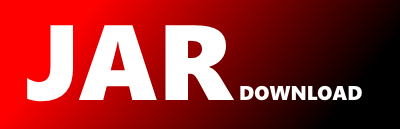
microsoft.dynamics.crm.entity.Goal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.GoalCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.GoalRequest;
import microsoft.dynamics.crm.entity.request.GoalrollupqueryRequest;
import microsoft.dynamics.crm.entity.request.MetricRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"computedtargetasoftodaypercentageachieved",
"stretchtargetstring",
"amountdatatype",
"_owningbusinessunit_value",
"createdon",
"_rollupqueryinprogressintegerid_value",
"_createdby_value",
"inprogressinteger",
"entityimage_url",
"overriddencreatedon",
"_rollupqueryactualintegerid_value",
"fiscalperiod",
"_modifiedby_value",
"isoverridden",
"title",
"goalenddate",
"_owningteam_value",
"_rollupqueryactualdecimalid_value",
"_rollupqueryactualmoneyid_value",
"exchangerate",
"inprogressdecimal",
"consideronlygoalownersrecords",
"computedtargetasoftodayinteger",
"goalid",
"entityimageid",
"targetdecimal",
"computedtargetasoftodaymoney",
"isamount",
"modifiedon",
"actualinteger",
"customrollupfieldmoney",
"entityimage",
"versionnumber",
"entityimage_timestamp",
"_ownerid_value",
"percentage",
"actualstring",
"actualmoney",
"stretchtargetinteger",
"lastrolledupdate",
"actualmoney_base",
"computedtargetasoftodaymoney_base",
"customrollupfieldmoney_base",
"_modifiedonbehalfby_value",
"targetinteger",
"inprogressstring",
"goalstartdate",
"inprogressmoney_base",
"targetstring",
"timezoneruleversionnumber",
"statuscode",
"isoverride",
"_goalownerid_value",
"_rollupquerycustommoneyid_value",
"_owninguser_value",
"targetmoney",
"_transactioncurrencyid_value",
"_rollupqueryinprogressdecimalid_value",
"statecode",
"stretchtargetmoney",
"importsequencenumber",
"treeid",
"_goalwitherrorid_value",
"rolluponlyfromchildgoals",
"isfiscalperiodgoal",
"actualdecimal",
"customrollupfieldstring",
"_rollupqueryinprogressmoneyid_value",
"_rollupquerycustomdecimalid_value",
"utcconversiontimezonecode",
"_rollupquerycustomintegerid_value",
"stretchtargetmoney_base",
"_metricid_value",
"computedtargetasoftodaydecimal",
"_createdonbehalfby_value",
"customrollupfielddecimal",
"fiscalyear",
"stretchtargetdecimal",
"targetmoney_base",
"inprogressmoney",
"depth",
"rolluperrorcode",
"_parentgoalid_value",
"customrollupfieldinteger"})
@JsonInclude(Include.NON_NULL)
public class Goal extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.goal";
}
@JsonProperty("computedtargetasoftodaypercentageachieved")
protected BigDecimal computedtargetasoftodaypercentageachieved;
@JsonProperty("stretchtargetstring")
protected String stretchtargetstring;
@JsonProperty("amountdatatype")
protected Integer amountdatatype;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("_rollupqueryinprogressintegerid_value")
protected UUID _rollupqueryinprogressintegerid_value;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("inprogressinteger")
protected Integer inprogressinteger;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("_rollupqueryactualintegerid_value")
protected UUID _rollupqueryactualintegerid_value;
@JsonProperty("fiscalperiod")
protected Integer fiscalperiod;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("isoverridden")
protected Boolean isoverridden;
@JsonProperty("title")
protected String title;
@JsonProperty("goalenddate")
protected OffsetDateTime goalenddate;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("_rollupqueryactualdecimalid_value")
protected UUID _rollupqueryactualdecimalid_value;
@JsonProperty("_rollupqueryactualmoneyid_value")
protected UUID _rollupqueryactualmoneyid_value;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("inprogressdecimal")
protected BigDecimal inprogressdecimal;
@JsonProperty("consideronlygoalownersrecords")
protected Boolean consideronlygoalownersrecords;
@JsonProperty("computedtargetasoftodayinteger")
protected Integer computedtargetasoftodayinteger;
@JsonProperty("goalid")
protected UUID goalid;
@JsonProperty("entityimageid")
protected UUID entityimageid;
@JsonProperty("targetdecimal")
protected BigDecimal targetdecimal;
@JsonProperty("computedtargetasoftodaymoney")
protected BigDecimal computedtargetasoftodaymoney;
@JsonProperty("isamount")
protected Boolean isamount;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("actualinteger")
protected Integer actualinteger;
@JsonProperty("customrollupfieldmoney")
protected BigDecimal customrollupfieldmoney;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("percentage")
protected BigDecimal percentage;
@JsonProperty("actualstring")
protected String actualstring;
@JsonProperty("actualmoney")
protected BigDecimal actualmoney;
@JsonProperty("stretchtargetinteger")
protected Integer stretchtargetinteger;
@JsonProperty("lastrolledupdate")
protected OffsetDateTime lastrolledupdate;
@JsonProperty("actualmoney_base")
protected BigDecimal actualmoney_base;
@JsonProperty("computedtargetasoftodaymoney_base")
protected BigDecimal computedtargetasoftodaymoney_base;
@JsonProperty("customrollupfieldmoney_base")
protected BigDecimal customrollupfieldmoney_base;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("targetinteger")
protected Integer targetinteger;
@JsonProperty("inprogressstring")
protected String inprogressstring;
@JsonProperty("goalstartdate")
protected OffsetDateTime goalstartdate;
@JsonProperty("inprogressmoney_base")
protected BigDecimal inprogressmoney_base;
@JsonProperty("targetstring")
protected String targetstring;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("isoverride")
protected Boolean isoverride;
@JsonProperty("_goalownerid_value")
protected UUID _goalownerid_value;
@JsonProperty("_rollupquerycustommoneyid_value")
protected UUID _rollupquerycustommoneyid_value;
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("targetmoney")
protected BigDecimal targetmoney;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("_rollupqueryinprogressdecimalid_value")
protected UUID _rollupqueryinprogressdecimalid_value;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("stretchtargetmoney")
protected BigDecimal stretchtargetmoney;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("treeid")
protected UUID treeid;
@JsonProperty("_goalwitherrorid_value")
protected UUID _goalwitherrorid_value;
@JsonProperty("rolluponlyfromchildgoals")
protected Boolean rolluponlyfromchildgoals;
@JsonProperty("isfiscalperiodgoal")
protected Boolean isfiscalperiodgoal;
@JsonProperty("actualdecimal")
protected BigDecimal actualdecimal;
@JsonProperty("customrollupfieldstring")
protected String customrollupfieldstring;
@JsonProperty("_rollupqueryinprogressmoneyid_value")
protected UUID _rollupqueryinprogressmoneyid_value;
@JsonProperty("_rollupquerycustomdecimalid_value")
protected UUID _rollupquerycustomdecimalid_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("_rollupquerycustomintegerid_value")
protected UUID _rollupquerycustomintegerid_value;
@JsonProperty("stretchtargetmoney_base")
protected BigDecimal stretchtargetmoney_base;
@JsonProperty("_metricid_value")
protected UUID _metricid_value;
@JsonProperty("computedtargetasoftodaydecimal")
protected BigDecimal computedtargetasoftodaydecimal;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("customrollupfielddecimal")
protected BigDecimal customrollupfielddecimal;
@JsonProperty("fiscalyear")
protected Integer fiscalyear;
@JsonProperty("stretchtargetdecimal")
protected BigDecimal stretchtargetdecimal;
@JsonProperty("targetmoney_base")
protected BigDecimal targetmoney_base;
@JsonProperty("inprogressmoney")
protected BigDecimal inprogressmoney;
@JsonProperty("depth")
protected Integer depth;
@JsonProperty("rolluperrorcode")
protected Integer rolluperrorcode;
@JsonProperty("_parentgoalid_value")
protected UUID _parentgoalid_value;
@JsonProperty("customrollupfieldinteger")
protected Integer customrollupfieldinteger;
protected Goal() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderGoal() {
return new Builder();
}
public static final class Builder {
private BigDecimal computedtargetasoftodaypercentageachieved;
private String stretchtargetstring;
private Integer amountdatatype;
private UUID _owningbusinessunit_value;
private OffsetDateTime createdon;
private UUID _rollupqueryinprogressintegerid_value;
private UUID _createdby_value;
private Integer inprogressinteger;
private String entityimage_url;
private OffsetDateTime overriddencreatedon;
private UUID _rollupqueryactualintegerid_value;
private Integer fiscalperiod;
private UUID _modifiedby_value;
private Boolean isoverridden;
private String title;
private OffsetDateTime goalenddate;
private UUID _owningteam_value;
private UUID _rollupqueryactualdecimalid_value;
private UUID _rollupqueryactualmoneyid_value;
private BigDecimal exchangerate;
private BigDecimal inprogressdecimal;
private Boolean consideronlygoalownersrecords;
private Integer computedtargetasoftodayinteger;
private UUID goalid;
private UUID entityimageid;
private BigDecimal targetdecimal;
private BigDecimal computedtargetasoftodaymoney;
private Boolean isamount;
private OffsetDateTime modifiedon;
private Integer actualinteger;
private BigDecimal customrollupfieldmoney;
private byte[] entityimage;
private Long versionnumber;
private Long entityimage_timestamp;
private UUID _ownerid_value;
private BigDecimal percentage;
private String actualstring;
private BigDecimal actualmoney;
private Integer stretchtargetinteger;
private OffsetDateTime lastrolledupdate;
private BigDecimal actualmoney_base;
private BigDecimal computedtargetasoftodaymoney_base;
private BigDecimal customrollupfieldmoney_base;
private UUID _modifiedonbehalfby_value;
private Integer targetinteger;
private String inprogressstring;
private OffsetDateTime goalstartdate;
private BigDecimal inprogressmoney_base;
private String targetstring;
private Integer timezoneruleversionnumber;
private Integer statuscode;
private Boolean isoverride;
private UUID _goalownerid_value;
private UUID _rollupquerycustommoneyid_value;
private UUID _owninguser_value;
private BigDecimal targetmoney;
private UUID _transactioncurrencyid_value;
private UUID _rollupqueryinprogressdecimalid_value;
private Integer statecode;
private BigDecimal stretchtargetmoney;
private Integer importsequencenumber;
private UUID treeid;
private UUID _goalwitherrorid_value;
private Boolean rolluponlyfromchildgoals;
private Boolean isfiscalperiodgoal;
private BigDecimal actualdecimal;
private String customrollupfieldstring;
private UUID _rollupqueryinprogressmoneyid_value;
private UUID _rollupquerycustomdecimalid_value;
private Integer utcconversiontimezonecode;
private UUID _rollupquerycustomintegerid_value;
private BigDecimal stretchtargetmoney_base;
private UUID _metricid_value;
private BigDecimal computedtargetasoftodaydecimal;
private UUID _createdonbehalfby_value;
private BigDecimal customrollupfielddecimal;
private Integer fiscalyear;
private BigDecimal stretchtargetdecimal;
private BigDecimal targetmoney_base;
private BigDecimal inprogressmoney;
private Integer depth;
private Integer rolluperrorcode;
private UUID _parentgoalid_value;
private Integer customrollupfieldinteger;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder computedtargetasoftodaypercentageachieved(BigDecimal computedtargetasoftodaypercentageachieved) {
this.computedtargetasoftodaypercentageachieved = computedtargetasoftodaypercentageachieved;
this.changedFields = changedFields.add("computedtargetasoftodaypercentageachieved");
return this;
}
public Builder stretchtargetstring(String stretchtargetstring) {
this.stretchtargetstring = stretchtargetstring;
this.changedFields = changedFields.add("stretchtargetstring");
return this;
}
public Builder amountdatatype(Integer amountdatatype) {
this.amountdatatype = amountdatatype;
this.changedFields = changedFields.add("amountdatatype");
return this;
}
public Builder _owningbusinessunit_value(UUID _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder _rollupqueryinprogressintegerid_value(UUID _rollupqueryinprogressintegerid_value) {
this._rollupqueryinprogressintegerid_value = _rollupqueryinprogressintegerid_value;
this.changedFields = changedFields.add("_rollupqueryinprogressintegerid_value");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder inprogressinteger(Integer inprogressinteger) {
this.inprogressinteger = inprogressinteger;
this.changedFields = changedFields.add("inprogressinteger");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder _rollupqueryactualintegerid_value(UUID _rollupqueryactualintegerid_value) {
this._rollupqueryactualintegerid_value = _rollupqueryactualintegerid_value;
this.changedFields = changedFields.add("_rollupqueryactualintegerid_value");
return this;
}
public Builder fiscalperiod(Integer fiscalperiod) {
this.fiscalperiod = fiscalperiod;
this.changedFields = changedFields.add("fiscalperiod");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder isoverridden(Boolean isoverridden) {
this.isoverridden = isoverridden;
this.changedFields = changedFields.add("isoverridden");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("title");
return this;
}
public Builder goalenddate(OffsetDateTime goalenddate) {
this.goalenddate = goalenddate;
this.changedFields = changedFields.add("goalenddate");
return this;
}
public Builder _owningteam_value(UUID _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder _rollupqueryactualdecimalid_value(UUID _rollupqueryactualdecimalid_value) {
this._rollupqueryactualdecimalid_value = _rollupqueryactualdecimalid_value;
this.changedFields = changedFields.add("_rollupqueryactualdecimalid_value");
return this;
}
public Builder _rollupqueryactualmoneyid_value(UUID _rollupqueryactualmoneyid_value) {
this._rollupqueryactualmoneyid_value = _rollupqueryactualmoneyid_value;
this.changedFields = changedFields.add("_rollupqueryactualmoneyid_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder inprogressdecimal(BigDecimal inprogressdecimal) {
this.inprogressdecimal = inprogressdecimal;
this.changedFields = changedFields.add("inprogressdecimal");
return this;
}
public Builder consideronlygoalownersrecords(Boolean consideronlygoalownersrecords) {
this.consideronlygoalownersrecords = consideronlygoalownersrecords;
this.changedFields = changedFields.add("consideronlygoalownersrecords");
return this;
}
public Builder computedtargetasoftodayinteger(Integer computedtargetasoftodayinteger) {
this.computedtargetasoftodayinteger = computedtargetasoftodayinteger;
this.changedFields = changedFields.add("computedtargetasoftodayinteger");
return this;
}
public Builder goalid(UUID goalid) {
this.goalid = goalid;
this.changedFields = changedFields.add("goalid");
return this;
}
public Builder entityimageid(UUID entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder targetdecimal(BigDecimal targetdecimal) {
this.targetdecimal = targetdecimal;
this.changedFields = changedFields.add("targetdecimal");
return this;
}
public Builder computedtargetasoftodaymoney(BigDecimal computedtargetasoftodaymoney) {
this.computedtargetasoftodaymoney = computedtargetasoftodaymoney;
this.changedFields = changedFields.add("computedtargetasoftodaymoney");
return this;
}
public Builder isamount(Boolean isamount) {
this.isamount = isamount;
this.changedFields = changedFields.add("isamount");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder actualinteger(Integer actualinteger) {
this.actualinteger = actualinteger;
this.changedFields = changedFields.add("actualinteger");
return this;
}
public Builder customrollupfieldmoney(BigDecimal customrollupfieldmoney) {
this.customrollupfieldmoney = customrollupfieldmoney;
this.changedFields = changedFields.add("customrollupfieldmoney");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder _ownerid_value(UUID _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder percentage(BigDecimal percentage) {
this.percentage = percentage;
this.changedFields = changedFields.add("percentage");
return this;
}
public Builder actualstring(String actualstring) {
this.actualstring = actualstring;
this.changedFields = changedFields.add("actualstring");
return this;
}
public Builder actualmoney(BigDecimal actualmoney) {
this.actualmoney = actualmoney;
this.changedFields = changedFields.add("actualmoney");
return this;
}
public Builder stretchtargetinteger(Integer stretchtargetinteger) {
this.stretchtargetinteger = stretchtargetinteger;
this.changedFields = changedFields.add("stretchtargetinteger");
return this;
}
public Builder lastrolledupdate(OffsetDateTime lastrolledupdate) {
this.lastrolledupdate = lastrolledupdate;
this.changedFields = changedFields.add("lastrolledupdate");
return this;
}
public Builder actualmoney_base(BigDecimal actualmoney_base) {
this.actualmoney_base = actualmoney_base;
this.changedFields = changedFields.add("actualmoney_base");
return this;
}
public Builder computedtargetasoftodaymoney_base(BigDecimal computedtargetasoftodaymoney_base) {
this.computedtargetasoftodaymoney_base = computedtargetasoftodaymoney_base;
this.changedFields = changedFields.add("computedtargetasoftodaymoney_base");
return this;
}
public Builder customrollupfieldmoney_base(BigDecimal customrollupfieldmoney_base) {
this.customrollupfieldmoney_base = customrollupfieldmoney_base;
this.changedFields = changedFields.add("customrollupfieldmoney_base");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder targetinteger(Integer targetinteger) {
this.targetinteger = targetinteger;
this.changedFields = changedFields.add("targetinteger");
return this;
}
public Builder inprogressstring(String inprogressstring) {
this.inprogressstring = inprogressstring;
this.changedFields = changedFields.add("inprogressstring");
return this;
}
public Builder goalstartdate(OffsetDateTime goalstartdate) {
this.goalstartdate = goalstartdate;
this.changedFields = changedFields.add("goalstartdate");
return this;
}
public Builder inprogressmoney_base(BigDecimal inprogressmoney_base) {
this.inprogressmoney_base = inprogressmoney_base;
this.changedFields = changedFields.add("inprogressmoney_base");
return this;
}
public Builder targetstring(String targetstring) {
this.targetstring = targetstring;
this.changedFields = changedFields.add("targetstring");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder isoverride(Boolean isoverride) {
this.isoverride = isoverride;
this.changedFields = changedFields.add("isoverride");
return this;
}
public Builder _goalownerid_value(UUID _goalownerid_value) {
this._goalownerid_value = _goalownerid_value;
this.changedFields = changedFields.add("_goalownerid_value");
return this;
}
public Builder _rollupquerycustommoneyid_value(UUID _rollupquerycustommoneyid_value) {
this._rollupquerycustommoneyid_value = _rollupquerycustommoneyid_value;
this.changedFields = changedFields.add("_rollupquerycustommoneyid_value");
return this;
}
public Builder _owninguser_value(UUID _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder targetmoney(BigDecimal targetmoney) {
this.targetmoney = targetmoney;
this.changedFields = changedFields.add("targetmoney");
return this;
}
public Builder _transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder _rollupqueryinprogressdecimalid_value(UUID _rollupqueryinprogressdecimalid_value) {
this._rollupqueryinprogressdecimalid_value = _rollupqueryinprogressdecimalid_value;
this.changedFields = changedFields.add("_rollupqueryinprogressdecimalid_value");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder stretchtargetmoney(BigDecimal stretchtargetmoney) {
this.stretchtargetmoney = stretchtargetmoney;
this.changedFields = changedFields.add("stretchtargetmoney");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder treeid(UUID treeid) {
this.treeid = treeid;
this.changedFields = changedFields.add("treeid");
return this;
}
public Builder _goalwitherrorid_value(UUID _goalwitherrorid_value) {
this._goalwitherrorid_value = _goalwitherrorid_value;
this.changedFields = changedFields.add("_goalwitherrorid_value");
return this;
}
public Builder rolluponlyfromchildgoals(Boolean rolluponlyfromchildgoals) {
this.rolluponlyfromchildgoals = rolluponlyfromchildgoals;
this.changedFields = changedFields.add("rolluponlyfromchildgoals");
return this;
}
public Builder isfiscalperiodgoal(Boolean isfiscalperiodgoal) {
this.isfiscalperiodgoal = isfiscalperiodgoal;
this.changedFields = changedFields.add("isfiscalperiodgoal");
return this;
}
public Builder actualdecimal(BigDecimal actualdecimal) {
this.actualdecimal = actualdecimal;
this.changedFields = changedFields.add("actualdecimal");
return this;
}
public Builder customrollupfieldstring(String customrollupfieldstring) {
this.customrollupfieldstring = customrollupfieldstring;
this.changedFields = changedFields.add("customrollupfieldstring");
return this;
}
public Builder _rollupqueryinprogressmoneyid_value(UUID _rollupqueryinprogressmoneyid_value) {
this._rollupqueryinprogressmoneyid_value = _rollupqueryinprogressmoneyid_value;
this.changedFields = changedFields.add("_rollupqueryinprogressmoneyid_value");
return this;
}
public Builder _rollupquerycustomdecimalid_value(UUID _rollupquerycustomdecimalid_value) {
this._rollupquerycustomdecimalid_value = _rollupquerycustomdecimalid_value;
this.changedFields = changedFields.add("_rollupquerycustomdecimalid_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder _rollupquerycustomintegerid_value(UUID _rollupquerycustomintegerid_value) {
this._rollupquerycustomintegerid_value = _rollupquerycustomintegerid_value;
this.changedFields = changedFields.add("_rollupquerycustomintegerid_value");
return this;
}
public Builder stretchtargetmoney_base(BigDecimal stretchtargetmoney_base) {
this.stretchtargetmoney_base = stretchtargetmoney_base;
this.changedFields = changedFields.add("stretchtargetmoney_base");
return this;
}
public Builder _metricid_value(UUID _metricid_value) {
this._metricid_value = _metricid_value;
this.changedFields = changedFields.add("_metricid_value");
return this;
}
public Builder computedtargetasoftodaydecimal(BigDecimal computedtargetasoftodaydecimal) {
this.computedtargetasoftodaydecimal = computedtargetasoftodaydecimal;
this.changedFields = changedFields.add("computedtargetasoftodaydecimal");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder customrollupfielddecimal(BigDecimal customrollupfielddecimal) {
this.customrollupfielddecimal = customrollupfielddecimal;
this.changedFields = changedFields.add("customrollupfielddecimal");
return this;
}
public Builder fiscalyear(Integer fiscalyear) {
this.fiscalyear = fiscalyear;
this.changedFields = changedFields.add("fiscalyear");
return this;
}
public Builder stretchtargetdecimal(BigDecimal stretchtargetdecimal) {
this.stretchtargetdecimal = stretchtargetdecimal;
this.changedFields = changedFields.add("stretchtargetdecimal");
return this;
}
public Builder targetmoney_base(BigDecimal targetmoney_base) {
this.targetmoney_base = targetmoney_base;
this.changedFields = changedFields.add("targetmoney_base");
return this;
}
public Builder inprogressmoney(BigDecimal inprogressmoney) {
this.inprogressmoney = inprogressmoney;
this.changedFields = changedFields.add("inprogressmoney");
return this;
}
public Builder depth(Integer depth) {
this.depth = depth;
this.changedFields = changedFields.add("depth");
return this;
}
public Builder rolluperrorcode(Integer rolluperrorcode) {
this.rolluperrorcode = rolluperrorcode;
this.changedFields = changedFields.add("rolluperrorcode");
return this;
}
public Builder _parentgoalid_value(UUID _parentgoalid_value) {
this._parentgoalid_value = _parentgoalid_value;
this.changedFields = changedFields.add("_parentgoalid_value");
return this;
}
public Builder customrollupfieldinteger(Integer customrollupfieldinteger) {
this.customrollupfieldinteger = customrollupfieldinteger;
this.changedFields = changedFields.add("customrollupfieldinteger");
return this;
}
public Goal build() {
Goal _x = new Goal();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.goal";
_x.computedtargetasoftodaypercentageachieved = computedtargetasoftodaypercentageachieved;
_x.stretchtargetstring = stretchtargetstring;
_x.amountdatatype = amountdatatype;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.createdon = createdon;
_x._rollupqueryinprogressintegerid_value = _rollupqueryinprogressintegerid_value;
_x._createdby_value = _createdby_value;
_x.inprogressinteger = inprogressinteger;
_x.entityimage_url = entityimage_url;
_x.overriddencreatedon = overriddencreatedon;
_x._rollupqueryactualintegerid_value = _rollupqueryactualintegerid_value;
_x.fiscalperiod = fiscalperiod;
_x._modifiedby_value = _modifiedby_value;
_x.isoverridden = isoverridden;
_x.title = title;
_x.goalenddate = goalenddate;
_x._owningteam_value = _owningteam_value;
_x._rollupqueryactualdecimalid_value = _rollupqueryactualdecimalid_value;
_x._rollupqueryactualmoneyid_value = _rollupqueryactualmoneyid_value;
_x.exchangerate = exchangerate;
_x.inprogressdecimal = inprogressdecimal;
_x.consideronlygoalownersrecords = consideronlygoalownersrecords;
_x.computedtargetasoftodayinteger = computedtargetasoftodayinteger;
_x.goalid = goalid;
_x.entityimageid = entityimageid;
_x.targetdecimal = targetdecimal;
_x.computedtargetasoftodaymoney = computedtargetasoftodaymoney;
_x.isamount = isamount;
_x.modifiedon = modifiedon;
_x.actualinteger = actualinteger;
_x.customrollupfieldmoney = customrollupfieldmoney;
_x.entityimage = entityimage;
_x.versionnumber = versionnumber;
_x.entityimage_timestamp = entityimage_timestamp;
_x._ownerid_value = _ownerid_value;
_x.percentage = percentage;
_x.actualstring = actualstring;
_x.actualmoney = actualmoney;
_x.stretchtargetinteger = stretchtargetinteger;
_x.lastrolledupdate = lastrolledupdate;
_x.actualmoney_base = actualmoney_base;
_x.computedtargetasoftodaymoney_base = computedtargetasoftodaymoney_base;
_x.customrollupfieldmoney_base = customrollupfieldmoney_base;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.targetinteger = targetinteger;
_x.inprogressstring = inprogressstring;
_x.goalstartdate = goalstartdate;
_x.inprogressmoney_base = inprogressmoney_base;
_x.targetstring = targetstring;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.statuscode = statuscode;
_x.isoverride = isoverride;
_x._goalownerid_value = _goalownerid_value;
_x._rollupquerycustommoneyid_value = _rollupquerycustommoneyid_value;
_x._owninguser_value = _owninguser_value;
_x.targetmoney = targetmoney;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x._rollupqueryinprogressdecimalid_value = _rollupqueryinprogressdecimalid_value;
_x.statecode = statecode;
_x.stretchtargetmoney = stretchtargetmoney;
_x.importsequencenumber = importsequencenumber;
_x.treeid = treeid;
_x._goalwitherrorid_value = _goalwitherrorid_value;
_x.rolluponlyfromchildgoals = rolluponlyfromchildgoals;
_x.isfiscalperiodgoal = isfiscalperiodgoal;
_x.actualdecimal = actualdecimal;
_x.customrollupfieldstring = customrollupfieldstring;
_x._rollupqueryinprogressmoneyid_value = _rollupqueryinprogressmoneyid_value;
_x._rollupquerycustomdecimalid_value = _rollupquerycustomdecimalid_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x._rollupquerycustomintegerid_value = _rollupquerycustomintegerid_value;
_x.stretchtargetmoney_base = stretchtargetmoney_base;
_x._metricid_value = _metricid_value;
_x.computedtargetasoftodaydecimal = computedtargetasoftodaydecimal;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.customrollupfielddecimal = customrollupfielddecimal;
_x.fiscalyear = fiscalyear;
_x.stretchtargetdecimal = stretchtargetdecimal;
_x.targetmoney_base = targetmoney_base;
_x.inprogressmoney = inprogressmoney;
_x.depth = depth;
_x.rolluperrorcode = rolluperrorcode;
_x._parentgoalid_value = _parentgoalid_value;
_x.customrollupfieldinteger = customrollupfieldinteger;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && goalid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(goalid, UUID.class));
}
}
@Property(name="computedtargetasoftodaypercentageachieved")
@JsonIgnore
public Optional getComputedtargetasoftodaypercentageachieved() {
return Optional.ofNullable(computedtargetasoftodaypercentageachieved);
}
public Goal withComputedtargetasoftodaypercentageachieved(BigDecimal computedtargetasoftodaypercentageachieved) {
Goal _x = _copy();
_x.changedFields = changedFields.add("computedtargetasoftodaypercentageachieved");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.computedtargetasoftodaypercentageachieved = computedtargetasoftodaypercentageachieved;
return _x;
}
@Property(name="stretchtargetstring")
@JsonIgnore
public Optional getStretchtargetstring() {
return Optional.ofNullable(stretchtargetstring);
}
public Goal withStretchtargetstring(String stretchtargetstring) {
Goal _x = _copy();
_x.changedFields = changedFields.add("stretchtargetstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.stretchtargetstring = stretchtargetstring;
return _x;
}
@Property(name="amountdatatype")
@JsonIgnore
public Optional getAmountdatatype() {
return Optional.ofNullable(amountdatatype);
}
public Goal withAmountdatatype(Integer amountdatatype) {
Goal _x = _copy();
_x.changedFields = changedFields.add("amountdatatype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.amountdatatype = amountdatatype;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Goal with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Goal withCreatedon(OffsetDateTime createdon) {
Goal _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.createdon = createdon;
return _x;
}
@Property(name="_rollupqueryinprogressintegerid_value")
@JsonIgnore
public Optional get_rollupqueryinprogressintegerid_value() {
return Optional.ofNullable(_rollupqueryinprogressintegerid_value);
}
public Goal with_rollupqueryinprogressintegerid_value(UUID _rollupqueryinprogressintegerid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryinprogressintegerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryinprogressintegerid_value = _rollupqueryinprogressintegerid_value;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Goal with_createdby_value(UUID _createdby_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="inprogressinteger")
@JsonIgnore
public Optional getInprogressinteger() {
return Optional.ofNullable(inprogressinteger);
}
public Goal withInprogressinteger(Integer inprogressinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("inprogressinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.inprogressinteger = inprogressinteger;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Goal withEntityimage_url(String entityimage_url) {
Goal _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Goal withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Goal _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="_rollupqueryactualintegerid_value")
@JsonIgnore
public Optional get_rollupqueryactualintegerid_value() {
return Optional.ofNullable(_rollupqueryactualintegerid_value);
}
public Goal with_rollupqueryactualintegerid_value(UUID _rollupqueryactualintegerid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryactualintegerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryactualintegerid_value = _rollupqueryactualintegerid_value;
return _x;
}
@Property(name="fiscalperiod")
@JsonIgnore
public Optional getFiscalperiod() {
return Optional.ofNullable(fiscalperiod);
}
public Goal withFiscalperiod(Integer fiscalperiod) {
Goal _x = _copy();
_x.changedFields = changedFields.add("fiscalperiod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.fiscalperiod = fiscalperiod;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Goal with_modifiedby_value(UUID _modifiedby_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="isoverridden")
@JsonIgnore
public Optional getIsoverridden() {
return Optional.ofNullable(isoverridden);
}
public Goal withIsoverridden(Boolean isoverridden) {
Goal _x = _copy();
_x.changedFields = changedFields.add("isoverridden");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.isoverridden = isoverridden;
return _x;
}
@Property(name="title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public Goal withTitle(String title) {
Goal _x = _copy();
_x.changedFields = changedFields.add("title");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.title = title;
return _x;
}
@Property(name="goalenddate")
@JsonIgnore
public Optional getGoalenddate() {
return Optional.ofNullable(goalenddate);
}
public Goal withGoalenddate(OffsetDateTime goalenddate) {
Goal _x = _copy();
_x.changedFields = changedFields.add("goalenddate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.goalenddate = goalenddate;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Goal with_owningteam_value(UUID _owningteam_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="_rollupqueryactualdecimalid_value")
@JsonIgnore
public Optional get_rollupqueryactualdecimalid_value() {
return Optional.ofNullable(_rollupqueryactualdecimalid_value);
}
public Goal with_rollupqueryactualdecimalid_value(UUID _rollupqueryactualdecimalid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryactualdecimalid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryactualdecimalid_value = _rollupqueryactualdecimalid_value;
return _x;
}
@Property(name="_rollupqueryactualmoneyid_value")
@JsonIgnore
public Optional get_rollupqueryactualmoneyid_value() {
return Optional.ofNullable(_rollupqueryactualmoneyid_value);
}
public Goal with_rollupqueryactualmoneyid_value(UUID _rollupqueryactualmoneyid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryactualmoneyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryactualmoneyid_value = _rollupqueryactualmoneyid_value;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Goal withExchangerate(BigDecimal exchangerate) {
Goal _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="inprogressdecimal")
@JsonIgnore
public Optional getInprogressdecimal() {
return Optional.ofNullable(inprogressdecimal);
}
public Goal withInprogressdecimal(BigDecimal inprogressdecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("inprogressdecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.inprogressdecimal = inprogressdecimal;
return _x;
}
@Property(name="consideronlygoalownersrecords")
@JsonIgnore
public Optional getConsideronlygoalownersrecords() {
return Optional.ofNullable(consideronlygoalownersrecords);
}
public Goal withConsideronlygoalownersrecords(Boolean consideronlygoalownersrecords) {
Goal _x = _copy();
_x.changedFields = changedFields.add("consideronlygoalownersrecords");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.consideronlygoalownersrecords = consideronlygoalownersrecords;
return _x;
}
@Property(name="computedtargetasoftodayinteger")
@JsonIgnore
public Optional getComputedtargetasoftodayinteger() {
return Optional.ofNullable(computedtargetasoftodayinteger);
}
public Goal withComputedtargetasoftodayinteger(Integer computedtargetasoftodayinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("computedtargetasoftodayinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.computedtargetasoftodayinteger = computedtargetasoftodayinteger;
return _x;
}
@Property(name="goalid")
@JsonIgnore
public Optional getGoalid() {
return Optional.ofNullable(goalid);
}
public Goal withGoalid(UUID goalid) {
Goal _x = _copy();
_x.changedFields = changedFields.add("goalid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.goalid = goalid;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Goal withEntityimageid(UUID entityimageid) {
Goal _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="targetdecimal")
@JsonIgnore
public Optional getTargetdecimal() {
return Optional.ofNullable(targetdecimal);
}
public Goal withTargetdecimal(BigDecimal targetdecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("targetdecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.targetdecimal = targetdecimal;
return _x;
}
@Property(name="computedtargetasoftodaymoney")
@JsonIgnore
public Optional getComputedtargetasoftodaymoney() {
return Optional.ofNullable(computedtargetasoftodaymoney);
}
public Goal withComputedtargetasoftodaymoney(BigDecimal computedtargetasoftodaymoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("computedtargetasoftodaymoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.computedtargetasoftodaymoney = computedtargetasoftodaymoney;
return _x;
}
@Property(name="isamount")
@JsonIgnore
public Optional getIsamount() {
return Optional.ofNullable(isamount);
}
public Goal withIsamount(Boolean isamount) {
Goal _x = _copy();
_x.changedFields = changedFields.add("isamount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.isamount = isamount;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Goal withModifiedon(OffsetDateTime modifiedon) {
Goal _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="actualinteger")
@JsonIgnore
public Optional getActualinteger() {
return Optional.ofNullable(actualinteger);
}
public Goal withActualinteger(Integer actualinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("actualinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.actualinteger = actualinteger;
return _x;
}
@Property(name="customrollupfieldmoney")
@JsonIgnore
public Optional getCustomrollupfieldmoney() {
return Optional.ofNullable(customrollupfieldmoney);
}
public Goal withCustomrollupfieldmoney(BigDecimal customrollupfieldmoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("customrollupfieldmoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.customrollupfieldmoney = customrollupfieldmoney;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Goal withEntityimage(byte[] entityimage) {
Goal _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.entityimage = entityimage;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Goal withVersionnumber(Long versionnumber) {
Goal _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Goal withEntityimage_timestamp(Long entityimage_timestamp) {
Goal _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Goal with_ownerid_value(UUID _ownerid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="percentage")
@JsonIgnore
public Optional getPercentage() {
return Optional.ofNullable(percentage);
}
public Goal withPercentage(BigDecimal percentage) {
Goal _x = _copy();
_x.changedFields = changedFields.add("percentage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.percentage = percentage;
return _x;
}
@Property(name="actualstring")
@JsonIgnore
public Optional getActualstring() {
return Optional.ofNullable(actualstring);
}
public Goal withActualstring(String actualstring) {
Goal _x = _copy();
_x.changedFields = changedFields.add("actualstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.actualstring = actualstring;
return _x;
}
@Property(name="actualmoney")
@JsonIgnore
public Optional getActualmoney() {
return Optional.ofNullable(actualmoney);
}
public Goal withActualmoney(BigDecimal actualmoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("actualmoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.actualmoney = actualmoney;
return _x;
}
@Property(name="stretchtargetinteger")
@JsonIgnore
public Optional getStretchtargetinteger() {
return Optional.ofNullable(stretchtargetinteger);
}
public Goal withStretchtargetinteger(Integer stretchtargetinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("stretchtargetinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.stretchtargetinteger = stretchtargetinteger;
return _x;
}
@Property(name="lastrolledupdate")
@JsonIgnore
public Optional getLastrolledupdate() {
return Optional.ofNullable(lastrolledupdate);
}
public Goal withLastrolledupdate(OffsetDateTime lastrolledupdate) {
Goal _x = _copy();
_x.changedFields = changedFields.add("lastrolledupdate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.lastrolledupdate = lastrolledupdate;
return _x;
}
@Property(name="actualmoney_base")
@JsonIgnore
public Optional getActualmoney_base() {
return Optional.ofNullable(actualmoney_base);
}
public Goal withActualmoney_base(BigDecimal actualmoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("actualmoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.actualmoney_base = actualmoney_base;
return _x;
}
@Property(name="computedtargetasoftodaymoney_base")
@JsonIgnore
public Optional getComputedtargetasoftodaymoney_base() {
return Optional.ofNullable(computedtargetasoftodaymoney_base);
}
public Goal withComputedtargetasoftodaymoney_base(BigDecimal computedtargetasoftodaymoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("computedtargetasoftodaymoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.computedtargetasoftodaymoney_base = computedtargetasoftodaymoney_base;
return _x;
}
@Property(name="customrollupfieldmoney_base")
@JsonIgnore
public Optional getCustomrollupfieldmoney_base() {
return Optional.ofNullable(customrollupfieldmoney_base);
}
public Goal withCustomrollupfieldmoney_base(BigDecimal customrollupfieldmoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("customrollupfieldmoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.customrollupfieldmoney_base = customrollupfieldmoney_base;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Goal with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="targetinteger")
@JsonIgnore
public Optional getTargetinteger() {
return Optional.ofNullable(targetinteger);
}
public Goal withTargetinteger(Integer targetinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("targetinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.targetinteger = targetinteger;
return _x;
}
@Property(name="inprogressstring")
@JsonIgnore
public Optional getInprogressstring() {
return Optional.ofNullable(inprogressstring);
}
public Goal withInprogressstring(String inprogressstring) {
Goal _x = _copy();
_x.changedFields = changedFields.add("inprogressstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.inprogressstring = inprogressstring;
return _x;
}
@Property(name="goalstartdate")
@JsonIgnore
public Optional getGoalstartdate() {
return Optional.ofNullable(goalstartdate);
}
public Goal withGoalstartdate(OffsetDateTime goalstartdate) {
Goal _x = _copy();
_x.changedFields = changedFields.add("goalstartdate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.goalstartdate = goalstartdate;
return _x;
}
@Property(name="inprogressmoney_base")
@JsonIgnore
public Optional getInprogressmoney_base() {
return Optional.ofNullable(inprogressmoney_base);
}
public Goal withInprogressmoney_base(BigDecimal inprogressmoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("inprogressmoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.inprogressmoney_base = inprogressmoney_base;
return _x;
}
@Property(name="targetstring")
@JsonIgnore
public Optional getTargetstring() {
return Optional.ofNullable(targetstring);
}
public Goal withTargetstring(String targetstring) {
Goal _x = _copy();
_x.changedFields = changedFields.add("targetstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.targetstring = targetstring;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Goal withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Goal _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Goal withStatuscode(Integer statuscode) {
Goal _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.statuscode = statuscode;
return _x;
}
@Property(name="isoverride")
@JsonIgnore
public Optional getIsoverride() {
return Optional.ofNullable(isoverride);
}
public Goal withIsoverride(Boolean isoverride) {
Goal _x = _copy();
_x.changedFields = changedFields.add("isoverride");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.isoverride = isoverride;
return _x;
}
@Property(name="_goalownerid_value")
@JsonIgnore
public Optional get_goalownerid_value() {
return Optional.ofNullable(_goalownerid_value);
}
public Goal with_goalownerid_value(UUID _goalownerid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_goalownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._goalownerid_value = _goalownerid_value;
return _x;
}
@Property(name="_rollupquerycustommoneyid_value")
@JsonIgnore
public Optional get_rollupquerycustommoneyid_value() {
return Optional.ofNullable(_rollupquerycustommoneyid_value);
}
public Goal with_rollupquerycustommoneyid_value(UUID _rollupquerycustommoneyid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupquerycustommoneyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupquerycustommoneyid_value = _rollupquerycustommoneyid_value;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Goal with_owninguser_value(UUID _owninguser_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="targetmoney")
@JsonIgnore
public Optional getTargetmoney() {
return Optional.ofNullable(targetmoney);
}
public Goal withTargetmoney(BigDecimal targetmoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("targetmoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.targetmoney = targetmoney;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Goal with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="_rollupqueryinprogressdecimalid_value")
@JsonIgnore
public Optional get_rollupqueryinprogressdecimalid_value() {
return Optional.ofNullable(_rollupqueryinprogressdecimalid_value);
}
public Goal with_rollupqueryinprogressdecimalid_value(UUID _rollupqueryinprogressdecimalid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryinprogressdecimalid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryinprogressdecimalid_value = _rollupqueryinprogressdecimalid_value;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Goal withStatecode(Integer statecode) {
Goal _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.statecode = statecode;
return _x;
}
@Property(name="stretchtargetmoney")
@JsonIgnore
public Optional getStretchtargetmoney() {
return Optional.ofNullable(stretchtargetmoney);
}
public Goal withStretchtargetmoney(BigDecimal stretchtargetmoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("stretchtargetmoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.stretchtargetmoney = stretchtargetmoney;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Goal withImportsequencenumber(Integer importsequencenumber) {
Goal _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="treeid")
@JsonIgnore
public Optional getTreeid() {
return Optional.ofNullable(treeid);
}
public Goal withTreeid(UUID treeid) {
Goal _x = _copy();
_x.changedFields = changedFields.add("treeid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.treeid = treeid;
return _x;
}
@Property(name="_goalwitherrorid_value")
@JsonIgnore
public Optional get_goalwitherrorid_value() {
return Optional.ofNullable(_goalwitherrorid_value);
}
public Goal with_goalwitherrorid_value(UUID _goalwitherrorid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_goalwitherrorid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._goalwitherrorid_value = _goalwitherrorid_value;
return _x;
}
@Property(name="rolluponlyfromchildgoals")
@JsonIgnore
public Optional getRolluponlyfromchildgoals() {
return Optional.ofNullable(rolluponlyfromchildgoals);
}
public Goal withRolluponlyfromchildgoals(Boolean rolluponlyfromchildgoals) {
Goal _x = _copy();
_x.changedFields = changedFields.add("rolluponlyfromchildgoals");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.rolluponlyfromchildgoals = rolluponlyfromchildgoals;
return _x;
}
@Property(name="isfiscalperiodgoal")
@JsonIgnore
public Optional getIsfiscalperiodgoal() {
return Optional.ofNullable(isfiscalperiodgoal);
}
public Goal withIsfiscalperiodgoal(Boolean isfiscalperiodgoal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("isfiscalperiodgoal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.isfiscalperiodgoal = isfiscalperiodgoal;
return _x;
}
@Property(name="actualdecimal")
@JsonIgnore
public Optional getActualdecimal() {
return Optional.ofNullable(actualdecimal);
}
public Goal withActualdecimal(BigDecimal actualdecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("actualdecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.actualdecimal = actualdecimal;
return _x;
}
@Property(name="customrollupfieldstring")
@JsonIgnore
public Optional getCustomrollupfieldstring() {
return Optional.ofNullable(customrollupfieldstring);
}
public Goal withCustomrollupfieldstring(String customrollupfieldstring) {
Goal _x = _copy();
_x.changedFields = changedFields.add("customrollupfieldstring");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.customrollupfieldstring = customrollupfieldstring;
return _x;
}
@Property(name="_rollupqueryinprogressmoneyid_value")
@JsonIgnore
public Optional get_rollupqueryinprogressmoneyid_value() {
return Optional.ofNullable(_rollupqueryinprogressmoneyid_value);
}
public Goal with_rollupqueryinprogressmoneyid_value(UUID _rollupqueryinprogressmoneyid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupqueryinprogressmoneyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupqueryinprogressmoneyid_value = _rollupqueryinprogressmoneyid_value;
return _x;
}
@Property(name="_rollupquerycustomdecimalid_value")
@JsonIgnore
public Optional get_rollupquerycustomdecimalid_value() {
return Optional.ofNullable(_rollupquerycustomdecimalid_value);
}
public Goal with_rollupquerycustomdecimalid_value(UUID _rollupquerycustomdecimalid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupquerycustomdecimalid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupquerycustomdecimalid_value = _rollupquerycustomdecimalid_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Goal withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Goal _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="_rollupquerycustomintegerid_value")
@JsonIgnore
public Optional get_rollupquerycustomintegerid_value() {
return Optional.ofNullable(_rollupquerycustomintegerid_value);
}
public Goal with_rollupquerycustomintegerid_value(UUID _rollupquerycustomintegerid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_rollupquerycustomintegerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._rollupquerycustomintegerid_value = _rollupquerycustomintegerid_value;
return _x;
}
@Property(name="stretchtargetmoney_base")
@JsonIgnore
public Optional getStretchtargetmoney_base() {
return Optional.ofNullable(stretchtargetmoney_base);
}
public Goal withStretchtargetmoney_base(BigDecimal stretchtargetmoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("stretchtargetmoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.stretchtargetmoney_base = stretchtargetmoney_base;
return _x;
}
@Property(name="_metricid_value")
@JsonIgnore
public Optional get_metricid_value() {
return Optional.ofNullable(_metricid_value);
}
public Goal with_metricid_value(UUID _metricid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_metricid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._metricid_value = _metricid_value;
return _x;
}
@Property(name="computedtargetasoftodaydecimal")
@JsonIgnore
public Optional getComputedtargetasoftodaydecimal() {
return Optional.ofNullable(computedtargetasoftodaydecimal);
}
public Goal withComputedtargetasoftodaydecimal(BigDecimal computedtargetasoftodaydecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("computedtargetasoftodaydecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.computedtargetasoftodaydecimal = computedtargetasoftodaydecimal;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Goal with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="customrollupfielddecimal")
@JsonIgnore
public Optional getCustomrollupfielddecimal() {
return Optional.ofNullable(customrollupfielddecimal);
}
public Goal withCustomrollupfielddecimal(BigDecimal customrollupfielddecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("customrollupfielddecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.customrollupfielddecimal = customrollupfielddecimal;
return _x;
}
@Property(name="fiscalyear")
@JsonIgnore
public Optional getFiscalyear() {
return Optional.ofNullable(fiscalyear);
}
public Goal withFiscalyear(Integer fiscalyear) {
Goal _x = _copy();
_x.changedFields = changedFields.add("fiscalyear");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.fiscalyear = fiscalyear;
return _x;
}
@Property(name="stretchtargetdecimal")
@JsonIgnore
public Optional getStretchtargetdecimal() {
return Optional.ofNullable(stretchtargetdecimal);
}
public Goal withStretchtargetdecimal(BigDecimal stretchtargetdecimal) {
Goal _x = _copy();
_x.changedFields = changedFields.add("stretchtargetdecimal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.stretchtargetdecimal = stretchtargetdecimal;
return _x;
}
@Property(name="targetmoney_base")
@JsonIgnore
public Optional getTargetmoney_base() {
return Optional.ofNullable(targetmoney_base);
}
public Goal withTargetmoney_base(BigDecimal targetmoney_base) {
Goal _x = _copy();
_x.changedFields = changedFields.add("targetmoney_base");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.targetmoney_base = targetmoney_base;
return _x;
}
@Property(name="inprogressmoney")
@JsonIgnore
public Optional getInprogressmoney() {
return Optional.ofNullable(inprogressmoney);
}
public Goal withInprogressmoney(BigDecimal inprogressmoney) {
Goal _x = _copy();
_x.changedFields = changedFields.add("inprogressmoney");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.inprogressmoney = inprogressmoney;
return _x;
}
@Property(name="depth")
@JsonIgnore
public Optional getDepth() {
return Optional.ofNullable(depth);
}
public Goal withDepth(Integer depth) {
Goal _x = _copy();
_x.changedFields = changedFields.add("depth");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.depth = depth;
return _x;
}
@Property(name="rolluperrorcode")
@JsonIgnore
public Optional getRolluperrorcode() {
return Optional.ofNullable(rolluperrorcode);
}
public Goal withRolluperrorcode(Integer rolluperrorcode) {
Goal _x = _copy();
_x.changedFields = changedFields.add("rolluperrorcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.rolluperrorcode = rolluperrorcode;
return _x;
}
@Property(name="_parentgoalid_value")
@JsonIgnore
public Optional get_parentgoalid_value() {
return Optional.ofNullable(_parentgoalid_value);
}
public Goal with_parentgoalid_value(UUID _parentgoalid_value) {
Goal _x = _copy();
_x.changedFields = changedFields.add("_parentgoalid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x._parentgoalid_value = _parentgoalid_value;
return _x;
}
@Property(name="customrollupfieldinteger")
@JsonIgnore
public Optional getCustomrollupfieldinteger() {
return Optional.ofNullable(customrollupfieldinteger);
}
public Goal withCustomrollupfieldinteger(Integer customrollupfieldinteger) {
Goal _x = _copy();
_x.changedFields = changedFields.add("customrollupfieldinteger");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.goal");
_x.customrollupfieldinteger = customrollupfieldinteger;
return _x;
}
public Goal withUnmappedField(String name, Object value) {
Goal _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="rollupqueryactualintegerid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryactualintegerid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryactualintegerid"), RequestHelper.getValue(unmappedFields, "rollupqueryactualintegerid"));
}
@NavigationProperty(name="rollupqueryactualmoneyid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryactualmoneyid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryactualmoneyid"), RequestHelper.getValue(unmappedFields, "rollupqueryactualmoneyid"));
}
@NavigationProperty(name="rollupqueryactualdecimalid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryactualdecimalid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryactualdecimalid"), RequestHelper.getValue(unmappedFields, "rollupqueryactualdecimalid"));
}
@NavigationProperty(name="rollupquerycustomintegerid")
@JsonIgnore
public GoalrollupqueryRequest getRollupquerycustomintegerid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupquerycustomintegerid"), RequestHelper.getValue(unmappedFields, "rollupquerycustomintegerid"));
}
@NavigationProperty(name="rollupquerycustommoneyid")
@JsonIgnore
public GoalrollupqueryRequest getRollupquerycustommoneyid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupquerycustommoneyid"), RequestHelper.getValue(unmappedFields, "rollupquerycustommoneyid"));
}
@NavigationProperty(name="rollupquerycustomdecimalid")
@JsonIgnore
public GoalrollupqueryRequest getRollupquerycustomdecimalid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupquerycustomdecimalid"), RequestHelper.getValue(unmappedFields, "rollupquerycustomdecimalid"));
}
@NavigationProperty(name="rollupqueryinprogressintegerid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryinprogressintegerid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryinprogressintegerid"), RequestHelper.getValue(unmappedFields, "rollupqueryinprogressintegerid"));
}
@NavigationProperty(name="rollupqueryinprogressmoneyid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryinprogressmoneyid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryinprogressmoneyid"), RequestHelper.getValue(unmappedFields, "rollupqueryinprogressmoneyid"));
}
@NavigationProperty(name="rollupqueryinprogressdecimalid")
@JsonIgnore
public GoalrollupqueryRequest getRollupqueryinprogressdecimalid() {
return new GoalrollupqueryRequest(contextPath.addSegment("rollupqueryinprogressdecimalid"), RequestHelper.getValue(unmappedFields, "rollupqueryinprogressdecimalid"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"), RequestHelper.getValue(unmappedFields, "owninguser"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="goalownerid_systemuser")
@JsonIgnore
public SystemuserRequest getGoalownerid_systemuser() {
return new SystemuserRequest(contextPath.addSegment("goalownerid_systemuser"), RequestHelper.getValue(unmappedFields, "goalownerid_systemuser"));
}
@NavigationProperty(name="parentgoalid")
@JsonIgnore
public GoalRequest getParentgoalid() {
return new GoalRequest(contextPath.addSegment("parentgoalid"), RequestHelper.getValue(unmappedFields, "parentgoalid"));
}
@NavigationProperty(name="goal_parent_goal")
@JsonIgnore
public GoalCollectionRequest getGoal_parent_goal() {
return new GoalCollectionRequest(
contextPath.addSegment("goal_parent_goal"), RequestHelper.getValue(unmappedFields, "goal_parent_goal"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@NavigationProperty(name="metricid")
@JsonIgnore
public MetricRequest getMetricid() {
return new MetricRequest(contextPath.addSegment("metricid"), RequestHelper.getValue(unmappedFields, "metricid"));
}
@NavigationProperty(name="Goal_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getGoal_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Goal_DuplicateMatchingRecord"), RequestHelper.getValue(unmappedFields, "Goal_DuplicateMatchingRecord"));
}
@NavigationProperty(name="goalwitherrorid")
@JsonIgnore
public GoalRequest getGoalwitherrorid() {
return new GoalRequest(contextPath.addSegment("goalwitherrorid"), RequestHelper.getValue(unmappedFields, "goalwitherrorid"));
}
@NavigationProperty(name="Goal_RollupError_Goal")
@JsonIgnore
public GoalCollectionRequest getGoal_RollupError_Goal() {
return new GoalCollectionRequest(
contextPath.addSegment("Goal_RollupError_Goal"), RequestHelper.getValue(unmappedFields, "Goal_RollupError_Goal"));
}
@NavigationProperty(name="goal_connections2")
@JsonIgnore
public ConnectionCollectionRequest getGoal_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("goal_connections2"), RequestHelper.getValue(unmappedFields, "goal_connections2"));
}
@NavigationProperty(name="Goal_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getGoal_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Goal_SyncErrors"), RequestHelper.getValue(unmappedFields, "Goal_SyncErrors"));
}
@NavigationProperty(name="Goal_Annotation")
@JsonIgnore
public AnnotationCollectionRequest getGoal_Annotation() {
return new AnnotationCollectionRequest(
contextPath.addSegment("Goal_Annotation"), RequestHelper.getValue(unmappedFields, "Goal_Annotation"));
}
@NavigationProperty(name="goal_connections1")
@JsonIgnore
public ConnectionCollectionRequest getGoal_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("goal_connections1"), RequestHelper.getValue(unmappedFields, "goal_connections1"));
}
@NavigationProperty(name="Goal_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getGoal_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Goal_AsyncOperations"), RequestHelper.getValue(unmappedFields, "Goal_AsyncOperations"));
}
@NavigationProperty(name="Goal_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getGoal_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("Goal_DuplicateBaseRecord"), RequestHelper.getValue(unmappedFields, "Goal_DuplicateBaseRecord"));
}
@NavigationProperty(name="Goal_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getGoal_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Goal_ProcessSessions"), RequestHelper.getValue(unmappedFields, "Goal_ProcessSessions"));
}
@NavigationProperty(name="goal_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getGoal_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("goal_principalobjectattributeaccess"), RequestHelper.getValue(unmappedFields, "goal_principalobjectattributeaccess"));
}
@NavigationProperty(name="goalownerid_team")
@JsonIgnore
public TeamRequest getGoalownerid_team() {
return new TeamRequest(contextPath.addSegment("goalownerid_team"), RequestHelper.getValue(unmappedFields, "goalownerid_team"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Goal patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Goal _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Goal put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Goal _x = _copy();
_x.changedFields = null;
return _x;
}
private Goal _copy() {
Goal _x = new Goal();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.computedtargetasoftodaypercentageachieved = computedtargetasoftodaypercentageachieved;
_x.stretchtargetstring = stretchtargetstring;
_x.amountdatatype = amountdatatype;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.createdon = createdon;
_x._rollupqueryinprogressintegerid_value = _rollupqueryinprogressintegerid_value;
_x._createdby_value = _createdby_value;
_x.inprogressinteger = inprogressinteger;
_x.entityimage_url = entityimage_url;
_x.overriddencreatedon = overriddencreatedon;
_x._rollupqueryactualintegerid_value = _rollupqueryactualintegerid_value;
_x.fiscalperiod = fiscalperiod;
_x._modifiedby_value = _modifiedby_value;
_x.isoverridden = isoverridden;
_x.title = title;
_x.goalenddate = goalenddate;
_x._owningteam_value = _owningteam_value;
_x._rollupqueryactualdecimalid_value = _rollupqueryactualdecimalid_value;
_x._rollupqueryactualmoneyid_value = _rollupqueryactualmoneyid_value;
_x.exchangerate = exchangerate;
_x.inprogressdecimal = inprogressdecimal;
_x.consideronlygoalownersrecords = consideronlygoalownersrecords;
_x.computedtargetasoftodayinteger = computedtargetasoftodayinteger;
_x.goalid = goalid;
_x.entityimageid = entityimageid;
_x.targetdecimal = targetdecimal;
_x.computedtargetasoftodaymoney = computedtargetasoftodaymoney;
_x.isamount = isamount;
_x.modifiedon = modifiedon;
_x.actualinteger = actualinteger;
_x.customrollupfieldmoney = customrollupfieldmoney;
_x.entityimage = entityimage;
_x.versionnumber = versionnumber;
_x.entityimage_timestamp = entityimage_timestamp;
_x._ownerid_value = _ownerid_value;
_x.percentage = percentage;
_x.actualstring = actualstring;
_x.actualmoney = actualmoney;
_x.stretchtargetinteger = stretchtargetinteger;
_x.lastrolledupdate = lastrolledupdate;
_x.actualmoney_base = actualmoney_base;
_x.computedtargetasoftodaymoney_base = computedtargetasoftodaymoney_base;
_x.customrollupfieldmoney_base = customrollupfieldmoney_base;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.targetinteger = targetinteger;
_x.inprogressstring = inprogressstring;
_x.goalstartdate = goalstartdate;
_x.inprogressmoney_base = inprogressmoney_base;
_x.targetstring = targetstring;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.statuscode = statuscode;
_x.isoverride = isoverride;
_x._goalownerid_value = _goalownerid_value;
_x._rollupquerycustommoneyid_value = _rollupquerycustommoneyid_value;
_x._owninguser_value = _owninguser_value;
_x.targetmoney = targetmoney;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x._rollupqueryinprogressdecimalid_value = _rollupqueryinprogressdecimalid_value;
_x.statecode = statecode;
_x.stretchtargetmoney = stretchtargetmoney;
_x.importsequencenumber = importsequencenumber;
_x.treeid = treeid;
_x._goalwitherrorid_value = _goalwitherrorid_value;
_x.rolluponlyfromchildgoals = rolluponlyfromchildgoals;
_x.isfiscalperiodgoal = isfiscalperiodgoal;
_x.actualdecimal = actualdecimal;
_x.customrollupfieldstring = customrollupfieldstring;
_x._rollupqueryinprogressmoneyid_value = _rollupqueryinprogressmoneyid_value;
_x._rollupquerycustomdecimalid_value = _rollupquerycustomdecimalid_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x._rollupquerycustomintegerid_value = _rollupquerycustomintegerid_value;
_x.stretchtargetmoney_base = stretchtargetmoney_base;
_x._metricid_value = _metricid_value;
_x.computedtargetasoftodaydecimal = computedtargetasoftodaydecimal;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.customrollupfielddecimal = customrollupfielddecimal;
_x.fiscalyear = fiscalyear;
_x.stretchtargetdecimal = stretchtargetdecimal;
_x.targetmoney_base = targetmoney_base;
_x.inprogressmoney = inprogressmoney;
_x.depth = depth;
_x.rolluperrorcode = rolluperrorcode;
_x._parentgoalid_value = _parentgoalid_value;
_x.customrollupfieldinteger = customrollupfieldinteger;
return _x;
}
@Action(name = "Recalculate")
@JsonIgnore
public ActionRequestNoReturn recalculate() {
Map _parameters = ParameterMap.empty();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.Recalculate"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Goal[");
b.append("computedtargetasoftodaypercentageachieved=");
b.append(this.computedtargetasoftodaypercentageachieved);
b.append(", ");
b.append("stretchtargetstring=");
b.append(this.stretchtargetstring);
b.append(", ");
b.append("amountdatatype=");
b.append(this.amountdatatype);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("_rollupqueryinprogressintegerid_value=");
b.append(this._rollupqueryinprogressintegerid_value);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("inprogressinteger=");
b.append(this.inprogressinteger);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("_rollupqueryactualintegerid_value=");
b.append(this._rollupqueryactualintegerid_value);
b.append(", ");
b.append("fiscalperiod=");
b.append(this.fiscalperiod);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("isoverridden=");
b.append(this.isoverridden);
b.append(", ");
b.append("title=");
b.append(this.title);
b.append(", ");
b.append("goalenddate=");
b.append(this.goalenddate);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("_rollupqueryactualdecimalid_value=");
b.append(this._rollupqueryactualdecimalid_value);
b.append(", ");
b.append("_rollupqueryactualmoneyid_value=");
b.append(this._rollupqueryactualmoneyid_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("inprogressdecimal=");
b.append(this.inprogressdecimal);
b.append(", ");
b.append("consideronlygoalownersrecords=");
b.append(this.consideronlygoalownersrecords);
b.append(", ");
b.append("computedtargetasoftodayinteger=");
b.append(this.computedtargetasoftodayinteger);
b.append(", ");
b.append("goalid=");
b.append(this.goalid);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("targetdecimal=");
b.append(this.targetdecimal);
b.append(", ");
b.append("computedtargetasoftodaymoney=");
b.append(this.computedtargetasoftodaymoney);
b.append(", ");
b.append("isamount=");
b.append(this.isamount);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("actualinteger=");
b.append(this.actualinteger);
b.append(", ");
b.append("customrollupfieldmoney=");
b.append(this.customrollupfieldmoney);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("percentage=");
b.append(this.percentage);
b.append(", ");
b.append("actualstring=");
b.append(this.actualstring);
b.append(", ");
b.append("actualmoney=");
b.append(this.actualmoney);
b.append(", ");
b.append("stretchtargetinteger=");
b.append(this.stretchtargetinteger);
b.append(", ");
b.append("lastrolledupdate=");
b.append(this.lastrolledupdate);
b.append(", ");
b.append("actualmoney_base=");
b.append(this.actualmoney_base);
b.append(", ");
b.append("computedtargetasoftodaymoney_base=");
b.append(this.computedtargetasoftodaymoney_base);
b.append(", ");
b.append("customrollupfieldmoney_base=");
b.append(this.customrollupfieldmoney_base);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("targetinteger=");
b.append(this.targetinteger);
b.append(", ");
b.append("inprogressstring=");
b.append(this.inprogressstring);
b.append(", ");
b.append("goalstartdate=");
b.append(this.goalstartdate);
b.append(", ");
b.append("inprogressmoney_base=");
b.append(this.inprogressmoney_base);
b.append(", ");
b.append("targetstring=");
b.append(this.targetstring);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("isoverride=");
b.append(this.isoverride);
b.append(", ");
b.append("_goalownerid_value=");
b.append(this._goalownerid_value);
b.append(", ");
b.append("_rollupquerycustommoneyid_value=");
b.append(this._rollupquerycustommoneyid_value);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("targetmoney=");
b.append(this.targetmoney);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("_rollupqueryinprogressdecimalid_value=");
b.append(this._rollupqueryinprogressdecimalid_value);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("stretchtargetmoney=");
b.append(this.stretchtargetmoney);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("treeid=");
b.append(this.treeid);
b.append(", ");
b.append("_goalwitherrorid_value=");
b.append(this._goalwitherrorid_value);
b.append(", ");
b.append("rolluponlyfromchildgoals=");
b.append(this.rolluponlyfromchildgoals);
b.append(", ");
b.append("isfiscalperiodgoal=");
b.append(this.isfiscalperiodgoal);
b.append(", ");
b.append("actualdecimal=");
b.append(this.actualdecimal);
b.append(", ");
b.append("customrollupfieldstring=");
b.append(this.customrollupfieldstring);
b.append(", ");
b.append("_rollupqueryinprogressmoneyid_value=");
b.append(this._rollupqueryinprogressmoneyid_value);
b.append(", ");
b.append("_rollupquerycustomdecimalid_value=");
b.append(this._rollupquerycustomdecimalid_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("_rollupquerycustomintegerid_value=");
b.append(this._rollupquerycustomintegerid_value);
b.append(", ");
b.append("stretchtargetmoney_base=");
b.append(this.stretchtargetmoney_base);
b.append(", ");
b.append("_metricid_value=");
b.append(this._metricid_value);
b.append(", ");
b.append("computedtargetasoftodaydecimal=");
b.append(this.computedtargetasoftodaydecimal);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("customrollupfielddecimal=");
b.append(this.customrollupfielddecimal);
b.append(", ");
b.append("fiscalyear=");
b.append(this.fiscalyear);
b.append(", ");
b.append("stretchtargetdecimal=");
b.append(this.stretchtargetdecimal);
b.append(", ");
b.append("targetmoney_base=");
b.append(this.targetmoney_base);
b.append(", ");
b.append("inprogressmoney=");
b.append(this.inprogressmoney);
b.append(", ");
b.append("depth=");
b.append(this.depth);
b.append(", ");
b.append("rolluperrorcode=");
b.append(this.rolluperrorcode);
b.append(", ");
b.append("_parentgoalid_value=");
b.append(this._parentgoalid_value);
b.append(", ");
b.append("customrollupfieldinteger=");
b.append(this.customrollupfieldinteger);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy