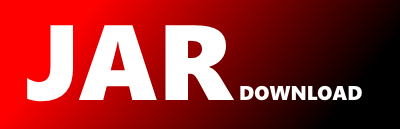
microsoft.dynamics.crm.entity.RelationshipMetadataBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.enums.RelationshipType;
import microsoft.dynamics.crm.enums.SecurityTypes;
@JsonPropertyOrder({
"@odata.type",
"IsCustomRelationship",
"IsCustomizable",
"IsValidForAdvancedFind",
"SchemaName",
"SecurityTypes",
"IsManaged",
"RelationshipType",
"IntroducedVersion"})
@JsonInclude(Include.NON_NULL)
public class RelationshipMetadataBase extends MetadataBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.RelationshipMetadataBase";
}
@JsonProperty("IsCustomRelationship")
protected Boolean isCustomRelationship;
@JsonProperty("IsCustomizable")
protected BooleanManagedProperty isCustomizable;
@JsonProperty("IsValidForAdvancedFind")
protected Boolean isValidForAdvancedFind;
@JsonProperty("SchemaName")
protected String schemaName;
@JsonProperty("SecurityTypes")
protected SecurityTypes securityTypes;
@JsonProperty("IsManaged")
protected Boolean isManaged;
@JsonProperty("RelationshipType")
protected RelationshipType relationshipType;
@JsonProperty("IntroducedVersion")
protected String introducedVersion;
protected RelationshipMetadataBase() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && metadataId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(metadataId, UUID.class));
}
}
@Property(name="IsCustomRelationship")
@JsonIgnore
public Optional getIsCustomRelationship() {
return Optional.ofNullable(isCustomRelationship);
}
public RelationshipMetadataBase withIsCustomRelationship(Boolean isCustomRelationship) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("IsCustomRelationship");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.isCustomRelationship = isCustomRelationship;
return _x;
}
@Property(name="IsCustomizable")
@JsonIgnore
public Optional getIsCustomizable() {
return Optional.ofNullable(isCustomizable);
}
public RelationshipMetadataBase withIsCustomizable(BooleanManagedProperty isCustomizable) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("IsCustomizable");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.isCustomizable = isCustomizable;
return _x;
}
@Property(name="IsValidForAdvancedFind")
@JsonIgnore
public Optional getIsValidForAdvancedFind() {
return Optional.ofNullable(isValidForAdvancedFind);
}
public RelationshipMetadataBase withIsValidForAdvancedFind(Boolean isValidForAdvancedFind) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("IsValidForAdvancedFind");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.isValidForAdvancedFind = isValidForAdvancedFind;
return _x;
}
@Property(name="SchemaName")
@JsonIgnore
public Optional getSchemaName() {
return Optional.ofNullable(schemaName);
}
public RelationshipMetadataBase withSchemaName(String schemaName) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("SchemaName");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.schemaName = schemaName;
return _x;
}
@Property(name="SecurityTypes")
@JsonIgnore
public Optional getSecurityTypes() {
return Optional.ofNullable(securityTypes);
}
public RelationshipMetadataBase withSecurityTypes(SecurityTypes securityTypes) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("SecurityTypes");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.securityTypes = securityTypes;
return _x;
}
@Property(name="IsManaged")
@JsonIgnore
public Optional getIsManaged() {
return Optional.ofNullable(isManaged);
}
public RelationshipMetadataBase withIsManaged(Boolean isManaged) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("IsManaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.isManaged = isManaged;
return _x;
}
@Property(name="RelationshipType")
@JsonIgnore
public Optional getRelationshipType() {
return Optional.ofNullable(relationshipType);
}
public RelationshipMetadataBase withRelationshipType(RelationshipType relationshipType) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("RelationshipType");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.relationshipType = relationshipType;
return _x;
}
@Property(name="IntroducedVersion")
@JsonIgnore
public Optional getIntroducedVersion() {
return Optional.ofNullable(introducedVersion);
}
public RelationshipMetadataBase withIntroducedVersion(String introducedVersion) {
RelationshipMetadataBase _x = _copy();
_x.changedFields = changedFields.add("IntroducedVersion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.RelationshipMetadataBase");
_x.introducedVersion = introducedVersion;
return _x;
}
public RelationshipMetadataBase withUnmappedField(String name, Object value) {
RelationshipMetadataBase _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public RelationshipMetadataBase patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
RelationshipMetadataBase _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public RelationshipMetadataBase put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
RelationshipMetadataBase _x = _copy();
_x.changedFields = null;
return _x;
}
private RelationshipMetadataBase _copy() {
RelationshipMetadataBase _x = new RelationshipMetadataBase();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.metadataId = metadataId;
_x.hasChanged = hasChanged;
_x.isCustomRelationship = isCustomRelationship;
_x.isCustomizable = isCustomizable;
_x.isValidForAdvancedFind = isValidForAdvancedFind;
_x.schemaName = schemaName;
_x.securityTypes = securityTypes;
_x.isManaged = isManaged;
_x.relationshipType = relationshipType;
_x.introducedVersion = introducedVersion;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("RelationshipMetadataBase[");
b.append("MetadataId=");
b.append(this.metadataId);
b.append(", ");
b.append("HasChanged=");
b.append(this.hasChanged);
b.append(", ");
b.append("IsCustomRelationship=");
b.append(this.isCustomRelationship);
b.append(", ");
b.append("IsCustomizable=");
b.append(this.isCustomizable);
b.append(", ");
b.append("IsValidForAdvancedFind=");
b.append(this.isValidForAdvancedFind);
b.append(", ");
b.append("SchemaName=");
b.append(this.schemaName);
b.append(", ");
b.append("SecurityTypes=");
b.append(this.securityTypes);
b.append(", ");
b.append("IsManaged=");
b.append(this.isManaged);
b.append(", ");
b.append("RelationshipType=");
b.append(this.relationshipType);
b.append(", ");
b.append("IntroducedVersion=");
b.append(this.introducedVersion);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy