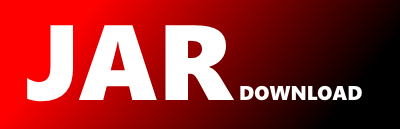
microsoft.dynamics.crm.entity.EntityMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.complex.EntitySetting;
import microsoft.dynamics.crm.complex.Label;
import microsoft.dynamics.crm.complex.SecurityPrivilegeMetadata;
import microsoft.dynamics.crm.entity.collection.request.AttributeMetadataCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EntityKeyMetadataCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ManyToManyRelationshipMetadataCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OneToManyRelationshipMetadataCollectionRequest;
import microsoft.dynamics.crm.enums.OwnershipTypes;
@JsonPropertyOrder({
"@odata.type",
"ActivityTypeMask",
"AutoRouteToOwnerQueue",
"CanTriggerWorkflow",
"Description",
"DisplayCollectionName",
"DisplayName",
"EntityHelpUrlEnabled",
"EntityHelpUrl",
"IsDocumentManagementEnabled",
"IsOneNoteIntegrationEnabled",
"IsInteractionCentricEnabled",
"IsKnowledgeManagementEnabled",
"IsSLAEnabled",
"IsBPFEntity",
"IsDocumentRecommendationsEnabled",
"IsMSTeamsIntegrationEnabled",
"SettingOf",
"DataProviderId",
"DataSourceId",
"AutoCreateAccessTeams",
"IsActivity",
"IsActivityParty",
"IsAuditEnabled",
"IsAvailableOffline",
"IsChildEntity",
"IsAIRUpdated",
"IsValidForQueue",
"IsConnectionsEnabled",
"IconLargeName",
"IconMediumName",
"IconSmallName",
"IconVectorName",
"IsCustomEntity",
"IsBusinessProcessEnabled",
"IsCustomizable",
"IsRenameable",
"IsMappable",
"IsDuplicateDetectionEnabled",
"CanCreateAttributes",
"CanCreateForms",
"CanCreateViews",
"CanCreateCharts",
"CanBeRelatedEntityInRelationship",
"CanBePrimaryEntityInRelationship",
"CanBeInManyToMany",
"CanBeInCustomEntityAssociation",
"CanEnableSyncToExternalSearchIndex",
"SyncToExternalSearchIndex",
"CanModifyAdditionalSettings",
"CanChangeHierarchicalRelationship",
"IsOptimisticConcurrencyEnabled",
"ChangeTrackingEnabled",
"CanChangeTrackingBeEnabled",
"IsImportable",
"IsIntersect",
"IsMailMergeEnabled",
"IsManaged",
"IsEnabledForCharts",
"IsEnabledForTrace",
"IsValidForAdvancedFind",
"IsVisibleInMobile",
"IsVisibleInMobileClient",
"IsReadOnlyInMobileClient",
"IsOfflineInMobileClient",
"DaysSinceRecordLastModified",
"MobileOfflineFilters",
"IsReadingPaneEnabled",
"IsQuickCreateEnabled",
"LogicalName",
"ObjectTypeCode",
"OwnershipType",
"PrimaryNameAttribute",
"PrimaryImageAttribute",
"PrimaryIdAttribute",
"Privileges",
"RecurrenceBaseEntityLogicalName",
"ReportViewName",
"SchemaName",
"IntroducedVersion",
"IsStateModelAware",
"EnforceStateTransitions",
"ExternalName",
"EntityColor",
"LogicalCollectionName",
"ExternalCollectionName",
"CollectionSchemaName",
"EntitySetName",
"IsEnabledForExternalChannels",
"IsPrivate",
"UsesBusinessDataLabelTable",
"IsLogicalEntity",
"HasNotes",
"HasActivities",
"HasFeedback",
"IsSolutionAware",
"Settings",
"CreatedOn",
"ModifiedOn",
"Attributes",
"Keys"})
@JsonInclude(Include.NON_NULL)
public class EntityMetadata extends MetadataBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.EntityMetadata";
}
@JsonProperty("ActivityTypeMask")
protected Integer activityTypeMask;
@JsonProperty("AutoRouteToOwnerQueue")
protected Boolean autoRouteToOwnerQueue;
@JsonProperty("CanTriggerWorkflow")
protected Boolean canTriggerWorkflow;
@JsonProperty("Description")
protected Label description;
@JsonProperty("DisplayCollectionName")
protected Label displayCollectionName;
@JsonProperty("DisplayName")
protected Label displayName;
@JsonProperty("EntityHelpUrlEnabled")
protected Boolean entityHelpUrlEnabled;
@JsonProperty("EntityHelpUrl")
protected String entityHelpUrl;
@JsonProperty("IsDocumentManagementEnabled")
protected Boolean isDocumentManagementEnabled;
@JsonProperty("IsOneNoteIntegrationEnabled")
protected Boolean isOneNoteIntegrationEnabled;
@JsonProperty("IsInteractionCentricEnabled")
protected Boolean isInteractionCentricEnabled;
@JsonProperty("IsKnowledgeManagementEnabled")
protected Boolean isKnowledgeManagementEnabled;
@JsonProperty("IsSLAEnabled")
protected Boolean isSLAEnabled;
@JsonProperty("IsBPFEntity")
protected Boolean isBPFEntity;
@JsonProperty("IsDocumentRecommendationsEnabled")
protected Boolean isDocumentRecommendationsEnabled;
@JsonProperty("IsMSTeamsIntegrationEnabled")
protected Boolean isMSTeamsIntegrationEnabled;
@JsonProperty("SettingOf")
protected String settingOf;
@JsonProperty("DataProviderId")
protected UUID dataProviderId;
@JsonProperty("DataSourceId")
protected UUID dataSourceId;
@JsonProperty("AutoCreateAccessTeams")
protected Boolean autoCreateAccessTeams;
@JsonProperty("IsActivity")
protected Boolean isActivity;
@JsonProperty("IsActivityParty")
protected Boolean isActivityParty;
@JsonProperty("IsAuditEnabled")
protected BooleanManagedProperty isAuditEnabled;
@JsonProperty("IsAvailableOffline")
protected Boolean isAvailableOffline;
@JsonProperty("IsChildEntity")
protected Boolean isChildEntity;
@JsonProperty("IsAIRUpdated")
protected Boolean isAIRUpdated;
@JsonProperty("IsValidForQueue")
protected BooleanManagedProperty isValidForQueue;
@JsonProperty("IsConnectionsEnabled")
protected BooleanManagedProperty isConnectionsEnabled;
@JsonProperty("IconLargeName")
protected String iconLargeName;
@JsonProperty("IconMediumName")
protected String iconMediumName;
@JsonProperty("IconSmallName")
protected String iconSmallName;
@JsonProperty("IconVectorName")
protected String iconVectorName;
@JsonProperty("IsCustomEntity")
protected Boolean isCustomEntity;
@JsonProperty("IsBusinessProcessEnabled")
protected Boolean isBusinessProcessEnabled;
@JsonProperty("IsCustomizable")
protected BooleanManagedProperty isCustomizable;
@JsonProperty("IsRenameable")
protected BooleanManagedProperty isRenameable;
@JsonProperty("IsMappable")
protected BooleanManagedProperty isMappable;
@JsonProperty("IsDuplicateDetectionEnabled")
protected BooleanManagedProperty isDuplicateDetectionEnabled;
@JsonProperty("CanCreateAttributes")
protected BooleanManagedProperty canCreateAttributes;
@JsonProperty("CanCreateForms")
protected BooleanManagedProperty canCreateForms;
@JsonProperty("CanCreateViews")
protected BooleanManagedProperty canCreateViews;
@JsonProperty("CanCreateCharts")
protected BooleanManagedProperty canCreateCharts;
@JsonProperty("CanBeRelatedEntityInRelationship")
protected BooleanManagedProperty canBeRelatedEntityInRelationship;
@JsonProperty("CanBePrimaryEntityInRelationship")
protected BooleanManagedProperty canBePrimaryEntityInRelationship;
@JsonProperty("CanBeInManyToMany")
protected BooleanManagedProperty canBeInManyToMany;
@JsonProperty("CanBeInCustomEntityAssociation")
protected BooleanManagedProperty canBeInCustomEntityAssociation;
@JsonProperty("CanEnableSyncToExternalSearchIndex")
protected BooleanManagedProperty canEnableSyncToExternalSearchIndex;
@JsonProperty("SyncToExternalSearchIndex")
protected Boolean syncToExternalSearchIndex;
@JsonProperty("CanModifyAdditionalSettings")
protected BooleanManagedProperty canModifyAdditionalSettings;
@JsonProperty("CanChangeHierarchicalRelationship")
protected BooleanManagedProperty canChangeHierarchicalRelationship;
@JsonProperty("IsOptimisticConcurrencyEnabled")
protected Boolean isOptimisticConcurrencyEnabled;
@JsonProperty("ChangeTrackingEnabled")
protected Boolean changeTrackingEnabled;
@JsonProperty("CanChangeTrackingBeEnabled")
protected BooleanManagedProperty canChangeTrackingBeEnabled;
@JsonProperty("IsImportable")
protected Boolean isImportable;
@JsonProperty("IsIntersect")
protected Boolean isIntersect;
@JsonProperty("IsMailMergeEnabled")
protected BooleanManagedProperty isMailMergeEnabled;
@JsonProperty("IsManaged")
protected Boolean isManaged;
@JsonProperty("IsEnabledForCharts")
protected Boolean isEnabledForCharts;
@JsonProperty("IsEnabledForTrace")
protected Boolean isEnabledForTrace;
@JsonProperty("IsValidForAdvancedFind")
protected Boolean isValidForAdvancedFind;
@JsonProperty("IsVisibleInMobile")
protected BooleanManagedProperty isVisibleInMobile;
@JsonProperty("IsVisibleInMobileClient")
protected BooleanManagedProperty isVisibleInMobileClient;
@JsonProperty("IsReadOnlyInMobileClient")
protected BooleanManagedProperty isReadOnlyInMobileClient;
@JsonProperty("IsOfflineInMobileClient")
protected BooleanManagedProperty isOfflineInMobileClient;
@JsonProperty("DaysSinceRecordLastModified")
protected Integer daysSinceRecordLastModified;
@JsonProperty("MobileOfflineFilters")
protected String mobileOfflineFilters;
@JsonProperty("IsReadingPaneEnabled")
protected Boolean isReadingPaneEnabled;
@JsonProperty("IsQuickCreateEnabled")
protected Boolean isQuickCreateEnabled;
@JsonProperty("LogicalName")
protected String logicalName;
@JsonProperty("ObjectTypeCode")
protected Integer objectTypeCode;
@JsonProperty("OwnershipType")
protected OwnershipTypes ownershipType;
@JsonProperty("PrimaryNameAttribute")
protected String primaryNameAttribute;
@JsonProperty("PrimaryImageAttribute")
protected String primaryImageAttribute;
@JsonProperty("PrimaryIdAttribute")
protected String primaryIdAttribute;
@JsonProperty("Privileges")
protected List privileges;
@JsonProperty("Privileges@nextLink")
protected String privilegesNextLink;
@JsonProperty("RecurrenceBaseEntityLogicalName")
protected String recurrenceBaseEntityLogicalName;
@JsonProperty("ReportViewName")
protected String reportViewName;
@JsonProperty("SchemaName")
protected String schemaName;
@JsonProperty("IntroducedVersion")
protected String introducedVersion;
@JsonProperty("IsStateModelAware")
protected Boolean isStateModelAware;
@JsonProperty("EnforceStateTransitions")
protected Boolean enforceStateTransitions;
@JsonProperty("ExternalName")
protected String externalName;
@JsonProperty("EntityColor")
protected String entityColor;
@JsonProperty("LogicalCollectionName")
protected String logicalCollectionName;
@JsonProperty("ExternalCollectionName")
protected String externalCollectionName;
@JsonProperty("CollectionSchemaName")
protected String collectionSchemaName;
@JsonProperty("EntitySetName")
protected String entitySetName;
@JsonProperty("IsEnabledForExternalChannels")
protected Boolean isEnabledForExternalChannels;
@JsonProperty("IsPrivate")
protected Boolean isPrivate;
@JsonProperty("UsesBusinessDataLabelTable")
protected Boolean usesBusinessDataLabelTable;
@JsonProperty("IsLogicalEntity")
protected Boolean isLogicalEntity;
@JsonProperty("HasNotes")
protected Boolean hasNotes;
@JsonProperty("HasActivities")
protected Boolean hasActivities;
@JsonProperty("HasFeedback")
protected Boolean hasFeedback;
@JsonProperty("IsSolutionAware")
protected Boolean isSolutionAware;
@JsonProperty("Settings")
protected List settings;
@JsonProperty("Settings@nextLink")
protected String settingsNextLink;
@JsonProperty("CreatedOn")
protected OffsetDateTime createdOn;
@JsonProperty("ModifiedOn")
protected OffsetDateTime modifiedOn;
@JsonProperty("Attributes")
protected List attributes;
@JsonProperty("Keys")
protected List keys;
protected EntityMetadata() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEntityMetadata() {
return new Builder();
}
public static final class Builder {
private UUID metadataId;
private Boolean hasChanged;
private Integer activityTypeMask;
private Boolean autoRouteToOwnerQueue;
private Boolean canTriggerWorkflow;
private Label description;
private Label displayCollectionName;
private Label displayName;
private Boolean entityHelpUrlEnabled;
private String entityHelpUrl;
private Boolean isDocumentManagementEnabled;
private Boolean isOneNoteIntegrationEnabled;
private Boolean isInteractionCentricEnabled;
private Boolean isKnowledgeManagementEnabled;
private Boolean isSLAEnabled;
private Boolean isBPFEntity;
private Boolean isDocumentRecommendationsEnabled;
private Boolean isMSTeamsIntegrationEnabled;
private String settingOf;
private UUID dataProviderId;
private UUID dataSourceId;
private Boolean autoCreateAccessTeams;
private Boolean isActivity;
private Boolean isActivityParty;
private BooleanManagedProperty isAuditEnabled;
private Boolean isAvailableOffline;
private Boolean isChildEntity;
private Boolean isAIRUpdated;
private BooleanManagedProperty isValidForQueue;
private BooleanManagedProperty isConnectionsEnabled;
private String iconLargeName;
private String iconMediumName;
private String iconSmallName;
private String iconVectorName;
private Boolean isCustomEntity;
private Boolean isBusinessProcessEnabled;
private BooleanManagedProperty isCustomizable;
private BooleanManagedProperty isRenameable;
private BooleanManagedProperty isMappable;
private BooleanManagedProperty isDuplicateDetectionEnabled;
private BooleanManagedProperty canCreateAttributes;
private BooleanManagedProperty canCreateForms;
private BooleanManagedProperty canCreateViews;
private BooleanManagedProperty canCreateCharts;
private BooleanManagedProperty canBeRelatedEntityInRelationship;
private BooleanManagedProperty canBePrimaryEntityInRelationship;
private BooleanManagedProperty canBeInManyToMany;
private BooleanManagedProperty canBeInCustomEntityAssociation;
private BooleanManagedProperty canEnableSyncToExternalSearchIndex;
private Boolean syncToExternalSearchIndex;
private BooleanManagedProperty canModifyAdditionalSettings;
private BooleanManagedProperty canChangeHierarchicalRelationship;
private Boolean isOptimisticConcurrencyEnabled;
private Boolean changeTrackingEnabled;
private BooleanManagedProperty canChangeTrackingBeEnabled;
private Boolean isImportable;
private Boolean isIntersect;
private BooleanManagedProperty isMailMergeEnabled;
private Boolean isManaged;
private Boolean isEnabledForCharts;
private Boolean isEnabledForTrace;
private Boolean isValidForAdvancedFind;
private BooleanManagedProperty isVisibleInMobile;
private BooleanManagedProperty isVisibleInMobileClient;
private BooleanManagedProperty isReadOnlyInMobileClient;
private BooleanManagedProperty isOfflineInMobileClient;
private Integer daysSinceRecordLastModified;
private String mobileOfflineFilters;
private Boolean isReadingPaneEnabled;
private Boolean isQuickCreateEnabled;
private String logicalName;
private Integer objectTypeCode;
private OwnershipTypes ownershipType;
private String primaryNameAttribute;
private String primaryImageAttribute;
private String primaryIdAttribute;
private List privileges;
private String privilegesNextLink;
private String recurrenceBaseEntityLogicalName;
private String reportViewName;
private String schemaName;
private String introducedVersion;
private Boolean isStateModelAware;
private Boolean enforceStateTransitions;
private String externalName;
private String entityColor;
private String logicalCollectionName;
private String externalCollectionName;
private String collectionSchemaName;
private String entitySetName;
private Boolean isEnabledForExternalChannels;
private Boolean isPrivate;
private Boolean usesBusinessDataLabelTable;
private Boolean isLogicalEntity;
private Boolean hasNotes;
private Boolean hasActivities;
private Boolean hasFeedback;
private Boolean isSolutionAware;
private List settings;
private String settingsNextLink;
private OffsetDateTime createdOn;
private OffsetDateTime modifiedOn;
private List attributes;
private List keys;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder metadataId(UUID metadataId) {
this.metadataId = metadataId;
this.changedFields = changedFields.add("MetadataId");
return this;
}
public Builder hasChanged(Boolean hasChanged) {
this.hasChanged = hasChanged;
this.changedFields = changedFields.add("HasChanged");
return this;
}
public Builder activityTypeMask(Integer activityTypeMask) {
this.activityTypeMask = activityTypeMask;
this.changedFields = changedFields.add("ActivityTypeMask");
return this;
}
public Builder autoRouteToOwnerQueue(Boolean autoRouteToOwnerQueue) {
this.autoRouteToOwnerQueue = autoRouteToOwnerQueue;
this.changedFields = changedFields.add("AutoRouteToOwnerQueue");
return this;
}
public Builder canTriggerWorkflow(Boolean canTriggerWorkflow) {
this.canTriggerWorkflow = canTriggerWorkflow;
this.changedFields = changedFields.add("CanTriggerWorkflow");
return this;
}
public Builder description(Label description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder displayCollectionName(Label displayCollectionName) {
this.displayCollectionName = displayCollectionName;
this.changedFields = changedFields.add("DisplayCollectionName");
return this;
}
public Builder displayName(Label displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("DisplayName");
return this;
}
public Builder entityHelpUrlEnabled(Boolean entityHelpUrlEnabled) {
this.entityHelpUrlEnabled = entityHelpUrlEnabled;
this.changedFields = changedFields.add("EntityHelpUrlEnabled");
return this;
}
public Builder entityHelpUrl(String entityHelpUrl) {
this.entityHelpUrl = entityHelpUrl;
this.changedFields = changedFields.add("EntityHelpUrl");
return this;
}
public Builder isDocumentManagementEnabled(Boolean isDocumentManagementEnabled) {
this.isDocumentManagementEnabled = isDocumentManagementEnabled;
this.changedFields = changedFields.add("IsDocumentManagementEnabled");
return this;
}
public Builder isOneNoteIntegrationEnabled(Boolean isOneNoteIntegrationEnabled) {
this.isOneNoteIntegrationEnabled = isOneNoteIntegrationEnabled;
this.changedFields = changedFields.add("IsOneNoteIntegrationEnabled");
return this;
}
public Builder isInteractionCentricEnabled(Boolean isInteractionCentricEnabled) {
this.isInteractionCentricEnabled = isInteractionCentricEnabled;
this.changedFields = changedFields.add("IsInteractionCentricEnabled");
return this;
}
public Builder isKnowledgeManagementEnabled(Boolean isKnowledgeManagementEnabled) {
this.isKnowledgeManagementEnabled = isKnowledgeManagementEnabled;
this.changedFields = changedFields.add("IsKnowledgeManagementEnabled");
return this;
}
public Builder isSLAEnabled(Boolean isSLAEnabled) {
this.isSLAEnabled = isSLAEnabled;
this.changedFields = changedFields.add("IsSLAEnabled");
return this;
}
public Builder isBPFEntity(Boolean isBPFEntity) {
this.isBPFEntity = isBPFEntity;
this.changedFields = changedFields.add("IsBPFEntity");
return this;
}
public Builder isDocumentRecommendationsEnabled(Boolean isDocumentRecommendationsEnabled) {
this.isDocumentRecommendationsEnabled = isDocumentRecommendationsEnabled;
this.changedFields = changedFields.add("IsDocumentRecommendationsEnabled");
return this;
}
public Builder isMSTeamsIntegrationEnabled(Boolean isMSTeamsIntegrationEnabled) {
this.isMSTeamsIntegrationEnabled = isMSTeamsIntegrationEnabled;
this.changedFields = changedFields.add("IsMSTeamsIntegrationEnabled");
return this;
}
public Builder settingOf(String settingOf) {
this.settingOf = settingOf;
this.changedFields = changedFields.add("SettingOf");
return this;
}
public Builder dataProviderId(UUID dataProviderId) {
this.dataProviderId = dataProviderId;
this.changedFields = changedFields.add("DataProviderId");
return this;
}
public Builder dataSourceId(UUID dataSourceId) {
this.dataSourceId = dataSourceId;
this.changedFields = changedFields.add("DataSourceId");
return this;
}
public Builder autoCreateAccessTeams(Boolean autoCreateAccessTeams) {
this.autoCreateAccessTeams = autoCreateAccessTeams;
this.changedFields = changedFields.add("AutoCreateAccessTeams");
return this;
}
public Builder isActivity(Boolean isActivity) {
this.isActivity = isActivity;
this.changedFields = changedFields.add("IsActivity");
return this;
}
public Builder isActivityParty(Boolean isActivityParty) {
this.isActivityParty = isActivityParty;
this.changedFields = changedFields.add("IsActivityParty");
return this;
}
public Builder isAuditEnabled(BooleanManagedProperty isAuditEnabled) {
this.isAuditEnabled = isAuditEnabled;
this.changedFields = changedFields.add("IsAuditEnabled");
return this;
}
public Builder isAvailableOffline(Boolean isAvailableOffline) {
this.isAvailableOffline = isAvailableOffline;
this.changedFields = changedFields.add("IsAvailableOffline");
return this;
}
public Builder isChildEntity(Boolean isChildEntity) {
this.isChildEntity = isChildEntity;
this.changedFields = changedFields.add("IsChildEntity");
return this;
}
public Builder isAIRUpdated(Boolean isAIRUpdated) {
this.isAIRUpdated = isAIRUpdated;
this.changedFields = changedFields.add("IsAIRUpdated");
return this;
}
public Builder isValidForQueue(BooleanManagedProperty isValidForQueue) {
this.isValidForQueue = isValidForQueue;
this.changedFields = changedFields.add("IsValidForQueue");
return this;
}
public Builder isConnectionsEnabled(BooleanManagedProperty isConnectionsEnabled) {
this.isConnectionsEnabled = isConnectionsEnabled;
this.changedFields = changedFields.add("IsConnectionsEnabled");
return this;
}
public Builder iconLargeName(String iconLargeName) {
this.iconLargeName = iconLargeName;
this.changedFields = changedFields.add("IconLargeName");
return this;
}
public Builder iconMediumName(String iconMediumName) {
this.iconMediumName = iconMediumName;
this.changedFields = changedFields.add("IconMediumName");
return this;
}
public Builder iconSmallName(String iconSmallName) {
this.iconSmallName = iconSmallName;
this.changedFields = changedFields.add("IconSmallName");
return this;
}
public Builder iconVectorName(String iconVectorName) {
this.iconVectorName = iconVectorName;
this.changedFields = changedFields.add("IconVectorName");
return this;
}
public Builder isCustomEntity(Boolean isCustomEntity) {
this.isCustomEntity = isCustomEntity;
this.changedFields = changedFields.add("IsCustomEntity");
return this;
}
public Builder isBusinessProcessEnabled(Boolean isBusinessProcessEnabled) {
this.isBusinessProcessEnabled = isBusinessProcessEnabled;
this.changedFields = changedFields.add("IsBusinessProcessEnabled");
return this;
}
public Builder isCustomizable(BooleanManagedProperty isCustomizable) {
this.isCustomizable = isCustomizable;
this.changedFields = changedFields.add("IsCustomizable");
return this;
}
public Builder isRenameable(BooleanManagedProperty isRenameable) {
this.isRenameable = isRenameable;
this.changedFields = changedFields.add("IsRenameable");
return this;
}
public Builder isMappable(BooleanManagedProperty isMappable) {
this.isMappable = isMappable;
this.changedFields = changedFields.add("IsMappable");
return this;
}
public Builder isDuplicateDetectionEnabled(BooleanManagedProperty isDuplicateDetectionEnabled) {
this.isDuplicateDetectionEnabled = isDuplicateDetectionEnabled;
this.changedFields = changedFields.add("IsDuplicateDetectionEnabled");
return this;
}
public Builder canCreateAttributes(BooleanManagedProperty canCreateAttributes) {
this.canCreateAttributes = canCreateAttributes;
this.changedFields = changedFields.add("CanCreateAttributes");
return this;
}
public Builder canCreateForms(BooleanManagedProperty canCreateForms) {
this.canCreateForms = canCreateForms;
this.changedFields = changedFields.add("CanCreateForms");
return this;
}
public Builder canCreateViews(BooleanManagedProperty canCreateViews) {
this.canCreateViews = canCreateViews;
this.changedFields = changedFields.add("CanCreateViews");
return this;
}
public Builder canCreateCharts(BooleanManagedProperty canCreateCharts) {
this.canCreateCharts = canCreateCharts;
this.changedFields = changedFields.add("CanCreateCharts");
return this;
}
public Builder canBeRelatedEntityInRelationship(BooleanManagedProperty canBeRelatedEntityInRelationship) {
this.canBeRelatedEntityInRelationship = canBeRelatedEntityInRelationship;
this.changedFields = changedFields.add("CanBeRelatedEntityInRelationship");
return this;
}
public Builder canBePrimaryEntityInRelationship(BooleanManagedProperty canBePrimaryEntityInRelationship) {
this.canBePrimaryEntityInRelationship = canBePrimaryEntityInRelationship;
this.changedFields = changedFields.add("CanBePrimaryEntityInRelationship");
return this;
}
public Builder canBeInManyToMany(BooleanManagedProperty canBeInManyToMany) {
this.canBeInManyToMany = canBeInManyToMany;
this.changedFields = changedFields.add("CanBeInManyToMany");
return this;
}
public Builder canBeInCustomEntityAssociation(BooleanManagedProperty canBeInCustomEntityAssociation) {
this.canBeInCustomEntityAssociation = canBeInCustomEntityAssociation;
this.changedFields = changedFields.add("CanBeInCustomEntityAssociation");
return this;
}
public Builder canEnableSyncToExternalSearchIndex(BooleanManagedProperty canEnableSyncToExternalSearchIndex) {
this.canEnableSyncToExternalSearchIndex = canEnableSyncToExternalSearchIndex;
this.changedFields = changedFields.add("CanEnableSyncToExternalSearchIndex");
return this;
}
public Builder syncToExternalSearchIndex(Boolean syncToExternalSearchIndex) {
this.syncToExternalSearchIndex = syncToExternalSearchIndex;
this.changedFields = changedFields.add("SyncToExternalSearchIndex");
return this;
}
public Builder canModifyAdditionalSettings(BooleanManagedProperty canModifyAdditionalSettings) {
this.canModifyAdditionalSettings = canModifyAdditionalSettings;
this.changedFields = changedFields.add("CanModifyAdditionalSettings");
return this;
}
public Builder canChangeHierarchicalRelationship(BooleanManagedProperty canChangeHierarchicalRelationship) {
this.canChangeHierarchicalRelationship = canChangeHierarchicalRelationship;
this.changedFields = changedFields.add("CanChangeHierarchicalRelationship");
return this;
}
public Builder isOptimisticConcurrencyEnabled(Boolean isOptimisticConcurrencyEnabled) {
this.isOptimisticConcurrencyEnabled = isOptimisticConcurrencyEnabled;
this.changedFields = changedFields.add("IsOptimisticConcurrencyEnabled");
return this;
}
public Builder changeTrackingEnabled(Boolean changeTrackingEnabled) {
this.changeTrackingEnabled = changeTrackingEnabled;
this.changedFields = changedFields.add("ChangeTrackingEnabled");
return this;
}
public Builder canChangeTrackingBeEnabled(BooleanManagedProperty canChangeTrackingBeEnabled) {
this.canChangeTrackingBeEnabled = canChangeTrackingBeEnabled;
this.changedFields = changedFields.add("CanChangeTrackingBeEnabled");
return this;
}
public Builder isImportable(Boolean isImportable) {
this.isImportable = isImportable;
this.changedFields = changedFields.add("IsImportable");
return this;
}
public Builder isIntersect(Boolean isIntersect) {
this.isIntersect = isIntersect;
this.changedFields = changedFields.add("IsIntersect");
return this;
}
public Builder isMailMergeEnabled(BooleanManagedProperty isMailMergeEnabled) {
this.isMailMergeEnabled = isMailMergeEnabled;
this.changedFields = changedFields.add("IsMailMergeEnabled");
return this;
}
public Builder isManaged(Boolean isManaged) {
this.isManaged = isManaged;
this.changedFields = changedFields.add("IsManaged");
return this;
}
public Builder isEnabledForCharts(Boolean isEnabledForCharts) {
this.isEnabledForCharts = isEnabledForCharts;
this.changedFields = changedFields.add("IsEnabledForCharts");
return this;
}
public Builder isEnabledForTrace(Boolean isEnabledForTrace) {
this.isEnabledForTrace = isEnabledForTrace;
this.changedFields = changedFields.add("IsEnabledForTrace");
return this;
}
public Builder isValidForAdvancedFind(Boolean isValidForAdvancedFind) {
this.isValidForAdvancedFind = isValidForAdvancedFind;
this.changedFields = changedFields.add("IsValidForAdvancedFind");
return this;
}
public Builder isVisibleInMobile(BooleanManagedProperty isVisibleInMobile) {
this.isVisibleInMobile = isVisibleInMobile;
this.changedFields = changedFields.add("IsVisibleInMobile");
return this;
}
public Builder isVisibleInMobileClient(BooleanManagedProperty isVisibleInMobileClient) {
this.isVisibleInMobileClient = isVisibleInMobileClient;
this.changedFields = changedFields.add("IsVisibleInMobileClient");
return this;
}
public Builder isReadOnlyInMobileClient(BooleanManagedProperty isReadOnlyInMobileClient) {
this.isReadOnlyInMobileClient = isReadOnlyInMobileClient;
this.changedFields = changedFields.add("IsReadOnlyInMobileClient");
return this;
}
public Builder isOfflineInMobileClient(BooleanManagedProperty isOfflineInMobileClient) {
this.isOfflineInMobileClient = isOfflineInMobileClient;
this.changedFields = changedFields.add("IsOfflineInMobileClient");
return this;
}
public Builder daysSinceRecordLastModified(Integer daysSinceRecordLastModified) {
this.daysSinceRecordLastModified = daysSinceRecordLastModified;
this.changedFields = changedFields.add("DaysSinceRecordLastModified");
return this;
}
public Builder mobileOfflineFilters(String mobileOfflineFilters) {
this.mobileOfflineFilters = mobileOfflineFilters;
this.changedFields = changedFields.add("MobileOfflineFilters");
return this;
}
public Builder isReadingPaneEnabled(Boolean isReadingPaneEnabled) {
this.isReadingPaneEnabled = isReadingPaneEnabled;
this.changedFields = changedFields.add("IsReadingPaneEnabled");
return this;
}
public Builder isQuickCreateEnabled(Boolean isQuickCreateEnabled) {
this.isQuickCreateEnabled = isQuickCreateEnabled;
this.changedFields = changedFields.add("IsQuickCreateEnabled");
return this;
}
public Builder logicalName(String logicalName) {
this.logicalName = logicalName;
this.changedFields = changedFields.add("LogicalName");
return this;
}
public Builder objectTypeCode(Integer objectTypeCode) {
this.objectTypeCode = objectTypeCode;
this.changedFields = changedFields.add("ObjectTypeCode");
return this;
}
public Builder ownershipType(OwnershipTypes ownershipType) {
this.ownershipType = ownershipType;
this.changedFields = changedFields.add("OwnershipType");
return this;
}
public Builder primaryNameAttribute(String primaryNameAttribute) {
this.primaryNameAttribute = primaryNameAttribute;
this.changedFields = changedFields.add("PrimaryNameAttribute");
return this;
}
public Builder primaryImageAttribute(String primaryImageAttribute) {
this.primaryImageAttribute = primaryImageAttribute;
this.changedFields = changedFields.add("PrimaryImageAttribute");
return this;
}
public Builder primaryIdAttribute(String primaryIdAttribute) {
this.primaryIdAttribute = primaryIdAttribute;
this.changedFields = changedFields.add("PrimaryIdAttribute");
return this;
}
public Builder privileges(List privileges) {
this.privileges = privileges;
this.changedFields = changedFields.add("Privileges");
return this;
}
public Builder privileges(SecurityPrivilegeMetadata... privileges) {
return privileges(Arrays.asList(privileges));
}
public Builder privilegesNextLink(String privilegesNextLink) {
this.privilegesNextLink = privilegesNextLink;
this.changedFields = changedFields.add("Privileges");
return this;
}
public Builder recurrenceBaseEntityLogicalName(String recurrenceBaseEntityLogicalName) {
this.recurrenceBaseEntityLogicalName = recurrenceBaseEntityLogicalName;
this.changedFields = changedFields.add("RecurrenceBaseEntityLogicalName");
return this;
}
public Builder reportViewName(String reportViewName) {
this.reportViewName = reportViewName;
this.changedFields = changedFields.add("ReportViewName");
return this;
}
public Builder schemaName(String schemaName) {
this.schemaName = schemaName;
this.changedFields = changedFields.add("SchemaName");
return this;
}
public Builder introducedVersion(String introducedVersion) {
this.introducedVersion = introducedVersion;
this.changedFields = changedFields.add("IntroducedVersion");
return this;
}
public Builder isStateModelAware(Boolean isStateModelAware) {
this.isStateModelAware = isStateModelAware;
this.changedFields = changedFields.add("IsStateModelAware");
return this;
}
public Builder enforceStateTransitions(Boolean enforceStateTransitions) {
this.enforceStateTransitions = enforceStateTransitions;
this.changedFields = changedFields.add("EnforceStateTransitions");
return this;
}
public Builder externalName(String externalName) {
this.externalName = externalName;
this.changedFields = changedFields.add("ExternalName");
return this;
}
public Builder entityColor(String entityColor) {
this.entityColor = entityColor;
this.changedFields = changedFields.add("EntityColor");
return this;
}
public Builder logicalCollectionName(String logicalCollectionName) {
this.logicalCollectionName = logicalCollectionName;
this.changedFields = changedFields.add("LogicalCollectionName");
return this;
}
public Builder externalCollectionName(String externalCollectionName) {
this.externalCollectionName = externalCollectionName;
this.changedFields = changedFields.add("ExternalCollectionName");
return this;
}
public Builder collectionSchemaName(String collectionSchemaName) {
this.collectionSchemaName = collectionSchemaName;
this.changedFields = changedFields.add("CollectionSchemaName");
return this;
}
public Builder entitySetName(String entitySetName) {
this.entitySetName = entitySetName;
this.changedFields = changedFields.add("EntitySetName");
return this;
}
public Builder isEnabledForExternalChannels(Boolean isEnabledForExternalChannels) {
this.isEnabledForExternalChannels = isEnabledForExternalChannels;
this.changedFields = changedFields.add("IsEnabledForExternalChannels");
return this;
}
public Builder isPrivate(Boolean isPrivate) {
this.isPrivate = isPrivate;
this.changedFields = changedFields.add("IsPrivate");
return this;
}
public Builder usesBusinessDataLabelTable(Boolean usesBusinessDataLabelTable) {
this.usesBusinessDataLabelTable = usesBusinessDataLabelTable;
this.changedFields = changedFields.add("UsesBusinessDataLabelTable");
return this;
}
public Builder isLogicalEntity(Boolean isLogicalEntity) {
this.isLogicalEntity = isLogicalEntity;
this.changedFields = changedFields.add("IsLogicalEntity");
return this;
}
public Builder hasNotes(Boolean hasNotes) {
this.hasNotes = hasNotes;
this.changedFields = changedFields.add("HasNotes");
return this;
}
public Builder hasActivities(Boolean hasActivities) {
this.hasActivities = hasActivities;
this.changedFields = changedFields.add("HasActivities");
return this;
}
public Builder hasFeedback(Boolean hasFeedback) {
this.hasFeedback = hasFeedback;
this.changedFields = changedFields.add("HasFeedback");
return this;
}
public Builder isSolutionAware(Boolean isSolutionAware) {
this.isSolutionAware = isSolutionAware;
this.changedFields = changedFields.add("IsSolutionAware");
return this;
}
public Builder settings(List settings) {
this.settings = settings;
this.changedFields = changedFields.add("Settings");
return this;
}
public Builder settings(EntitySetting... settings) {
return settings(Arrays.asList(settings));
}
public Builder settingsNextLink(String settingsNextLink) {
this.settingsNextLink = settingsNextLink;
this.changedFields = changedFields.add("Settings");
return this;
}
public Builder createdOn(OffsetDateTime createdOn) {
this.createdOn = createdOn;
this.changedFields = changedFields.add("CreatedOn");
return this;
}
public Builder modifiedOn(OffsetDateTime modifiedOn) {
this.modifiedOn = modifiedOn;
this.changedFields = changedFields.add("ModifiedOn");
return this;
}
public Builder attributes(List attributes) {
this.attributes = attributes;
this.changedFields = changedFields.add("Attributes");
return this;
}
public Builder attributes(AttributeMetadata... attributes) {
return attributes(Arrays.asList(attributes));
}
public Builder keys(List keys) {
this.keys = keys;
this.changedFields = changedFields.add("Keys");
return this;
}
public Builder keys(EntityKeyMetadata... keys) {
return keys(Arrays.asList(keys));
}
public EntityMetadata build() {
EntityMetadata _x = new EntityMetadata();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.EntityMetadata";
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
_x.activityTypeMask = activityTypeMask;
_x.autoRouteToOwnerQueue = autoRouteToOwnerQueue;
_x.canTriggerWorkflow = canTriggerWorkflow;
_x.description = description;
_x.displayCollectionName = displayCollectionName;
_x.displayName = displayName;
_x.entityHelpUrlEnabled = entityHelpUrlEnabled;
_x.entityHelpUrl = entityHelpUrl;
_x.isDocumentManagementEnabled = isDocumentManagementEnabled;
_x.isOneNoteIntegrationEnabled = isOneNoteIntegrationEnabled;
_x.isInteractionCentricEnabled = isInteractionCentricEnabled;
_x.isKnowledgeManagementEnabled = isKnowledgeManagementEnabled;
_x.isSLAEnabled = isSLAEnabled;
_x.isBPFEntity = isBPFEntity;
_x.isDocumentRecommendationsEnabled = isDocumentRecommendationsEnabled;
_x.isMSTeamsIntegrationEnabled = isMSTeamsIntegrationEnabled;
_x.settingOf = settingOf;
_x.dataProviderId = dataProviderId;
_x.dataSourceId = dataSourceId;
_x.autoCreateAccessTeams = autoCreateAccessTeams;
_x.isActivity = isActivity;
_x.isActivityParty = isActivityParty;
_x.isAuditEnabled = isAuditEnabled;
_x.isAvailableOffline = isAvailableOffline;
_x.isChildEntity = isChildEntity;
_x.isAIRUpdated = isAIRUpdated;
_x.isValidForQueue = isValidForQueue;
_x.isConnectionsEnabled = isConnectionsEnabled;
_x.iconLargeName = iconLargeName;
_x.iconMediumName = iconMediumName;
_x.iconSmallName = iconSmallName;
_x.iconVectorName = iconVectorName;
_x.isCustomEntity = isCustomEntity;
_x.isBusinessProcessEnabled = isBusinessProcessEnabled;
_x.isCustomizable = isCustomizable;
_x.isRenameable = isRenameable;
_x.isMappable = isMappable;
_x.isDuplicateDetectionEnabled = isDuplicateDetectionEnabled;
_x.canCreateAttributes = canCreateAttributes;
_x.canCreateForms = canCreateForms;
_x.canCreateViews = canCreateViews;
_x.canCreateCharts = canCreateCharts;
_x.canBeRelatedEntityInRelationship = canBeRelatedEntityInRelationship;
_x.canBePrimaryEntityInRelationship = canBePrimaryEntityInRelationship;
_x.canBeInManyToMany = canBeInManyToMany;
_x.canBeInCustomEntityAssociation = canBeInCustomEntityAssociation;
_x.canEnableSyncToExternalSearchIndex = canEnableSyncToExternalSearchIndex;
_x.syncToExternalSearchIndex = syncToExternalSearchIndex;
_x.canModifyAdditionalSettings = canModifyAdditionalSettings;
_x.canChangeHierarchicalRelationship = canChangeHierarchicalRelationship;
_x.isOptimisticConcurrencyEnabled = isOptimisticConcurrencyEnabled;
_x.changeTrackingEnabled = changeTrackingEnabled;
_x.canChangeTrackingBeEnabled = canChangeTrackingBeEnabled;
_x.isImportable = isImportable;
_x.isIntersect = isIntersect;
_x.isMailMergeEnabled = isMailMergeEnabled;
_x.isManaged = isManaged;
_x.isEnabledForCharts = isEnabledForCharts;
_x.isEnabledForTrace = isEnabledForTrace;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.isVisibleInMobile = isVisibleInMobile;
_x.isVisibleInMobileClient = isVisibleInMobileClient;
_x.isReadOnlyInMobileClient = isReadOnlyInMobileClient;
_x.isOfflineInMobileClient = isOfflineInMobileClient;
_x.daysSinceRecordLastModified = daysSinceRecordLastModified;
_x.mobileOfflineFilters = mobileOfflineFilters;
_x.isReadingPaneEnabled = isReadingPaneEnabled;
_x.isQuickCreateEnabled = isQuickCreateEnabled;
_x.logicalName = logicalName;
_x.objectTypeCode = objectTypeCode;
_x.ownershipType = ownershipType;
_x.primaryNameAttribute = primaryNameAttribute;
_x.primaryImageAttribute = primaryImageAttribute;
_x.primaryIdAttribute = primaryIdAttribute;
_x.privileges = privileges;
_x.privilegesNextLink = privilegesNextLink;
_x.recurrenceBaseEntityLogicalName = recurrenceBaseEntityLogicalName;
_x.reportViewName = reportViewName;
_x.schemaName = schemaName;
_x.introducedVersion = introducedVersion;
_x.isStateModelAware = isStateModelAware;
_x.enforceStateTransitions = enforceStateTransitions;
_x.externalName = externalName;
_x.entityColor = entityColor;
_x.logicalCollectionName = logicalCollectionName;
_x.externalCollectionName = externalCollectionName;
_x.collectionSchemaName = collectionSchemaName;
_x.entitySetName = entitySetName;
_x.isEnabledForExternalChannels = isEnabledForExternalChannels;
_x.isPrivate = isPrivate;
_x.usesBusinessDataLabelTable = usesBusinessDataLabelTable;
_x.isLogicalEntity = isLogicalEntity;
_x.hasNotes = hasNotes;
_x.hasActivities = hasActivities;
_x.hasFeedback = hasFeedback;
_x.isSolutionAware = isSolutionAware;
_x.settings = settings;
_x.settingsNextLink = settingsNextLink;
_x.createdOn = createdOn;
_x.modifiedOn = modifiedOn;
_x.attributes = attributes;
_x.keys = keys;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && metadataId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(metadataId, UUID.class));
}
}
@Property(name="ActivityTypeMask")
@JsonIgnore
public Optional getActivityTypeMask() {
return Optional.ofNullable(activityTypeMask);
}
public EntityMetadata withActivityTypeMask(Integer activityTypeMask) {
EntityMetadata _x = _copy();
_x.changedFields = changedFields.add("ActivityTypeMask");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityMetadata");
_x.activityTypeMask = activityTypeMask;
return _x;
}
@Property(name="AutoRouteToOwnerQueue")
@JsonIgnore
public Optional getAutoRouteToOwnerQueue() {
return Optional.ofNullable(autoRouteToOwnerQueue);
}
public EntityMetadata withAutoRouteToOwnerQueue(Boolean autoRouteToOwnerQueue) {
EntityMetadata _x = _copy();
_x.changedFields = changedFields.add("AutoRouteToOwnerQueue");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityMetadata");
_x.autoRouteToOwnerQueue = autoRouteToOwnerQueue;
return _x;
}
@Property(name="CanTriggerWorkflow")
@JsonIgnore
public Optional getCanTriggerWorkflow() {
return Optional.ofNullable(canTriggerWorkflow);
}
public EntityMetadata withCanTriggerWorkflow(Boolean canTriggerWorkflow) {
EntityMetadata _x = _copy();
_x.changedFields = changedFields.add("CanTriggerWorkflow");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.EntityMetadata");
_x.canTriggerWorkflow = canTriggerWorkflow;
return _x;
}
@Property(name="Description")
@JsonIgnore
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy