donotfax);
}
public Account withDonotfax(Boolean donotfax) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotfax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotfax = donotfax;
return _x;
}
@Property(name="aging90_base")
@JsonIgnore
public Optional getAging90_base() {
return Optional.ofNullable(aging90_base);
}
public Account withAging90_base(BigDecimal aging90_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging90_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging90_base = aging90_base;
return _x;
}
@Property(name="businesstypecode")
@JsonIgnore
public Optional getBusinesstypecode() {
return Optional.ofNullable(businesstypecode);
}
public Account withBusinesstypecode(Integer businesstypecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("businesstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.businesstypecode = businesstypecode;
return _x;
}
@Property(name="address2_line3")
@JsonIgnore
public Optional getAddress2_line3() {
return Optional.ofNullable(address2_line3);
}
public Account withAddress2_line3(String address2_line3) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_line3 = address2_line3;
return _x;
}
@Property(name="address2_utcoffset")
@JsonIgnore
public Optional getAddress2_utcoffset() {
return Optional.ofNullable(address2_utcoffset);
}
public Account withAddress2_utcoffset(Integer address2_utcoffset) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_utcoffset = address2_utcoffset;
return _x;
}
@Property(name="donotphone")
@JsonIgnore
public Optional getDonotphone() {
return Optional.ofNullable(donotphone);
}
public Account withDonotphone(Boolean donotphone) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotphone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotphone = donotphone;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Account withExchangerate(BigDecimal exchangerate) {
Account _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Account with_ownerid_value(UUID _ownerid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Account with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Account withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="ftpsiteurl")
@JsonIgnore
public Optional getFtpsiteurl() {
return Optional.ofNullable(ftpsiteurl);
}
public Account withFtpsiteurl(String ftpsiteurl) {
Account _x = _copy();
_x.changedFields = changedFields.add("ftpsiteurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.ftpsiteurl = ftpsiteurl;
return _x;
}
@Property(name="shippingmethodcode")
@JsonIgnore
public Optional getShippingmethodcode() {
return Optional.ofNullable(shippingmethodcode);
}
public Account withShippingmethodcode(Integer shippingmethodcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.shippingmethodcode = shippingmethodcode;
return _x;
}
@Property(name="stockexchange")
@JsonIgnore
public Optional getStockexchange() {
return Optional.ofNullable(stockexchange);
}
public Account withStockexchange(String stockexchange) {
Account _x = _copy();
_x.changedFields = changedFields.add("stockexchange");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.stockexchange = stockexchange;
return _x;
}
@Property(name="address2_telephone3")
@JsonIgnore
public Optional getAddress2_telephone3() {
return Optional.ofNullable(address2_telephone3);
}
public Account withAddress2_telephone3(String address2_telephone3) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_telephone3 = address2_telephone3;
return _x;
}
@Property(name="address2_line1")
@JsonIgnore
public Optional getAddress2_line1() {
return Optional.ofNullable(address2_line1);
}
public Account withAddress2_line1(String address2_line1) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_line1 = address2_line1;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Account with_owningteam_value(UUID _owningteam_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="aging60")
@JsonIgnore
public Optional getAging60() {
return Optional.ofNullable(aging60);
}
public Account withAging60(BigDecimal aging60) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging60");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging60 = aging60;
return _x;
}
@Property(name="participatesinworkflow")
@JsonIgnore
public Optional getParticipatesinworkflow() {
return Optional.ofNullable(participatesinworkflow);
}
public Account withParticipatesinworkflow(Boolean participatesinworkflow) {
Account _x = _copy();
_x.changedFields = changedFields.add("participatesinworkflow");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.participatesinworkflow = participatesinworkflow;
return _x;
}
@Property(name="address1_postalcode")
@JsonIgnore
public Optional getAddress1_postalcode() {
return Optional.ofNullable(address1_postalcode);
}
public Account withAddress1_postalcode(String address1_postalcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_postalcode = address1_postalcode;
return _x;
}
@Property(name="address2_addressid")
@JsonIgnore
public Optional getAddress2_addressid() {
return Optional.ofNullable(address2_addressid);
}
public Account withAddress2_addressid(UUID address2_addressid) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_addressid = address2_addressid;
return _x;
}
@Property(name="marketcap")
@JsonIgnore
public Optional getMarketcap() {
return Optional.ofNullable(marketcap);
}
public Account withMarketcap(BigDecimal marketcap) {
Account _x = _copy();
_x.changedFields = changedFields.add("marketcap");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.marketcap = marketcap;
return _x;
}
@Property(name="address2_addresstypecode")
@JsonIgnore
public Optional getAddress2_addresstypecode() {
return Optional.ofNullable(address2_addresstypecode);
}
public Account withAddress2_addresstypecode(Integer address2_addresstypecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_addresstypecode = address2_addresstypecode;
return _x;
}
@Property(name="aging90")
@JsonIgnore
public Optional getAging90() {
return Optional.ofNullable(aging90);
}
public Account withAging90(BigDecimal aging90) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging90");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging90 = aging90;
return _x;
}
@Property(name="donotbulkemail")
@JsonIgnore
public Optional getDonotbulkemail() {
return Optional.ofNullable(donotbulkemail);
}
public Account withDonotbulkemail(Boolean donotbulkemail) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotbulkemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotbulkemail = donotbulkemail;
return _x;
}
@Property(name="aging30")
@JsonIgnore
public Optional getAging30() {
return Optional.ofNullable(aging30);
}
public Account withAging30(BigDecimal aging30) {
Account _x = _copy();
_x.changedFields = changedFields.add("aging30");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.aging30 = aging30;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Account withEntityimage_url(String entityimage_url) {
Account _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="sic")
@JsonIgnore
public Optional getSic() {
return Optional.ofNullable(sic);
}
public Account withSic(String sic) {
Account _x = _copy();
_x.changedFields = changedFields.add("sic");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.sic = sic;
return _x;
}
@Property(name="sharesoutstanding")
@JsonIgnore
public Optional getSharesoutstanding() {
return Optional.ofNullable(sharesoutstanding);
}
public Account withSharesoutstanding(Integer sharesoutstanding) {
Account _x = _copy();
_x.changedFields = changedFields.add("sharesoutstanding");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.sharesoutstanding = sharesoutstanding;
return _x;
}
@Property(name="accountcategorycode")
@JsonIgnore
public Optional getAccountcategorycode() {
return Optional.ofNullable(accountcategorycode);
}
public Account withAccountcategorycode(Integer accountcategorycode) {
Account _x = _copy();
_x.changedFields = changedFields.add("accountcategorycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.accountcategorycode = accountcategorycode;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Account with_owninguser_value(UUID _owninguser_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="stageid")
@JsonIgnore
public Optional getStageid() {
return Optional.ofNullable(stageid);
}
public Account withStageid(UUID stageid) {
Account _x = _copy();
_x.changedFields = changedFields.add("stageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.stageid = stageid;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Account withVersionnumber(Long versionnumber) {
Account _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="preferredappointmenttimecode")
@JsonIgnore
public Optional getPreferredappointmenttimecode() {
return Optional.ofNullable(preferredappointmenttimecode);
}
public Account withPreferredappointmenttimecode(Integer preferredappointmenttimecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("preferredappointmenttimecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.preferredappointmenttimecode = preferredappointmenttimecode;
return _x;
}
@Property(name="donotsendmm")
@JsonIgnore
public Optional getDonotsendmm() {
return Optional.ofNullable(donotsendmm);
}
public Account withDonotsendmm(Boolean donotsendmm) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotsendmm");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotsendmm = donotsendmm;
return _x;
}
@Property(name="address1_name")
@JsonIgnore
public Optional getAddress1_name() {
return Optional.ofNullable(address1_name);
}
public Account withAddress1_name(String address1_name) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_name = address1_name;
return _x;
}
@Property(name="fax")
@JsonIgnore
public Optional getFax() {
return Optional.ofNullable(fax);
}
public Account withFax(String fax) {
Account _x = _copy();
_x.changedFields = changedFields.add("fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.fax = fax;
return _x;
}
@Property(name="address1_fax")
@JsonIgnore
public Optional getAddress1_fax() {
return Optional.ofNullable(address1_fax);
}
public Account withAddress1_fax(String address1_fax) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_fax = address1_fax;
return _x;
}
@Property(name="merged")
@JsonIgnore
public Optional getMerged() {
return Optional.ofNullable(merged);
}
public Account withMerged(Boolean merged) {
Account _x = _copy();
_x.changedFields = changedFields.add("merged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.merged = merged;
return _x;
}
@Property(name="address1_city")
@JsonIgnore
public Optional getAddress1_city() {
return Optional.ofNullable(address1_city);
}
public Account withAddress1_city(String address1_city) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_city = address1_city;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Account withDescription(String description) {
Account _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.description = description;
return _x;
}
@Property(name="address2_stateorprovince")
@JsonIgnore
public Optional getAddress2_stateorprovince() {
return Optional.ofNullable(address2_stateorprovince);
}
public Account withAddress2_stateorprovince(String address2_stateorprovince) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_stateorprovince = address2_stateorprovince;
return _x;
}
@Property(name="yominame")
@JsonIgnore
public Optional getYominame() {
return Optional.ofNullable(yominame);
}
public Account withYominame(String yominame) {
Account _x = _copy();
_x.changedFields = changedFields.add("yominame");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.yominame = yominame;
return _x;
}
@Property(name="donotpostalmail")
@JsonIgnore
public Optional getDonotpostalmail() {
return Optional.ofNullable(donotpostalmail);
}
public Account withDonotpostalmail(Boolean donotpostalmail) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotpostalmail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotpostalmail = donotpostalmail;
return _x;
}
@Property(name="address1_country")
@JsonIgnore
public Optional getAddress1_country() {
return Optional.ofNullable(address1_country);
}
public Account withAddress1_country(String address1_country) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_country = address1_country;
return _x;
}
@Property(name="donotemail")
@JsonIgnore
public Optional getDonotemail() {
return Optional.ofNullable(donotemail);
}
public Account withDonotemail(Boolean donotemail) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotemail = donotemail;
return _x;
}
@Property(name="address1_composite")
@JsonIgnore
public Optional getAddress1_composite() {
return Optional.ofNullable(address1_composite);
}
public Account withAddress1_composite(String address1_composite) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_composite = address1_composite;
return _x;
}
@Property(name="_slainvokedid_value")
@JsonIgnore
public Optional get_slainvokedid_value() {
return Optional.ofNullable(_slainvokedid_value);
}
public Account with_slainvokedid_value(UUID _slainvokedid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_slainvokedid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._slainvokedid_value = _slainvokedid_value;
return _x;
}
@Property(name="address1_primarycontactname")
@JsonIgnore
public Optional getAddress1_primarycontactname() {
return Optional.ofNullable(address1_primarycontactname);
}
public Account withAddress1_primarycontactname(String address1_primarycontactname) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_primarycontactname = address1_primarycontactname;
return _x;
}
@Property(name="onholdtime")
@JsonIgnore
public Optional getOnholdtime() {
return Optional.ofNullable(onholdtime);
}
public Account withOnholdtime(Integer onholdtime) {
Account _x = _copy();
_x.changedFields = changedFields.add("onholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.onholdtime = onholdtime;
return _x;
}
@Property(name="_createdbyexternalparty_value")
@JsonIgnore
public Optional get_createdbyexternalparty_value() {
return Optional.ofNullable(_createdbyexternalparty_value);
}
public Account with_createdbyexternalparty_value(UUID _createdbyexternalparty_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_createdbyexternalparty_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
return _x;
}
@Property(name="marketingonly")
@JsonIgnore
public Optional getMarketingonly() {
return Optional.ofNullable(marketingonly);
}
public Account withMarketingonly(Boolean marketingonly) {
Account _x = _copy();
_x.changedFields = changedFields.add("marketingonly");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.marketingonly = marketingonly;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Account withStatuscode(Integer statuscode) {
Account _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.statuscode = statuscode;
return _x;
}
@Property(name="traversedpath")
@JsonIgnore
public Optional getTraversedpath() {
return Optional.ofNullable(traversedpath);
}
public Account withTraversedpath(String traversedpath) {
Account _x = _copy();
_x.changedFields = changedFields.add("traversedpath");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.traversedpath = traversedpath;
return _x;
}
@Property(name="processid")
@JsonIgnore
public Optional getProcessid() {
return Optional.ofNullable(processid);
}
public Account withProcessid(UUID processid) {
Account _x = _copy();
_x.changedFields = changedFields.add("processid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.processid = processid;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Account with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="_modifiedbyexternalparty_value")
@JsonIgnore
public Optional get_modifiedbyexternalparty_value() {
return Optional.ofNullable(_modifiedbyexternalparty_value);
}
public Account with_modifiedbyexternalparty_value(UUID _modifiedbyexternalparty_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_modifiedbyexternalparty_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
return _x;
}
@Property(name="address2_postalcode")
@JsonIgnore
public Optional getAddress2_postalcode() {
return Optional.ofNullable(address2_postalcode);
}
public Account withAddress2_postalcode(String address2_postalcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_postalcode = address2_postalcode;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Account with_modifiedby_value(UUID _modifiedby_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="address1_shippingmethodcode")
@JsonIgnore
public Optional getAddress1_shippingmethodcode() {
return Optional.ofNullable(address1_shippingmethodcode);
}
public Account withAddress1_shippingmethodcode(Integer address1_shippingmethodcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_shippingmethodcode = address1_shippingmethodcode;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Account with_createdby_value(UUID _createdby_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Account withEntityimage_timestamp(Long entityimage_timestamp) {
Account _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="address1_upszone")
@JsonIgnore
public Optional getAddress1_upszone() {
return Optional.ofNullable(address1_upszone);
}
public Account withAddress1_upszone(String address1_upszone) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_upszone = address1_upszone;
return _x;
}
@Property(name="address2_primarycontactname")
@JsonIgnore
public Optional getAddress2_primarycontactname() {
return Optional.ofNullable(address2_primarycontactname);
}
public Account withAddress2_primarycontactname(String address2_primarycontactname) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_primarycontactname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_primarycontactname = address2_primarycontactname;
return _x;
}
@Property(name="primarytwitterid")
@JsonIgnore
public Optional getPrimarytwitterid() {
return Optional.ofNullable(primarytwitterid);
}
public Account withPrimarytwitterid(String primarytwitterid) {
Account _x = _copy();
_x.changedFields = changedFields.add("primarytwitterid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.primarytwitterid = primarytwitterid;
return _x;
}
@Property(name="customertypecode")
@JsonIgnore
public Optional getCustomertypecode() {
return Optional.ofNullable(customertypecode);
}
public Account withCustomertypecode(Integer customertypecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("customertypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.customertypecode = customertypecode;
return _x;
}
@Property(name="address2_postofficebox")
@JsonIgnore
public Optional getAddress2_postofficebox() {
return Optional.ofNullable(address2_postofficebox);
}
public Account withAddress2_postofficebox(String address2_postofficebox) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_postofficebox = address2_postofficebox;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Account withStatecode(Integer statecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.statecode = statecode;
return _x;
}
@Property(name="address2_city")
@JsonIgnore
public Optional getAddress2_city() {
return Optional.ofNullable(address2_city);
}
public Account withAddress2_city(String address2_city) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_city = address2_city;
return _x;
}
@Property(name="_primarycontactid_value")
@JsonIgnore
public Optional get_primarycontactid_value() {
return Optional.ofNullable(_primarycontactid_value);
}
public Account with_primarycontactid_value(UUID _primarycontactid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_primarycontactid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._primarycontactid_value = _primarycontactid_value;
return _x;
}
@Property(name="address1_freighttermscode")
@JsonIgnore
public Optional getAddress1_freighttermscode() {
return Optional.ofNullable(address1_freighttermscode);
}
public Account withAddress1_freighttermscode(Integer address1_freighttermscode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_freighttermscode = address1_freighttermscode;
return _x;
}
@Property(name="address1_longitude")
@JsonIgnore
public Optional getAddress1_longitude() {
return Optional.ofNullable(address1_longitude);
}
public Account withAddress1_longitude(Double address1_longitude) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_longitude = address1_longitude;
return _x;
}
@Property(name="telephone2")
@JsonIgnore
public Optional getTelephone2() {
return Optional.ofNullable(telephone2);
}
public Account withTelephone2(String telephone2) {
Account _x = _copy();
_x.changedFields = changedFields.add("telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.telephone2 = telephone2;
return _x;
}
@Property(name="address1_addresstypecode")
@JsonIgnore
public Optional getAddress1_addresstypecode() {
return Optional.ofNullable(address1_addresstypecode);
}
public Account withAddress1_addresstypecode(Integer address1_addresstypecode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_addresstypecode = address1_addresstypecode;
return _x;
}
@Property(name="donotbulkpostalmail")
@JsonIgnore
public Optional getDonotbulkpostalmail() {
return Optional.ofNullable(donotbulkpostalmail);
}
public Account withDonotbulkpostalmail(Boolean donotbulkpostalmail) {
Account _x = _copy();
_x.changedFields = changedFields.add("donotbulkpostalmail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.donotbulkpostalmail = donotbulkpostalmail;
return _x;
}
@Property(name="lastusedincampaign")
@JsonIgnore
public Optional getLastusedincampaign() {
return Optional.ofNullable(lastusedincampaign);
}
public Account withLastusedincampaign(OffsetDateTime lastusedincampaign) {
Account _x = _copy();
_x.changedFields = changedFields.add("lastusedincampaign");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.lastusedincampaign = lastusedincampaign;
return _x;
}
@Property(name="_preferredsystemuserid_value")
@JsonIgnore
public Optional get_preferredsystemuserid_value() {
return Optional.ofNullable(_preferredsystemuserid_value);
}
public Account with_preferredsystemuserid_value(UUID _preferredsystemuserid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_preferredsystemuserid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
return _x;
}
@Property(name="creditlimit_base")
@JsonIgnore
public Optional getCreditlimit_base() {
return Optional.ofNullable(creditlimit_base);
}
public Account withCreditlimit_base(BigDecimal creditlimit_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("creditlimit_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.creditlimit_base = creditlimit_base;
return _x;
}
@Property(name="telephone3")
@JsonIgnore
public Optional getTelephone3() {
return Optional.ofNullable(telephone3);
}
public Account withTelephone3(String telephone3) {
Account _x = _copy();
_x.changedFields = changedFields.add("telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.telephone3 = telephone3;
return _x;
}
@Property(name="address1_addressid")
@JsonIgnore
public Optional getAddress1_addressid() {
return Optional.ofNullable(address1_addressid);
}
public Account withAddress1_addressid(UUID address1_addressid) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_addressid = address1_addressid;
return _x;
}
@Property(name="preferredappointmentdaycode")
@JsonIgnore
public Optional getPreferredappointmentdaycode() {
return Optional.ofNullable(preferredappointmentdaycode);
}
public Account withPreferredappointmentdaycode(Integer preferredappointmentdaycode) {
Account _x = _copy();
_x.changedFields = changedFields.add("preferredappointmentdaycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.preferredappointmentdaycode = preferredappointmentdaycode;
return _x;
}
@Property(name="websiteurl")
@JsonIgnore
public Optional getWebsiteurl() {
return Optional.ofNullable(websiteurl);
}
public Account withWebsiteurl(String websiteurl) {
Account _x = _copy();
_x.changedFields = changedFields.add("websiteurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.websiteurl = websiteurl;
return _x;
}
@Property(name="accountclassificationcode")
@JsonIgnore
public Optional getAccountclassificationcode() {
return Optional.ofNullable(accountclassificationcode);
}
public Account withAccountclassificationcode(Integer accountclassificationcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("accountclassificationcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.accountclassificationcode = accountclassificationcode;
return _x;
}
@Property(name="address2_composite")
@JsonIgnore
public Optional getAddress2_composite() {
return Optional.ofNullable(address2_composite);
}
public Account withAddress2_composite(String address2_composite) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_composite = address2_composite;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Account withName(String name) {
Account _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.name = name;
return _x;
}
@Property(name="address2_telephone1")
@JsonIgnore
public Optional getAddress2_telephone1() {
return Optional.ofNullable(address2_telephone1);
}
public Account withAddress2_telephone1(String address2_telephone1) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_telephone1 = address2_telephone1;
return _x;
}
@Property(name="creditlimit")
@JsonIgnore
public Optional getCreditlimit() {
return Optional.ofNullable(creditlimit);
}
public Account withCreditlimit(BigDecimal creditlimit) {
Account _x = _copy();
_x.changedFields = changedFields.add("creditlimit");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.creditlimit = creditlimit;
return _x;
}
@Property(name="lastonholdtime")
@JsonIgnore
public Optional getLastonholdtime() {
return Optional.ofNullable(lastonholdtime);
}
public Account withLastonholdtime(OffsetDateTime lastonholdtime) {
Account _x = _copy();
_x.changedFields = changedFields.add("lastonholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.lastonholdtime = lastonholdtime;
return _x;
}
@Property(name="address2_line2")
@JsonIgnore
public Optional getAddress2_line2() {
return Optional.ofNullable(address2_line2);
}
public Account withAddress2_line2(String address2_line2) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_line2 = address2_line2;
return _x;
}
@Property(name="creditonhold")
@JsonIgnore
public Optional getCreditonhold() {
return Optional.ofNullable(creditonhold);
}
public Account withCreditonhold(Boolean creditonhold) {
Account _x = _copy();
_x.changedFields = changedFields.add("creditonhold");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.creditonhold = creditonhold;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Account withEntityimageid(UUID entityimageid) {
Account _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="accountnumber")
@JsonIgnore
public Optional getAccountnumber() {
return Optional.ofNullable(accountnumber);
}
public Account withAccountnumber(String accountnumber) {
Account _x = _copy();
_x.changedFields = changedFields.add("accountnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.accountnumber = accountnumber;
return _x;
}
@Property(name="followemail")
@JsonIgnore
public Optional getFollowemail() {
return Optional.ofNullable(followemail);
}
public Account withFollowemail(Boolean followemail) {
Account _x = _copy();
_x.changedFields = changedFields.add("followemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.followemail = followemail;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Account withModifiedon(OffsetDateTime modifiedon) {
Account _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="address1_county")
@JsonIgnore
public Optional getAddress1_county() {
return Optional.ofNullable(address1_county);
}
public Account withAddress1_county(String address1_county) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_county = address1_county;
return _x;
}
@Property(name="marketcap_base")
@JsonIgnore
public Optional getMarketcap_base() {
return Optional.ofNullable(marketcap_base);
}
public Account withMarketcap_base(BigDecimal marketcap_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("marketcap_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.marketcap_base = marketcap_base;
return _x;
}
@Property(name="address2_upszone")
@JsonIgnore
public Optional getAddress2_upszone() {
return Optional.ofNullable(address2_upszone);
}
public Account withAddress2_upszone(String address2_upszone) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_upszone = address2_upszone;
return _x;
}
@Property(name="address2_country")
@JsonIgnore
public Optional getAddress2_country() {
return Optional.ofNullable(address2_country);
}
public Account withAddress2_country(String address2_country) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_country = address2_country;
return _x;
}
@Property(name="address1_telephone2")
@JsonIgnore
public Optional getAddress1_telephone2() {
return Optional.ofNullable(address1_telephone2);
}
public Account withAddress1_telephone2(String address1_telephone2) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_telephone2 = address1_telephone2;
return _x;
}
@Property(name="address2_freighttermscode")
@JsonIgnore
public Optional getAddress2_freighttermscode() {
return Optional.ofNullable(address2_freighttermscode);
}
public Account withAddress2_freighttermscode(Integer address2_freighttermscode) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_freighttermscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_freighttermscode = address2_freighttermscode;
return _x;
}
@Property(name="address1_utcoffset")
@JsonIgnore
public Optional getAddress1_utcoffset() {
return Optional.ofNullable(address1_utcoffset);
}
public Account withAddress1_utcoffset(Integer address1_utcoffset) {
Account _x = _copy();
_x.changedFields = changedFields.add("address1_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address1_utcoffset = address1_utcoffset;
return _x;
}
@Property(name="emailaddress2")
@JsonIgnore
public Optional getEmailaddress2() {
return Optional.ofNullable(emailaddress2);
}
public Account withEmailaddress2(String emailaddress2) {
Account _x = _copy();
_x.changedFields = changedFields.add("emailaddress2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.emailaddress2 = emailaddress2;
return _x;
}
@Property(name="_parentaccountid_value")
@JsonIgnore
public Optional get_parentaccountid_value() {
return Optional.ofNullable(_parentaccountid_value);
}
public Account with_parentaccountid_value(UUID _parentaccountid_value) {
Account _x = _copy();
_x.changedFields = changedFields.add("_parentaccountid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x._parentaccountid_value = _parentaccountid_value;
return _x;
}
@Property(name="preferredcontactmethodcode")
@JsonIgnore
public Optional getPreferredcontactmethodcode() {
return Optional.ofNullable(preferredcontactmethodcode);
}
public Account withPreferredcontactmethodcode(Integer preferredcontactmethodcode) {
Account _x = _copy();
_x.changedFields = changedFields.add("preferredcontactmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.preferredcontactmethodcode = preferredcontactmethodcode;
return _x;
}
@Property(name="revenue_base")
@JsonIgnore
public Optional getRevenue_base() {
return Optional.ofNullable(revenue_base);
}
public Account withRevenue_base(BigDecimal revenue_base) {
Account _x = _copy();
_x.changedFields = changedFields.add("revenue_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.revenue_base = revenue_base;
return _x;
}
@Property(name="address2_telephone2")
@JsonIgnore
public Optional getAddress2_telephone2() {
return Optional.ofNullable(address2_telephone2);
}
public Account withAddress2_telephone2(String address2_telephone2) {
Account _x = _copy();
_x.changedFields = changedFields.add("address2_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.account");
_x.address2_telephone2 = address2_telephone2;
return _x;
}
public Account withUnmappedField(String name, Object value) {
Account _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="account_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getAccount_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("account_principalobjectattributeaccess"), RequestHelper.getValue(unmappedFields, "account_principalobjectattributeaccess"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="Account_ActivityPointers")
@JsonIgnore
public ActivitypointerCollectionRequest getAccount_ActivityPointers() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("Account_ActivityPointers"), RequestHelper.getValue(unmappedFields, "Account_ActivityPointers"));
}
@NavigationProperty(name="Account_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getAccount_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Account_SyncErrors"), RequestHelper.getValue(unmappedFields, "Account_SyncErrors"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="Account_Email_SendersAccount")
@JsonIgnore
public EmailCollectionRequest getAccount_Email_SendersAccount() {
return new EmailCollectionRequest(
contextPath.addSegment("Account_Email_SendersAccount"), RequestHelper.getValue(unmappedFields, "Account_Email_SendersAccount"));
}
@NavigationProperty(name="Account_Email_EmailSender")
@JsonIgnore
public EmailCollectionRequest getAccount_Email_EmailSender() {
return new EmailCollectionRequest(
contextPath.addSegment("Account_Email_EmailSender"), RequestHelper.getValue(unmappedFields, "Account_Email_EmailSender"));
}
@NavigationProperty(name="Account_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getAccount_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Account_Annotation"), RequestHelper.getValue(unmappedFields, "Account_Annotation"));
}
@NavigationProperty(name="Account_SharepointDocumentLocation")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getAccount_SharepointDocumentLocation() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("Account_SharepointDocumentLocation"), RequestHelper.getValue(unmappedFields, "Account_SharepointDocumentLocation"));
}
@NavigationProperty(name="sla_account_sla")
@JsonIgnore
public SlaRequest getSla_account_sla() {
return new SlaRequest(contextPath.addSegment("sla_account_sla"), RequestHelper.getValue(unmappedFields, "sla_account_sla"));
}
@NavigationProperty(name="account_connections2")
@JsonIgnore
public ConnectionCollectionRequest getAccount_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("account_connections2"), RequestHelper.getValue(unmappedFields, "account_connections2"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="account_PostFollows")
@JsonIgnore
public PostfollowCollectionRequest getAccount_PostFollows() {
return new PostfollowCollectionRequest(
contextPath.addSegment("account_PostFollows"), RequestHelper.getValue(unmappedFields, "account_PostFollows"));
}
@NavigationProperty(name="account_PostRegardings")
@JsonIgnore
public PostregardingCollectionRequest getAccount_PostRegardings() {
return new PostregardingCollectionRequest(
contextPath.addSegment("account_PostRegardings"), RequestHelper.getValue(unmappedFields, "account_PostRegardings"));
}
@NavigationProperty(name="Account_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getAccount_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Account_ProcessSessions"), RequestHelper.getValue(unmappedFields, "Account_ProcessSessions"));
}
@NavigationProperty(name="Account_Faxes")
@JsonIgnore
public FaxCollectionRequest getAccount_Faxes() {
return new FaxCollectionRequest(
contextPath.addSegment("Account_Faxes"), RequestHelper.getValue(unmappedFields, "Account_Faxes"));
}
@NavigationProperty(name="masterid")
@JsonIgnore
public AccountRequest getMasterid() {
return new AccountRequest(contextPath.addSegment("masterid"), RequestHelper.getValue(unmappedFields, "masterid"));
}
@NavigationProperty(name="account_master_account")
@JsonIgnore
public AccountCollectionRequest getAccount_master_account() {
return new AccountCollectionRequest(
contextPath.addSegment("account_master_account"), RequestHelper.getValue(unmappedFields, "account_master_account"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="Account_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getAccount_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Account_AsyncOperations"), RequestHelper.getValue(unmappedFields, "Account_AsyncOperations"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@NavigationProperty(name="Account_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getAccount_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Account_DuplicateBaseRecord"), RequestHelper.getValue(unmappedFields, "Account_DuplicateBaseRecord"));
}
@NavigationProperty(name="preferredsystemuserid")
@JsonIgnore
public SystemuserRequest getPreferredsystemuserid() {
return new SystemuserRequest(contextPath.addSegment("preferredsystemuserid"), RequestHelper.getValue(unmappedFields, "preferredsystemuserid"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="Account_RecurringAppointmentMasters")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getAccount_RecurringAppointmentMasters() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("Account_RecurringAppointmentMasters"), RequestHelper.getValue(unmappedFields, "Account_RecurringAppointmentMasters"));
}
@NavigationProperty(name="Account_Phonecalls")
@JsonIgnore
public PhonecallCollectionRequest getAccount_Phonecalls() {
return new PhonecallCollectionRequest(
contextPath.addSegment("Account_Phonecalls"), RequestHelper.getValue(unmappedFields, "Account_Phonecalls"));
}
@NavigationProperty(name="primarycontactid")
@JsonIgnore
public ContactRequest getPrimarycontactid() {
return new ContactRequest(contextPath.addSegment("primarycontactid"), RequestHelper.getValue(unmappedFields, "primarycontactid"));
}
@NavigationProperty(name="Account_SocialActivities")
@JsonIgnore
public SocialactivityCollectionRequest getAccount_SocialActivities() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("Account_SocialActivities"), RequestHelper.getValue(unmappedFields, "Account_SocialActivities"));
}
@NavigationProperty(name="Account_MailboxTrackingFolder")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getAccount_MailboxTrackingFolder() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("Account_MailboxTrackingFolder"), RequestHelper.getValue(unmappedFields, "Account_MailboxTrackingFolder"));
}
@NavigationProperty(name="SocialActivity_PostAuthorAccount_accounts")
@JsonIgnore
public SocialactivityCollectionRequest getSocialActivity_PostAuthorAccount_accounts() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("SocialActivity_PostAuthorAccount_accounts"), RequestHelper.getValue(unmappedFields, "SocialActivity_PostAuthorAccount_accounts"));
}
@NavigationProperty(name="Account_CustomerAddress")
@JsonIgnore
public CustomeraddressCollectionRequest getAccount_CustomerAddress() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("Account_CustomerAddress"), RequestHelper.getValue(unmappedFields, "Account_CustomerAddress"));
}
@NavigationProperty(name="slakpiinstance_account")
@JsonIgnore
public SlakpiinstanceCollectionRequest getSlakpiinstance_account() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("slakpiinstance_account"), RequestHelper.getValue(unmappedFields, "slakpiinstance_account"));
}
@NavigationProperty(name="Account_Tasks")
@JsonIgnore
public TaskCollectionRequest getAccount_Tasks() {
return new TaskCollectionRequest(
contextPath.addSegment("Account_Tasks"), RequestHelper.getValue(unmappedFields, "Account_Tasks"));
}
@NavigationProperty(name="slainvokedid_account_sla")
@JsonIgnore
public SlaRequest getSlainvokedid_account_sla() {
return new SlaRequest(contextPath.addSegment("slainvokedid_account_sla"), RequestHelper.getValue(unmappedFields, "slainvokedid_account_sla"));
}
@NavigationProperty(name="Account_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getAccount_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Account_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "Account_BulkDeleteFailures"));
}
@NavigationProperty(name="SocialActivity_PostAuthor_accounts")
@JsonIgnore
public SocialactivityCollectionRequest getSocialActivity_PostAuthor_accounts() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("SocialActivity_PostAuthor_accounts"), RequestHelper.getValue(unmappedFields, "SocialActivity_PostAuthor_accounts"));
}
@NavigationProperty(name="contact_customer_accounts")
@JsonIgnore
public ContactCollectionRequest getContact_customer_accounts() {
return new ContactCollectionRequest(
contextPath.addSegment("contact_customer_accounts"), RequestHelper.getValue(unmappedFields, "contact_customer_accounts"));
}
@NavigationProperty(name="Account_Appointments")
@JsonIgnore
public AppointmentCollectionRequest getAccount_Appointments() {
return new AppointmentCollectionRequest(
contextPath.addSegment("Account_Appointments"), RequestHelper.getValue(unmappedFields, "Account_Appointments"));
}
@NavigationProperty(name="Account_Emails")
@JsonIgnore
public EmailCollectionRequest getAccount_Emails() {
return new EmailCollectionRequest(
contextPath.addSegment("Account_Emails"), RequestHelper.getValue(unmappedFields, "Account_Emails"));
}
@NavigationProperty(name="parentaccountid")
@JsonIgnore
public AccountRequest getParentaccountid() {
return new AccountRequest(contextPath.addSegment("parentaccountid"), RequestHelper.getValue(unmappedFields, "parentaccountid"));
}
@NavigationProperty(name="account_parent_account")
@JsonIgnore
public AccountCollectionRequest getAccount_parent_account() {
return new AccountCollectionRequest(
contextPath.addSegment("account_parent_account"), RequestHelper.getValue(unmappedFields, "account_parent_account"));
}
@NavigationProperty(name="Socialprofile_customer_accounts")
@JsonIgnore
public SocialprofileCollectionRequest getSocialprofile_customer_accounts() {
return new SocialprofileCollectionRequest(
contextPath.addSegment("Socialprofile_customer_accounts"), RequestHelper.getValue(unmappedFields, "Socialprofile_customer_accounts"));
}
@NavigationProperty(name="Account_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getAccount_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Account_DuplicateMatchingRecord"), RequestHelper.getValue(unmappedFields, "Account_DuplicateMatchingRecord"));
}
@NavigationProperty(name="account_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getAccount_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("account_activity_parties"), RequestHelper.getValue(unmappedFields, "account_activity_parties"));
}
@NavigationProperty(name="account_connections1")
@JsonIgnore
public ConnectionCollectionRequest getAccount_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("account_connections1"), RequestHelper.getValue(unmappedFields, "account_connections1"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="account_actioncard")
@JsonIgnore
public ActioncardCollectionRequest getAccount_actioncard() {
return new ActioncardCollectionRequest(
contextPath.addSegment("account_actioncard"), RequestHelper.getValue(unmappedFields, "account_actioncard"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"), RequestHelper.getValue(unmappedFields, "owninguser"));
}
@NavigationProperty(name="Account_Letters")
@JsonIgnore
public LetterCollectionRequest getAccount_Letters() {
return new LetterCollectionRequest(
contextPath.addSegment("Account_Letters"), RequestHelper.getValue(unmappedFields, "Account_Letters"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"), RequestHelper.getValue(unmappedFields, "stageid_processstage"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Account patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Account _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Account put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Account _x = _copy();
_x.changedFields = null;
return _x;
}
private Account _copy() {
Account _x = new Account();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.overriddencreatedon = overriddencreatedon;
_x.territorycode = territorycode;
_x.accountid = accountid;
_x.address1_telephone1 = address1_telephone1;
_x.address1_postofficebox = address1_postofficebox;
_x.address1_line2 = address1_line2;
_x.emailaddress3 = emailaddress3;
_x.address1_telephone3 = address1_telephone3;
_x.address2_county = address2_county;
_x.createdon = createdon;
_x.numberofemployees = numberofemployees;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.timespentbymeonemailandmeetings = timespentbymeonemailandmeetings;
_x.address2_longitude = address2_longitude;
_x.ownershipcode = ownershipcode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.primarysatoriid = primarysatoriid;
_x._masterid_value = _masterid_value;
_x.address2_latitude = address2_latitude;
_x.address2_fax = address2_fax;
_x._slaid_value = _slaid_value;
_x.aging60_base = aging60_base;
_x.telephone1 = telephone1;
_x.entityimage = entityimage;
_x.revenue = revenue;
_x.industrycode = industrycode;
_x.aging30_base = aging30_base;
_x.emailaddress1 = emailaddress1;
_x.address1_latitude = address1_latitude;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.tickersymbol = tickersymbol;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.customersizecode = customersizecode;
_x.address2_name = address2_name;
_x.address1_stateorprovince = address1_stateorprovince;
_x.address1_line3 = address1_line3;
_x.accountratingcode = accountratingcode;
_x.address1_line1 = address1_line1;
_x.importsequencenumber = importsequencenumber;
_x.paymenttermscode = paymenttermscode;
_x.donotfax = donotfax;
_x.aging90_base = aging90_base;
_x.businesstypecode = businesstypecode;
_x.address2_line3 = address2_line3;
_x.address2_utcoffset = address2_utcoffset;
_x.donotphone = donotphone;
_x.exchangerate = exchangerate;
_x._ownerid_value = _ownerid_value;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.ftpsiteurl = ftpsiteurl;
_x.shippingmethodcode = shippingmethodcode;
_x.stockexchange = stockexchange;
_x.address2_telephone3 = address2_telephone3;
_x.address2_line1 = address2_line1;
_x._owningteam_value = _owningteam_value;
_x.aging60 = aging60;
_x.participatesinworkflow = participatesinworkflow;
_x.address1_postalcode = address1_postalcode;
_x.address2_addressid = address2_addressid;
_x.marketcap = marketcap;
_x.address2_addresstypecode = address2_addresstypecode;
_x.aging90 = aging90;
_x.donotbulkemail = donotbulkemail;
_x.aging30 = aging30;
_x.entityimage_url = entityimage_url;
_x.sic = sic;
_x.sharesoutstanding = sharesoutstanding;
_x.accountcategorycode = accountcategorycode;
_x._owninguser_value = _owninguser_value;
_x.stageid = stageid;
_x.versionnumber = versionnumber;
_x.preferredappointmenttimecode = preferredappointmenttimecode;
_x.donotsendmm = donotsendmm;
_x.address1_name = address1_name;
_x.fax = fax;
_x.address1_fax = address1_fax;
_x.merged = merged;
_x.address1_city = address1_city;
_x.description = description;
_x.address2_stateorprovince = address2_stateorprovince;
_x.yominame = yominame;
_x.donotpostalmail = donotpostalmail;
_x.address1_country = address1_country;
_x.donotemail = donotemail;
_x.address1_composite = address1_composite;
_x._slainvokedid_value = _slainvokedid_value;
_x.address1_primarycontactname = address1_primarycontactname;
_x.onholdtime = onholdtime;
_x._createdbyexternalparty_value = _createdbyexternalparty_value;
_x.marketingonly = marketingonly;
_x.statuscode = statuscode;
_x.traversedpath = traversedpath;
_x.processid = processid;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._modifiedbyexternalparty_value = _modifiedbyexternalparty_value;
_x.address2_postalcode = address2_postalcode;
_x._modifiedby_value = _modifiedby_value;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x._createdby_value = _createdby_value;
_x.entityimage_timestamp = entityimage_timestamp;
_x.address1_upszone = address1_upszone;
_x.address2_primarycontactname = address2_primarycontactname;
_x.primarytwitterid = primarytwitterid;
_x.customertypecode = customertypecode;
_x.address2_postofficebox = address2_postofficebox;
_x.statecode = statecode;
_x.address2_city = address2_city;
_x._primarycontactid_value = _primarycontactid_value;
_x.address1_freighttermscode = address1_freighttermscode;
_x.address1_longitude = address1_longitude;
_x.telephone2 = telephone2;
_x.address1_addresstypecode = address1_addresstypecode;
_x.donotbulkpostalmail = donotbulkpostalmail;
_x.lastusedincampaign = lastusedincampaign;
_x._preferredsystemuserid_value = _preferredsystemuserid_value;
_x.creditlimit_base = creditlimit_base;
_x.telephone3 = telephone3;
_x.address1_addressid = address1_addressid;
_x.preferredappointmentdaycode = preferredappointmentdaycode;
_x.websiteurl = websiteurl;
_x.accountclassificationcode = accountclassificationcode;
_x.address2_composite = address2_composite;
_x.name = name;
_x.address2_telephone1 = address2_telephone1;
_x.creditlimit = creditlimit;
_x.lastonholdtime = lastonholdtime;
_x.address2_line2 = address2_line2;
_x.creditonhold = creditonhold;
_x.entityimageid = entityimageid;
_x.accountnumber = accountnumber;
_x.followemail = followemail;
_x.modifiedon = modifiedon;
_x.address1_county = address1_county;
_x.marketcap_base = marketcap_base;
_x.address2_upszone = address2_upszone;
_x.address2_country = address2_country;
_x.address1_telephone2 = address1_telephone2;
_x.address2_freighttermscode = address2_freighttermscode;
_x.address1_utcoffset = address1_utcoffset;
_x.emailaddress2 = emailaddress2;
_x._parentaccountid_value = _parentaccountid_value;
_x.preferredcontactmethodcode = preferredcontactmethodcode;
_x.revenue_base = revenue_base;
_x.address2_telephone2 = address2_telephone2;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Account[");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("territorycode=");
b.append(this.territorycode);
b.append(", ");
b.append("accountid=");
b.append(this.accountid);
b.append(", ");
b.append("address1_telephone1=");
b.append(this.address1_telephone1);
b.append(", ");
b.append("address1_postofficebox=");
b.append(this.address1_postofficebox);
b.append(", ");
b.append("address1_line2=");
b.append(this.address1_line2);
b.append(", ");
b.append("emailaddress3=");
b.append(this.emailaddress3);
b.append(", ");
b.append("address1_telephone3=");
b.append(this.address1_telephone3);
b.append(", ");
b.append("address2_county=");
b.append(this.address2_county);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("numberofemployees=");
b.append(this.numberofemployees);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("timespentbymeonemailandmeetings=");
b.append(this.timespentbymeonemailandmeetings);
b.append(", ");
b.append("address2_longitude=");
b.append(this.address2_longitude);
b.append(", ");
b.append("ownershipcode=");
b.append(this.ownershipcode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("primarysatoriid=");
b.append(this.primarysatoriid);
b.append(", ");
b.append("_masterid_value=");
b.append(this._masterid_value);
b.append(", ");
b.append("address2_latitude=");
b.append(this.address2_latitude);
b.append(", ");
b.append("address2_fax=");
b.append(this.address2_fax);
b.append(", ");
b.append("_slaid_value=");
b.append(this._slaid_value);
b.append(", ");
b.append("aging60_base=");
b.append(this.aging60_base);
b.append(", ");
b.append("telephone1=");
b.append(this.telephone1);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("revenue=");
b.append(this.revenue);
b.append(", ");
b.append("industrycode=");
b.append(this.industrycode);
b.append(", ");
b.append("aging30_base=");
b.append(this.aging30_base);
b.append(", ");
b.append("emailaddress1=");
b.append(this.emailaddress1);
b.append(", ");
b.append("address1_latitude=");
b.append(this.address1_latitude);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("tickersymbol=");
b.append(this.tickersymbol);
b.append(", ");
b.append("address2_shippingmethodcode=");
b.append(this.address2_shippingmethodcode);
b.append(", ");
b.append("customersizecode=");
b.append(this.customersizecode);
b.append(", ");
b.append("address2_name=");
b.append(this.address2_name);
b.append(", ");
b.append("address1_stateorprovince=");
b.append(this.address1_stateorprovince);
b.append(", ");
b.append("address1_line3=");
b.append(this.address1_line3);
b.append(", ");
b.append("accountratingcode=");
b.append(this.accountratingcode);
b.append(", ");
b.append("address1_line1=");
b.append(this.address1_line1);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("paymenttermscode=");
b.append(this.paymenttermscode);
b.append(", ");
b.append("donotfax=");
b.append(this.donotfax);
b.append(", ");
b.append("aging90_base=");
b.append(this.aging90_base);
b.append(", ");
b.append("businesstypecode=");
b.append(this.businesstypecode);
b.append(", ");
b.append("address2_line3=");
b.append(this.address2_line3);
b.append(", ");
b.append("address2_utcoffset=");
b.append(this.address2_utcoffset);
b.append(", ");
b.append("donotphone=");
b.append(this.donotphone);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("ftpsiteurl=");
b.append(this.ftpsiteurl);
b.append(", ");
b.append("shippingmethodcode=");
b.append(this.shippingmethodcode);
b.append(", ");
b.append("stockexchange=");
b.append(this.stockexchange);
b.append(", ");
b.append("address2_telephone3=");
b.append(this.address2_telephone3);
b.append(", ");
b.append("address2_line1=");
b.append(this.address2_line1);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("aging60=");
b.append(this.aging60);
b.append(", ");
b.append("participatesinworkflow=");
b.append(this.participatesinworkflow);
b.append(", ");
b.append("address1_postalcode=");
b.append(this.address1_postalcode);
b.append(", ");
b.append("address2_addressid=");
b.append(this.address2_addressid);
b.append(", ");
b.append("marketcap=");
b.append(this.marketcap);
b.append(", ");
b.append("address2_addresstypecode=");
b.append(this.address2_addresstypecode);
b.append(", ");
b.append("aging90=");
b.append(this.aging90);
b.append(", ");
b.append("donotbulkemail=");
b.append(this.donotbulkemail);
b.append(", ");
b.append("aging30=");
b.append(this.aging30);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("sic=");
b.append(this.sic);
b.append(", ");
b.append("sharesoutstanding=");
b.append(this.sharesoutstanding);
b.append(", ");
b.append("accountcategorycode=");
b.append(this.accountcategorycode);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("preferredappointmenttimecode=");
b.append(this.preferredappointmenttimecode);
b.append(", ");
b.append("donotsendmm=");
b.append(this.donotsendmm);
b.append(", ");
b.append("address1_name=");
b.append(this.address1_name);
b.append(", ");
b.append("fax=");
b.append(this.fax);
b.append(", ");
b.append("address1_fax=");
b.append(this.address1_fax);
b.append(", ");
b.append("merged=");
b.append(this.merged);
b.append(", ");
b.append("address1_city=");
b.append(this.address1_city);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("address2_stateorprovince=");
b.append(this.address2_stateorprovince);
b.append(", ");
b.append("yominame=");
b.append(this.yominame);
b.append(", ");
b.append("donotpostalmail=");
b.append(this.donotpostalmail);
b.append(", ");
b.append("address1_country=");
b.append(this.address1_country);
b.append(", ");
b.append("donotemail=");
b.append(this.donotemail);
b.append(", ");
b.append("address1_composite=");
b.append(this.address1_composite);
b.append(", ");
b.append("_slainvokedid_value=");
b.append(this._slainvokedid_value);
b.append(", ");
b.append("address1_primarycontactname=");
b.append(this.address1_primarycontactname);
b.append(", ");
b.append("onholdtime=");
b.append(this.onholdtime);
b.append(", ");
b.append("_createdbyexternalparty_value=");
b.append(this._createdbyexternalparty_value);
b.append(", ");
b.append("marketingonly=");
b.append(this.marketingonly);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("_modifiedbyexternalparty_value=");
b.append(this._modifiedbyexternalparty_value);
b.append(", ");
b.append("address2_postalcode=");
b.append(this.address2_postalcode);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("address1_shippingmethodcode=");
b.append(this.address1_shippingmethodcode);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("address1_upszone=");
b.append(this.address1_upszone);
b.append(", ");
b.append("address2_primarycontactname=");
b.append(this.address2_primarycontactname);
b.append(", ");
b.append("primarytwitterid=");
b.append(this.primarytwitterid);
b.append(", ");
b.append("customertypecode=");
b.append(this.customertypecode);
b.append(", ");
b.append("address2_postofficebox=");
b.append(this.address2_postofficebox);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("address2_city=");
b.append(this.address2_city);
b.append(", ");
b.append("_primarycontactid_value=");
b.append(this._primarycontactid_value);
b.append(", ");
b.append("address1_freighttermscode=");
b.append(this.address1_freighttermscode);
b.append(", ");
b.append("address1_longitude=");
b.append(this.address1_longitude);
b.append(", ");
b.append("telephone2=");
b.append(this.telephone2);
b.append(", ");
b.append("address1_addresstypecode=");
b.append(this.address1_addresstypecode);
b.append(", ");
b.append("donotbulkpostalmail=");
b.append(this.donotbulkpostalmail);
b.append(", ");
b.append("lastusedincampaign=");
b.append(this.lastusedincampaign);
b.append(", ");
b.append("_preferredsystemuserid_value=");
b.append(this._preferredsystemuserid_value);
b.append(", ");
b.append("creditlimit_base=");
b.append(this.creditlimit_base);
b.append(", ");
b.append("telephone3=");
b.append(this.telephone3);
b.append(", ");
b.append("address1_addressid=");
b.append(this.address1_addressid);
b.append(", ");
b.append("preferredappointmentdaycode=");
b.append(this.preferredappointmentdaycode);
b.append(", ");
b.append("websiteurl=");
b.append(this.websiteurl);
b.append(", ");
b.append("accountclassificationcode=");
b.append(this.accountclassificationcode);
b.append(", ");
b.append("address2_composite=");
b.append(this.address2_composite);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("address2_telephone1=");
b.append(this.address2_telephone1);
b.append(", ");
b.append("creditlimit=");
b.append(this.creditlimit);
b.append(", ");
b.append("lastonholdtime=");
b.append(this.lastonholdtime);
b.append(", ");
b.append("address2_line2=");
b.append(this.address2_line2);
b.append(", ");
b.append("creditonhold=");
b.append(this.creditonhold);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("accountnumber=");
b.append(this.accountnumber);
b.append(", ");
b.append("followemail=");
b.append(this.followemail);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("address1_county=");
b.append(this.address1_county);
b.append(", ");
b.append("marketcap_base=");
b.append(this.marketcap_base);
b.append(", ");
b.append("address2_upszone=");
b.append(this.address2_upszone);
b.append(", ");
b.append("address2_country=");
b.append(this.address2_country);
b.append(", ");
b.append("address1_telephone2=");
b.append(this.address1_telephone2);
b.append(", ");
b.append("address2_freighttermscode=");
b.append(this.address2_freighttermscode);
b.append(", ");
b.append("address1_utcoffset=");
b.append(this.address1_utcoffset);
b.append(", ");
b.append("emailaddress2=");
b.append(this.emailaddress2);
b.append(", ");
b.append("_parentaccountid_value=");
b.append(this._parentaccountid_value);
b.append(", ");
b.append("preferredcontactmethodcode=");
b.append(this.preferredcontactmethodcode);
b.append(", ");
b.append("revenue_base=");
b.append(this.revenue_base);
b.append(", ");
b.append("address2_telephone2=");
b.append(this.address2_telephone2);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy