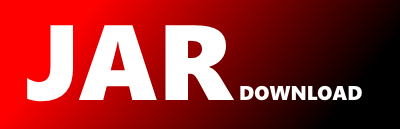
microsoft.dynamics.crm.entity.Knowledgearticle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypointerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppointmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CategoryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExpiredprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FaxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FeedbackCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KnowledgearticleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KnowledgearticleviewsCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LetterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.NewprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PhonecallCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostfollowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostregardingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurringappointmentmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SharepointdocumentlocationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialactivityCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TaskCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TeamCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TranslationprocessCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.KnowledgearticleRequest;
import microsoft.dynamics.crm.entity.request.LanguagelocaleRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.SubjectRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"_owninguser_value",
"_languagelocaleid_value",
"publishstatusid",
"expiredreviewoptions",
"_modifiedby_value",
"knowledgearticleviews_date",
"importsequencenumber",
"description",
"title",
"versionnumber",
"content",
"_modifiedonbehalfby_value",
"rating_date",
"rating_state",
"overriddencreatedon",
"_parentarticlecontentid_value",
"knowledgearticleviews",
"_owningbusinessunit_value",
"timezoneruleversionnumber",
"keywords",
"isprimary",
"_primaryauthorid_value",
"_ownerid_value",
"_owningteam_value",
"exchangerate",
"updatecontent",
"expirationstateid",
"modifiedon",
"knowledgearticleviews_state",
"knowledgearticleid",
"rating_sum",
"createdon",
"stageid",
"expirationstatusid",
"statecode",
"isrootarticle",
"utcconversiontimezonecode",
"rating_count",
"_subjectid_value",
"islatestversion",
"publishon",
"processid",
"scheduledstatusid",
"_previousarticlecontentid_value",
"minorversionnumber",
"_createdonbehalfby_value",
"majorversionnumber",
"articlepublicnumber",
"traversedpath",
"statuscode",
"expirationdate",
"_rootarticleid_value",
"rating",
"_createdby_value",
"_transactioncurrencyid_value",
"readyforreview",
"review",
"isinternal",
"setcategoryassociations"})
@JsonInclude(Include.NON_NULL)
public class Knowledgearticle extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.knowledgearticle";
}
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("_languagelocaleid_value")
protected UUID _languagelocaleid_value;
@JsonProperty("publishstatusid")
protected Integer publishstatusid;
@JsonProperty("expiredreviewoptions")
protected Integer expiredreviewoptions;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("knowledgearticleviews_date")
protected OffsetDateTime knowledgearticleviews_date;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("description")
protected String description;
@JsonProperty("title")
protected String title;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("content")
protected String content;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("rating_date")
protected OffsetDateTime rating_date;
@JsonProperty("rating_state")
protected Integer rating_state;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("_parentarticlecontentid_value")
protected UUID _parentarticlecontentid_value;
@JsonProperty("knowledgearticleviews")
protected Integer knowledgearticleviews;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("keywords")
protected String keywords;
@JsonProperty("isprimary")
protected Boolean isprimary;
@JsonProperty("_primaryauthorid_value")
protected UUID _primaryauthorid_value;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("updatecontent")
protected Boolean updatecontent;
@JsonProperty("expirationstateid")
protected Integer expirationstateid;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("knowledgearticleviews_state")
protected Integer knowledgearticleviews_state;
@JsonProperty("knowledgearticleid")
protected UUID knowledgearticleid;
@JsonProperty("rating_sum")
protected BigDecimal rating_sum;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("stageid")
protected UUID stageid;
@JsonProperty("expirationstatusid")
protected Integer expirationstatusid;
@JsonProperty("statecode")
protected Integer statecode;
@JsonProperty("isrootarticle")
protected Boolean isrootarticle;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("rating_count")
protected Integer rating_count;
@JsonProperty("_subjectid_value")
protected UUID _subjectid_value;
@JsonProperty("islatestversion")
protected Boolean islatestversion;
@JsonProperty("publishon")
protected OffsetDateTime publishon;
@JsonProperty("processid")
protected UUID processid;
@JsonProperty("scheduledstatusid")
protected Integer scheduledstatusid;
@JsonProperty("_previousarticlecontentid_value")
protected UUID _previousarticlecontentid_value;
@JsonProperty("minorversionnumber")
protected Integer minorversionnumber;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("majorversionnumber")
protected Integer majorversionnumber;
@JsonProperty("articlepublicnumber")
protected String articlepublicnumber;
@JsonProperty("traversedpath")
protected String traversedpath;
@JsonProperty("statuscode")
protected Integer statuscode;
@JsonProperty("expirationdate")
protected OffsetDateTime expirationdate;
@JsonProperty("_rootarticleid_value")
protected UUID _rootarticleid_value;
@JsonProperty("rating")
protected BigDecimal rating;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("readyforreview")
protected Boolean readyforreview;
@JsonProperty("review")
protected Integer review;
@JsonProperty("isinternal")
protected Boolean isinternal;
@JsonProperty("setcategoryassociations")
protected Boolean setcategoryassociations;
protected Knowledgearticle() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderKnowledgearticle() {
return new Builder();
}
public static final class Builder {
private UUID _owninguser_value;
private UUID _languagelocaleid_value;
private Integer publishstatusid;
private Integer expiredreviewoptions;
private UUID _modifiedby_value;
private OffsetDateTime knowledgearticleviews_date;
private Integer importsequencenumber;
private String description;
private String title;
private Long versionnumber;
private String content;
private UUID _modifiedonbehalfby_value;
private OffsetDateTime rating_date;
private Integer rating_state;
private OffsetDateTime overriddencreatedon;
private UUID _parentarticlecontentid_value;
private Integer knowledgearticleviews;
private UUID _owningbusinessunit_value;
private Integer timezoneruleversionnumber;
private String keywords;
private Boolean isprimary;
private UUID _primaryauthorid_value;
private UUID _ownerid_value;
private UUID _owningteam_value;
private BigDecimal exchangerate;
private Boolean updatecontent;
private Integer expirationstateid;
private OffsetDateTime modifiedon;
private Integer knowledgearticleviews_state;
private UUID knowledgearticleid;
private BigDecimal rating_sum;
private OffsetDateTime createdon;
private UUID stageid;
private Integer expirationstatusid;
private Integer statecode;
private Boolean isrootarticle;
private Integer utcconversiontimezonecode;
private Integer rating_count;
private UUID _subjectid_value;
private Boolean islatestversion;
private OffsetDateTime publishon;
private UUID processid;
private Integer scheduledstatusid;
private UUID _previousarticlecontentid_value;
private Integer minorversionnumber;
private UUID _createdonbehalfby_value;
private Integer majorversionnumber;
private String articlepublicnumber;
private String traversedpath;
private Integer statuscode;
private OffsetDateTime expirationdate;
private UUID _rootarticleid_value;
private BigDecimal rating;
private UUID _createdby_value;
private UUID _transactioncurrencyid_value;
private Boolean readyforreview;
private Integer review;
private Boolean isinternal;
private Boolean setcategoryassociations;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder _owninguser_value(UUID _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder _languagelocaleid_value(UUID _languagelocaleid_value) {
this._languagelocaleid_value = _languagelocaleid_value;
this.changedFields = changedFields.add("_languagelocaleid_value");
return this;
}
public Builder publishstatusid(Integer publishstatusid) {
this.publishstatusid = publishstatusid;
this.changedFields = changedFields.add("publishstatusid");
return this;
}
public Builder expiredreviewoptions(Integer expiredreviewoptions) {
this.expiredreviewoptions = expiredreviewoptions;
this.changedFields = changedFields.add("expiredreviewoptions");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder knowledgearticleviews_date(OffsetDateTime knowledgearticleviews_date) {
this.knowledgearticleviews_date = knowledgearticleviews_date;
this.changedFields = changedFields.add("knowledgearticleviews_date");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("title");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder content(String content) {
this.content = content;
this.changedFields = changedFields.add("content");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder rating_date(OffsetDateTime rating_date) {
this.rating_date = rating_date;
this.changedFields = changedFields.add("rating_date");
return this;
}
public Builder rating_state(Integer rating_state) {
this.rating_state = rating_state;
this.changedFields = changedFields.add("rating_state");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder _parentarticlecontentid_value(UUID _parentarticlecontentid_value) {
this._parentarticlecontentid_value = _parentarticlecontentid_value;
this.changedFields = changedFields.add("_parentarticlecontentid_value");
return this;
}
public Builder knowledgearticleviews(Integer knowledgearticleviews) {
this.knowledgearticleviews = knowledgearticleviews;
this.changedFields = changedFields.add("knowledgearticleviews");
return this;
}
public Builder _owningbusinessunit_value(UUID _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder keywords(String keywords) {
this.keywords = keywords;
this.changedFields = changedFields.add("keywords");
return this;
}
public Builder isprimary(Boolean isprimary) {
this.isprimary = isprimary;
this.changedFields = changedFields.add("isprimary");
return this;
}
public Builder _primaryauthorid_value(UUID _primaryauthorid_value) {
this._primaryauthorid_value = _primaryauthorid_value;
this.changedFields = changedFields.add("_primaryauthorid_value");
return this;
}
public Builder _ownerid_value(UUID _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder _owningteam_value(UUID _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder updatecontent(Boolean updatecontent) {
this.updatecontent = updatecontent;
this.changedFields = changedFields.add("updatecontent");
return this;
}
public Builder expirationstateid(Integer expirationstateid) {
this.expirationstateid = expirationstateid;
this.changedFields = changedFields.add("expirationstateid");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder knowledgearticleviews_state(Integer knowledgearticleviews_state) {
this.knowledgearticleviews_state = knowledgearticleviews_state;
this.changedFields = changedFields.add("knowledgearticleviews_state");
return this;
}
public Builder knowledgearticleid(UUID knowledgearticleid) {
this.knowledgearticleid = knowledgearticleid;
this.changedFields = changedFields.add("knowledgearticleid");
return this;
}
public Builder rating_sum(BigDecimal rating_sum) {
this.rating_sum = rating_sum;
this.changedFields = changedFields.add("rating_sum");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder stageid(UUID stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder expirationstatusid(Integer expirationstatusid) {
this.expirationstatusid = expirationstatusid;
this.changedFields = changedFields.add("expirationstatusid");
return this;
}
public Builder statecode(Integer statecode) {
this.statecode = statecode;
this.changedFields = changedFields.add("statecode");
return this;
}
public Builder isrootarticle(Boolean isrootarticle) {
this.isrootarticle = isrootarticle;
this.changedFields = changedFields.add("isrootarticle");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder rating_count(Integer rating_count) {
this.rating_count = rating_count;
this.changedFields = changedFields.add("rating_count");
return this;
}
public Builder _subjectid_value(UUID _subjectid_value) {
this._subjectid_value = _subjectid_value;
this.changedFields = changedFields.add("_subjectid_value");
return this;
}
public Builder islatestversion(Boolean islatestversion) {
this.islatestversion = islatestversion;
this.changedFields = changedFields.add("islatestversion");
return this;
}
public Builder publishon(OffsetDateTime publishon) {
this.publishon = publishon;
this.changedFields = changedFields.add("publishon");
return this;
}
public Builder processid(UUID processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder scheduledstatusid(Integer scheduledstatusid) {
this.scheduledstatusid = scheduledstatusid;
this.changedFields = changedFields.add("scheduledstatusid");
return this;
}
public Builder _previousarticlecontentid_value(UUID _previousarticlecontentid_value) {
this._previousarticlecontentid_value = _previousarticlecontentid_value;
this.changedFields = changedFields.add("_previousarticlecontentid_value");
return this;
}
public Builder minorversionnumber(Integer minorversionnumber) {
this.minorversionnumber = minorversionnumber;
this.changedFields = changedFields.add("minorversionnumber");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder majorversionnumber(Integer majorversionnumber) {
this.majorversionnumber = majorversionnumber;
this.changedFields = changedFields.add("majorversionnumber");
return this;
}
public Builder articlepublicnumber(String articlepublicnumber) {
this.articlepublicnumber = articlepublicnumber;
this.changedFields = changedFields.add("articlepublicnumber");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder statuscode(Integer statuscode) {
this.statuscode = statuscode;
this.changedFields = changedFields.add("statuscode");
return this;
}
public Builder expirationdate(OffsetDateTime expirationdate) {
this.expirationdate = expirationdate;
this.changedFields = changedFields.add("expirationdate");
return this;
}
public Builder _rootarticleid_value(UUID _rootarticleid_value) {
this._rootarticleid_value = _rootarticleid_value;
this.changedFields = changedFields.add("_rootarticleid_value");
return this;
}
public Builder rating(BigDecimal rating) {
this.rating = rating;
this.changedFields = changedFields.add("rating");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder _transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder readyforreview(Boolean readyforreview) {
this.readyforreview = readyforreview;
this.changedFields = changedFields.add("readyforreview");
return this;
}
public Builder review(Integer review) {
this.review = review;
this.changedFields = changedFields.add("review");
return this;
}
public Builder isinternal(Boolean isinternal) {
this.isinternal = isinternal;
this.changedFields = changedFields.add("isinternal");
return this;
}
public Builder setcategoryassociations(Boolean setcategoryassociations) {
this.setcategoryassociations = setcategoryassociations;
this.changedFields = changedFields.add("setcategoryassociations");
return this;
}
public Knowledgearticle build() {
Knowledgearticle _x = new Knowledgearticle();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.knowledgearticle";
_x._owninguser_value = _owninguser_value;
_x._languagelocaleid_value = _languagelocaleid_value;
_x.publishstatusid = publishstatusid;
_x.expiredreviewoptions = expiredreviewoptions;
_x._modifiedby_value = _modifiedby_value;
_x.knowledgearticleviews_date = knowledgearticleviews_date;
_x.importsequencenumber = importsequencenumber;
_x.description = description;
_x.title = title;
_x.versionnumber = versionnumber;
_x.content = content;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.rating_date = rating_date;
_x.rating_state = rating_state;
_x.overriddencreatedon = overriddencreatedon;
_x._parentarticlecontentid_value = _parentarticlecontentid_value;
_x.knowledgearticleviews = knowledgearticleviews;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.keywords = keywords;
_x.isprimary = isprimary;
_x._primaryauthorid_value = _primaryauthorid_value;
_x._ownerid_value = _ownerid_value;
_x._owningteam_value = _owningteam_value;
_x.exchangerate = exchangerate;
_x.updatecontent = updatecontent;
_x.expirationstateid = expirationstateid;
_x.modifiedon = modifiedon;
_x.knowledgearticleviews_state = knowledgearticleviews_state;
_x.knowledgearticleid = knowledgearticleid;
_x.rating_sum = rating_sum;
_x.createdon = createdon;
_x.stageid = stageid;
_x.expirationstatusid = expirationstatusid;
_x.statecode = statecode;
_x.isrootarticle = isrootarticle;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.rating_count = rating_count;
_x._subjectid_value = _subjectid_value;
_x.islatestversion = islatestversion;
_x.publishon = publishon;
_x.processid = processid;
_x.scheduledstatusid = scheduledstatusid;
_x._previousarticlecontentid_value = _previousarticlecontentid_value;
_x.minorversionnumber = minorversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.majorversionnumber = majorversionnumber;
_x.articlepublicnumber = articlepublicnumber;
_x.traversedpath = traversedpath;
_x.statuscode = statuscode;
_x.expirationdate = expirationdate;
_x._rootarticleid_value = _rootarticleid_value;
_x.rating = rating;
_x._createdby_value = _createdby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.readyforreview = readyforreview;
_x.review = review;
_x.isinternal = isinternal;
_x.setcategoryassociations = setcategoryassociations;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && knowledgearticleid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(knowledgearticleid, UUID.class));
}
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Knowledgearticle with_owninguser_value(UUID _owninguser_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="_languagelocaleid_value")
@JsonIgnore
public Optional get_languagelocaleid_value() {
return Optional.ofNullable(_languagelocaleid_value);
}
public Knowledgearticle with_languagelocaleid_value(UUID _languagelocaleid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_languagelocaleid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._languagelocaleid_value = _languagelocaleid_value;
return _x;
}
@Property(name="publishstatusid")
@JsonIgnore
public Optional getPublishstatusid() {
return Optional.ofNullable(publishstatusid);
}
public Knowledgearticle withPublishstatusid(Integer publishstatusid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("publishstatusid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.publishstatusid = publishstatusid;
return _x;
}
@Property(name="expiredreviewoptions")
@JsonIgnore
public Optional getExpiredreviewoptions() {
return Optional.ofNullable(expiredreviewoptions);
}
public Knowledgearticle withExpiredreviewoptions(Integer expiredreviewoptions) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("expiredreviewoptions");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.expiredreviewoptions = expiredreviewoptions;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Knowledgearticle with_modifiedby_value(UUID _modifiedby_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="knowledgearticleviews_date")
@JsonIgnore
public Optional getKnowledgearticleviews_date() {
return Optional.ofNullable(knowledgearticleviews_date);
}
public Knowledgearticle withKnowledgearticleviews_date(OffsetDateTime knowledgearticleviews_date) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("knowledgearticleviews_date");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.knowledgearticleviews_date = knowledgearticleviews_date;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Knowledgearticle withImportsequencenumber(Integer importsequencenumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Knowledgearticle withDescription(String description) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.description = description;
return _x;
}
@Property(name="title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public Knowledgearticle withTitle(String title) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("title");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.title = title;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Knowledgearticle withVersionnumber(Long versionnumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="content")
@JsonIgnore
public Optional getContent() {
return Optional.ofNullable(content);
}
public Knowledgearticle withContent(String content) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("content");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.content = content;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Knowledgearticle with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="rating_date")
@JsonIgnore
public Optional getRating_date() {
return Optional.ofNullable(rating_date);
}
public Knowledgearticle withRating_date(OffsetDateTime rating_date) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("rating_date");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.rating_date = rating_date;
return _x;
}
@Property(name="rating_state")
@JsonIgnore
public Optional getRating_state() {
return Optional.ofNullable(rating_state);
}
public Knowledgearticle withRating_state(Integer rating_state) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("rating_state");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.rating_state = rating_state;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Knowledgearticle withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="_parentarticlecontentid_value")
@JsonIgnore
public Optional get_parentarticlecontentid_value() {
return Optional.ofNullable(_parentarticlecontentid_value);
}
public Knowledgearticle with_parentarticlecontentid_value(UUID _parentarticlecontentid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_parentarticlecontentid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._parentarticlecontentid_value = _parentarticlecontentid_value;
return _x;
}
@Property(name="knowledgearticleviews")
@JsonIgnore
public Optional getKnowledgearticleviews() {
return Optional.ofNullable(knowledgearticleviews);
}
public Knowledgearticle withKnowledgearticleviews(Integer knowledgearticleviews) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("knowledgearticleviews");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.knowledgearticleviews = knowledgearticleviews;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Knowledgearticle with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Knowledgearticle withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="keywords")
@JsonIgnore
public Optional getKeywords() {
return Optional.ofNullable(keywords);
}
public Knowledgearticle withKeywords(String keywords) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("keywords");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.keywords = keywords;
return _x;
}
@Property(name="isprimary")
@JsonIgnore
public Optional getIsprimary() {
return Optional.ofNullable(isprimary);
}
public Knowledgearticle withIsprimary(Boolean isprimary) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("isprimary");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.isprimary = isprimary;
return _x;
}
@Property(name="_primaryauthorid_value")
@JsonIgnore
public Optional get_primaryauthorid_value() {
return Optional.ofNullable(_primaryauthorid_value);
}
public Knowledgearticle with_primaryauthorid_value(UUID _primaryauthorid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_primaryauthorid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._primaryauthorid_value = _primaryauthorid_value;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Knowledgearticle with_ownerid_value(UUID _ownerid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Knowledgearticle with_owningteam_value(UUID _owningteam_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Knowledgearticle withExchangerate(BigDecimal exchangerate) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="updatecontent")
@JsonIgnore
public Optional getUpdatecontent() {
return Optional.ofNullable(updatecontent);
}
public Knowledgearticle withUpdatecontent(Boolean updatecontent) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("updatecontent");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.updatecontent = updatecontent;
return _x;
}
@Property(name="expirationstateid")
@JsonIgnore
public Optional getExpirationstateid() {
return Optional.ofNullable(expirationstateid);
}
public Knowledgearticle withExpirationstateid(Integer expirationstateid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("expirationstateid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.expirationstateid = expirationstateid;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Knowledgearticle withModifiedon(OffsetDateTime modifiedon) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="knowledgearticleviews_state")
@JsonIgnore
public Optional getKnowledgearticleviews_state() {
return Optional.ofNullable(knowledgearticleviews_state);
}
public Knowledgearticle withKnowledgearticleviews_state(Integer knowledgearticleviews_state) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("knowledgearticleviews_state");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.knowledgearticleviews_state = knowledgearticleviews_state;
return _x;
}
@Property(name="knowledgearticleid")
@JsonIgnore
public Optional getKnowledgearticleid() {
return Optional.ofNullable(knowledgearticleid);
}
public Knowledgearticle withKnowledgearticleid(UUID knowledgearticleid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("knowledgearticleid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.knowledgearticleid = knowledgearticleid;
return _x;
}
@Property(name="rating_sum")
@JsonIgnore
public Optional getRating_sum() {
return Optional.ofNullable(rating_sum);
}
public Knowledgearticle withRating_sum(BigDecimal rating_sum) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("rating_sum");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.rating_sum = rating_sum;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Knowledgearticle withCreatedon(OffsetDateTime createdon) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.createdon = createdon;
return _x;
}
@Property(name="stageid")
@JsonIgnore
public Optional getStageid() {
return Optional.ofNullable(stageid);
}
public Knowledgearticle withStageid(UUID stageid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("stageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.stageid = stageid;
return _x;
}
@Property(name="expirationstatusid")
@JsonIgnore
public Optional getExpirationstatusid() {
return Optional.ofNullable(expirationstatusid);
}
public Knowledgearticle withExpirationstatusid(Integer expirationstatusid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("expirationstatusid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.expirationstatusid = expirationstatusid;
return _x;
}
@Property(name="statecode")
@JsonIgnore
public Optional getStatecode() {
return Optional.ofNullable(statecode);
}
public Knowledgearticle withStatecode(Integer statecode) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("statecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.statecode = statecode;
return _x;
}
@Property(name="isrootarticle")
@JsonIgnore
public Optional getIsrootarticle() {
return Optional.ofNullable(isrootarticle);
}
public Knowledgearticle withIsrootarticle(Boolean isrootarticle) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("isrootarticle");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.isrootarticle = isrootarticle;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Knowledgearticle withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="rating_count")
@JsonIgnore
public Optional getRating_count() {
return Optional.ofNullable(rating_count);
}
public Knowledgearticle withRating_count(Integer rating_count) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("rating_count");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.rating_count = rating_count;
return _x;
}
@Property(name="_subjectid_value")
@JsonIgnore
public Optional get_subjectid_value() {
return Optional.ofNullable(_subjectid_value);
}
public Knowledgearticle with_subjectid_value(UUID _subjectid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_subjectid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._subjectid_value = _subjectid_value;
return _x;
}
@Property(name="islatestversion")
@JsonIgnore
public Optional getIslatestversion() {
return Optional.ofNullable(islatestversion);
}
public Knowledgearticle withIslatestversion(Boolean islatestversion) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("islatestversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.islatestversion = islatestversion;
return _x;
}
@Property(name="publishon")
@JsonIgnore
public Optional getPublishon() {
return Optional.ofNullable(publishon);
}
public Knowledgearticle withPublishon(OffsetDateTime publishon) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("publishon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.publishon = publishon;
return _x;
}
@Property(name="processid")
@JsonIgnore
public Optional getProcessid() {
return Optional.ofNullable(processid);
}
public Knowledgearticle withProcessid(UUID processid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("processid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.processid = processid;
return _x;
}
@Property(name="scheduledstatusid")
@JsonIgnore
public Optional getScheduledstatusid() {
return Optional.ofNullable(scheduledstatusid);
}
public Knowledgearticle withScheduledstatusid(Integer scheduledstatusid) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("scheduledstatusid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.scheduledstatusid = scheduledstatusid;
return _x;
}
@Property(name="_previousarticlecontentid_value")
@JsonIgnore
public Optional get_previousarticlecontentid_value() {
return Optional.ofNullable(_previousarticlecontentid_value);
}
public Knowledgearticle with_previousarticlecontentid_value(UUID _previousarticlecontentid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_previousarticlecontentid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._previousarticlecontentid_value = _previousarticlecontentid_value;
return _x;
}
@Property(name="minorversionnumber")
@JsonIgnore
public Optional getMinorversionnumber() {
return Optional.ofNullable(minorversionnumber);
}
public Knowledgearticle withMinorversionnumber(Integer minorversionnumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("minorversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.minorversionnumber = minorversionnumber;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Knowledgearticle with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="majorversionnumber")
@JsonIgnore
public Optional getMajorversionnumber() {
return Optional.ofNullable(majorversionnumber);
}
public Knowledgearticle withMajorversionnumber(Integer majorversionnumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("majorversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.majorversionnumber = majorversionnumber;
return _x;
}
@Property(name="articlepublicnumber")
@JsonIgnore
public Optional getArticlepublicnumber() {
return Optional.ofNullable(articlepublicnumber);
}
public Knowledgearticle withArticlepublicnumber(String articlepublicnumber) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("articlepublicnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.articlepublicnumber = articlepublicnumber;
return _x;
}
@Property(name="traversedpath")
@JsonIgnore
public Optional getTraversedpath() {
return Optional.ofNullable(traversedpath);
}
public Knowledgearticle withTraversedpath(String traversedpath) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("traversedpath");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.traversedpath = traversedpath;
return _x;
}
@Property(name="statuscode")
@JsonIgnore
public Optional getStatuscode() {
return Optional.ofNullable(statuscode);
}
public Knowledgearticle withStatuscode(Integer statuscode) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("statuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.statuscode = statuscode;
return _x;
}
@Property(name="expirationdate")
@JsonIgnore
public Optional getExpirationdate() {
return Optional.ofNullable(expirationdate);
}
public Knowledgearticle withExpirationdate(OffsetDateTime expirationdate) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("expirationdate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.expirationdate = expirationdate;
return _x;
}
@Property(name="_rootarticleid_value")
@JsonIgnore
public Optional get_rootarticleid_value() {
return Optional.ofNullable(_rootarticleid_value);
}
public Knowledgearticle with_rootarticleid_value(UUID _rootarticleid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_rootarticleid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._rootarticleid_value = _rootarticleid_value;
return _x;
}
@Property(name="rating")
@JsonIgnore
public Optional getRating() {
return Optional.ofNullable(rating);
}
public Knowledgearticle withRating(BigDecimal rating) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("rating");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.rating = rating;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Knowledgearticle with_createdby_value(UUID _createdby_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Knowledgearticle with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="readyforreview")
@JsonIgnore
public Optional getReadyforreview() {
return Optional.ofNullable(readyforreview);
}
public Knowledgearticle withReadyforreview(Boolean readyforreview) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("readyforreview");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.readyforreview = readyforreview;
return _x;
}
@Property(name="review")
@JsonIgnore
public Optional getReview() {
return Optional.ofNullable(review);
}
public Knowledgearticle withReview(Integer review) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("review");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.review = review;
return _x;
}
@Property(name="isinternal")
@JsonIgnore
public Optional getIsinternal() {
return Optional.ofNullable(isinternal);
}
public Knowledgearticle withIsinternal(Boolean isinternal) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("isinternal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.isinternal = isinternal;
return _x;
}
@Property(name="setcategoryassociations")
@JsonIgnore
public Optional getSetcategoryassociations() {
return Optional.ofNullable(setcategoryassociations);
}
public Knowledgearticle withSetcategoryassociations(Boolean setcategoryassociations) {
Knowledgearticle _x = _copy();
_x.changedFields = changedFields.add("setcategoryassociations");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.knowledgearticle");
_x.setcategoryassociations = setcategoryassociations;
return _x;
}
public Knowledgearticle withUnmappedField(String name, Object value) {
Knowledgearticle _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"), RequestHelper.getValue(unmappedFields, "owninguser"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="knowledgearticle_connections1")
@JsonIgnore
public ConnectionCollectionRequest getKnowledgearticle_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("knowledgearticle_connections1"), RequestHelper.getValue(unmappedFields, "knowledgearticle_connections1"));
}
@NavigationProperty(name="knowledgearticle_connections2")
@JsonIgnore
public ConnectionCollectionRequest getKnowledgearticle_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("knowledgearticle_connections2"), RequestHelper.getValue(unmappedFields, "knowledgearticle_connections2"));
}
@NavigationProperty(name="knowledgearticle_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getKnowledgearticle_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("knowledgearticle_DuplicateMatchingRecord"), RequestHelper.getValue(unmappedFields, "knowledgearticle_DuplicateMatchingRecord"));
}
@NavigationProperty(name="knowledgearticle_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getKnowledgearticle_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("knowledgearticle_DuplicateBaseRecord"), RequestHelper.getValue(unmappedFields, "knowledgearticle_DuplicateBaseRecord"));
}
@NavigationProperty(name="knowledgearticle_SharePointDocumentLocations")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getKnowledgearticle_SharePointDocumentLocations() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("knowledgearticle_SharePointDocumentLocations"), RequestHelper.getValue(unmappedFields, "knowledgearticle_SharePointDocumentLocations"));
}
@NavigationProperty(name="knowledgearticle_QueueItems")
@JsonIgnore
public QueueitemCollectionRequest getKnowledgearticle_QueueItems() {
return new QueueitemCollectionRequest(
contextPath.addSegment("knowledgearticle_QueueItems"), RequestHelper.getValue(unmappedFields, "knowledgearticle_QueueItems"));
}
@NavigationProperty(name="knowledgearticle_Annotations")
@JsonIgnore
public AnnotationCollectionRequest getKnowledgearticle_Annotations() {
return new AnnotationCollectionRequest(
contextPath.addSegment("knowledgearticle_Annotations"), RequestHelper.getValue(unmappedFields, "knowledgearticle_Annotations"));
}
@NavigationProperty(name="knowledgearticle_Teams")
@JsonIgnore
public TeamCollectionRequest getKnowledgearticle_Teams() {
return new TeamCollectionRequest(
contextPath.addSegment("knowledgearticle_Teams"), RequestHelper.getValue(unmappedFields, "knowledgearticle_Teams"));
}
@NavigationProperty(name="knowledgearticle_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getKnowledgearticle_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("knowledgearticle_AsyncOperations"), RequestHelper.getValue(unmappedFields, "knowledgearticle_AsyncOperations"));
}
@NavigationProperty(name="knowledgearticle_ProcessSession")
@JsonIgnore
public ProcesssessionCollectionRequest getKnowledgearticle_ProcessSession() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("knowledgearticle_ProcessSession"), RequestHelper.getValue(unmappedFields, "knowledgearticle_ProcessSession"));
}
@NavigationProperty(name="knowledgearticle_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getKnowledgearticle_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("knowledgearticle_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "knowledgearticle_BulkDeleteFailures"));
}
@NavigationProperty(name="knowledgearticle_PrincipalObjectAttributeAccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getKnowledgearticle_PrincipalObjectAttributeAccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("knowledgearticle_PrincipalObjectAttributeAccess"), RequestHelper.getValue(unmappedFields, "knowledgearticle_PrincipalObjectAttributeAccess"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"), RequestHelper.getValue(unmappedFields, "stageid_processstage"));
}
@NavigationProperty(name="knowledgearticle_expiredprocess")
@JsonIgnore
public ExpiredprocessCollectionRequest getKnowledgearticle_expiredprocess() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("knowledgearticle_expiredprocess"), RequestHelper.getValue(unmappedFields, "knowledgearticle_expiredprocess"));
}
@NavigationProperty(name="knowledgearticle_translationprocess")
@JsonIgnore
public TranslationprocessCollectionRequest getKnowledgearticle_translationprocess() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("knowledgearticle_translationprocess"), RequestHelper.getValue(unmappedFields, "knowledgearticle_translationprocess"));
}
@NavigationProperty(name="KnowledgeArticle_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getKnowledgeArticle_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("KnowledgeArticle_SyncErrors"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_SyncErrors"));
}
@NavigationProperty(name="languagelocaleid")
@JsonIgnore
public LanguagelocaleRequest getLanguagelocaleid() {
return new LanguagelocaleRequest(contextPath.addSegment("languagelocaleid"), RequestHelper.getValue(unmappedFields, "languagelocaleid"));
}
@NavigationProperty(name="KnowledgeArticle_RecurringAppointmentMasters")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getKnowledgeArticle_RecurringAppointmentMasters() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("KnowledgeArticle_RecurringAppointmentMasters"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_RecurringAppointmentMasters"));
}
@NavigationProperty(name="KnowledgeArticle_Letters")
@JsonIgnore
public LetterCollectionRequest getKnowledgeArticle_Letters() {
return new LetterCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Letters"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Letters"));
}
@NavigationProperty(name="KnowledgeArticle_SocialActivities")
@JsonIgnore
public SocialactivityCollectionRequest getKnowledgeArticle_SocialActivities() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("KnowledgeArticle_SocialActivities"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_SocialActivities"));
}
@NavigationProperty(name="KnowledgeArticle_ActivityPointers")
@JsonIgnore
public ActivitypointerCollectionRequest getKnowledgeArticle_ActivityPointers() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("KnowledgeArticle_ActivityPointers"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_ActivityPointers"));
}
@NavigationProperty(name="knowledgearticle_newprocess")
@JsonIgnore
public NewprocessCollectionRequest getKnowledgearticle_newprocess() {
return new NewprocessCollectionRequest(
contextPath.addSegment("knowledgearticle_newprocess"), RequestHelper.getValue(unmappedFields, "knowledgearticle_newprocess"));
}
@NavigationProperty(name="KnowledgeArticle_Faxes")
@JsonIgnore
public FaxCollectionRequest getKnowledgeArticle_Faxes() {
return new FaxCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Faxes"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Faxes"));
}
@NavigationProperty(name="PreviousArticleContentId")
@JsonIgnore
public KnowledgearticleRequest getPreviousArticleContentId() {
return new KnowledgearticleRequest(contextPath.addSegment("PreviousArticleContentId"), RequestHelper.getValue(unmappedFields, "PreviousArticleContentId"));
}
@NavigationProperty(name="knowledgearticle_previousarticle_contentid")
@JsonIgnore
public KnowledgearticleCollectionRequest getKnowledgearticle_previousarticle_contentid() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("knowledgearticle_previousarticle_contentid"), RequestHelper.getValue(unmappedFields, "knowledgearticle_previousarticle_contentid"));
}
@NavigationProperty(name="RootArticleId")
@JsonIgnore
public KnowledgearticleRequest getRootArticleId() {
return new KnowledgearticleRequest(contextPath.addSegment("RootArticleId"), RequestHelper.getValue(unmappedFields, "RootArticleId"));
}
@NavigationProperty(name="knowledgearticle_rootarticle_id")
@JsonIgnore
public KnowledgearticleCollectionRequest getKnowledgearticle_rootarticle_id() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("knowledgearticle_rootarticle_id"), RequestHelper.getValue(unmappedFields, "knowledgearticle_rootarticle_id"));
}
@NavigationProperty(name="knowledgearticle_views")
@JsonIgnore
public KnowledgearticleviewsCollectionRequest getKnowledgearticle_views() {
return new KnowledgearticleviewsCollectionRequest(
contextPath.addSegment("knowledgearticle_views"), RequestHelper.getValue(unmappedFields, "knowledgearticle_views"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@NavigationProperty(name="KnowledgeArticle_Phonecalls")
@JsonIgnore
public PhonecallCollectionRequest getKnowledgeArticle_Phonecalls() {
return new PhonecallCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Phonecalls"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Phonecalls"));
}
@NavigationProperty(name="ParentArticleContentId")
@JsonIgnore
public KnowledgearticleRequest getParentArticleContentId() {
return new KnowledgearticleRequest(contextPath.addSegment("ParentArticleContentId"), RequestHelper.getValue(unmappedFields, "ParentArticleContentId"));
}
@NavigationProperty(name="knowledgearticle_parentarticle_contentid")
@JsonIgnore
public KnowledgearticleCollectionRequest getKnowledgearticle_parentarticle_contentid() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("knowledgearticle_parentarticle_contentid"), RequestHelper.getValue(unmappedFields, "knowledgearticle_parentarticle_contentid"));
}
@NavigationProperty(name="knowledgearticle_category")
@JsonIgnore
public CategoryCollectionRequest getKnowledgearticle_category() {
return new CategoryCollectionRequest(
contextPath.addSegment("knowledgearticle_category"), RequestHelper.getValue(unmappedFields, "knowledgearticle_category"));
}
@NavigationProperty(name="knowledgearticle_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getKnowledgearticle_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("knowledgearticle_activity_parties"), RequestHelper.getValue(unmappedFields, "knowledgearticle_activity_parties"));
}
@NavigationProperty(name="KnowledgeArticle_Emails")
@JsonIgnore
public EmailCollectionRequest getKnowledgeArticle_Emails() {
return new EmailCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Emails"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Emails"));
}
@NavigationProperty(name="KnowledgeArticle_Tasks")
@JsonIgnore
public TaskCollectionRequest getKnowledgeArticle_Tasks() {
return new TaskCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Tasks"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Tasks"));
}
@NavigationProperty(name="primaryauthorid")
@JsonIgnore
public SystemuserRequest getPrimaryauthorid() {
return new SystemuserRequest(contextPath.addSegment("primaryauthorid"), RequestHelper.getValue(unmappedFields, "primaryauthorid"));
}
@NavigationProperty(name="KnowledgeArticle_Appointments")
@JsonIgnore
public AppointmentCollectionRequest getKnowledgeArticle_Appointments() {
return new AppointmentCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Appointments"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Appointments"));
}
@NavigationProperty(name="knowledgearticle_PostRegardings")
@JsonIgnore
public PostregardingCollectionRequest getKnowledgearticle_PostRegardings() {
return new PostregardingCollectionRequest(
contextPath.addSegment("knowledgearticle_PostRegardings"), RequestHelper.getValue(unmappedFields, "knowledgearticle_PostRegardings"));
}
@NavigationProperty(name="knowledgearticle_PostFollows")
@JsonIgnore
public PostfollowCollectionRequest getKnowledgearticle_PostFollows() {
return new PostfollowCollectionRequest(
contextPath.addSegment("knowledgearticle_PostFollows"), RequestHelper.getValue(unmappedFields, "knowledgearticle_PostFollows"));
}
@NavigationProperty(name="KnowledgeArticle_Feedback")
@JsonIgnore
public FeedbackCollectionRequest getKnowledgeArticle_Feedback() {
return new FeedbackCollectionRequest(
contextPath.addSegment("KnowledgeArticle_Feedback"), RequestHelper.getValue(unmappedFields, "KnowledgeArticle_Feedback"));
}
@NavigationProperty(name="subjectid")
@JsonIgnore
public SubjectRequest getSubjectid() {
return new SubjectRequest(contextPath.addSegment("subjectid"), RequestHelper.getValue(unmappedFields, "subjectid"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Knowledgearticle patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Knowledgearticle _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Knowledgearticle put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Knowledgearticle _x = _copy();
_x.changedFields = null;
return _x;
}
private Knowledgearticle _copy() {
Knowledgearticle _x = new Knowledgearticle();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x._owninguser_value = _owninguser_value;
_x._languagelocaleid_value = _languagelocaleid_value;
_x.publishstatusid = publishstatusid;
_x.expiredreviewoptions = expiredreviewoptions;
_x._modifiedby_value = _modifiedby_value;
_x.knowledgearticleviews_date = knowledgearticleviews_date;
_x.importsequencenumber = importsequencenumber;
_x.description = description;
_x.title = title;
_x.versionnumber = versionnumber;
_x.content = content;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.rating_date = rating_date;
_x.rating_state = rating_state;
_x.overriddencreatedon = overriddencreatedon;
_x._parentarticlecontentid_value = _parentarticlecontentid_value;
_x.knowledgearticleviews = knowledgearticleviews;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.keywords = keywords;
_x.isprimary = isprimary;
_x._primaryauthorid_value = _primaryauthorid_value;
_x._ownerid_value = _ownerid_value;
_x._owningteam_value = _owningteam_value;
_x.exchangerate = exchangerate;
_x.updatecontent = updatecontent;
_x.expirationstateid = expirationstateid;
_x.modifiedon = modifiedon;
_x.knowledgearticleviews_state = knowledgearticleviews_state;
_x.knowledgearticleid = knowledgearticleid;
_x.rating_sum = rating_sum;
_x.createdon = createdon;
_x.stageid = stageid;
_x.expirationstatusid = expirationstatusid;
_x.statecode = statecode;
_x.isrootarticle = isrootarticle;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.rating_count = rating_count;
_x._subjectid_value = _subjectid_value;
_x.islatestversion = islatestversion;
_x.publishon = publishon;
_x.processid = processid;
_x.scheduledstatusid = scheduledstatusid;
_x._previousarticlecontentid_value = _previousarticlecontentid_value;
_x.minorversionnumber = minorversionnumber;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.majorversionnumber = majorversionnumber;
_x.articlepublicnumber = articlepublicnumber;
_x.traversedpath = traversedpath;
_x.statuscode = statuscode;
_x.expirationdate = expirationdate;
_x._rootarticleid_value = _rootarticleid_value;
_x.rating = rating;
_x._createdby_value = _createdby_value;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.readyforreview = readyforreview;
_x.review = review;
_x.isinternal = isinternal;
_x.setcategoryassociations = setcategoryassociations;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Knowledgearticle[");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("_languagelocaleid_value=");
b.append(this._languagelocaleid_value);
b.append(", ");
b.append("publishstatusid=");
b.append(this.publishstatusid);
b.append(", ");
b.append("expiredreviewoptions=");
b.append(this.expiredreviewoptions);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("knowledgearticleviews_date=");
b.append(this.knowledgearticleviews_date);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("title=");
b.append(this.title);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("content=");
b.append(this.content);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("rating_date=");
b.append(this.rating_date);
b.append(", ");
b.append("rating_state=");
b.append(this.rating_state);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("_parentarticlecontentid_value=");
b.append(this._parentarticlecontentid_value);
b.append(", ");
b.append("knowledgearticleviews=");
b.append(this.knowledgearticleviews);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("keywords=");
b.append(this.keywords);
b.append(", ");
b.append("isprimary=");
b.append(this.isprimary);
b.append(", ");
b.append("_primaryauthorid_value=");
b.append(this._primaryauthorid_value);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("updatecontent=");
b.append(this.updatecontent);
b.append(", ");
b.append("expirationstateid=");
b.append(this.expirationstateid);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("knowledgearticleviews_state=");
b.append(this.knowledgearticleviews_state);
b.append(", ");
b.append("knowledgearticleid=");
b.append(this.knowledgearticleid);
b.append(", ");
b.append("rating_sum=");
b.append(this.rating_sum);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("expirationstatusid=");
b.append(this.expirationstatusid);
b.append(", ");
b.append("statecode=");
b.append(this.statecode);
b.append(", ");
b.append("isrootarticle=");
b.append(this.isrootarticle);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("rating_count=");
b.append(this.rating_count);
b.append(", ");
b.append("_subjectid_value=");
b.append(this._subjectid_value);
b.append(", ");
b.append("islatestversion=");
b.append(this.islatestversion);
b.append(", ");
b.append("publishon=");
b.append(this.publishon);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("scheduledstatusid=");
b.append(this.scheduledstatusid);
b.append(", ");
b.append("_previousarticlecontentid_value=");
b.append(this._previousarticlecontentid_value);
b.append(", ");
b.append("minorversionnumber=");
b.append(this.minorversionnumber);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("majorversionnumber=");
b.append(this.majorversionnumber);
b.append(", ");
b.append("articlepublicnumber=");
b.append(this.articlepublicnumber);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("statuscode=");
b.append(this.statuscode);
b.append(", ");
b.append("expirationdate=");
b.append(this.expirationdate);
b.append(", ");
b.append("_rootarticleid_value=");
b.append(this._rootarticleid_value);
b.append(", ");
b.append("rating=");
b.append(this.rating);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("readyforreview=");
b.append(this.readyforreview);
b.append(", ");
b.append("review=");
b.append(this.review);
b.append(", ");
b.append("isinternal=");
b.append(this.isinternal);
b.append(", ");
b.append("setcategoryassociations=");
b.append(this.setcategoryassociations);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy