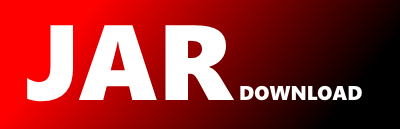
microsoft.dynamics.crm.entity.Systemform Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.FunctionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesstriggerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SystemformCollectionRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.SystemformRequest;
@JsonPropertyOrder({
"@odata.type",
"formjson",
"formactivationstate",
"overwritetime",
"canbedeleted",
"componentstate",
"_organizationid_value",
"version",
"description",
"istabletenabled",
"isdefault",
"introducedversion",
"objecttypecode",
"ismanaged",
"versionnumber",
"type",
"formidunique",
"formpresentation",
"formid",
"solutionid",
"name",
"uniquename",
"formxml",
"isdesktopenabled",
"iscustomizable",
"publishedon",
"_ancestorformid_value",
"isairmerged"})
@JsonInclude(Include.NON_NULL)
public class Systemform extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.systemform";
}
@JsonProperty("formjson")
protected String formjson;
@JsonProperty("formactivationstate")
protected Integer formactivationstate;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("canbedeleted")
protected BooleanManagedProperty canbedeleted;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("_organizationid_value")
protected UUID _organizationid_value;
@JsonProperty("version")
protected Integer version;
@JsonProperty("description")
protected String description;
@JsonProperty("istabletenabled")
protected Boolean istabletenabled;
@JsonProperty("isdefault")
protected Boolean isdefault;
@JsonProperty("introducedversion")
protected String introducedversion;
@JsonProperty("objecttypecode")
protected String objecttypecode;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("type")
protected Integer type;
@JsonProperty("formidunique")
protected UUID formidunique;
@JsonProperty("formpresentation")
protected Integer formpresentation;
@JsonProperty("formid")
protected UUID formid;
@JsonProperty("solutionid")
protected UUID solutionid;
@JsonProperty("name")
protected String name;
@JsonProperty("uniquename")
protected String uniquename;
@JsonProperty("formxml")
protected String formxml;
@JsonProperty("isdesktopenabled")
protected Boolean isdesktopenabled;
@JsonProperty("iscustomizable")
protected BooleanManagedProperty iscustomizable;
@JsonProperty("publishedon")
protected OffsetDateTime publishedon;
@JsonProperty("_ancestorformid_value")
protected UUID _ancestorformid_value;
@JsonProperty("isairmerged")
protected Boolean isairmerged;
protected Systemform() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSystemform() {
return new Builder();
}
public static final class Builder {
private String formjson;
private Integer formactivationstate;
private OffsetDateTime overwritetime;
private BooleanManagedProperty canbedeleted;
private Integer componentstate;
private UUID _organizationid_value;
private Integer version;
private String description;
private Boolean istabletenabled;
private Boolean isdefault;
private String introducedversion;
private String objecttypecode;
private Boolean ismanaged;
private Long versionnumber;
private Integer type;
private UUID formidunique;
private Integer formpresentation;
private UUID formid;
private UUID solutionid;
private String name;
private String uniquename;
private String formxml;
private Boolean isdesktopenabled;
private BooleanManagedProperty iscustomizable;
private OffsetDateTime publishedon;
private UUID _ancestorformid_value;
private Boolean isairmerged;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder formjson(String formjson) {
this.formjson = formjson;
this.changedFields = changedFields.add("formjson");
return this;
}
public Builder formactivationstate(Integer formactivationstate) {
this.formactivationstate = formactivationstate;
this.changedFields = changedFields.add("formactivationstate");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder canbedeleted(BooleanManagedProperty canbedeleted) {
this.canbedeleted = canbedeleted;
this.changedFields = changedFields.add("canbedeleted");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder _organizationid_value(UUID _organizationid_value) {
this._organizationid_value = _organizationid_value;
this.changedFields = changedFields.add("_organizationid_value");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder istabletenabled(Boolean istabletenabled) {
this.istabletenabled = istabletenabled;
this.changedFields = changedFields.add("istabletenabled");
return this;
}
public Builder isdefault(Boolean isdefault) {
this.isdefault = isdefault;
this.changedFields = changedFields.add("isdefault");
return this;
}
public Builder introducedversion(String introducedversion) {
this.introducedversion = introducedversion;
this.changedFields = changedFields.add("introducedversion");
return this;
}
public Builder objecttypecode(String objecttypecode) {
this.objecttypecode = objecttypecode;
this.changedFields = changedFields.add("objecttypecode");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder type(Integer type) {
this.type = type;
this.changedFields = changedFields.add("type");
return this;
}
public Builder formidunique(UUID formidunique) {
this.formidunique = formidunique;
this.changedFields = changedFields.add("formidunique");
return this;
}
public Builder formpresentation(Integer formpresentation) {
this.formpresentation = formpresentation;
this.changedFields = changedFields.add("formpresentation");
return this;
}
public Builder formid(UUID formid) {
this.formid = formid;
this.changedFields = changedFields.add("formid");
return this;
}
public Builder solutionid(UUID solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder uniquename(String uniquename) {
this.uniquename = uniquename;
this.changedFields = changedFields.add("uniquename");
return this;
}
public Builder formxml(String formxml) {
this.formxml = formxml;
this.changedFields = changedFields.add("formxml");
return this;
}
public Builder isdesktopenabled(Boolean isdesktopenabled) {
this.isdesktopenabled = isdesktopenabled;
this.changedFields = changedFields.add("isdesktopenabled");
return this;
}
public Builder iscustomizable(BooleanManagedProperty iscustomizable) {
this.iscustomizable = iscustomizable;
this.changedFields = changedFields.add("iscustomizable");
return this;
}
public Builder publishedon(OffsetDateTime publishedon) {
this.publishedon = publishedon;
this.changedFields = changedFields.add("publishedon");
return this;
}
public Builder _ancestorformid_value(UUID _ancestorformid_value) {
this._ancestorformid_value = _ancestorformid_value;
this.changedFields = changedFields.add("_ancestorformid_value");
return this;
}
public Builder isairmerged(Boolean isairmerged) {
this.isairmerged = isairmerged;
this.changedFields = changedFields.add("isairmerged");
return this;
}
public Systemform build() {
Systemform _x = new Systemform();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.systemform";
_x.formjson = formjson;
_x.formactivationstate = formactivationstate;
_x.overwritetime = overwritetime;
_x.canbedeleted = canbedeleted;
_x.componentstate = componentstate;
_x._organizationid_value = _organizationid_value;
_x.version = version;
_x.description = description;
_x.istabletenabled = istabletenabled;
_x.isdefault = isdefault;
_x.introducedversion = introducedversion;
_x.objecttypecode = objecttypecode;
_x.ismanaged = ismanaged;
_x.versionnumber = versionnumber;
_x.type = type;
_x.formidunique = formidunique;
_x.formpresentation = formpresentation;
_x.formid = formid;
_x.solutionid = solutionid;
_x.name = name;
_x.uniquename = uniquename;
_x.formxml = formxml;
_x.isdesktopenabled = isdesktopenabled;
_x.iscustomizable = iscustomizable;
_x.publishedon = publishedon;
_x._ancestorformid_value = _ancestorformid_value;
_x.isairmerged = isairmerged;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && formid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(formid, UUID.class));
}
}
@Property(name="formjson")
@JsonIgnore
public Optional getFormjson() {
return Optional.ofNullable(formjson);
}
public Systemform withFormjson(String formjson) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formjson");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formjson = formjson;
return _x;
}
@Property(name="formactivationstate")
@JsonIgnore
public Optional getFormactivationstate() {
return Optional.ofNullable(formactivationstate);
}
public Systemform withFormactivationstate(Integer formactivationstate) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formactivationstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formactivationstate = formactivationstate;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Systemform withOverwritetime(OffsetDateTime overwritetime) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="canbedeleted")
@JsonIgnore
public Optional getCanbedeleted() {
return Optional.ofNullable(canbedeleted);
}
public Systemform withCanbedeleted(BooleanManagedProperty canbedeleted) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("canbedeleted");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.canbedeleted = canbedeleted;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Systemform withComponentstate(Integer componentstate) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.componentstate = componentstate;
return _x;
}
@Property(name="_organizationid_value")
@JsonIgnore
public Optional get_organizationid_value() {
return Optional.ofNullable(_organizationid_value);
}
public Systemform with_organizationid_value(UUID _organizationid_value) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("_organizationid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x._organizationid_value = _organizationid_value;
return _x;
}
@Property(name="version")
@JsonIgnore
public Optional getVersion() {
return Optional.ofNullable(version);
}
public Systemform withVersion(Integer version) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("version");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.version = version;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Systemform withDescription(String description) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.description = description;
return _x;
}
@Property(name="istabletenabled")
@JsonIgnore
public Optional getIstabletenabled() {
return Optional.ofNullable(istabletenabled);
}
public Systemform withIstabletenabled(Boolean istabletenabled) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("istabletenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.istabletenabled = istabletenabled;
return _x;
}
@Property(name="isdefault")
@JsonIgnore
public Optional getIsdefault() {
return Optional.ofNullable(isdefault);
}
public Systemform withIsdefault(Boolean isdefault) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("isdefault");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.isdefault = isdefault;
return _x;
}
@Property(name="introducedversion")
@JsonIgnore
public Optional getIntroducedversion() {
return Optional.ofNullable(introducedversion);
}
public Systemform withIntroducedversion(String introducedversion) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("introducedversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.introducedversion = introducedversion;
return _x;
}
@Property(name="objecttypecode")
@JsonIgnore
public Optional getObjecttypecode() {
return Optional.ofNullable(objecttypecode);
}
public Systemform withObjecttypecode(String objecttypecode) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("objecttypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.objecttypecode = objecttypecode;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Systemform withIsmanaged(Boolean ismanaged) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Systemform withVersionnumber(Long versionnumber) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public Systemform withType(Integer type) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("type");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.type = type;
return _x;
}
@Property(name="formidunique")
@JsonIgnore
public Optional getFormidunique() {
return Optional.ofNullable(formidunique);
}
public Systemform withFormidunique(UUID formidunique) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formidunique = formidunique;
return _x;
}
@Property(name="formpresentation")
@JsonIgnore
public Optional getFormpresentation() {
return Optional.ofNullable(formpresentation);
}
public Systemform withFormpresentation(Integer formpresentation) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formpresentation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formpresentation = formpresentation;
return _x;
}
@Property(name="formid")
@JsonIgnore
public Optional getFormid() {
return Optional.ofNullable(formid);
}
public Systemform withFormid(UUID formid) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formid = formid;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Systemform withSolutionid(UUID solutionid) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.solutionid = solutionid;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public Systemform withName(String name) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.name = name;
return _x;
}
@Property(name="uniquename")
@JsonIgnore
public Optional getUniquename() {
return Optional.ofNullable(uniquename);
}
public Systemform withUniquename(String uniquename) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("uniquename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.uniquename = uniquename;
return _x;
}
@Property(name="formxml")
@JsonIgnore
public Optional getFormxml() {
return Optional.ofNullable(formxml);
}
public Systemform withFormxml(String formxml) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("formxml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.formxml = formxml;
return _x;
}
@Property(name="isdesktopenabled")
@JsonIgnore
public Optional getIsdesktopenabled() {
return Optional.ofNullable(isdesktopenabled);
}
public Systemform withIsdesktopenabled(Boolean isdesktopenabled) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("isdesktopenabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.isdesktopenabled = isdesktopenabled;
return _x;
}
@Property(name="iscustomizable")
@JsonIgnore
public Optional getIscustomizable() {
return Optional.ofNullable(iscustomizable);
}
public Systemform withIscustomizable(BooleanManagedProperty iscustomizable) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("iscustomizable");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.iscustomizable = iscustomizable;
return _x;
}
@Property(name="publishedon")
@JsonIgnore
public Optional getPublishedon() {
return Optional.ofNullable(publishedon);
}
public Systemform withPublishedon(OffsetDateTime publishedon) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("publishedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.publishedon = publishedon;
return _x;
}
@Property(name="_ancestorformid_value")
@JsonIgnore
public Optional get_ancestorformid_value() {
return Optional.ofNullable(_ancestorformid_value);
}
public Systemform with_ancestorformid_value(UUID _ancestorformid_value) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("_ancestorformid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x._ancestorformid_value = _ancestorformid_value;
return _x;
}
@Property(name="isairmerged")
@JsonIgnore
public Optional getIsairmerged() {
return Optional.ofNullable(isairmerged);
}
public Systemform withIsairmerged(Boolean isairmerged) {
Systemform _x = _copy();
_x.changedFields = changedFields.add("isairmerged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemform");
_x.isairmerged = isairmerged;
return _x;
}
public Systemform withUnmappedField(String name, Object value) {
Systemform _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="SystemForm_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getSystemForm_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("SystemForm_AsyncOperations"), RequestHelper.getValue(unmappedFields, "SystemForm_AsyncOperations"));
}
@NavigationProperty(name="processtrigger_systemform")
@JsonIgnore
public ProcesstriggerCollectionRequest getProcesstrigger_systemform() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("processtrigger_systemform"), RequestHelper.getValue(unmappedFields, "processtrigger_systemform"));
}
@NavigationProperty(name="SystemForm_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getSystemForm_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("SystemForm_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "SystemForm_BulkDeleteFailures"));
}
@NavigationProperty(name="ancestorformid")
@JsonIgnore
public SystemformRequest getAncestorformid() {
return new SystemformRequest(contextPath.addSegment("ancestorformid"), RequestHelper.getValue(unmappedFields, "ancestorformid"));
}
@NavigationProperty(name="form_ancestor_form")
@JsonIgnore
public SystemformCollectionRequest getForm_ancestor_form() {
return new SystemformCollectionRequest(
contextPath.addSegment("form_ancestor_form"), RequestHelper.getValue(unmappedFields, "form_ancestor_form"));
}
@NavigationProperty(name="organizationid")
@JsonIgnore
public OrganizationRequest getOrganizationid() {
return new OrganizationRequest(contextPath.addSegment("organizationid"), RequestHelper.getValue(unmappedFields, "organizationid"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Systemform patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Systemform _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Systemform put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Systemform _x = _copy();
_x.changedFields = null;
return _x;
}
private Systemform _copy() {
Systemform _x = new Systemform();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.formjson = formjson;
_x.formactivationstate = formactivationstate;
_x.overwritetime = overwritetime;
_x.canbedeleted = canbedeleted;
_x.componentstate = componentstate;
_x._organizationid_value = _organizationid_value;
_x.version = version;
_x.description = description;
_x.istabletenabled = istabletenabled;
_x.isdefault = isdefault;
_x.introducedversion = introducedversion;
_x.objecttypecode = objecttypecode;
_x.ismanaged = ismanaged;
_x.versionnumber = versionnumber;
_x.type = type;
_x.formidunique = formidunique;
_x.formpresentation = formpresentation;
_x.formid = formid;
_x.solutionid = solutionid;
_x.name = name;
_x.uniquename = uniquename;
_x.formxml = formxml;
_x.isdesktopenabled = isdesktopenabled;
_x.iscustomizable = iscustomizable;
_x.publishedon = publishedon;
_x._ancestorformid_value = _ancestorformid_value;
_x.isairmerged = isairmerged;
return _x;
}
@Action(name = "CopySystemForm")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped copySystemForm(Systemform target) {
Map _parameters = ParameterMap
.put("Target", "Microsoft.Dynamics.CRM.systemform", target)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.CopySystemForm"), Systemform.class, _parameters);
}
@Function(name = "RetrieveUnpublished")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUnpublished() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUnpublished"), Systemform.class, _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Systemform[");
b.append("formjson=");
b.append(this.formjson);
b.append(", ");
b.append("formactivationstate=");
b.append(this.formactivationstate);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("canbedeleted=");
b.append(this.canbedeleted);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("_organizationid_value=");
b.append(this._organizationid_value);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("istabletenabled=");
b.append(this.istabletenabled);
b.append(", ");
b.append("isdefault=");
b.append(this.isdefault);
b.append(", ");
b.append("introducedversion=");
b.append(this.introducedversion);
b.append(", ");
b.append("objecttypecode=");
b.append(this.objecttypecode);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("type=");
b.append(this.type);
b.append(", ");
b.append("formidunique=");
b.append(this.formidunique);
b.append(", ");
b.append("formpresentation=");
b.append(this.formpresentation);
b.append(", ");
b.append("formid=");
b.append(this.formid);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("uniquename=");
b.append(this.uniquename);
b.append(", ");
b.append("formxml=");
b.append(this.formxml);
b.append(", ");
b.append("isdesktopenabled=");
b.append(this.isdesktopenabled);
b.append(", ");
b.append("iscustomizable=");
b.append(this.iscustomizable);
b.append(", ");
b.append("publishedon=");
b.append(this.publishedon);
b.append(", ");
b.append("_ancestorformid_value=");
b.append(this._ancestorformid_value);
b.append(", ");
b.append("isairmerged=");
b.append(this.isairmerged);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy