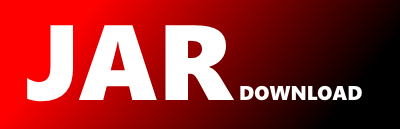
microsoft.dynamics.crm.entity.Emailserverprofile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OrganizationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TracelogCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
@JsonPropertyOrder({
"@odata.type",
"exchangeonlinetenantid",
"oauthclientsecret",
"outgoingserverlocation",
"timeoutmailboxconnection",
"_incomingpartnerapplication_value",
"timeoutmailboxconnectionafteramount",
"name",
"incominguseimpersonation",
"_createdonbehalfby_value",
"usedefaulttenantid",
"isincomingpasswordset",
"oauthclientid",
"incomingpassword",
"statuscode",
"outgoingusername",
"_owningbusinessunit_value",
"utcconversiontimezonecode",
"lastcrmmessage",
"outgoingpassword",
"incomingportnumber",
"encodingcodepage",
"isoauthclientsecretset",
"sendemailalert",
"emailservertypename",
"processemailsreceivedafter",
"lastauthorizationstatus",
"entityimage",
"_ownerid_value",
"lasttesttotalexecutiontime",
"useautodiscover",
"_outgoingpartnerapplication_value",
"outgoingauthenticationprotocol",
"incomingusessl",
"createdon",
"outgoingautograntdelegateaccess",
"incomingcredentialretrieval",
"incomingauthenticationprotocol",
"maxconcurrentconnections",
"owneremailaddress",
"outgoingportnumber",
"outgoingusessl",
"_modifiedby_value",
"outgoingcredentialretrieval",
"lasttestrequest",
"lasttestresponse",
"versionnumber",
"usesamesettingsforoutgoingconnections",
"exchangeversion",
"emailserverprofileid",
"_organizationid_value",
"minpollingintervalinminutes",
"entityimage_timestamp",
"timezoneruleversionnumber",
"incomingusername",
"description",
"entityimage_url",
"isoutgoingpasswordset",
"incomingserverlocation",
"lasttestvalidationstatus",
"lasttestexecutionstatus",
"entityimageid",
"moveundeliveredemails",
"defaultserverlocation",
"outgoinguseimpersonation",
"modifiedon",
"_owningteam_value",
"statecode",
"_owninguser_value",
"lastteststarttime",
"_modifiedonbehalfby_value",
"_createdby_value",
"servertype"})
@JsonInclude(Include.NON_NULL)
public class Emailserverprofile extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.emailserverprofile";
}
@JsonProperty("exchangeonlinetenantid")
protected String exchangeonlinetenantid;
@JsonProperty("oauthclientsecret")
protected String oauthclientsecret;
@JsonProperty("outgoingserverlocation")
protected String outgoingserverlocation;
@JsonProperty("timeoutmailboxconnection")
protected Boolean timeoutmailboxconnection;
@JsonProperty("_incomingpartnerapplication_value")
protected UUID _incomingpartnerapplication_value;
@JsonProperty("timeoutmailboxconnectionafteramount")
protected Integer timeoutmailboxconnectionafteramount;
@JsonProperty("name")
protected String name;
@JsonProperty("incominguseimpersonation")
protected Boolean incominguseimpersonation;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("usedefaulttenantid")
protected Boolean usedefaulttenantid;
@JsonProperty("isincomingpasswordset")
protected Boolean isincomingpasswordset;
@JsonProperty("oauthclientid")
protected String oauthclientid;
@JsonProperty("incomingpassword")
protected String incomingpassword;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("outgoingusername")
protected String outgoingusername;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("lastcrmmessage")
protected String lastcrmmessage;
@JsonProperty("outgoingpassword")
protected String outgoingpassword;
@JsonProperty("incomingportnumber")
protected Integer incomingportnumber;
@JsonProperty("encodingcodepage")
protected String encodingcodepage;
@JsonProperty("isoauthclientsecretset")
protected Boolean isoauthclientsecretset;
@JsonProperty("sendemailalert")
protected Boolean sendemailalert;
@JsonProperty("emailservertypename")
protected String emailservertypename;
@JsonProperty("processemailsreceivedafter")
protected OffsetDateTime processemailsreceivedafter;
@JsonProperty("lastauthorizationstatus")
protected Integer lastauthorizationstatus;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("lasttesttotalexecutiontime")
protected Long lasttesttotalexecutiontime;
@JsonProperty("useautodiscover")
protected Boolean useautodiscover;
@JsonProperty("_outgoingpartnerapplication_value")
protected UUID _outgoingpartnerapplication_value;
@JsonProperty("outgoingauthenticationprotocol")
protected Integer outgoingauthenticationprotocol;
@JsonProperty("incomingusessl")
protected Boolean incomingusessl;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("outgoingautograntdelegateaccess")
protected Boolean outgoingautograntdelegateaccess;
@JsonProperty("incomingcredentialretrieval")
protected Integer incomingcredentialretrieval;
@JsonProperty("incomingauthenticationprotocol")
protected Integer incomingauthenticationprotocol;
@JsonProperty("maxconcurrentconnections")
protected Integer maxconcurrentconnections;
@JsonProperty("owneremailaddress")
protected String owneremailaddress;
@JsonProperty("outgoingportnumber")
protected Integer outgoingportnumber;
@JsonProperty("outgoingusessl")
protected Boolean outgoingusessl;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("outgoingcredentialretrieval")
protected Integer outgoingcredentialretrieval;
@JsonProperty("lasttestrequest")
protected String lasttestrequest;
@JsonProperty("lasttestresponse")
protected String lasttestresponse;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("usesamesettingsforoutgoingconnections")
protected Boolean usesamesettingsforoutgoingconnections;
@JsonProperty("exchangeversion")
protected Integer exchangeversion;
@JsonProperty("emailserverprofileid")
protected UUID emailserverprofileid;
@JsonProperty("_organizationid_value")
protected UUID _organizationid_value;
@JsonProperty("minpollingintervalinminutes")
protected Integer minpollingintervalinminutes;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("incomingusername")
protected String incomingusername;
@JsonProperty("description")
protected String description;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("isoutgoingpasswordset")
protected Boolean isoutgoingpasswordset;
@JsonProperty("incomingserverlocation")
protected String incomingserverlocation;
@JsonProperty("lasttestvalidationstatus")
protected Integer lasttestvalidationstatus;
@JsonProperty("lasttestexecutionstatus")
protected Integer lasttestexecutionstatus;
@JsonProperty("entityimageid")
protected UUID entityimageid;
@JsonProperty("moveundeliveredemails")
protected Boolean moveundeliveredemails;
@JsonProperty("defaultserverlocation")
protected String defaultserverlocation;
@JsonProperty("outgoinguseimpersonation")
protected Boolean outgoinguseimpersonation;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("lastteststarttime")
protected OffsetDateTime lastteststarttime;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("servertype")
protected Integer servertype;
protected Emailserverprofile() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEmailserverprofile() {
return new Builder();
}
public static final class Builder {
private String exchangeonlinetenantid;
private String oauthclientsecret;
private String outgoingserverlocation;
private Boolean timeoutmailboxconnection;
private UUID _incomingpartnerapplication_value;
private Integer timeoutmailboxconnectionafteramount;
private String name;
private Boolean incominguseimpersonation;
private UUID _createdonbehalfby_value;
private Boolean usedefaulttenantid;
private Boolean isincomingpasswordset;
private String oauthclientid;
private String incomingpassword;
private Integer statuscode;
private String outgoingusername;
private UUID _owningbusinessunit_value;
private Integer utcconversiontimezonecode;
private String lastcrmmessage;
private String outgoingpassword;
private Integer incomingportnumber;
private String encodingcodepage;
private Boolean isoauthclientsecretset;
private Boolean sendemailalert;
private String emailservertypename;
private OffsetDateTime processemailsreceivedafter;
private Integer lastauthorizationstatus;
private byte[] entityimage;
private UUID _ownerid_value;
private Long lasttesttotalexecutiontime;
private Boolean useautodiscover;
private UUID _outgoingpartnerapplication_value;
private Integer outgoingauthenticationprotocol;
private Boolean incomingusessl;
private OffsetDateTime createdon;
private Boolean outgoingautograntdelegateaccess;
private Integer incomingcredentialretrieval;
private Integer incomingauthenticationprotocol;
private Integer maxconcurrentconnections;
private String owneremailaddress;
private Integer outgoingportnumber;
private Boolean outgoingusessl;
private UUID _modifiedby_value;
private Integer outgoingcredentialretrieval;
private String lasttestrequest;
private String lasttestresponse;
private Long versionnumber;
private Boolean usesamesettingsforoutgoingconnections;
private Integer exchangeversion;
private UUID emailserverprofileid;
private UUID _organizationid_value;
private Integer minpollingintervalinminutes;
private Long entityimage_timestamp;
private Integer timezoneruleversionnumber;
private String incomingusername;
private String description;
private String entityimage_url;
private Boolean isoutgoingpasswordset;
private String incomingserverlocation;
private Integer lasttestvalidationstatus;
private Integer lasttestexecutionstatus;
private UUID entityimageid;
private Boolean moveundeliveredemails;
private String defaultserverlocation;
private Boolean outgoinguseimpersonation;
private OffsetDateTime modifiedon;
private UUID _owningteam_value;
private Integer statecode;
private UUID _owninguser_value;
private OffsetDateTime lastteststarttime;
private UUID _modifiedonbehalfby_value;
private UUID _createdby_value;
private Integer servertype;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder exchangeonlinetenantid(String exchangeonlinetenantid) {
this.exchangeonlinetenantid = exchangeonlinetenantid;
this.changedFields = changedFields.add("exchangeonlinetenantid");
return this;
}
public Builder oauthclientsecret(String oauthclientsecret) {
this.oauthclientsecret = oauthclientsecret;
this.changedFields = changedFields.add("oauthclientsecret");
return this;
}
public Builder outgoingserverlocation(String outgoingserverlocation) {
this.outgoingserverlocation = outgoingserverlocation;
this.changedFields = changedFields.add("outgoingserverlocation");
return this;
}
public Builder timeoutmailboxconnection(Boolean timeoutmailboxconnection) {
this.timeoutmailboxconnection = timeoutmailboxconnection;
this.changedFields = changedFields.add("timeoutmailboxconnection");
return this;
}
public Builder _incomingpartnerapplication_value(UUID _incomingpartnerapplication_value) {
this._incomingpartnerapplication_value = _incomingpartnerapplication_value;
this.changedFields = changedFields.add("_incomingpartnerapplication_value");
return this;
}
public Builder timeoutmailboxconnectionafteramount(Integer timeoutmailboxconnectionafteramount) {
this.timeoutmailboxconnectionafteramount = timeoutmailboxconnectionafteramount;
this.changedFields = changedFields.add("timeoutmailboxconnectionafteramount");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder incominguseimpersonation(Boolean incominguseimpersonation) {
this.incominguseimpersonation = incominguseimpersonation;
this.changedFields = changedFields.add("incominguseimpersonation");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder usedefaulttenantid(Boolean usedefaulttenantid) {
this.usedefaulttenantid = usedefaulttenantid;
this.changedFields = changedFields.add("usedefaulttenantid");
return this;
}
public Builder isincomingpasswordset(Boolean isincomingpasswordset) {
this.isincomingpasswordset = isincomingpasswordset;
this.changedFields = changedFields.add("isincomingpasswordset");
return this;
}
public Builder oauthclientid(String oauthclientid) {
this.oauthclientid = oauthclientid;
this.changedFields = changedFields.add("oauthclientid");
return this;
}
public Builder incomingpassword(String incomingpassword) {
this.incomingpassword = incomingpassword;
this.changedFields = changedFields.add("incomingpassword");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder outgoingusername(String outgoingusername) {
this.outgoingusername = outgoingusername;
this.changedFields = changedFields.add("outgoingusername");
return this;
}
public Builder _owningbusinessunit_value(UUID _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder lastcrmmessage(String lastcrmmessage) {
this.lastcrmmessage = lastcrmmessage;
this.changedFields = changedFields.add("lastcrmmessage");
return this;
}
public Builder outgoingpassword(String outgoingpassword) {
this.outgoingpassword = outgoingpassword;
this.changedFields = changedFields.add("outgoingpassword");
return this;
}
public Builder incomingportnumber(Integer incomingportnumber) {
this.incomingportnumber = incomingportnumber;
this.changedFields = changedFields.add("incomingportnumber");
return this;
}
public Builder encodingcodepage(String encodingcodepage) {
this.encodingcodepage = encodingcodepage;
this.changedFields = changedFields.add("encodingcodepage");
return this;
}
public Builder isoauthclientsecretset(Boolean isoauthclientsecretset) {
this.isoauthclientsecretset = isoauthclientsecretset;
this.changedFields = changedFields.add("isoauthclientsecretset");
return this;
}
public Builder sendemailalert(Boolean sendemailalert) {
this.sendemailalert = sendemailalert;
this.changedFields = changedFields.add("sendemailalert");
return this;
}
public Builder emailservertypename(String emailservertypename) {
this.emailservertypename = emailservertypename;
this.changedFields = changedFields.add("emailservertypename");
return this;
}
public Builder processemailsreceivedafter(OffsetDateTime processemailsreceivedafter) {
this.processemailsreceivedafter = processemailsreceivedafter;
this.changedFields = changedFields.add("processemailsreceivedafter");
return this;
}
public Builder lastauthorizationstatus(Integer lastauthorizationstatus) {
this.lastauthorizationstatus = lastauthorizationstatus;
this.changedFields = changedFields.add("lastauthorizationstatus");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder _ownerid_value(UUID _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder lasttesttotalexecutiontime(Long lasttesttotalexecutiontime) {
this.lasttesttotalexecutiontime = lasttesttotalexecutiontime;
this.changedFields = changedFields.add("lasttesttotalexecutiontime");
return this;
}
public Builder useautodiscover(Boolean useautodiscover) {
this.useautodiscover = useautodiscover;
this.changedFields = changedFields.add("useautodiscover");
return this;
}
public Builder _outgoingpartnerapplication_value(UUID _outgoingpartnerapplication_value) {
this._outgoingpartnerapplication_value = _outgoingpartnerapplication_value;
this.changedFields = changedFields.add("_outgoingpartnerapplication_value");
return this;
}
public Builder outgoingauthenticationprotocol(Integer outgoingauthenticationprotocol) {
this.outgoingauthenticationprotocol = outgoingauthenticationprotocol;
this.changedFields = changedFields.add("outgoingauthenticationprotocol");
return this;
}
public Builder incomingusessl(Boolean incomingusessl) {
this.incomingusessl = incomingusessl;
this.changedFields = changedFields.add("incomingusessl");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder outgoingautograntdelegateaccess(Boolean outgoingautograntdelegateaccess) {
this.outgoingautograntdelegateaccess = outgoingautograntdelegateaccess;
this.changedFields = changedFields.add("outgoingautograntdelegateaccess");
return this;
}
public Builder incomingcredentialretrieval(Integer incomingcredentialretrieval) {
this.incomingcredentialretrieval = incomingcredentialretrieval;
this.changedFields = changedFields.add("incomingcredentialretrieval");
return this;
}
public Builder incomingauthenticationprotocol(Integer incomingauthenticationprotocol) {
this.incomingauthenticationprotocol = incomingauthenticationprotocol;
this.changedFields = changedFields.add("incomingauthenticationprotocol");
return this;
}
public Builder maxconcurrentconnections(Integer maxconcurrentconnections) {
this.maxconcurrentconnections = maxconcurrentconnections;
this.changedFields = changedFields.add("maxconcurrentconnections");
return this;
}
public Builder owneremailaddress(String owneremailaddress) {
this.owneremailaddress = owneremailaddress;
this.changedFields = changedFields.add("owneremailaddress");
return this;
}
public Builder outgoingportnumber(Integer outgoingportnumber) {
this.outgoingportnumber = outgoingportnumber;
this.changedFields = changedFields.add("outgoingportnumber");
return this;
}
public Builder outgoingusessl(Boolean outgoingusessl) {
this.outgoingusessl = outgoingusessl;
this.changedFields = changedFields.add("outgoingusessl");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder outgoingcredentialretrieval(Integer outgoingcredentialretrieval) {
this.outgoingcredentialretrieval = outgoingcredentialretrieval;
this.changedFields = changedFields.add("outgoingcredentialretrieval");
return this;
}
public Builder lasttestrequest(String lasttestrequest) {
this.lasttestrequest = lasttestrequest;
this.changedFields = changedFields.add("lasttestrequest");
return this;
}
public Builder lasttestresponse(String lasttestresponse) {
this.lasttestresponse = lasttestresponse;
this.changedFields = changedFields.add("lasttestresponse");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder usesamesettingsforoutgoingconnections(Boolean usesamesettingsforoutgoingconnections) {
this.usesamesettingsforoutgoingconnections = usesamesettingsforoutgoingconnections;
this.changedFields = changedFields.add("usesamesettingsforoutgoingconnections");
return this;
}
public Builder exchangeversion(Integer exchangeversion) {
this.exchangeversion = exchangeversion;
this.changedFields = changedFields.add("exchangeversion");
return this;
}
public Builder emailserverprofileid(UUID emailserverprofileid) {
this.emailserverprofileid = emailserverprofileid;
this.changedFields = changedFields.add("emailserverprofileid");
return this;
}
public Builder _organizationid_value(UUID _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder minpollingintervalinminutes(Integer minpollingintervalinminutes) {
this.minpollingintervalinminutes = minpollingintervalinminutes;
this.changedFields = changedFields.add("minpollingintervalinminutes");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder incomingusername(String incomingusername) {
this.incomingusername = incomingusername;
this.changedFields = changedFields.add("incomingusername");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder isoutgoingpasswordset(Boolean isoutgoingpasswordset) {
this.isoutgoingpasswordset = isoutgoingpasswordset;
this.changedFields = changedFields.add("isoutgoingpasswordset");
return this;
}
public Builder incomingserverlocation(String incomingserverlocation) {
this.incomingserverlocation = incomingserverlocation;
this.changedFields = changedFields.add("incomingserverlocation");
return this;
}
public Builder lasttestvalidationstatus(Integer lasttestvalidationstatus) {
this.lasttestvalidationstatus = lasttestvalidationstatus;
this.changedFields = changedFields.add("lasttestvalidationstatus");
return this;
}
public Builder lasttestexecutionstatus(Integer lasttestexecutionstatus) {
this.lasttestexecutionstatus = lasttestexecutionstatus;
this.changedFields = changedFields.add("lasttestexecutionstatus");
return this;
}
public Builder entityimageid(UUID entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder moveundeliveredemails(Boolean moveundeliveredemails) {
this.moveundeliveredemails = moveundeliveredemails;
this.changedFields = changedFields.add("moveundeliveredemails");
return this;
}
public Builder defaultserverlocation(String defaultserverlocation) {
this.defaultserverlocation = defaultserverlocation;
this.changedFields = changedFields.add("defaultserverlocation");
return this;
}
public Builder outgoinguseimpersonation(Boolean outgoinguseimpersonation) {
this.outgoinguseimpersonation = outgoinguseimpersonation;
this.changedFields = changedFields.add("outgoinguseimpersonation");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder _owningteam_value(UUID _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder _owninguser_value(UUID _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder lastteststarttime(OffsetDateTime lastteststarttime) {
this.lastteststarttime = lastteststarttime;
this.changedFields = changedFields.add("lastteststarttime");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder servertype(Integer servertype) {
this.servertype = servertype;
this.changedFields = changedFields.add("servertype");
return this;
}
public Emailserverprofile build() {
Emailserverprofile _x = new Emailserverprofile();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.emailserverprofile";
_x.exchangeonlinetenantid = exchangeonlinetenantid;
_x.oauthclientsecret = oauthclientsecret;
_x.outgoingserverlocation = outgoingserverlocation;
_x.timeoutmailboxconnection = timeoutmailboxconnection;
_x._incomingpartnerapplication_value = _incomingpartnerapplication_value;
_x.timeoutmailboxconnectionafteramount = timeoutmailboxconnectionafteramount;
_x.name = name;
_x.incominguseimpersonation = incominguseimpersonation;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.usedefaulttenantid = usedefaulttenantid;
_x.isincomingpasswordset = isincomingpasswordset;
_x.oauthclientid = oauthclientid;
_x.incomingpassword = incomingpassword;
_x.statuscode = statuscode;
_x.outgoingusername = outgoingusername;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.lastcrmmessage = lastcrmmessage;
_x.outgoingpassword = outgoingpassword;
_x.incomingportnumber = incomingportnumber;
_x.encodingcodepage = encodingcodepage;
_x.isoauthclientsecretset = isoauthclientsecretset;
_x.sendemailalert = sendemailalert;
_x.emailservertypename = emailservertypename;
_x.processemailsreceivedafter = processemailsreceivedafter;
_x.lastauthorizationstatus = lastauthorizationstatus;
_x.entityimage = entityimage;
_x._ownerid_value = _ownerid_value;
_x.lasttesttotalexecutiontime = lasttesttotalexecutiontime;
_x.useautodiscover = useautodiscover;
_x._outgoingpartnerapplication_value = _outgoingpartnerapplication_value;
_x.outgoingauthenticationprotocol = outgoingauthenticationprotocol;
_x.incomingusessl = incomingusessl;
_x.createdon = createdon;
_x.outgoingautograntdelegateaccess = outgoingautograntdelegateaccess;
_x.incomingcredentialretrieval = incomingcredentialretrieval;
_x.incomingauthenticationprotocol = incomingauthenticationprotocol;
_x.maxconcurrentconnections = maxconcurrentconnections;
_x.owneremailaddress = owneremailaddress;
_x.outgoingportnumber = outgoingportnumber;
_x.outgoingusessl = outgoingusessl;
_x._modifiedby_value = _modifiedby_value;
_x.outgoingcredentialretrieval = outgoingcredentialretrieval;
_x.lasttestrequest = lasttestrequest;
_x.lasttestresponse = lasttestresponse;
_x.versionnumber = versionnumber;
_x.usesamesettingsforoutgoingconnections = usesamesettingsforoutgoingconnections;
_x.exchangeversion = exchangeversion;
_x.emailserverprofileid = emailserverprofileid;
_x._organizationid_value = _organizationid_value;
_x.minpollingintervalinminutes = minpollingintervalinminutes;
_x.entityimage_timestamp = entityimage_timestamp;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.incomingusername = incomingusername;
_x.description = description;
_x.entityimage_url = entityimage_url;
_x.isoutgoingpasswordset = isoutgoingpasswordset;
_x.incomingserverlocation = incomingserverlocation;
_x.lasttestvalidationstatus = lasttestvalidationstatus;
_x.lasttestexecutionstatus = lasttestexecutionstatus;
_x.entityimageid = entityimageid;
_x.moveundeliveredemails = moveundeliveredemails;
_x.defaultserverlocation = defaultserverlocation;
_x.outgoinguseimpersonation = outgoinguseimpersonation;
_x.modifiedon = modifiedon;
_x._owningteam_value = _owningteam_value;
_x.statecode = statecode;
_x._owninguser_value = _owninguser_value;
_x.lastteststarttime = lastteststarttime;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x._createdby_value = _createdby_value;
_x.servertype = servertype;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && emailserverprofileid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(emailserverprofileid, UUID.class));
}
}
@Property(name="exchangeonlinetenantid")
@JsonIgnore
public Optional getExchangeonlinetenantid() {
return Optional.ofNullable(exchangeonlinetenantid);
}
public Emailserverprofile withExchangeonlinetenantid(String exchangeonlinetenantid) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("exchangeonlinetenantid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.exchangeonlinetenantid = exchangeonlinetenantid;
return _x;
}
@Property(name="oauthclientsecret")
@JsonIgnore
public Optional getOauthclientsecret() {
return Optional.ofNullable(oauthclientsecret);
}
public Emailserverprofile withOauthclientsecret(String oauthclientsecret) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("oauthclientsecret");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.oauthclientsecret = oauthclientsecret;
return _x;
}
@Property(name="outgoingserverlocation")
@JsonIgnore
public Optional getOutgoingserverlocation() {
return Optional.ofNullable(outgoingserverlocation);
}
public Emailserverprofile withOutgoingserverlocation(String outgoingserverlocation) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingserverlocation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingserverlocation = outgoingserverlocation;
return _x;
}
@Property(name="timeoutmailboxconnection")
@JsonIgnore
public Optional getTimeoutmailboxconnection() {
return Optional.ofNullable(timeoutmailboxconnection);
}
public Emailserverprofile withTimeoutmailboxconnection(Boolean timeoutmailboxconnection) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("timeoutmailboxconnection");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.timeoutmailboxconnection = timeoutmailboxconnection;
return _x;
}
@Property(name="_incomingpartnerapplication_value")
@JsonIgnore
public Optional get_incomingpartnerapplication_value() {
return Optional.ofNullable(_incomingpartnerapplication_value);
}
public Emailserverprofile with_incomingpartnerapplication_value(UUID _incomingpartnerapplication_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_incomingpartnerapplication_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._incomingpartnerapplication_value = _incomingpartnerapplication_value;
return _x;
}
@Property(name="timeoutmailboxconnectionafteramount")
@JsonIgnore
public Optional getTimeoutmailboxconnectionafteramount() {
return Optional.ofNullable(timeoutmailboxconnectionafteramount);
}
public Emailserverprofile withTimeoutmailboxconnectionafteramount(Integer timeoutmailboxconnectionafteramount) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("timeoutmailboxconnectionafteramount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.timeoutmailboxconnectionafteramount = timeoutmailboxconnectionafteramount;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Emailserverprofile withName(String name) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.name = name;
return _x;
}
@Property(name="incominguseimpersonation")
@JsonIgnore
public Optional getIncominguseimpersonation() {
return Optional.ofNullable(incominguseimpersonation);
}
public Emailserverprofile withIncominguseimpersonation(Boolean incominguseimpersonation) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incominguseimpersonation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incominguseimpersonation = incominguseimpersonation;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Emailserverprofile with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="usedefaulttenantid")
@JsonIgnore
public Optional getUsedefaulttenantid() {
return Optional.ofNullable(usedefaulttenantid);
}
public Emailserverprofile withUsedefaulttenantid(Boolean usedefaulttenantid) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("usedefaulttenantid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.usedefaulttenantid = usedefaulttenantid;
return _x;
}
@Property(name="isincomingpasswordset")
@JsonIgnore
public Optional getIsincomingpasswordset() {
return Optional.ofNullable(isincomingpasswordset);
}
public Emailserverprofile withIsincomingpasswordset(Boolean isincomingpasswordset) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("isincomingpasswordset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.isincomingpasswordset = isincomingpasswordset;
return _x;
}
@Property(name="oauthclientid")
@JsonIgnore
public Optional getOauthclientid() {
return Optional.ofNullable(oauthclientid);
}
public Emailserverprofile withOauthclientid(String oauthclientid) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("oauthclientid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.oauthclientid = oauthclientid;
return _x;
}
@Property(name="incomingpassword")
@JsonIgnore
public Optional getIncomingpassword() {
return Optional.ofNullable(incomingpassword);
}
public Emailserverprofile withIncomingpassword(String incomingpassword) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingpassword");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingpassword = incomingpassword;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Emailserverprofile withStatuscode(Integer statuscode) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.statuscode = statuscode;
return _x;
}
@Property(name="outgoingusername")
@JsonIgnore
public Optional getOutgoingusername() {
return Optional.ofNullable(outgoingusername);
}
public Emailserverprofile withOutgoingusername(String outgoingusername) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingusername");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingusername = outgoingusername;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Emailserverprofile with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Emailserverprofile withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="lastcrmmessage")
@JsonIgnore
public Optional getLastcrmmessage() {
return Optional.ofNullable(lastcrmmessage);
}
public Emailserverprofile withLastcrmmessage(String lastcrmmessage) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lastcrmmessage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lastcrmmessage = lastcrmmessage;
return _x;
}
@Property(name="outgoingpassword")
@JsonIgnore
public Optional getOutgoingpassword() {
return Optional.ofNullable(outgoingpassword);
}
public Emailserverprofile withOutgoingpassword(String outgoingpassword) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingpassword");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingpassword = outgoingpassword;
return _x;
}
@Property(name="incomingportnumber")
@JsonIgnore
public Optional getIncomingportnumber() {
return Optional.ofNullable(incomingportnumber);
}
public Emailserverprofile withIncomingportnumber(Integer incomingportnumber) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingportnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingportnumber = incomingportnumber;
return _x;
}
@Property(name="encodingcodepage")
@JsonIgnore
public Optional getEncodingcodepage() {
return Optional.ofNullable(encodingcodepage);
}
public Emailserverprofile withEncodingcodepage(String encodingcodepage) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("encodingcodepage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.encodingcodepage = encodingcodepage;
return _x;
}
@Property(name="isoauthclientsecretset")
@JsonIgnore
public Optional getIsoauthclientsecretset() {
return Optional.ofNullable(isoauthclientsecretset);
}
public Emailserverprofile withIsoauthclientsecretset(Boolean isoauthclientsecretset) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("isoauthclientsecretset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.isoauthclientsecretset = isoauthclientsecretset;
return _x;
}
@Property(name="sendemailalert")
@JsonIgnore
public Optional getSendemailalert() {
return Optional.ofNullable(sendemailalert);
}
public Emailserverprofile withSendemailalert(Boolean sendemailalert) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("sendemailalert");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.sendemailalert = sendemailalert;
return _x;
}
@Property(name="emailservertypename")
@JsonIgnore
public Optional getEmailservertypename() {
return Optional.ofNullable(emailservertypename);
}
public Emailserverprofile withEmailservertypename(String emailservertypename) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("emailservertypename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.emailservertypename = emailservertypename;
return _x;
}
@Property(name="processemailsreceivedafter")
@JsonIgnore
public Optional getProcessemailsreceivedafter() {
return Optional.ofNullable(processemailsreceivedafter);
}
public Emailserverprofile withProcessemailsreceivedafter(OffsetDateTime processemailsreceivedafter) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("processemailsreceivedafter");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.processemailsreceivedafter = processemailsreceivedafter;
return _x;
}
@Property(name="lastauthorizationstatus")
@JsonIgnore
public Optional getLastauthorizationstatus() {
return Optional.ofNullable(lastauthorizationstatus);
}
public Emailserverprofile withLastauthorizationstatus(Integer lastauthorizationstatus) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lastauthorizationstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lastauthorizationstatus = lastauthorizationstatus;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Emailserverprofile withEntityimage(byte[] entityimage) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.entityimage = entityimage;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Emailserverprofile with_ownerid_value(UUID _ownerid_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="lasttesttotalexecutiontime")
@JsonIgnore
public Optional getLasttesttotalexecutiontime() {
return Optional.ofNullable(lasttesttotalexecutiontime);
}
public Emailserverprofile withLasttesttotalexecutiontime(Long lasttesttotalexecutiontime) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lasttesttotalexecutiontime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lasttesttotalexecutiontime = lasttesttotalexecutiontime;
return _x;
}
@Property(name="useautodiscover")
@JsonIgnore
public Optional getUseautodiscover() {
return Optional.ofNullable(useautodiscover);
}
public Emailserverprofile withUseautodiscover(Boolean useautodiscover) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("useautodiscover");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.useautodiscover = useautodiscover;
return _x;
}
@Property(name="_outgoingpartnerapplication_value")
@JsonIgnore
public Optional get_outgoingpartnerapplication_value() {
return Optional.ofNullable(_outgoingpartnerapplication_value);
}
public Emailserverprofile with_outgoingpartnerapplication_value(UUID _outgoingpartnerapplication_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_outgoingpartnerapplication_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._outgoingpartnerapplication_value = _outgoingpartnerapplication_value;
return _x;
}
@Property(name="outgoingauthenticationprotocol")
@JsonIgnore
public Optional getOutgoingauthenticationprotocol() {
return Optional.ofNullable(outgoingauthenticationprotocol);
}
public Emailserverprofile withOutgoingauthenticationprotocol(Integer outgoingauthenticationprotocol) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingauthenticationprotocol");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingauthenticationprotocol = outgoingauthenticationprotocol;
return _x;
}
@Property(name="incomingusessl")
@JsonIgnore
public Optional getIncomingusessl() {
return Optional.ofNullable(incomingusessl);
}
public Emailserverprofile withIncomingusessl(Boolean incomingusessl) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingusessl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingusessl = incomingusessl;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Emailserverprofile withCreatedon(OffsetDateTime createdon) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.createdon = createdon;
return _x;
}
@Property(name="outgoingautograntdelegateaccess")
@JsonIgnore
public Optional getOutgoingautograntdelegateaccess() {
return Optional.ofNullable(outgoingautograntdelegateaccess);
}
public Emailserverprofile withOutgoingautograntdelegateaccess(Boolean outgoingautograntdelegateaccess) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingautograntdelegateaccess");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingautograntdelegateaccess = outgoingautograntdelegateaccess;
return _x;
}
@Property(name="incomingcredentialretrieval")
@JsonIgnore
public Optional getIncomingcredentialretrieval() {
return Optional.ofNullable(incomingcredentialretrieval);
}
public Emailserverprofile withIncomingcredentialretrieval(Integer incomingcredentialretrieval) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingcredentialretrieval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingcredentialretrieval = incomingcredentialretrieval;
return _x;
}
@Property(name="incomingauthenticationprotocol")
@JsonIgnore
public Optional getIncomingauthenticationprotocol() {
return Optional.ofNullable(incomingauthenticationprotocol);
}
public Emailserverprofile withIncomingauthenticationprotocol(Integer incomingauthenticationprotocol) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingauthenticationprotocol");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingauthenticationprotocol = incomingauthenticationprotocol;
return _x;
}
@Property(name="maxconcurrentconnections")
@JsonIgnore
public Optional getMaxconcurrentconnections() {
return Optional.ofNullable(maxconcurrentconnections);
}
public Emailserverprofile withMaxconcurrentconnections(Integer maxconcurrentconnections) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("maxconcurrentconnections");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.maxconcurrentconnections = maxconcurrentconnections;
return _x;
}
@Property(name="owneremailaddress")
@JsonIgnore
public Optional getOwneremailaddress() {
return Optional.ofNullable(owneremailaddress);
}
public Emailserverprofile withOwneremailaddress(String owneremailaddress) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("owneremailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.owneremailaddress = owneremailaddress;
return _x;
}
@Property(name="outgoingportnumber")
@JsonIgnore
public Optional getOutgoingportnumber() {
return Optional.ofNullable(outgoingportnumber);
}
public Emailserverprofile withOutgoingportnumber(Integer outgoingportnumber) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingportnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingportnumber = outgoingportnumber;
return _x;
}
@Property(name="outgoingusessl")
@JsonIgnore
public Optional getOutgoingusessl() {
return Optional.ofNullable(outgoingusessl);
}
public Emailserverprofile withOutgoingusessl(Boolean outgoingusessl) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingusessl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingusessl = outgoingusessl;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Emailserverprofile with_modifiedby_value(UUID _modifiedby_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="outgoingcredentialretrieval")
@JsonIgnore
public Optional getOutgoingcredentialretrieval() {
return Optional.ofNullable(outgoingcredentialretrieval);
}
public Emailserverprofile withOutgoingcredentialretrieval(Integer outgoingcredentialretrieval) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoingcredentialretrieval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoingcredentialretrieval = outgoingcredentialretrieval;
return _x;
}
@Property(name="lasttestrequest")
@JsonIgnore
public Optional getLasttestrequest() {
return Optional.ofNullable(lasttestrequest);
}
public Emailserverprofile withLasttestrequest(String lasttestrequest) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lasttestrequest");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lasttestrequest = lasttestrequest;
return _x;
}
@Property(name="lasttestresponse")
@JsonIgnore
public Optional getLasttestresponse() {
return Optional.ofNullable(lasttestresponse);
}
public Emailserverprofile withLasttestresponse(String lasttestresponse) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lasttestresponse");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lasttestresponse = lasttestresponse;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Emailserverprofile withVersionnumber(Long versionnumber) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="usesamesettingsforoutgoingconnections")
@JsonIgnore
public Optional getUsesamesettingsforoutgoingconnections() {
return Optional.ofNullable(usesamesettingsforoutgoingconnections);
}
public Emailserverprofile withUsesamesettingsforoutgoingconnections(Boolean usesamesettingsforoutgoingconnections) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("usesamesettingsforoutgoingconnections");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.usesamesettingsforoutgoingconnections = usesamesettingsforoutgoingconnections;
return _x;
}
@Property(name="exchangeversion")
@JsonIgnore
public Optional getExchangeversion() {
return Optional.ofNullable(exchangeversion);
}
public Emailserverprofile withExchangeversion(Integer exchangeversion) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("exchangeversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.exchangeversion = exchangeversion;
return _x;
}
@Property(name="emailserverprofileid")
@JsonIgnore
public Optional getEmailserverprofileid() {
return Optional.ofNullable(emailserverprofileid);
}
public Emailserverprofile withEmailserverprofileid(UUID emailserverprofileid) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("emailserverprofileid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.emailserverprofileid = emailserverprofileid;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Emailserverprofile with_organizationid_value(UUID _organizationid_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="minpollingintervalinminutes")
@JsonIgnore
public Optional getMinpollingintervalinminutes() {
return Optional.ofNullable(minpollingintervalinminutes);
}
public Emailserverprofile withMinpollingintervalinminutes(Integer minpollingintervalinminutes) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("minpollingintervalinminutes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.minpollingintervalinminutes = minpollingintervalinminutes;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Emailserverprofile withEntityimage_timestamp(Long entityimage_timestamp) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Emailserverprofile withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="incomingusername")
@JsonIgnore
public Optional getIncomingusername() {
return Optional.ofNullable(incomingusername);
}
public Emailserverprofile withIncomingusername(String incomingusername) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingusername");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingusername = incomingusername;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Emailserverprofile withDescription(String description) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.description = description;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Emailserverprofile withEntityimage_url(String entityimage_url) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="isoutgoingpasswordset")
@JsonIgnore
public Optional getIsoutgoingpasswordset() {
return Optional.ofNullable(isoutgoingpasswordset);
}
public Emailserverprofile withIsoutgoingpasswordset(Boolean isoutgoingpasswordset) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("isoutgoingpasswordset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.isoutgoingpasswordset = isoutgoingpasswordset;
return _x;
}
@Property(name="incomingserverlocation")
@JsonIgnore
public Optional getIncomingserverlocation() {
return Optional.ofNullable(incomingserverlocation);
}
public Emailserverprofile withIncomingserverlocation(String incomingserverlocation) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("incomingserverlocation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.incomingserverlocation = incomingserverlocation;
return _x;
}
@Property(name="lasttestvalidationstatus")
@JsonIgnore
public Optional getLasttestvalidationstatus() {
return Optional.ofNullable(lasttestvalidationstatus);
}
public Emailserverprofile withLasttestvalidationstatus(Integer lasttestvalidationstatus) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lasttestvalidationstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lasttestvalidationstatus = lasttestvalidationstatus;
return _x;
}
@Property(name="lasttestexecutionstatus")
@JsonIgnore
public Optional getLasttestexecutionstatus() {
return Optional.ofNullable(lasttestexecutionstatus);
}
public Emailserverprofile withLasttestexecutionstatus(Integer lasttestexecutionstatus) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lasttestexecutionstatus");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lasttestexecutionstatus = lasttestexecutionstatus;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Emailserverprofile withEntityimageid(UUID entityimageid) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="moveundeliveredemails")
@JsonIgnore
public Optional getMoveundeliveredemails() {
return Optional.ofNullable(moveundeliveredemails);
}
public Emailserverprofile withMoveundeliveredemails(Boolean moveundeliveredemails) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("moveundeliveredemails");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.moveundeliveredemails = moveundeliveredemails;
return _x;
}
@Property(name="defaultserverlocation")
@JsonIgnore
public Optional getDefaultserverlocation() {
return Optional.ofNullable(defaultserverlocation);
}
public Emailserverprofile withDefaultserverlocation(String defaultserverlocation) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("defaultserverlocation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.defaultserverlocation = defaultserverlocation;
return _x;
}
@Property(name="outgoinguseimpersonation")
@JsonIgnore
public Optional getOutgoinguseimpersonation() {
return Optional.ofNullable(outgoinguseimpersonation);
}
public Emailserverprofile withOutgoinguseimpersonation(Boolean outgoinguseimpersonation) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("outgoinguseimpersonation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.outgoinguseimpersonation = outgoinguseimpersonation;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Emailserverprofile withModifiedon(OffsetDateTime modifiedon) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Emailserverprofile with_owningteam_value(UUID _owningteam_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Emailserverprofile withStatecode(Integer statecode) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.statecode = statecode;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Emailserverprofile with_owninguser_value(UUID _owninguser_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="lastteststarttime")
@JsonIgnore
public Optional getLastteststarttime() {
return Optional.ofNullable(lastteststarttime);
}
public Emailserverprofile withLastteststarttime(OffsetDateTime lastteststarttime) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("lastteststarttime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.lastteststarttime = lastteststarttime;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Emailserverprofile with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Emailserverprofile with_createdby_value(UUID _createdby_value) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="servertype")
@JsonIgnore
public Optional getServertype() {
return Optional.ofNullable(servertype);
}
public Emailserverprofile withServertype(Integer servertype) {
Emailserverprofile _x = _copy();
_x.changedFields = changedFields.add("servertype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.emailserverprofile");
_x.servertype = servertype;
return _x;
}
public Emailserverprofile withUnmappedField(String name, Object value) {
Emailserverprofile _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="emailserverprofile_mailbox")
@JsonIgnore
public MailboxCollectionRequest getEmailserverprofile_mailbox() {
return new MailboxCollectionRequest(
contextPath.addSegment("emailserverprofile_mailbox"), RequestHelper.getValue(unmappedFields, "emailserverprofile_mailbox"));
}
@NavigationProperty(name="emailserverprofile_asyncoperations")
@JsonIgnore
public AsyncoperationCollectionRequest getEmailserverprofile_asyncoperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("emailserverprofile_asyncoperations"), RequestHelper.getValue(unmappedFields, "emailserverprofile_asyncoperations"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"), RequestHelper.getValue(unmappedFields, "organizationid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="emailserverprofile_bulkdeletefailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getEmailserverprofile_bulkdeletefailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("emailserverprofile_bulkdeletefailures"), RequestHelper.getValue(unmappedFields, "emailserverprofile_bulkdeletefailures"));
}
@NavigationProperty(name="EmailServerProfile_Organization")
@JsonIgnore
public OrganizationCollectionRequest getEmailServerProfile_Organization() {
return new OrganizationCollectionRequest(
contextPath.addSegment("EmailServerProfile_Organization"), RequestHelper.getValue(unmappedFields, "EmailServerProfile_Organization"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="tracelog_EmailServerProfile")
@JsonIgnore
public TracelogCollectionRequest getTracelog_EmailServerProfile() {
return new TracelogCollectionRequest(
contextPath.addSegment("tracelog_EmailServerProfile"), RequestHelper.getValue(unmappedFields, "tracelog_EmailServerProfile"));
}
@NavigationProperty(name="EmailServerProfile_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getEmailServerProfile_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("EmailServerProfile_Annotation"), RequestHelper.getValue(unmappedFields, "EmailServerProfile_Annotation"));
}
@NavigationProperty(name="emailserverprofile_duplicatematchingrecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getEmailserverprofile_duplicatematchingrecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("emailserverprofile_duplicatematchingrecord"), RequestHelper.getValue(unmappedFields, "emailserverprofile_duplicatematchingrecord"));
}
@NavigationProperty(name="EmailServerProfile_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getEmailServerProfile_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("EmailServerProfile_SyncErrors"), RequestHelper.getValue(unmappedFields, "EmailServerProfile_SyncErrors"));
}
@NavigationProperty(name="emailserverprofile_duplicatebaserecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getEmailserverprofile_duplicatebaserecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("emailserverprofile_duplicatebaserecord"), RequestHelper.getValue(unmappedFields, "emailserverprofile_duplicatebaserecord"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Emailserverprofile patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Emailserverprofile _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Emailserverprofile put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Emailserverprofile _x = _copy();
_x.changedFields = null;
return _x;
}
private Emailserverprofile _copy() {
Emailserverprofile _x = new Emailserverprofile();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.exchangeonlinetenantid = exchangeonlinetenantid;
_x.oauthclientsecret = oauthclientsecret;
_x.outgoingserverlocation = outgoingserverlocation;
_x.timeoutmailboxconnection = timeoutmailboxconnection;
_x._incomingpartnerapplication_value = _incomingpartnerapplication_value;
_x.timeoutmailboxconnectionafteramount = timeoutmailboxconnectionafteramount;
_x.name = name;
_x.incominguseimpersonation = incominguseimpersonation;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.usedefaulttenantid = usedefaulttenantid;
_x.isincomingpasswordset = isincomingpasswordset;
_x.oauthclientid = oauthclientid;
_x.incomingpassword = incomingpassword;
_x.statuscode = statuscode;
_x.outgoingusername = outgoingusername;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.lastcrmmessage = lastcrmmessage;
_x.outgoingpassword = outgoingpassword;
_x.incomingportnumber = incomingportnumber;
_x.encodingcodepage = encodingcodepage;
_x.isoauthclientsecretset = isoauthclientsecretset;
_x.sendemailalert = sendemailalert;
_x.emailservertypename = emailservertypename;
_x.processemailsreceivedafter = processemailsreceivedafter;
_x.lastauthorizationstatus = lastauthorizationstatus;
_x.entityimage = entityimage;
_x._ownerid_value = _ownerid_value;
_x.lasttesttotalexecutiontime = lasttesttotalexecutiontime;
_x.useautodiscover = useautodiscover;
_x._outgoingpartnerapplication_value = _outgoingpartnerapplication_value;
_x.outgoingauthenticationprotocol = outgoingauthenticationprotocol;
_x.incomingusessl = incomingusessl;
_x.createdon = createdon;
_x.outgoingautograntdelegateaccess = outgoingautograntdelegateaccess;
_x.incomingcredentialretrieval = incomingcredentialretrieval;
_x.incomingauthenticationprotocol = incomingauthenticationprotocol;
_x.maxconcurrentconnections = maxconcurrentconnections;
_x.owneremailaddress = owneremailaddress;
_x.outgoingportnumber = outgoingportnumber;
_x.outgoingusessl = outgoingusessl;
_x._modifiedby_value = _modifiedby_value;
_x.outgoingcredentialretrieval = outgoingcredentialretrieval;
_x.lasttestrequest = lasttestrequest;
_x.lasttestresponse = lasttestresponse;
_x.versionnumber = versionnumber;
_x.usesamesettingsforoutgoingconnections = usesamesettingsforoutgoingconnections;
_x.exchangeversion = exchangeversion;
_x.emailserverprofileid = emailserverprofileid;
_x._organizationid_value = _organizationid_value;
_x.minpollingintervalinminutes = minpollingintervalinminutes;
_x.entityimage_timestamp = entityimage_timestamp;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.incomingusername = incomingusername;
_x.description = description;
_x.entityimage_url = entityimage_url;
_x.isoutgoingpasswordset = isoutgoingpasswordset;
_x.incomingserverlocation = incomingserverlocation;
_x.lasttestvalidationstatus = lasttestvalidationstatus;
_x.lasttestexecutionstatus = lasttestexecutionstatus;
_x.entityimageid = entityimageid;
_x.moveundeliveredemails = moveundeliveredemails;
_x.defaultserverlocation = defaultserverlocation;
_x.outgoinguseimpersonation = outgoinguseimpersonation;
_x.modifiedon = modifiedon;
_x._owningteam_value = _owningteam_value;
_x.statecode = statecode;
_x._owninguser_value = _owninguser_value;
_x.lastteststarttime = lastteststarttime;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x._createdby_value = _createdby_value;
_x.servertype = servertype;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Emailserverprofile[");
b.append("exchangeonlinetenantid=");
b.append(this.exchangeonlinetenantid);
b.append(", ");
b.append("oauthclientsecret=");
b.append(this.oauthclientsecret);
b.append(", ");
b.append("outgoingserverlocation=");
b.append(this.outgoingserverlocation);
b.append(", ");
b.append("timeoutmailboxconnection=");
b.append(this.timeoutmailboxconnection);
b.append(", ");
b.append("_incomingpartnerapplication_value=");
b.append(this._incomingpartnerapplication_value);
b.append(", ");
b.append("timeoutmailboxconnectionafteramount=");
b.append(this.timeoutmailboxconnectionafteramount);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("incominguseimpersonation=");
b.append(this.incominguseimpersonation);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("usedefaulttenantid=");
b.append(this.usedefaulttenantid);
b.append(", ");
b.append("isincomingpasswordset=");
b.append(this.isincomingpasswordset);
b.append(", ");
b.append("oauthclientid=");
b.append(this.oauthclientid);
b.append(", ");
b.append("incomingpassword=");
b.append(this.incomingpassword);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("outgoingusername=");
b.append(this.outgoingusername);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("lastcrmmessage=");
b.append(this.lastcrmmessage);
b.append(", ");
b.append("outgoingpassword=");
b.append(this.outgoingpassword);
b.append(", ");
b.append("incomingportnumber=");
b.append(this.incomingportnumber);
b.append(", ");
b.append("encodingcodepage=");
b.append(this.encodingcodepage);
b.append(", ");
b.append("isoauthclientsecretset=");
b.append(this.isoauthclientsecretset);
b.append(", ");
b.append("sendemailalert=");
b.append(this.sendemailalert);
b.append(", ");
b.append("emailservertypename=");
b.append(this.emailservertypename);
b.append(", ");
b.append("processemailsreceivedafter=");
b.append(this.processemailsreceivedafter);
b.append(", ");
b.append("lastauthorizationstatus=");
b.append(this.lastauthorizationstatus);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("lasttesttotalexecutiontime=");
b.append(this.lasttesttotalexecutiontime);
b.append(", ");
b.append("useautodiscover=");
b.append(this.useautodiscover);
b.append(", ");
b.append("_outgoingpartnerapplication_value=");
b.append(this._outgoingpartnerapplication_value);
b.append(", ");
b.append("outgoingauthenticationprotocol=");
b.append(this.outgoingauthenticationprotocol);
b.append(", ");
b.append("incomingusessl=");
b.append(this.incomingusessl);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("outgoingautograntdelegateaccess=");
b.append(this.outgoingautograntdelegateaccess);
b.append(", ");
b.append("incomingcredentialretrieval=");
b.append(this.incomingcredentialretrieval);
b.append(", ");
b.append("incomingauthenticationprotocol=");
b.append(this.incomingauthenticationprotocol);
b.append(", ");
b.append("maxconcurrentconnections=");
b.append(this.maxconcurrentconnections);
b.append(", ");
b.append("owneremailaddress=");
b.append(this.owneremailaddress);
b.append(", ");
b.append("outgoingportnumber=");
b.append(this.outgoingportnumber);
b.append(", ");
b.append("outgoingusessl=");
b.append(this.outgoingusessl);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("outgoingcredentialretrieval=");
b.append(this.outgoingcredentialretrieval);
b.append(", ");
b.append("lasttestrequest=");
b.append(this.lasttestrequest);
b.append(", ");
b.append("lasttestresponse=");
b.append(this.lasttestresponse);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("usesamesettingsforoutgoingconnections=");
b.append(this.usesamesettingsforoutgoingconnections);
b.append(", ");
b.append("exchangeversion=");
b.append(this.exchangeversion);
b.append(", ");
b.append("emailserverprofileid=");
b.append(this.emailserverprofileid);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("minpollingintervalinminutes=");
b.append(this.minpollingintervalinminutes);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("incomingusername=");
b.append(this.incomingusername);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("isoutgoingpasswordset=");
b.append(this.isoutgoingpasswordset);
b.append(", ");
b.append("incomingserverlocation=");
b.append(this.incomingserverlocation);
b.append(", ");
b.append("lasttestvalidationstatus=");
b.append(this.lasttestvalidationstatus);
b.append(", ");
b.append("lasttestexecutionstatus=");
b.append(this.lasttestexecutionstatus);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("moveundeliveredemails=");
b.append(this.moveundeliveredemails);
b.append(", ");
b.append("defaultserverlocation=");
b.append(this.defaultserverlocation);
b.append(", ");
b.append("outgoinguseimpersonation=");
b.append(this.outgoinguseimpersonation);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("lastteststarttime=");
b.append(this.lastteststarttime);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("servertype=");
b.append(this.servertype);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy