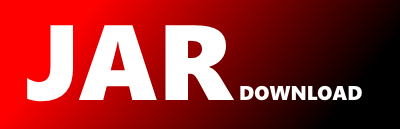
microsoft.dynamics.crm.entity.Officegraphdocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"_modifiedonbehalfby_value",
"utcconversiontimezonecode",
"modifiedby",
"fileextension",
"createdby",
"readurl",
"viewcount",
"sitetitle",
"documentid",
"officegraphdocumentid",
"_transactioncurrencyid_value",
"_createdonbehalfby_value",
"createdtime",
"documentpreviewmetadata",
"documentlastmodifiedon",
"previewimageurl",
"rank",
"authornames",
"modifiedtime",
"versionnumber",
"title",
"secondaryfileextension",
"querytype",
"weblocationurl",
"filetype",
"timezoneruleversionnumber",
"siteurl",
"_organizationid_value",
"exchangerate",
"documentlastmodifiedby"})
@JsonInclude(Include.NON_NULL)
public class Officegraphdocument extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.officegraphdocument";
}
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("modifiedby")
protected String modifiedby;
@JsonProperty("fileextension")
protected String fileextension;
@JsonProperty("createdby")
protected String createdby;
@JsonProperty("readurl")
protected String readurl;
@JsonProperty("viewcount")
protected Integer viewcount;
@JsonProperty("sitetitle")
protected String sitetitle;
@JsonProperty("documentid")
protected String documentid;
@JsonProperty("officegraphdocumentid")
protected UUID officegraphdocumentid;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("createdtime")
protected OffsetDateTime createdtime;
@JsonProperty("documentpreviewmetadata")
protected String documentpreviewmetadata;
@JsonProperty("documentlastmodifiedon")
protected OffsetDateTime documentlastmodifiedon;
@JsonProperty("previewimageurl")
protected String previewimageurl;
@JsonProperty("rank")
protected Integer rank;
@JsonProperty("authornames")
protected String authornames;
@JsonProperty("modifiedtime")
protected OffsetDateTime modifiedtime;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("title")
protected String title;
@JsonProperty("secondaryfileextension")
protected String secondaryfileextension;
@JsonProperty("querytype")
protected Integer querytype;
@JsonProperty("weblocationurl")
protected String weblocationurl;
@JsonProperty("filetype")
protected String filetype;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("siteurl")
protected String siteurl;
@JsonProperty("_organizationid_value")
protected UUID _organizationid_value;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("documentlastmodifiedby")
protected String documentlastmodifiedby;
protected Officegraphdocument() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderOfficegraphdocument() {
return new Builder();
}
public static final class Builder {
private UUID _modifiedonbehalfby_value;
private Integer utcconversiontimezonecode;
private String modifiedby;
private String fileextension;
private String createdby;
private String readurl;
private Integer viewcount;
private String sitetitle;
private String documentid;
private UUID officegraphdocumentid;
private UUID _transactioncurrencyid_value;
private UUID _createdonbehalfby_value;
private OffsetDateTime createdtime;
private String documentpreviewmetadata;
private OffsetDateTime documentlastmodifiedon;
private String previewimageurl;
private Integer rank;
private String authornames;
private OffsetDateTime modifiedtime;
private Long versionnumber;
private String title;
private String secondaryfileextension;
private Integer querytype;
private String weblocationurl;
private String filetype;
private Integer timezoneruleversionnumber;
private String siteurl;
private UUID _organizationid_value;
private BigDecimal exchangerate;
private String documentlastmodifiedby;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder modifiedby(String modifiedby) {
this.modifiedby = modifiedby;
this.changedFields = changedFields.add("modifiedby");
return this;
}
public Builder fileextension(String fileextension) {
this.fileextension = fileextension;
this.changedFields = changedFields.add("fileextension");
return this;
}
public Builder createdby(String createdby) {
this.createdby = createdby;
this.changedFields = changedFields.add("createdby");
return this;
}
public Builder readurl(String readurl) {
this.readurl = readurl;
this.changedFields = changedFields.add("readurl");
return this;
}
public Builder viewcount(Integer viewcount) {
this.viewcount = viewcount;
this.changedFields = changedFields.add("viewcount");
return this;
}
public Builder sitetitle(String sitetitle) {
this.sitetitle = sitetitle;
this.changedFields = changedFields.add("sitetitle");
return this;
}
public Builder documentid(String documentid) {
this.documentid = documentid;
this.changedFields = changedFields.add("documentid");
return this;
}
public Builder officegraphdocumentid(UUID officegraphdocumentid) {
this.officegraphdocumentid = officegraphdocumentid;
this.changedFields = changedFields.add("officegraphdocumentid");
return this;
}
public Builder _transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder createdtime(OffsetDateTime createdtime) {
this.createdtime = createdtime;
this.changedFields = changedFields.add("createdtime");
return this;
}
public Builder documentpreviewmetadata(String documentpreviewmetadata) {
this.documentpreviewmetadata = documentpreviewmetadata;
this.changedFields = changedFields.add("documentpreviewmetadata");
return this;
}
public Builder documentlastmodifiedon(OffsetDateTime documentlastmodifiedon) {
this.documentlastmodifiedon = documentlastmodifiedon;
this.changedFields = changedFields.add("documentlastmodifiedon");
return this;
}
public Builder previewimageurl(String previewimageurl) {
this.previewimageurl = previewimageurl;
this.changedFields = changedFields.add("previewimageurl");
return this;
}
public Builder rank(Integer rank) {
this.rank = rank;
this.changedFields = changedFields.add("rank");
return this;
}
public Builder authornames(String authornames) {
this.authornames = authornames;
this.changedFields = changedFields.add("authornames");
return this;
}
public Builder modifiedtime(OffsetDateTime modifiedtime) {
this.modifiedtime = modifiedtime;
this.changedFields = changedFields.add("modifiedtime");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("title");
return this;
}
public Builder secondaryfileextension(String secondaryfileextension) {
this.secondaryfileextension = secondaryfileextension;
this.changedFields = changedFields.add("secondaryfileextension");
return this;
}
public Builder querytype(Integer querytype) {
this.querytype = querytype;
this.changedFields = changedFields.add("querytype");
return this;
}
public Builder weblocationurl(String weblocationurl) {
this.weblocationurl = weblocationurl;
this.changedFields = changedFields.add("weblocationurl");
return this;
}
public Builder filetype(String filetype) {
this.filetype = filetype;
this.changedFields = changedFields.add("filetype");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder siteurl(String siteurl) {
this.siteurl = siteurl;
this.changedFields = changedFields.add("siteurl");
return this;
}
public Builder _organizationid_value(UUID _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder documentlastmodifiedby(String documentlastmodifiedby) {
this.documentlastmodifiedby = documentlastmodifiedby;
this.changedFields = changedFields.add("documentlastmodifiedby");
return this;
}
public Officegraphdocument build() {
Officegraphdocument _x = new Officegraphdocument();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.officegraphdocument";
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.modifiedby = modifiedby;
_x.fileextension = fileextension;
_x.createdby = createdby;
_x.readurl = readurl;
_x.viewcount = viewcount;
_x.sitetitle = sitetitle;
_x.documentid = documentid;
_x.officegraphdocumentid = officegraphdocumentid;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.createdtime = createdtime;
_x.documentpreviewmetadata = documentpreviewmetadata;
_x.documentlastmodifiedon = documentlastmodifiedon;
_x.previewimageurl = previewimageurl;
_x.rank = rank;
_x.authornames = authornames;
_x.modifiedtime = modifiedtime;
_x.versionnumber = versionnumber;
_x.title = title;
_x.secondaryfileextension = secondaryfileextension;
_x.querytype = querytype;
_x.weblocationurl = weblocationurl;
_x.filetype = filetype;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.siteurl = siteurl;
_x._organizationid_value = _organizationid_value;
_x.exchangerate = exchangerate;
_x.documentlastmodifiedby = documentlastmodifiedby;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && officegraphdocumentid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(officegraphdocumentid, UUID.class));
}
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Officegraphdocument with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Officegraphdocument withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="modifiedby")
@JsonIgnore
public Optional getModifiedby() {
return Optional.ofNullable(modifiedby);
}
public Officegraphdocument withModifiedby(String modifiedby) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("modifiedby");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.modifiedby = modifiedby;
return _x;
}
@Property(name="fileextension")
@JsonIgnore
public Optional getFileextension() {
return Optional.ofNullable(fileextension);
}
public Officegraphdocument withFileextension(String fileextension) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("fileextension");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.fileextension = fileextension;
return _x;
}
@Property(name="createdby")
@JsonIgnore
public Optional getCreatedby() {
return Optional.ofNullable(createdby);
}
public Officegraphdocument withCreatedby(String createdby) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("createdby");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.createdby = createdby;
return _x;
}
@Property(name="readurl")
@JsonIgnore
public Optional getReadurl() {
return Optional.ofNullable(readurl);
}
public Officegraphdocument withReadurl(String readurl) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("readurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.readurl = readurl;
return _x;
}
@Property(name="viewcount")
@JsonIgnore
public Optional getViewcount() {
return Optional.ofNullable(viewcount);
}
public Officegraphdocument withViewcount(Integer viewcount) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("viewcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.viewcount = viewcount;
return _x;
}
@Property(name="sitetitle")
@JsonIgnore
public Optional getSitetitle() {
return Optional.ofNullable(sitetitle);
}
public Officegraphdocument withSitetitle(String sitetitle) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("sitetitle");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.sitetitle = sitetitle;
return _x;
}
@Property(name="documentid")
@JsonIgnore
public Optional getDocumentid() {
return Optional.ofNullable(documentid);
}
public Officegraphdocument withDocumentid(String documentid) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("documentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.documentid = documentid;
return _x;
}
@Property(name="officegraphdocumentid")
@JsonIgnore
public Optional getOfficegraphdocumentid() {
return Optional.ofNullable(officegraphdocumentid);
}
public Officegraphdocument withOfficegraphdocumentid(UUID officegraphdocumentid) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("officegraphdocumentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.officegraphdocumentid = officegraphdocumentid;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Officegraphdocument with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Officegraphdocument with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="createdtime")
@JsonIgnore
public Optional getCreatedtime() {
return Optional.ofNullable(createdtime);
}
public Officegraphdocument withCreatedtime(OffsetDateTime createdtime) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("createdtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.createdtime = createdtime;
return _x;
}
@Property(name="documentpreviewmetadata")
@JsonIgnore
public Optional getDocumentpreviewmetadata() {
return Optional.ofNullable(documentpreviewmetadata);
}
public Officegraphdocument withDocumentpreviewmetadata(String documentpreviewmetadata) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("documentpreviewmetadata");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.documentpreviewmetadata = documentpreviewmetadata;
return _x;
}
@Property(name="documentlastmodifiedon")
@JsonIgnore
public Optional getDocumentlastmodifiedon() {
return Optional.ofNullable(documentlastmodifiedon);
}
public Officegraphdocument withDocumentlastmodifiedon(OffsetDateTime documentlastmodifiedon) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("documentlastmodifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.documentlastmodifiedon = documentlastmodifiedon;
return _x;
}
@Property(name="previewimageurl")
@JsonIgnore
public Optional getPreviewimageurl() {
return Optional.ofNullable(previewimageurl);
}
public Officegraphdocument withPreviewimageurl(String previewimageurl) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("previewimageurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.previewimageurl = previewimageurl;
return _x;
}
@Property(name="rank")
@JsonIgnore
public Optional getRank() {
return Optional.ofNullable(rank);
}
public Officegraphdocument withRank(Integer rank) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("rank");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.rank = rank;
return _x;
}
@Property(name="authornames")
@JsonIgnore
public Optional getAuthornames() {
return Optional.ofNullable(authornames);
}
public Officegraphdocument withAuthornames(String authornames) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("authornames");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.authornames = authornames;
return _x;
}
@Property(name="modifiedtime")
@JsonIgnore
public Optional getModifiedtime() {
return Optional.ofNullable(modifiedtime);
}
public Officegraphdocument withModifiedtime(OffsetDateTime modifiedtime) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("modifiedtime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.modifiedtime = modifiedtime;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Officegraphdocument withVersionnumber(Long versionnumber) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public Officegraphdocument withTitle(String title) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("title");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.title = title;
return _x;
}
@Property(name="secondaryfileextension")
@JsonIgnore
public Optional getSecondaryfileextension() {
return Optional.ofNullable(secondaryfileextension);
}
public Officegraphdocument withSecondaryfileextension(String secondaryfileextension) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("secondaryfileextension");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.secondaryfileextension = secondaryfileextension;
return _x;
}
@Property(name="querytype")
@JsonIgnore
public Optional getQuerytype() {
return Optional.ofNullable(querytype);
}
public Officegraphdocument withQuerytype(Integer querytype) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("querytype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.querytype = querytype;
return _x;
}
@Property(name="weblocationurl")
@JsonIgnore
public Optional getWeblocationurl() {
return Optional.ofNullable(weblocationurl);
}
public Officegraphdocument withWeblocationurl(String weblocationurl) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("weblocationurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.weblocationurl = weblocationurl;
return _x;
}
@Property(name="filetype")
@JsonIgnore
public Optional getFiletype() {
return Optional.ofNullable(filetype);
}
public Officegraphdocument withFiletype(String filetype) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("filetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.filetype = filetype;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Officegraphdocument withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="siteurl")
@JsonIgnore
public Optional getSiteurl() {
return Optional.ofNullable(siteurl);
}
public Officegraphdocument withSiteurl(String siteurl) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("siteurl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.siteurl = siteurl;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Officegraphdocument with_organizationid_value(UUID _organizationid_value) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Officegraphdocument withExchangerate(BigDecimal exchangerate) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="documentlastmodifiedby")
@JsonIgnore
public Optional getDocumentlastmodifiedby() {
return Optional.ofNullable(documentlastmodifiedby);
}
public Officegraphdocument withDocumentlastmodifiedby(String documentlastmodifiedby) {
Officegraphdocument _x = _copy();
_x.changedFields = changedFields.add("documentlastmodifiedby");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.officegraphdocument");
_x.documentlastmodifiedby = documentlastmodifiedby;
return _x;
}
public Officegraphdocument withUnmappedField(String name, Object value) {
Officegraphdocument _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"), RequestHelper.getValue(unmappedFields, "organizationid"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Officegraphdocument patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Officegraphdocument _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Officegraphdocument put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Officegraphdocument _x = _copy();
_x.changedFields = null;
return _x;
}
private Officegraphdocument _copy() {
Officegraphdocument _x = new Officegraphdocument();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.modifiedby = modifiedby;
_x.fileextension = fileextension;
_x.createdby = createdby;
_x.readurl = readurl;
_x.viewcount = viewcount;
_x.sitetitle = sitetitle;
_x.documentid = documentid;
_x.officegraphdocumentid = officegraphdocumentid;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.createdtime = createdtime;
_x.documentpreviewmetadata = documentpreviewmetadata;
_x.documentlastmodifiedon = documentlastmodifiedon;
_x.previewimageurl = previewimageurl;
_x.rank = rank;
_x.authornames = authornames;
_x.modifiedtime = modifiedtime;
_x.versionnumber = versionnumber;
_x.title = title;
_x.secondaryfileextension = secondaryfileextension;
_x.querytype = querytype;
_x.weblocationurl = weblocationurl;
_x.filetype = filetype;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.siteurl = siteurl;
_x._organizationid_value = _organizationid_value;
_x.exchangerate = exchangerate;
_x.documentlastmodifiedby = documentlastmodifiedby;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Officegraphdocument[");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("modifiedby=");
b.append(this.modifiedby);
b.append(", ");
b.append("fileextension=");
b.append(this.fileextension);
b.append(", ");
b.append("createdby=");
b.append(this.createdby);
b.append(", ");
b.append("readurl=");
b.append(this.readurl);
b.append(", ");
b.append("viewcount=");
b.append(this.viewcount);
b.append(", ");
b.append("sitetitle=");
b.append(this.sitetitle);
b.append(", ");
b.append("documentid=");
b.append(this.documentid);
b.append(", ");
b.append("officegraphdocumentid=");
b.append(this.officegraphdocumentid);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("createdtime=");
b.append(this.createdtime);
b.append(", ");
b.append("documentpreviewmetadata=");
b.append(this.documentpreviewmetadata);
b.append(", ");
b.append("documentlastmodifiedon=");
b.append(this.documentlastmodifiedon);
b.append(", ");
b.append("previewimageurl=");
b.append(this.previewimageurl);
b.append(", ");
b.append("rank=");
b.append(this.rank);
b.append(", ");
b.append("authornames=");
b.append(this.authornames);
b.append(", ");
b.append("modifiedtime=");
b.append(this.modifiedtime);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("title=");
b.append(this.title);
b.append(", ");
b.append("secondaryfileextension=");
b.append(this.secondaryfileextension);
b.append(", ");
b.append("querytype=");
b.append(this.querytype);
b.append(", ");
b.append("weblocationurl=");
b.append(this.weblocationurl);
b.append(", ");
b.append("filetype=");
b.append(this.filetype);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("siteurl=");
b.append(this.siteurl);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("documentlastmodifiedby=");
b.append(this.documentlastmodifiedby);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy