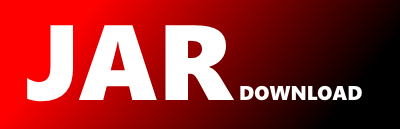
microsoft.dynamics.crm.entity.Invaliddependency Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import java.util.UUID;
@JsonPropertyOrder({
"@odata.type",
"existingcomponenttype",
"isexistingnoderequiredcomponent",
"missingcomponentlookuptype",
"invaliddependencyid",
"missingcomponentid",
"existingdependencytype",
"missingcomponentinfo",
"missingcomponenttype",
"existingcomponentid"})
@JsonInclude(Include.NON_NULL)
public class Invaliddependency extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.invaliddependency";
}
@JsonProperty("existingcomponenttype")
protected Integer existingcomponenttype;
@JsonProperty("isexistingnoderequiredcomponent")
protected Boolean isexistingnoderequiredcomponent;
@JsonProperty("missingcomponentlookuptype")
protected Integer missingcomponentlookuptype;
@JsonProperty("invaliddependencyid")
protected UUID invaliddependencyid;
@JsonProperty("missingcomponentid")
protected UUID missingcomponentid;
@JsonProperty("existingdependencytype")
protected Integer existingdependencytype;
@JsonProperty("missingcomponentinfo")
protected String missingcomponentinfo;
@JsonProperty("missingcomponenttype")
protected Integer missingcomponenttype;
@JsonProperty("existingcomponentid")
protected UUID existingcomponentid;
protected Invaliddependency() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderInvaliddependency() {
return new Builder();
}
public static final class Builder {
private Integer existingcomponenttype;
private Boolean isexistingnoderequiredcomponent;
private Integer missingcomponentlookuptype;
private UUID invaliddependencyid;
private UUID missingcomponentid;
private Integer existingdependencytype;
private String missingcomponentinfo;
private Integer missingcomponenttype;
private UUID existingcomponentid;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder existingcomponenttype(Integer existingcomponenttype) {
this.existingcomponenttype = existingcomponenttype;
this.changedFields = changedFields.add("existingcomponenttype");
return this;
}
public Builder isexistingnoderequiredcomponent(Boolean isexistingnoderequiredcomponent) {
this.isexistingnoderequiredcomponent = isexistingnoderequiredcomponent;
this.changedFields = changedFields.add("isexistingnoderequiredcomponent");
return this;
}
public Builder missingcomponentlookuptype(Integer missingcomponentlookuptype) {
this.missingcomponentlookuptype = missingcomponentlookuptype;
this.changedFields = changedFields.add("missingcomponentlookuptype");
return this;
}
public Builder invaliddependencyid(UUID invaliddependencyid) {
this.invaliddependencyid = invaliddependencyid;
this.changedFields = changedFields.add("invaliddependencyid");
return this;
}
public Builder missingcomponentid(UUID missingcomponentid) {
this.missingcomponentid = missingcomponentid;
this.changedFields = changedFields.add("missingcomponentid");
return this;
}
public Builder existingdependencytype(Integer existingdependencytype) {
this.existingdependencytype = existingdependencytype;
this.changedFields = changedFields.add("existingdependencytype");
return this;
}
public Builder missingcomponentinfo(String missingcomponentinfo) {
this.missingcomponentinfo = missingcomponentinfo;
this.changedFields = changedFields.add("missingcomponentinfo");
return this;
}
public Builder missingcomponenttype(Integer missingcomponenttype) {
this.missingcomponenttype = missingcomponenttype;
this.changedFields = changedFields.add("missingcomponenttype");
return this;
}
public Builder existingcomponentid(UUID existingcomponentid) {
this.existingcomponentid = existingcomponentid;
this.changedFields = changedFields.add("existingcomponentid");
return this;
}
public Invaliddependency build() {
Invaliddependency _x = new Invaliddependency();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.invaliddependency";
_x.existingcomponenttype = existingcomponenttype;
_x.isexistingnoderequiredcomponent = isexistingnoderequiredcomponent;
_x.missingcomponentlookuptype = missingcomponentlookuptype;
_x.invaliddependencyid = invaliddependencyid;
_x.missingcomponentid = missingcomponentid;
_x.existingdependencytype = existingdependencytype;
_x.missingcomponentinfo = missingcomponentinfo;
_x.missingcomponenttype = missingcomponenttype;
_x.existingcomponentid = existingcomponentid;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && invaliddependencyid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(invaliddependencyid, UUID.class));
}
}
@Property(name="existingcomponenttype")
@JsonIgnore
public Optional getExistingcomponenttype() {
return Optional.ofNullable(existingcomponenttype);
}
public Invaliddependency withExistingcomponenttype(Integer existingcomponenttype) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("existingcomponenttype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.existingcomponenttype = existingcomponenttype;
return _x;
}
@Property(name="isexistingnoderequiredcomponent")
@JsonIgnore
public Optional getIsexistingnoderequiredcomponent() {
return Optional.ofNullable(isexistingnoderequiredcomponent);
}
public Invaliddependency withIsexistingnoderequiredcomponent(Boolean isexistingnoderequiredcomponent) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("isexistingnoderequiredcomponent");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.isexistingnoderequiredcomponent = isexistingnoderequiredcomponent;
return _x;
}
@Property(name="missingcomponentlookuptype")
@JsonIgnore
public Optional getMissingcomponentlookuptype() {
return Optional.ofNullable(missingcomponentlookuptype);
}
public Invaliddependency withMissingcomponentlookuptype(Integer missingcomponentlookuptype) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("missingcomponentlookuptype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.missingcomponentlookuptype = missingcomponentlookuptype;
return _x;
}
@Property(name="invaliddependencyid")
@JsonIgnore
public Optional getInvaliddependencyid() {
return Optional.ofNullable(invaliddependencyid);
}
public Invaliddependency withInvaliddependencyid(UUID invaliddependencyid) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("invaliddependencyid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.invaliddependencyid = invaliddependencyid;
return _x;
}
@Property(name="missingcomponentid")
@JsonIgnore
public Optional getMissingcomponentid() {
return Optional.ofNullable(missingcomponentid);
}
public Invaliddependency withMissingcomponentid(UUID missingcomponentid) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("missingcomponentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.missingcomponentid = missingcomponentid;
return _x;
}
@Property(name="existingdependencytype")
@JsonIgnore
public Optional getExistingdependencytype() {
return Optional.ofNullable(existingdependencytype);
}
public Invaliddependency withExistingdependencytype(Integer existingdependencytype) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("existingdependencytype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.existingdependencytype = existingdependencytype;
return _x;
}
@Property(name="missingcomponentinfo")
@JsonIgnore
public Optional getMissingcomponentinfo() {
return Optional.ofNullable(missingcomponentinfo);
}
public Invaliddependency withMissingcomponentinfo(String missingcomponentinfo) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("missingcomponentinfo");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.missingcomponentinfo = missingcomponentinfo;
return _x;
}
@Property(name="missingcomponenttype")
@JsonIgnore
public Optional getMissingcomponenttype() {
return Optional.ofNullable(missingcomponenttype);
}
public Invaliddependency withMissingcomponenttype(Integer missingcomponenttype) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("missingcomponenttype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.missingcomponenttype = missingcomponenttype;
return _x;
}
@Property(name="existingcomponentid")
@JsonIgnore
public Optional getExistingcomponentid() {
return Optional.ofNullable(existingcomponentid);
}
public Invaliddependency withExistingcomponentid(UUID existingcomponentid) {
Invaliddependency _x = _copy();
_x.changedFields = changedFields.add("existingcomponentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.invaliddependency");
_x.existingcomponentid = existingcomponentid;
return _x;
}
public Invaliddependency withUnmappedField(String name, Object value) {
Invaliddependency _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Invaliddependency patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Invaliddependency _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Invaliddependency put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Invaliddependency _x = _copy();
_x.changedFields = null;
return _x;
}
private Invaliddependency _copy() {
Invaliddependency _x = new Invaliddependency();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.existingcomponenttype = existingcomponenttype;
_x.isexistingnoderequiredcomponent = isexistingnoderequiredcomponent;
_x.missingcomponentlookuptype = missingcomponentlookuptype;
_x.invaliddependencyid = invaliddependencyid;
_x.missingcomponentid = missingcomponentid;
_x.existingdependencytype = existingdependencytype;
_x.missingcomponentinfo = missingcomponentinfo;
_x.missingcomponenttype = missingcomponenttype;
_x.existingcomponentid = existingcomponentid;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Invaliddependency[");
b.append("existingcomponenttype=");
b.append(this.existingcomponenttype);
b.append(", ");
b.append("isexistingnoderequiredcomponent=");
b.append(this.isexistingnoderequiredcomponent);
b.append(", ");
b.append("missingcomponentlookuptype=");
b.append(this.missingcomponentlookuptype);
b.append(", ");
b.append("invaliddependencyid=");
b.append(this.invaliddependencyid);
b.append(", ");
b.append("missingcomponentid=");
b.append(this.missingcomponentid);
b.append(", ");
b.append("existingdependencytype=");
b.append(this.existingdependencytype);
b.append(", ");
b.append("missingcomponentinfo=");
b.append(this.missingcomponentinfo);
b.append(", ");
b.append("missingcomponenttype=");
b.append(this.missingcomponenttype);
b.append(", ");
b.append("existingcomponentid=");
b.append(this.existingcomponentid);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy