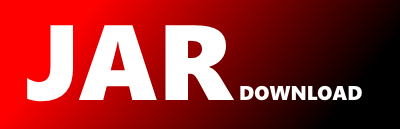
microsoft.dynamics.crm.entity.Activitypointer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.collection.request.ActivitymimeattachmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppointmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FaxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LetterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PhonecallCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurrenceruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurringappointmentmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialactivityCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TaskCollectionRequest;
import microsoft.dynamics.crm.entity.request.AccountRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.ContactRequest;
import microsoft.dynamics.crm.entity.request.InteractionforemailRequest;
import microsoft.dynamics.crm.entity.request.KnowledgearticleRequest;
import microsoft.dynamics.crm.entity.request.KnowledgebaserecordRequest;
import microsoft.dynamics.crm.entity.request.MailboxRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SlaRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"lastonholdtime",
"actualdurationminutes",
"_owningteam_value",
"exchangeitemid",
"ismapiprivate",
"createdon",
"seriesid",
"leftvoicemail",
"deliverylastattemptedon",
"isbilled",
"isworkflowcreated",
"_sendermailboxid_value",
"description",
"_regardingobjectid_value",
"onholdtime",
"_modifiedby_value",
"community",
"activityid",
"sortdate",
"instancetypecode",
"timezoneruleversionnumber",
"_createdonbehalfby_value",
"_transactioncurrencyid_value",
"versionnumber",
"processid",
"scheduledend",
"prioritycode",
"_slaid_value",
"stageid",
"actualstart",
"_owningbusinessunit_value",
"_owninguser_value",
"utcconversiontimezonecode",
"exchangeweblink",
"scheduleddurationminutes",
"senton",
"scheduledstart",
"statecode",
"subject",
"postponeactivityprocessinguntil",
"_modifiedonbehalfby_value",
"exchangerate",
"isregularactivity",
"deliveryprioritycode",
"activityadditionalparams",
"traversedpath",
"_createdby_value",
"activitytypecode",
"_ownerid_value",
"modifiedon",
"_slainvokedid_value",
"statuscode",
"actualend"})
@JsonInclude(Include.NON_NULL)
public class Activitypointer extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.activitypointer";
}
@JsonProperty("lastonholdtime")
protected OffsetDateTime lastonholdtime;
@JsonProperty("actualdurationminutes")
protected Integer actualdurationminutes;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("exchangeitemid")
protected String exchangeitemid;
@JsonProperty("ismapiprivate")
protected Boolean ismapiprivate;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("seriesid")
protected UUID seriesid;
@JsonProperty("leftvoicemail")
protected Boolean leftvoicemail;
@JsonProperty("deliverylastattemptedon")
protected OffsetDateTime deliverylastattemptedon;
@JsonProperty("isbilled")
protected Boolean isbilled;
@JsonProperty("isworkflowcreated")
protected Boolean isworkflowcreated;
@JsonProperty("_sendermailboxid_value")
protected UUID _sendermailboxid_value;
@JsonProperty("description")
protected String description;
@JsonProperty("_regardingobjectid_value")
protected UUID _regardingobjectid_value;
@JsonProperty("onholdtime")
protected Integer onholdtime;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("community")
protected Integer community;
@JsonProperty("activityid")
protected UUID activityid;
@JsonProperty("sortdate")
protected OffsetDateTime sortdate;
@JsonProperty("instancetypecode")
protected Integer instancetypecode;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("processid")
protected UUID processid;
@JsonProperty("scheduledend")
protected OffsetDateTime scheduledend;
@JsonProperty("prioritycode")
protected Integer prioritycode;
@JsonProperty("_slaid_value")
protected UUID _slaid_value;
@JsonProperty("stageid")
protected UUID stageid;
@JsonProperty("actualstart")
protected OffsetDateTime actualstart;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("exchangeweblink")
protected String exchangeweblink;
@JsonProperty("scheduleddurationminutes")
protected Integer scheduleddurationminutes;
@JsonProperty("senton")
protected OffsetDateTime senton;
@JsonProperty("scheduledstart")
protected OffsetDateTime scheduledstart;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("subject")
protected String subject;
@JsonProperty("postponeactivityprocessinguntil")
protected OffsetDateTime postponeactivityprocessinguntil;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("isregularactivity")
protected Boolean isregularactivity;
@JsonProperty("deliveryprioritycode")
protected Integer deliveryprioritycode;
@JsonProperty("activityadditionalparams")
protected String activityadditionalparams;
@JsonProperty("traversedpath")
protected String traversedpath;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("activitytypecode")
protected String activitytypecode;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("_slainvokedid_value")
protected UUID _slainvokedid_value;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("actualend")
protected OffsetDateTime actualend;
protected Activitypointer() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && activityid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(activityid, UUID.class));
}
}
@Property(name="lastonholdtime")
@JsonIgnore
public Optional getLastonholdtime() {
return Optional.ofNullable(lastonholdtime);
}
public Activitypointer withLastonholdtime(OffsetDateTime lastonholdtime) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("lastonholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.lastonholdtime = lastonholdtime;
return _x;
}
@Property(name="actualdurationminutes")
@JsonIgnore
public Optional getActualdurationminutes() {
return Optional.ofNullable(actualdurationminutes);
}
public Activitypointer withActualdurationminutes(Integer actualdurationminutes) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("actualdurationminutes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.actualdurationminutes = actualdurationminutes;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Activitypointer with_owningteam_value(UUID _owningteam_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="exchangeitemid")
@JsonIgnore
public Optional getExchangeitemid() {
return Optional.ofNullable(exchangeitemid);
}
public Activitypointer withExchangeitemid(String exchangeitemid) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("exchangeitemid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.exchangeitemid = exchangeitemid;
return _x;
}
@Property(name="ismapiprivate")
@JsonIgnore
public Optional getIsmapiprivate() {
return Optional.ofNullable(ismapiprivate);
}
public Activitypointer withIsmapiprivate(Boolean ismapiprivate) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("ismapiprivate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.ismapiprivate = ismapiprivate;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Activitypointer withCreatedon(OffsetDateTime createdon) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.createdon = createdon;
return _x;
}
@Property(name="seriesid")
@JsonIgnore
public Optional getSeriesid() {
return Optional.ofNullable(seriesid);
}
public Activitypointer withSeriesid(UUID seriesid) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("seriesid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.seriesid = seriesid;
return _x;
}
@Property(name="leftvoicemail")
@JsonIgnore
public Optional getLeftvoicemail() {
return Optional.ofNullable(leftvoicemail);
}
public Activitypointer withLeftvoicemail(Boolean leftvoicemail) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("leftvoicemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.leftvoicemail = leftvoicemail;
return _x;
}
@Property(name="deliverylastattemptedon")
@JsonIgnore
public Optional getDeliverylastattemptedon() {
return Optional.ofNullable(deliverylastattemptedon);
}
public Activitypointer withDeliverylastattemptedon(OffsetDateTime deliverylastattemptedon) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("deliverylastattemptedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.deliverylastattemptedon = deliverylastattemptedon;
return _x;
}
@Property(name="isbilled")
@JsonIgnore
public Optional getIsbilled() {
return Optional.ofNullable(isbilled);
}
public Activitypointer withIsbilled(Boolean isbilled) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("isbilled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.isbilled = isbilled;
return _x;
}
@Property(name="isworkflowcreated")
@JsonIgnore
public Optional getIsworkflowcreated() {
return Optional.ofNullable(isworkflowcreated);
}
public Activitypointer withIsworkflowcreated(Boolean isworkflowcreated) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("isworkflowcreated");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.isworkflowcreated = isworkflowcreated;
return _x;
}
@Property(name="_sendermailboxid_value")
@JsonIgnore
public Optional get_sendermailboxid_value() {
return Optional.ofNullable(_sendermailboxid_value);
}
public Activitypointer with_sendermailboxid_value(UUID _sendermailboxid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_sendermailboxid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._sendermailboxid_value = _sendermailboxid_value;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Activitypointer withDescription(String description) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.description = description;
return _x;
}
@Property(name="_regardingobjectid_value")
@JsonIgnore
public Optional get_regardingobjectid_value() {
return Optional.ofNullable(_regardingobjectid_value);
}
public Activitypointer with_regardingobjectid_value(UUID _regardingobjectid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_regardingobjectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._regardingobjectid_value = _regardingobjectid_value;
return _x;
}
@Property(name="onholdtime")
@JsonIgnore
public Optional getOnholdtime() {
return Optional.ofNullable(onholdtime);
}
public Activitypointer withOnholdtime(Integer onholdtime) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("onholdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.onholdtime = onholdtime;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Activitypointer with_modifiedby_value(UUID _modifiedby_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="community")
@JsonIgnore
public Optional getCommunity() {
return Optional.ofNullable(community);
}
public Activitypointer withCommunity(Integer community) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("community");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.community = community;
return _x;
}
@Property(name="activityid")
@JsonIgnore
public Optional getActivityid() {
return Optional.ofNullable(activityid);
}
public Activitypointer withActivityid(UUID activityid) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("activityid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.activityid = activityid;
return _x;
}
@Property(name="sortdate")
@JsonIgnore
public Optional getSortdate() {
return Optional.ofNullable(sortdate);
}
public Activitypointer withSortdate(OffsetDateTime sortdate) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("sortdate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.sortdate = sortdate;
return _x;
}
@Property(name="instancetypecode")
@JsonIgnore
public Optional getInstancetypecode() {
return Optional.ofNullable(instancetypecode);
}
public Activitypointer withInstancetypecode(Integer instancetypecode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("instancetypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.instancetypecode = instancetypecode;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Activitypointer withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Activitypointer with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Activitypointer with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Activitypointer withVersionnumber(Long versionnumber) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="processid")
@JsonIgnore
public Optional getProcessid() {
return Optional.ofNullable(processid);
}
public Activitypointer withProcessid(UUID processid) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("processid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.processid = processid;
return _x;
}
@Property(name="scheduledend")
@JsonIgnore
public Optional getScheduledend() {
return Optional.ofNullable(scheduledend);
}
public Activitypointer withScheduledend(OffsetDateTime scheduledend) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("scheduledend");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.scheduledend = scheduledend;
return _x;
}
@Property(name="prioritycode")
@JsonIgnore
public Optional getPrioritycode() {
return Optional.ofNullable(prioritycode);
}
public Activitypointer withPrioritycode(Integer prioritycode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("prioritycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.prioritycode = prioritycode;
return _x;
}
@Property(name="_slaid_value")
@JsonIgnore
public Optional get_slaid_value() {
return Optional.ofNullable(_slaid_value);
}
public Activitypointer with_slaid_value(UUID _slaid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_slaid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._slaid_value = _slaid_value;
return _x;
}
@Property(name="stageid")
@JsonIgnore
public Optional getStageid() {
return Optional.ofNullable(stageid);
}
public Activitypointer withStageid(UUID stageid) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("stageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.stageid = stageid;
return _x;
}
@Property(name="actualstart")
@JsonIgnore
public Optional getActualstart() {
return Optional.ofNullable(actualstart);
}
public Activitypointer withActualstart(OffsetDateTime actualstart) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("actualstart");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.actualstart = actualstart;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Activitypointer with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Activitypointer with_owninguser_value(UUID _owninguser_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Activitypointer withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="exchangeweblink")
@JsonIgnore
public Optional getExchangeweblink() {
return Optional.ofNullable(exchangeweblink);
}
public Activitypointer withExchangeweblink(String exchangeweblink) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("exchangeweblink");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.exchangeweblink = exchangeweblink;
return _x;
}
@Property(name="scheduleddurationminutes")
@JsonIgnore
public Optional getScheduleddurationminutes() {
return Optional.ofNullable(scheduleddurationminutes);
}
public Activitypointer withScheduleddurationminutes(Integer scheduleddurationminutes) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("scheduleddurationminutes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.scheduleddurationminutes = scheduleddurationminutes;
return _x;
}
@Property(name="senton")
@JsonIgnore
public Optional getSenton() {
return Optional.ofNullable(senton);
}
public Activitypointer withSenton(OffsetDateTime senton) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("senton");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.senton = senton;
return _x;
}
@Property(name="scheduledstart")
@JsonIgnore
public Optional getScheduledstart() {
return Optional.ofNullable(scheduledstart);
}
public Activitypointer withScheduledstart(OffsetDateTime scheduledstart) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("scheduledstart");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.scheduledstart = scheduledstart;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Activitypointer withStatecode(Integer statecode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.statecode = statecode;
return _x;
}
@Property(name="subject")
@JsonIgnore
public Optional getSubject() {
return Optional.ofNullable(subject);
}
public Activitypointer withSubject(String subject) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("subject");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.subject = subject;
return _x;
}
@Property(name="postponeactivityprocessinguntil")
@JsonIgnore
public Optional getPostponeactivityprocessinguntil() {
return Optional.ofNullable(postponeactivityprocessinguntil);
}
public Activitypointer withPostponeactivityprocessinguntil(OffsetDateTime postponeactivityprocessinguntil) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("postponeactivityprocessinguntil");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Activitypointer with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Activitypointer withExchangerate(BigDecimal exchangerate) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="isregularactivity")
@JsonIgnore
public Optional getIsregularactivity() {
return Optional.ofNullable(isregularactivity);
}
public Activitypointer withIsregularactivity(Boolean isregularactivity) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("isregularactivity");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.isregularactivity = isregularactivity;
return _x;
}
@Property(name="deliveryprioritycode")
@JsonIgnore
public Optional getDeliveryprioritycode() {
return Optional.ofNullable(deliveryprioritycode);
}
public Activitypointer withDeliveryprioritycode(Integer deliveryprioritycode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("deliveryprioritycode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.deliveryprioritycode = deliveryprioritycode;
return _x;
}
@Property(name="activityadditionalparams")
@JsonIgnore
public Optional getActivityadditionalparams() {
return Optional.ofNullable(activityadditionalparams);
}
public Activitypointer withActivityadditionalparams(String activityadditionalparams) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("activityadditionalparams");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.activityadditionalparams = activityadditionalparams;
return _x;
}
@Property(name="traversedpath")
@JsonIgnore
public Optional getTraversedpath() {
return Optional.ofNullable(traversedpath);
}
public Activitypointer withTraversedpath(String traversedpath) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("traversedpath");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.traversedpath = traversedpath;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Activitypointer with_createdby_value(UUID _createdby_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="activitytypecode")
@JsonIgnore
public Optional getActivitytypecode() {
return Optional.ofNullable(activitytypecode);
}
public Activitypointer withActivitytypecode(String activitytypecode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("activitytypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.activitytypecode = activitytypecode;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Activitypointer with_ownerid_value(UUID _ownerid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Activitypointer withModifiedon(OffsetDateTime modifiedon) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="_slainvokedid_value")
@JsonIgnore
public Optional get_slainvokedid_value() {
return Optional.ofNullable(_slainvokedid_value);
}
public Activitypointer with_slainvokedid_value(UUID _slainvokedid_value) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("_slainvokedid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x._slainvokedid_value = _slainvokedid_value;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Activitypointer withStatuscode(Integer statuscode) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.statuscode = statuscode;
return _x;
}
@Property(name="actualend")
@JsonIgnore
public Optional getActualend() {
return Optional.ofNullable(actualend);
}
public Activitypointer withActualend(OffsetDateTime actualend) {
Activitypointer _x = _copy();
_x.changedFields = changedFields.add("actualend");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.activitypointer");
_x.actualend = actualend;
return _x;
}
public Activitypointer withUnmappedField(String name, Object value) {
Activitypointer _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="regardingobjectid_new_interactionforemail")
@JsonIgnore
public InteractionforemailRequest getRegardingobjectid_new_interactionforemail() {
return new InteractionforemailRequest(contextPath.addSegment("regardingobjectid_new_interactionforemail"), RequestHelper.getValue(unmappedFields, "regardingobjectid_new_interactionforemail"));
}
@NavigationProperty(name="regardingobjectid_knowledgebaserecord")
@JsonIgnore
public KnowledgebaserecordRequest getRegardingobjectid_knowledgebaserecord() {
return new KnowledgebaserecordRequest(contextPath.addSegment("regardingobjectid_knowledgebaserecord"), RequestHelper.getValue(unmappedFields, "regardingobjectid_knowledgebaserecord"));
}
@NavigationProperty(name="regardingobjectid_account")
@JsonIgnore
public AccountRequest getRegardingobjectid_account() {
return new AccountRequest(contextPath.addSegment("regardingobjectid_account"), RequestHelper.getValue(unmappedFields, "regardingobjectid_account"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="regardingobjectid_contact")
@JsonIgnore
public ContactRequest getRegardingobjectid_contact() {
return new ContactRequest(contextPath.addSegment("regardingobjectid_contact"), RequestHelper.getValue(unmappedFields, "regardingobjectid_contact"));
}
@NavigationProperty(name="activity_pointer_socialactivity")
@JsonIgnore
public SocialactivityCollectionRequest getActivity_pointer_socialactivity() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("activity_pointer_socialactivity"), RequestHelper.getValue(unmappedFields, "activity_pointer_socialactivity"));
}
@NavigationProperty(name="activity_pointer_recurringappointmentmaster")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getActivity_pointer_recurringappointmentmaster() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("activity_pointer_recurringappointmentmaster"), RequestHelper.getValue(unmappedFields, "activity_pointer_recurringappointmentmaster"));
}
@NavigationProperty(name="activity_pointer_email")
@JsonIgnore
public EmailCollectionRequest getActivity_pointer_email() {
return new EmailCollectionRequest(
contextPath.addSegment("activity_pointer_email"), RequestHelper.getValue(unmappedFields, "activity_pointer_email"));
}
@NavigationProperty(name="sendermailboxid")
@JsonIgnore
public MailboxRequest getSendermailboxid() {
return new MailboxRequest(contextPath.addSegment("sendermailboxid"), RequestHelper.getValue(unmappedFields, "sendermailboxid"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@NavigationProperty(name="ActivityPointer_QueueItem")
@JsonIgnore
public QueueitemCollectionRequest getActivityPointer_QueueItem() {
return new QueueitemCollectionRequest(
contextPath.addSegment("ActivityPointer_QueueItem"), RequestHelper.getValue(unmappedFields, "ActivityPointer_QueueItem"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"), RequestHelper.getValue(unmappedFields, "owninguser"));
}
@NavigationProperty(name="sla_activitypointer_sla")
@JsonIgnore
public SlaRequest getSla_activitypointer_sla() {
return new SlaRequest(contextPath.addSegment("sla_activitypointer_sla"), RequestHelper.getValue(unmappedFields, "sla_activitypointer_sla"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="regardingobjectid_knowledgearticle")
@JsonIgnore
public KnowledgearticleRequest getRegardingobjectid_knowledgearticle() {
return new KnowledgearticleRequest(contextPath.addSegment("regardingobjectid_knowledgearticle"), RequestHelper.getValue(unmappedFields, "regardingobjectid_knowledgearticle"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="activity_pointer_activity_mime_attachment")
@JsonIgnore
public ActivitymimeattachmentCollectionRequest getActivity_pointer_activity_mime_attachment() {
return new ActivitymimeattachmentCollectionRequest(
contextPath.addSegment("activity_pointer_activity_mime_attachment"), RequestHelper.getValue(unmappedFields, "activity_pointer_activity_mime_attachment"));
}
@NavigationProperty(name="activity_pointer_fax")
@JsonIgnore
public FaxCollectionRequest getActivity_pointer_fax() {
return new FaxCollectionRequest(
contextPath.addSegment("activity_pointer_fax"), RequestHelper.getValue(unmappedFields, "activity_pointer_fax"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="activity_pointer_task")
@JsonIgnore
public TaskCollectionRequest getActivity_pointer_task() {
return new TaskCollectionRequest(
contextPath.addSegment("activity_pointer_task"), RequestHelper.getValue(unmappedFields, "activity_pointer_task"));
}
@NavigationProperty(name="activity_pointer_phonecall")
@JsonIgnore
public PhonecallCollectionRequest getActivity_pointer_phonecall() {
return new PhonecallCollectionRequest(
contextPath.addSegment("activity_pointer_phonecall"), RequestHelper.getValue(unmappedFields, "activity_pointer_phonecall"));
}
@NavigationProperty(name="activity_pointer_appointment")
@JsonIgnore
public AppointmentCollectionRequest getActivity_pointer_appointment() {
return new AppointmentCollectionRequest(
contextPath.addSegment("activity_pointer_appointment"), RequestHelper.getValue(unmappedFields, "activity_pointer_appointment"));
}
@NavigationProperty(name="activity_pointer_letter")
@JsonIgnore
public LetterCollectionRequest getActivity_pointer_letter() {
return new LetterCollectionRequest(
contextPath.addSegment("activity_pointer_letter"), RequestHelper.getValue(unmappedFields, "activity_pointer_letter"));
}
@NavigationProperty(name="activitypointer_connections2")
@JsonIgnore
public ConnectionCollectionRequest getActivitypointer_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("activitypointer_connections2"), RequestHelper.getValue(unmappedFields, "activitypointer_connections2"));
}
@NavigationProperty(name="slakpiinstance_activitypointer")
@JsonIgnore
public SlakpiinstanceCollectionRequest getSlakpiinstance_activitypointer() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("slakpiinstance_activitypointer"), RequestHelper.getValue(unmappedFields, "slakpiinstance_activitypointer"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="ActivityPointer_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getActivityPointer_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("ActivityPointer_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "ActivityPointer_BulkDeleteFailures"));
}
@NavigationProperty(name="slainvokedid_activitypointer_sla")
@JsonIgnore
public SlaRequest getSlainvokedid_activitypointer_sla() {
return new SlaRequest(contextPath.addSegment("slainvokedid_activitypointer_sla"), RequestHelper.getValue(unmappedFields, "slainvokedid_activitypointer_sla"));
}
@NavigationProperty(name="activitypointer_connections1")
@JsonIgnore
public ConnectionCollectionRequest getActivitypointer_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("activitypointer_connections1"), RequestHelper.getValue(unmappedFields, "activitypointer_connections1"));
}
@NavigationProperty(name="ActivityPointer_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getActivityPointer_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("ActivityPointer_AsyncOperations"), RequestHelper.getValue(unmappedFields, "ActivityPointer_AsyncOperations"));
}
@NavigationProperty(name="activity_pointer_recurrencerule")
@JsonIgnore
public RecurrenceruleCollectionRequest getActivity_pointer_recurrencerule() {
return new RecurrenceruleCollectionRequest(
contextPath.addSegment("activity_pointer_recurrencerule"), RequestHelper.getValue(unmappedFields, "activity_pointer_recurrencerule"));
}
@NavigationProperty(name="activitypointer_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getActivitypointer_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("activitypointer_activity_parties"), RequestHelper.getValue(unmappedFields, "activitypointer_activity_parties"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Activitypointer patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Activitypointer _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Activitypointer put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Activitypointer _x = _copy();
_x.changedFields = null;
return _x;
}
private Activitypointer _copy() {
Activitypointer _x = new Activitypointer();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.lastonholdtime = lastonholdtime;
_x.actualdurationminutes = actualdurationminutes;
_x._owningteam_value = _owningteam_value;
_x.exchangeitemid = exchangeitemid;
_x.ismapiprivate = ismapiprivate;
_x.createdon = createdon;
_x.seriesid = seriesid;
_x.leftvoicemail = leftvoicemail;
_x.deliverylastattemptedon = deliverylastattemptedon;
_x.isbilled = isbilled;
_x.isworkflowcreated = isworkflowcreated;
_x._sendermailboxid_value = _sendermailboxid_value;
_x.description = description;
_x._regardingobjectid_value = _regardingobjectid_value;
_x.onholdtime = onholdtime;
_x._modifiedby_value = _modifiedby_value;
_x.community = community;
_x.activityid = activityid;
_x.sortdate = sortdate;
_x.instancetypecode = instancetypecode;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.versionnumber = versionnumber;
_x.processid = processid;
_x.scheduledend = scheduledend;
_x.prioritycode = prioritycode;
_x._slaid_value = _slaid_value;
_x.stageid = stageid;
_x.actualstart = actualstart;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._owninguser_value = _owninguser_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.exchangeweblink = exchangeweblink;
_x.scheduleddurationminutes = scheduleddurationminutes;
_x.senton = senton;
_x.scheduledstart = scheduledstart;
_x.statecode = statecode;
_x.subject = subject;
_x.postponeactivityprocessinguntil = postponeactivityprocessinguntil;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.isregularactivity = isregularactivity;
_x.deliveryprioritycode = deliveryprioritycode;
_x.activityadditionalparams = activityadditionalparams;
_x.traversedpath = traversedpath;
_x._createdby_value = _createdby_value;
_x.activitytypecode = activitytypecode;
_x._ownerid_value = _ownerid_value;
_x.modifiedon = modifiedon;
_x._slainvokedid_value = _slainvokedid_value;
_x.statuscode = statuscode;
_x.actualend = actualend;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Activitypointer[");
b.append("lastonholdtime=");
b.append(this.lastonholdtime);
b.append(", ");
b.append("actualdurationminutes=");
b.append(this.actualdurationminutes);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("exchangeitemid=");
b.append(this.exchangeitemid);
b.append(", ");
b.append("ismapiprivate=");
b.append(this.ismapiprivate);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("seriesid=");
b.append(this.seriesid);
b.append(", ");
b.append("leftvoicemail=");
b.append(this.leftvoicemail);
b.append(", ");
b.append("deliverylastattemptedon=");
b.append(this.deliverylastattemptedon);
b.append(", ");
b.append("isbilled=");
b.append(this.isbilled);
b.append(", ");
b.append("isworkflowcreated=");
b.append(this.isworkflowcreated);
b.append(", ");
b.append("_sendermailboxid_value=");
b.append(this._sendermailboxid_value);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("_regardingobjectid_value=");
b.append(this._regardingobjectid_value);
b.append(", ");
b.append("onholdtime=");
b.append(this.onholdtime);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("community=");
b.append(this.community);
b.append(", ");
b.append("activityid=");
b.append(this.activityid);
b.append(", ");
b.append("sortdate=");
b.append(this.sortdate);
b.append(", ");
b.append("instancetypecode=");
b.append(this.instancetypecode);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("scheduledend=");
b.append(this.scheduledend);
b.append(", ");
b.append("prioritycode=");
b.append(this.prioritycode);
b.append(", ");
b.append("_slaid_value=");
b.append(this._slaid_value);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("actualstart=");
b.append(this.actualstart);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("exchangeweblink=");
b.append(this.exchangeweblink);
b.append(", ");
b.append("scheduleddurationminutes=");
b.append(this.scheduleddurationminutes);
b.append(", ");
b.append("senton=");
b.append(this.senton);
b.append(", ");
b.append("scheduledstart=");
b.append(this.scheduledstart);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("subject=");
b.append(this.subject);
b.append(", ");
b.append("postponeactivityprocessinguntil=");
b.append(this.postponeactivityprocessinguntil);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("isregularactivity=");
b.append(this.isregularactivity);
b.append(", ");
b.append("deliveryprioritycode=");
b.append(this.deliveryprioritycode);
b.append(", ");
b.append("activityadditionalparams=");
b.append(this.activityadditionalparams);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("activitytypecode=");
b.append(this.activitytypecode);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("_slainvokedid_value=");
b.append(this._slainvokedid_value);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("actualend=");
b.append(this.actualend);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy