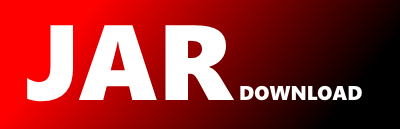
microsoft.dynamics.crm.entity.Systemuser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.FunctionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.complex.AddUserToRecordTeamResponse;
import microsoft.dynamics.crm.complex.RemoveUserFromRecordTeamResponse;
import microsoft.dynamics.crm.complex.RetrievePrincipalAccessInfoResponse;
import microsoft.dynamics.crm.complex.RetrievePrincipalAccessResponse;
import microsoft.dynamics.crm.complex.RetrievePrincipalAttributePrivilegesResponse;
import microsoft.dynamics.crm.complex.RetrievePrincipalSyncAttributeMappingsResponse;
import microsoft.dynamics.crm.complex.RetrieveUserLicenseInfoResponse;
import microsoft.dynamics.crm.complex.RetrieveUserPrivilegeByPrivilegeIdResponse;
import microsoft.dynamics.crm.complex.RetrieveUserPrivilegeByPrivilegeNameResponse;
import microsoft.dynamics.crm.complex.RetrieveUserPrivilegesResponse;
import microsoft.dynamics.crm.complex.RetrieveUsersPrivilegesThroughTeamsResponse;
import microsoft.dynamics.crm.entity.collection.request.AccountCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AciviewmapperCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActioncardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypartyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ActivitypointerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnotationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AnnualfiscalcalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppconfigCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppconfiginstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppconfigmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ApplicationuserCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppmoduleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppmodulecomponentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AppointmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AuditCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeleteoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BusinessunitCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BusinessunitnewsarticleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CalendarruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CallbackregistrationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CategoryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ColumnmappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionreferenceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectionroleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ConnectorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ContactCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomcontrolCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomcontroldefaultconfigCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomcontrolresourceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.CustomeraddressCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DatalakeworkspaceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DatalakeworkspacepermissionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DisplaystringCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DocumenttemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicaterecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicateruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.DuplicateruleconditionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailserverprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EnvironmentvariabledefinitionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EnvironmentvariablevalueCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExchangesyncidmappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExpiredprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ExportsolutionuploadCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FaxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FeedbackCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FieldsecurityprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FixedmonthlyfiscalcalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.FlowsessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_crewCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_eventCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_teamCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Ggw_team_applicationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.GoalCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.GoalrollupqueryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportdataCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportentitymappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportfileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportjobCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportlogCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ImportmapCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.InteractionforemailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KbarticleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KbarticlecommentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KbarticletemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KnowledgearticleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KnowledgearticleviewsCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.KnowledgebaserecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LetterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.LookupmappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailboxtrackingfolderCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MailmergetemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MetricCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MobileofflineprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MobileofflineprofileitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MobileofflineprofileitemassociationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.MonthlyfiscalcalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibdatasetCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibdatasetfileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibdatasetrecordCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibdatasetscontainerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibfileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aibfileattacheddataCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aifptrainingdocumentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aimodelCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiodimageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiodlabelCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiodtrainingboundingboxCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aiodtrainingimageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_aitemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_analysiscomponentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_analysisjobCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_analysisresultCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_analysisresultdetailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_dataflowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_helppageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_knowledgearticleimageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_knowledgearticletemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_richtextfileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_serviceconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_slakpiCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_solutionhealthruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_solutionhealthruleargumentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.Msdyn_solutionhealthrulesetCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.NavigationsettingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.NewprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OfficegraphdocumentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OrganizationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OwnermappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PersonaldocumenttemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PhonecallCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PicklistmappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PluginassemblyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PlugintracelogCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PlugintypeCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PlugintypestatisticCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PositionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostcommentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostfollowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostlikeCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PostregardingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PrincipalobjectattributeaccessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcessstageparameterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesstriggerCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PublisherCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.PublisheraddressCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QuarterlyfiscalcalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.QueueitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecommendeddocumentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurrenceruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RecurringappointmentmasterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ReportCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ReportcategoryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RoleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.RollupfieldCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SavedqueryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SavedqueryvisualizationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessagefilterCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessageprocessingstepCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessageprocessingstepimageCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SdkmessageprocessingstepsecureconfigCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SemiannualfiscalcalendarCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ServiceendpointCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ServiceplanCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SharepointdocumentlocationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SharepointsiteCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SimilarityruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SitemapCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlaitemCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SlakpiinstanceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialactivityCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SocialprofileCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutioncomponentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutioncomponentattributeconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutioncomponentconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SolutioncomponentrelationshipconfigurationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.StagesolutionuploadCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SubjectCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SystemuserCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TaskCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TeamCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TeamtemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TemplateCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TerritoryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ThemeCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TimezonedefinitionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TimezonelocalizednameCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TimezoneruleCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TracelogCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TransactioncurrencyCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TransformationmappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TransformationparametermappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.TranslationprocessCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.UserformCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.UsermappingCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.UserqueryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.UserqueryvisualizationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.UsersettingsCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WebresourceCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WebwizardCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowbinaryCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.WorkflowlogCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.CalendarRequest;
import microsoft.dynamics.crm.entity.request.MailboxRequest;
import microsoft.dynamics.crm.entity.request.MobileofflineprofileRequest;
import microsoft.dynamics.crm.entity.request.OrganizationRequest;
import microsoft.dynamics.crm.entity.request.PositionRequest;
import microsoft.dynamics.crm.entity.request.ProcessstageRequest;
import microsoft.dynamics.crm.entity.request.QueueRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TerritoryRequest;
import microsoft.dynamics.crm.entity.request.TransactioncurrencyRequest;
@JsonPropertyOrder({
"@odata.type",
"title",
"address1_fax",
"organizationid",
"nickname",
"defaultodbfoldername",
"address1_stateorprovince",
"_modifiedby_value",
"applicationid",
"address1_upszone",
"photourl",
"address1_latitude",
"address1_shippingmethodcode",
"versionnumber",
"address1_utcoffset",
"_createdonbehalfby_value",
"homephone",
"address2_latitude",
"governmentid",
"_parentsystemuserid_value",
"salutation",
"address2_longitude",
"_createdby_value",
"overriddencreatedon",
"address1_telephone3",
"mobilephone",
"_queueid_value",
"preferredaddresscode",
"address2_city",
"address1_addressid",
"address1_name",
"address2_stateorprovince",
"address2_line2",
"userpuid",
"firstname",
"passporthi",
"address2_name",
"_territoryid_value",
"address2_shippingmethodcode",
"disabledreason",
"address1_postofficebox",
"address1_composite",
"setupuser",
"entityimage_timestamp",
"internalemailaddress",
"isemailaddressapprovedbyo365admin",
"address1_county",
"_businessunitid_value",
"address1_telephone1",
"invitestatuscode",
"entityimageid",
"address2_line3",
"userlicensetype",
"incomingemaildeliverymethod",
"skills",
"outgoingemaildeliverymethod",
"address2_postalcode",
"passportlo",
"issyncwithdirectory",
"importsequencenumber",
"modifiedon",
"sharepointemailaddress",
"yammeruserid",
"address1_longitude",
"defaultfilterspopulated",
"stageid",
"isintegrationuser",
"personalemailaddress",
"utcconversiontimezonecode",
"address2_telephone2",
"preferredemailcode",
"address2_composite",
"preferredphonecode",
"_mobileofflineprofileid_value",
"windowsliveid",
"address1_line1",
"yomimiddlename",
"entityimage",
"emailrouteraccessapproval",
"_calendarid_value",
"address1_line3",
"yomifirstname",
"address2_country",
"fullname",
"azureactivedirectoryobjectid",
"systemuserid",
"entityimage_url",
"address1_line2",
"address2_upszone",
"address1_city",
"address2_fax",
"_positionid_value",
"address2_line1",
"_transactioncurrencyid_value",
"address2_telephone1",
"middlename",
"isdisabled",
"_defaultmailbox_value",
"address1_postalcode",
"employeeid",
"lastname",
"mobilealertemail",
"timezoneruleversionnumber",
"identityid",
"traversedpath",
"address2_county",
"address1_addresstypecode",
"address2_telephone3",
"yomilastname",
"displayinserviceviews",
"yomifullname",
"address2_addresstypecode",
"createdon",
"accessmode",
"yammeremailaddress",
"_modifiedonbehalfby_value",
"exchangerate",
"jobtitle",
"address2_postofficebox",
"caltype",
"address2_addressid",
"processid",
"applicationiduri",
"address2_utcoffset",
"islicensed",
"address1_telephone2",
"address1_country",
"domainname"})
@JsonInclude(Include.NON_NULL)
public class Systemuser extends Principal implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.systemuser";
}
@JsonProperty("title")
protected String title;
@JsonProperty("address1_fax")
protected String address1_fax;
@JsonProperty("organizationid")
protected UUID organizationid;
@JsonProperty("nickname")
protected String nickname;
@JsonProperty("defaultodbfoldername")
protected String defaultodbfoldername;
@JsonProperty("address1_stateorprovince")
protected String address1_stateorprovince;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("applicationid")
protected UUID applicationid;
@JsonProperty("address1_upszone")
protected String address1_upszone;
@JsonProperty("photourl")
protected String photourl;
@JsonProperty("address1_latitude")
protected Double address1_latitude;
@JsonProperty("address1_shippingmethodcode")
protected Integer address1_shippingmethodcode;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("address1_utcoffset")
protected Integer address1_utcoffset;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
@JsonProperty("homephone")
protected String homephone;
@JsonProperty("address2_latitude")
protected Double address2_latitude;
@JsonProperty("governmentid")
protected String governmentid;
@JsonProperty("_parentsystemuserid_value")
protected UUID _parentsystemuserid_value;
@JsonProperty("salutation")
protected String salutation;
@JsonProperty("address2_longitude")
protected Double address2_longitude;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("overriddencreatedon")
protected OffsetDateTime overriddencreatedon;
@JsonProperty("address1_telephone3")
protected String address1_telephone3;
@JsonProperty("mobilephone")
protected String mobilephone;
@JsonProperty("_queueid_value")
protected UUID _queueid_value;
@JsonProperty("preferredaddresscode")
protected Integer preferredaddresscode;
@JsonProperty("address2_city")
protected String address2_city;
@JsonProperty("address1_addressid")
protected UUID address1_addressid;
@JsonProperty("address1_name")
protected String address1_name;
@JsonProperty("address2_stateorprovince")
protected String address2_stateorprovince;
@JsonProperty("address2_line2")
protected String address2_line2;
@JsonProperty("userpuid")
protected String userpuid;
@JsonProperty("firstname")
protected String firstname;
@JsonProperty("passporthi")
protected Integer passporthi;
@JsonProperty("address2_name")
protected String address2_name;
@JsonProperty("_territoryid_value")
protected UUID _territoryid_value;
@JsonProperty("address2_shippingmethodcode")
protected Integer address2_shippingmethodcode;
@JsonProperty("disabledreason")
protected String disabledreason;
@JsonProperty("address1_postofficebox")
protected String address1_postofficebox;
@JsonProperty("address1_composite")
protected String address1_composite;
@JsonProperty("setupuser")
protected Boolean setupuser;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("internalemailaddress")
protected String internalemailaddress;
@JsonProperty("isemailaddressapprovedbyo365admin")
protected Boolean isemailaddressapprovedbyo365admin;
@JsonProperty("address1_county")
protected String address1_county;
@JsonProperty("_businessunitid_value")
protected UUID _businessunitid_value;
@JsonProperty("address1_telephone1")
protected String address1_telephone1;
@JsonProperty("invitestatuscode")
protected Integer invitestatuscode;
@JsonProperty("entityimageid")
protected UUID entityimageid;
@JsonProperty("address2_line3")
protected String address2_line3;
@JsonProperty("userlicensetype")
protected Integer userlicensetype;
@JsonProperty("incomingemaildeliverymethod")
protected Integer incomingemaildeliverymethod;
@JsonProperty("skills")
protected String skills;
@JsonProperty("outgoingemaildeliverymethod")
protected Integer outgoingemaildeliverymethod;
@JsonProperty("address2_postalcode")
protected String address2_postalcode;
@JsonProperty("passportlo")
protected Integer passportlo;
@JsonProperty("issyncwithdirectory")
protected Boolean issyncwithdirectory;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("sharepointemailaddress")
protected String sharepointemailaddress;
@JsonProperty("yammeruserid")
protected String yammeruserid;
@JsonProperty("address1_longitude")
protected Double address1_longitude;
@JsonProperty("defaultfilterspopulated")
protected Boolean defaultfilterspopulated;
@JsonProperty("stageid")
protected UUID stageid;
@JsonProperty("isintegrationuser")
protected Boolean isintegrationuser;
@JsonProperty("personalemailaddress")
protected String personalemailaddress;
@JsonProperty("utcconversiontimezonecode")
protected Integer utcconversiontimezonecode;
@JsonProperty("address2_telephone2")
protected String address2_telephone2;
@JsonProperty("preferredemailcode")
protected Integer preferredemailcode;
@JsonProperty("address2_composite")
protected String address2_composite;
@JsonProperty("preferredphonecode")
protected Integer preferredphonecode;
@JsonProperty("_mobileofflineprofileid_value")
protected UUID _mobileofflineprofileid_value;
@JsonProperty("windowsliveid")
protected String windowsliveid;
@JsonProperty("address1_line1")
protected String address1_line1;
@JsonProperty("yomimiddlename")
protected String yomimiddlename;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("emailrouteraccessapproval")
protected Integer emailrouteraccessapproval;
@JsonProperty("_calendarid_value")
protected UUID _calendarid_value;
@JsonProperty("address1_line3")
protected String address1_line3;
@JsonProperty("yomifirstname")
protected String yomifirstname;
@JsonProperty("address2_country")
protected String address2_country;
@JsonProperty("fullname")
protected String fullname;
@JsonProperty("azureactivedirectoryobjectid")
protected UUID azureactivedirectoryobjectid;
@JsonProperty("systemuserid")
protected UUID systemuserid;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("address1_line2")
protected String address1_line2;
@JsonProperty("address2_upszone")
protected String address2_upszone;
@JsonProperty("address1_city")
protected String address1_city;
@JsonProperty("address2_fax")
protected String address2_fax;
@JsonProperty("_positionid_value")
protected UUID _positionid_value;
@JsonProperty("address2_line1")
protected String address2_line1;
@JsonProperty("_transactioncurrencyid_value")
protected UUID _transactioncurrencyid_value;
@JsonProperty("address2_telephone1")
protected String address2_telephone1;
@JsonProperty("middlename")
protected String middlename;
@JsonProperty("isdisabled")
protected Boolean isdisabled;
@JsonProperty("_defaultmailbox_value")
protected UUID _defaultmailbox_value;
@JsonProperty("address1_postalcode")
protected String address1_postalcode;
@JsonProperty("employeeid")
protected String employeeid;
@JsonProperty("lastname")
protected String lastname;
@JsonProperty("mobilealertemail")
protected String mobilealertemail;
@JsonProperty("timezoneruleversionnumber")
protected Integer timezoneruleversionnumber;
@JsonProperty("identityid")
protected Integer identityid;
@JsonProperty("traversedpath")
protected String traversedpath;
@JsonProperty("address2_county")
protected String address2_county;
@JsonProperty("address1_addresstypecode")
protected Integer address1_addresstypecode;
@JsonProperty("address2_telephone3")
protected String address2_telephone3;
@JsonProperty("yomilastname")
protected String yomilastname;
@JsonProperty("displayinserviceviews")
protected Boolean displayinserviceviews;
@JsonProperty("yomifullname")
protected String yomifullname;
@JsonProperty("address2_addresstypecode")
protected Integer address2_addresstypecode;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("accessmode")
protected Integer accessmode;
@JsonProperty("yammeremailaddress")
protected String yammeremailaddress;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("exchangerate")
protected BigDecimal exchangerate;
@JsonProperty("jobtitle")
protected String jobtitle;
@JsonProperty("address2_postofficebox")
protected String address2_postofficebox;
@JsonProperty("caltype")
protected Integer caltype;
@JsonProperty("address2_addressid")
protected UUID address2_addressid;
@JsonProperty("processid")
protected UUID processid;
@JsonProperty("applicationiduri")
protected String applicationiduri;
@JsonProperty("address2_utcoffset")
protected Integer address2_utcoffset;
@JsonProperty("islicensed")
protected Boolean islicensed;
@JsonProperty("address1_telephone2")
protected String address1_telephone2;
@JsonProperty("address1_country")
protected String address1_country;
@JsonProperty("domainname")
protected String domainname;
protected Systemuser() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSystemuser() {
return new Builder();
}
public static final class Builder {
private UUID ownerid;
private String title;
private String address1_fax;
private UUID organizationid;
private String nickname;
private String defaultodbfoldername;
private String address1_stateorprovince;
private UUID _modifiedby_value;
private UUID applicationid;
private String address1_upszone;
private String photourl;
private Double address1_latitude;
private Integer address1_shippingmethodcode;
private Long versionnumber;
private Integer address1_utcoffset;
private UUID _createdonbehalfby_value;
private String homephone;
private Double address2_latitude;
private String governmentid;
private UUID _parentsystemuserid_value;
private String salutation;
private Double address2_longitude;
private UUID _createdby_value;
private OffsetDateTime overriddencreatedon;
private String address1_telephone3;
private String mobilephone;
private UUID _queueid_value;
private Integer preferredaddresscode;
private String address2_city;
private UUID address1_addressid;
private String address1_name;
private String address2_stateorprovince;
private String address2_line2;
private String userpuid;
private String firstname;
private Integer passporthi;
private String address2_name;
private UUID _territoryid_value;
private Integer address2_shippingmethodcode;
private String disabledreason;
private String address1_postofficebox;
private String address1_composite;
private Boolean setupuser;
private Long entityimage_timestamp;
private String internalemailaddress;
private Boolean isemailaddressapprovedbyo365admin;
private String address1_county;
private UUID _businessunitid_value;
private String address1_telephone1;
private Integer invitestatuscode;
private UUID entityimageid;
private String address2_line3;
private Integer userlicensetype;
private Integer incomingemaildeliverymethod;
private String skills;
private Integer outgoingemaildeliverymethod;
private String address2_postalcode;
private Integer passportlo;
private Boolean issyncwithdirectory;
private Integer importsequencenumber;
private OffsetDateTime modifiedon;
private String sharepointemailaddress;
private String yammeruserid;
private Double address1_longitude;
private Boolean defaultfilterspopulated;
private UUID stageid;
private Boolean isintegrationuser;
private String personalemailaddress;
private Integer utcconversiontimezonecode;
private String address2_telephone2;
private Integer preferredemailcode;
private String address2_composite;
private Integer preferredphonecode;
private UUID _mobileofflineprofileid_value;
private String windowsliveid;
private String address1_line1;
private String yomimiddlename;
private byte[] entityimage;
private Integer emailrouteraccessapproval;
private UUID _calendarid_value;
private String address1_line3;
private String yomifirstname;
private String address2_country;
private String fullname;
private UUID azureactivedirectoryobjectid;
private UUID systemuserid;
private String entityimage_url;
private String address1_line2;
private String address2_upszone;
private String address1_city;
private String address2_fax;
private UUID _positionid_value;
private String address2_line1;
private UUID _transactioncurrencyid_value;
private String address2_telephone1;
private String middlename;
private Boolean isdisabled;
private UUID _defaultmailbox_value;
private String address1_postalcode;
private String employeeid;
private String lastname;
private String mobilealertemail;
private Integer timezoneruleversionnumber;
private Integer identityid;
private String traversedpath;
private String address2_county;
private Integer address1_addresstypecode;
private String address2_telephone3;
private String yomilastname;
private Boolean displayinserviceviews;
private String yomifullname;
private Integer address2_addresstypecode;
private OffsetDateTime createdon;
private Integer accessmode;
private String yammeremailaddress;
private UUID _modifiedonbehalfby_value;
private BigDecimal exchangerate;
private String jobtitle;
private String address2_postofficebox;
private Integer caltype;
private UUID address2_addressid;
private UUID processid;
private String applicationiduri;
private Integer address2_utcoffset;
private Boolean islicensed;
private String address1_telephone2;
private String address1_country;
private String domainname;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder ownerid(UUID ownerid) {
this.ownerid = ownerid;
this.changedFields = changedFields.add("ownerid");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("title");
return this;
}
public Builder address1_fax(String address1_fax) {
this.address1_fax = address1_fax;
this.changedFields = changedFields.add("address1_fax");
return this;
}
public Builder organizationid(UUID organizationid) {
this.organizationid = organizationid;
this.changedFields = changedFields.add("organizationid");
return this;
}
public Builder nickname(String nickname) {
this.nickname = nickname;
this.changedFields = changedFields.add("nickname");
return this;
}
public Builder defaultodbfoldername(String defaultodbfoldername) {
this.defaultodbfoldername = defaultodbfoldername;
this.changedFields = changedFields.add("defaultodbfoldername");
return this;
}
public Builder address1_stateorprovince(String address1_stateorprovince) {
this.address1_stateorprovince = address1_stateorprovince;
this.changedFields = changedFields.add("address1_stateorprovince");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder applicationid(UUID applicationid) {
this.applicationid = applicationid;
this.changedFields = changedFields.add("applicationid");
return this;
}
public Builder address1_upszone(String address1_upszone) {
this.address1_upszone = address1_upszone;
this.changedFields = changedFields.add("address1_upszone");
return this;
}
public Builder photourl(String photourl) {
this.photourl = photourl;
this.changedFields = changedFields.add("photourl");
return this;
}
public Builder address1_latitude(Double address1_latitude) {
this.address1_latitude = address1_latitude;
this.changedFields = changedFields.add("address1_latitude");
return this;
}
public Builder address1_shippingmethodcode(Integer address1_shippingmethodcode) {
this.address1_shippingmethodcode = address1_shippingmethodcode;
this.changedFields = changedFields.add("address1_shippingmethodcode");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder address1_utcoffset(Integer address1_utcoffset) {
this.address1_utcoffset = address1_utcoffset;
this.changedFields = changedFields.add("address1_utcoffset");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Builder homephone(String homephone) {
this.homephone = homephone;
this.changedFields = changedFields.add("homephone");
return this;
}
public Builder address2_latitude(Double address2_latitude) {
this.address2_latitude = address2_latitude;
this.changedFields = changedFields.add("address2_latitude");
return this;
}
public Builder governmentid(String governmentid) {
this.governmentid = governmentid;
this.changedFields = changedFields.add("governmentid");
return this;
}
public Builder _parentsystemuserid_value(UUID _parentsystemuserid_value) {
this._parentsystemuserid_value = _parentsystemuserid_value;
this.changedFields = changedFields.add("_parentsystemuserid_value");
return this;
}
public Builder salutation(String salutation) {
this.salutation = salutation;
this.changedFields = changedFields.add("salutation");
return this;
}
public Builder address2_longitude(Double address2_longitude) {
this.address2_longitude = address2_longitude;
this.changedFields = changedFields.add("address2_longitude");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder overriddencreatedon(OffsetDateTime overriddencreatedon) {
this.overriddencreatedon = overriddencreatedon;
this.changedFields = changedFields.add("overriddencreatedon");
return this;
}
public Builder address1_telephone3(String address1_telephone3) {
this.address1_telephone3 = address1_telephone3;
this.changedFields = changedFields.add("address1_telephone3");
return this;
}
public Builder mobilephone(String mobilephone) {
this.mobilephone = mobilephone;
this.changedFields = changedFields.add("mobilephone");
return this;
}
public Builder _queueid_value(UUID _queueid_value) {
this._queueid_value = _queueid_value;
this.changedFields = changedFields.add("_queueid_value");
return this;
}
public Builder preferredaddresscode(Integer preferredaddresscode) {
this.preferredaddresscode = preferredaddresscode;
this.changedFields = changedFields.add("preferredaddresscode");
return this;
}
public Builder address2_city(String address2_city) {
this.address2_city = address2_city;
this.changedFields = changedFields.add("address2_city");
return this;
}
public Builder address1_addressid(UUID address1_addressid) {
this.address1_addressid = address1_addressid;
this.changedFields = changedFields.add("address1_addressid");
return this;
}
public Builder address1_name(String address1_name) {
this.address1_name = address1_name;
this.changedFields = changedFields.add("address1_name");
return this;
}
public Builder address2_stateorprovince(String address2_stateorprovince) {
this.address2_stateorprovince = address2_stateorprovince;
this.changedFields = changedFields.add("address2_stateorprovince");
return this;
}
public Builder address2_line2(String address2_line2) {
this.address2_line2 = address2_line2;
this.changedFields = changedFields.add("address2_line2");
return this;
}
public Builder userpuid(String userpuid) {
this.userpuid = userpuid;
this.changedFields = changedFields.add("userpuid");
return this;
}
public Builder firstname(String firstname) {
this.firstname = firstname;
this.changedFields = changedFields.add("firstname");
return this;
}
public Builder passporthi(Integer passporthi) {
this.passporthi = passporthi;
this.changedFields = changedFields.add("passporthi");
return this;
}
public Builder address2_name(String address2_name) {
this.address2_name = address2_name;
this.changedFields = changedFields.add("address2_name");
return this;
}
public Builder _territoryid_value(UUID _territoryid_value) {
this._territoryid_value = _territoryid_value;
this.changedFields = changedFields.add("_territoryid_value");
return this;
}
public Builder address2_shippingmethodcode(Integer address2_shippingmethodcode) {
this.address2_shippingmethodcode = address2_shippingmethodcode;
this.changedFields = changedFields.add("address2_shippingmethodcode");
return this;
}
public Builder disabledreason(String disabledreason) {
this.disabledreason = disabledreason;
this.changedFields = changedFields.add("disabledreason");
return this;
}
public Builder address1_postofficebox(String address1_postofficebox) {
this.address1_postofficebox = address1_postofficebox;
this.changedFields = changedFields.add("address1_postofficebox");
return this;
}
public Builder address1_composite(String address1_composite) {
this.address1_composite = address1_composite;
this.changedFields = changedFields.add("address1_composite");
return this;
}
public Builder setupuser(Boolean setupuser) {
this.setupuser = setupuser;
this.changedFields = changedFields.add("setupuser");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder internalemailaddress(String internalemailaddress) {
this.internalemailaddress = internalemailaddress;
this.changedFields = changedFields.add("internalemailaddress");
return this;
}
public Builder isemailaddressapprovedbyo365admin(Boolean isemailaddressapprovedbyo365admin) {
this.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
this.changedFields = changedFields.add("isemailaddressapprovedbyo365admin");
return this;
}
public Builder address1_county(String address1_county) {
this.address1_county = address1_county;
this.changedFields = changedFields.add("address1_county");
return this;
}
public Builder _businessunitid_value(UUID _businessunitid_value) {
this._businessunitid_value = _businessunitid_value;
this.changedFields = changedFields.add("_businessunitid_value");
return this;
}
public Builder address1_telephone1(String address1_telephone1) {
this.address1_telephone1 = address1_telephone1;
this.changedFields = changedFields.add("address1_telephone1");
return this;
}
public Builder invitestatuscode(Integer invitestatuscode) {
this.invitestatuscode = invitestatuscode;
this.changedFields = changedFields.add("invitestatuscode");
return this;
}
public Builder entityimageid(UUID entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder address2_line3(String address2_line3) {
this.address2_line3 = address2_line3;
this.changedFields = changedFields.add("address2_line3");
return this;
}
public Builder userlicensetype(Integer userlicensetype) {
this.userlicensetype = userlicensetype;
this.changedFields = changedFields.add("userlicensetype");
return this;
}
public Builder incomingemaildeliverymethod(Integer incomingemaildeliverymethod) {
this.incomingemaildeliverymethod = incomingemaildeliverymethod;
this.changedFields = changedFields.add("incomingemaildeliverymethod");
return this;
}
public Builder skills(String skills) {
this.skills = skills;
this.changedFields = changedFields.add("skills");
return this;
}
public Builder outgoingemaildeliverymethod(Integer outgoingemaildeliverymethod) {
this.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
this.changedFields = changedFields.add("outgoingemaildeliverymethod");
return this;
}
public Builder address2_postalcode(String address2_postalcode) {
this.address2_postalcode = address2_postalcode;
this.changedFields = changedFields.add("address2_postalcode");
return this;
}
public Builder passportlo(Integer passportlo) {
this.passportlo = passportlo;
this.changedFields = changedFields.add("passportlo");
return this;
}
public Builder issyncwithdirectory(Boolean issyncwithdirectory) {
this.issyncwithdirectory = issyncwithdirectory;
this.changedFields = changedFields.add("issyncwithdirectory");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder sharepointemailaddress(String sharepointemailaddress) {
this.sharepointemailaddress = sharepointemailaddress;
this.changedFields = changedFields.add("sharepointemailaddress");
return this;
}
public Builder yammeruserid(String yammeruserid) {
this.yammeruserid = yammeruserid;
this.changedFields = changedFields.add("yammeruserid");
return this;
}
public Builder address1_longitude(Double address1_longitude) {
this.address1_longitude = address1_longitude;
this.changedFields = changedFields.add("address1_longitude");
return this;
}
public Builder defaultfilterspopulated(Boolean defaultfilterspopulated) {
this.defaultfilterspopulated = defaultfilterspopulated;
this.changedFields = changedFields.add("defaultfilterspopulated");
return this;
}
public Builder stageid(UUID stageid) {
this.stageid = stageid;
this.changedFields = changedFields.add("stageid");
return this;
}
public Builder isintegrationuser(Boolean isintegrationuser) {
this.isintegrationuser = isintegrationuser;
this.changedFields = changedFields.add("isintegrationuser");
return this;
}
public Builder personalemailaddress(String personalemailaddress) {
this.personalemailaddress = personalemailaddress;
this.changedFields = changedFields.add("personalemailaddress");
return this;
}
public Builder utcconversiontimezonecode(Integer utcconversiontimezonecode) {
this.utcconversiontimezonecode = utcconversiontimezonecode;
this.changedFields = changedFields.add("utcconversiontimezonecode");
return this;
}
public Builder address2_telephone2(String address2_telephone2) {
this.address2_telephone2 = address2_telephone2;
this.changedFields = changedFields.add("address2_telephone2");
return this;
}
public Builder preferredemailcode(Integer preferredemailcode) {
this.preferredemailcode = preferredemailcode;
this.changedFields = changedFields.add("preferredemailcode");
return this;
}
public Builder address2_composite(String address2_composite) {
this.address2_composite = address2_composite;
this.changedFields = changedFields.add("address2_composite");
return this;
}
public Builder preferredphonecode(Integer preferredphonecode) {
this.preferredphonecode = preferredphonecode;
this.changedFields = changedFields.add("preferredphonecode");
return this;
}
public Builder _mobileofflineprofileid_value(UUID _mobileofflineprofileid_value) {
this._mobileofflineprofileid_value = _mobileofflineprofileid_value;
this.changedFields = changedFields.add("_mobileofflineprofileid_value");
return this;
}
public Builder windowsliveid(String windowsliveid) {
this.windowsliveid = windowsliveid;
this.changedFields = changedFields.add("windowsliveid");
return this;
}
public Builder address1_line1(String address1_line1) {
this.address1_line1 = address1_line1;
this.changedFields = changedFields.add("address1_line1");
return this;
}
public Builder yomimiddlename(String yomimiddlename) {
this.yomimiddlename = yomimiddlename;
this.changedFields = changedFields.add("yomimiddlename");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder emailrouteraccessapproval(Integer emailrouteraccessapproval) {
this.emailrouteraccessapproval = emailrouteraccessapproval;
this.changedFields = changedFields.add("emailrouteraccessapproval");
return this;
}
public Builder _calendarid_value(UUID _calendarid_value) {
this._calendarid_value = _calendarid_value;
this.changedFields = changedFields.add("_calendarid_value");
return this;
}
public Builder address1_line3(String address1_line3) {
this.address1_line3 = address1_line3;
this.changedFields = changedFields.add("address1_line3");
return this;
}
public Builder yomifirstname(String yomifirstname) {
this.yomifirstname = yomifirstname;
this.changedFields = changedFields.add("yomifirstname");
return this;
}
public Builder address2_country(String address2_country) {
this.address2_country = address2_country;
this.changedFields = changedFields.add("address2_country");
return this;
}
public Builder fullname(String fullname) {
this.fullname = fullname;
this.changedFields = changedFields.add("fullname");
return this;
}
public Builder azureactivedirectoryobjectid(UUID azureactivedirectoryobjectid) {
this.azureactivedirectoryobjectid = azureactivedirectoryobjectid;
this.changedFields = changedFields.add("azureactivedirectoryobjectid");
return this;
}
public Builder systemuserid(UUID systemuserid) {
this.systemuserid = systemuserid;
this.changedFields = changedFields.add("systemuserid");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder address1_line2(String address1_line2) {
this.address1_line2 = address1_line2;
this.changedFields = changedFields.add("address1_line2");
return this;
}
public Builder address2_upszone(String address2_upszone) {
this.address2_upszone = address2_upszone;
this.changedFields = changedFields.add("address2_upszone");
return this;
}
public Builder address1_city(String address1_city) {
this.address1_city = address1_city;
this.changedFields = changedFields.add("address1_city");
return this;
}
public Builder address2_fax(String address2_fax) {
this.address2_fax = address2_fax;
this.changedFields = changedFields.add("address2_fax");
return this;
}
public Builder _positionid_value(UUID _positionid_value) {
this._positionid_value = _positionid_value;
this.changedFields = changedFields.add("_positionid_value");
return this;
}
public Builder address2_line1(String address2_line1) {
this.address2_line1 = address2_line1;
this.changedFields = changedFields.add("address2_line1");
return this;
}
public Builder _transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
this._transactioncurrencyid_value = _transactioncurrencyid_value;
this.changedFields = changedFields.add("_transactioncurrencyid_value");
return this;
}
public Builder address2_telephone1(String address2_telephone1) {
this.address2_telephone1 = address2_telephone1;
this.changedFields = changedFields.add("address2_telephone1");
return this;
}
public Builder middlename(String middlename) {
this.middlename = middlename;
this.changedFields = changedFields.add("middlename");
return this;
}
public Builder isdisabled(Boolean isdisabled) {
this.isdisabled = isdisabled;
this.changedFields = changedFields.add("isdisabled");
return this;
}
public Builder _defaultmailbox_value(UUID _defaultmailbox_value) {
this._defaultmailbox_value = _defaultmailbox_value;
this.changedFields = changedFields.add("_defaultmailbox_value");
return this;
}
public Builder address1_postalcode(String address1_postalcode) {
this.address1_postalcode = address1_postalcode;
this.changedFields = changedFields.add("address1_postalcode");
return this;
}
public Builder employeeid(String employeeid) {
this.employeeid = employeeid;
this.changedFields = changedFields.add("employeeid");
return this;
}
public Builder lastname(String lastname) {
this.lastname = lastname;
this.changedFields = changedFields.add("lastname");
return this;
}
public Builder mobilealertemail(String mobilealertemail) {
this.mobilealertemail = mobilealertemail;
this.changedFields = changedFields.add("mobilealertemail");
return this;
}
public Builder timezoneruleversionnumber(Integer timezoneruleversionnumber) {
this.timezoneruleversionnumber = timezoneruleversionnumber;
this.changedFields = changedFields.add("timezoneruleversionnumber");
return this;
}
public Builder identityid(Integer identityid) {
this.identityid = identityid;
this.changedFields = changedFields.add("identityid");
return this;
}
public Builder traversedpath(String traversedpath) {
this.traversedpath = traversedpath;
this.changedFields = changedFields.add("traversedpath");
return this;
}
public Builder address2_county(String address2_county) {
this.address2_county = address2_county;
this.changedFields = changedFields.add("address2_county");
return this;
}
public Builder address1_addresstypecode(Integer address1_addresstypecode) {
this.address1_addresstypecode = address1_addresstypecode;
this.changedFields = changedFields.add("address1_addresstypecode");
return this;
}
public Builder address2_telephone3(String address2_telephone3) {
this.address2_telephone3 = address2_telephone3;
this.changedFields = changedFields.add("address2_telephone3");
return this;
}
public Builder yomilastname(String yomilastname) {
this.yomilastname = yomilastname;
this.changedFields = changedFields.add("yomilastname");
return this;
}
public Builder displayinserviceviews(Boolean displayinserviceviews) {
this.displayinserviceviews = displayinserviceviews;
this.changedFields = changedFields.add("displayinserviceviews");
return this;
}
public Builder yomifullname(String yomifullname) {
this.yomifullname = yomifullname;
this.changedFields = changedFields.add("yomifullname");
return this;
}
public Builder address2_addresstypecode(Integer address2_addresstypecode) {
this.address2_addresstypecode = address2_addresstypecode;
this.changedFields = changedFields.add("address2_addresstypecode");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder accessmode(Integer accessmode) {
this.accessmode = accessmode;
this.changedFields = changedFields.add("accessmode");
return this;
}
public Builder yammeremailaddress(String yammeremailaddress) {
this.yammeremailaddress = yammeremailaddress;
this.changedFields = changedFields.add("yammeremailaddress");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder exchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
this.changedFields = changedFields.add("exchangerate");
return this;
}
public Builder jobtitle(String jobtitle) {
this.jobtitle = jobtitle;
this.changedFields = changedFields.add("jobtitle");
return this;
}
public Builder address2_postofficebox(String address2_postofficebox) {
this.address2_postofficebox = address2_postofficebox;
this.changedFields = changedFields.add("address2_postofficebox");
return this;
}
public Builder caltype(Integer caltype) {
this.caltype = caltype;
this.changedFields = changedFields.add("caltype");
return this;
}
public Builder address2_addressid(UUID address2_addressid) {
this.address2_addressid = address2_addressid;
this.changedFields = changedFields.add("address2_addressid");
return this;
}
public Builder processid(UUID processid) {
this.processid = processid;
this.changedFields = changedFields.add("processid");
return this;
}
public Builder applicationiduri(String applicationiduri) {
this.applicationiduri = applicationiduri;
this.changedFields = changedFields.add("applicationiduri");
return this;
}
public Builder address2_utcoffset(Integer address2_utcoffset) {
this.address2_utcoffset = address2_utcoffset;
this.changedFields = changedFields.add("address2_utcoffset");
return this;
}
public Builder islicensed(Boolean islicensed) {
this.islicensed = islicensed;
this.changedFields = changedFields.add("islicensed");
return this;
}
public Builder address1_telephone2(String address1_telephone2) {
this.address1_telephone2 = address1_telephone2;
this.changedFields = changedFields.add("address1_telephone2");
return this;
}
public Builder address1_country(String address1_country) {
this.address1_country = address1_country;
this.changedFields = changedFields.add("address1_country");
return this;
}
public Builder domainname(String domainname) {
this.domainname = domainname;
this.changedFields = changedFields.add("domainname");
return this;
}
public Systemuser build() {
Systemuser _x = new Systemuser();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.systemuser";
_x.ownerid = ownerid;
_x.title = title;
_x.address1_fax = address1_fax;
_x.organizationid = organizationid;
_x.nickname = nickname;
_x.defaultodbfoldername = defaultodbfoldername;
_x.address1_stateorprovince = address1_stateorprovince;
_x._modifiedby_value = _modifiedby_value;
_x.applicationid = applicationid;
_x.address1_upszone = address1_upszone;
_x.photourl = photourl;
_x.address1_latitude = address1_latitude;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x.versionnumber = versionnumber;
_x.address1_utcoffset = address1_utcoffset;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.homephone = homephone;
_x.address2_latitude = address2_latitude;
_x.governmentid = governmentid;
_x._parentsystemuserid_value = _parentsystemuserid_value;
_x.salutation = salutation;
_x.address2_longitude = address2_longitude;
_x._createdby_value = _createdby_value;
_x.overriddencreatedon = overriddencreatedon;
_x.address1_telephone3 = address1_telephone3;
_x.mobilephone = mobilephone;
_x._queueid_value = _queueid_value;
_x.preferredaddresscode = preferredaddresscode;
_x.address2_city = address2_city;
_x.address1_addressid = address1_addressid;
_x.address1_name = address1_name;
_x.address2_stateorprovince = address2_stateorprovince;
_x.address2_line2 = address2_line2;
_x.userpuid = userpuid;
_x.firstname = firstname;
_x.passporthi = passporthi;
_x.address2_name = address2_name;
_x._territoryid_value = _territoryid_value;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.disabledreason = disabledreason;
_x.address1_postofficebox = address1_postofficebox;
_x.address1_composite = address1_composite;
_x.setupuser = setupuser;
_x.entityimage_timestamp = entityimage_timestamp;
_x.internalemailaddress = internalemailaddress;
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
_x.address1_county = address1_county;
_x._businessunitid_value = _businessunitid_value;
_x.address1_telephone1 = address1_telephone1;
_x.invitestatuscode = invitestatuscode;
_x.entityimageid = entityimageid;
_x.address2_line3 = address2_line3;
_x.userlicensetype = userlicensetype;
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
_x.skills = skills;
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
_x.address2_postalcode = address2_postalcode;
_x.passportlo = passportlo;
_x.issyncwithdirectory = issyncwithdirectory;
_x.importsequencenumber = importsequencenumber;
_x.modifiedon = modifiedon;
_x.sharepointemailaddress = sharepointemailaddress;
_x.yammeruserid = yammeruserid;
_x.address1_longitude = address1_longitude;
_x.defaultfilterspopulated = defaultfilterspopulated;
_x.stageid = stageid;
_x.isintegrationuser = isintegrationuser;
_x.personalemailaddress = personalemailaddress;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.address2_telephone2 = address2_telephone2;
_x.preferredemailcode = preferredemailcode;
_x.address2_composite = address2_composite;
_x.preferredphonecode = preferredphonecode;
_x._mobileofflineprofileid_value = _mobileofflineprofileid_value;
_x.windowsliveid = windowsliveid;
_x.address1_line1 = address1_line1;
_x.yomimiddlename = yomimiddlename;
_x.entityimage = entityimage;
_x.emailrouteraccessapproval = emailrouteraccessapproval;
_x._calendarid_value = _calendarid_value;
_x.address1_line3 = address1_line3;
_x.yomifirstname = yomifirstname;
_x.address2_country = address2_country;
_x.fullname = fullname;
_x.azureactivedirectoryobjectid = azureactivedirectoryobjectid;
_x.systemuserid = systemuserid;
_x.entityimage_url = entityimage_url;
_x.address1_line2 = address1_line2;
_x.address2_upszone = address2_upszone;
_x.address1_city = address1_city;
_x.address2_fax = address2_fax;
_x._positionid_value = _positionid_value;
_x.address2_line1 = address2_line1;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.address2_telephone1 = address2_telephone1;
_x.middlename = middlename;
_x.isdisabled = isdisabled;
_x._defaultmailbox_value = _defaultmailbox_value;
_x.address1_postalcode = address1_postalcode;
_x.employeeid = employeeid;
_x.lastname = lastname;
_x.mobilealertemail = mobilealertemail;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.identityid = identityid;
_x.traversedpath = traversedpath;
_x.address2_county = address2_county;
_x.address1_addresstypecode = address1_addresstypecode;
_x.address2_telephone3 = address2_telephone3;
_x.yomilastname = yomilastname;
_x.displayinserviceviews = displayinserviceviews;
_x.yomifullname = yomifullname;
_x.address2_addresstypecode = address2_addresstypecode;
_x.createdon = createdon;
_x.accessmode = accessmode;
_x.yammeremailaddress = yammeremailaddress;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.jobtitle = jobtitle;
_x.address2_postofficebox = address2_postofficebox;
_x.caltype = caltype;
_x.address2_addressid = address2_addressid;
_x.processid = processid;
_x.applicationiduri = applicationiduri;
_x.address2_utcoffset = address2_utcoffset;
_x.islicensed = islicensed;
_x.address1_telephone2 = address1_telephone2;
_x.address1_country = address1_country;
_x.domainname = domainname;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && ownerid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(ownerid, UUID.class));
}
}
@Property(name="title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public Systemuser withTitle(String title) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("title");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.title = title;
return _x;
}
@Property(name="address1_fax")
@JsonIgnore
public Optional getAddress1_fax() {
return Optional.ofNullable(address1_fax);
}
public Systemuser withAddress1_fax(String address1_fax) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_fax = address1_fax;
return _x;
}
@Property(name="organizationid")
@JsonIgnore
public Optional getOrganizationid() {
return Optional.ofNullable(organizationid);
}
public Systemuser withOrganizationid(UUID organizationid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("organizationid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.organizationid = organizationid;
return _x;
}
@Property(name="nickname")
@JsonIgnore
public Optional getNickname() {
return Optional.ofNullable(nickname);
}
public Systemuser withNickname(String nickname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("nickname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.nickname = nickname;
return _x;
}
@Property(name="defaultodbfoldername")
@JsonIgnore
public Optional getDefaultodbfoldername() {
return Optional.ofNullable(defaultodbfoldername);
}
public Systemuser withDefaultodbfoldername(String defaultodbfoldername) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("defaultodbfoldername");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.defaultodbfoldername = defaultodbfoldername;
return _x;
}
@Property(name="address1_stateorprovince")
@JsonIgnore
public Optional getAddress1_stateorprovince() {
return Optional.ofNullable(address1_stateorprovince);
}
public Systemuser withAddress1_stateorprovince(String address1_stateorprovince) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_stateorprovince = address1_stateorprovince;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Systemuser with_modifiedby_value(UUID _modifiedby_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="applicationid")
@JsonIgnore
public Optional getApplicationid() {
return Optional.ofNullable(applicationid);
}
public Systemuser withApplicationid(UUID applicationid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("applicationid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.applicationid = applicationid;
return _x;
}
@Property(name="address1_upszone")
@JsonIgnore
public Optional getAddress1_upszone() {
return Optional.ofNullable(address1_upszone);
}
public Systemuser withAddress1_upszone(String address1_upszone) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_upszone = address1_upszone;
return _x;
}
@Property(name="photourl")
@JsonIgnore
public Optional getPhotourl() {
return Optional.ofNullable(photourl);
}
public Systemuser withPhotourl(String photourl) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("photourl");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.photourl = photourl;
return _x;
}
@Property(name="address1_latitude")
@JsonIgnore
public Optional getAddress1_latitude() {
return Optional.ofNullable(address1_latitude);
}
public Systemuser withAddress1_latitude(Double address1_latitude) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_latitude = address1_latitude;
return _x;
}
@Property(name="address1_shippingmethodcode")
@JsonIgnore
public Optional getAddress1_shippingmethodcode() {
return Optional.ofNullable(address1_shippingmethodcode);
}
public Systemuser withAddress1_shippingmethodcode(Integer address1_shippingmethodcode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_shippingmethodcode = address1_shippingmethodcode;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Systemuser withVersionnumber(Long versionnumber) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="address1_utcoffset")
@JsonIgnore
public Optional getAddress1_utcoffset() {
return Optional.ofNullable(address1_utcoffset);
}
public Systemuser withAddress1_utcoffset(Integer address1_utcoffset) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_utcoffset = address1_utcoffset;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Systemuser with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Property(name="homephone")
@JsonIgnore
public Optional getHomephone() {
return Optional.ofNullable(homephone);
}
public Systemuser withHomephone(String homephone) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("homephone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.homephone = homephone;
return _x;
}
@Property(name="address2_latitude")
@JsonIgnore
public Optional getAddress2_latitude() {
return Optional.ofNullable(address2_latitude);
}
public Systemuser withAddress2_latitude(Double address2_latitude) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_latitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_latitude = address2_latitude;
return _x;
}
@Property(name="governmentid")
@JsonIgnore
public Optional getGovernmentid() {
return Optional.ofNullable(governmentid);
}
public Systemuser withGovernmentid(String governmentid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("governmentid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.governmentid = governmentid;
return _x;
}
@Property(name="_parentsystemuserid_value")
@JsonIgnore
public Optional get_parentsystemuserid_value() {
return Optional.ofNullable(_parentsystemuserid_value);
}
public Systemuser with_parentsystemuserid_value(UUID _parentsystemuserid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_parentsystemuserid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._parentsystemuserid_value = _parentsystemuserid_value;
return _x;
}
@Property(name="salutation")
@JsonIgnore
public Optional getSalutation() {
return Optional.ofNullable(salutation);
}
public Systemuser withSalutation(String salutation) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("salutation");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.salutation = salutation;
return _x;
}
@Property(name="address2_longitude")
@JsonIgnore
public Optional getAddress2_longitude() {
return Optional.ofNullable(address2_longitude);
}
public Systemuser withAddress2_longitude(Double address2_longitude) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_longitude = address2_longitude;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Systemuser with_createdby_value(UUID _createdby_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="overriddencreatedon")
@JsonIgnore
public Optional getOverriddencreatedon() {
return Optional.ofNullable(overriddencreatedon);
}
public Systemuser withOverriddencreatedon(OffsetDateTime overriddencreatedon) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("overriddencreatedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.overriddencreatedon = overriddencreatedon;
return _x;
}
@Property(name="address1_telephone3")
@JsonIgnore
public Optional getAddress1_telephone3() {
return Optional.ofNullable(address1_telephone3);
}
public Systemuser withAddress1_telephone3(String address1_telephone3) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_telephone3 = address1_telephone3;
return _x;
}
@Property(name="mobilephone")
@JsonIgnore
public Optional getMobilephone() {
return Optional.ofNullable(mobilephone);
}
public Systemuser withMobilephone(String mobilephone) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("mobilephone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.mobilephone = mobilephone;
return _x;
}
@Property(name="_queueid_value")
@JsonIgnore
public Optional get_queueid_value() {
return Optional.ofNullable(_queueid_value);
}
public Systemuser with_queueid_value(UUID _queueid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_queueid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._queueid_value = _queueid_value;
return _x;
}
@Property(name="preferredaddresscode")
@JsonIgnore
public Optional getPreferredaddresscode() {
return Optional.ofNullable(preferredaddresscode);
}
public Systemuser withPreferredaddresscode(Integer preferredaddresscode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("preferredaddresscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.preferredaddresscode = preferredaddresscode;
return _x;
}
@Property(name="address2_city")
@JsonIgnore
public Optional getAddress2_city() {
return Optional.ofNullable(address2_city);
}
public Systemuser withAddress2_city(String address2_city) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_city = address2_city;
return _x;
}
@Property(name="address1_addressid")
@JsonIgnore
public Optional getAddress1_addressid() {
return Optional.ofNullable(address1_addressid);
}
public Systemuser withAddress1_addressid(UUID address1_addressid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_addressid = address1_addressid;
return _x;
}
@Property(name="address1_name")
@JsonIgnore
public Optional getAddress1_name() {
return Optional.ofNullable(address1_name);
}
public Systemuser withAddress1_name(String address1_name) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_name = address1_name;
return _x;
}
@Property(name="address2_stateorprovince")
@JsonIgnore
public Optional getAddress2_stateorprovince() {
return Optional.ofNullable(address2_stateorprovince);
}
public Systemuser withAddress2_stateorprovince(String address2_stateorprovince) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_stateorprovince");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_stateorprovince = address2_stateorprovince;
return _x;
}
@Property(name="address2_line2")
@JsonIgnore
public Optional getAddress2_line2() {
return Optional.ofNullable(address2_line2);
}
public Systemuser withAddress2_line2(String address2_line2) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_line2 = address2_line2;
return _x;
}
@Property(name="userpuid")
@JsonIgnore
public Optional getUserpuid() {
return Optional.ofNullable(userpuid);
}
public Systemuser withUserpuid(String userpuid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("userpuid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.userpuid = userpuid;
return _x;
}
@Property(name="firstname")
@JsonIgnore
public Optional getFirstname() {
return Optional.ofNullable(firstname);
}
public Systemuser withFirstname(String firstname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("firstname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.firstname = firstname;
return _x;
}
@Property(name="passporthi")
@JsonIgnore
public Optional getPassporthi() {
return Optional.ofNullable(passporthi);
}
public Systemuser withPassporthi(Integer passporthi) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("passporthi");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.passporthi = passporthi;
return _x;
}
@Property(name="address2_name")
@JsonIgnore
public Optional getAddress2_name() {
return Optional.ofNullable(address2_name);
}
public Systemuser withAddress2_name(String address2_name) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_name");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_name = address2_name;
return _x;
}
@Property(name="_territoryid_value")
@JsonIgnore
public Optional get_territoryid_value() {
return Optional.ofNullable(_territoryid_value);
}
public Systemuser with_territoryid_value(UUID _territoryid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_territoryid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._territoryid_value = _territoryid_value;
return _x;
}
@Property(name="address2_shippingmethodcode")
@JsonIgnore
public Optional getAddress2_shippingmethodcode() {
return Optional.ofNullable(address2_shippingmethodcode);
}
public Systemuser withAddress2_shippingmethodcode(Integer address2_shippingmethodcode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_shippingmethodcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_shippingmethodcode = address2_shippingmethodcode;
return _x;
}
@Property(name="disabledreason")
@JsonIgnore
public Optional getDisabledreason() {
return Optional.ofNullable(disabledreason);
}
public Systemuser withDisabledreason(String disabledreason) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("disabledreason");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.disabledreason = disabledreason;
return _x;
}
@Property(name="address1_postofficebox")
@JsonIgnore
public Optional getAddress1_postofficebox() {
return Optional.ofNullable(address1_postofficebox);
}
public Systemuser withAddress1_postofficebox(String address1_postofficebox) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_postofficebox = address1_postofficebox;
return _x;
}
@Property(name="address1_composite")
@JsonIgnore
public Optional getAddress1_composite() {
return Optional.ofNullable(address1_composite);
}
public Systemuser withAddress1_composite(String address1_composite) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_composite = address1_composite;
return _x;
}
@Property(name="setupuser")
@JsonIgnore
public Optional getSetupuser() {
return Optional.ofNullable(setupuser);
}
public Systemuser withSetupuser(Boolean setupuser) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("setupuser");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.setupuser = setupuser;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Systemuser withEntityimage_timestamp(Long entityimage_timestamp) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="internalemailaddress")
@JsonIgnore
public Optional getInternalemailaddress() {
return Optional.ofNullable(internalemailaddress);
}
public Systemuser withInternalemailaddress(String internalemailaddress) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("internalemailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.internalemailaddress = internalemailaddress;
return _x;
}
@Property(name="isemailaddressapprovedbyo365admin")
@JsonIgnore
public Optional getIsemailaddressapprovedbyo365admin() {
return Optional.ofNullable(isemailaddressapprovedbyo365admin);
}
public Systemuser withIsemailaddressapprovedbyo365admin(Boolean isemailaddressapprovedbyo365admin) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("isemailaddressapprovedbyo365admin");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
return _x;
}
@Property(name="address1_county")
@JsonIgnore
public Optional getAddress1_county() {
return Optional.ofNullable(address1_county);
}
public Systemuser withAddress1_county(String address1_county) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_county = address1_county;
return _x;
}
@Property(name="_businessunitid_value")
@JsonIgnore
public Optional get_businessunitid_value() {
return Optional.ofNullable(_businessunitid_value);
}
public Systemuser with_businessunitid_value(UUID _businessunitid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_businessunitid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._businessunitid_value = _businessunitid_value;
return _x;
}
@Property(name="address1_telephone1")
@JsonIgnore
public Optional getAddress1_telephone1() {
return Optional.ofNullable(address1_telephone1);
}
public Systemuser withAddress1_telephone1(String address1_telephone1) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_telephone1 = address1_telephone1;
return _x;
}
@Property(name="invitestatuscode")
@JsonIgnore
public Optional getInvitestatuscode() {
return Optional.ofNullable(invitestatuscode);
}
public Systemuser withInvitestatuscode(Integer invitestatuscode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("invitestatuscode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.invitestatuscode = invitestatuscode;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Systemuser withEntityimageid(UUID entityimageid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="address2_line3")
@JsonIgnore
public Optional getAddress2_line3() {
return Optional.ofNullable(address2_line3);
}
public Systemuser withAddress2_line3(String address2_line3) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_line3 = address2_line3;
return _x;
}
@Property(name="userlicensetype")
@JsonIgnore
public Optional getUserlicensetype() {
return Optional.ofNullable(userlicensetype);
}
public Systemuser withUserlicensetype(Integer userlicensetype) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("userlicensetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.userlicensetype = userlicensetype;
return _x;
}
@Property(name="incomingemaildeliverymethod")
@JsonIgnore
public Optional getIncomingemaildeliverymethod() {
return Optional.ofNullable(incomingemaildeliverymethod);
}
public Systemuser withIncomingemaildeliverymethod(Integer incomingemaildeliverymethod) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("incomingemaildeliverymethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
return _x;
}
@Property(name="skills")
@JsonIgnore
public Optional getSkills() {
return Optional.ofNullable(skills);
}
public Systemuser withSkills(String skills) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("skills");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.skills = skills;
return _x;
}
@Property(name="outgoingemaildeliverymethod")
@JsonIgnore
public Optional getOutgoingemaildeliverymethod() {
return Optional.ofNullable(outgoingemaildeliverymethod);
}
public Systemuser withOutgoingemaildeliverymethod(Integer outgoingemaildeliverymethod) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("outgoingemaildeliverymethod");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
return _x;
}
@Property(name="address2_postalcode")
@JsonIgnore
public Optional getAddress2_postalcode() {
return Optional.ofNullable(address2_postalcode);
}
public Systemuser withAddress2_postalcode(String address2_postalcode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_postalcode = address2_postalcode;
return _x;
}
@Property(name="passportlo")
@JsonIgnore
public Optional getPassportlo() {
return Optional.ofNullable(passportlo);
}
public Systemuser withPassportlo(Integer passportlo) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("passportlo");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.passportlo = passportlo;
return _x;
}
@Property(name="issyncwithdirectory")
@JsonIgnore
public Optional getIssyncwithdirectory() {
return Optional.ofNullable(issyncwithdirectory);
}
public Systemuser withIssyncwithdirectory(Boolean issyncwithdirectory) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("issyncwithdirectory");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.issyncwithdirectory = issyncwithdirectory;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Systemuser withImportsequencenumber(Integer importsequencenumber) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Systemuser withModifiedon(OffsetDateTime modifiedon) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="sharepointemailaddress")
@JsonIgnore
public Optional getSharepointemailaddress() {
return Optional.ofNullable(sharepointemailaddress);
}
public Systemuser withSharepointemailaddress(String sharepointemailaddress) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("sharepointemailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.sharepointemailaddress = sharepointemailaddress;
return _x;
}
@Property(name="yammeruserid")
@JsonIgnore
public Optional getYammeruserid() {
return Optional.ofNullable(yammeruserid);
}
public Systemuser withYammeruserid(String yammeruserid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yammeruserid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yammeruserid = yammeruserid;
return _x;
}
@Property(name="address1_longitude")
@JsonIgnore
public Optional getAddress1_longitude() {
return Optional.ofNullable(address1_longitude);
}
public Systemuser withAddress1_longitude(Double address1_longitude) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_longitude");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_longitude = address1_longitude;
return _x;
}
@Property(name="defaultfilterspopulated")
@JsonIgnore
public Optional getDefaultfilterspopulated() {
return Optional.ofNullable(defaultfilterspopulated);
}
public Systemuser withDefaultfilterspopulated(Boolean defaultfilterspopulated) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("defaultfilterspopulated");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.defaultfilterspopulated = defaultfilterspopulated;
return _x;
}
@Property(name="stageid")
@JsonIgnore
public Optional getStageid() {
return Optional.ofNullable(stageid);
}
public Systemuser withStageid(UUID stageid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("stageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.stageid = stageid;
return _x;
}
@Property(name="isintegrationuser")
@JsonIgnore
public Optional getIsintegrationuser() {
return Optional.ofNullable(isintegrationuser);
}
public Systemuser withIsintegrationuser(Boolean isintegrationuser) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("isintegrationuser");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.isintegrationuser = isintegrationuser;
return _x;
}
@Property(name="personalemailaddress")
@JsonIgnore
public Optional getPersonalemailaddress() {
return Optional.ofNullable(personalemailaddress);
}
public Systemuser withPersonalemailaddress(String personalemailaddress) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("personalemailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.personalemailaddress = personalemailaddress;
return _x;
}
@Property(name="utcconversiontimezonecode")
@JsonIgnore
public Optional getUtcconversiontimezonecode() {
return Optional.ofNullable(utcconversiontimezonecode);
}
public Systemuser withUtcconversiontimezonecode(Integer utcconversiontimezonecode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("utcconversiontimezonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.utcconversiontimezonecode = utcconversiontimezonecode;
return _x;
}
@Property(name="address2_telephone2")
@JsonIgnore
public Optional getAddress2_telephone2() {
return Optional.ofNullable(address2_telephone2);
}
public Systemuser withAddress2_telephone2(String address2_telephone2) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_telephone2 = address2_telephone2;
return _x;
}
@Property(name="preferredemailcode")
@JsonIgnore
public Optional getPreferredemailcode() {
return Optional.ofNullable(preferredemailcode);
}
public Systemuser withPreferredemailcode(Integer preferredemailcode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("preferredemailcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.preferredemailcode = preferredemailcode;
return _x;
}
@Property(name="address2_composite")
@JsonIgnore
public Optional getAddress2_composite() {
return Optional.ofNullable(address2_composite);
}
public Systemuser withAddress2_composite(String address2_composite) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_composite");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_composite = address2_composite;
return _x;
}
@Property(name="preferredphonecode")
@JsonIgnore
public Optional getPreferredphonecode() {
return Optional.ofNullable(preferredphonecode);
}
public Systemuser withPreferredphonecode(Integer preferredphonecode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("preferredphonecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.preferredphonecode = preferredphonecode;
return _x;
}
@Property(name="_mobileofflineprofileid_value")
@JsonIgnore
public Optional get_mobileofflineprofileid_value() {
return Optional.ofNullable(_mobileofflineprofileid_value);
}
public Systemuser with_mobileofflineprofileid_value(UUID _mobileofflineprofileid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_mobileofflineprofileid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._mobileofflineprofileid_value = _mobileofflineprofileid_value;
return _x;
}
@Property(name="windowsliveid")
@JsonIgnore
public Optional getWindowsliveid() {
return Optional.ofNullable(windowsliveid);
}
public Systemuser withWindowsliveid(String windowsliveid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("windowsliveid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.windowsliveid = windowsliveid;
return _x;
}
@Property(name="address1_line1")
@JsonIgnore
public Optional getAddress1_line1() {
return Optional.ofNullable(address1_line1);
}
public Systemuser withAddress1_line1(String address1_line1) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_line1 = address1_line1;
return _x;
}
@Property(name="yomimiddlename")
@JsonIgnore
public Optional getYomimiddlename() {
return Optional.ofNullable(yomimiddlename);
}
public Systemuser withYomimiddlename(String yomimiddlename) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yomimiddlename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yomimiddlename = yomimiddlename;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Systemuser withEntityimage(byte[] entityimage) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.entityimage = entityimage;
return _x;
}
@Property(name="emailrouteraccessapproval")
@JsonIgnore
public Optional getEmailrouteraccessapproval() {
return Optional.ofNullable(emailrouteraccessapproval);
}
public Systemuser withEmailrouteraccessapproval(Integer emailrouteraccessapproval) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("emailrouteraccessapproval");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.emailrouteraccessapproval = emailrouteraccessapproval;
return _x;
}
@Property(name="_calendarid_value")
@JsonIgnore
public Optional get_calendarid_value() {
return Optional.ofNullable(_calendarid_value);
}
public Systemuser with_calendarid_value(UUID _calendarid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_calendarid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._calendarid_value = _calendarid_value;
return _x;
}
@Property(name="address1_line3")
@JsonIgnore
public Optional getAddress1_line3() {
return Optional.ofNullable(address1_line3);
}
public Systemuser withAddress1_line3(String address1_line3) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_line3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_line3 = address1_line3;
return _x;
}
@Property(name="yomifirstname")
@JsonIgnore
public Optional getYomifirstname() {
return Optional.ofNullable(yomifirstname);
}
public Systemuser withYomifirstname(String yomifirstname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yomifirstname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yomifirstname = yomifirstname;
return _x;
}
@Property(name="address2_country")
@JsonIgnore
public Optional getAddress2_country() {
return Optional.ofNullable(address2_country);
}
public Systemuser withAddress2_country(String address2_country) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_country = address2_country;
return _x;
}
@Property(name="fullname")
@JsonIgnore
public Optional getFullname() {
return Optional.ofNullable(fullname);
}
public Systemuser withFullname(String fullname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("fullname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.fullname = fullname;
return _x;
}
@Property(name="azureactivedirectoryobjectid")
@JsonIgnore
public Optional getAzureactivedirectoryobjectid() {
return Optional.ofNullable(azureactivedirectoryobjectid);
}
public Systemuser withAzureactivedirectoryobjectid(UUID azureactivedirectoryobjectid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("azureactivedirectoryobjectid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.azureactivedirectoryobjectid = azureactivedirectoryobjectid;
return _x;
}
@Property(name="systemuserid")
@JsonIgnore
public Optional getSystemuserid() {
return Optional.ofNullable(systemuserid);
}
public Systemuser withSystemuserid(UUID systemuserid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("systemuserid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.systemuserid = systemuserid;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Systemuser withEntityimage_url(String entityimage_url) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="address1_line2")
@JsonIgnore
public Optional getAddress1_line2() {
return Optional.ofNullable(address1_line2);
}
public Systemuser withAddress1_line2(String address1_line2) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_line2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_line2 = address1_line2;
return _x;
}
@Property(name="address2_upszone")
@JsonIgnore
public Optional getAddress2_upszone() {
return Optional.ofNullable(address2_upszone);
}
public Systemuser withAddress2_upszone(String address2_upszone) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_upszone");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_upszone = address2_upszone;
return _x;
}
@Property(name="address1_city")
@JsonIgnore
public Optional getAddress1_city() {
return Optional.ofNullable(address1_city);
}
public Systemuser withAddress1_city(String address1_city) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_city");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_city = address1_city;
return _x;
}
@Property(name="address2_fax")
@JsonIgnore
public Optional getAddress2_fax() {
return Optional.ofNullable(address2_fax);
}
public Systemuser withAddress2_fax(String address2_fax) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_fax");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_fax = address2_fax;
return _x;
}
@Property(name="_positionid_value")
@JsonIgnore
public Optional get_positionid_value() {
return Optional.ofNullable(_positionid_value);
}
public Systemuser with_positionid_value(UUID _positionid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_positionid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._positionid_value = _positionid_value;
return _x;
}
@Property(name="address2_line1")
@JsonIgnore
public Optional getAddress2_line1() {
return Optional.ofNullable(address2_line1);
}
public Systemuser withAddress2_line1(String address2_line1) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_line1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_line1 = address2_line1;
return _x;
}
@Property(name="_transactioncurrencyid_value")
@JsonIgnore
public Optional get_transactioncurrencyid_value() {
return Optional.ofNullable(_transactioncurrencyid_value);
}
public Systemuser with_transactioncurrencyid_value(UUID _transactioncurrencyid_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_transactioncurrencyid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
return _x;
}
@Property(name="address2_telephone1")
@JsonIgnore
public Optional getAddress2_telephone1() {
return Optional.ofNullable(address2_telephone1);
}
public Systemuser withAddress2_telephone1(String address2_telephone1) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_telephone1");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_telephone1 = address2_telephone1;
return _x;
}
@Property(name="middlename")
@JsonIgnore
public Optional getMiddlename() {
return Optional.ofNullable(middlename);
}
public Systemuser withMiddlename(String middlename) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("middlename");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.middlename = middlename;
return _x;
}
@Property(name="isdisabled")
@JsonIgnore
public Optional getIsdisabled() {
return Optional.ofNullable(isdisabled);
}
public Systemuser withIsdisabled(Boolean isdisabled) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("isdisabled");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.isdisabled = isdisabled;
return _x;
}
@Property(name="_defaultmailbox_value")
@JsonIgnore
public Optional get_defaultmailbox_value() {
return Optional.ofNullable(_defaultmailbox_value);
}
public Systemuser with_defaultmailbox_value(UUID _defaultmailbox_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_defaultmailbox_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._defaultmailbox_value = _defaultmailbox_value;
return _x;
}
@Property(name="address1_postalcode")
@JsonIgnore
public Optional getAddress1_postalcode() {
return Optional.ofNullable(address1_postalcode);
}
public Systemuser withAddress1_postalcode(String address1_postalcode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_postalcode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_postalcode = address1_postalcode;
return _x;
}
@Property(name="employeeid")
@JsonIgnore
public Optional getEmployeeid() {
return Optional.ofNullable(employeeid);
}
public Systemuser withEmployeeid(String employeeid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("employeeid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.employeeid = employeeid;
return _x;
}
@Property(name="lastname")
@JsonIgnore
public Optional getLastname() {
return Optional.ofNullable(lastname);
}
public Systemuser withLastname(String lastname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("lastname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.lastname = lastname;
return _x;
}
@Property(name="mobilealertemail")
@JsonIgnore
public Optional getMobilealertemail() {
return Optional.ofNullable(mobilealertemail);
}
public Systemuser withMobilealertemail(String mobilealertemail) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("mobilealertemail");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.mobilealertemail = mobilealertemail;
return _x;
}
@Property(name="timezoneruleversionnumber")
@JsonIgnore
public Optional getTimezoneruleversionnumber() {
return Optional.ofNullable(timezoneruleversionnumber);
}
public Systemuser withTimezoneruleversionnumber(Integer timezoneruleversionnumber) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("timezoneruleversionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.timezoneruleversionnumber = timezoneruleversionnumber;
return _x;
}
@Property(name="identityid")
@JsonIgnore
public Optional getIdentityid() {
return Optional.ofNullable(identityid);
}
public Systemuser withIdentityid(Integer identityid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("identityid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.identityid = identityid;
return _x;
}
@Property(name="traversedpath")
@JsonIgnore
public Optional getTraversedpath() {
return Optional.ofNullable(traversedpath);
}
public Systemuser withTraversedpath(String traversedpath) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("traversedpath");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.traversedpath = traversedpath;
return _x;
}
@Property(name="address2_county")
@JsonIgnore
public Optional getAddress2_county() {
return Optional.ofNullable(address2_county);
}
public Systemuser withAddress2_county(String address2_county) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_county");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_county = address2_county;
return _x;
}
@Property(name="address1_addresstypecode")
@JsonIgnore
public Optional getAddress1_addresstypecode() {
return Optional.ofNullable(address1_addresstypecode);
}
public Systemuser withAddress1_addresstypecode(Integer address1_addresstypecode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_addresstypecode = address1_addresstypecode;
return _x;
}
@Property(name="address2_telephone3")
@JsonIgnore
public Optional getAddress2_telephone3() {
return Optional.ofNullable(address2_telephone3);
}
public Systemuser withAddress2_telephone3(String address2_telephone3) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_telephone3");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_telephone3 = address2_telephone3;
return _x;
}
@Property(name="yomilastname")
@JsonIgnore
public Optional getYomilastname() {
return Optional.ofNullable(yomilastname);
}
public Systemuser withYomilastname(String yomilastname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yomilastname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yomilastname = yomilastname;
return _x;
}
@Property(name="displayinserviceviews")
@JsonIgnore
public Optional getDisplayinserviceviews() {
return Optional.ofNullable(displayinserviceviews);
}
public Systemuser withDisplayinserviceviews(Boolean displayinserviceviews) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("displayinserviceviews");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.displayinserviceviews = displayinserviceviews;
return _x;
}
@Property(name="yomifullname")
@JsonIgnore
public Optional getYomifullname() {
return Optional.ofNullable(yomifullname);
}
public Systemuser withYomifullname(String yomifullname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yomifullname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yomifullname = yomifullname;
return _x;
}
@Property(name="address2_addresstypecode")
@JsonIgnore
public Optional getAddress2_addresstypecode() {
return Optional.ofNullable(address2_addresstypecode);
}
public Systemuser withAddress2_addresstypecode(Integer address2_addresstypecode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_addresstypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_addresstypecode = address2_addresstypecode;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Systemuser withCreatedon(OffsetDateTime createdon) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.createdon = createdon;
return _x;
}
@Property(name="accessmode")
@JsonIgnore
public Optional getAccessmode() {
return Optional.ofNullable(accessmode);
}
public Systemuser withAccessmode(Integer accessmode) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("accessmode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.accessmode = accessmode;
return _x;
}
@Property(name="yammeremailaddress")
@JsonIgnore
public Optional getYammeremailaddress() {
return Optional.ofNullable(yammeremailaddress);
}
public Systemuser withYammeremailaddress(String yammeremailaddress) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("yammeremailaddress");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.yammeremailaddress = yammeremailaddress;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Systemuser with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="exchangerate")
@JsonIgnore
public Optional getExchangerate() {
return Optional.ofNullable(exchangerate);
}
public Systemuser withExchangerate(BigDecimal exchangerate) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("exchangerate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.exchangerate = exchangerate;
return _x;
}
@Property(name="jobtitle")
@JsonIgnore
public Optional getJobtitle() {
return Optional.ofNullable(jobtitle);
}
public Systemuser withJobtitle(String jobtitle) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("jobtitle");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.jobtitle = jobtitle;
return _x;
}
@Property(name="address2_postofficebox")
@JsonIgnore
public Optional getAddress2_postofficebox() {
return Optional.ofNullable(address2_postofficebox);
}
public Systemuser withAddress2_postofficebox(String address2_postofficebox) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_postofficebox");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_postofficebox = address2_postofficebox;
return _x;
}
@Property(name="caltype")
@JsonIgnore
public Optional getCaltype() {
return Optional.ofNullable(caltype);
}
public Systemuser withCaltype(Integer caltype) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("caltype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.caltype = caltype;
return _x;
}
@Property(name="address2_addressid")
@JsonIgnore
public Optional getAddress2_addressid() {
return Optional.ofNullable(address2_addressid);
}
public Systemuser withAddress2_addressid(UUID address2_addressid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_addressid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_addressid = address2_addressid;
return _x;
}
@Property(name="processid")
@JsonIgnore
public Optional getProcessid() {
return Optional.ofNullable(processid);
}
public Systemuser withProcessid(UUID processid) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("processid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.processid = processid;
return _x;
}
@Property(name="applicationiduri")
@JsonIgnore
public Optional getApplicationiduri() {
return Optional.ofNullable(applicationiduri);
}
public Systemuser withApplicationiduri(String applicationiduri) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("applicationiduri");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.applicationiduri = applicationiduri;
return _x;
}
@Property(name="address2_utcoffset")
@JsonIgnore
public Optional getAddress2_utcoffset() {
return Optional.ofNullable(address2_utcoffset);
}
public Systemuser withAddress2_utcoffset(Integer address2_utcoffset) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address2_utcoffset");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address2_utcoffset = address2_utcoffset;
return _x;
}
@Property(name="islicensed")
@JsonIgnore
public Optional getIslicensed() {
return Optional.ofNullable(islicensed);
}
public Systemuser withIslicensed(Boolean islicensed) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("islicensed");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.islicensed = islicensed;
return _x;
}
@Property(name="address1_telephone2")
@JsonIgnore
public Optional getAddress1_telephone2() {
return Optional.ofNullable(address1_telephone2);
}
public Systemuser withAddress1_telephone2(String address1_telephone2) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_telephone2");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_telephone2 = address1_telephone2;
return _x;
}
@Property(name="address1_country")
@JsonIgnore
public Optional getAddress1_country() {
return Optional.ofNullable(address1_country);
}
public Systemuser withAddress1_country(String address1_country) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("address1_country");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.address1_country = address1_country;
return _x;
}
@Property(name="domainname")
@JsonIgnore
public Optional getDomainname() {
return Optional.ofNullable(domainname);
}
public Systemuser withDomainname(String domainname) {
Systemuser _x = _copy();
_x.changedFields = changedFields.add("domainname");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.systemuser");
_x.domainname = domainname;
return _x;
}
public Systemuser withUnmappedField(String name, Object value) {
Systemuser _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="systemuserroles_association")
@JsonIgnore
public RoleCollectionRequest getSystemuserroles_association() {
return new RoleCollectionRequest(
contextPath.addSegment("systemuserroles_association"), RequestHelper.getValue(unmappedFields, "systemuserroles_association"));
}
@NavigationProperty(name="teammembership_association")
@JsonIgnore
public TeamCollectionRequest getTeammembership_association() {
return new TeamCollectionRequest(
contextPath.addSegment("teammembership_association"), RequestHelper.getValue(unmappedFields, "teammembership_association"));
}
@NavigationProperty(name="systemuser_principalobjectattributeaccess_principalid")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getSystemuser_principalobjectattributeaccess_principalid() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("systemuser_principalobjectattributeaccess_principalid"), RequestHelper.getValue(unmappedFields, "systemuser_principalobjectattributeaccess_principalid"));
}
@NavigationProperty(name="user_exchangesyncidmapping")
@JsonIgnore
public ExchangesyncidmappingCollectionRequest getUser_exchangesyncidmapping() {
return new ExchangesyncidmappingCollectionRequest(
contextPath.addSegment("user_exchangesyncidmapping"), RequestHelper.getValue(unmappedFields, "user_exchangesyncidmapping"));
}
@NavigationProperty(name="lk_theme_createdby")
@JsonIgnore
public ThemeCollectionRequest getLk_theme_createdby() {
return new ThemeCollectionRequest(
contextPath.addSegment("lk_theme_createdby"), RequestHelper.getValue(unmappedFields, "lk_theme_createdby"));
}
@NavigationProperty(name="lk_theme_createdonbehalfby")
@JsonIgnore
public ThemeCollectionRequest getLk_theme_createdonbehalfby() {
return new ThemeCollectionRequest(
contextPath.addSegment("lk_theme_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_theme_createdonbehalfby"));
}
@NavigationProperty(name="lk_theme_modifiedby")
@JsonIgnore
public ThemeCollectionRequest getLk_theme_modifiedby() {
return new ThemeCollectionRequest(
contextPath.addSegment("lk_theme_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_theme_modifiedby"));
}
@NavigationProperty(name="lk_theme_modifiedonbehalfby")
@JsonIgnore
public ThemeCollectionRequest getLk_theme_modifiedonbehalfby() {
return new ThemeCollectionRequest(
contextPath.addSegment("lk_theme_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_theme_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_usermapping_createdby")
@JsonIgnore
public UsermappingCollectionRequest getLk_usermapping_createdby() {
return new UsermappingCollectionRequest(
contextPath.addSegment("lk_usermapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_usermapping_createdby"));
}
@NavigationProperty(name="lk_usermapping_createdonbehalfby")
@JsonIgnore
public UsermappingCollectionRequest getLk_usermapping_createdonbehalfby() {
return new UsermappingCollectionRequest(
contextPath.addSegment("lk_usermapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_usermapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_usermapping_modifiedby")
@JsonIgnore
public UsermappingCollectionRequest getLk_usermapping_modifiedby() {
return new UsermappingCollectionRequest(
contextPath.addSegment("lk_usermapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_usermapping_modifiedby"));
}
@NavigationProperty(name="lk_usermapping_modifiedonbehalfby")
@JsonIgnore
public UsermappingCollectionRequest getLk_usermapping_modifiedonbehalfby() {
return new UsermappingCollectionRequest(
contextPath.addSegment("lk_usermapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_usermapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_new_interactionforemail_createdby")
@JsonIgnore
public InteractionforemailCollectionRequest getLk_new_interactionforemail_createdby() {
return new InteractionforemailCollectionRequest(
contextPath.addSegment("lk_new_interactionforemail_createdby"), RequestHelper.getValue(unmappedFields, "lk_new_interactionforemail_createdby"));
}
@NavigationProperty(name="lk_new_interactionforemail_createdonbehalfby")
@JsonIgnore
public InteractionforemailCollectionRequest getLk_new_interactionforemail_createdonbehalfby() {
return new InteractionforemailCollectionRequest(
contextPath.addSegment("lk_new_interactionforemail_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_new_interactionforemail_createdonbehalfby"));
}
@NavigationProperty(name="lk_new_interactionforemail_modifiedby")
@JsonIgnore
public InteractionforemailCollectionRequest getLk_new_interactionforemail_modifiedby() {
return new InteractionforemailCollectionRequest(
contextPath.addSegment("lk_new_interactionforemail_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_new_interactionforemail_modifiedby"));
}
@NavigationProperty(name="lk_new_interactionforemail_modifiedonbehalfby")
@JsonIgnore
public InteractionforemailCollectionRequest getLk_new_interactionforemail_modifiedonbehalfby() {
return new InteractionforemailCollectionRequest(
contextPath.addSegment("lk_new_interactionforemail_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_new_interactionforemail_modifiedonbehalfby"));
}
@NavigationProperty(name="user_new_interactionforemail")
@JsonIgnore
public InteractionforemailCollectionRequest getUser_new_interactionforemail() {
return new InteractionforemailCollectionRequest(
contextPath.addSegment("user_new_interactionforemail"), RequestHelper.getValue(unmappedFields, "user_new_interactionforemail"));
}
@NavigationProperty(name="lk_knowledgearticle_createdby")
@JsonIgnore
public KnowledgearticleCollectionRequest getLk_knowledgearticle_createdby() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("lk_knowledgearticle_createdby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticle_createdby"));
}
@NavigationProperty(name="lk_knowledgearticle_createdonbehalfby")
@JsonIgnore
public KnowledgearticleCollectionRequest getLk_knowledgearticle_createdonbehalfby() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("lk_knowledgearticle_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticle_createdonbehalfby"));
}
@NavigationProperty(name="lk_knowledgearticle_modifiedby")
@JsonIgnore
public KnowledgearticleCollectionRequest getLk_knowledgearticle_modifiedby() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("lk_knowledgearticle_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticle_modifiedby"));
}
@NavigationProperty(name="lk_knowledgearticle_modifiedonbehalfby")
@JsonIgnore
public KnowledgearticleCollectionRequest getLk_knowledgearticle_modifiedonbehalfby() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("lk_knowledgearticle_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticle_modifiedonbehalfby"));
}
@NavigationProperty(name="user_knowledgearticle")
@JsonIgnore
public KnowledgearticleCollectionRequest getUser_knowledgearticle() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("user_knowledgearticle"), RequestHelper.getValue(unmappedFields, "user_knowledgearticle"));
}
@NavigationProperty(name="user_sharepointsite")
@JsonIgnore
public SharepointsiteCollectionRequest getUser_sharepointsite() {
return new SharepointsiteCollectionRequest(
contextPath.addSegment("user_sharepointsite"), RequestHelper.getValue(unmappedFields, "user_sharepointsite"));
}
@NavigationProperty(name="user_sharepointdocumentlocation")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getUser_sharepointdocumentlocation() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("user_sharepointdocumentlocation"), RequestHelper.getValue(unmappedFields, "user_sharepointdocumentlocation"));
}
@NavigationProperty(name="lk_goal_createdby")
@JsonIgnore
public GoalCollectionRequest getLk_goal_createdby() {
return new GoalCollectionRequest(
contextPath.addSegment("lk_goal_createdby"), RequestHelper.getValue(unmappedFields, "lk_goal_createdby"));
}
@NavigationProperty(name="lk_goal_createdonbehalfby")
@JsonIgnore
public GoalCollectionRequest getLk_goal_createdonbehalfby() {
return new GoalCollectionRequest(
contextPath.addSegment("lk_goal_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_goal_createdonbehalfby"));
}
@NavigationProperty(name="lk_goal_modifiedby")
@JsonIgnore
public GoalCollectionRequest getLk_goal_modifiedby() {
return new GoalCollectionRequest(
contextPath.addSegment("lk_goal_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_goal_modifiedby"));
}
@NavigationProperty(name="lk_goal_modifiedonbehalfby")
@JsonIgnore
public GoalCollectionRequest getLk_goal_modifiedonbehalfby() {
return new GoalCollectionRequest(
contextPath.addSegment("lk_goal_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_goal_modifiedonbehalfby"));
}
@NavigationProperty(name="user_goal")
@JsonIgnore
public GoalCollectionRequest getUser_goal() {
return new GoalCollectionRequest(
contextPath.addSegment("user_goal"), RequestHelper.getValue(unmappedFields, "user_goal"));
}
@NavigationProperty(name="user_goal_goalowner")
@JsonIgnore
public GoalCollectionRequest getUser_goal_goalowner() {
return new GoalCollectionRequest(
contextPath.addSegment("user_goal_goalowner"), RequestHelper.getValue(unmappedFields, "user_goal_goalowner"));
}
@NavigationProperty(name="lk_metric_createdby")
@JsonIgnore
public MetricCollectionRequest getLk_metric_createdby() {
return new MetricCollectionRequest(
contextPath.addSegment("lk_metric_createdby"), RequestHelper.getValue(unmappedFields, "lk_metric_createdby"));
}
@NavigationProperty(name="lk_metric_createdonbehalfby")
@JsonIgnore
public MetricCollectionRequest getLk_metric_createdonbehalfby() {
return new MetricCollectionRequest(
contextPath.addSegment("lk_metric_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_metric_createdonbehalfby"));
}
@NavigationProperty(name="lk_metric_modifiedby")
@JsonIgnore
public MetricCollectionRequest getLk_metric_modifiedby() {
return new MetricCollectionRequest(
contextPath.addSegment("lk_metric_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_metric_modifiedby"));
}
@NavigationProperty(name="lk_metric_modifiedonbehalfby")
@JsonIgnore
public MetricCollectionRequest getLk_metric_modifiedonbehalfby() {
return new MetricCollectionRequest(
contextPath.addSegment("lk_metric_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_metric_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_rollupfield_createdby")
@JsonIgnore
public RollupfieldCollectionRequest getLk_rollupfield_createdby() {
return new RollupfieldCollectionRequest(
contextPath.addSegment("lk_rollupfield_createdby"), RequestHelper.getValue(unmappedFields, "lk_rollupfield_createdby"));
}
@NavigationProperty(name="lk_rollupfield_createdonbehalfby")
@JsonIgnore
public RollupfieldCollectionRequest getLk_rollupfield_createdonbehalfby() {
return new RollupfieldCollectionRequest(
contextPath.addSegment("lk_rollupfield_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_rollupfield_createdonbehalfby"));
}
@NavigationProperty(name="lk_rollupfield_modifiedby")
@JsonIgnore
public RollupfieldCollectionRequest getLk_rollupfield_modifiedby() {
return new RollupfieldCollectionRequest(
contextPath.addSegment("lk_rollupfield_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_rollupfield_modifiedby"));
}
@NavigationProperty(name="lk_rollupfield_modifiedonbehalfby")
@JsonIgnore
public RollupfieldCollectionRequest getLk_rollupfield_modifiedonbehalfby() {
return new RollupfieldCollectionRequest(
contextPath.addSegment("lk_rollupfield_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_rollupfield_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_goalrollupquery_createdby")
@JsonIgnore
public GoalrollupqueryCollectionRequest getLk_goalrollupquery_createdby() {
return new GoalrollupqueryCollectionRequest(
contextPath.addSegment("lk_goalrollupquery_createdby"), RequestHelper.getValue(unmappedFields, "lk_goalrollupquery_createdby"));
}
@NavigationProperty(name="lk_goalrollupquery_createdonbehalfby")
@JsonIgnore
public GoalrollupqueryCollectionRequest getLk_goalrollupquery_createdonbehalfby() {
return new GoalrollupqueryCollectionRequest(
contextPath.addSegment("lk_goalrollupquery_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_goalrollupquery_createdonbehalfby"));
}
@NavigationProperty(name="lk_goalrollupquery_modifiedby")
@JsonIgnore
public GoalrollupqueryCollectionRequest getLk_goalrollupquery_modifiedby() {
return new GoalrollupqueryCollectionRequest(
contextPath.addSegment("lk_goalrollupquery_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_goalrollupquery_modifiedby"));
}
@NavigationProperty(name="lk_goalrollupquery_modifiedonbehalfby")
@JsonIgnore
public GoalrollupqueryCollectionRequest getLk_goalrollupquery_modifiedonbehalfby() {
return new GoalrollupqueryCollectionRequest(
contextPath.addSegment("lk_goalrollupquery_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_goalrollupquery_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_emailserverprofile_createdonbehalfby")
@JsonIgnore
public EmailserverprofileCollectionRequest getLk_emailserverprofile_createdonbehalfby() {
return new EmailserverprofileCollectionRequest(
contextPath.addSegment("lk_emailserverprofile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_emailserverprofile_createdonbehalfby"));
}
@NavigationProperty(name="lk_emailserverprofile_modifiedonbehalfby")
@JsonIgnore
public EmailserverprofileCollectionRequest getLk_emailserverprofile_modifiedonbehalfby() {
return new EmailserverprofileCollectionRequest(
contextPath.addSegment("lk_emailserverprofile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_emailserverprofile_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_mailbox_createdby")
@JsonIgnore
public MailboxCollectionRequest getLk_mailbox_createdby() {
return new MailboxCollectionRequest(
contextPath.addSegment("lk_mailbox_createdby"), RequestHelper.getValue(unmappedFields, "lk_mailbox_createdby"));
}
@NavigationProperty(name="lk_mailbox_createdonbehalfby")
@JsonIgnore
public MailboxCollectionRequest getLk_mailbox_createdonbehalfby() {
return new MailboxCollectionRequest(
contextPath.addSegment("lk_mailbox_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailbox_createdonbehalfby"));
}
@NavigationProperty(name="lk_mailbox_modifiedby")
@JsonIgnore
public MailboxCollectionRequest getLk_mailbox_modifiedby() {
return new MailboxCollectionRequest(
contextPath.addSegment("lk_mailbox_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_mailbox_modifiedby"));
}
@NavigationProperty(name="lk_mailbox_modifiedonbehalfby")
@JsonIgnore
public MailboxCollectionRequest getLk_mailbox_modifiedonbehalfby() {
return new MailboxCollectionRequest(
contextPath.addSegment("lk_mailbox_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailbox_modifiedonbehalfby"));
}
@NavigationProperty(name="user_mailbox")
@JsonIgnore
public MailboxCollectionRequest getUser_mailbox() {
return new MailboxCollectionRequest(
contextPath.addSegment("user_mailbox"), RequestHelper.getValue(unmappedFields, "user_mailbox"));
}
@NavigationProperty(name="mailbox_regarding_systemuser")
@JsonIgnore
public MailboxCollectionRequest getMailbox_regarding_systemuser() {
return new MailboxCollectionRequest(
contextPath.addSegment("mailbox_regarding_systemuser"), RequestHelper.getValue(unmappedFields, "mailbox_regarding_systemuser"));
}
@NavigationProperty(name="defaultmailbox")
@JsonIgnore
public MailboxRequest getDefaultmailbox() {
return new MailboxRequest(contextPath.addSegment("defaultmailbox"), RequestHelper.getValue(unmappedFields, "defaultmailbox"));
}
@NavigationProperty(name="lk_post_createdby")
@JsonIgnore
public PostCollectionRequest getLk_post_createdby() {
return new PostCollectionRequest(
contextPath.addSegment("lk_post_createdby"), RequestHelper.getValue(unmappedFields, "lk_post_createdby"));
}
@NavigationProperty(name="lk_post_createdonbehalfby")
@JsonIgnore
public PostCollectionRequest getLk_post_createdonbehalfby() {
return new PostCollectionRequest(
contextPath.addSegment("lk_post_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_post_createdonbehalfby"));
}
@NavigationProperty(name="lk_post_modifiedby")
@JsonIgnore
public PostCollectionRequest getLk_post_modifiedby() {
return new PostCollectionRequest(
contextPath.addSegment("lk_post_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_post_modifiedby"));
}
@NavigationProperty(name="lk_post_modifiedonbehalfby")
@JsonIgnore
public PostCollectionRequest getLk_post_modifiedonbehalfby() {
return new PostCollectionRequest(
contextPath.addSegment("lk_post_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_post_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_position_createdby")
@JsonIgnore
public PositionCollectionRequest getLk_position_createdby() {
return new PositionCollectionRequest(
contextPath.addSegment("lk_position_createdby"), RequestHelper.getValue(unmappedFields, "lk_position_createdby"));
}
@NavigationProperty(name="lk_position_createdonbehalfby")
@JsonIgnore
public PositionCollectionRequest getLk_position_createdonbehalfby() {
return new PositionCollectionRequest(
contextPath.addSegment("lk_position_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_position_createdonbehalfby"));
}
@NavigationProperty(name="lk_position_modifiedby")
@JsonIgnore
public PositionCollectionRequest getLk_position_modifiedby() {
return new PositionCollectionRequest(
contextPath.addSegment("lk_position_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_position_modifiedby"));
}
@NavigationProperty(name="lk_position_modifiedonbehalfby")
@JsonIgnore
public PositionCollectionRequest getLk_position_modifiedonbehalfby() {
return new PositionCollectionRequest(
contextPath.addSegment("lk_position_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_position_modifiedonbehalfby"));
}
@NavigationProperty(name="positionid")
@JsonIgnore
public PositionRequest getPositionid() {
return new PositionRequest(contextPath.addSegment("positionid"), RequestHelper.getValue(unmappedFields, "positionid"));
}
@NavigationProperty(name="lk_solution_createdby")
@JsonIgnore
public SolutionCollectionRequest getLk_solution_createdby() {
return new SolutionCollectionRequest(
contextPath.addSegment("lk_solution_createdby"), RequestHelper.getValue(unmappedFields, "lk_solution_createdby"));
}
@NavigationProperty(name="lk_solution_modifiedby")
@JsonIgnore
public SolutionCollectionRequest getLk_solution_modifiedby() {
return new SolutionCollectionRequest(
contextPath.addSegment("lk_solution_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_solution_modifiedby"));
}
@NavigationProperty(name="lk_publisher_createdby")
@JsonIgnore
public PublisherCollectionRequest getLk_publisher_createdby() {
return new PublisherCollectionRequest(
contextPath.addSegment("lk_publisher_createdby"), RequestHelper.getValue(unmappedFields, "lk_publisher_createdby"));
}
@NavigationProperty(name="lk_publisher_modifiedby")
@JsonIgnore
public PublisherCollectionRequest getLk_publisher_modifiedby() {
return new PublisherCollectionRequest(
contextPath.addSegment("lk_publisher_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_publisher_modifiedby"));
}
@NavigationProperty(name="lk_officegraphdocument_createdonbehalfby")
@JsonIgnore
public OfficegraphdocumentCollectionRequest getLk_officegraphdocument_createdonbehalfby() {
return new OfficegraphdocumentCollectionRequest(
contextPath.addSegment("lk_officegraphdocument_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_officegraphdocument_createdonbehalfby"));
}
@NavigationProperty(name="lk_officegraphdocument_modifiedonbehalfby")
@JsonIgnore
public OfficegraphdocumentCollectionRequest getLk_officegraphdocument_modifiedonbehalfby() {
return new OfficegraphdocumentCollectionRequest(
contextPath.addSegment("lk_officegraphdocument_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_officegraphdocument_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_similarityrule_createdonbehalfby")
@JsonIgnore
public SimilarityruleCollectionRequest getLk_similarityrule_createdonbehalfby() {
return new SimilarityruleCollectionRequest(
contextPath.addSegment("lk_similarityrule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_similarityrule_createdonbehalfby"));
}
@NavigationProperty(name="lk_similarityrule_modifiedonbehalfby")
@JsonIgnore
public SimilarityruleCollectionRequest getLk_similarityrule_modifiedonbehalfby() {
return new SimilarityruleCollectionRequest(
contextPath.addSegment("lk_similarityrule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_similarityrule_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_recommendeddocument_createdby")
@JsonIgnore
public RecommendeddocumentCollectionRequest getLk_recommendeddocument_createdby() {
return new RecommendeddocumentCollectionRequest(
contextPath.addSegment("lk_recommendeddocument_createdby"), RequestHelper.getValue(unmappedFields, "lk_recommendeddocument_createdby"));
}
@NavigationProperty(name="lk_recommendeddocument_createdonbehalfby")
@JsonIgnore
public RecommendeddocumentCollectionRequest getLk_recommendeddocument_createdonbehalfby() {
return new RecommendeddocumentCollectionRequest(
contextPath.addSegment("lk_recommendeddocument_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recommendeddocument_createdonbehalfby"));
}
@NavigationProperty(name="lk_recommendeddocument_modifiedby")
@JsonIgnore
public RecommendeddocumentCollectionRequest getLk_recommendeddocument_modifiedby() {
return new RecommendeddocumentCollectionRequest(
contextPath.addSegment("lk_recommendeddocument_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_recommendeddocument_modifiedby"));
}
@NavigationProperty(name="lk_recommendeddocument_modifiedonbehalfby")
@JsonIgnore
public RecommendeddocumentCollectionRequest getLk_recommendeddocument_modifiedonbehalfby() {
return new RecommendeddocumentCollectionRequest(
contextPath.addSegment("lk_recommendeddocument_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recommendeddocument_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_KnowledgeBaseRecord_createdby")
@JsonIgnore
public KnowledgebaserecordCollectionRequest getLk_KnowledgeBaseRecord_createdby() {
return new KnowledgebaserecordCollectionRequest(
contextPath.addSegment("lk_KnowledgeBaseRecord_createdby"), RequestHelper.getValue(unmappedFields, "lk_KnowledgeBaseRecord_createdby"));
}
@NavigationProperty(name="lk_KnowledgeBaseRecord_createdonbehalfby")
@JsonIgnore
public KnowledgebaserecordCollectionRequest getLk_KnowledgeBaseRecord_createdonbehalfby() {
return new KnowledgebaserecordCollectionRequest(
contextPath.addSegment("lk_KnowledgeBaseRecord_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_KnowledgeBaseRecord_createdonbehalfby"));
}
@NavigationProperty(name="lk_KnowledgeBaseRecord_modifiedby")
@JsonIgnore
public KnowledgebaserecordCollectionRequest getLk_KnowledgeBaseRecord_modifiedby() {
return new KnowledgebaserecordCollectionRequest(
contextPath.addSegment("lk_KnowledgeBaseRecord_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_KnowledgeBaseRecord_modifiedby"));
}
@NavigationProperty(name="lk_KnowledgeBaseRecord_modifiedonbehalfby")
@JsonIgnore
public KnowledgebaserecordCollectionRequest getLk_KnowledgeBaseRecord_modifiedonbehalfby() {
return new KnowledgebaserecordCollectionRequest(
contextPath.addSegment("lk_KnowledgeBaseRecord_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_KnowledgeBaseRecord_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_monthlyfiscalcalendar_modifiedby")
@JsonIgnore
public MonthlyfiscalcalendarCollectionRequest getLk_monthlyfiscalcalendar_modifiedby() {
return new MonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_monthlyfiscalcalendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_monthlyfiscalcalendar_modifiedby"));
}
@NavigationProperty(name="lk_slakpiinstancebase_createdonbehalfby")
@JsonIgnore
public SlakpiinstanceCollectionRequest getLk_slakpiinstancebase_createdonbehalfby() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("lk_slakpiinstancebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slakpiinstancebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitemassocaition_modifiedonbehalfby")
@JsonIgnore
public MobileofflineprofileitemassociationCollectionRequest getLk_mobileofflineprofileitemassocaition_modifiedonbehalfby() {
return new MobileofflineprofileitemassociationCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitemassocaition_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitemassocaition_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_userqueryvisualizationbase_modifiedonbehalfby")
@JsonIgnore
public UserqueryvisualizationCollectionRequest getLk_userqueryvisualizationbase_modifiedonbehalfby() {
return new UserqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_userqueryvisualizationbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userqueryvisualizationbase_modifiedonbehalfby"));
}
@NavigationProperty(name="SystemUser_SyncError")
@JsonIgnore
public SyncerrorCollectionRequest getSystemUser_SyncError() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("SystemUser_SyncError"), RequestHelper.getValue(unmappedFields, "SystemUser_SyncError"));
}
@NavigationProperty(name="SystemUser_ImportData")
@JsonIgnore
public ImportdataCollectionRequest getSystemUser_ImportData() {
return new ImportdataCollectionRequest(
contextPath.addSegment("SystemUser_ImportData"), RequestHelper.getValue(unmappedFields, "SystemUser_ImportData"));
}
@NavigationProperty(name="lk_recurrencerulebase_createdonbehalfby")
@JsonIgnore
public RecurrenceruleCollectionRequest getLk_recurrencerulebase_createdonbehalfby() {
return new RecurrenceruleCollectionRequest(
contextPath.addSegment("lk_recurrencerulebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recurrencerulebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_fieldsecurityprofile_createdonbehalfby")
@JsonIgnore
public FieldsecurityprofileCollectionRequest getLk_fieldsecurityprofile_createdonbehalfby() {
return new FieldsecurityprofileCollectionRequest(
contextPath.addSegment("lk_fieldsecurityprofile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fieldsecurityprofile_createdonbehalfby"));
}
@NavigationProperty(name="lk_importbase_createdby")
@JsonIgnore
public ImportCollectionRequest getLk_importbase_createdby() {
return new ImportCollectionRequest(
contextPath.addSegment("lk_importbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importbase_createdby"));
}
@NavigationProperty(name="lk_slaitembase_modifiedonbehalfby")
@JsonIgnore
public SlaitemCollectionRequest getLk_slaitembase_modifiedonbehalfby() {
return new SlaitemCollectionRequest(
contextPath.addSegment("lk_slaitembase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slaitembase_modifiedonbehalfby"));
}
@NavigationProperty(name="createdby_sdkmessage")
@JsonIgnore
public SdkmessageCollectionRequest getCreatedby_sdkmessage() {
return new SdkmessageCollectionRequest(
contextPath.addSegment("createdby_sdkmessage"), RequestHelper.getValue(unmappedFields, "createdby_sdkmessage"));
}
@NavigationProperty(name="lk_processsession_canceledby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_canceledby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_canceledby"), RequestHelper.getValue(unmappedFields, "lk_processsession_canceledby"));
}
@NavigationProperty(name="lk_duplicaterule_createdonbehalfby")
@JsonIgnore
public DuplicateruleCollectionRequest getLk_duplicaterule_createdonbehalfby() {
return new DuplicateruleCollectionRequest(
contextPath.addSegment("lk_duplicaterule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterule_createdonbehalfby"));
}
@NavigationProperty(name="lk_monthlyfiscalcalendar_modifiedonbehalfby")
@JsonIgnore
public MonthlyfiscalcalendarCollectionRequest getLk_monthlyfiscalcalendar_modifiedonbehalfby() {
return new MonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_monthlyfiscalcalendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_monthlyfiscalcalendar_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_lookupmapping_modifiedby")
@JsonIgnore
public LookupmappingCollectionRequest getLk_lookupmapping_modifiedby() {
return new LookupmappingCollectionRequest(
contextPath.addSegment("lk_lookupmapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_lookupmapping_modifiedby"));
}
@NavigationProperty(name="lk_kbarticletemplatebase_createdby")
@JsonIgnore
public KbarticletemplateCollectionRequest getLk_kbarticletemplatebase_createdby() {
return new KbarticletemplateCollectionRequest(
contextPath.addSegment("lk_kbarticletemplatebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_kbarticletemplatebase_createdby"));
}
@NavigationProperty(name="lk_savedquerybase_modifiedby")
@JsonIgnore
public SavedqueryCollectionRequest getLk_savedquerybase_modifiedby() {
return new SavedqueryCollectionRequest(
contextPath.addSegment("lk_savedquerybase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_savedquerybase_modifiedby"));
}
@NavigationProperty(name="lk_teamtemplate_createdby")
@JsonIgnore
public TeamtemplateCollectionRequest getLk_teamtemplate_createdby() {
return new TeamtemplateCollectionRequest(
contextPath.addSegment("lk_teamtemplate_createdby"), RequestHelper.getValue(unmappedFields, "lk_teamtemplate_createdby"));
}
@NavigationProperty(name="lk_accountbase_createdby")
@JsonIgnore
public AccountCollectionRequest getLk_accountbase_createdby() {
return new AccountCollectionRequest(
contextPath.addSegment("lk_accountbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_accountbase_createdby"));
}
@NavigationProperty(name="createdby_pluginassembly")
@JsonIgnore
public PluginassemblyCollectionRequest getCreatedby_pluginassembly() {
return new PluginassemblyCollectionRequest(
contextPath.addSegment("createdby_pluginassembly"), RequestHelper.getValue(unmappedFields, "createdby_pluginassembly"));
}
@NavigationProperty(name="lk_kbarticle_createdonbehalfby")
@JsonIgnore
public KbarticleCollectionRequest getLk_kbarticle_createdonbehalfby() {
return new KbarticleCollectionRequest(
contextPath.addSegment("lk_kbarticle_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticle_createdonbehalfby"));
}
@NavigationProperty(name="user_userform")
@JsonIgnore
public UserformCollectionRequest getUser_userform() {
return new UserformCollectionRequest(
contextPath.addSegment("user_userform"), RequestHelper.getValue(unmappedFields, "user_userform"));
}
@NavigationProperty(name="lk_organization_modifiedonbehalfby")
@JsonIgnore
public OrganizationCollectionRequest getLk_organization_modifiedonbehalfby() {
return new OrganizationCollectionRequest(
contextPath.addSegment("lk_organization_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_organization_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_solutionbase_modifiedonbehalfby")
@JsonIgnore
public SolutionCollectionRequest getLk_solutionbase_modifiedonbehalfby() {
return new SolutionCollectionRequest(
contextPath.addSegment("lk_solutionbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutionbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_timezonerule_createdby")
@JsonIgnore
public TimezoneruleCollectionRequest getLk_timezonerule_createdby() {
return new TimezoneruleCollectionRequest(
contextPath.addSegment("lk_timezonerule_createdby"), RequestHelper.getValue(unmappedFields, "lk_timezonerule_createdby"));
}
@NavigationProperty(name="modifiedby_connection")
@JsonIgnore
public ConnectionCollectionRequest getModifiedby_connection() {
return new ConnectionCollectionRequest(
contextPath.addSegment("modifiedby_connection"), RequestHelper.getValue(unmappedFields, "modifiedby_connection"));
}
@NavigationProperty(name="systemuser_connections1")
@JsonIgnore
public ConnectionCollectionRequest getSystemuser_connections1() {
return new ConnectionCollectionRequest(
contextPath.addSegment("systemuser_connections1"), RequestHelper.getValue(unmappedFields, "systemuser_connections1"));
}
@NavigationProperty(name="lk_report_modifiedonbehalfby")
@JsonIgnore
public ReportCollectionRequest getLk_report_modifiedonbehalfby() {
return new ReportCollectionRequest(
contextPath.addSegment("lk_report_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_report_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_tracelog_createdby")
@JsonIgnore
public TracelogCollectionRequest getLk_tracelog_createdby() {
return new TracelogCollectionRequest(
contextPath.addSegment("lk_tracelog_createdby"), RequestHelper.getValue(unmappedFields, "lk_tracelog_createdby"));
}
@NavigationProperty(name="lk_calendar_createdonbehalfby")
@JsonIgnore
public CalendarCollectionRequest getLk_calendar_createdonbehalfby() {
return new CalendarCollectionRequest(
contextPath.addSegment("lk_calendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_calendar_createdonbehalfby"));
}
@NavigationProperty(name="lk_activitypointer_createdby")
@JsonIgnore
public ActivitypointerCollectionRequest getLk_activitypointer_createdby() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("lk_activitypointer_createdby"), RequestHelper.getValue(unmappedFields, "lk_activitypointer_createdby"));
}
@NavigationProperty(name="lk_queueitembase_createdby")
@JsonIgnore
public QueueitemCollectionRequest getLk_queueitembase_createdby() {
return new QueueitemCollectionRequest(
contextPath.addSegment("lk_queueitembase_createdby"), RequestHelper.getValue(unmappedFields, "lk_queueitembase_createdby"));
}
@NavigationProperty(name="systemuser_navigationsetting_modifiedby")
@JsonIgnore
public NavigationsettingCollectionRequest getSystemuser_navigationsetting_modifiedby() {
return new NavigationsettingCollectionRequest(
contextPath.addSegment("systemuser_navigationsetting_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_navigationsetting_modifiedby"));
}
@NavigationProperty(name="systemuser_SiteMap_modifiedonbehalfby")
@JsonIgnore
public SitemapCollectionRequest getSystemuser_SiteMap_modifiedonbehalfby() {
return new SitemapCollectionRequest(
contextPath.addSegment("systemuser_SiteMap_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_SiteMap_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_annotationbase_modifiedonbehalfby")
@JsonIgnore
public AnnotationCollectionRequest getLk_annotationbase_modifiedonbehalfby() {
return new AnnotationCollectionRequest(
contextPath.addSegment("lk_annotationbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_annotationbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_slakpiinstancebase_createdby")
@JsonIgnore
public SlakpiinstanceCollectionRequest getLk_slakpiinstancebase_createdby() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("lk_slakpiinstancebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_slakpiinstancebase_createdby"));
}
@NavigationProperty(name="systemuser_appmodule_modifiedonbehalfby")
@JsonIgnore
public AppmoduleCollectionRequest getSystemuser_appmodule_modifiedonbehalfby() {
return new AppmoduleCollectionRequest(
contextPath.addSegment("systemuser_appmodule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appmodule_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_role_createdonbehalfby")
@JsonIgnore
public RoleCollectionRequest getLk_role_createdonbehalfby() {
return new RoleCollectionRequest(
contextPath.addSegment("lk_role_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_role_createdonbehalfby"));
}
@NavigationProperty(name="lk_socialactivitybase_modifiedonbehalfby")
@JsonIgnore
public SocialactivityCollectionRequest getLk_socialactivitybase_modifiedonbehalfby() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("lk_socialactivitybase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_socialactivitybase_modifiedonbehalfby"));
}
@NavigationProperty(name="user_recurringappointmentmaster")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getUser_recurringappointmentmaster() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("user_recurringappointmentmaster"), RequestHelper.getValue(unmappedFields, "user_recurringappointmentmaster"));
}
@NavigationProperty(name="lk_customeraddress_modifiedonbehalfby")
@JsonIgnore
public CustomeraddressCollectionRequest getLk_customeraddress_modifiedonbehalfby() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("lk_customeraddress_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customeraddress_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_businessunitbase_modifiedby")
@JsonIgnore
public BusinessunitCollectionRequest getLk_businessunitbase_modifiedby() {
return new BusinessunitCollectionRequest(
contextPath.addSegment("lk_businessunitbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_businessunitbase_modifiedby"));
}
@NavigationProperty(name="modifiedby_plugintype")
@JsonIgnore
public PlugintypeCollectionRequest getModifiedby_plugintype() {
return new PlugintypeCollectionRequest(
contextPath.addSegment("modifiedby_plugintype"), RequestHelper.getValue(unmappedFields, "modifiedby_plugintype"));
}
@NavigationProperty(name="lk_timezonelocalizedname_createdonbehalfby")
@JsonIgnore
public TimezonelocalizednameCollectionRequest getLk_timezonelocalizedname_createdonbehalfby() {
return new TimezonelocalizednameCollectionRequest(
contextPath.addSegment("lk_timezonelocalizedname_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonelocalizedname_createdonbehalfby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstepimage_createdonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepimageCollectionRequest getLk_sdkmessageprocessingstepimage_createdonbehalfby() {
return new SdkmessageprocessingstepimageCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstepimage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstepimage_createdonbehalfby"));
}
@NavigationProperty(name="createdby_connection")
@JsonIgnore
public ConnectionCollectionRequest getCreatedby_connection() {
return new ConnectionCollectionRequest(
contextPath.addSegment("createdby_connection"), RequestHelper.getValue(unmappedFields, "createdby_connection"));
}
@NavigationProperty(name="lk_savedqueryvisualizationbase_createdonbehalfby")
@JsonIgnore
public SavedqueryvisualizationCollectionRequest getLk_savedqueryvisualizationbase_createdonbehalfby() {
return new SavedqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_savedqueryvisualizationbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_savedqueryvisualizationbase_createdonbehalfby"));
}
@NavigationProperty(name="SystemUser_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getSystemUser_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("SystemUser_ProcessSessions"), RequestHelper.getValue(unmappedFields, "SystemUser_ProcessSessions"));
}
@NavigationProperty(name="lk_importdatabase_modifiedby")
@JsonIgnore
public ImportdataCollectionRequest getLk_importdatabase_modifiedby() {
return new ImportdataCollectionRequest(
contextPath.addSegment("lk_importdatabase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importdatabase_modifiedby"));
}
@NavigationProperty(name="workflow_createdonbehalfby")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_createdonbehalfby() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "workflow_createdonbehalfby"));
}
@NavigationProperty(name="lk_slabase_createdby")
@JsonIgnore
public SlaCollectionRequest getLk_slabase_createdby() {
return new SlaCollectionRequest(
contextPath.addSegment("lk_slabase_createdby"), RequestHelper.getValue(unmappedFields, "lk_slabase_createdby"));
}
@NavigationProperty(name="lk_processtriggerbase_createdonbehalfby")
@JsonIgnore
public ProcesstriggerCollectionRequest getLk_processtriggerbase_createdonbehalfby() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("lk_processtriggerbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processtriggerbase_createdonbehalfby"));
}
@NavigationProperty(name="systemuser_connections2")
@JsonIgnore
public ConnectionCollectionRequest getSystemuser_connections2() {
return new ConnectionCollectionRequest(
contextPath.addSegment("systemuser_connections2"), RequestHelper.getValue(unmappedFields, "systemuser_connections2"));
}
@NavigationProperty(name="modifiedby_connection_role")
@JsonIgnore
public ConnectionroleCollectionRequest getModifiedby_connection_role() {
return new ConnectionroleCollectionRequest(
contextPath.addSegment("modifiedby_connection_role"), RequestHelper.getValue(unmappedFields, "modifiedby_connection_role"));
}
@NavigationProperty(name="appmodulecomponent_modifiedby")
@JsonIgnore
public AppmodulecomponentCollectionRequest getAppmodulecomponent_modifiedby() {
return new AppmodulecomponentCollectionRequest(
contextPath.addSegment("appmodulecomponent_modifiedby"), RequestHelper.getValue(unmappedFields, "appmodulecomponent_modifiedby"));
}
@NavigationProperty(name="lk_serviceendpointbase_createdonbehalfby")
@JsonIgnore
public ServiceendpointCollectionRequest getLk_serviceendpointbase_createdonbehalfby() {
return new ServiceendpointCollectionRequest(
contextPath.addSegment("lk_serviceendpointbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_serviceendpointbase_createdonbehalfby"));
}
@NavigationProperty(name="modifiedby_sdkmessageprocessingstepimage")
@JsonIgnore
public SdkmessageprocessingstepimageCollectionRequest getModifiedby_sdkmessageprocessingstepimage() {
return new SdkmessageprocessingstepimageCollectionRequest(
contextPath.addSegment("modifiedby_sdkmessageprocessingstepimage"), RequestHelper.getValue(unmappedFields, "modifiedby_sdkmessageprocessingstepimage"));
}
@NavigationProperty(name="lk_importentitymapping_modifiedby")
@JsonIgnore
public ImportentitymappingCollectionRequest getLk_importentitymapping_modifiedby() {
return new ImportentitymappingCollectionRequest(
contextPath.addSegment("lk_importentitymapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importentitymapping_modifiedby"));
}
@NavigationProperty(name="lk_slabase_createdonbehalfby")
@JsonIgnore
public SlaCollectionRequest getLk_slabase_createdonbehalfby() {
return new SlaCollectionRequest(
contextPath.addSegment("lk_slabase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slabase_createdonbehalfby"));
}
@NavigationProperty(name="lk_processtriggerbase_modifiedby")
@JsonIgnore
public ProcesstriggerCollectionRequest getLk_processtriggerbase_modifiedby() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("lk_processtriggerbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_processtriggerbase_modifiedby"));
}
@NavigationProperty(name="lk_accountbase_modifiedby")
@JsonIgnore
public AccountCollectionRequest getLk_accountbase_modifiedby() {
return new AccountCollectionRequest(
contextPath.addSegment("lk_accountbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_accountbase_modifiedby"));
}
@NavigationProperty(name="lk_PostFollow_createdby")
@JsonIgnore
public PostfollowCollectionRequest getLk_PostFollow_createdby() {
return new PostfollowCollectionRequest(
contextPath.addSegment("lk_PostFollow_createdby"), RequestHelper.getValue(unmappedFields, "lk_PostFollow_createdby"));
}
@NavigationProperty(name="systemuser_PostFollows")
@JsonIgnore
public PostfollowCollectionRequest getSystemuser_PostFollows() {
return new PostfollowCollectionRequest(
contextPath.addSegment("systemuser_PostFollows"), RequestHelper.getValue(unmappedFields, "systemuser_PostFollows"));
}
@NavigationProperty(name="systemuser_PostRegardings")
@JsonIgnore
public PostregardingCollectionRequest getSystemuser_PostRegardings() {
return new PostregardingCollectionRequest(
contextPath.addSegment("systemuser_PostRegardings"), RequestHelper.getValue(unmappedFields, "systemuser_PostRegardings"));
}
@NavigationProperty(name="lk_postcomment_createdby")
@JsonIgnore
public PostcommentCollectionRequest getLk_postcomment_createdby() {
return new PostcommentCollectionRequest(
contextPath.addSegment("lk_postcomment_createdby"), RequestHelper.getValue(unmappedFields, "lk_postcomment_createdby"));
}
@NavigationProperty(name="user_owner_postfollows")
@JsonIgnore
public PostfollowCollectionRequest getUser_owner_postfollows() {
return new PostfollowCollectionRequest(
contextPath.addSegment("user_owner_postfollows"), RequestHelper.getValue(unmappedFields, "user_owner_postfollows"));
}
@NavigationProperty(name="lk_postfollow_createdonbehalfby")
@JsonIgnore
public PostfollowCollectionRequest getLk_postfollow_createdonbehalfby() {
return new PostfollowCollectionRequest(
contextPath.addSegment("lk_postfollow_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_postfollow_createdonbehalfby"));
}
@NavigationProperty(name="lk_postcomment_createdonbehalfby")
@JsonIgnore
public PostcommentCollectionRequest getLk_postcomment_createdonbehalfby() {
return new PostcommentCollectionRequest(
contextPath.addSegment("lk_postcomment_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_postcomment_createdonbehalfby"));
}
@NavigationProperty(name="lk_postlike_createdonbehalfby")
@JsonIgnore
public PostlikeCollectionRequest getLk_postlike_createdonbehalfby() {
return new PostlikeCollectionRequest(
contextPath.addSegment("lk_postlike_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_postlike_createdonbehalfby"));
}
@NavigationProperty(name="lk_postlike_createdby")
@JsonIgnore
public PostlikeCollectionRequest getLk_postlike_createdby() {
return new PostlikeCollectionRequest(
contextPath.addSegment("lk_postlike_createdby"), RequestHelper.getValue(unmappedFields, "lk_postlike_createdby"));
}
@NavigationProperty(name="lk_calendar_modifiedby")
@JsonIgnore
public CalendarCollectionRequest getLk_calendar_modifiedby() {
return new CalendarCollectionRequest(
contextPath.addSegment("lk_calendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_calendar_modifiedby"));
}
@NavigationProperty(name="lk_sharepointdocumentlocationbase_createdonbehalfby")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getLk_sharepointdocumentlocationbase_createdonbehalfby() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("lk_sharepointdocumentlocationbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sharepointdocumentlocationbase_createdonbehalfby"));
}
@NavigationProperty(name="SystemUser_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getSystemUser_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("SystemUser_SyncErrors"), RequestHelper.getValue(unmappedFields, "SystemUser_SyncErrors"));
}
@NavigationProperty(name="lk_quarterlyfiscalcalendar_createdonbehalfby")
@JsonIgnore
public QuarterlyfiscalcalendarCollectionRequest getLk_quarterlyfiscalcalendar_createdonbehalfby() {
return new QuarterlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_quarterlyfiscalcalendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_quarterlyfiscalcalendar_createdonbehalfby"));
}
@NavigationProperty(name="lk_transformationparametermapping_modifiedonbehalfby")
@JsonIgnore
public TransformationparametermappingCollectionRequest getLk_transformationparametermapping_modifiedonbehalfby() {
return new TransformationparametermappingCollectionRequest(
contextPath.addSegment("lk_transformationparametermapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transformationparametermapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_timezonedefinition_modifiedby")
@JsonIgnore
public TimezonedefinitionCollectionRequest getLk_timezonedefinition_modifiedby() {
return new TimezonedefinitionCollectionRequest(
contextPath.addSegment("lk_timezonedefinition_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_timezonedefinition_modifiedby"));
}
@NavigationProperty(name="lk_picklistmapping_modifiedby")
@JsonIgnore
public PicklistmappingCollectionRequest getLk_picklistmapping_modifiedby() {
return new PicklistmappingCollectionRequest(
contextPath.addSegment("lk_picklistmapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_picklistmapping_modifiedby"));
}
@NavigationProperty(name="lk_templatebase_createdby")
@JsonIgnore
public TemplateCollectionRequest getLk_templatebase_createdby() {
return new TemplateCollectionRequest(
contextPath.addSegment("lk_templatebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_templatebase_createdby"));
}
@NavigationProperty(name="lk_plugintracelogbase_createdonbehalfby")
@JsonIgnore
public PlugintracelogCollectionRequest getLk_plugintracelogbase_createdonbehalfby() {
return new PlugintracelogCollectionRequest(
contextPath.addSegment("lk_plugintracelogbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_plugintracelogbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_workflowlog_createdonbehalfby")
@JsonIgnore
public WorkflowlogCollectionRequest getLk_workflowlog_createdonbehalfby() {
return new WorkflowlogCollectionRequest(
contextPath.addSegment("lk_workflowlog_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_workflowlog_createdonbehalfby"));
}
@NavigationProperty(name="lk_userqueryvisualizationbase_createdonbehalfby")
@JsonIgnore
public UserqueryvisualizationCollectionRequest getLk_userqueryvisualizationbase_createdonbehalfby() {
return new UserqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_userqueryvisualizationbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userqueryvisualizationbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_bulkdeleteoperationbase_modifiedby")
@JsonIgnore
public BulkdeleteoperationCollectionRequest getLk_bulkdeleteoperationbase_modifiedby() {
return new BulkdeleteoperationCollectionRequest(
contextPath.addSegment("lk_bulkdeleteoperationbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_bulkdeleteoperationbase_modifiedby"));
}
@NavigationProperty(name="lk_sharepointsitebase_modifiedby")
@JsonIgnore
public SharepointsiteCollectionRequest getLk_sharepointsitebase_modifiedby() {
return new SharepointsiteCollectionRequest(
contextPath.addSegment("lk_sharepointsitebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_sharepointsitebase_modifiedby"));
}
@NavigationProperty(name="lk_report_createdonbehalfby")
@JsonIgnore
public ReportCollectionRequest getLk_report_createdonbehalfby() {
return new ReportCollectionRequest(
contextPath.addSegment("lk_report_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_report_createdonbehalfby"));
}
@NavigationProperty(name="createdby_plugintracelog")
@JsonIgnore
public PlugintracelogCollectionRequest getCreatedby_plugintracelog() {
return new PlugintracelogCollectionRequest(
contextPath.addSegment("createdby_plugintracelog"), RequestHelper.getValue(unmappedFields, "createdby_plugintracelog"));
}
@NavigationProperty(name="createdby_plugintypestatistic")
@JsonIgnore
public PlugintypestatisticCollectionRequest getCreatedby_plugintypestatistic() {
return new PlugintypestatisticCollectionRequest(
contextPath.addSegment("createdby_plugintypestatistic"), RequestHelper.getValue(unmappedFields, "createdby_plugintypestatistic"));
}
@NavigationProperty(name="lk_DisplayStringbase_modifiedonbehalfby")
@JsonIgnore
public DisplaystringCollectionRequest getLk_DisplayStringbase_modifiedonbehalfby() {
return new DisplaystringCollectionRequest(
contextPath.addSegment("lk_DisplayStringbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_DisplayStringbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_monthlyfiscalcalendar_salespersonid")
@JsonIgnore
public MonthlyfiscalcalendarCollectionRequest getLk_monthlyfiscalcalendar_salespersonid() {
return new MonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_monthlyfiscalcalendar_salespersonid"), RequestHelper.getValue(unmappedFields, "lk_monthlyfiscalcalendar_salespersonid"));
}
@NavigationProperty(name="systemuser_SiteMap_modifiedby")
@JsonIgnore
public SitemapCollectionRequest getSystemuser_SiteMap_modifiedby() {
return new SitemapCollectionRequest(
contextPath.addSegment("systemuser_SiteMap_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_SiteMap_modifiedby"));
}
@NavigationProperty(name="lk_tracelog_modifiedby")
@JsonIgnore
public TracelogCollectionRequest getLk_tracelog_modifiedby() {
return new TracelogCollectionRequest(
contextPath.addSegment("lk_tracelog_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_tracelog_modifiedby"));
}
@NavigationProperty(name="lk_duplicaterule_modifiedonbehalfby")
@JsonIgnore
public DuplicateruleCollectionRequest getLk_duplicaterule_modifiedonbehalfby() {
return new DuplicateruleCollectionRequest(
contextPath.addSegment("lk_duplicaterule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterule_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_savedquery_createdonbehalfby")
@JsonIgnore
public SavedqueryCollectionRequest getLk_savedquery_createdonbehalfby() {
return new SavedqueryCollectionRequest(
contextPath.addSegment("lk_savedquery_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_savedquery_createdonbehalfby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitemassocaition_modifiedby")
@JsonIgnore
public MobileofflineprofileitemassociationCollectionRequest getLk_mobileofflineprofileitemassocaition_modifiedby() {
return new MobileofflineprofileitemassociationCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitemassocaition_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitemassocaition_modifiedby"));
}
@NavigationProperty(name="lk_queueitembase_modifiedby")
@JsonIgnore
public QueueitemCollectionRequest getLk_queueitembase_modifiedby() {
return new QueueitemCollectionRequest(
contextPath.addSegment("lk_queueitembase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_queueitembase_modifiedby"));
}
@NavigationProperty(name="lk_rolebase_modifiedby")
@JsonIgnore
public RoleCollectionRequest getLk_rolebase_modifiedby() {
return new RoleCollectionRequest(
contextPath.addSegment("lk_rolebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_rolebase_modifiedby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstep_createdonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getLk_sdkmessageprocessingstep_createdonbehalfby() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstep_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstep_createdonbehalfby"));
}
@NavigationProperty(name="lk_newprocess_createdby")
@JsonIgnore
public NewprocessCollectionRequest getLk_newprocess_createdby() {
return new NewprocessCollectionRequest(
contextPath.addSegment("lk_newprocess_createdby"), RequestHelper.getValue(unmappedFields, "lk_newprocess_createdby"));
}
@NavigationProperty(name="lk_importjobbase_createdonbehalfby")
@JsonIgnore
public ImportjobCollectionRequest getLk_importjobbase_createdonbehalfby() {
return new ImportjobCollectionRequest(
contextPath.addSegment("lk_importjobbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importjobbase_createdonbehalfby"));
}
@NavigationProperty(name="createdby_sdkmessageprocessingstepimage")
@JsonIgnore
public SdkmessageprocessingstepimageCollectionRequest getCreatedby_sdkmessageprocessingstepimage() {
return new SdkmessageprocessingstepimageCollectionRequest(
contextPath.addSegment("createdby_sdkmessageprocessingstepimage"), RequestHelper.getValue(unmappedFields, "createdby_sdkmessageprocessingstepimage"));
}
@NavigationProperty(name="systemuser_SiteMap_createdonbehalfby")
@JsonIgnore
public SitemapCollectionRequest getSystemuser_SiteMap_createdonbehalfby() {
return new SitemapCollectionRequest(
contextPath.addSegment("systemuser_SiteMap_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_SiteMap_createdonbehalfby"));
}
@NavigationProperty(name="lk_businessunit_createdonbehalfby")
@JsonIgnore
public BusinessunitCollectionRequest getLk_businessunit_createdonbehalfby() {
return new BusinessunitCollectionRequest(
contextPath.addSegment("lk_businessunit_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_businessunit_createdonbehalfby"));
}
@NavigationProperty(name="lk_customcontrol_modifiedonbehalfby")
@JsonIgnore
public CustomcontrolCollectionRequest getLk_customcontrol_modifiedonbehalfby() {
return new CustomcontrolCollectionRequest(
contextPath.addSegment("lk_customcontrol_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontrol_modifiedonbehalfby"));
}
@NavigationProperty(name="createdby_serviceendpoint")
@JsonIgnore
public ServiceendpointCollectionRequest getCreatedby_serviceendpoint() {
return new ServiceendpointCollectionRequest(
contextPath.addSegment("createdby_serviceendpoint"), RequestHelper.getValue(unmappedFields, "createdby_serviceendpoint"));
}
@NavigationProperty(name="lk_socialactivitybase_createdonbehalfby")
@JsonIgnore
public SocialactivityCollectionRequest getLk_socialactivitybase_createdonbehalfby() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("lk_socialactivitybase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_socialactivitybase_createdonbehalfby"));
}
@NavigationProperty(name="lk_letter_modifiedonbehalfby")
@JsonIgnore
public LetterCollectionRequest getLk_letter_modifiedonbehalfby() {
return new LetterCollectionRequest(
contextPath.addSegment("lk_letter_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_letter_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_timezonerule_createdonbehalfby")
@JsonIgnore
public TimezoneruleCollectionRequest getLk_timezonerule_createdonbehalfby() {
return new TimezoneruleCollectionRequest(
contextPath.addSegment("lk_timezonerule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonerule_createdonbehalfby"));
}
@NavigationProperty(name="lk_personaldocumenttemplatebase_modifiedonbehalfby")
@JsonIgnore
public PersonaldocumenttemplateCollectionRequest getLk_personaldocumenttemplatebase_modifiedonbehalfby() {
return new PersonaldocumenttemplateCollectionRequest(
contextPath.addSegment("lk_personaldocumenttemplatebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_personaldocumenttemplatebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_teamtemplate_createdonbehalfby")
@JsonIgnore
public TeamtemplateCollectionRequest getLk_teamtemplate_createdonbehalfby() {
return new TeamtemplateCollectionRequest(
contextPath.addSegment("lk_teamtemplate_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_teamtemplate_createdonbehalfby"));
}
@NavigationProperty(name="SystemUser_ImportLogs")
@JsonIgnore
public ImportlogCollectionRequest getSystemUser_ImportLogs() {
return new ImportlogCollectionRequest(
contextPath.addSegment("SystemUser_ImportLogs"), RequestHelper.getValue(unmappedFields, "SystemUser_ImportLogs"));
}
@NavigationProperty(name="lk_teamtemplate_modifiedby")
@JsonIgnore
public TeamtemplateCollectionRequest getLk_teamtemplate_modifiedby() {
return new TeamtemplateCollectionRequest(
contextPath.addSegment("lk_teamtemplate_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_teamtemplate_modifiedby"));
}
@NavigationProperty(name="lk_columnmapping_modifiedby")
@JsonIgnore
public ColumnmappingCollectionRequest getLk_columnmapping_modifiedby() {
return new ColumnmappingCollectionRequest(
contextPath.addSegment("lk_columnmapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_columnmapping_modifiedby"));
}
@NavigationProperty(name="lk_timezonedefinition_createdonbehalfby")
@JsonIgnore
public TimezonedefinitionCollectionRequest getLk_timezonedefinition_createdonbehalfby() {
return new TimezonedefinitionCollectionRequest(
contextPath.addSegment("lk_timezonedefinition_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonedefinition_createdonbehalfby"));
}
@NavigationProperty(name="lk_reportcategory_modifiedonbehalfby")
@JsonIgnore
public ReportcategoryCollectionRequest getLk_reportcategory_modifiedonbehalfby() {
return new ReportcategoryCollectionRequest(
contextPath.addSegment("lk_reportcategory_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_reportcategory_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_expiredprocess_createdby")
@JsonIgnore
public ExpiredprocessCollectionRequest getLk_expiredprocess_createdby() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("lk_expiredprocess_createdby"), RequestHelper.getValue(unmappedFields, "lk_expiredprocess_createdby"));
}
@NavigationProperty(name="webresource_modifiedby")
@JsonIgnore
public WebresourceCollectionRequest getWebresource_modifiedby() {
return new WebresourceCollectionRequest(
contextPath.addSegment("webresource_modifiedby"), RequestHelper.getValue(unmappedFields, "webresource_modifiedby"));
}
@NavigationProperty(name="lk_reportcategorybase_createdby")
@JsonIgnore
public ReportcategoryCollectionRequest getLk_reportcategorybase_createdby() {
return new ReportcategoryCollectionRequest(
contextPath.addSegment("lk_reportcategorybase_createdby"), RequestHelper.getValue(unmappedFields, "lk_reportcategorybase_createdby"));
}
@NavigationProperty(name="lk_userquery_modifiedby")
@JsonIgnore
public UserqueryCollectionRequest getLk_userquery_modifiedby() {
return new UserqueryCollectionRequest(
contextPath.addSegment("lk_userquery_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_userquery_modifiedby"));
}
@NavigationProperty(name="lk_customcontrol_createdby")
@JsonIgnore
public CustomcontrolCollectionRequest getLk_customcontrol_createdby() {
return new CustomcontrolCollectionRequest(
contextPath.addSegment("lk_customcontrol_createdby"), RequestHelper.getValue(unmappedFields, "lk_customcontrol_createdby"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="lk_systemuser_modifiedonbehalfby")
@JsonIgnore
public SystemuserCollectionRequest getLk_systemuser_modifiedonbehalfby() {
return new SystemuserCollectionRequest(
contextPath.addSegment("lk_systemuser_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_systemuser_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_importlogbase_createdby")
@JsonIgnore
public ImportlogCollectionRequest getLk_importlogbase_createdby() {
return new ImportlogCollectionRequest(
contextPath.addSegment("lk_importlogbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importlogbase_createdby"));
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="lk_systemuserbase_createdby")
@JsonIgnore
public SystemuserCollectionRequest getLk_systemuserbase_createdby() {
return new SystemuserCollectionRequest(
contextPath.addSegment("lk_systemuserbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_systemuserbase_createdby"));
}
@NavigationProperty(name="lk_sharepointdocumentlocationbase_modifiedby")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getLk_sharepointdocumentlocationbase_modifiedby() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("lk_sharepointdocumentlocationbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_sharepointdocumentlocationbase_modifiedby"));
}
@NavigationProperty(name="lk_queueitembase_workerid")
@JsonIgnore
public QueueitemCollectionRequest getLk_queueitembase_workerid() {
return new QueueitemCollectionRequest(
contextPath.addSegment("lk_queueitembase_workerid"), RequestHelper.getValue(unmappedFields, "lk_queueitembase_workerid"));
}
@NavigationProperty(name="lk_solutioncomponentbase_createdonbehalfby")
@JsonIgnore
public SolutioncomponentCollectionRequest getLk_solutioncomponentbase_createdonbehalfby() {
return new SolutioncomponentCollectionRequest(
contextPath.addSegment("lk_solutioncomponentbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentbase_createdonbehalfby"));
}
@NavigationProperty(name="systemuserprofiles_association")
@JsonIgnore
public FieldsecurityprofileCollectionRequest getSystemuserprofiles_association() {
return new FieldsecurityprofileCollectionRequest(
contextPath.addSegment("systemuserprofiles_association"), RequestHelper.getValue(unmappedFields, "systemuserprofiles_association"));
}
@NavigationProperty(name="lk_translationprocess_modifiedby")
@JsonIgnore
public TranslationprocessCollectionRequest getLk_translationprocess_modifiedby() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("lk_translationprocess_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_translationprocess_modifiedby"));
}
@NavigationProperty(name="lk_semiannualfiscalcalendar_modifiedonbehalfby")
@JsonIgnore
public SemiannualfiscalcalendarCollectionRequest getLk_semiannualfiscalcalendar_modifiedonbehalfby() {
return new SemiannualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_semiannualfiscalcalendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_semiannualfiscalcalendar_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_publisherbase_modifiedonbehalfby")
@JsonIgnore
public PublisherCollectionRequest getLk_publisherbase_modifiedonbehalfby() {
return new PublisherCollectionRequest(
contextPath.addSegment("lk_publisherbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_publisherbase_modifiedonbehalfby"));
}
@NavigationProperty(name="transactioncurrencyid")
@JsonIgnore
public TransactioncurrencyRequest getTransactioncurrencyid() {
return new TransactioncurrencyRequest(contextPath.addSegment("transactioncurrencyid"), RequestHelper.getValue(unmappedFields, "transactioncurrencyid"));
}
@NavigationProperty(name="lk_ACIViewMapper_createdby")
@JsonIgnore
public AciviewmapperCollectionRequest getLk_ACIViewMapper_createdby() {
return new AciviewmapperCollectionRequest(
contextPath.addSegment("lk_ACIViewMapper_createdby"), RequestHelper.getValue(unmappedFields, "lk_ACIViewMapper_createdby"));
}
@NavigationProperty(name="lk_importlog_createdonbehalfby")
@JsonIgnore
public ImportlogCollectionRequest getLk_importlog_createdonbehalfby() {
return new ImportlogCollectionRequest(
contextPath.addSegment("lk_importlog_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importlog_createdonbehalfby"));
}
@NavigationProperty(name="lk_importdata_createdonbehalfby")
@JsonIgnore
public ImportdataCollectionRequest getLk_importdata_createdonbehalfby() {
return new ImportdataCollectionRequest(
contextPath.addSegment("lk_importdata_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importdata_createdonbehalfby"));
}
@NavigationProperty(name="lk_letter_createdonbehalfby")
@JsonIgnore
public LetterCollectionRequest getLk_letter_createdonbehalfby() {
return new LetterCollectionRequest(
contextPath.addSegment("lk_letter_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_letter_createdonbehalfby"));
}
@NavigationProperty(name="modifiedby_serviceendpoint")
@JsonIgnore
public ServiceendpointCollectionRequest getModifiedby_serviceendpoint() {
return new ServiceendpointCollectionRequest(
contextPath.addSegment("modifiedby_serviceendpoint"), RequestHelper.getValue(unmappedFields, "modifiedby_serviceendpoint"));
}
@NavigationProperty(name="lk_annualfiscalcalendar_modifiedby")
@JsonIgnore
public AnnualfiscalcalendarCollectionRequest getLk_annualfiscalcalendar_modifiedby() {
return new AnnualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_annualfiscalcalendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_annualfiscalcalendar_modifiedby"));
}
@NavigationProperty(name="lk_importmap_createdonbehalfby")
@JsonIgnore
public ImportmapCollectionRequest getLk_importmap_createdonbehalfby() {
return new ImportmapCollectionRequest(
contextPath.addSegment("lk_importmap_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importmap_createdonbehalfby"));
}
@NavigationProperty(name="lk_knowledgearticleviews_createdonbehalfby")
@JsonIgnore
public KnowledgearticleviewsCollectionRequest getLk_knowledgearticleviews_createdonbehalfby() {
return new KnowledgearticleviewsCollectionRequest(
contextPath.addSegment("lk_knowledgearticleviews_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticleviews_createdonbehalfby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitem_createdonbehalfby")
@JsonIgnore
public MobileofflineprofileitemCollectionRequest getLk_mobileofflineprofileitem_createdonbehalfby() {
return new MobileofflineprofileitemCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitem_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitem_createdonbehalfby"));
}
@NavigationProperty(name="lk_annotationbase_createdby")
@JsonIgnore
public AnnotationCollectionRequest getLk_annotationbase_createdby() {
return new AnnotationCollectionRequest(
contextPath.addSegment("lk_annotationbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_annotationbase_createdby"));
}
@NavigationProperty(name="lk_plugintype_createdonbehalfby")
@JsonIgnore
public PlugintypeCollectionRequest getLk_plugintype_createdonbehalfby() {
return new PlugintypeCollectionRequest(
contextPath.addSegment("lk_plugintype_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_plugintype_createdonbehalfby"));
}
@NavigationProperty(name="createdby_connection_role")
@JsonIgnore
public ConnectionroleCollectionRequest getCreatedby_connection_role() {
return new ConnectionroleCollectionRequest(
contextPath.addSegment("createdby_connection_role"), RequestHelper.getValue(unmappedFields, "createdby_connection_role"));
}
@NavigationProperty(name="lk_customeraddressbase_createdby")
@JsonIgnore
public CustomeraddressCollectionRequest getLk_customeraddressbase_createdby() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("lk_customeraddressbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_customeraddressbase_createdby"));
}
@NavigationProperty(name="lk_timezonedefinition_createdby")
@JsonIgnore
public TimezonedefinitionCollectionRequest getLk_timezonedefinition_createdby() {
return new TimezonedefinitionCollectionRequest(
contextPath.addSegment("lk_timezonedefinition_createdby"), RequestHelper.getValue(unmappedFields, "lk_timezonedefinition_createdby"));
}
@NavigationProperty(name="lk_documenttemplatebase_createdonbehalfby")
@JsonIgnore
public DocumenttemplateCollectionRequest getLk_documenttemplatebase_createdonbehalfby() {
return new DocumenttemplateCollectionRequest(
contextPath.addSegment("lk_documenttemplatebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_documenttemplatebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_recurringappointmentmaster_createdonbehalfby")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getLk_recurringappointmentmaster_createdonbehalfby() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("lk_recurringappointmentmaster_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recurringappointmentmaster_createdonbehalfby"));
}
@NavigationProperty(name="lk_personaldocumenttemplatebase_createdby")
@JsonIgnore
public PersonaldocumenttemplateCollectionRequest getLk_personaldocumenttemplatebase_createdby() {
return new PersonaldocumenttemplateCollectionRequest(
contextPath.addSegment("lk_personaldocumenttemplatebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_personaldocumenttemplatebase_createdby"));
}
@NavigationProperty(name="lk_semiannualfiscalcalendar_createdonbehalfby")
@JsonIgnore
public SemiannualfiscalcalendarCollectionRequest getLk_semiannualfiscalcalendar_createdonbehalfby() {
return new SemiannualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_semiannualfiscalcalendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_semiannualfiscalcalendar_createdonbehalfby"));
}
@NavigationProperty(name="SystemUser_DuplicateBaseRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getSystemUser_DuplicateBaseRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("SystemUser_DuplicateBaseRecord"), RequestHelper.getValue(unmappedFields, "SystemUser_DuplicateBaseRecord"));
}
@NavigationProperty(name="lk_mailboxtrackingfolder_modifiedonbehalfby")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getLk_mailboxtrackingfolder_modifiedonbehalfby() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("lk_mailboxtrackingfolder_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailboxtrackingfolder_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_recurringappointmentmaster_createdby")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getLk_recurringappointmentmaster_createdby() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("lk_recurringappointmentmaster_createdby"), RequestHelper.getValue(unmappedFields, "lk_recurringappointmentmaster_createdby"));
}
@NavigationProperty(name="lk_sharepointsitebase_createdby")
@JsonIgnore
public SharepointsiteCollectionRequest getLk_sharepointsitebase_createdby() {
return new SharepointsiteCollectionRequest(
contextPath.addSegment("lk_sharepointsitebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_sharepointsitebase_createdby"));
}
@NavigationProperty(name="lk_phonecall_modifiedonbehalfby")
@JsonIgnore
public PhonecallCollectionRequest getLk_phonecall_modifiedonbehalfby() {
return new PhonecallCollectionRequest(
contextPath.addSegment("lk_phonecall_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_phonecall_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticlecommentbase_createdby")
@JsonIgnore
public KbarticlecommentCollectionRequest getLk_kbarticlecommentbase_createdby() {
return new KbarticlecommentCollectionRequest(
contextPath.addSegment("lk_kbarticlecommentbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlecommentbase_createdby"));
}
@NavigationProperty(name="lk_recurrencerulebase_modifiedonbehalfby")
@JsonIgnore
public RecurrenceruleCollectionRequest getLk_recurrencerulebase_modifiedonbehalfby() {
return new RecurrenceruleCollectionRequest(
contextPath.addSegment("lk_recurrencerulebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recurrencerulebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_quarterlyfiscalcalendar_modifiedby")
@JsonIgnore
public QuarterlyfiscalcalendarCollectionRequest getLk_quarterlyfiscalcalendar_modifiedby() {
return new QuarterlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_quarterlyfiscalcalendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_quarterlyfiscalcalendar_modifiedby"));
}
@NavigationProperty(name="lk_solutioncomponentbase_modifiedonbehalfby")
@JsonIgnore
public SolutioncomponentCollectionRequest getLk_solutioncomponentbase_modifiedonbehalfby() {
return new SolutioncomponentCollectionRequest(
contextPath.addSegment("lk_solutioncomponentbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_role_modifiedonbehalfby")
@JsonIgnore
public RoleCollectionRequest getLk_role_modifiedonbehalfby() {
return new RoleCollectionRequest(
contextPath.addSegment("lk_role_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_role_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_duplicaterulebase_modifiedby")
@JsonIgnore
public DuplicateruleCollectionRequest getLk_duplicaterulebase_modifiedby() {
return new DuplicateruleCollectionRequest(
contextPath.addSegment("lk_duplicaterulebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterulebase_modifiedby"));
}
@NavigationProperty(name="lk_subjectbase_createdby")
@JsonIgnore
public SubjectCollectionRequest getLk_subjectbase_createdby() {
return new SubjectCollectionRequest(
contextPath.addSegment("lk_subjectbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_subjectbase_createdby"));
}
@NavigationProperty(name="lk_contact_createdonbehalfby")
@JsonIgnore
public ContactCollectionRequest getLk_contact_createdonbehalfby() {
return new ContactCollectionRequest(
contextPath.addSegment("lk_contact_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_contact_createdonbehalfby"));
}
@NavigationProperty(name="lk_semiannualfiscalcalendar_createdby")
@JsonIgnore
public SemiannualfiscalcalendarCollectionRequest getLk_semiannualfiscalcalendar_createdby() {
return new SemiannualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_semiannualfiscalcalendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_semiannualfiscalcalendar_createdby"));
}
@NavigationProperty(name="lk_SocialProfile_modifiedonbehalfby")
@JsonIgnore
public SocialprofileCollectionRequest getLk_SocialProfile_modifiedonbehalfby() {
return new SocialprofileCollectionRequest(
contextPath.addSegment("lk_SocialProfile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_SocialProfile_modifiedonbehalfby"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="lk_systemuserbase_modifiedby")
@JsonIgnore
public SystemuserCollectionRequest getLk_systemuserbase_modifiedby() {
return new SystemuserCollectionRequest(
contextPath.addSegment("lk_systemuserbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_systemuserbase_modifiedby"));
}
@NavigationProperty(name="lk_knowledgearticleviews_modifiedonbehalfby")
@JsonIgnore
public KnowledgearticleviewsCollectionRequest getLk_knowledgearticleviews_modifiedonbehalfby() {
return new KnowledgearticleviewsCollectionRequest(
contextPath.addSegment("lk_knowledgearticleviews_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticleviews_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_processsessionbase_modifiedonbehalfby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsessionbase_modifiedonbehalfby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsessionbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processsessionbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_lookupmapping_createdby")
@JsonIgnore
public LookupmappingCollectionRequest getLk_lookupmapping_createdby() {
return new LookupmappingCollectionRequest(
contextPath.addSegment("lk_lookupmapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_lookupmapping_createdby"));
}
@NavigationProperty(name="lk_importentitymapping_createdby")
@JsonIgnore
public ImportentitymappingCollectionRequest getLk_importentitymapping_createdby() {
return new ImportentitymappingCollectionRequest(
contextPath.addSegment("lk_importentitymapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_importentitymapping_createdby"));
}
@NavigationProperty(name="lk_kbarticlecomment_createdonbehalfby")
@JsonIgnore
public KbarticlecommentCollectionRequest getLk_kbarticlecomment_createdonbehalfby() {
return new KbarticlecommentCollectionRequest(
contextPath.addSegment("lk_kbarticlecomment_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlecomment_createdonbehalfby"));
}
@NavigationProperty(name="lk_team_modifiedonbehalfby")
@JsonIgnore
public TeamCollectionRequest getLk_team_modifiedonbehalfby() {
return new TeamCollectionRequest(
contextPath.addSegment("lk_team_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_team_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_teamtemplate_modifiedonbehalfby")
@JsonIgnore
public TeamtemplateCollectionRequest getLk_teamtemplate_modifiedonbehalfby() {
return new TeamtemplateCollectionRequest(
contextPath.addSegment("lk_teamtemplate_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_teamtemplate_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_fax_createdby")
@JsonIgnore
public FaxCollectionRequest getLk_fax_createdby() {
return new FaxCollectionRequest(
contextPath.addSegment("lk_fax_createdby"), RequestHelper.getValue(unmappedFields, "lk_fax_createdby"));
}
@NavigationProperty(name="lk_DisplayStringbase_createdby")
@JsonIgnore
public DisplaystringCollectionRequest getLk_DisplayStringbase_createdby() {
return new DisplaystringCollectionRequest(
contextPath.addSegment("lk_DisplayStringbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_DisplayStringbase_createdby"));
}
@NavigationProperty(name="lk_publisheraddressbase_createdby")
@JsonIgnore
public PublisheraddressCollectionRequest getLk_publisheraddressbase_createdby() {
return new PublisheraddressCollectionRequest(
contextPath.addSegment("lk_publisheraddressbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_publisheraddressbase_createdby"));
}
@NavigationProperty(name="lk_templatebase_modifiedby")
@JsonIgnore
public TemplateCollectionRequest getLk_templatebase_modifiedby() {
return new TemplateCollectionRequest(
contextPath.addSegment("lk_templatebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_templatebase_modifiedby"));
}
@NavigationProperty(name="user_activity")
@JsonIgnore
public ActivitypointerCollectionRequest getUser_activity() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("user_activity"), RequestHelper.getValue(unmappedFields, "user_activity"));
}
@NavigationProperty(name="lk_MobileOfflineProfile_modifiedonbehalfby")
@JsonIgnore
public MobileofflineprofileCollectionRequest getLk_MobileOfflineProfile_modifiedonbehalfby() {
return new MobileofflineprofileCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfile_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_fax_createdonbehalfby")
@JsonIgnore
public FaxCollectionRequest getLk_fax_createdonbehalfby() {
return new FaxCollectionRequest(
contextPath.addSegment("lk_fax_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fax_createdonbehalfby"));
}
@NavigationProperty(name="lk_lookupmapping_modifiedonbehalfby")
@JsonIgnore
public LookupmappingCollectionRequest getLk_lookupmapping_modifiedonbehalfby() {
return new LookupmappingCollectionRequest(
contextPath.addSegment("lk_lookupmapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_lookupmapping_modifiedonbehalfby"));
}
@NavigationProperty(name="SystemUser_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getSystemUser_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("SystemUser_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "SystemUser_BulkDeleteFailures"));
}
@NavigationProperty(name="organizationid_organization")
@JsonIgnore
public OrganizationRequest getOrganizationid_organization() {
return new OrganizationRequest(contextPath.addSegment("organizationid_organization"), RequestHelper.getValue(unmappedFields, "organizationid_organization"));
}
@NavigationProperty(name="lk_customeraddress_createdonbehalfby")
@JsonIgnore
public CustomeraddressCollectionRequest getLk_customeraddress_createdonbehalfby() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("lk_customeraddress_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customeraddress_createdonbehalfby"));
}
@NavigationProperty(name="lk_calendarrule_modifiedby")
@JsonIgnore
public CalendarruleCollectionRequest getLk_calendarrule_modifiedby() {
return new CalendarruleCollectionRequest(
contextPath.addSegment("lk_calendarrule_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_calendarrule_modifiedby"));
}
@NavigationProperty(name="system_user_accounts")
@JsonIgnore
public AccountCollectionRequest getSystem_user_accounts() {
return new AccountCollectionRequest(
contextPath.addSegment("system_user_accounts"), RequestHelper.getValue(unmappedFields, "system_user_accounts"));
}
@NavigationProperty(name="lk_savedqueryvisualizationbase_createdby")
@JsonIgnore
public SavedqueryvisualizationCollectionRequest getLk_savedqueryvisualizationbase_createdby() {
return new SavedqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_savedqueryvisualizationbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_savedqueryvisualizationbase_createdby"));
}
@NavigationProperty(name="lk_columnmapping_createdby")
@JsonIgnore
public ColumnmappingCollectionRequest getLk_columnmapping_createdby() {
return new ColumnmappingCollectionRequest(
contextPath.addSegment("lk_columnmapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_columnmapping_createdby"));
}
@NavigationProperty(name="lk_connectionbase_createdonbehalfby")
@JsonIgnore
public ConnectionCollectionRequest getLk_connectionbase_createdonbehalfby() {
return new ConnectionCollectionRequest(
contextPath.addSegment("lk_connectionbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionbase_createdonbehalfby"));
}
@NavigationProperty(name="impersonatinguserid_sdkmessageprocessingstep")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getImpersonatinguserid_sdkmessageprocessingstep() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("impersonatinguserid_sdkmessageprocessingstep"), RequestHelper.getValue(unmappedFields, "impersonatinguserid_sdkmessageprocessingstep"));
}
@NavigationProperty(name="lk_userquery_createdonbehalfby")
@JsonIgnore
public UserqueryCollectionRequest getLk_userquery_createdonbehalfby() {
return new UserqueryCollectionRequest(
contextPath.addSegment("lk_userquery_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userquery_createdonbehalfby"));
}
@NavigationProperty(name="lk_accountbase_createdonbehalfby")
@JsonIgnore
public AccountCollectionRequest getLk_accountbase_createdonbehalfby() {
return new AccountCollectionRequest(
contextPath.addSegment("lk_accountbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_accountbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_publisheraddressbase_modifiedonbehalfby")
@JsonIgnore
public PublisheraddressCollectionRequest getLk_publisheraddressbase_modifiedonbehalfby() {
return new PublisheraddressCollectionRequest(
contextPath.addSegment("lk_publisheraddressbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_publisheraddressbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_userqueryvisualization_modifiedby")
@JsonIgnore
public UserqueryvisualizationCollectionRequest getLk_userqueryvisualization_modifiedby() {
return new UserqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_userqueryvisualization_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_userqueryvisualization_modifiedby"));
}
@NavigationProperty(name="systemuser_appconfig_modifiedby")
@JsonIgnore
public AppconfigCollectionRequest getSystemuser_appconfig_modifiedby() {
return new AppconfigCollectionRequest(
contextPath.addSegment("systemuser_appconfig_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfig_modifiedby"));
}
@NavigationProperty(name="lk_connectionrolebase_modifiedonbehalfby")
@JsonIgnore
public ConnectionroleCollectionRequest getLk_connectionrolebase_modifiedonbehalfby() {
return new ConnectionroleCollectionRequest(
contextPath.addSegment("lk_connectionrolebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionrolebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_calendar_createdby")
@JsonIgnore
public CalendarCollectionRequest getLk_calendar_createdby() {
return new CalendarCollectionRequest(
contextPath.addSegment("lk_calendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_calendar_createdby"));
}
@NavigationProperty(name="annotation_owning_user")
@JsonIgnore
public AnnotationCollectionRequest getAnnotation_owning_user() {
return new AnnotationCollectionRequest(
contextPath.addSegment("annotation_owning_user"), RequestHelper.getValue(unmappedFields, "annotation_owning_user"));
}
@NavigationProperty(name="systemuser_appconfigmaster_createdonbehalfby")
@JsonIgnore
public AppconfigmasterCollectionRequest getSystemuser_appconfigmaster_createdonbehalfby() {
return new AppconfigmasterCollectionRequest(
contextPath.addSegment("systemuser_appconfigmaster_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfigmaster_createdonbehalfby"));
}
@NavigationProperty(name="lk_newprocess_modifiedby")
@JsonIgnore
public NewprocessCollectionRequest getLk_newprocess_modifiedby() {
return new NewprocessCollectionRequest(
contextPath.addSegment("lk_newprocess_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_newprocess_modifiedby"));
}
@NavigationProperty(name="calendarid")
@JsonIgnore
public CalendarRequest getCalendarid() {
return new CalendarRequest(contextPath.addSegment("calendarid"), RequestHelper.getValue(unmappedFields, "calendarid"));
}
@NavigationProperty(name="lk_tracelog_createdonbehalfby")
@JsonIgnore
public TracelogCollectionRequest getLk_tracelog_createdonbehalfby() {
return new TracelogCollectionRequest(
contextPath.addSegment("lk_tracelog_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_tracelog_createdonbehalfby"));
}
@NavigationProperty(name="lk_mailboxtrackingfolder_createdonbehalfby")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getLk_mailboxtrackingfolder_createdonbehalfby() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("lk_mailboxtrackingfolder_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailboxtrackingfolder_createdonbehalfby"));
}
@NavigationProperty(name="lk_category_modifiedonbehalfby")
@JsonIgnore
public CategoryCollectionRequest getLk_category_modifiedonbehalfby() {
return new CategoryCollectionRequest(
contextPath.addSegment("lk_category_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_category_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_asyncoperation_createdby")
@JsonIgnore
public AsyncoperationCollectionRequest getLk_asyncoperation_createdby() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_createdby"), RequestHelper.getValue(unmappedFields, "lk_asyncoperation_createdby"));
}
@NavigationProperty(name="systemuser_appmodule_createdby")
@JsonIgnore
public AppmoduleCollectionRequest getSystemuser_appmodule_createdby() {
return new AppmoduleCollectionRequest(
contextPath.addSegment("systemuser_appmodule_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_appmodule_createdby"));
}
@NavigationProperty(name="lk_processsession_createdby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_createdby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_createdby"), RequestHelper.getValue(unmappedFields, "lk_processsession_createdby"));
}
@NavigationProperty(name="lk_audit_callinguserid")
@JsonIgnore
public AuditCollectionRequest getLk_audit_callinguserid() {
return new AuditCollectionRequest(
contextPath.addSegment("lk_audit_callinguserid"), RequestHelper.getValue(unmappedFields, "lk_audit_callinguserid"));
}
@NavigationProperty(name="SystemUser_Imports")
@JsonIgnore
public ImportCollectionRequest getSystemUser_Imports() {
return new ImportCollectionRequest(
contextPath.addSegment("SystemUser_Imports"), RequestHelper.getValue(unmappedFields, "SystemUser_Imports"));
}
@NavigationProperty(name="lk_transactioncurrency_createdonbehalfby")
@JsonIgnore
public TransactioncurrencyCollectionRequest getLk_transactioncurrency_createdonbehalfby() {
return new TransactioncurrencyCollectionRequest(
contextPath.addSegment("lk_transactioncurrency_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transactioncurrency_createdonbehalfby"));
}
@NavigationProperty(name="system_user_activity_parties")
@JsonIgnore
public ActivitypartyCollectionRequest getSystem_user_activity_parties() {
return new ActivitypartyCollectionRequest(
contextPath.addSegment("system_user_activity_parties"), RequestHelper.getValue(unmappedFields, "system_user_activity_parties"));
}
@NavigationProperty(name="lk_emailserverprofile_createdby")
@JsonIgnore
public EmailserverprofileCollectionRequest getLk_emailserverprofile_createdby() {
return new EmailserverprofileCollectionRequest(
contextPath.addSegment("lk_emailserverprofile_createdby"), RequestHelper.getValue(unmappedFields, "lk_emailserverprofile_createdby"));
}
@NavigationProperty(name="lk_slaitembase_createdonbehalfby")
@JsonIgnore
public SlaitemCollectionRequest getLk_slaitembase_createdonbehalfby() {
return new SlaitemCollectionRequest(
contextPath.addSegment("lk_slaitembase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slaitembase_createdonbehalfby"));
}
@NavigationProperty(name="lk_businessunitnewsarticlebase_createdby")
@JsonIgnore
public BusinessunitnewsarticleCollectionRequest getLk_businessunitnewsarticlebase_createdby() {
return new BusinessunitnewsarticleCollectionRequest(
contextPath.addSegment("lk_businessunitnewsarticlebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_businessunitnewsarticlebase_createdby"));
}
@NavigationProperty(name="lk_pluginassembly_modifiedonbehalfby")
@JsonIgnore
public PluginassemblyCollectionRequest getLk_pluginassembly_modifiedonbehalfby() {
return new PluginassemblyCollectionRequest(
contextPath.addSegment("lk_pluginassembly_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_pluginassembly_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_contact_modifiedonbehalfby")
@JsonIgnore
public ContactCollectionRequest getLk_contact_modifiedonbehalfby() {
return new ContactCollectionRequest(
contextPath.addSegment("lk_contact_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_contact_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_subjectbase_modifiedby")
@JsonIgnore
public SubjectCollectionRequest getLk_subjectbase_modifiedby() {
return new SubjectCollectionRequest(
contextPath.addSegment("lk_subjectbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_subjectbase_modifiedby"));
}
@NavigationProperty(name="lk_phonecall_createdby")
@JsonIgnore
public PhonecallCollectionRequest getLk_phonecall_createdby() {
return new PhonecallCollectionRequest(
contextPath.addSegment("lk_phonecall_createdby"), RequestHelper.getValue(unmappedFields, "lk_phonecall_createdby"));
}
@NavigationProperty(name="lk_quarterlyfiscalcalendar_createdby")
@JsonIgnore
public QuarterlyfiscalcalendarCollectionRequest getLk_quarterlyfiscalcalendar_createdby() {
return new QuarterlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_quarterlyfiscalcalendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_quarterlyfiscalcalendar_createdby"));
}
@NavigationProperty(name="lk_actioncardbase_modifiedonbehalfby")
@JsonIgnore
public ActioncardCollectionRequest getLk_actioncardbase_modifiedonbehalfby() {
return new ActioncardCollectionRequest(
contextPath.addSegment("lk_actioncardbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_actioncardbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_tracelog_modifiedonbehalfby")
@JsonIgnore
public TracelogCollectionRequest getLk_tracelog_modifiedonbehalfby() {
return new TracelogCollectionRequest(
contextPath.addSegment("lk_tracelog_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_tracelog_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_appmodulecomponent_modifiedonbehalfby")
@JsonIgnore
public AppmodulecomponentCollectionRequest getLk_appmodulecomponent_modifiedonbehalfby() {
return new AppmodulecomponentCollectionRequest(
contextPath.addSegment("lk_appmodulecomponent_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_appmodulecomponent_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_subject_modifiedonbehalfby")
@JsonIgnore
public SubjectCollectionRequest getLk_subject_modifiedonbehalfby() {
return new SubjectCollectionRequest(
contextPath.addSegment("lk_subject_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_subject_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_customcontrolresource_createdby")
@JsonIgnore
public CustomcontrolresourceCollectionRequest getLk_customcontrolresource_createdby() {
return new CustomcontrolresourceCollectionRequest(
contextPath.addSegment("lk_customcontrolresource_createdby"), RequestHelper.getValue(unmappedFields, "lk_customcontrolresource_createdby"));
}
@NavigationProperty(name="lk_picklistmapping_createdonbehalfby")
@JsonIgnore
public PicklistmappingCollectionRequest getLk_picklistmapping_createdonbehalfby() {
return new PicklistmappingCollectionRequest(
contextPath.addSegment("lk_picklistmapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_picklistmapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_fixedmonthlyfiscalcalendar_createdonbehalfby")
@JsonIgnore
public FixedmonthlyfiscalcalendarCollectionRequest getLk_fixedmonthlyfiscalcalendar_createdonbehalfby() {
return new FixedmonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fixedmonthlyfiscalcalendar_createdonbehalfby"));
}
@NavigationProperty(name="modifiedby_sdkmessagefilter")
@JsonIgnore
public SdkmessagefilterCollectionRequest getModifiedby_sdkmessagefilter() {
return new SdkmessagefilterCollectionRequest(
contextPath.addSegment("modifiedby_sdkmessagefilter"), RequestHelper.getValue(unmappedFields, "modifiedby_sdkmessagefilter"));
}
@NavigationProperty(name="lk_sharepointsitebase_createdonbehalfby")
@JsonIgnore
public SharepointsiteCollectionRequest getLk_sharepointsitebase_createdonbehalfby() {
return new SharepointsiteCollectionRequest(
contextPath.addSegment("lk_sharepointsitebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sharepointsitebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_reportcategory_createdonbehalfby")
@JsonIgnore
public ReportcategoryCollectionRequest getLk_reportcategory_createdonbehalfby() {
return new ReportcategoryCollectionRequest(
contextPath.addSegment("lk_reportcategory_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_reportcategory_createdonbehalfby"));
}
@NavigationProperty(name="lk_templatebase_createdonbehalfby")
@JsonIgnore
public TemplateCollectionRequest getLk_templatebase_createdonbehalfby() {
return new TemplateCollectionRequest(
contextPath.addSegment("lk_templatebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_templatebase_createdonbehalfby"));
}
@NavigationProperty(name="user_email")
@JsonIgnore
public EmailCollectionRequest getUser_email() {
return new EmailCollectionRequest(
contextPath.addSegment("user_email"), RequestHelper.getValue(unmappedFields, "user_email"));
}
@NavigationProperty(name="user_fax")
@JsonIgnore
public FaxCollectionRequest getUser_fax() {
return new FaxCollectionRequest(
contextPath.addSegment("user_fax"), RequestHelper.getValue(unmappedFields, "user_fax"));
}
@NavigationProperty(name="lk_feedback_modifiedby")
@JsonIgnore
public FeedbackCollectionRequest getLk_feedback_modifiedby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_feedback_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_feedback_modifiedby"));
}
@NavigationProperty(name="lk_contactbase_createdby")
@JsonIgnore
public ContactCollectionRequest getLk_contactbase_createdby() {
return new ContactCollectionRequest(
contextPath.addSegment("lk_contactbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_contactbase_createdby"));
}
@NavigationProperty(name="lk_timezonelocalizedname_modifiedonbehalfby")
@JsonIgnore
public TimezonelocalizednameCollectionRequest getLk_timezonelocalizedname_modifiedonbehalfby() {
return new TimezonelocalizednameCollectionRequest(
contextPath.addSegment("lk_timezonelocalizedname_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonelocalizedname_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_email_modifiedby")
@JsonIgnore
public EmailCollectionRequest getLk_email_modifiedby() {
return new EmailCollectionRequest(
contextPath.addSegment("lk_email_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_email_modifiedby"));
}
@NavigationProperty(name="lk_phonecall_createdonbehalfby")
@JsonIgnore
public PhonecallCollectionRequest getLk_phonecall_createdonbehalfby() {
return new PhonecallCollectionRequest(
contextPath.addSegment("lk_phonecall_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_phonecall_createdonbehalfby"));
}
@NavigationProperty(name="lk_businessunitbase_createdby")
@JsonIgnore
public BusinessunitCollectionRequest getLk_businessunitbase_createdby() {
return new BusinessunitCollectionRequest(
contextPath.addSegment("lk_businessunitbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_businessunitbase_createdby"));
}
@NavigationProperty(name="lk_category_createdonbehalfby")
@JsonIgnore
public CategoryCollectionRequest getLk_category_createdonbehalfby() {
return new CategoryCollectionRequest(
contextPath.addSegment("lk_category_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_category_createdonbehalfby"));
}
@NavigationProperty(name="lk_slakpiinstancebase_modifiedby")
@JsonIgnore
public SlakpiinstanceCollectionRequest getLk_slakpiinstancebase_modifiedby() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("lk_slakpiinstancebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_slakpiinstancebase_modifiedby"));
}
@NavigationProperty(name="queue_primary_user")
@JsonIgnore
public QueueCollectionRequest getQueue_primary_user() {
return new QueueCollectionRequest(
contextPath.addSegment("queue_primary_user"), RequestHelper.getValue(unmappedFields, "queue_primary_user"));
}
@NavigationProperty(name="lk_importjobbase_modifiedonbehalfby")
@JsonIgnore
public ImportjobCollectionRequest getLk_importjobbase_modifiedonbehalfby() {
return new ImportjobCollectionRequest(
contextPath.addSegment("lk_importjobbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importjobbase_modifiedonbehalfby"));
}
@NavigationProperty(name="SystemUser_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getSystemUser_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("SystemUser_AsyncOperations"), RequestHelper.getValue(unmappedFields, "SystemUser_AsyncOperations"));
}
@NavigationProperty(name="lk_organization_createdonbehalfby")
@JsonIgnore
public OrganizationCollectionRequest getLk_organization_createdonbehalfby() {
return new OrganizationCollectionRequest(
contextPath.addSegment("lk_organization_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_organization_createdonbehalfby"));
}
@NavigationProperty(name="lk_DisplayStringbase_createdonbehalfby")
@JsonIgnore
public DisplaystringCollectionRequest getLk_DisplayStringbase_createdonbehalfby() {
return new DisplaystringCollectionRequest(
contextPath.addSegment("lk_DisplayStringbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_DisplayStringbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_calendarrule_modifiedonbehalfby")
@JsonIgnore
public CalendarruleCollectionRequest getLk_calendarrule_modifiedonbehalfby() {
return new CalendarruleCollectionRequest(
contextPath.addSegment("lk_calendarrule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_calendarrule_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_importjobbase_createdby")
@JsonIgnore
public ImportjobCollectionRequest getLk_importjobbase_createdby() {
return new ImportjobCollectionRequest(
contextPath.addSegment("lk_importjobbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importjobbase_createdby"));
}
@NavigationProperty(name="SystemUser_ImportFiles")
@JsonIgnore
public ImportfileCollectionRequest getSystemUser_ImportFiles() {
return new ImportfileCollectionRequest(
contextPath.addSegment("SystemUser_ImportFiles"), RequestHelper.getValue(unmappedFields, "SystemUser_ImportFiles"));
}
@NavigationProperty(name="lk_columnmapping_createdonbehalfby")
@JsonIgnore
public ColumnmappingCollectionRequest getLk_columnmapping_createdonbehalfby() {
return new ColumnmappingCollectionRequest(
contextPath.addSegment("lk_columnmapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_columnmapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_importfilebase_modifiedonbehalfby")
@JsonIgnore
public ImportfileCollectionRequest getLk_importfilebase_modifiedonbehalfby() {
return new ImportfileCollectionRequest(
contextPath.addSegment("lk_importfilebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importfilebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_mailboxtrackingfolder_createdby")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getLk_mailboxtrackingfolder_createdby() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("lk_mailboxtrackingfolder_createdby"), RequestHelper.getValue(unmappedFields, "lk_mailboxtrackingfolder_createdby"));
}
@NavigationProperty(name="socialProfile_owning_user")
@JsonIgnore
public SocialprofileCollectionRequest getSocialProfile_owning_user() {
return new SocialprofileCollectionRequest(
contextPath.addSegment("socialProfile_owning_user"), RequestHelper.getValue(unmappedFields, "socialProfile_owning_user"));
}
@NavigationProperty(name="lk_appmodulecomponent_createdonbehalfby")
@JsonIgnore
public AppmodulecomponentCollectionRequest getLk_appmodulecomponent_createdonbehalfby() {
return new AppmodulecomponentCollectionRequest(
contextPath.addSegment("lk_appmodulecomponent_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_appmodulecomponent_createdonbehalfby"));
}
@NavigationProperty(name="lk_ACIViewMapper_createdonbehalfby")
@JsonIgnore
public AciviewmapperCollectionRequest getLk_ACIViewMapper_createdonbehalfby() {
return new AciviewmapperCollectionRequest(
contextPath.addSegment("lk_ACIViewMapper_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ACIViewMapper_createdonbehalfby"));
}
@NavigationProperty(name="lk_businessunit_modifiedonbehalfby")
@JsonIgnore
public BusinessunitCollectionRequest getLk_businessunit_modifiedonbehalfby() {
return new BusinessunitCollectionRequest(
contextPath.addSegment("lk_businessunit_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_businessunit_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_appointment_modifiedonbehalfby")
@JsonIgnore
public AppointmentCollectionRequest getLk_appointment_modifiedonbehalfby() {
return new AppointmentCollectionRequest(
contextPath.addSegment("lk_appointment_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_appointment_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstepimage_modifiedonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepimageCollectionRequest getLk_sdkmessageprocessingstepimage_modifiedonbehalfby() {
return new SdkmessageprocessingstepimageCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstepimage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstepimage_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticletemplate_createdonbehalfby")
@JsonIgnore
public KbarticletemplateCollectionRequest getLk_kbarticletemplate_createdonbehalfby() {
return new KbarticletemplateCollectionRequest(
contextPath.addSegment("lk_kbarticletemplate_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticletemplate_createdonbehalfby"));
}
@NavigationProperty(name="lk_documenttemplatebase_modifiedonbehalfby")
@JsonIgnore
public DocumenttemplateCollectionRequest getLk_documenttemplatebase_modifiedonbehalfby() {
return new DocumenttemplateCollectionRequest(
contextPath.addSegment("lk_documenttemplatebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_documenttemplatebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_processsession_executedby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_executedby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_executedby"), RequestHelper.getValue(unmappedFields, "lk_processsession_executedby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstep_modifiedonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getLk_sdkmessageprocessingstep_modifiedonbehalfby() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstep_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstep_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_monthlyfiscalcalendar_createdby")
@JsonIgnore
public MonthlyfiscalcalendarCollectionRequest getLk_monthlyfiscalcalendar_createdby() {
return new MonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_monthlyfiscalcalendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_monthlyfiscalcalendar_createdby"));
}
@NavigationProperty(name="lk_syncerrorbase_modifiedby")
@JsonIgnore
public SyncerrorCollectionRequest getLk_syncerrorbase_modifiedby() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("lk_syncerrorbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_syncerrorbase_modifiedby"));
}
@NavigationProperty(name="systemuser_appconfig_modifiedonbehalfby")
@JsonIgnore
public AppconfigCollectionRequest getSystemuser_appconfig_modifiedonbehalfby() {
return new AppconfigCollectionRequest(
contextPath.addSegment("systemuser_appconfig_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfig_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_reportbase_createdby")
@JsonIgnore
public ReportCollectionRequest getLk_reportbase_createdby() {
return new ReportCollectionRequest(
contextPath.addSegment("lk_reportbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_reportbase_createdby"));
}
@NavigationProperty(name="lk_documenttemplatebase_createdby")
@JsonIgnore
public DocumenttemplateCollectionRequest getLk_documenttemplatebase_createdby() {
return new DocumenttemplateCollectionRequest(
contextPath.addSegment("lk_documenttemplatebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_documenttemplatebase_createdby"));
}
@NavigationProperty(name="lk_subject_createdonbehalfby")
@JsonIgnore
public SubjectCollectionRequest getLk_subject_createdonbehalfby() {
return new SubjectCollectionRequest(
contextPath.addSegment("lk_subject_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_subject_createdonbehalfby"));
}
@NavigationProperty(name="lk_recurringappointmentmaster_modifiedonbehalfby")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getLk_recurringappointmentmaster_modifiedonbehalfby() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("lk_recurringappointmentmaster_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_recurringappointmentmaster_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_reportbase_modifiedby")
@JsonIgnore
public ReportCollectionRequest getLk_reportbase_modifiedby() {
return new ReportCollectionRequest(
contextPath.addSegment("lk_reportbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_reportbase_modifiedby"));
}
@NavigationProperty(name="lk_userformbase_modifiedonbehalfby")
@JsonIgnore
public UserformCollectionRequest getLk_userformbase_modifiedonbehalfby() {
return new UserformCollectionRequest(
contextPath.addSegment("lk_userformbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userformbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_bulkdeleteoperationbase_createdby")
@JsonIgnore
public BulkdeleteoperationCollectionRequest getLk_bulkdeleteoperationbase_createdby() {
return new BulkdeleteoperationCollectionRequest(
contextPath.addSegment("lk_bulkdeleteoperationbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_bulkdeleteoperationbase_createdby"));
}
@NavigationProperty(name="lk_transformationmapping_modifiedonbehalfby")
@JsonIgnore
public TransformationmappingCollectionRequest getLk_transformationmapping_modifiedonbehalfby() {
return new TransformationmappingCollectionRequest(
contextPath.addSegment("lk_transformationmapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transformationmapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_feedback_modifiedonbehalfby")
@JsonIgnore
public FeedbackCollectionRequest getLk_feedback_modifiedonbehalfby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_feedback_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_feedback_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_slabase_modifiedby")
@JsonIgnore
public SlaCollectionRequest getLk_slabase_modifiedby() {
return new SlaCollectionRequest(
contextPath.addSegment("lk_slabase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_slabase_modifiedby"));
}
@NavigationProperty(name="lk_personaldocumenttemplatebase_createdonbehalfby")
@JsonIgnore
public PersonaldocumenttemplateCollectionRequest getLk_personaldocumenttemplatebase_createdonbehalfby() {
return new PersonaldocumenttemplateCollectionRequest(
contextPath.addSegment("lk_personaldocumenttemplatebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_personaldocumenttemplatebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_activitypointer_modifiedonbehalfby")
@JsonIgnore
public ActivitypointerCollectionRequest getLk_activitypointer_modifiedonbehalfby() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("lk_activitypointer_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_activitypointer_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_socialactivity_createdby")
@JsonIgnore
public SocialactivityCollectionRequest getLk_socialactivity_createdby() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("lk_socialactivity_createdby"), RequestHelper.getValue(unmappedFields, "lk_socialactivity_createdby"));
}
@NavigationProperty(name="systemuser_navigationsetting_createdonbehalfby")
@JsonIgnore
public NavigationsettingCollectionRequest getSystemuser_navigationsetting_createdonbehalfby() {
return new NavigationsettingCollectionRequest(
contextPath.addSegment("systemuser_navigationsetting_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_navigationsetting_createdonbehalfby"));
}
@NavigationProperty(name="lk_fax_modifiedby")
@JsonIgnore
public FaxCollectionRequest getLk_fax_modifiedby() {
return new FaxCollectionRequest(
contextPath.addSegment("lk_fax_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_fax_modifiedby"));
}
@NavigationProperty(name="lk_userformbase_createdonbehalfby")
@JsonIgnore
public UserformCollectionRequest getLk_userformbase_createdonbehalfby() {
return new UserformCollectionRequest(
contextPath.addSegment("lk_userformbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userformbase_createdonbehalfby"));
}
@NavigationProperty(name="modifiedby_sdkmessageprocessingstep")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getModifiedby_sdkmessageprocessingstep() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("modifiedby_sdkmessageprocessingstep"), RequestHelper.getValue(unmappedFields, "modifiedby_sdkmessageprocessingstep"));
}
@NavigationProperty(name="createdby_plugintype")
@JsonIgnore
public PlugintypeCollectionRequest getCreatedby_plugintype() {
return new PlugintypeCollectionRequest(
contextPath.addSegment("createdby_plugintype"), RequestHelper.getValue(unmappedFields, "createdby_plugintype"));
}
@NavigationProperty(name="lk_sdkmessage_createdonbehalfby")
@JsonIgnore
public SdkmessageCollectionRequest getLk_sdkmessage_createdonbehalfby() {
return new SdkmessageCollectionRequest(
contextPath.addSegment("lk_sdkmessage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessage_createdonbehalfby"));
}
@NavigationProperty(name="lk_customcontroldefaultconfig_createdonbehalfby")
@JsonIgnore
public CustomcontroldefaultconfigCollectionRequest getLk_customcontroldefaultconfig_createdonbehalfby() {
return new CustomcontroldefaultconfigCollectionRequest(
contextPath.addSegment("lk_customcontroldefaultconfig_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontroldefaultconfig_createdonbehalfby"));
}
@NavigationProperty(name="lk_pluginassembly_createdonbehalfby")
@JsonIgnore
public PluginassemblyCollectionRequest getLk_pluginassembly_createdonbehalfby() {
return new PluginassemblyCollectionRequest(
contextPath.addSegment("lk_pluginassembly_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_pluginassembly_createdonbehalfby"));
}
@NavigationProperty(name="createdby_sdkmessagefilter")
@JsonIgnore
public SdkmessagefilterCollectionRequest getCreatedby_sdkmessagefilter() {
return new SdkmessagefilterCollectionRequest(
contextPath.addSegment("createdby_sdkmessagefilter"), RequestHelper.getValue(unmappedFields, "createdby_sdkmessagefilter"));
}
@NavigationProperty(name="lk_knowledgearticleviews_modifiedby")
@JsonIgnore
public KnowledgearticleviewsCollectionRequest getLk_knowledgearticleviews_modifiedby() {
return new KnowledgearticleviewsCollectionRequest(
contextPath.addSegment("lk_knowledgearticleviews_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticleviews_modifiedby"));
}
@NavigationProperty(name="lk_connectionrolebase_createdonbehalfby")
@JsonIgnore
public ConnectionroleCollectionRequest getLk_connectionrolebase_createdonbehalfby() {
return new ConnectionroleCollectionRequest(
contextPath.addSegment("lk_connectionrolebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionrolebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_workflowlog_modifiedonbehalfby")
@JsonIgnore
public WorkflowlogCollectionRequest getLk_workflowlog_modifiedonbehalfby() {
return new WorkflowlogCollectionRequest(
contextPath.addSegment("lk_workflowlog_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_workflowlog_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_feedback_closedby")
@JsonIgnore
public FeedbackCollectionRequest getLk_feedback_closedby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_feedback_closedby"), RequestHelper.getValue(unmappedFields, "lk_feedback_closedby"));
}
@NavigationProperty(name="systemuser_appconfigmaster_createdby")
@JsonIgnore
public AppconfigmasterCollectionRequest getSystemuser_appconfigmaster_createdby() {
return new AppconfigmasterCollectionRequest(
contextPath.addSegment("systemuser_appconfigmaster_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfigmaster_createdby"));
}
@NavigationProperty(name="lk_usersettingsbase_modifiedby")
@JsonIgnore
public UsersettingsCollectionRequest getLk_usersettingsbase_modifiedby() {
return new UsersettingsCollectionRequest(
contextPath.addSegment("lk_usersettingsbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_usersettingsbase_modifiedby"));
}
@NavigationProperty(name="lk_sharepointdocumentlocationbase_createdby")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getLk_sharepointdocumentlocationbase_createdby() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("lk_sharepointdocumentlocationbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_sharepointdocumentlocationbase_createdby"));
}
@NavigationProperty(name="lk_fax_modifiedonbehalfby")
@JsonIgnore
public FaxCollectionRequest getLk_fax_modifiedonbehalfby() {
return new FaxCollectionRequest(
contextPath.addSegment("lk_fax_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fax_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_feedback_createdonbehalfby")
@JsonIgnore
public FeedbackCollectionRequest getLk_feedback_createdonbehalfby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_feedback_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_feedback_createdonbehalfby"));
}
@NavigationProperty(name="systemuser_appconfiginstance_createdonbehalfby")
@JsonIgnore
public AppconfiginstanceCollectionRequest getSystemuser_appconfiginstance_createdonbehalfby() {
return new AppconfiginstanceCollectionRequest(
contextPath.addSegment("systemuser_appconfiginstance_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfiginstance_createdonbehalfby"));
}
@NavigationProperty(name="systemuser_SiteMap_createdby")
@JsonIgnore
public SitemapCollectionRequest getSystemuser_SiteMap_createdby() {
return new SitemapCollectionRequest(
contextPath.addSegment("systemuser_SiteMap_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_SiteMap_createdby"));
}
@NavigationProperty(name="lk_asyncoperation_modifiedby")
@JsonIgnore
public AsyncoperationCollectionRequest getLk_asyncoperation_modifiedby() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_asyncoperation_modifiedby"));
}
@NavigationProperty(name="lk_savedquery_modifiedonbehalfby")
@JsonIgnore
public SavedqueryCollectionRequest getLk_savedquery_modifiedonbehalfby() {
return new SavedqueryCollectionRequest(
contextPath.addSegment("lk_savedquery_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_savedquery_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_workflowlog_modifiedby")
@JsonIgnore
public WorkflowlogCollectionRequest getLk_workflowlog_modifiedby() {
return new WorkflowlogCollectionRequest(
contextPath.addSegment("lk_workflowlog_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_workflowlog_modifiedby"));
}
@NavigationProperty(name="lk_processtriggerbase_modifiedonbehalfby")
@JsonIgnore
public ProcesstriggerCollectionRequest getLk_processtriggerbase_modifiedonbehalfby() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("lk_processtriggerbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processtriggerbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_webwizard_createdonbehalfby")
@JsonIgnore
public WebwizardCollectionRequest getLk_webwizard_createdonbehalfby() {
return new WebwizardCollectionRequest(
contextPath.addSegment("lk_webwizard_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_webwizard_createdonbehalfby"));
}
@NavigationProperty(name="lk_webwizard_modifiedonbehalfby")
@JsonIgnore
public WebwizardCollectionRequest getLk_webwizard_modifiedonbehalfby() {
return new WebwizardCollectionRequest(
contextPath.addSegment("lk_webwizard_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_webwizard_modifiedonbehalfby"));
}
@NavigationProperty(name="systemuser_appconfig_createdonbehalfby")
@JsonIgnore
public AppconfigCollectionRequest getSystemuser_appconfig_createdonbehalfby() {
return new AppconfigCollectionRequest(
contextPath.addSegment("systemuser_appconfig_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfig_createdonbehalfby"));
}
@NavigationProperty(name="lk_appointment_modifiedby")
@JsonIgnore
public AppointmentCollectionRequest getLk_appointment_modifiedby() {
return new AppointmentCollectionRequest(
contextPath.addSegment("lk_appointment_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_appointment_modifiedby"));
}
@NavigationProperty(name="lk_organizationbase_createdby")
@JsonIgnore
public OrganizationCollectionRequest getLk_organizationbase_createdby() {
return new OrganizationCollectionRequest(
contextPath.addSegment("lk_organizationbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_organizationbase_createdby"));
}
@NavigationProperty(name="lk_timezonelocalizedname_modifiedby")
@JsonIgnore
public TimezonelocalizednameCollectionRequest getLk_timezonelocalizedname_modifiedby() {
return new TimezonelocalizednameCollectionRequest(
contextPath.addSegment("lk_timezonelocalizedname_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_timezonelocalizedname_modifiedby"));
}
@NavigationProperty(name="lk_activitypointer_createdonbehalfby")
@JsonIgnore
public ActivitypointerCollectionRequest getLk_activitypointer_createdonbehalfby() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("lk_activitypointer_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_activitypointer_createdonbehalfby"));
}
@NavigationProperty(name="lk_duplicaterulecondition_createdonbehalfby")
@JsonIgnore
public DuplicateruleconditionCollectionRequest getLk_duplicaterulecondition_createdonbehalfby() {
return new DuplicateruleconditionCollectionRequest(
contextPath.addSegment("lk_duplicaterulecondition_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterulecondition_createdonbehalfby"));
}
@NavigationProperty(name="lk_mailmergetemplate_createdonbehalfby")
@JsonIgnore
public MailmergetemplateCollectionRequest getLk_mailmergetemplate_createdonbehalfby() {
return new MailmergetemplateCollectionRequest(
contextPath.addSegment("lk_mailmergetemplate_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailmergetemplate_createdonbehalfby"));
}
@NavigationProperty(name="lk_importdata_modifiedonbehalfby")
@JsonIgnore
public ImportdataCollectionRequest getLk_importdata_modifiedonbehalfby() {
return new ImportdataCollectionRequest(
contextPath.addSegment("lk_importdata_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importdata_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_transformationmapping_createdonbehalfby")
@JsonIgnore
public TransformationmappingCollectionRequest getLk_transformationmapping_createdonbehalfby() {
return new TransformationmappingCollectionRequest(
contextPath.addSegment("lk_transformationmapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transformationmapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_semiannualfiscalcalendar_salespersonid")
@JsonIgnore
public SemiannualfiscalcalendarCollectionRequest getLk_semiannualfiscalcalendar_salespersonid() {
return new SemiannualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_semiannualfiscalcalendar_salespersonid"), RequestHelper.getValue(unmappedFields, "lk_semiannualfiscalcalendar_salespersonid"));
}
@NavigationProperty(name="lk_transformationparametermapping_createdonbehalfby")
@JsonIgnore
public TransformationparametermappingCollectionRequest getLk_transformationparametermapping_createdonbehalfby() {
return new TransformationparametermappingCollectionRequest(
contextPath.addSegment("lk_transformationparametermapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transformationparametermapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_connectionbase_modifiedonbehalfby")
@JsonIgnore
public ConnectionCollectionRequest getLk_connectionbase_modifiedonbehalfby() {
return new ConnectionCollectionRequest(
contextPath.addSegment("lk_connectionbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_plugintypestatisticbase_createdonbehalfby")
@JsonIgnore
public PlugintypestatisticCollectionRequest getLk_plugintypestatisticbase_createdonbehalfby() {
return new PlugintypestatisticCollectionRequest(
contextPath.addSegment("lk_plugintypestatisticbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_plugintypestatisticbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_transformationmapping_createdby")
@JsonIgnore
public TransformationmappingCollectionRequest getLk_transformationmapping_createdby() {
return new TransformationmappingCollectionRequest(
contextPath.addSegment("lk_transformationmapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_transformationmapping_createdby"));
}
@NavigationProperty(name="lk_MobileOfflineProfile_createdby")
@JsonIgnore
public MobileofflineprofileCollectionRequest getLk_MobileOfflineProfile_createdby() {
return new MobileofflineprofileCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfile_createdby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfile_createdby"));
}
@NavigationProperty(name="lk_syncerrorbase_createdby")
@JsonIgnore
public SyncerrorCollectionRequest getLk_syncerrorbase_createdby() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("lk_syncerrorbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_syncerrorbase_createdby"));
}
@NavigationProperty(name="lk_documenttemplatebase_modifiedby")
@JsonIgnore
public DocumenttemplateCollectionRequest getLk_documenttemplatebase_modifiedby() {
return new DocumenttemplateCollectionRequest(
contextPath.addSegment("lk_documenttemplatebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_documenttemplatebase_modifiedby"));
}
@NavigationProperty(name="lk_task_modifiedby")
@JsonIgnore
public TaskCollectionRequest getLk_task_modifiedby() {
return new TaskCollectionRequest(
contextPath.addSegment("lk_task_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_task_modifiedby"));
}
@NavigationProperty(name="lk_kbarticlebase_createdby")
@JsonIgnore
public KbarticleCollectionRequest getLk_kbarticlebase_createdby() {
return new KbarticleCollectionRequest(
contextPath.addSegment("lk_kbarticlebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlebase_createdby"));
}
@NavigationProperty(name="lk_task_createdby")
@JsonIgnore
public TaskCollectionRequest getLk_task_createdby() {
return new TaskCollectionRequest(
contextPath.addSegment("lk_task_createdby"), RequestHelper.getValue(unmappedFields, "lk_task_createdby"));
}
@NavigationProperty(name="lk_translationprocess_modifiedonbehalfby")
@JsonIgnore
public TranslationprocessCollectionRequest getLk_translationprocess_modifiedonbehalfby() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("lk_translationprocess_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_translationprocess_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_timezonedefinition_modifiedonbehalfby")
@JsonIgnore
public TimezonedefinitionCollectionRequest getLk_timezonedefinition_modifiedonbehalfby() {
return new TimezonedefinitionCollectionRequest(
contextPath.addSegment("lk_timezonedefinition_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonedefinition_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_duplicaterulecondition_modifiedonbehalfby")
@JsonIgnore
public DuplicateruleconditionCollectionRequest getLk_duplicaterulecondition_modifiedonbehalfby() {
return new DuplicateruleconditionCollectionRequest(
contextPath.addSegment("lk_duplicaterulecondition_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterulecondition_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_asyncoperation_createdonbehalfby")
@JsonIgnore
public AsyncoperationCollectionRequest getLk_asyncoperation_createdonbehalfby() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_asyncoperation_createdonbehalfby"));
}
@NavigationProperty(name="lk_activitypointer_modifiedby")
@JsonIgnore
public ActivitypointerCollectionRequest getLk_activitypointer_modifiedby() {
return new ActivitypointerCollectionRequest(
contextPath.addSegment("lk_activitypointer_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_activitypointer_modifiedby"));
}
@NavigationProperty(name="lk_queuebase_createdby")
@JsonIgnore
public QueueCollectionRequest getLk_queuebase_createdby() {
return new QueueCollectionRequest(
contextPath.addSegment("lk_queuebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_queuebase_createdby"));
}
@NavigationProperty(name="lk_transformationmapping_modifiedby")
@JsonIgnore
public TransformationmappingCollectionRequest getLk_transformationmapping_modifiedby() {
return new TransformationmappingCollectionRequest(
contextPath.addSegment("lk_transformationmapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_transformationmapping_modifiedby"));
}
@NavigationProperty(name="systemuser_appconfig_createdby")
@JsonIgnore
public AppconfigCollectionRequest getSystemuser_appconfig_createdby() {
return new AppconfigCollectionRequest(
contextPath.addSegment("systemuser_appconfig_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfig_createdby"));
}
@NavigationProperty(name="user_settings")
@JsonIgnore
public UsersettingsCollectionRequest getUser_settings() {
return new UsersettingsCollectionRequest(
contextPath.addSegment("user_settings"), RequestHelper.getValue(unmappedFields, "user_settings"));
}
@NavigationProperty(name="systemuser_appconfiginstance_modifiedby")
@JsonIgnore
public AppconfiginstanceCollectionRequest getSystemuser_appconfiginstance_modifiedby() {
return new AppconfiginstanceCollectionRequest(
contextPath.addSegment("systemuser_appconfiginstance_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfiginstance_modifiedby"));
}
@NavigationProperty(name="lk_translationprocess_createdby")
@JsonIgnore
public TranslationprocessCollectionRequest getLk_translationprocess_createdby() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("lk_translationprocess_createdby"), RequestHelper.getValue(unmappedFields, "lk_translationprocess_createdby"));
}
@NavigationProperty(name="systemuser_navigationsetting_modifiedonbehalfby")
@JsonIgnore
public NavigationsettingCollectionRequest getSystemuser_navigationsetting_modifiedonbehalfby() {
return new NavigationsettingCollectionRequest(
contextPath.addSegment("systemuser_navigationsetting_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_navigationsetting_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_queueitem_modifiedonbehalfby")
@JsonIgnore
public QueueitemCollectionRequest getLk_queueitem_modifiedonbehalfby() {
return new QueueitemCollectionRequest(
contextPath.addSegment("lk_queueitem_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_queueitem_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_annotationbase_createdonbehalfby")
@JsonIgnore
public AnnotationCollectionRequest getLk_annotationbase_createdonbehalfby() {
return new AnnotationCollectionRequest(
contextPath.addSegment("lk_annotationbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_annotationbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_actioncardbase_createdonbehalfby")
@JsonIgnore
public ActioncardCollectionRequest getLk_actioncardbase_createdonbehalfby() {
return new ActioncardCollectionRequest(
contextPath.addSegment("lk_actioncardbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_actioncardbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_timezonelocalizedname_createdby")
@JsonIgnore
public TimezonelocalizednameCollectionRequest getLk_timezonelocalizedname_createdby() {
return new TimezonelocalizednameCollectionRequest(
contextPath.addSegment("lk_timezonelocalizedname_createdby"), RequestHelper.getValue(unmappedFields, "lk_timezonelocalizedname_createdby"));
}
@NavigationProperty(name="systemuser_appconfiginstance_modifiedonbehalfby")
@JsonIgnore
public AppconfiginstanceCollectionRequest getSystemuser_appconfiginstance_modifiedonbehalfby() {
return new AppconfiginstanceCollectionRequest(
contextPath.addSegment("systemuser_appconfiginstance_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfiginstance_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_import_createdonbehalfby")
@JsonIgnore
public ImportCollectionRequest getLk_import_createdonbehalfby() {
return new ImportCollectionRequest(
contextPath.addSegment("lk_import_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_import_createdonbehalfby"));
}
@NavigationProperty(name="lk_mailmergetemplate_modifiedonbehalfby")
@JsonIgnore
public MailmergetemplateCollectionRequest getLk_mailmergetemplate_modifiedonbehalfby() {
return new MailmergetemplateCollectionRequest(
contextPath.addSegment("lk_mailmergetemplate_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mailmergetemplate_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_customcontroldefaultconfig_modifiedby")
@JsonIgnore
public CustomcontroldefaultconfigCollectionRequest getLk_customcontroldefaultconfig_modifiedby() {
return new CustomcontroldefaultconfigCollectionRequest(
contextPath.addSegment("lk_customcontroldefaultconfig_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_customcontroldefaultconfig_modifiedby"));
}
@NavigationProperty(name="lk_customcontrol_createdonbehalfby")
@JsonIgnore
public CustomcontrolCollectionRequest getLk_customcontrol_createdonbehalfby() {
return new CustomcontrolCollectionRequest(
contextPath.addSegment("lk_customcontrol_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontrol_createdonbehalfby"));
}
@NavigationProperty(name="lk_usersettings_modifiedonbehalfby")
@JsonIgnore
public UsersettingsCollectionRequest getLk_usersettings_modifiedonbehalfby() {
return new UsersettingsCollectionRequest(
contextPath.addSegment("lk_usersettings_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_usersettings_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_ownermapping_modifiedonbehalfby")
@JsonIgnore
public OwnermappingCollectionRequest getLk_ownermapping_modifiedonbehalfby() {
return new OwnermappingCollectionRequest(
contextPath.addSegment("lk_ownermapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ownermapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_MobileOfflineProfile_modifiedby")
@JsonIgnore
public MobileofflineprofileCollectionRequest getLk_MobileOfflineProfile_modifiedby() {
return new MobileofflineprofileCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfile_modifiedby"));
}
@NavigationProperty(name="lk_lookupmapping_createdonbehalfby")
@JsonIgnore
public LookupmappingCollectionRequest getLk_lookupmapping_createdonbehalfby() {
return new LookupmappingCollectionRequest(
contextPath.addSegment("lk_lookupmapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_lookupmapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_plugintype_modifiedonbehalfby")
@JsonIgnore
public PlugintypeCollectionRequest getLk_plugintype_modifiedonbehalfby() {
return new PlugintypeCollectionRequest(
contextPath.addSegment("lk_plugintype_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_plugintype_modifiedonbehalfby"));
}
@NavigationProperty(name="appmodulecomponent_createdby")
@JsonIgnore
public AppmodulecomponentCollectionRequest getAppmodulecomponent_createdby() {
return new AppmodulecomponentCollectionRequest(
contextPath.addSegment("appmodulecomponent_createdby"), RequestHelper.getValue(unmappedFields, "appmodulecomponent_createdby"));
}
@NavigationProperty(name="lk_processsession_modifiedby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_modifiedby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_processsession_modifiedby"));
}
@NavigationProperty(name="lk_transformationparametermapping_modifiedby")
@JsonIgnore
public TransformationparametermappingCollectionRequest getLk_transformationparametermapping_modifiedby() {
return new TransformationparametermappingCollectionRequest(
contextPath.addSegment("lk_transformationparametermapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_transformationparametermapping_modifiedby"));
}
@NavigationProperty(name="lk_savedqueryvisualizationbase_modifiedonbehalfby")
@JsonIgnore
public SavedqueryvisualizationCollectionRequest getLk_savedqueryvisualizationbase_modifiedonbehalfby() {
return new SavedqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_savedqueryvisualizationbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_savedqueryvisualizationbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticlecomment_modifiedonbehalfby")
@JsonIgnore
public KbarticlecommentCollectionRequest getLk_kbarticlecomment_modifiedonbehalfby() {
return new KbarticlecommentCollectionRequest(
contextPath.addSegment("lk_kbarticlecomment_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlecomment_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_rolebase_createdby")
@JsonIgnore
public RoleCollectionRequest getLk_rolebase_createdby() {
return new RoleCollectionRequest(
contextPath.addSegment("lk_rolebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_rolebase_createdby"));
}
@NavigationProperty(name="lk_bulkdeleteoperation_createdonbehalfby")
@JsonIgnore
public BulkdeleteoperationCollectionRequest getLk_bulkdeleteoperation_createdonbehalfby() {
return new BulkdeleteoperationCollectionRequest(
contextPath.addSegment("lk_bulkdeleteoperation_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_bulkdeleteoperation_createdonbehalfby"));
}
@NavigationProperty(name="lk_knowledgearticleviews_createdby")
@JsonIgnore
public KnowledgearticleviewsCollectionRequest getLk_knowledgearticleviews_createdby() {
return new KnowledgearticleviewsCollectionRequest(
contextPath.addSegment("lk_knowledgearticleviews_createdby"), RequestHelper.getValue(unmappedFields, "lk_knowledgearticleviews_createdby"));
}
@NavigationProperty(name="lk_customeraddressbase_modifiedby")
@JsonIgnore
public CustomeraddressCollectionRequest getLk_customeraddressbase_modifiedby() {
return new CustomeraddressCollectionRequest(
contextPath.addSegment("lk_customeraddressbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_customeraddressbase_modifiedby"));
}
@NavigationProperty(name="lk_translationprocess_createdonbehalfby")
@JsonIgnore
public TranslationprocessCollectionRequest getLk_translationprocess_createdonbehalfby() {
return new TranslationprocessCollectionRequest(
contextPath.addSegment("lk_translationprocess_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_translationprocess_createdonbehalfby"));
}
@NavigationProperty(name="lk_email_createdonbehalfby")
@JsonIgnore
public EmailCollectionRequest getLk_email_createdonbehalfby() {
return new EmailCollectionRequest(
contextPath.addSegment("lk_email_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_email_createdonbehalfby"));
}
@NavigationProperty(name="lk_category_modifiedby")
@JsonIgnore
public CategoryCollectionRequest getLk_category_modifiedby() {
return new CategoryCollectionRequest(
contextPath.addSegment("lk_category_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_category_modifiedby"));
}
@NavigationProperty(name="lk_quarterlyfiscalcalendar_modifiedonbehalfby")
@JsonIgnore
public QuarterlyfiscalcalendarCollectionRequest getLk_quarterlyfiscalcalendar_modifiedonbehalfby() {
return new QuarterlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_quarterlyfiscalcalendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_quarterlyfiscalcalendar_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_importfilebase_createdonbehalfby")
@JsonIgnore
public ImportfileCollectionRequest getLk_importfilebase_createdonbehalfby() {
return new ImportfileCollectionRequest(
contextPath.addSegment("lk_importfilebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importfilebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_sdkmessagefilter_createdonbehalfby")
@JsonIgnore
public SdkmessagefilterCollectionRequest getLk_sdkmessagefilter_createdonbehalfby() {
return new SdkmessagefilterCollectionRequest(
contextPath.addSegment("lk_sdkmessagefilter_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessagefilter_createdonbehalfby"));
}
@NavigationProperty(name="lk_annotationbase_modifiedby")
@JsonIgnore
public AnnotationCollectionRequest getLk_annotationbase_modifiedby() {
return new AnnotationCollectionRequest(
contextPath.addSegment("lk_annotationbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_annotationbase_modifiedby"));
}
@NavigationProperty(name="lk_queue_modifiedonbehalfby")
@JsonIgnore
public QueueCollectionRequest getLk_queue_modifiedonbehalfby() {
return new QueueCollectionRequest(
contextPath.addSegment("lk_queue_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_queue_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_sharepointdocumentlocationbase_modifiedonbehalfby")
@JsonIgnore
public SharepointdocumentlocationCollectionRequest getLk_sharepointdocumentlocationbase_modifiedonbehalfby() {
return new SharepointdocumentlocationCollectionRequest(
contextPath.addSegment("lk_sharepointdocumentlocationbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sharepointdocumentlocationbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_socialactivity_modifiedby")
@JsonIgnore
public SocialactivityCollectionRequest getLk_socialactivity_modifiedby() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("lk_socialactivity_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_socialactivity_modifiedby"));
}
@NavigationProperty(name="lk_calendar_modifiedonbehalfby")
@JsonIgnore
public CalendarCollectionRequest getLk_calendar_modifiedonbehalfby() {
return new CalendarCollectionRequest(
contextPath.addSegment("lk_calendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_calendar_modifiedonbehalfby"));
}
@NavigationProperty(name="system_user_contacts")
@JsonIgnore
public ContactCollectionRequest getSystem_user_contacts() {
return new ContactCollectionRequest(
contextPath.addSegment("system_user_contacts"), RequestHelper.getValue(unmappedFields, "system_user_contacts"));
}
@NavigationProperty(name="lk_webresourcebase_createdonbehalfby")
@JsonIgnore
public WebresourceCollectionRequest getLk_webresourcebase_createdonbehalfby() {
return new WebresourceCollectionRequest(
contextPath.addSegment("lk_webresourcebase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_webresourcebase_createdonbehalfby"));
}
@NavigationProperty(name="lk_feedback_createdby")
@JsonIgnore
public FeedbackCollectionRequest getLk_feedback_createdby() {
return new FeedbackCollectionRequest(
contextPath.addSegment("lk_feedback_createdby"), RequestHelper.getValue(unmappedFields, "lk_feedback_createdby"));
}
@NavigationProperty(name="lk_fixedmonthlyfiscalcalendar_createdby")
@JsonIgnore
public FixedmonthlyfiscalcalendarCollectionRequest getLk_fixedmonthlyfiscalcalendar_createdby() {
return new FixedmonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_fixedmonthlyfiscalcalendar_createdby"));
}
@NavigationProperty(name="lk_fieldsecurityprofile_modifiedonbehalfby")
@JsonIgnore
public FieldsecurityprofileCollectionRequest getLk_fieldsecurityprofile_modifiedonbehalfby() {
return new FieldsecurityprofileCollectionRequest(
contextPath.addSegment("lk_fieldsecurityprofile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fieldsecurityprofile_modifiedonbehalfby"));
}
@NavigationProperty(name="createdby_sdkmessageprocessingstepsecureconfig")
@JsonIgnore
public SdkmessageprocessingstepsecureconfigCollectionRequest getCreatedby_sdkmessageprocessingstepsecureconfig() {
return new SdkmessageprocessingstepsecureconfigCollectionRequest(
contextPath.addSegment("createdby_sdkmessageprocessingstepsecureconfig"), RequestHelper.getValue(unmappedFields, "createdby_sdkmessageprocessingstepsecureconfig"));
}
@NavigationProperty(name="lk_processsessionbase_createdonbehalfby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsessionbase_createdonbehalfby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsessionbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processsessionbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_webwizard_modifiedby")
@JsonIgnore
public WebwizardCollectionRequest getLk_webwizard_modifiedby() {
return new WebwizardCollectionRequest(
contextPath.addSegment("lk_webwizard_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_webwizard_modifiedby"));
}
@NavigationProperty(name="lk_teambase_modifiedby")
@JsonIgnore
public TeamCollectionRequest getLk_teambase_modifiedby() {
return new TeamCollectionRequest(
contextPath.addSegment("lk_teambase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_teambase_modifiedby"));
}
@NavigationProperty(name="lk_ACIViewMapper_modifiedonbehalfby")
@JsonIgnore
public AciviewmapperCollectionRequest getLk_ACIViewMapper_modifiedonbehalfby() {
return new AciviewmapperCollectionRequest(
contextPath.addSegment("lk_ACIViewMapper_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ACIViewMapper_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_annualfiscalcalendar_createdby")
@JsonIgnore
public AnnualfiscalcalendarCollectionRequest getLk_annualfiscalcalendar_createdby() {
return new AnnualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_annualfiscalcalendar_createdby"), RequestHelper.getValue(unmappedFields, "lk_annualfiscalcalendar_createdby"));
}
@NavigationProperty(name="lk_customcontroldefaultconfig_createdby")
@JsonIgnore
public CustomcontroldefaultconfigCollectionRequest getLk_customcontroldefaultconfig_createdby() {
return new CustomcontroldefaultconfigCollectionRequest(
contextPath.addSegment("lk_customcontroldefaultconfig_createdby"), RequestHelper.getValue(unmappedFields, "lk_customcontroldefaultconfig_createdby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitem_modifiedonbehalfby")
@JsonIgnore
public MobileofflineprofileitemCollectionRequest getLk_mobileofflineprofileitem_modifiedonbehalfby() {
return new MobileofflineprofileitemCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitem_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitem_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_annualfiscalcalendar_createdonbehalfby")
@JsonIgnore
public AnnualfiscalcalendarCollectionRequest getLk_annualfiscalcalendar_createdonbehalfby() {
return new AnnualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_annualfiscalcalendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_annualfiscalcalendar_createdonbehalfby"));
}
@NavigationProperty(name="queuemembership_association")
@JsonIgnore
public QueueCollectionRequest getQueuemembership_association() {
return new QueueCollectionRequest(
contextPath.addSegment("queuemembership_association"), RequestHelper.getValue(unmappedFields, "queuemembership_association"));
}
@NavigationProperty(name="lk_solutionbase_createdonbehalfby")
@JsonIgnore
public SolutionCollectionRequest getLk_solutionbase_createdonbehalfby() {
return new SolutionCollectionRequest(
contextPath.addSegment("lk_solutionbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutionbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_sharepointsitebase_modifiedonbehalfby")
@JsonIgnore
public SharepointsiteCollectionRequest getLk_sharepointsitebase_modifiedonbehalfby() {
return new SharepointsiteCollectionRequest(
contextPath.addSegment("lk_sharepointsitebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sharepointsitebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_syncerrorbase_createdonbehalfby")
@JsonIgnore
public SyncerrorCollectionRequest getLk_syncerrorbase_createdonbehalfby() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("lk_syncerrorbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_syncerrorbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_customcontrolresource_modifiedby")
@JsonIgnore
public CustomcontrolresourceCollectionRequest getLk_customcontrolresource_modifiedby() {
return new CustomcontrolresourceCollectionRequest(
contextPath.addSegment("lk_customcontrolresource_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_customcontrolresource_modifiedby"));
}
@NavigationProperty(name="modifiedby_pluginassembly")
@JsonIgnore
public PluginassemblyCollectionRequest getModifiedby_pluginassembly() {
return new PluginassemblyCollectionRequest(
contextPath.addSegment("modifiedby_pluginassembly"), RequestHelper.getValue(unmappedFields, "modifiedby_pluginassembly"));
}
@NavigationProperty(name="lk_recurrencerule_modifiedby")
@JsonIgnore
public RecurrenceruleCollectionRequest getLk_recurrencerule_modifiedby() {
return new RecurrenceruleCollectionRequest(
contextPath.addSegment("lk_recurrencerule_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_recurrencerule_modifiedby"));
}
@NavigationProperty(name="knowledgearticle_primaryauthorid")
@JsonIgnore
public KnowledgearticleCollectionRequest getKnowledgearticle_primaryauthorid() {
return new KnowledgearticleCollectionRequest(
contextPath.addSegment("knowledgearticle_primaryauthorid"), RequestHelper.getValue(unmappedFields, "knowledgearticle_primaryauthorid"));
}
@NavigationProperty(name="lk_publisheraddressbase_createdonbehalfby")
@JsonIgnore
public PublisheraddressCollectionRequest getLk_publisheraddressbase_createdonbehalfby() {
return new PublisheraddressCollectionRequest(
contextPath.addSegment("lk_publisheraddressbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_publisheraddressbase_createdonbehalfby"));
}
@NavigationProperty(name="lk_transactioncurrencybase_modifiedby")
@JsonIgnore
public TransactioncurrencyCollectionRequest getLk_transactioncurrencybase_modifiedby() {
return new TransactioncurrencyCollectionRequest(
contextPath.addSegment("lk_transactioncurrencybase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_transactioncurrencybase_modifiedby"));
}
@NavigationProperty(name="contact_owning_user")
@JsonIgnore
public ContactCollectionRequest getContact_owning_user() {
return new ContactCollectionRequest(
contextPath.addSegment("contact_owning_user"), RequestHelper.getValue(unmappedFields, "contact_owning_user"));
}
@NavigationProperty(name="lk_appointment_createdonbehalfby")
@JsonIgnore
public AppointmentCollectionRequest getLk_appointment_createdonbehalfby() {
return new AppointmentCollectionRequest(
contextPath.addSegment("lk_appointment_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_appointment_createdonbehalfby"));
}
@NavigationProperty(name="lk_MobileOfflineProfileItemAssociation_createdby")
@JsonIgnore
public MobileofflineprofileitemassociationCollectionRequest getLk_MobileOfflineProfileItemAssociation_createdby() {
return new MobileofflineprofileitemassociationCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfileItemAssociation_createdby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfileItemAssociation_createdby"));
}
@NavigationProperty(name="lk_actioncardbase_createdby")
@JsonIgnore
public ActioncardCollectionRequest getLk_actioncardbase_createdby() {
return new ActioncardCollectionRequest(
contextPath.addSegment("lk_actioncardbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_actioncardbase_createdby"));
}
@NavigationProperty(name="lk_quarterlyfiscalcalendar_salespersonid")
@JsonIgnore
public QuarterlyfiscalcalendarCollectionRequest getLk_quarterlyfiscalcalendar_salespersonid() {
return new QuarterlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_quarterlyfiscalcalendar_salespersonid"), RequestHelper.getValue(unmappedFields, "lk_quarterlyfiscalcalendar_salespersonid"));
}
@NavigationProperty(name="lk_importlogbase_modifiedby")
@JsonIgnore
public ImportlogCollectionRequest getLk_importlogbase_modifiedby() {
return new ImportlogCollectionRequest(
contextPath.addSegment("lk_importlogbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importlogbase_modifiedby"));
}
@NavigationProperty(name="lk_recurrencerule_createdby")
@JsonIgnore
public RecurrenceruleCollectionRequest getLk_recurrencerule_createdby() {
return new RecurrenceruleCollectionRequest(
contextPath.addSegment("lk_recurrencerule_createdby"), RequestHelper.getValue(unmappedFields, "lk_recurrencerule_createdby"));
}
@NavigationProperty(name="lk_businessunitnewsarticlebase_modifiedby")
@JsonIgnore
public BusinessunitnewsarticleCollectionRequest getLk_businessunitnewsarticlebase_modifiedby() {
return new BusinessunitnewsarticleCollectionRequest(
contextPath.addSegment("lk_businessunitnewsarticlebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_businessunitnewsarticlebase_modifiedby"));
}
@NavigationProperty(name="lk_customcontrolresource_createdonbehalfby")
@JsonIgnore
public CustomcontrolresourceCollectionRequest getLk_customcontrolresource_createdonbehalfby() {
return new CustomcontrolresourceCollectionRequest(
contextPath.addSegment("lk_customcontrolresource_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontrolresource_createdonbehalfby"));
}
@NavigationProperty(name="lk_importmap_modifiedonbehalfby")
@JsonIgnore
public ImportmapCollectionRequest getLk_importmap_modifiedonbehalfby() {
return new ImportmapCollectionRequest(
contextPath.addSegment("lk_importmap_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importmap_modifiedonbehalfby"));
}
@NavigationProperty(name="modifiedby_sdkmessageprocessingstepsecureconfig")
@JsonIgnore
public SdkmessageprocessingstepsecureconfigCollectionRequest getModifiedby_sdkmessageprocessingstepsecureconfig() {
return new SdkmessageprocessingstepsecureconfigCollectionRequest(
contextPath.addSegment("modifiedby_sdkmessageprocessingstepsecureconfig"), RequestHelper.getValue(unmappedFields, "modifiedby_sdkmessageprocessingstepsecureconfig"));
}
@NavigationProperty(name="stageid_processstage")
@JsonIgnore
public ProcessstageRequest getStageid_processstage() {
return new ProcessstageRequest(contextPath.addSegment("stageid_processstage"), RequestHelper.getValue(unmappedFields, "stageid_processstage"));
}
@NavigationProperty(name="lk_category_createdby")
@JsonIgnore
public CategoryCollectionRequest getLk_category_createdby() {
return new CategoryCollectionRequest(
contextPath.addSegment("lk_category_createdby"), RequestHelper.getValue(unmappedFields, "lk_category_createdby"));
}
@NavigationProperty(name="systemuser_appmodule_modifiedby")
@JsonIgnore
public AppmoduleCollectionRequest getSystemuser_appmodule_modifiedby() {
return new AppmoduleCollectionRequest(
contextPath.addSegment("systemuser_appmodule_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_appmodule_modifiedby"));
}
@NavigationProperty(name="lk_processsession_startedby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_startedby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_startedby"), RequestHelper.getValue(unmappedFields, "lk_processsession_startedby"));
}
@NavigationProperty(name="systemuser_navigationsetting_createdby")
@JsonIgnore
public NavigationsettingCollectionRequest getSystemuser_navigationsetting_createdby() {
return new NavigationsettingCollectionRequest(
contextPath.addSegment("systemuser_navigationsetting_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_navigationsetting_createdby"));
}
@NavigationProperty(name="lk_newprocess_createdonbehalfby")
@JsonIgnore
public NewprocessCollectionRequest getLk_newprocess_createdonbehalfby() {
return new NewprocessCollectionRequest(
contextPath.addSegment("lk_newprocess_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_newprocess_createdonbehalfby"));
}
@NavigationProperty(name="lk_reportcategorybase_modifiedby")
@JsonIgnore
public ReportcategoryCollectionRequest getLk_reportcategorybase_modifiedby() {
return new ReportcategoryCollectionRequest(
contextPath.addSegment("lk_reportcategorybase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_reportcategorybase_modifiedby"));
}
@NavigationProperty(name="lk_task_createdonbehalfby")
@JsonIgnore
public TaskCollectionRequest getLk_task_createdonbehalfby() {
return new TaskCollectionRequest(
contextPath.addSegment("lk_task_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_task_createdonbehalfby"));
}
@NavigationProperty(name="user_letter")
@JsonIgnore
public LetterCollectionRequest getUser_letter() {
return new LetterCollectionRequest(
contextPath.addSegment("user_letter"), RequestHelper.getValue(unmappedFields, "user_letter"));
}
@NavigationProperty(name="lk_teambase_createdby")
@JsonIgnore
public TeamCollectionRequest getLk_teambase_createdby() {
return new TeamCollectionRequest(
contextPath.addSegment("lk_teambase_createdby"), RequestHelper.getValue(unmappedFields, "lk_teambase_createdby"));
}
@NavigationProperty(name="lk_slabase_modifiedonbehalfby")
@JsonIgnore
public SlaCollectionRequest getLk_slabase_modifiedonbehalfby() {
return new SlaCollectionRequest(
contextPath.addSegment("lk_slabase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slabase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_usersettings_createdonbehalfby")
@JsonIgnore
public UsersettingsCollectionRequest getLk_usersettings_createdonbehalfby() {
return new UsersettingsCollectionRequest(
contextPath.addSegment("lk_usersettings_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_usersettings_createdonbehalfby"));
}
@NavigationProperty(name="lk_calendarrule_createdby")
@JsonIgnore
public CalendarruleCollectionRequest getLk_calendarrule_createdby() {
return new CalendarruleCollectionRequest(
contextPath.addSegment("lk_calendarrule_createdby"), RequestHelper.getValue(unmappedFields, "lk_calendarrule_createdby"));
}
@NavigationProperty(name="lk_importentitymapping_createdonbehalfby")
@JsonIgnore
public ImportentitymappingCollectionRequest getLk_importentitymapping_createdonbehalfby() {
return new ImportentitymappingCollectionRequest(
contextPath.addSegment("lk_importentitymapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importentitymapping_createdonbehalfby"));
}
@NavigationProperty(name="lk_customcontrolresource_modifiedonbehalfby")
@JsonIgnore
public CustomcontrolresourceCollectionRequest getLk_customcontrolresource_modifiedonbehalfby() {
return new CustomcontrolresourceCollectionRequest(
contextPath.addSegment("lk_customcontrolresource_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontrolresource_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitem_modifiedby")
@JsonIgnore
public MobileofflineprofileitemCollectionRequest getLk_mobileofflineprofileitem_modifiedby() {
return new MobileofflineprofileitemCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitem_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitem_modifiedby"));
}
@NavigationProperty(name="parentsystemuserid")
@JsonIgnore
public SystemuserRequest getParentsystemuserid() {
return new SystemuserRequest(contextPath.addSegment("parentsystemuserid"), RequestHelper.getValue(unmappedFields, "parentsystemuserid"));
}
@NavigationProperty(name="user_parent_user")
@JsonIgnore
public SystemuserCollectionRequest getUser_parent_user() {
return new SystemuserCollectionRequest(
contextPath.addSegment("user_parent_user"), RequestHelper.getValue(unmappedFields, "user_parent_user"));
}
@NavigationProperty(name="lk_personaldocumenttemplatebase_modifiedby")
@JsonIgnore
public PersonaldocumenttemplateCollectionRequest getLk_personaldocumenttemplatebase_modifiedby() {
return new PersonaldocumenttemplateCollectionRequest(
contextPath.addSegment("lk_personaldocumenttemplatebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_personaldocumenttemplatebase_modifiedby"));
}
@NavigationProperty(name="ImportFile_SystemUser")
@JsonIgnore
public ImportfileCollectionRequest getImportFile_SystemUser() {
return new ImportfileCollectionRequest(
contextPath.addSegment("ImportFile_SystemUser"), RequestHelper.getValue(unmappedFields, "ImportFile_SystemUser"));
}
@NavigationProperty(name="lk_savedquerybase_createdby")
@JsonIgnore
public SavedqueryCollectionRequest getLk_savedquerybase_createdby() {
return new SavedqueryCollectionRequest(
contextPath.addSegment("lk_savedquerybase_createdby"), RequestHelper.getValue(unmappedFields, "lk_savedquerybase_createdby"));
}
@NavigationProperty(name="SystemUser_DuplicateMatchingRecord")
@JsonIgnore
public DuplicaterecordCollectionRequest getSystemUser_DuplicateMatchingRecord() {
return new DuplicaterecordCollectionRequest(
contextPath.addSegment("SystemUser_DuplicateMatchingRecord"), RequestHelper.getValue(unmappedFields, "SystemUser_DuplicateMatchingRecord"));
}
@NavigationProperty(name="lk_email_modifiedonbehalfby")
@JsonIgnore
public EmailCollectionRequest getLk_email_modifiedonbehalfby() {
return new EmailCollectionRequest(
contextPath.addSegment("lk_email_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_email_modifiedonbehalfby"));
}
@NavigationProperty(name="user_socialactivity")
@JsonIgnore
public SocialactivityCollectionRequest getUser_socialactivity() {
return new SocialactivityCollectionRequest(
contextPath.addSegment("user_socialactivity"), RequestHelper.getValue(unmappedFields, "user_socialactivity"));
}
@NavigationProperty(name="lk_fixedmonthlyfiscalcalendar_modifiedby")
@JsonIgnore
public FixedmonthlyfiscalcalendarCollectionRequest getLk_fixedmonthlyfiscalcalendar_modifiedby() {
return new FixedmonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_fixedmonthlyfiscalcalendar_modifiedby"));
}
@NavigationProperty(name="lk_businessunitnewsarticle_modifiedonbehalfby")
@JsonIgnore
public BusinessunitnewsarticleCollectionRequest getLk_businessunitnewsarticle_modifiedonbehalfby() {
return new BusinessunitnewsarticleCollectionRequest(
contextPath.addSegment("lk_businessunitnewsarticle_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_businessunitnewsarticle_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_phonecall_modifiedby")
@JsonIgnore
public PhonecallCollectionRequest getLk_phonecall_modifiedby() {
return new PhonecallCollectionRequest(
contextPath.addSegment("lk_phonecall_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_phonecall_modifiedby"));
}
@NavigationProperty(name="lk_annualfiscalcalendar_modifiedonbehalfby")
@JsonIgnore
public AnnualfiscalcalendarCollectionRequest getLk_annualfiscalcalendar_modifiedonbehalfby() {
return new AnnualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_annualfiscalcalendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_annualfiscalcalendar_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticletemplate_modifiedonbehalfby")
@JsonIgnore
public KbarticletemplateCollectionRequest getLk_kbarticletemplate_modifiedonbehalfby() {
return new KbarticletemplateCollectionRequest(
contextPath.addSegment("lk_kbarticletemplate_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticletemplate_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_organizationbase_modifiedby")
@JsonIgnore
public OrganizationCollectionRequest getLk_organizationbase_modifiedby() {
return new OrganizationCollectionRequest(
contextPath.addSegment("lk_organizationbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_organizationbase_modifiedby"));
}
@NavigationProperty(name="lk_ownermapping_createdonbehalfby")
@JsonIgnore
public OwnermappingCollectionRequest getLk_ownermapping_createdonbehalfby() {
return new OwnermappingCollectionRequest(
contextPath.addSegment("lk_ownermapping_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ownermapping_createdonbehalfby"));
}
@NavigationProperty(name="createdby_sdkmessageprocessingstep")
@JsonIgnore
public SdkmessageprocessingstepCollectionRequest getCreatedby_sdkmessageprocessingstep() {
return new SdkmessageprocessingstepCollectionRequest(
contextPath.addSegment("createdby_sdkmessageprocessingstep"), RequestHelper.getValue(unmappedFields, "createdby_sdkmessageprocessingstep"));
}
@NavigationProperty(name="lk_syncerrorbase_modifiedonbehalfby")
@JsonIgnore
public SyncerrorCollectionRequest getLk_syncerrorbase_modifiedonbehalfby() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("lk_syncerrorbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_syncerrorbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_appointment_createdby")
@JsonIgnore
public AppointmentCollectionRequest getLk_appointment_createdby() {
return new AppointmentCollectionRequest(
contextPath.addSegment("lk_appointment_createdby"), RequestHelper.getValue(unmappedFields, "lk_appointment_createdby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepsecureconfigCollectionRequest getLk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby() {
return new SdkmessageprocessingstepsecureconfigCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_importjobbase_modifiedby")
@JsonIgnore
public ImportjobCollectionRequest getLk_importjobbase_modifiedby() {
return new ImportjobCollectionRequest(
contextPath.addSegment("lk_importjobbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importjobbase_modifiedby"));
}
@NavigationProperty(name="mobileofflineprofileid")
@JsonIgnore
public MobileofflineprofileRequest getMobileofflineprofileid() {
return new MobileofflineprofileRequest(contextPath.addSegment("mobileofflineprofileid"), RequestHelper.getValue(unmappedFields, "mobileofflineprofileid"));
}
@NavigationProperty(name="lk_mailmergetemplatebase_modifiedby")
@JsonIgnore
public MailmergetemplateCollectionRequest getLk_mailmergetemplatebase_modifiedby() {
return new MailmergetemplateCollectionRequest(
contextPath.addSegment("lk_mailmergetemplatebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_mailmergetemplatebase_modifiedby"));
}
@NavigationProperty(name="lk_import_modifiedonbehalfby")
@JsonIgnore
public ImportCollectionRequest getLk_import_modifiedonbehalfby() {
return new ImportCollectionRequest(
contextPath.addSegment("lk_import_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_import_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_monthlyfiscalcalendar_createdonbehalfby")
@JsonIgnore
public MonthlyfiscalcalendarCollectionRequest getLk_monthlyfiscalcalendar_createdonbehalfby() {
return new MonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_monthlyfiscalcalendar_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_monthlyfiscalcalendar_createdonbehalfby"));
}
@NavigationProperty(name="queueid")
@JsonIgnore
public QueueRequest getQueueid() {
return new QueueRequest(contextPath.addSegment("queueid"), RequestHelper.getValue(unmappedFields, "queueid"));
}
@NavigationProperty(name="lk_userquery_modifiedonbehalfby")
@JsonIgnore
public UserqueryCollectionRequest getLk_userquery_modifiedonbehalfby() {
return new UserqueryCollectionRequest(
contextPath.addSegment("lk_userquery_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_userquery_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_timezonerule_modifiedonbehalfby")
@JsonIgnore
public TimezoneruleCollectionRequest getLk_timezonerule_modifiedonbehalfby() {
return new TimezoneruleCollectionRequest(
contextPath.addSegment("lk_timezonerule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_timezonerule_modifiedonbehalfby"));
}
@NavigationProperty(name="user_userqueryvisualizations")
@JsonIgnore
public UserqueryvisualizationCollectionRequest getUser_userqueryvisualizations() {
return new UserqueryvisualizationCollectionRequest(
contextPath.addSegment("user_userqueryvisualizations"), RequestHelper.getValue(unmappedFields, "user_userqueryvisualizations"));
}
@NavigationProperty(name="lk_userform_createdby")
@JsonIgnore
public UserformCollectionRequest getLk_userform_createdby() {
return new UserformCollectionRequest(
contextPath.addSegment("lk_userform_createdby"), RequestHelper.getValue(unmappedFields, "lk_userform_createdby"));
}
@NavigationProperty(name="user_task")
@JsonIgnore
public TaskCollectionRequest getUser_task() {
return new TaskCollectionRequest(
contextPath.addSegment("user_task"), RequestHelper.getValue(unmappedFields, "user_task"));
}
@NavigationProperty(name="lk_MobileOfflineProfile_createdonbehalfby")
@JsonIgnore
public MobileofflineprofileCollectionRequest getLk_MobileOfflineProfile_createdonbehalfby() {
return new MobileofflineprofileCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfile_createdonbehalfby"));
}
@NavigationProperty(name="lk_webwizard_createdby")
@JsonIgnore
public WebwizardCollectionRequest getLk_webwizard_createdby() {
return new WebwizardCollectionRequest(
contextPath.addSegment("lk_webwizard_createdby"), RequestHelper.getValue(unmappedFields, "lk_webwizard_createdby"));
}
@NavigationProperty(name="lk_mailmergetemplatebase_createdby")
@JsonIgnore
public MailmergetemplateCollectionRequest getLk_mailmergetemplatebase_createdby() {
return new MailmergetemplateCollectionRequest(
contextPath.addSegment("lk_mailmergetemplatebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_mailmergetemplatebase_createdby"));
}
@NavigationProperty(name="lk_calendarrule_createdonbehalfby")
@JsonIgnore
public CalendarruleCollectionRequest getLk_calendarrule_createdonbehalfby() {
return new CalendarruleCollectionRequest(
contextPath.addSegment("lk_calendarrule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_calendarrule_createdonbehalfby"));
}
@NavigationProperty(name="lk_email_createdby")
@JsonIgnore
public EmailCollectionRequest getLk_email_createdby() {
return new EmailCollectionRequest(
contextPath.addSegment("lk_email_createdby"), RequestHelper.getValue(unmappedFields, "lk_email_createdby"));
}
@NavigationProperty(name="lk_queue_createdonbehalfby")
@JsonIgnore
public QueueCollectionRequest getLk_queue_createdonbehalfby() {
return new QueueCollectionRequest(
contextPath.addSegment("lk_queue_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_queue_createdonbehalfby"));
}
@NavigationProperty(name="lk_userquery_createdby")
@JsonIgnore
public UserqueryCollectionRequest getLk_userquery_createdby() {
return new UserqueryCollectionRequest(
contextPath.addSegment("lk_userquery_createdby"), RequestHelper.getValue(unmappedFields, "lk_userquery_createdby"));
}
@NavigationProperty(name="systemuser_appconfigmaster_modifiedonbehalfby")
@JsonIgnore
public AppconfigmasterCollectionRequest getSystemuser_appconfigmaster_modifiedonbehalfby() {
return new AppconfigmasterCollectionRequest(
contextPath.addSegment("systemuser_appconfigmaster_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfigmaster_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_queuebase_modifiedby")
@JsonIgnore
public QueueCollectionRequest getLk_queuebase_modifiedby() {
return new QueueCollectionRequest(
contextPath.addSegment("lk_queuebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_queuebase_modifiedby"));
}
@NavigationProperty(name="lk_audit_userid")
@JsonIgnore
public AuditCollectionRequest getLk_audit_userid() {
return new AuditCollectionRequest(
contextPath.addSegment("lk_audit_userid"), RequestHelper.getValue(unmappedFields, "lk_audit_userid"));
}
@NavigationProperty(name="modifiedby_sdkmessage")
@JsonIgnore
public SdkmessageCollectionRequest getModifiedby_sdkmessage() {
return new SdkmessageCollectionRequest(
contextPath.addSegment("modifiedby_sdkmessage"), RequestHelper.getValue(unmappedFields, "modifiedby_sdkmessage"));
}
@NavigationProperty(name="lk_transformationparametermapping_createdby")
@JsonIgnore
public TransformationparametermappingCollectionRequest getLk_transformationparametermapping_createdby() {
return new TransformationparametermappingCollectionRequest(
contextPath.addSegment("lk_transformationparametermapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_transformationparametermapping_createdby"));
}
@NavigationProperty(name="lk_fieldsecurityprofile_modifiedby")
@JsonIgnore
public FieldsecurityprofileCollectionRequest getLk_fieldsecurityprofile_modifiedby() {
return new FieldsecurityprofileCollectionRequest(
contextPath.addSegment("lk_fieldsecurityprofile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_fieldsecurityprofile_modifiedby"));
}
@NavigationProperty(name="lk_customcontroldefaultconfig_modifiedonbehalfby")
@JsonIgnore
public CustomcontroldefaultconfigCollectionRequest getLk_customcontroldefaultconfig_modifiedonbehalfby() {
return new CustomcontroldefaultconfigCollectionRequest(
contextPath.addSegment("lk_customcontroldefaultconfig_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_customcontroldefaultconfig_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_picklistmapping_createdby")
@JsonIgnore
public PicklistmappingCollectionRequest getLk_picklistmapping_createdby() {
return new PicklistmappingCollectionRequest(
contextPath.addSegment("lk_picklistmapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_picklistmapping_createdby"));
}
@NavigationProperty(name="lk_asyncoperation_modifiedonbehalfby")
@JsonIgnore
public AsyncoperationCollectionRequest getLk_asyncoperation_modifiedonbehalfby() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("lk_asyncoperation_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_asyncoperation_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_publisherbase_createdonbehalfby")
@JsonIgnore
public PublisherCollectionRequest getLk_publisherbase_createdonbehalfby() {
return new PublisherCollectionRequest(
contextPath.addSegment("lk_publisherbase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_publisherbase_createdonbehalfby"));
}
@NavigationProperty(name="SystemUser_Email_EmailSender")
@JsonIgnore
public EmailCollectionRequest getSystemUser_Email_EmailSender() {
return new EmailCollectionRequest(
contextPath.addSegment("SystemUser_Email_EmailSender"), RequestHelper.getValue(unmappedFields, "SystemUser_Email_EmailSender"));
}
@NavigationProperty(name="lk_recurringappointmentmaster_modifiedby")
@JsonIgnore
public RecurringappointmentmasterCollectionRequest getLk_recurringappointmentmaster_modifiedby() {
return new RecurringappointmentmasterCollectionRequest(
contextPath.addSegment("lk_recurringappointmentmaster_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_recurringappointmentmaster_modifiedby"));
}
@NavigationProperty(name="SystemUser_ImportMaps")
@JsonIgnore
public ImportmapCollectionRequest getSystemUser_ImportMaps() {
return new ImportmapCollectionRequest(
contextPath.addSegment("SystemUser_ImportMaps"), RequestHelper.getValue(unmappedFields, "SystemUser_ImportMaps"));
}
@NavigationProperty(name="workflow_modifiedonbehalfby")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_modifiedonbehalfby() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "workflow_modifiedonbehalfby"));
}
@NavigationProperty(name="systemuser_principalobjectattributeaccess")
@JsonIgnore
public PrincipalobjectattributeaccessCollectionRequest getSystemuser_principalobjectattributeaccess() {
return new PrincipalobjectattributeaccessCollectionRequest(
contextPath.addSegment("systemuser_principalobjectattributeaccess"), RequestHelper.getValue(unmappedFields, "systemuser_principalobjectattributeaccess"));
}
@NavigationProperty(name="lk_slaitembase_modifiedby")
@JsonIgnore
public SlaitemCollectionRequest getLk_slaitembase_modifiedby() {
return new SlaitemCollectionRequest(
contextPath.addSegment("lk_slaitembase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_slaitembase_modifiedby"));
}
@NavigationProperty(name="lk_mobileofflineprofileitemassociation_createdonbehalfby")
@JsonIgnore
public MobileofflineprofileitemassociationCollectionRequest getLk_mobileofflineprofileitemassociation_createdonbehalfby() {
return new MobileofflineprofileitemassociationCollectionRequest(
contextPath.addSegment("lk_mobileofflineprofileitemassociation_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_mobileofflineprofileitemassociation_createdonbehalfby"));
}
@NavigationProperty(name="lk_annualfiscalcalendar_salespersonid")
@JsonIgnore
public AnnualfiscalcalendarCollectionRequest getLk_annualfiscalcalendar_salespersonid() {
return new AnnualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_annualfiscalcalendar_salespersonid"), RequestHelper.getValue(unmappedFields, "lk_annualfiscalcalendar_salespersonid"));
}
@NavigationProperty(name="lk_emailserverprofile_modifiedby")
@JsonIgnore
public EmailserverprofileCollectionRequest getLk_emailserverprofile_modifiedby() {
return new EmailserverprofileCollectionRequest(
contextPath.addSegment("lk_emailserverprofile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_emailserverprofile_modifiedby"));
}
@NavigationProperty(name="lk_slakpiinstancebase_modifiedonbehalfby")
@JsonIgnore
public SlakpiinstanceCollectionRequest getLk_slakpiinstancebase_modifiedonbehalfby() {
return new SlakpiinstanceCollectionRequest(
contextPath.addSegment("lk_slakpiinstancebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_slakpiinstancebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_duplicateruleconditionbase_modifiedby")
@JsonIgnore
public DuplicateruleconditionCollectionRequest getLk_duplicateruleconditionbase_modifiedby() {
return new DuplicateruleconditionCollectionRequest(
contextPath.addSegment("lk_duplicateruleconditionbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_duplicateruleconditionbase_modifiedby"));
}
@NavigationProperty(name="system_user_email_templates")
@JsonIgnore
public TemplateCollectionRequest getSystem_user_email_templates() {
return new TemplateCollectionRequest(
contextPath.addSegment("system_user_email_templates"), RequestHelper.getValue(unmappedFields, "system_user_email_templates"));
}
@NavigationProperty(name="lk_letter_modifiedby")
@JsonIgnore
public LetterCollectionRequest getLk_letter_modifiedby() {
return new LetterCollectionRequest(
contextPath.addSegment("lk_letter_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_letter_modifiedby"));
}
@NavigationProperty(name="workflow_createdby")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_createdby() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_createdby"), RequestHelper.getValue(unmappedFields, "workflow_createdby"));
}
@NavigationProperty(name="lk_publisheraddressbase_modifiedby")
@JsonIgnore
public PublisheraddressCollectionRequest getLk_publisheraddressbase_modifiedby() {
return new PublisheraddressCollectionRequest(
contextPath.addSegment("lk_publisheraddressbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_publisheraddressbase_modifiedby"));
}
@NavigationProperty(name="lk_picklistmapping_modifiedonbehalfby")
@JsonIgnore
public PicklistmappingCollectionRequest getLk_picklistmapping_modifiedonbehalfby() {
return new PicklistmappingCollectionRequest(
contextPath.addSegment("lk_picklistmapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_picklistmapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_ownermapping_modifiedby")
@JsonIgnore
public OwnermappingCollectionRequest getLk_ownermapping_modifiedby() {
return new OwnermappingCollectionRequest(
contextPath.addSegment("lk_ownermapping_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ownermapping_modifiedby"));
}
@NavigationProperty(name="lk_task_modifiedonbehalfby")
@JsonIgnore
public TaskCollectionRequest getLk_task_modifiedonbehalfby() {
return new TaskCollectionRequest(
contextPath.addSegment("lk_task_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_task_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_userform_modifiedby")
@JsonIgnore
public UserformCollectionRequest getLk_userform_modifiedby() {
return new UserformCollectionRequest(
contextPath.addSegment("lk_userform_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_userform_modifiedby"));
}
@NavigationProperty(name="lk_DisplayStringbase_modifiedby")
@JsonIgnore
public DisplaystringCollectionRequest getLk_DisplayStringbase_modifiedby() {
return new DisplaystringCollectionRequest(
contextPath.addSegment("lk_DisplayStringbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_DisplayStringbase_modifiedby"));
}
@NavigationProperty(name="lk_importdatabase_createdby")
@JsonIgnore
public ImportdataCollectionRequest getLk_importdatabase_createdby() {
return new ImportdataCollectionRequest(
contextPath.addSegment("lk_importdatabase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importdatabase_createdby"));
}
@NavigationProperty(name="user_slabase")
@JsonIgnore
public SlaCollectionRequest getUser_slabase() {
return new SlaCollectionRequest(
contextPath.addSegment("user_slabase"), RequestHelper.getValue(unmappedFields, "user_slabase"));
}
@NavigationProperty(name="lk_importfilebase_modifiedby")
@JsonIgnore
public ImportfileCollectionRequest getLk_importfilebase_modifiedby() {
return new ImportfileCollectionRequest(
contextPath.addSegment("lk_importfilebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importfilebase_modifiedby"));
}
@NavigationProperty(name="lk_team_createdonbehalfby")
@JsonIgnore
public TeamCollectionRequest getLk_team_createdonbehalfby() {
return new TeamCollectionRequest(
contextPath.addSegment("lk_team_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_team_createdonbehalfby"));
}
@NavigationProperty(name="lk_templatebase_modifiedonbehalfby")
@JsonIgnore
public TemplateCollectionRequest getLk_templatebase_modifiedonbehalfby() {
return new TemplateCollectionRequest(
contextPath.addSegment("lk_templatebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_templatebase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_slaitembase_createdby")
@JsonIgnore
public SlaitemCollectionRequest getLk_slaitembase_createdby() {
return new SlaitemCollectionRequest(
contextPath.addSegment("lk_slaitembase_createdby"), RequestHelper.getValue(unmappedFields, "lk_slaitembase_createdby"));
}
@NavigationProperty(name="businessunitid")
@JsonIgnore
public BusinessunitRequest getBusinessunitid() {
return new BusinessunitRequest(contextPath.addSegment("businessunitid"), RequestHelper.getValue(unmappedFields, "businessunitid"));
}
@NavigationProperty(name="lk_importmapbase_modifiedby")
@JsonIgnore
public ImportmapCollectionRequest getLk_importmapbase_modifiedby() {
return new ImportmapCollectionRequest(
contextPath.addSegment("lk_importmapbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importmapbase_modifiedby"));
}
@NavigationProperty(name="lk_newprocess_modifiedonbehalfby")
@JsonIgnore
public NewprocessCollectionRequest getLk_newprocess_modifiedonbehalfby() {
return new NewprocessCollectionRequest(
contextPath.addSegment("lk_newprocess_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_newprocess_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_usersettingsbase_createdby")
@JsonIgnore
public UsersettingsCollectionRequest getLk_usersettingsbase_createdby() {
return new UsersettingsCollectionRequest(
contextPath.addSegment("lk_usersettingsbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_usersettingsbase_createdby"));
}
@NavigationProperty(name="lk_webresourcebase_modifiedonbehalfby")
@JsonIgnore
public WebresourceCollectionRequest getLk_webresourcebase_modifiedonbehalfby() {
return new WebresourceCollectionRequest(
contextPath.addSegment("lk_webresourcebase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_webresourcebase_modifiedonbehalfby"));
}
@NavigationProperty(name="webresource_createdby")
@JsonIgnore
public WebresourceCollectionRequest getWebresource_createdby() {
return new WebresourceCollectionRequest(
contextPath.addSegment("webresource_createdby"), RequestHelper.getValue(unmappedFields, "webresource_createdby"));
}
@NavigationProperty(name="lk_processtriggerbase_createdby")
@JsonIgnore
public ProcesstriggerCollectionRequest getLk_processtriggerbase_createdby() {
return new ProcesstriggerCollectionRequest(
contextPath.addSegment("lk_processtriggerbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_processtriggerbase_createdby"));
}
@NavigationProperty(name="lk_expiredprocess_modifiedonbehalfby")
@JsonIgnore
public ExpiredprocessCollectionRequest getLk_expiredprocess_modifiedonbehalfby() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("lk_expiredprocess_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_expiredprocess_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_ownermapping_createdby")
@JsonIgnore
public OwnermappingCollectionRequest getLk_ownermapping_createdby() {
return new OwnermappingCollectionRequest(
contextPath.addSegment("lk_ownermapping_createdby"), RequestHelper.getValue(unmappedFields, "lk_ownermapping_createdby"));
}
@NavigationProperty(name="user_accounts")
@JsonIgnore
public AccountCollectionRequest getUser_accounts() {
return new AccountCollectionRequest(
contextPath.addSegment("user_accounts"), RequestHelper.getValue(unmappedFields, "user_accounts"));
}
@NavigationProperty(name="lk_kbarticlebase_modifiedby")
@JsonIgnore
public KbarticleCollectionRequest getLk_kbarticlebase_modifiedby() {
return new KbarticleCollectionRequest(
contextPath.addSegment("lk_kbarticlebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlebase_modifiedby"));
}
@NavigationProperty(name="lk_workflowlog_createdby")
@JsonIgnore
public WorkflowlogCollectionRequest getLk_workflowlog_createdby() {
return new WorkflowlogCollectionRequest(
contextPath.addSegment("lk_workflowlog_createdby"), RequestHelper.getValue(unmappedFields, "lk_workflowlog_createdby"));
}
@NavigationProperty(name="lk_bulkdeleteoperation_modifiedonbehalfby")
@JsonIgnore
public BulkdeleteoperationCollectionRequest getLk_bulkdeleteoperation_modifiedonbehalfby() {
return new BulkdeleteoperationCollectionRequest(
contextPath.addSegment("lk_bulkdeleteoperation_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_bulkdeleteoperation_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_userqueryvisualization_createdby")
@JsonIgnore
public UserqueryvisualizationCollectionRequest getLk_userqueryvisualization_createdby() {
return new UserqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_userqueryvisualization_createdby"), RequestHelper.getValue(unmappedFields, "lk_userqueryvisualization_createdby"));
}
@NavigationProperty(name="lk_importfilebase_createdby")
@JsonIgnore
public ImportfileCollectionRequest getLk_importfilebase_createdby() {
return new ImportfileCollectionRequest(
contextPath.addSegment("lk_importfilebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importfilebase_createdby"));
}
@NavigationProperty(name="lk_contactbase_modifiedby")
@JsonIgnore
public ContactCollectionRequest getLk_contactbase_modifiedby() {
return new ContactCollectionRequest(
contextPath.addSegment("lk_contactbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_contactbase_modifiedby"));
}
@NavigationProperty(name="lk_MobileOfflineProfileItem_createdby")
@JsonIgnore
public MobileofflineprofileitemCollectionRequest getLk_MobileOfflineProfileItem_createdby() {
return new MobileofflineprofileitemCollectionRequest(
contextPath.addSegment("lk_MobileOfflineProfileItem_createdby"), RequestHelper.getValue(unmappedFields, "lk_MobileOfflineProfileItem_createdby"));
}
@NavigationProperty(name="workflow_modifiedby")
@JsonIgnore
public WorkflowCollectionRequest getWorkflow_modifiedby() {
return new WorkflowCollectionRequest(
contextPath.addSegment("workflow_modifiedby"), RequestHelper.getValue(unmappedFields, "workflow_modifiedby"));
}
@NavigationProperty(name="lk_sdkmessagefilter_modifiedonbehalfby")
@JsonIgnore
public SdkmessagefilterCollectionRequest getLk_sdkmessagefilter_modifiedonbehalfby() {
return new SdkmessagefilterCollectionRequest(
contextPath.addSegment("lk_sdkmessagefilter_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessagefilter_modifiedonbehalfby"));
}
@NavigationProperty(name="SystemUser_DuplicateRules")
@JsonIgnore
public DuplicateruleCollectionRequest getSystemUser_DuplicateRules() {
return new DuplicateruleCollectionRequest(
contextPath.addSegment("SystemUser_DuplicateRules"), RequestHelper.getValue(unmappedFields, "SystemUser_DuplicateRules"));
}
@NavigationProperty(name="lk_fixedmonthlyfiscalcalendar_salespersonid")
@JsonIgnore
public FixedmonthlyfiscalcalendarCollectionRequest getLk_fixedmonthlyfiscalcalendar_salespersonid() {
return new FixedmonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_salespersonid"), RequestHelper.getValue(unmappedFields, "lk_fixedmonthlyfiscalcalendar_salespersonid"));
}
@NavigationProperty(name="lk_actioncardbase_modifiedby")
@JsonIgnore
public ActioncardCollectionRequest getLk_actioncardbase_modifiedby() {
return new ActioncardCollectionRequest(
contextPath.addSegment("lk_actioncardbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_actioncardbase_modifiedby"));
}
@NavigationProperty(name="lk_ACIViewMapper_modifiedby")
@JsonIgnore
public AciviewmapperCollectionRequest getLk_ACIViewMapper_modifiedby() {
return new AciviewmapperCollectionRequest(
contextPath.addSegment("lk_ACIViewMapper_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ACIViewMapper_modifiedby"));
}
@NavigationProperty(name="lk_kbarticletemplatebase_modifiedby")
@JsonIgnore
public KbarticletemplateCollectionRequest getLk_kbarticletemplatebase_modifiedby() {
return new KbarticletemplateCollectionRequest(
contextPath.addSegment("lk_kbarticletemplatebase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_kbarticletemplatebase_modifiedby"));
}
@NavigationProperty(name="systemuser_appconfigmaster_modifiedby")
@JsonIgnore
public AppconfigmasterCollectionRequest getSystemuser_appconfigmaster_modifiedby() {
return new AppconfigmasterCollectionRequest(
contextPath.addSegment("systemuser_appconfigmaster_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfigmaster_modifiedby"));
}
@NavigationProperty(name="lk_savedqueryvisualizationbase_modifiedby")
@JsonIgnore
public SavedqueryvisualizationCollectionRequest getLk_savedqueryvisualizationbase_modifiedby() {
return new SavedqueryvisualizationCollectionRequest(
contextPath.addSegment("lk_savedqueryvisualizationbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_savedqueryvisualizationbase_modifiedby"));
}
@NavigationProperty(name="lk_semiannualfiscalcalendar_modifiedby")
@JsonIgnore
public SemiannualfiscalcalendarCollectionRequest getLk_semiannualfiscalcalendar_modifiedby() {
return new SemiannualfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_semiannualfiscalcalendar_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_semiannualfiscalcalendar_modifiedby"));
}
@NavigationProperty(name="modifiedby_plugintypestatistic")
@JsonIgnore
public PlugintypestatisticCollectionRequest getModifiedby_plugintypestatistic() {
return new PlugintypestatisticCollectionRequest(
contextPath.addSegment("modifiedby_plugintypestatistic"), RequestHelper.getValue(unmappedFields, "modifiedby_plugintypestatistic"));
}
@NavigationProperty(name="lk_letter_createdby")
@JsonIgnore
public LetterCollectionRequest getLk_letter_createdby() {
return new LetterCollectionRequest(
contextPath.addSegment("lk_letter_createdby"), RequestHelper.getValue(unmappedFields, "lk_letter_createdby"));
}
@NavigationProperty(name="systemuser_appmodule_createdonbehalfby")
@JsonIgnore
public AppmoduleCollectionRequest getSystemuser_appmodule_createdonbehalfby() {
return new AppmoduleCollectionRequest(
contextPath.addSegment("systemuser_appmodule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_appmodule_createdonbehalfby"));
}
@NavigationProperty(name="lk_businessunitnewsarticle_createdonbehalfby")
@JsonIgnore
public BusinessunitnewsarticleCollectionRequest getLk_businessunitnewsarticle_createdonbehalfby() {
return new BusinessunitnewsarticleCollectionRequest(
contextPath.addSegment("lk_businessunitnewsarticle_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_businessunitnewsarticle_createdonbehalfby"));
}
@NavigationProperty(name="lk_timezonerule_modifiedby")
@JsonIgnore
public TimezoneruleCollectionRequest getLk_timezonerule_modifiedby() {
return new TimezoneruleCollectionRequest(
contextPath.addSegment("lk_timezonerule_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_timezonerule_modifiedby"));
}
@NavigationProperty(name="lk_serviceendpointbase_modifiedonbehalfby")
@JsonIgnore
public ServiceendpointCollectionRequest getLk_serviceendpointbase_modifiedonbehalfby() {
return new ServiceendpointCollectionRequest(
contextPath.addSegment("lk_serviceendpointbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_serviceendpointbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticle_modifiedonbehalfby")
@JsonIgnore
public KbarticleCollectionRequest getLk_kbarticle_modifiedonbehalfby() {
return new KbarticleCollectionRequest(
contextPath.addSegment("lk_kbarticle_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_kbarticle_modifiedonbehalfby"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="lk_systemuser_createdonbehalfby")
@JsonIgnore
public SystemuserCollectionRequest getLk_systemuser_createdonbehalfby() {
return new SystemuserCollectionRequest(
contextPath.addSegment("lk_systemuser_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_systemuser_createdonbehalfby"));
}
@NavigationProperty(name="lk_SocialProfile_createdonbehalfby")
@JsonIgnore
public SocialprofileCollectionRequest getLk_SocialProfile_createdonbehalfby() {
return new SocialprofileCollectionRequest(
contextPath.addSegment("lk_SocialProfile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_SocialProfile_createdonbehalfby"));
}
@NavigationProperty(name="OwnerMapping_SystemUser")
@JsonIgnore
public OwnermappingCollectionRequest getOwnerMapping_SystemUser() {
return new OwnermappingCollectionRequest(
contextPath.addSegment("OwnerMapping_SystemUser"), RequestHelper.getValue(unmappedFields, "OwnerMapping_SystemUser"));
}
@NavigationProperty(name="system_user_workflow")
@JsonIgnore
public WorkflowCollectionRequest getSystem_user_workflow() {
return new WorkflowCollectionRequest(
contextPath.addSegment("system_user_workflow"), RequestHelper.getValue(unmappedFields, "system_user_workflow"));
}
@NavigationProperty(name="lk_importbase_modifiedby")
@JsonIgnore
public ImportCollectionRequest getLk_importbase_modifiedby() {
return new ImportCollectionRequest(
contextPath.addSegment("lk_importbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_importbase_modifiedby"));
}
@NavigationProperty(name="lk_teambase_administratorid")
@JsonIgnore
public TeamCollectionRequest getLk_teambase_administratorid() {
return new TeamCollectionRequest(
contextPath.addSegment("lk_teambase_administratorid"), RequestHelper.getValue(unmappedFields, "lk_teambase_administratorid"));
}
@NavigationProperty(name="lk_fieldsecurityprofile_createdby")
@JsonIgnore
public FieldsecurityprofileCollectionRequest getLk_fieldsecurityprofile_createdby() {
return new FieldsecurityprofileCollectionRequest(
contextPath.addSegment("lk_fieldsecurityprofile_createdby"), RequestHelper.getValue(unmappedFields, "lk_fieldsecurityprofile_createdby"));
}
@NavigationProperty(name="lk_fixedmonthlyfiscalcalendar_modifiedonbehalfby")
@JsonIgnore
public FixedmonthlyfiscalcalendarCollectionRequest getLk_fixedmonthlyfiscalcalendar_modifiedonbehalfby() {
return new FixedmonthlyfiscalcalendarCollectionRequest(
contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_fixedmonthlyfiscalcalendar_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_mailboxtrackingfolder_modifiedby")
@JsonIgnore
public MailboxtrackingfolderCollectionRequest getLk_mailboxtrackingfolder_modifiedby() {
return new MailboxtrackingfolderCollectionRequest(
contextPath.addSegment("lk_mailboxtrackingfolder_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_mailboxtrackingfolder_modifiedby"));
}
@NavigationProperty(name="lk_sdkmessageprocessingstepsecureconfig_createdonbehalfby")
@JsonIgnore
public SdkmessageprocessingstepsecureconfigCollectionRequest getLk_sdkmessageprocessingstepsecureconfig_createdonbehalfby() {
return new SdkmessageprocessingstepsecureconfigCollectionRequest(
contextPath.addSegment("lk_sdkmessageprocessingstepsecureconfig_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessageprocessingstepsecureconfig_createdonbehalfby"));
}
@NavigationProperty(name="lk_columnmapping_modifiedonbehalfby")
@JsonIgnore
public ColumnmappingCollectionRequest getLk_columnmapping_modifiedonbehalfby() {
return new ColumnmappingCollectionRequest(
contextPath.addSegment("lk_columnmapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_columnmapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_importmapbase_createdby")
@JsonIgnore
public ImportmapCollectionRequest getLk_importmapbase_createdby() {
return new ImportmapCollectionRequest(
contextPath.addSegment("lk_importmapbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_importmapbase_createdby"));
}
@NavigationProperty(name="lk_transactioncurrency_modifiedonbehalfby")
@JsonIgnore
public TransactioncurrencyCollectionRequest getLk_transactioncurrency_modifiedonbehalfby() {
return new TransactioncurrencyCollectionRequest(
contextPath.addSegment("lk_transactioncurrency_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_transactioncurrency_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_expiredprocess_modifiedby")
@JsonIgnore
public ExpiredprocessCollectionRequest getLk_expiredprocess_modifiedby() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("lk_expiredprocess_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_expiredprocess_modifiedby"));
}
@NavigationProperty(name="user_userquery")
@JsonIgnore
public UserqueryCollectionRequest getUser_userquery() {
return new UserqueryCollectionRequest(
contextPath.addSegment("user_userquery"), RequestHelper.getValue(unmappedFields, "user_userquery"));
}
@NavigationProperty(name="system_user_asyncoperation")
@JsonIgnore
public AsyncoperationCollectionRequest getSystem_user_asyncoperation() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("system_user_asyncoperation"), RequestHelper.getValue(unmappedFields, "system_user_asyncoperation"));
}
@NavigationProperty(name="lk_plugintypestatisticbase_modifiedonbehalfby")
@JsonIgnore
public PlugintypestatisticCollectionRequest getLk_plugintypestatisticbase_modifiedonbehalfby() {
return new PlugintypestatisticCollectionRequest(
contextPath.addSegment("lk_plugintypestatisticbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_plugintypestatisticbase_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_duplicateruleconditionbase_createdby")
@JsonIgnore
public DuplicateruleconditionCollectionRequest getLk_duplicateruleconditionbase_createdby() {
return new DuplicateruleconditionCollectionRequest(
contextPath.addSegment("lk_duplicateruleconditionbase_createdby"), RequestHelper.getValue(unmappedFields, "lk_duplicateruleconditionbase_createdby"));
}
@NavigationProperty(name="lk_processsession_completedby")
@JsonIgnore
public ProcesssessionCollectionRequest getLk_processsession_completedby() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("lk_processsession_completedby"), RequestHelper.getValue(unmappedFields, "lk_processsession_completedby"));
}
@NavigationProperty(name="lk_importentitymapping_modifiedonbehalfby")
@JsonIgnore
public ImportentitymappingCollectionRequest getLk_importentitymapping_modifiedonbehalfby() {
return new ImportentitymappingCollectionRequest(
contextPath.addSegment("lk_importentitymapping_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importentitymapping_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_accountbase_modifiedonbehalfby")
@JsonIgnore
public AccountCollectionRequest getLk_accountbase_modifiedonbehalfby() {
return new AccountCollectionRequest(
contextPath.addSegment("lk_accountbase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_accountbase_modifiedonbehalfby"));
}
@NavigationProperty(name="systemuser_appconfiginstance_createdby")
@JsonIgnore
public AppconfiginstanceCollectionRequest getSystemuser_appconfiginstance_createdby() {
return new AppconfiginstanceCollectionRequest(
contextPath.addSegment("systemuser_appconfiginstance_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_appconfiginstance_createdby"));
}
@NavigationProperty(name="lk_customcontrol_modifiedby")
@JsonIgnore
public CustomcontrolCollectionRequest getLk_customcontrol_modifiedby() {
return new CustomcontrolCollectionRequest(
contextPath.addSegment("lk_customcontrol_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_customcontrol_modifiedby"));
}
@NavigationProperty(name="lk_duplicaterulebase_createdby")
@JsonIgnore
public DuplicateruleCollectionRequest getLk_duplicaterulebase_createdby() {
return new DuplicateruleCollectionRequest(
contextPath.addSegment("lk_duplicaterulebase_createdby"), RequestHelper.getValue(unmappedFields, "lk_duplicaterulebase_createdby"));
}
@NavigationProperty(name="lk_queueitem_createdonbehalfby")
@JsonIgnore
public QueueitemCollectionRequest getLk_queueitem_createdonbehalfby() {
return new QueueitemCollectionRequest(
contextPath.addSegment("lk_queueitem_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_queueitem_createdonbehalfby"));
}
@NavigationProperty(name="lk_transactioncurrencybase_createdby")
@JsonIgnore
public TransactioncurrencyCollectionRequest getLk_transactioncurrencybase_createdby() {
return new TransactioncurrencyCollectionRequest(
contextPath.addSegment("lk_transactioncurrencybase_createdby"), RequestHelper.getValue(unmappedFields, "lk_transactioncurrencybase_createdby"));
}
@NavigationProperty(name="lk_importlog_modifiedonbehalfby")
@JsonIgnore
public ImportlogCollectionRequest getLk_importlog_modifiedonbehalfby() {
return new ImportlogCollectionRequest(
contextPath.addSegment("lk_importlog_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_importlog_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_sdkmessage_modifiedonbehalfby")
@JsonIgnore
public SdkmessageCollectionRequest getLk_sdkmessage_modifiedonbehalfby() {
return new SdkmessageCollectionRequest(
contextPath.addSegment("lk_sdkmessage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_sdkmessage_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_kbarticlecommentbase_modifiedby")
@JsonIgnore
public KbarticlecommentCollectionRequest getLk_kbarticlecommentbase_modifiedby() {
return new KbarticlecommentCollectionRequest(
contextPath.addSegment("lk_kbarticlecommentbase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_kbarticlecommentbase_modifiedby"));
}
@NavigationProperty(name="user_phonecall")
@JsonIgnore
public PhonecallCollectionRequest getUser_phonecall() {
return new PhonecallCollectionRequest(
contextPath.addSegment("user_phonecall"), RequestHelper.getValue(unmappedFields, "user_phonecall"));
}
@NavigationProperty(name="user_appointment")
@JsonIgnore
public AppointmentCollectionRequest getUser_appointment() {
return new AppointmentCollectionRequest(
contextPath.addSegment("user_appointment"), RequestHelper.getValue(unmappedFields, "user_appointment"));
}
@NavigationProperty(name="lk_expiredprocess_createdonbehalfby")
@JsonIgnore
public ExpiredprocessCollectionRequest getLk_expiredprocess_createdonbehalfby() {
return new ExpiredprocessCollectionRequest(
contextPath.addSegment("lk_expiredprocess_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_expiredprocess_createdonbehalfby"));
}
@NavigationProperty(name="systemuser_callbackregistration_modifiedonbehalfby")
@JsonIgnore
public CallbackregistrationCollectionRequest getSystemuser_callbackregistration_modifiedonbehalfby() {
return new CallbackregistrationCollectionRequest(
contextPath.addSegment("systemuser_callbackregistration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_callbackregistration_modifiedonbehalfby"));
}
@NavigationProperty(name="systemuser_callbackregistration_modifiedby")
@JsonIgnore
public CallbackregistrationCollectionRequest getSystemuser_callbackregistration_modifiedby() {
return new CallbackregistrationCollectionRequest(
contextPath.addSegment("systemuser_callbackregistration_modifiedby"), RequestHelper.getValue(unmappedFields, "systemuser_callbackregistration_modifiedby"));
}
@NavigationProperty(name="systemuser_callbackregistration_createdonbehalfby")
@JsonIgnore
public CallbackregistrationCollectionRequest getSystemuser_callbackregistration_createdonbehalfby() {
return new CallbackregistrationCollectionRequest(
contextPath.addSegment("systemuser_callbackregistration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "systemuser_callbackregistration_createdonbehalfby"));
}
@NavigationProperty(name="systemuser_callbackregistration_createdby")
@JsonIgnore
public CallbackregistrationCollectionRequest getSystemuser_callbackregistration_createdby() {
return new CallbackregistrationCollectionRequest(
contextPath.addSegment("systemuser_callbackregistration_createdby"), RequestHelper.getValue(unmappedFields, "systemuser_callbackregistration_createdby"));
}
@NavigationProperty(name="lk_solutioncomponentattributeconfiguration_createdby")
@JsonIgnore
public SolutioncomponentattributeconfigurationCollectionRequest getLk_solutioncomponentattributeconfiguration_createdby() {
return new SolutioncomponentattributeconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentattributeconfiguration_createdby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentattributeconfiguration_createdby"));
}
@NavigationProperty(name="lk_solutioncomponentattributeconfiguration_createdonbehalfby")
@JsonIgnore
public SolutioncomponentattributeconfigurationCollectionRequest getLk_solutioncomponentattributeconfiguration_createdonbehalfby() {
return new SolutioncomponentattributeconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentattributeconfiguration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentattributeconfiguration_createdonbehalfby"));
}
@NavigationProperty(name="lk_solutioncomponentattributeconfiguration_modifiedby")
@JsonIgnore
public SolutioncomponentattributeconfigurationCollectionRequest getLk_solutioncomponentattributeconfiguration_modifiedby() {
return new SolutioncomponentattributeconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentattributeconfiguration_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentattributeconfiguration_modifiedby"));
}
@NavigationProperty(name="lk_solutioncomponentattributeconfiguration_modifiedonbehalfby")
@JsonIgnore
public SolutioncomponentattributeconfigurationCollectionRequest getLk_solutioncomponentattributeconfiguration_modifiedonbehalfby() {
return new SolutioncomponentattributeconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentattributeconfiguration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentattributeconfiguration_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_solutioncomponentconfiguration_createdby")
@JsonIgnore
public SolutioncomponentconfigurationCollectionRequest getLk_solutioncomponentconfiguration_createdby() {
return new SolutioncomponentconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentconfiguration_createdby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentconfiguration_createdby"));
}
@NavigationProperty(name="lk_solutioncomponentconfiguration_createdonbehalfby")
@JsonIgnore
public SolutioncomponentconfigurationCollectionRequest getLk_solutioncomponentconfiguration_createdonbehalfby() {
return new SolutioncomponentconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentconfiguration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentconfiguration_createdonbehalfby"));
}
@NavigationProperty(name="lk_solutioncomponentconfiguration_modifiedby")
@JsonIgnore
public SolutioncomponentconfigurationCollectionRequest getLk_solutioncomponentconfiguration_modifiedby() {
return new SolutioncomponentconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentconfiguration_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentconfiguration_modifiedby"));
}
@NavigationProperty(name="lk_solutioncomponentconfiguration_modifiedonbehalfby")
@JsonIgnore
public SolutioncomponentconfigurationCollectionRequest getLk_solutioncomponentconfiguration_modifiedonbehalfby() {
return new SolutioncomponentconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentconfiguration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentconfiguration_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_solutioncomponentrelationshipconfiguration_createdby")
@JsonIgnore
public SolutioncomponentrelationshipconfigurationCollectionRequest getLk_solutioncomponentrelationshipconfiguration_createdby() {
return new SolutioncomponentrelationshipconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_createdby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentrelationshipconfiguration_createdby"));
}
@NavigationProperty(name="lk_solutioncomponentrelationshipconfiguration_createdonbehalfby")
@JsonIgnore
public SolutioncomponentrelationshipconfigurationCollectionRequest getLk_solutioncomponentrelationshipconfiguration_createdonbehalfby() {
return new SolutioncomponentrelationshipconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentrelationshipconfiguration_createdonbehalfby"));
}
@NavigationProperty(name="lk_solutioncomponentrelationshipconfiguration_modifiedby")
@JsonIgnore
public SolutioncomponentrelationshipconfigurationCollectionRequest getLk_solutioncomponentrelationshipconfiguration_modifiedby() {
return new SolutioncomponentrelationshipconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentrelationshipconfiguration_modifiedby"));
}
@NavigationProperty(name="lk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby")
@JsonIgnore
public SolutioncomponentrelationshipconfigurationCollectionRequest getLk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby() {
return new SolutioncomponentrelationshipconfigurationCollectionRequest(
contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_stagesolutionupload_createdby")
@JsonIgnore
public StagesolutionuploadCollectionRequest getLk_stagesolutionupload_createdby() {
return new StagesolutionuploadCollectionRequest(
contextPath.addSegment("lk_stagesolutionupload_createdby"), RequestHelper.getValue(unmappedFields, "lk_stagesolutionupload_createdby"));
}
@NavigationProperty(name="lk_stagesolutionupload_createdonbehalfby")
@JsonIgnore
public StagesolutionuploadCollectionRequest getLk_stagesolutionupload_createdonbehalfby() {
return new StagesolutionuploadCollectionRequest(
contextPath.addSegment("lk_stagesolutionupload_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_stagesolutionupload_createdonbehalfby"));
}
@NavigationProperty(name="lk_stagesolutionupload_modifiedby")
@JsonIgnore
public StagesolutionuploadCollectionRequest getLk_stagesolutionupload_modifiedby() {
return new StagesolutionuploadCollectionRequest(
contextPath.addSegment("lk_stagesolutionupload_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_stagesolutionupload_modifiedby"));
}
@NavigationProperty(name="lk_stagesolutionupload_modifiedonbehalfby")
@JsonIgnore
public StagesolutionuploadCollectionRequest getLk_stagesolutionupload_modifiedonbehalfby() {
return new StagesolutionuploadCollectionRequest(
contextPath.addSegment("lk_stagesolutionupload_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_stagesolutionupload_modifiedonbehalfby"));
}
@NavigationProperty(name="user_stagesolutionupload")
@JsonIgnore
public StagesolutionuploadCollectionRequest getUser_stagesolutionupload() {
return new StagesolutionuploadCollectionRequest(
contextPath.addSegment("user_stagesolutionupload"), RequestHelper.getValue(unmappedFields, "user_stagesolutionupload"));
}
@NavigationProperty(name="lk_exportsolutionupload_createdby")
@JsonIgnore
public ExportsolutionuploadCollectionRequest getLk_exportsolutionupload_createdby() {
return new ExportsolutionuploadCollectionRequest(
contextPath.addSegment("lk_exportsolutionupload_createdby"), RequestHelper.getValue(unmappedFields, "lk_exportsolutionupload_createdby"));
}
@NavigationProperty(name="lk_exportsolutionupload_createdonbehalfby")
@JsonIgnore
public ExportsolutionuploadCollectionRequest getLk_exportsolutionupload_createdonbehalfby() {
return new ExportsolutionuploadCollectionRequest(
contextPath.addSegment("lk_exportsolutionupload_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_exportsolutionupload_createdonbehalfby"));
}
@NavigationProperty(name="lk_exportsolutionupload_modifiedby")
@JsonIgnore
public ExportsolutionuploadCollectionRequest getLk_exportsolutionupload_modifiedby() {
return new ExportsolutionuploadCollectionRequest(
contextPath.addSegment("lk_exportsolutionupload_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_exportsolutionupload_modifiedby"));
}
@NavigationProperty(name="lk_exportsolutionupload_modifiedonbehalfby")
@JsonIgnore
public ExportsolutionuploadCollectionRequest getLk_exportsolutionupload_modifiedonbehalfby() {
return new ExportsolutionuploadCollectionRequest(
contextPath.addSegment("lk_exportsolutionupload_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_exportsolutionupload_modifiedonbehalfby"));
}
@NavigationProperty(name="user_exportsolutionupload")
@JsonIgnore
public ExportsolutionuploadCollectionRequest getUser_exportsolutionupload() {
return new ExportsolutionuploadCollectionRequest(
contextPath.addSegment("user_exportsolutionupload"), RequestHelper.getValue(unmappedFields, "user_exportsolutionupload"));
}
@NavigationProperty(name="lk_datalakeworkspace_createdby")
@JsonIgnore
public DatalakeworkspaceCollectionRequest getLk_datalakeworkspace_createdby() {
return new DatalakeworkspaceCollectionRequest(
contextPath.addSegment("lk_datalakeworkspace_createdby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspace_createdby"));
}
@NavigationProperty(name="lk_datalakeworkspace_createdonbehalfby")
@JsonIgnore
public DatalakeworkspaceCollectionRequest getLk_datalakeworkspace_createdonbehalfby() {
return new DatalakeworkspaceCollectionRequest(
contextPath.addSegment("lk_datalakeworkspace_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspace_createdonbehalfby"));
}
@NavigationProperty(name="lk_datalakeworkspace_modifiedby")
@JsonIgnore
public DatalakeworkspaceCollectionRequest getLk_datalakeworkspace_modifiedby() {
return new DatalakeworkspaceCollectionRequest(
contextPath.addSegment("lk_datalakeworkspace_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspace_modifiedby"));
}
@NavigationProperty(name="lk_datalakeworkspace_modifiedonbehalfby")
@JsonIgnore
public DatalakeworkspaceCollectionRequest getLk_datalakeworkspace_modifiedonbehalfby() {
return new DatalakeworkspaceCollectionRequest(
contextPath.addSegment("lk_datalakeworkspace_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspace_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_datalakeworkspacepermission_createdby")
@JsonIgnore
public DatalakeworkspacepermissionCollectionRequest getLk_datalakeworkspacepermission_createdby() {
return new DatalakeworkspacepermissionCollectionRequest(
contextPath.addSegment("lk_datalakeworkspacepermission_createdby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspacepermission_createdby"));
}
@NavigationProperty(name="lk_datalakeworkspacepermission_createdonbehalfby")
@JsonIgnore
public DatalakeworkspacepermissionCollectionRequest getLk_datalakeworkspacepermission_createdonbehalfby() {
return new DatalakeworkspacepermissionCollectionRequest(
contextPath.addSegment("lk_datalakeworkspacepermission_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspacepermission_createdonbehalfby"));
}
@NavigationProperty(name="lk_datalakeworkspacepermission_modifiedby")
@JsonIgnore
public DatalakeworkspacepermissionCollectionRequest getLk_datalakeworkspacepermission_modifiedby() {
return new DatalakeworkspacepermissionCollectionRequest(
contextPath.addSegment("lk_datalakeworkspacepermission_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspacepermission_modifiedby"));
}
@NavigationProperty(name="lk_datalakeworkspacepermission_modifiedonbehalfby")
@JsonIgnore
public DatalakeworkspacepermissionCollectionRequest getLk_datalakeworkspacepermission_modifiedonbehalfby() {
return new DatalakeworkspacepermissionCollectionRequest(
contextPath.addSegment("lk_datalakeworkspacepermission_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_datalakeworkspacepermission_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_applicationuser_createdby")
@JsonIgnore
public ApplicationuserCollectionRequest getLk_applicationuser_createdby() {
return new ApplicationuserCollectionRequest(
contextPath.addSegment("lk_applicationuser_createdby"), RequestHelper.getValue(unmappedFields, "lk_applicationuser_createdby"));
}
@NavigationProperty(name="lk_applicationuser_createdonbehalfby")
@JsonIgnore
public ApplicationuserCollectionRequest getLk_applicationuser_createdonbehalfby() {
return new ApplicationuserCollectionRequest(
contextPath.addSegment("lk_applicationuser_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_applicationuser_createdonbehalfby"));
}
@NavigationProperty(name="lk_applicationuser_modifiedby")
@JsonIgnore
public ApplicationuserCollectionRequest getLk_applicationuser_modifiedby() {
return new ApplicationuserCollectionRequest(
contextPath.addSegment("lk_applicationuser_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_applicationuser_modifiedby"));
}
@NavigationProperty(name="lk_applicationuser_modifiedonbehalfby")
@JsonIgnore
public ApplicationuserCollectionRequest getLk_applicationuser_modifiedonbehalfby() {
return new ApplicationuserCollectionRequest(
contextPath.addSegment("lk_applicationuser_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_applicationuser_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_serviceplan_createdby")
@JsonIgnore
public ServiceplanCollectionRequest getLk_serviceplan_createdby() {
return new ServiceplanCollectionRequest(
contextPath.addSegment("lk_serviceplan_createdby"), RequestHelper.getValue(unmappedFields, "lk_serviceplan_createdby"));
}
@NavigationProperty(name="lk_serviceplan_createdonbehalfby")
@JsonIgnore
public ServiceplanCollectionRequest getLk_serviceplan_createdonbehalfby() {
return new ServiceplanCollectionRequest(
contextPath.addSegment("lk_serviceplan_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_serviceplan_createdonbehalfby"));
}
@NavigationProperty(name="lk_serviceplan_modifiedby")
@JsonIgnore
public ServiceplanCollectionRequest getLk_serviceplan_modifiedby() {
return new ServiceplanCollectionRequest(
contextPath.addSegment("lk_serviceplan_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_serviceplan_modifiedby"));
}
@NavigationProperty(name="lk_serviceplan_modifiedonbehalfby")
@JsonIgnore
public ServiceplanCollectionRequest getLk_serviceplan_modifiedonbehalfby() {
return new ServiceplanCollectionRequest(
contextPath.addSegment("lk_serviceplan_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_serviceplan_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_connector_createdby")
@JsonIgnore
public ConnectorCollectionRequest getLk_connector_createdby() {
return new ConnectorCollectionRequest(
contextPath.addSegment("lk_connector_createdby"), RequestHelper.getValue(unmappedFields, "lk_connector_createdby"));
}
@NavigationProperty(name="lk_connector_createdonbehalfby")
@JsonIgnore
public ConnectorCollectionRequest getLk_connector_createdonbehalfby() {
return new ConnectorCollectionRequest(
contextPath.addSegment("lk_connector_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connector_createdonbehalfby"));
}
@NavigationProperty(name="lk_connector_modifiedby")
@JsonIgnore
public ConnectorCollectionRequest getLk_connector_modifiedby() {
return new ConnectorCollectionRequest(
contextPath.addSegment("lk_connector_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_connector_modifiedby"));
}
@NavigationProperty(name="lk_connector_modifiedonbehalfby")
@JsonIgnore
public ConnectorCollectionRequest getLk_connector_modifiedonbehalfby() {
return new ConnectorCollectionRequest(
contextPath.addSegment("lk_connector_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connector_modifiedonbehalfby"));
}
@NavigationProperty(name="user_connector")
@JsonIgnore
public ConnectorCollectionRequest getUser_connector() {
return new ConnectorCollectionRequest(
contextPath.addSegment("user_connector"), RequestHelper.getValue(unmappedFields, "user_connector"));
}
@NavigationProperty(name="lk_environmentvariabledefinition_createdby")
@JsonIgnore
public EnvironmentvariabledefinitionCollectionRequest getLk_environmentvariabledefinition_createdby() {
return new EnvironmentvariabledefinitionCollectionRequest(
contextPath.addSegment("lk_environmentvariabledefinition_createdby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariabledefinition_createdby"));
}
@NavigationProperty(name="lk_environmentvariabledefinition_createdonbehalfby")
@JsonIgnore
public EnvironmentvariabledefinitionCollectionRequest getLk_environmentvariabledefinition_createdonbehalfby() {
return new EnvironmentvariabledefinitionCollectionRequest(
contextPath.addSegment("lk_environmentvariabledefinition_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariabledefinition_createdonbehalfby"));
}
@NavigationProperty(name="lk_environmentvariabledefinition_modifiedby")
@JsonIgnore
public EnvironmentvariabledefinitionCollectionRequest getLk_environmentvariabledefinition_modifiedby() {
return new EnvironmentvariabledefinitionCollectionRequest(
contextPath.addSegment("lk_environmentvariabledefinition_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariabledefinition_modifiedby"));
}
@NavigationProperty(name="lk_environmentvariabledefinition_modifiedonbehalfby")
@JsonIgnore
public EnvironmentvariabledefinitionCollectionRequest getLk_environmentvariabledefinition_modifiedonbehalfby() {
return new EnvironmentvariabledefinitionCollectionRequest(
contextPath.addSegment("lk_environmentvariabledefinition_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariabledefinition_modifiedonbehalfby"));
}
@NavigationProperty(name="user_environmentvariabledefinition")
@JsonIgnore
public EnvironmentvariabledefinitionCollectionRequest getUser_environmentvariabledefinition() {
return new EnvironmentvariabledefinitionCollectionRequest(
contextPath.addSegment("user_environmentvariabledefinition"), RequestHelper.getValue(unmappedFields, "user_environmentvariabledefinition"));
}
@NavigationProperty(name="lk_environmentvariablevalue_createdby")
@JsonIgnore
public EnvironmentvariablevalueCollectionRequest getLk_environmentvariablevalue_createdby() {
return new EnvironmentvariablevalueCollectionRequest(
contextPath.addSegment("lk_environmentvariablevalue_createdby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariablevalue_createdby"));
}
@NavigationProperty(name="lk_environmentvariablevalue_createdonbehalfby")
@JsonIgnore
public EnvironmentvariablevalueCollectionRequest getLk_environmentvariablevalue_createdonbehalfby() {
return new EnvironmentvariablevalueCollectionRequest(
contextPath.addSegment("lk_environmentvariablevalue_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariablevalue_createdonbehalfby"));
}
@NavigationProperty(name="lk_environmentvariablevalue_modifiedby")
@JsonIgnore
public EnvironmentvariablevalueCollectionRequest getLk_environmentvariablevalue_modifiedby() {
return new EnvironmentvariablevalueCollectionRequest(
contextPath.addSegment("lk_environmentvariablevalue_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariablevalue_modifiedby"));
}
@NavigationProperty(name="lk_environmentvariablevalue_modifiedonbehalfby")
@JsonIgnore
public EnvironmentvariablevalueCollectionRequest getLk_environmentvariablevalue_modifiedonbehalfby() {
return new EnvironmentvariablevalueCollectionRequest(
contextPath.addSegment("lk_environmentvariablevalue_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_environmentvariablevalue_modifiedonbehalfby"));
}
@NavigationProperty(name="user_environmentvariablevalue")
@JsonIgnore
public EnvironmentvariablevalueCollectionRequest getUser_environmentvariablevalue() {
return new EnvironmentvariablevalueCollectionRequest(
contextPath.addSegment("user_environmentvariablevalue"), RequestHelper.getValue(unmappedFields, "user_environmentvariablevalue"));
}
@NavigationProperty(name="lk_processstageparameter_createdby")
@JsonIgnore
public ProcessstageparameterCollectionRequest getLk_processstageparameter_createdby() {
return new ProcessstageparameterCollectionRequest(
contextPath.addSegment("lk_processstageparameter_createdby"), RequestHelper.getValue(unmappedFields, "lk_processstageparameter_createdby"));
}
@NavigationProperty(name="lk_processstageparameter_createdonbehalfby")
@JsonIgnore
public ProcessstageparameterCollectionRequest getLk_processstageparameter_createdonbehalfby() {
return new ProcessstageparameterCollectionRequest(
contextPath.addSegment("lk_processstageparameter_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processstageparameter_createdonbehalfby"));
}
@NavigationProperty(name="lk_processstageparameter_modifiedby")
@JsonIgnore
public ProcessstageparameterCollectionRequest getLk_processstageparameter_modifiedby() {
return new ProcessstageparameterCollectionRequest(
contextPath.addSegment("lk_processstageparameter_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_processstageparameter_modifiedby"));
}
@NavigationProperty(name="lk_processstageparameter_modifiedonbehalfby")
@JsonIgnore
public ProcessstageparameterCollectionRequest getLk_processstageparameter_modifiedonbehalfby() {
return new ProcessstageparameterCollectionRequest(
contextPath.addSegment("lk_processstageparameter_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_processstageparameter_modifiedonbehalfby"));
}
@NavigationProperty(name="user_processstageparameter")
@JsonIgnore
public ProcessstageparameterCollectionRequest getUser_processstageparameter() {
return new ProcessstageparameterCollectionRequest(
contextPath.addSegment("user_processstageparameter"), RequestHelper.getValue(unmappedFields, "user_processstageparameter"));
}
@NavigationProperty(name="lk_flowsession_createdby")
@JsonIgnore
public FlowsessionCollectionRequest getLk_flowsession_createdby() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("lk_flowsession_createdby"), RequestHelper.getValue(unmappedFields, "lk_flowsession_createdby"));
}
@NavigationProperty(name="lk_flowsession_createdonbehalfby")
@JsonIgnore
public FlowsessionCollectionRequest getLk_flowsession_createdonbehalfby() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("lk_flowsession_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_flowsession_createdonbehalfby"));
}
@NavigationProperty(name="lk_flowsession_modifiedby")
@JsonIgnore
public FlowsessionCollectionRequest getLk_flowsession_modifiedby() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("lk_flowsession_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_flowsession_modifiedby"));
}
@NavigationProperty(name="lk_flowsession_modifiedonbehalfby")
@JsonIgnore
public FlowsessionCollectionRequest getLk_flowsession_modifiedonbehalfby() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("lk_flowsession_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_flowsession_modifiedonbehalfby"));
}
@NavigationProperty(name="user_flowsession")
@JsonIgnore
public FlowsessionCollectionRequest getUser_flowsession() {
return new FlowsessionCollectionRequest(
contextPath.addSegment("user_flowsession"), RequestHelper.getValue(unmappedFields, "user_flowsession"));
}
@NavigationProperty(name="lk_workflowbinary_createdby")
@JsonIgnore
public WorkflowbinaryCollectionRequest getLk_workflowbinary_createdby() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("lk_workflowbinary_createdby"), RequestHelper.getValue(unmappedFields, "lk_workflowbinary_createdby"));
}
@NavigationProperty(name="lk_workflowbinary_createdonbehalfby")
@JsonIgnore
public WorkflowbinaryCollectionRequest getLk_workflowbinary_createdonbehalfby() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("lk_workflowbinary_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_workflowbinary_createdonbehalfby"));
}
@NavigationProperty(name="lk_workflowbinary_modifiedby")
@JsonIgnore
public WorkflowbinaryCollectionRequest getLk_workflowbinary_modifiedby() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("lk_workflowbinary_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_workflowbinary_modifiedby"));
}
@NavigationProperty(name="lk_workflowbinary_modifiedonbehalfby")
@JsonIgnore
public WorkflowbinaryCollectionRequest getLk_workflowbinary_modifiedonbehalfby() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("lk_workflowbinary_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_workflowbinary_modifiedonbehalfby"));
}
@NavigationProperty(name="user_workflowbinary")
@JsonIgnore
public WorkflowbinaryCollectionRequest getUser_workflowbinary() {
return new WorkflowbinaryCollectionRequest(
contextPath.addSegment("user_workflowbinary"), RequestHelper.getValue(unmappedFields, "user_workflowbinary"));
}
@NavigationProperty(name="lk_connectionreference_createdby")
@JsonIgnore
public ConnectionreferenceCollectionRequest getLk_connectionreference_createdby() {
return new ConnectionreferenceCollectionRequest(
contextPath.addSegment("lk_connectionreference_createdby"), RequestHelper.getValue(unmappedFields, "lk_connectionreference_createdby"));
}
@NavigationProperty(name="lk_connectionreference_createdonbehalfby")
@JsonIgnore
public ConnectionreferenceCollectionRequest getLk_connectionreference_createdonbehalfby() {
return new ConnectionreferenceCollectionRequest(
contextPath.addSegment("lk_connectionreference_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionreference_createdonbehalfby"));
}
@NavigationProperty(name="lk_connectionreference_modifiedby")
@JsonIgnore
public ConnectionreferenceCollectionRequest getLk_connectionreference_modifiedby() {
return new ConnectionreferenceCollectionRequest(
contextPath.addSegment("lk_connectionreference_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_connectionreference_modifiedby"));
}
@NavigationProperty(name="lk_connectionreference_modifiedonbehalfby")
@JsonIgnore
public ConnectionreferenceCollectionRequest getLk_connectionreference_modifiedonbehalfby() {
return new ConnectionreferenceCollectionRequest(
contextPath.addSegment("lk_connectionreference_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_connectionreference_modifiedonbehalfby"));
}
@NavigationProperty(name="user_connectionreference")
@JsonIgnore
public ConnectionreferenceCollectionRequest getUser_connectionreference() {
return new ConnectionreferenceCollectionRequest(
contextPath.addSegment("user_connectionreference"), RequestHelper.getValue(unmappedFields, "user_connectionreference"));
}
@NavigationProperty(name="lk_msdyn_helppage_createdby")
@JsonIgnore
public Msdyn_helppageCollectionRequest getLk_msdyn_helppage_createdby() {
return new Msdyn_helppageCollectionRequest(
contextPath.addSegment("lk_msdyn_helppage_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_helppage_createdby"));
}
@NavigationProperty(name="lk_msdyn_helppage_createdonbehalfby")
@JsonIgnore
public Msdyn_helppageCollectionRequest getLk_msdyn_helppage_createdonbehalfby() {
return new Msdyn_helppageCollectionRequest(
contextPath.addSegment("lk_msdyn_helppage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_helppage_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_helppage_modifiedby")
@JsonIgnore
public Msdyn_helppageCollectionRequest getLk_msdyn_helppage_modifiedby() {
return new Msdyn_helppageCollectionRequest(
contextPath.addSegment("lk_msdyn_helppage_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_helppage_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_helppage_modifiedonbehalfby")
@JsonIgnore
public Msdyn_helppageCollectionRequest getLk_msdyn_helppage_modifiedonbehalfby() {
return new Msdyn_helppageCollectionRequest(
contextPath.addSegment("lk_msdyn_helppage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_helppage_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_territorybase_createdby")
@JsonIgnore
public TerritoryCollectionRequest getLk_territorybase_createdby() {
return new TerritoryCollectionRequest(
contextPath.addSegment("lk_territorybase_createdby"), RequestHelper.getValue(unmappedFields, "lk_territorybase_createdby"));
}
@NavigationProperty(name="lk_territorybase_createdonbehalfby")
@JsonIgnore
public TerritoryCollectionRequest getLk_territorybase_createdonbehalfby() {
return new TerritoryCollectionRequest(
contextPath.addSegment("lk_territorybase_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_territorybase_createdonbehalfby"));
}
@NavigationProperty(name="lk_territorybase_modifiedby")
@JsonIgnore
public TerritoryCollectionRequest getLk_territorybase_modifiedby() {
return new TerritoryCollectionRequest(
contextPath.addSegment("lk_territorybase_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_territorybase_modifiedby"));
}
@NavigationProperty(name="lk_territorybase_modifiedonbehalfby")
@JsonIgnore
public TerritoryCollectionRequest getLk_territorybase_modifiedonbehalfby() {
return new TerritoryCollectionRequest(
contextPath.addSegment("lk_territorybase_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_territorybase_modifiedonbehalfby"));
}
@NavigationProperty(name="system_user_territories")
@JsonIgnore
public TerritoryCollectionRequest getSystem_user_territories() {
return new TerritoryCollectionRequest(
contextPath.addSegment("system_user_territories"), RequestHelper.getValue(unmappedFields, "system_user_territories"));
}
@NavigationProperty(name="territoryid")
@JsonIgnore
public TerritoryRequest getTerritoryid() {
return new TerritoryRequest(contextPath.addSegment("territoryid"), RequestHelper.getValue(unmappedFields, "territoryid"));
}
@NavigationProperty(name="lk_msdyn_serviceconfiguration_createdby")
@JsonIgnore
public Msdyn_serviceconfigurationCollectionRequest getLk_msdyn_serviceconfiguration_createdby() {
return new Msdyn_serviceconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_serviceconfiguration_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_serviceconfiguration_createdby"));
}
@NavigationProperty(name="lk_msdyn_serviceconfiguration_createdonbehalfby")
@JsonIgnore
public Msdyn_serviceconfigurationCollectionRequest getLk_msdyn_serviceconfiguration_createdonbehalfby() {
return new Msdyn_serviceconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_serviceconfiguration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_serviceconfiguration_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_serviceconfiguration_modifiedby")
@JsonIgnore
public Msdyn_serviceconfigurationCollectionRequest getLk_msdyn_serviceconfiguration_modifiedby() {
return new Msdyn_serviceconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_serviceconfiguration_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_serviceconfiguration_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_serviceconfiguration_modifiedonbehalfby")
@JsonIgnore
public Msdyn_serviceconfigurationCollectionRequest getLk_msdyn_serviceconfiguration_modifiedonbehalfby() {
return new Msdyn_serviceconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_serviceconfiguration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_serviceconfiguration_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_serviceconfiguration")
@JsonIgnore
public Msdyn_serviceconfigurationCollectionRequest getUser_msdyn_serviceconfiguration() {
return new Msdyn_serviceconfigurationCollectionRequest(
contextPath.addSegment("user_msdyn_serviceconfiguration"), RequestHelper.getValue(unmappedFields, "user_msdyn_serviceconfiguration"));
}
@NavigationProperty(name="lk_msdyn_slakpi_createdby")
@JsonIgnore
public Msdyn_slakpiCollectionRequest getLk_msdyn_slakpi_createdby() {
return new Msdyn_slakpiCollectionRequest(
contextPath.addSegment("lk_msdyn_slakpi_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_slakpi_createdby"));
}
@NavigationProperty(name="lk_msdyn_slakpi_createdonbehalfby")
@JsonIgnore
public Msdyn_slakpiCollectionRequest getLk_msdyn_slakpi_createdonbehalfby() {
return new Msdyn_slakpiCollectionRequest(
contextPath.addSegment("lk_msdyn_slakpi_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_slakpi_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_slakpi_modifiedby")
@JsonIgnore
public Msdyn_slakpiCollectionRequest getLk_msdyn_slakpi_modifiedby() {
return new Msdyn_slakpiCollectionRequest(
contextPath.addSegment("lk_msdyn_slakpi_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_slakpi_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_slakpi_modifiedonbehalfby")
@JsonIgnore
public Msdyn_slakpiCollectionRequest getLk_msdyn_slakpi_modifiedonbehalfby() {
return new Msdyn_slakpiCollectionRequest(
contextPath.addSegment("lk_msdyn_slakpi_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_slakpi_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_slakpi")
@JsonIgnore
public Msdyn_slakpiCollectionRequest getUser_msdyn_slakpi() {
return new Msdyn_slakpiCollectionRequest(
contextPath.addSegment("user_msdyn_slakpi"), RequestHelper.getValue(unmappedFields, "user_msdyn_slakpi"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticleimage_createdby")
@JsonIgnore
public Msdyn_knowledgearticleimageCollectionRequest getLk_msdyn_knowledgearticleimage_createdby() {
return new Msdyn_knowledgearticleimageCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticleimage_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticleimage_createdby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticleimage_createdonbehalfby")
@JsonIgnore
public Msdyn_knowledgearticleimageCollectionRequest getLk_msdyn_knowledgearticleimage_createdonbehalfby() {
return new Msdyn_knowledgearticleimageCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticleimage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticleimage_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticleimage_modifiedby")
@JsonIgnore
public Msdyn_knowledgearticleimageCollectionRequest getLk_msdyn_knowledgearticleimage_modifiedby() {
return new Msdyn_knowledgearticleimageCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticleimage_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticleimage_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticleimage_modifiedonbehalfby")
@JsonIgnore
public Msdyn_knowledgearticleimageCollectionRequest getLk_msdyn_knowledgearticleimage_modifiedonbehalfby() {
return new Msdyn_knowledgearticleimageCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticleimage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticleimage_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_knowledgearticleimage")
@JsonIgnore
public Msdyn_knowledgearticleimageCollectionRequest getUser_msdyn_knowledgearticleimage() {
return new Msdyn_knowledgearticleimageCollectionRequest(
contextPath.addSegment("user_msdyn_knowledgearticleimage"), RequestHelper.getValue(unmappedFields, "user_msdyn_knowledgearticleimage"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticletemplate_createdby")
@JsonIgnore
public Msdyn_knowledgearticletemplateCollectionRequest getLk_msdyn_knowledgearticletemplate_createdby() {
return new Msdyn_knowledgearticletemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticletemplate_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticletemplate_createdby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticletemplate_createdonbehalfby")
@JsonIgnore
public Msdyn_knowledgearticletemplateCollectionRequest getLk_msdyn_knowledgearticletemplate_createdonbehalfby() {
return new Msdyn_knowledgearticletemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticletemplate_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticletemplate_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticletemplate_modifiedby")
@JsonIgnore
public Msdyn_knowledgearticletemplateCollectionRequest getLk_msdyn_knowledgearticletemplate_modifiedby() {
return new Msdyn_knowledgearticletemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticletemplate_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticletemplate_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_knowledgearticletemplate_modifiedonbehalfby")
@JsonIgnore
public Msdyn_knowledgearticletemplateCollectionRequest getLk_msdyn_knowledgearticletemplate_modifiedonbehalfby() {
return new Msdyn_knowledgearticletemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_knowledgearticletemplate_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_knowledgearticletemplate_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_knowledgearticletemplate")
@JsonIgnore
public Msdyn_knowledgearticletemplateCollectionRequest getUser_msdyn_knowledgearticletemplate() {
return new Msdyn_knowledgearticletemplateCollectionRequest(
contextPath.addSegment("user_msdyn_knowledgearticletemplate"), RequestHelper.getValue(unmappedFields, "user_msdyn_knowledgearticletemplate"));
}
@NavigationProperty(name="lk_msdyn_dataflow_createdby")
@JsonIgnore
public Msdyn_dataflowCollectionRequest getLk_msdyn_dataflow_createdby() {
return new Msdyn_dataflowCollectionRequest(
contextPath.addSegment("lk_msdyn_dataflow_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_dataflow_createdby"));
}
@NavigationProperty(name="lk_msdyn_dataflow_createdonbehalfby")
@JsonIgnore
public Msdyn_dataflowCollectionRequest getLk_msdyn_dataflow_createdonbehalfby() {
return new Msdyn_dataflowCollectionRequest(
contextPath.addSegment("lk_msdyn_dataflow_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_dataflow_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_dataflow_modifiedby")
@JsonIgnore
public Msdyn_dataflowCollectionRequest getLk_msdyn_dataflow_modifiedby() {
return new Msdyn_dataflowCollectionRequest(
contextPath.addSegment("lk_msdyn_dataflow_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_dataflow_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_dataflow_modifiedonbehalfby")
@JsonIgnore
public Msdyn_dataflowCollectionRequest getLk_msdyn_dataflow_modifiedonbehalfby() {
return new Msdyn_dataflowCollectionRequest(
contextPath.addSegment("lk_msdyn_dataflow_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_dataflow_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_dataflow")
@JsonIgnore
public Msdyn_dataflowCollectionRequest getUser_msdyn_dataflow() {
return new Msdyn_dataflowCollectionRequest(
contextPath.addSegment("user_msdyn_dataflow"), RequestHelper.getValue(unmappedFields, "user_msdyn_dataflow"));
}
@NavigationProperty(name="lk_msdyn_richtextfile_createdby")
@JsonIgnore
public Msdyn_richtextfileCollectionRequest getLk_msdyn_richtextfile_createdby() {
return new Msdyn_richtextfileCollectionRequest(
contextPath.addSegment("lk_msdyn_richtextfile_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_richtextfile_createdby"));
}
@NavigationProperty(name="lk_msdyn_richtextfile_createdonbehalfby")
@JsonIgnore
public Msdyn_richtextfileCollectionRequest getLk_msdyn_richtextfile_createdonbehalfby() {
return new Msdyn_richtextfileCollectionRequest(
contextPath.addSegment("lk_msdyn_richtextfile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_richtextfile_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_richtextfile_modifiedby")
@JsonIgnore
public Msdyn_richtextfileCollectionRequest getLk_msdyn_richtextfile_modifiedby() {
return new Msdyn_richtextfileCollectionRequest(
contextPath.addSegment("lk_msdyn_richtextfile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_richtextfile_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_richtextfile_modifiedonbehalfby")
@JsonIgnore
public Msdyn_richtextfileCollectionRequest getLk_msdyn_richtextfile_modifiedonbehalfby() {
return new Msdyn_richtextfileCollectionRequest(
contextPath.addSegment("lk_msdyn_richtextfile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_richtextfile_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_richtextfile")
@JsonIgnore
public Msdyn_richtextfileCollectionRequest getUser_msdyn_richtextfile() {
return new Msdyn_richtextfileCollectionRequest(
contextPath.addSegment("user_msdyn_richtextfile"), RequestHelper.getValue(unmappedFields, "user_msdyn_richtextfile"));
}
@NavigationProperty(name="lk_msdyn_aiconfiguration_createdby")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getLk_msdyn_aiconfiguration_createdby() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_aiconfiguration_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiconfiguration_createdby"));
}
@NavigationProperty(name="lk_msdyn_aiconfiguration_createdonbehalfby")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getLk_msdyn_aiconfiguration_createdonbehalfby() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_aiconfiguration_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiconfiguration_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aiconfiguration_modifiedby")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getLk_msdyn_aiconfiguration_modifiedby() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_aiconfiguration_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiconfiguration_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aiconfiguration_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getLk_msdyn_aiconfiguration_modifiedonbehalfby() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("lk_msdyn_aiconfiguration_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiconfiguration_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aiconfiguration")
@JsonIgnore
public Msdyn_aiconfigurationCollectionRequest getUser_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurationCollectionRequest(
contextPath.addSegment("user_msdyn_aiconfiguration"), RequestHelper.getValue(unmappedFields, "user_msdyn_aiconfiguration"));
}
@NavigationProperty(name="lk_msdyn_aimodel_createdby")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getLk_msdyn_aimodel_createdby() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("lk_msdyn_aimodel_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aimodel_createdby"));
}
@NavigationProperty(name="lk_msdyn_aimodel_createdonbehalfby")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getLk_msdyn_aimodel_createdonbehalfby() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("lk_msdyn_aimodel_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aimodel_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aimodel_modifiedby")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getLk_msdyn_aimodel_modifiedby() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("lk_msdyn_aimodel_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aimodel_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aimodel_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getLk_msdyn_aimodel_modifiedonbehalfby() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("lk_msdyn_aimodel_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aimodel_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aimodel")
@JsonIgnore
public Msdyn_aimodelCollectionRequest getUser_msdyn_aimodel() {
return new Msdyn_aimodelCollectionRequest(
contextPath.addSegment("user_msdyn_aimodel"), RequestHelper.getValue(unmappedFields, "user_msdyn_aimodel"));
}
@NavigationProperty(name="lk_msdyn_aitemplate_createdby")
@JsonIgnore
public Msdyn_aitemplateCollectionRequest getLk_msdyn_aitemplate_createdby() {
return new Msdyn_aitemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_aitemplate_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aitemplate_createdby"));
}
@NavigationProperty(name="lk_msdyn_aitemplate_createdonbehalfby")
@JsonIgnore
public Msdyn_aitemplateCollectionRequest getLk_msdyn_aitemplate_createdonbehalfby() {
return new Msdyn_aitemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_aitemplate_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aitemplate_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aitemplate_modifiedby")
@JsonIgnore
public Msdyn_aitemplateCollectionRequest getLk_msdyn_aitemplate_modifiedby() {
return new Msdyn_aitemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_aitemplate_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aitemplate_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aitemplate_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aitemplateCollectionRequest getLk_msdyn_aitemplate_modifiedonbehalfby() {
return new Msdyn_aitemplateCollectionRequest(
contextPath.addSegment("lk_msdyn_aitemplate_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aitemplate_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aitemplate")
@JsonIgnore
public Msdyn_aitemplateCollectionRequest getUser_msdyn_aitemplate() {
return new Msdyn_aitemplateCollectionRequest(
contextPath.addSegment("user_msdyn_aitemplate"), RequestHelper.getValue(unmappedFields, "user_msdyn_aitemplate"));
}
@NavigationProperty(name="lk_msdyn_aibdataset_createdby")
@JsonIgnore
public Msdyn_aibdatasetCollectionRequest getLk_msdyn_aibdataset_createdby() {
return new Msdyn_aibdatasetCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdataset_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdataset_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibdataset_createdonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetCollectionRequest getLk_msdyn_aibdataset_createdonbehalfby() {
return new Msdyn_aibdatasetCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdataset_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdataset_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibdataset_modifiedby")
@JsonIgnore
public Msdyn_aibdatasetCollectionRequest getLk_msdyn_aibdataset_modifiedby() {
return new Msdyn_aibdatasetCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdataset_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdataset_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibdataset_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetCollectionRequest getLk_msdyn_aibdataset_modifiedonbehalfby() {
return new Msdyn_aibdatasetCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdataset_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdataset_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibdataset")
@JsonIgnore
public Msdyn_aibdatasetCollectionRequest getUser_msdyn_aibdataset() {
return new Msdyn_aibdatasetCollectionRequest(
contextPath.addSegment("user_msdyn_aibdataset"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibdataset"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetfile_createdby")
@JsonIgnore
public Msdyn_aibdatasetfileCollectionRequest getLk_msdyn_aibdatasetfile_createdby() {
return new Msdyn_aibdatasetfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetfile_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetfile_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetfile_createdonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetfileCollectionRequest getLk_msdyn_aibdatasetfile_createdonbehalfby() {
return new Msdyn_aibdatasetfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetfile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetfile_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetfile_modifiedby")
@JsonIgnore
public Msdyn_aibdatasetfileCollectionRequest getLk_msdyn_aibdatasetfile_modifiedby() {
return new Msdyn_aibdatasetfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetfile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetfile_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetfile_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetfileCollectionRequest getLk_msdyn_aibdatasetfile_modifiedonbehalfby() {
return new Msdyn_aibdatasetfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetfile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetfile_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibdatasetfile")
@JsonIgnore
public Msdyn_aibdatasetfileCollectionRequest getUser_msdyn_aibdatasetfile() {
return new Msdyn_aibdatasetfileCollectionRequest(
contextPath.addSegment("user_msdyn_aibdatasetfile"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibdatasetfile"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetrecord_createdby")
@JsonIgnore
public Msdyn_aibdatasetrecordCollectionRequest getLk_msdyn_aibdatasetrecord_createdby() {
return new Msdyn_aibdatasetrecordCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetrecord_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetrecord_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetrecord_createdonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetrecordCollectionRequest getLk_msdyn_aibdatasetrecord_createdonbehalfby() {
return new Msdyn_aibdatasetrecordCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetrecord_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetrecord_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetrecord_modifiedby")
@JsonIgnore
public Msdyn_aibdatasetrecordCollectionRequest getLk_msdyn_aibdatasetrecord_modifiedby() {
return new Msdyn_aibdatasetrecordCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetrecord_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetrecord_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetrecord_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetrecordCollectionRequest getLk_msdyn_aibdatasetrecord_modifiedonbehalfby() {
return new Msdyn_aibdatasetrecordCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetrecord_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetrecord_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibdatasetrecord")
@JsonIgnore
public Msdyn_aibdatasetrecordCollectionRequest getUser_msdyn_aibdatasetrecord() {
return new Msdyn_aibdatasetrecordCollectionRequest(
contextPath.addSegment("user_msdyn_aibdatasetrecord"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibdatasetrecord"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetscontainer_createdby")
@JsonIgnore
public Msdyn_aibdatasetscontainerCollectionRequest getLk_msdyn_aibdatasetscontainer_createdby() {
return new Msdyn_aibdatasetscontainerCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetscontainer_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetscontainer_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetscontainer_createdonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetscontainerCollectionRequest getLk_msdyn_aibdatasetscontainer_createdonbehalfby() {
return new Msdyn_aibdatasetscontainerCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetscontainer_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetscontainer_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetscontainer_modifiedby")
@JsonIgnore
public Msdyn_aibdatasetscontainerCollectionRequest getLk_msdyn_aibdatasetscontainer_modifiedby() {
return new Msdyn_aibdatasetscontainerCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetscontainer_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetscontainer_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibdatasetscontainer_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibdatasetscontainerCollectionRequest getLk_msdyn_aibdatasetscontainer_modifiedonbehalfby() {
return new Msdyn_aibdatasetscontainerCollectionRequest(
contextPath.addSegment("lk_msdyn_aibdatasetscontainer_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibdatasetscontainer_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibdatasetscontainer")
@JsonIgnore
public Msdyn_aibdatasetscontainerCollectionRequest getUser_msdyn_aibdatasetscontainer() {
return new Msdyn_aibdatasetscontainerCollectionRequest(
contextPath.addSegment("user_msdyn_aibdatasetscontainer"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibdatasetscontainer"));
}
@NavigationProperty(name="lk_msdyn_aibfile_createdby")
@JsonIgnore
public Msdyn_aibfileCollectionRequest getLk_msdyn_aibfile_createdby() {
return new Msdyn_aibfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfile_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfile_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibfile_createdonbehalfby")
@JsonIgnore
public Msdyn_aibfileCollectionRequest getLk_msdyn_aibfile_createdonbehalfby() {
return new Msdyn_aibfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfile_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfile_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibfile_modifiedby")
@JsonIgnore
public Msdyn_aibfileCollectionRequest getLk_msdyn_aibfile_modifiedby() {
return new Msdyn_aibfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfile_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfile_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibfile_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibfileCollectionRequest getLk_msdyn_aibfile_modifiedonbehalfby() {
return new Msdyn_aibfileCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfile_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfile_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibfile")
@JsonIgnore
public Msdyn_aibfileCollectionRequest getUser_msdyn_aibfile() {
return new Msdyn_aibfileCollectionRequest(
contextPath.addSegment("user_msdyn_aibfile"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibfile"));
}
@NavigationProperty(name="lk_msdyn_aibfileattacheddata_createdby")
@JsonIgnore
public Msdyn_aibfileattacheddataCollectionRequest getLk_msdyn_aibfileattacheddata_createdby() {
return new Msdyn_aibfileattacheddataCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfileattacheddata_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfileattacheddata_createdby"));
}
@NavigationProperty(name="lk_msdyn_aibfileattacheddata_createdonbehalfby")
@JsonIgnore
public Msdyn_aibfileattacheddataCollectionRequest getLk_msdyn_aibfileattacheddata_createdonbehalfby() {
return new Msdyn_aibfileattacheddataCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfileattacheddata_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfileattacheddata_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aibfileattacheddata_modifiedby")
@JsonIgnore
public Msdyn_aibfileattacheddataCollectionRequest getLk_msdyn_aibfileattacheddata_modifiedby() {
return new Msdyn_aibfileattacheddataCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfileattacheddata_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfileattacheddata_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aibfileattacheddata_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aibfileattacheddataCollectionRequest getLk_msdyn_aibfileattacheddata_modifiedonbehalfby() {
return new Msdyn_aibfileattacheddataCollectionRequest(
contextPath.addSegment("lk_msdyn_aibfileattacheddata_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aibfileattacheddata_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aibfileattacheddata")
@JsonIgnore
public Msdyn_aibfileattacheddataCollectionRequest getUser_msdyn_aibfileattacheddata() {
return new Msdyn_aibfileattacheddataCollectionRequest(
contextPath.addSegment("user_msdyn_aibfileattacheddata"), RequestHelper.getValue(unmappedFields, "user_msdyn_aibfileattacheddata"));
}
@NavigationProperty(name="lk_msdyn_aifptrainingdocument_createdby")
@JsonIgnore
public Msdyn_aifptrainingdocumentCollectionRequest getLk_msdyn_aifptrainingdocument_createdby() {
return new Msdyn_aifptrainingdocumentCollectionRequest(
contextPath.addSegment("lk_msdyn_aifptrainingdocument_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aifptrainingdocument_createdby"));
}
@NavigationProperty(name="lk_msdyn_aifptrainingdocument_createdonbehalfby")
@JsonIgnore
public Msdyn_aifptrainingdocumentCollectionRequest getLk_msdyn_aifptrainingdocument_createdonbehalfby() {
return new Msdyn_aifptrainingdocumentCollectionRequest(
contextPath.addSegment("lk_msdyn_aifptrainingdocument_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aifptrainingdocument_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aifptrainingdocument_modifiedby")
@JsonIgnore
public Msdyn_aifptrainingdocumentCollectionRequest getLk_msdyn_aifptrainingdocument_modifiedby() {
return new Msdyn_aifptrainingdocumentCollectionRequest(
contextPath.addSegment("lk_msdyn_aifptrainingdocument_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aifptrainingdocument_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aifptrainingdocument_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aifptrainingdocumentCollectionRequest getLk_msdyn_aifptrainingdocument_modifiedonbehalfby() {
return new Msdyn_aifptrainingdocumentCollectionRequest(
contextPath.addSegment("lk_msdyn_aifptrainingdocument_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aifptrainingdocument_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aifptrainingdocument")
@JsonIgnore
public Msdyn_aifptrainingdocumentCollectionRequest getUser_msdyn_aifptrainingdocument() {
return new Msdyn_aifptrainingdocumentCollectionRequest(
contextPath.addSegment("user_msdyn_aifptrainingdocument"), RequestHelper.getValue(unmappedFields, "user_msdyn_aifptrainingdocument"));
}
@NavigationProperty(name="lk_msdyn_aiodimage_createdby")
@JsonIgnore
public Msdyn_aiodimageCollectionRequest getLk_msdyn_aiodimage_createdby() {
return new Msdyn_aiodimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodimage_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodimage_createdby"));
}
@NavigationProperty(name="lk_msdyn_aiodimage_createdonbehalfby")
@JsonIgnore
public Msdyn_aiodimageCollectionRequest getLk_msdyn_aiodimage_createdonbehalfby() {
return new Msdyn_aiodimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodimage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodimage_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aiodimage_modifiedby")
@JsonIgnore
public Msdyn_aiodimageCollectionRequest getLk_msdyn_aiodimage_modifiedby() {
return new Msdyn_aiodimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodimage_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodimage_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aiodimage_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aiodimageCollectionRequest getLk_msdyn_aiodimage_modifiedonbehalfby() {
return new Msdyn_aiodimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodimage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodimage_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aiodimage")
@JsonIgnore
public Msdyn_aiodimageCollectionRequest getUser_msdyn_aiodimage() {
return new Msdyn_aiodimageCollectionRequest(
contextPath.addSegment("user_msdyn_aiodimage"), RequestHelper.getValue(unmappedFields, "user_msdyn_aiodimage"));
}
@NavigationProperty(name="lk_msdyn_aiodlabel_createdby")
@JsonIgnore
public Msdyn_aiodlabelCollectionRequest getLk_msdyn_aiodlabel_createdby() {
return new Msdyn_aiodlabelCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodlabel_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodlabel_createdby"));
}
@NavigationProperty(name="lk_msdyn_aiodlabel_createdonbehalfby")
@JsonIgnore
public Msdyn_aiodlabelCollectionRequest getLk_msdyn_aiodlabel_createdonbehalfby() {
return new Msdyn_aiodlabelCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodlabel_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodlabel_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aiodlabel_modifiedby")
@JsonIgnore
public Msdyn_aiodlabelCollectionRequest getLk_msdyn_aiodlabel_modifiedby() {
return new Msdyn_aiodlabelCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodlabel_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodlabel_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aiodlabel_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aiodlabelCollectionRequest getLk_msdyn_aiodlabel_modifiedonbehalfby() {
return new Msdyn_aiodlabelCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodlabel_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodlabel_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aiodlabel")
@JsonIgnore
public Msdyn_aiodlabelCollectionRequest getUser_msdyn_aiodlabel() {
return new Msdyn_aiodlabelCollectionRequest(
contextPath.addSegment("user_msdyn_aiodlabel"), RequestHelper.getValue(unmappedFields, "user_msdyn_aiodlabel"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingboundingbox_createdby")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getLk_msdyn_aiodtrainingboundingbox_createdby() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingboundingbox_createdby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingboundingbox_createdonbehalfby")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getLk_msdyn_aiodtrainingboundingbox_createdonbehalfby() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingboundingbox_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingboundingbox_modifiedby")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getLk_msdyn_aiodtrainingboundingbox_modifiedby() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingboundingbox_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getLk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aiodtrainingboundingbox")
@JsonIgnore
public Msdyn_aiodtrainingboundingboxCollectionRequest getUser_msdyn_aiodtrainingboundingbox() {
return new Msdyn_aiodtrainingboundingboxCollectionRequest(
contextPath.addSegment("user_msdyn_aiodtrainingboundingbox"), RequestHelper.getValue(unmappedFields, "user_msdyn_aiodtrainingboundingbox"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingimage_createdby")
@JsonIgnore
public Msdyn_aiodtrainingimageCollectionRequest getLk_msdyn_aiodtrainingimage_createdby() {
return new Msdyn_aiodtrainingimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingimage_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingimage_createdby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingimage_createdonbehalfby")
@JsonIgnore
public Msdyn_aiodtrainingimageCollectionRequest getLk_msdyn_aiodtrainingimage_createdonbehalfby() {
return new Msdyn_aiodtrainingimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingimage_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingimage_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingimage_modifiedby")
@JsonIgnore
public Msdyn_aiodtrainingimageCollectionRequest getLk_msdyn_aiodtrainingimage_modifiedby() {
return new Msdyn_aiodtrainingimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingimage_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingimage_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_aiodtrainingimage_modifiedonbehalfby")
@JsonIgnore
public Msdyn_aiodtrainingimageCollectionRequest getLk_msdyn_aiodtrainingimage_modifiedonbehalfby() {
return new Msdyn_aiodtrainingimageCollectionRequest(
contextPath.addSegment("lk_msdyn_aiodtrainingimage_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_aiodtrainingimage_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_aiodtrainingimage")
@JsonIgnore
public Msdyn_aiodtrainingimageCollectionRequest getUser_msdyn_aiodtrainingimage() {
return new Msdyn_aiodtrainingimageCollectionRequest(
contextPath.addSegment("user_msdyn_aiodtrainingimage"), RequestHelper.getValue(unmappedFields, "user_msdyn_aiodtrainingimage"));
}
@NavigationProperty(name="lk_msdyn_analysiscomponent_createdby")
@JsonIgnore
public Msdyn_analysiscomponentCollectionRequest getLk_msdyn_analysiscomponent_createdby() {
return new Msdyn_analysiscomponentCollectionRequest(
contextPath.addSegment("lk_msdyn_analysiscomponent_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysiscomponent_createdby"));
}
@NavigationProperty(name="lk_msdyn_analysiscomponent_createdonbehalfby")
@JsonIgnore
public Msdyn_analysiscomponentCollectionRequest getLk_msdyn_analysiscomponent_createdonbehalfby() {
return new Msdyn_analysiscomponentCollectionRequest(
contextPath.addSegment("lk_msdyn_analysiscomponent_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysiscomponent_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_analysiscomponent_modifiedby")
@JsonIgnore
public Msdyn_analysiscomponentCollectionRequest getLk_msdyn_analysiscomponent_modifiedby() {
return new Msdyn_analysiscomponentCollectionRequest(
contextPath.addSegment("lk_msdyn_analysiscomponent_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysiscomponent_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_analysiscomponent_modifiedonbehalfby")
@JsonIgnore
public Msdyn_analysiscomponentCollectionRequest getLk_msdyn_analysiscomponent_modifiedonbehalfby() {
return new Msdyn_analysiscomponentCollectionRequest(
contextPath.addSegment("lk_msdyn_analysiscomponent_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysiscomponent_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_analysiscomponent")
@JsonIgnore
public Msdyn_analysiscomponentCollectionRequest getUser_msdyn_analysiscomponent() {
return new Msdyn_analysiscomponentCollectionRequest(
contextPath.addSegment("user_msdyn_analysiscomponent"), RequestHelper.getValue(unmappedFields, "user_msdyn_analysiscomponent"));
}
@NavigationProperty(name="lk_msdyn_analysisjob_createdby")
@JsonIgnore
public Msdyn_analysisjobCollectionRequest getLk_msdyn_analysisjob_createdby() {
return new Msdyn_analysisjobCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisjob_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisjob_createdby"));
}
@NavigationProperty(name="lk_msdyn_analysisjob_createdonbehalfby")
@JsonIgnore
public Msdyn_analysisjobCollectionRequest getLk_msdyn_analysisjob_createdonbehalfby() {
return new Msdyn_analysisjobCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisjob_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisjob_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_analysisjob_modifiedby")
@JsonIgnore
public Msdyn_analysisjobCollectionRequest getLk_msdyn_analysisjob_modifiedby() {
return new Msdyn_analysisjobCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisjob_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisjob_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_analysisjob_modifiedonbehalfby")
@JsonIgnore
public Msdyn_analysisjobCollectionRequest getLk_msdyn_analysisjob_modifiedonbehalfby() {
return new Msdyn_analysisjobCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisjob_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisjob_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_analysisjob")
@JsonIgnore
public Msdyn_analysisjobCollectionRequest getUser_msdyn_analysisjob() {
return new Msdyn_analysisjobCollectionRequest(
contextPath.addSegment("user_msdyn_analysisjob"), RequestHelper.getValue(unmappedFields, "user_msdyn_analysisjob"));
}
@NavigationProperty(name="lk_msdyn_analysisresult_createdby")
@JsonIgnore
public Msdyn_analysisresultCollectionRequest getLk_msdyn_analysisresult_createdby() {
return new Msdyn_analysisresultCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresult_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresult_createdby"));
}
@NavigationProperty(name="lk_msdyn_analysisresult_createdonbehalfby")
@JsonIgnore
public Msdyn_analysisresultCollectionRequest getLk_msdyn_analysisresult_createdonbehalfby() {
return new Msdyn_analysisresultCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresult_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresult_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_analysisresult_modifiedby")
@JsonIgnore
public Msdyn_analysisresultCollectionRequest getLk_msdyn_analysisresult_modifiedby() {
return new Msdyn_analysisresultCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresult_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresult_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_analysisresult_modifiedonbehalfby")
@JsonIgnore
public Msdyn_analysisresultCollectionRequest getLk_msdyn_analysisresult_modifiedonbehalfby() {
return new Msdyn_analysisresultCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresult_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresult_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_analysisresult")
@JsonIgnore
public Msdyn_analysisresultCollectionRequest getUser_msdyn_analysisresult() {
return new Msdyn_analysisresultCollectionRequest(
contextPath.addSegment("user_msdyn_analysisresult"), RequestHelper.getValue(unmappedFields, "user_msdyn_analysisresult"));
}
@NavigationProperty(name="lk_msdyn_analysisresultdetail_createdby")
@JsonIgnore
public Msdyn_analysisresultdetailCollectionRequest getLk_msdyn_analysisresultdetail_createdby() {
return new Msdyn_analysisresultdetailCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresultdetail_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresultdetail_createdby"));
}
@NavigationProperty(name="lk_msdyn_analysisresultdetail_createdonbehalfby")
@JsonIgnore
public Msdyn_analysisresultdetailCollectionRequest getLk_msdyn_analysisresultdetail_createdonbehalfby() {
return new Msdyn_analysisresultdetailCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresultdetail_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresultdetail_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_analysisresultdetail_modifiedby")
@JsonIgnore
public Msdyn_analysisresultdetailCollectionRequest getLk_msdyn_analysisresultdetail_modifiedby() {
return new Msdyn_analysisresultdetailCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresultdetail_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresultdetail_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_analysisresultdetail_modifiedonbehalfby")
@JsonIgnore
public Msdyn_analysisresultdetailCollectionRequest getLk_msdyn_analysisresultdetail_modifiedonbehalfby() {
return new Msdyn_analysisresultdetailCollectionRequest(
contextPath.addSegment("lk_msdyn_analysisresultdetail_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_analysisresultdetail_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_analysisresultdetail")
@JsonIgnore
public Msdyn_analysisresultdetailCollectionRequest getUser_msdyn_analysisresultdetail() {
return new Msdyn_analysisresultdetailCollectionRequest(
contextPath.addSegment("user_msdyn_analysisresultdetail"), RequestHelper.getValue(unmappedFields, "user_msdyn_analysisresultdetail"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthrule_createdby")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getLk_msdyn_solutionhealthrule_createdby() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthrule_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthrule_createdby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthrule_createdonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getLk_msdyn_solutionhealthrule_createdonbehalfby() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthrule_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthrule_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthrule_modifiedby")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getLk_msdyn_solutionhealthrule_modifiedby() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthrule_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthrule_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthrule_modifiedonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getLk_msdyn_solutionhealthrule_modifiedonbehalfby() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthrule_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthrule_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_solutionhealthrule")
@JsonIgnore
public Msdyn_solutionhealthruleCollectionRequest getUser_msdyn_solutionhealthrule() {
return new Msdyn_solutionhealthruleCollectionRequest(
contextPath.addSegment("user_msdyn_solutionhealthrule"), RequestHelper.getValue(unmappedFields, "user_msdyn_solutionhealthrule"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleargument_createdby")
@JsonIgnore
public Msdyn_solutionhealthruleargumentCollectionRequest getLk_msdyn_solutionhealthruleargument_createdby() {
return new Msdyn_solutionhealthruleargumentCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleargument_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleargument_createdby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleargument_createdonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthruleargumentCollectionRequest getLk_msdyn_solutionhealthruleargument_createdonbehalfby() {
return new Msdyn_solutionhealthruleargumentCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleargument_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleargument_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleargument_modifiedby")
@JsonIgnore
public Msdyn_solutionhealthruleargumentCollectionRequest getLk_msdyn_solutionhealthruleargument_modifiedby() {
return new Msdyn_solutionhealthruleargumentCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleargument_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleargument_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleargument_modifiedonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthruleargumentCollectionRequest getLk_msdyn_solutionhealthruleargument_modifiedonbehalfby() {
return new Msdyn_solutionhealthruleargumentCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleargument_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleargument_modifiedonbehalfby"));
}
@NavigationProperty(name="user_msdyn_solutionhealthruleargument")
@JsonIgnore
public Msdyn_solutionhealthruleargumentCollectionRequest getUser_msdyn_solutionhealthruleargument() {
return new Msdyn_solutionhealthruleargumentCollectionRequest(
contextPath.addSegment("user_msdyn_solutionhealthruleargument"), RequestHelper.getValue(unmappedFields, "user_msdyn_solutionhealthruleargument"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleset_createdby")
@JsonIgnore
public Msdyn_solutionhealthrulesetCollectionRequest getLk_msdyn_solutionhealthruleset_createdby() {
return new Msdyn_solutionhealthrulesetCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleset_createdby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleset_createdby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleset_createdonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthrulesetCollectionRequest getLk_msdyn_solutionhealthruleset_createdonbehalfby() {
return new Msdyn_solutionhealthrulesetCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleset_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleset_createdonbehalfby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleset_modifiedby")
@JsonIgnore
public Msdyn_solutionhealthrulesetCollectionRequest getLk_msdyn_solutionhealthruleset_modifiedby() {
return new Msdyn_solutionhealthrulesetCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleset_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleset_modifiedby"));
}
@NavigationProperty(name="lk_msdyn_solutionhealthruleset_modifiedonbehalfby")
@JsonIgnore
public Msdyn_solutionhealthrulesetCollectionRequest getLk_msdyn_solutionhealthruleset_modifiedonbehalfby() {
return new Msdyn_solutionhealthrulesetCollectionRequest(
contextPath.addSegment("lk_msdyn_solutionhealthruleset_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_msdyn_solutionhealthruleset_modifiedonbehalfby"));
}
@NavigationProperty(name="lk_ggw_event_createdby")
@JsonIgnore
public Ggw_eventCollectionRequest getLk_ggw_event_createdby() {
return new Ggw_eventCollectionRequest(
contextPath.addSegment("lk_ggw_event_createdby"), RequestHelper.getValue(unmappedFields, "lk_ggw_event_createdby"));
}
@NavigationProperty(name="lk_ggw_event_createdonbehalfby")
@JsonIgnore
public Ggw_eventCollectionRequest getLk_ggw_event_createdonbehalfby() {
return new Ggw_eventCollectionRequest(
contextPath.addSegment("lk_ggw_event_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_event_createdonbehalfby"));
}
@NavigationProperty(name="lk_ggw_event_modifiedby")
@JsonIgnore
public Ggw_eventCollectionRequest getLk_ggw_event_modifiedby() {
return new Ggw_eventCollectionRequest(
contextPath.addSegment("lk_ggw_event_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ggw_event_modifiedby"));
}
@NavigationProperty(name="lk_ggw_event_modifiedonbehalfby")
@JsonIgnore
public Ggw_eventCollectionRequest getLk_ggw_event_modifiedonbehalfby() {
return new Ggw_eventCollectionRequest(
contextPath.addSegment("lk_ggw_event_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_event_modifiedonbehalfby"));
}
@NavigationProperty(name="user_ggw_event")
@JsonIgnore
public Ggw_eventCollectionRequest getUser_ggw_event() {
return new Ggw_eventCollectionRequest(
contextPath.addSegment("user_ggw_event"), RequestHelper.getValue(unmappedFields, "user_ggw_event"));
}
@NavigationProperty(name="lk_ggw_team_createdby")
@JsonIgnore
public Ggw_teamCollectionRequest getLk_ggw_team_createdby() {
return new Ggw_teamCollectionRequest(
contextPath.addSegment("lk_ggw_team_createdby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_createdby"));
}
@NavigationProperty(name="lk_ggw_team_createdonbehalfby")
@JsonIgnore
public Ggw_teamCollectionRequest getLk_ggw_team_createdonbehalfby() {
return new Ggw_teamCollectionRequest(
contextPath.addSegment("lk_ggw_team_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_createdonbehalfby"));
}
@NavigationProperty(name="lk_ggw_team_modifiedby")
@JsonIgnore
public Ggw_teamCollectionRequest getLk_ggw_team_modifiedby() {
return new Ggw_teamCollectionRequest(
contextPath.addSegment("lk_ggw_team_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_modifiedby"));
}
@NavigationProperty(name="lk_ggw_team_modifiedonbehalfby")
@JsonIgnore
public Ggw_teamCollectionRequest getLk_ggw_team_modifiedonbehalfby() {
return new Ggw_teamCollectionRequest(
contextPath.addSegment("lk_ggw_team_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_modifiedonbehalfby"));
}
@NavigationProperty(name="user_ggw_team")
@JsonIgnore
public Ggw_teamCollectionRequest getUser_ggw_team() {
return new Ggw_teamCollectionRequest(
contextPath.addSegment("user_ggw_team"), RequestHelper.getValue(unmappedFields, "user_ggw_team"));
}
@NavigationProperty(name="lk_ggw_crew_createdby")
@JsonIgnore
public Ggw_crewCollectionRequest getLk_ggw_crew_createdby() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("lk_ggw_crew_createdby"), RequestHelper.getValue(unmappedFields, "lk_ggw_crew_createdby"));
}
@NavigationProperty(name="lk_ggw_crew_createdonbehalfby")
@JsonIgnore
public Ggw_crewCollectionRequest getLk_ggw_crew_createdonbehalfby() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("lk_ggw_crew_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_crew_createdonbehalfby"));
}
@NavigationProperty(name="lk_ggw_crew_modifiedby")
@JsonIgnore
public Ggw_crewCollectionRequest getLk_ggw_crew_modifiedby() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("lk_ggw_crew_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ggw_crew_modifiedby"));
}
@NavigationProperty(name="lk_ggw_crew_modifiedonbehalfby")
@JsonIgnore
public Ggw_crewCollectionRequest getLk_ggw_crew_modifiedonbehalfby() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("lk_ggw_crew_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_crew_modifiedonbehalfby"));
}
@NavigationProperty(name="user_ggw_crew")
@JsonIgnore
public Ggw_crewCollectionRequest getUser_ggw_crew() {
return new Ggw_crewCollectionRequest(
contextPath.addSegment("user_ggw_crew"), RequestHelper.getValue(unmappedFields, "user_ggw_crew"));
}
@NavigationProperty(name="lk_ggw_team_application_createdby")
@JsonIgnore
public Ggw_team_applicationCollectionRequest getLk_ggw_team_application_createdby() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("lk_ggw_team_application_createdby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_application_createdby"));
}
@NavigationProperty(name="lk_ggw_team_application_createdonbehalfby")
@JsonIgnore
public Ggw_team_applicationCollectionRequest getLk_ggw_team_application_createdonbehalfby() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("lk_ggw_team_application_createdonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_application_createdonbehalfby"));
}
@NavigationProperty(name="lk_ggw_team_application_modifiedby")
@JsonIgnore
public Ggw_team_applicationCollectionRequest getLk_ggw_team_application_modifiedby() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("lk_ggw_team_application_modifiedby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_application_modifiedby"));
}
@NavigationProperty(name="lk_ggw_team_application_modifiedonbehalfby")
@JsonIgnore
public Ggw_team_applicationCollectionRequest getLk_ggw_team_application_modifiedonbehalfby() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("lk_ggw_team_application_modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "lk_ggw_team_application_modifiedonbehalfby"));
}
@NavigationProperty(name="user_ggw_team_application")
@JsonIgnore
public Ggw_team_applicationCollectionRequest getUser_ggw_team_application() {
return new Ggw_team_applicationCollectionRequest(
contextPath.addSegment("user_ggw_team_application"), RequestHelper.getValue(unmappedFields, "user_ggw_team_application"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Systemuser patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Systemuser _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Systemuser put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Systemuser _x = _copy();
_x.changedFields = null;
return _x;
}
private Systemuser _copy() {
Systemuser _x = new Systemuser();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.ownerid = ownerid;
_x.title = title;
_x.address1_fax = address1_fax;
_x.organizationid = organizationid;
_x.nickname = nickname;
_x.defaultodbfoldername = defaultodbfoldername;
_x.address1_stateorprovince = address1_stateorprovince;
_x._modifiedby_value = _modifiedby_value;
_x.applicationid = applicationid;
_x.address1_upszone = address1_upszone;
_x.photourl = photourl;
_x.address1_latitude = address1_latitude;
_x.address1_shippingmethodcode = address1_shippingmethodcode;
_x.versionnumber = versionnumber;
_x.address1_utcoffset = address1_utcoffset;
_x._createdonbehalfby_value = _createdonbehalfby_value;
_x.homephone = homephone;
_x.address2_latitude = address2_latitude;
_x.governmentid = governmentid;
_x._parentsystemuserid_value = _parentsystemuserid_value;
_x.salutation = salutation;
_x.address2_longitude = address2_longitude;
_x._createdby_value = _createdby_value;
_x.overriddencreatedon = overriddencreatedon;
_x.address1_telephone3 = address1_telephone3;
_x.mobilephone = mobilephone;
_x._queueid_value = _queueid_value;
_x.preferredaddresscode = preferredaddresscode;
_x.address2_city = address2_city;
_x.address1_addressid = address1_addressid;
_x.address1_name = address1_name;
_x.address2_stateorprovince = address2_stateorprovince;
_x.address2_line2 = address2_line2;
_x.userpuid = userpuid;
_x.firstname = firstname;
_x.passporthi = passporthi;
_x.address2_name = address2_name;
_x._territoryid_value = _territoryid_value;
_x.address2_shippingmethodcode = address2_shippingmethodcode;
_x.disabledreason = disabledreason;
_x.address1_postofficebox = address1_postofficebox;
_x.address1_composite = address1_composite;
_x.setupuser = setupuser;
_x.entityimage_timestamp = entityimage_timestamp;
_x.internalemailaddress = internalemailaddress;
_x.isemailaddressapprovedbyo365admin = isemailaddressapprovedbyo365admin;
_x.address1_county = address1_county;
_x._businessunitid_value = _businessunitid_value;
_x.address1_telephone1 = address1_telephone1;
_x.invitestatuscode = invitestatuscode;
_x.entityimageid = entityimageid;
_x.address2_line3 = address2_line3;
_x.userlicensetype = userlicensetype;
_x.incomingemaildeliverymethod = incomingemaildeliverymethod;
_x.skills = skills;
_x.outgoingemaildeliverymethod = outgoingemaildeliverymethod;
_x.address2_postalcode = address2_postalcode;
_x.passportlo = passportlo;
_x.issyncwithdirectory = issyncwithdirectory;
_x.importsequencenumber = importsequencenumber;
_x.modifiedon = modifiedon;
_x.sharepointemailaddress = sharepointemailaddress;
_x.yammeruserid = yammeruserid;
_x.address1_longitude = address1_longitude;
_x.defaultfilterspopulated = defaultfilterspopulated;
_x.stageid = stageid;
_x.isintegrationuser = isintegrationuser;
_x.personalemailaddress = personalemailaddress;
_x.utcconversiontimezonecode = utcconversiontimezonecode;
_x.address2_telephone2 = address2_telephone2;
_x.preferredemailcode = preferredemailcode;
_x.address2_composite = address2_composite;
_x.preferredphonecode = preferredphonecode;
_x._mobileofflineprofileid_value = _mobileofflineprofileid_value;
_x.windowsliveid = windowsliveid;
_x.address1_line1 = address1_line1;
_x.yomimiddlename = yomimiddlename;
_x.entityimage = entityimage;
_x.emailrouteraccessapproval = emailrouteraccessapproval;
_x._calendarid_value = _calendarid_value;
_x.address1_line3 = address1_line3;
_x.yomifirstname = yomifirstname;
_x.address2_country = address2_country;
_x.fullname = fullname;
_x.azureactivedirectoryobjectid = azureactivedirectoryobjectid;
_x.systemuserid = systemuserid;
_x.entityimage_url = entityimage_url;
_x.address1_line2 = address1_line2;
_x.address2_upszone = address2_upszone;
_x.address1_city = address1_city;
_x.address2_fax = address2_fax;
_x._positionid_value = _positionid_value;
_x.address2_line1 = address2_line1;
_x._transactioncurrencyid_value = _transactioncurrencyid_value;
_x.address2_telephone1 = address2_telephone1;
_x.middlename = middlename;
_x.isdisabled = isdisabled;
_x._defaultmailbox_value = _defaultmailbox_value;
_x.address1_postalcode = address1_postalcode;
_x.employeeid = employeeid;
_x.lastname = lastname;
_x.mobilealertemail = mobilealertemail;
_x.timezoneruleversionnumber = timezoneruleversionnumber;
_x.identityid = identityid;
_x.traversedpath = traversedpath;
_x.address2_county = address2_county;
_x.address1_addresstypecode = address1_addresstypecode;
_x.address2_telephone3 = address2_telephone3;
_x.yomilastname = yomilastname;
_x.displayinserviceviews = displayinserviceviews;
_x.yomifullname = yomifullname;
_x.address2_addresstypecode = address2_addresstypecode;
_x.createdon = createdon;
_x.accessmode = accessmode;
_x.yammeremailaddress = yammeremailaddress;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.exchangerate = exchangerate;
_x.jobtitle = jobtitle;
_x.address2_postofficebox = address2_postofficebox;
_x.caltype = caltype;
_x.address2_addressid = address2_addressid;
_x.processid = processid;
_x.applicationiduri = applicationiduri;
_x.address2_utcoffset = address2_utcoffset;
_x.islicensed = islicensed;
_x.address1_telephone2 = address1_telephone2;
_x.address1_country = address1_country;
_x.domainname = domainname;
return _x;
}
@Action(name = "AddUserToRecordTeam")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped addUserToRecordTeam(Crmbaseentity record, Teamtemplate teamTemplate) {
Preconditions.checkNotNull(record, "record cannot be null");
Preconditions.checkNotNull(teamTemplate, "teamTemplate cannot be null");
Map _parameters = ParameterMap
.put("Record", "Microsoft.Dynamics.CRM.crmbaseentity", record)
.put("TeamTemplate", "Microsoft.Dynamics.CRM.teamtemplate", teamTemplate)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.AddUserToRecordTeam"), AddUserToRecordTeamResponse.class, _parameters);
}
@Action(name = "InstantiateFilters")
@JsonIgnore
public ActionRequestNoReturn instantiateFilters(List templateCollection) {
Preconditions.checkNotNull(templateCollection, "templateCollection cannot be null");
Map _parameters = ParameterMap
.put("TemplateCollection", "Collection(Microsoft.Dynamics.CRM.savedquery)", templateCollection)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.InstantiateFilters"), _parameters);
}
@Action(name = "ReassignObjectsSystemUser")
@JsonIgnore
public ActionRequestNoReturn reassignObjectsSystemUser(Crmbaseentity reassignPrincipal) {
Preconditions.checkNotNull(reassignPrincipal, "reassignPrincipal cannot be null");
Map _parameters = ParameterMap
.put("ReassignPrincipal", "Microsoft.Dynamics.CRM.crmbaseentity", reassignPrincipal)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.ReassignObjectsSystemUser"), _parameters);
}
@Action(name = "RemoveUserFromRecordTeam")
@JsonIgnore
public ActionRequestReturningNonCollectionUnwrapped removeUserFromRecordTeam(Crmbaseentity record, Teamtemplate teamTemplate) {
Preconditions.checkNotNull(record, "record cannot be null");
Preconditions.checkNotNull(teamTemplate, "teamTemplate cannot be null");
Map _parameters = ParameterMap
.put("Record", "Microsoft.Dynamics.CRM.crmbaseentity", record)
.put("TeamTemplate", "Microsoft.Dynamics.CRM.teamtemplate", teamTemplate)
.build();
return new ActionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RemoveUserFromRecordTeam"), RemoveUserFromRecordTeamResponse.class, _parameters);
}
@Action(name = "SetBusinessSystemUser")
@JsonIgnore
public ActionRequestNoReturn setBusinessSystemUser(Businessunit businessUnit, Crmbaseentity reassignPrincipal) {
Preconditions.checkNotNull(businessUnit, "businessUnit cannot be null");
Preconditions.checkNotNull(reassignPrincipal, "reassignPrincipal cannot be null");
Map _parameters = ParameterMap
.put("BusinessUnit", "Microsoft.Dynamics.CRM.businessunit", businessUnit)
.put("ReassignPrincipal", "Microsoft.Dynamics.CRM.crmbaseentity", reassignPrincipal)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.SetBusinessSystemUser"), _parameters);
}
@Action(name = "SetParentSystemUser")
@JsonIgnore
public ActionRequestNoReturn setParentSystemUser(Systemuser parent, Boolean keepChildUsers) {
Preconditions.checkNotNull(parent, "parent cannot be null");
Preconditions.checkNotNull(keepChildUsers, "keepChildUsers cannot be null");
Map _parameters = ParameterMap
.put("Parent", "Microsoft.Dynamics.CRM.systemuser", parent)
.put("KeepChildUsers", "Edm.Boolean", keepChildUsers)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.SetParentSystemUser"), _parameters);
}
@Function(name = "RetrieveAllChildUsersSystemUser")
@JsonIgnore
public CollectionPageNonEntityRequest retrieveAllChildUsersSystemUser() {
Map _parameters = ParameterMap.empty();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveAllChildUsersSystemUser"), Systemuser.class, _parameters);
}
@Function(name = "RetrievePrincipalAccess")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrievePrincipalAccess(Crmbaseentity target) {
Preconditions.checkNotNull(target, "target cannot be null");
Map _parameters = ParameterMap
.put("Target", "Microsoft.Dynamics.CRM.crmbaseentity", target)
.build();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrievePrincipalAccess"), RetrievePrincipalAccessResponse.class, _parameters);
}
@Function(name = "RetrievePrincipalAccessInfo")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrievePrincipalAccessInfo(UUID objectId, String entityName) {
Preconditions.checkNotNull(objectId, "objectId cannot be null");
Preconditions.checkNotNull(entityName, "entityName cannot be null");
Map _parameters = ParameterMap
.put("ObjectId", "Edm.Guid", objectId)
.put("EntityName", "Edm.String", Checks.checkIsAscii(entityName))
.build();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrievePrincipalAccessInfo"), RetrievePrincipalAccessInfoResponse.class, _parameters);
}
@Function(name = "RetrievePrincipalAttributePrivileges")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrievePrincipalAttributePrivileges() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrievePrincipalAttributePrivileges"), RetrievePrincipalAttributePrivilegesResponse.class, _parameters);
}
@Function(name = "RetrievePrincipalSyncAttributeMappings")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrievePrincipalSyncAttributeMappings() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrievePrincipalSyncAttributeMappings"), RetrievePrincipalSyncAttributeMappingsResponse.class, _parameters);
}
@Function(name = "RetrieveUserLicenseInfo")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUserLicenseInfo() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUserLicenseInfo"), RetrieveUserLicenseInfoResponse.class, _parameters);
}
@Function(name = "RetrieveUserPrivilegeByPrivilegeId")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUserPrivilegeByPrivilegeId(UUID privilegeId) {
Preconditions.checkNotNull(privilegeId, "privilegeId cannot be null");
Map _parameters = ParameterMap
.put("PrivilegeId", "Edm.Guid", privilegeId)
.build();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUserPrivilegeByPrivilegeId"), RetrieveUserPrivilegeByPrivilegeIdResponse.class, _parameters);
}
@Function(name = "RetrieveUserPrivilegeByPrivilegeName")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUserPrivilegeByPrivilegeName(String privilegeName) {
Preconditions.checkNotNull(privilegeName, "privilegeName cannot be null");
Map _parameters = ParameterMap
.put("PrivilegeName", "Edm.String", Checks.checkIsAscii(privilegeName))
.build();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUserPrivilegeByPrivilegeName"), RetrieveUserPrivilegeByPrivilegeNameResponse.class, _parameters);
}
@Function(name = "RetrieveUserPrivileges")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUserPrivileges() {
Map _parameters = ParameterMap.empty();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUserPrivileges"), RetrieveUserPrivilegesResponse.class, _parameters);
}
@Function(name = "RetrieveUserQueues")
@JsonIgnore
public CollectionPageNonEntityRequest retrieveUserQueues(Boolean includePublic) {
Preconditions.checkNotNull(includePublic, "includePublic cannot be null");
Map _parameters = ParameterMap
.put("IncludePublic", "Edm.Boolean", includePublic)
.build();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUserQueues"), Queue.class, _parameters);
}
@Function(name = "RetrieveUsersPrivilegesThroughTeams")
@JsonIgnore
public FunctionRequestReturningNonCollectionUnwrapped retrieveUsersPrivilegesThroughTeams(Boolean excludeOrgDisabledPrivileges, Boolean includeSetupUserFiltering) {
Preconditions.checkNotNull(excludeOrgDisabledPrivileges, "excludeOrgDisabledPrivileges cannot be null");
Preconditions.checkNotNull(includeSetupUserFiltering, "includeSetupUserFiltering cannot be null");
Map _parameters = ParameterMap
.put("ExcludeOrgDisabledPrivileges", "Edm.Boolean", excludeOrgDisabledPrivileges)
.put("IncludeSetupUserFiltering", "Edm.Boolean", includeSetupUserFiltering)
.build();
return new FunctionRequestReturningNonCollectionUnwrapped(this.contextPath.addActionOrFunctionSegment("Microsoft.Dynamics.CRM.RetrieveUsersPrivilegesThroughTeams"), RetrieveUsersPrivilegesThroughTeamsResponse.class, _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Systemuser[");
b.append("ownerid=");
b.append(this.ownerid);
b.append(", ");
b.append("title=");
b.append(this.title);
b.append(", ");
b.append("address1_fax=");
b.append(this.address1_fax);
b.append(", ");
b.append("organizationid=");
b.append(this.organizationid);
b.append(", ");
b.append("nickname=");
b.append(this.nickname);
b.append(", ");
b.append("defaultodbfoldername=");
b.append(this.defaultodbfoldername);
b.append(", ");
b.append("address1_stateorprovince=");
b.append(this.address1_stateorprovince);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("applicationid=");
b.append(this.applicationid);
b.append(", ");
b.append("address1_upszone=");
b.append(this.address1_upszone);
b.append(", ");
b.append("photourl=");
b.append(this.photourl);
b.append(", ");
b.append("address1_latitude=");
b.append(this.address1_latitude);
b.append(", ");
b.append("address1_shippingmethodcode=");
b.append(this.address1_shippingmethodcode);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("address1_utcoffset=");
b.append(this.address1_utcoffset);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append(", ");
b.append("homephone=");
b.append(this.homephone);
b.append(", ");
b.append("address2_latitude=");
b.append(this.address2_latitude);
b.append(", ");
b.append("governmentid=");
b.append(this.governmentid);
b.append(", ");
b.append("_parentsystemuserid_value=");
b.append(this._parentsystemuserid_value);
b.append(", ");
b.append("salutation=");
b.append(this.salutation);
b.append(", ");
b.append("address2_longitude=");
b.append(this.address2_longitude);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("overriddencreatedon=");
b.append(this.overriddencreatedon);
b.append(", ");
b.append("address1_telephone3=");
b.append(this.address1_telephone3);
b.append(", ");
b.append("mobilephone=");
b.append(this.mobilephone);
b.append(", ");
b.append("_queueid_value=");
b.append(this._queueid_value);
b.append(", ");
b.append("preferredaddresscode=");
b.append(this.preferredaddresscode);
b.append(", ");
b.append("address2_city=");
b.append(this.address2_city);
b.append(", ");
b.append("address1_addressid=");
b.append(this.address1_addressid);
b.append(", ");
b.append("address1_name=");
b.append(this.address1_name);
b.append(", ");
b.append("address2_stateorprovince=");
b.append(this.address2_stateorprovince);
b.append(", ");
b.append("address2_line2=");
b.append(this.address2_line2);
b.append(", ");
b.append("userpuid=");
b.append(this.userpuid);
b.append(", ");
b.append("firstname=");
b.append(this.firstname);
b.append(", ");
b.append("passporthi=");
b.append(this.passporthi);
b.append(", ");
b.append("address2_name=");
b.append(this.address2_name);
b.append(", ");
b.append("_territoryid_value=");
b.append(this._territoryid_value);
b.append(", ");
b.append("address2_shippingmethodcode=");
b.append(this.address2_shippingmethodcode);
b.append(", ");
b.append("disabledreason=");
b.append(this.disabledreason);
b.append(", ");
b.append("address1_postofficebox=");
b.append(this.address1_postofficebox);
b.append(", ");
b.append("address1_composite=");
b.append(this.address1_composite);
b.append(", ");
b.append("setupuser=");
b.append(this.setupuser);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("internalemailaddress=");
b.append(this.internalemailaddress);
b.append(", ");
b.append("isemailaddressapprovedbyo365admin=");
b.append(this.isemailaddressapprovedbyo365admin);
b.append(", ");
b.append("address1_county=");
b.append(this.address1_county);
b.append(", ");
b.append("_businessunitid_value=");
b.append(this._businessunitid_value);
b.append(", ");
b.append("address1_telephone1=");
b.append(this.address1_telephone1);
b.append(", ");
b.append("invitestatuscode=");
b.append(this.invitestatuscode);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("address2_line3=");
b.append(this.address2_line3);
b.append(", ");
b.append("userlicensetype=");
b.append(this.userlicensetype);
b.append(", ");
b.append("incomingemaildeliverymethod=");
b.append(this.incomingemaildeliverymethod);
b.append(", ");
b.append("skills=");
b.append(this.skills);
b.append(", ");
b.append("outgoingemaildeliverymethod=");
b.append(this.outgoingemaildeliverymethod);
b.append(", ");
b.append("address2_postalcode=");
b.append(this.address2_postalcode);
b.append(", ");
b.append("passportlo=");
b.append(this.passportlo);
b.append(", ");
b.append("issyncwithdirectory=");
b.append(this.issyncwithdirectory);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("sharepointemailaddress=");
b.append(this.sharepointemailaddress);
b.append(", ");
b.append("yammeruserid=");
b.append(this.yammeruserid);
b.append(", ");
b.append("address1_longitude=");
b.append(this.address1_longitude);
b.append(", ");
b.append("defaultfilterspopulated=");
b.append(this.defaultfilterspopulated);
b.append(", ");
b.append("stageid=");
b.append(this.stageid);
b.append(", ");
b.append("isintegrationuser=");
b.append(this.isintegrationuser);
b.append(", ");
b.append("personalemailaddress=");
b.append(this.personalemailaddress);
b.append(", ");
b.append("utcconversiontimezonecode=");
b.append(this.utcconversiontimezonecode);
b.append(", ");
b.append("address2_telephone2=");
b.append(this.address2_telephone2);
b.append(", ");
b.append("preferredemailcode=");
b.append(this.preferredemailcode);
b.append(", ");
b.append("address2_composite=");
b.append(this.address2_composite);
b.append(", ");
b.append("preferredphonecode=");
b.append(this.preferredphonecode);
b.append(", ");
b.append("_mobileofflineprofileid_value=");
b.append(this._mobileofflineprofileid_value);
b.append(", ");
b.append("windowsliveid=");
b.append(this.windowsliveid);
b.append(", ");
b.append("address1_line1=");
b.append(this.address1_line1);
b.append(", ");
b.append("yomimiddlename=");
b.append(this.yomimiddlename);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("emailrouteraccessapproval=");
b.append(this.emailrouteraccessapproval);
b.append(", ");
b.append("_calendarid_value=");
b.append(this._calendarid_value);
b.append(", ");
b.append("address1_line3=");
b.append(this.address1_line3);
b.append(", ");
b.append("yomifirstname=");
b.append(this.yomifirstname);
b.append(", ");
b.append("address2_country=");
b.append(this.address2_country);
b.append(", ");
b.append("fullname=");
b.append(this.fullname);
b.append(", ");
b.append("azureactivedirectoryobjectid=");
b.append(this.azureactivedirectoryobjectid);
b.append(", ");
b.append("systemuserid=");
b.append(this.systemuserid);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("address1_line2=");
b.append(this.address1_line2);
b.append(", ");
b.append("address2_upszone=");
b.append(this.address2_upszone);
b.append(", ");
b.append("address1_city=");
b.append(this.address1_city);
b.append(", ");
b.append("address2_fax=");
b.append(this.address2_fax);
b.append(", ");
b.append("_positionid_value=");
b.append(this._positionid_value);
b.append(", ");
b.append("address2_line1=");
b.append(this.address2_line1);
b.append(", ");
b.append("_transactioncurrencyid_value=");
b.append(this._transactioncurrencyid_value);
b.append(", ");
b.append("address2_telephone1=");
b.append(this.address2_telephone1);
b.append(", ");
b.append("middlename=");
b.append(this.middlename);
b.append(", ");
b.append("isdisabled=");
b.append(this.isdisabled);
b.append(", ");
b.append("_defaultmailbox_value=");
b.append(this._defaultmailbox_value);
b.append(", ");
b.append("address1_postalcode=");
b.append(this.address1_postalcode);
b.append(", ");
b.append("employeeid=");
b.append(this.employeeid);
b.append(", ");
b.append("lastname=");
b.append(this.lastname);
b.append(", ");
b.append("mobilealertemail=");
b.append(this.mobilealertemail);
b.append(", ");
b.append("timezoneruleversionnumber=");
b.append(this.timezoneruleversionnumber);
b.append(", ");
b.append("identityid=");
b.append(this.identityid);
b.append(", ");
b.append("traversedpath=");
b.append(this.traversedpath);
b.append(", ");
b.append("address2_county=");
b.append(this.address2_county);
b.append(", ");
b.append("address1_addresstypecode=");
b.append(this.address1_addresstypecode);
b.append(", ");
b.append("address2_telephone3=");
b.append(this.address2_telephone3);
b.append(", ");
b.append("yomilastname=");
b.append(this.yomilastname);
b.append(", ");
b.append("displayinserviceviews=");
b.append(this.displayinserviceviews);
b.append(", ");
b.append("yomifullname=");
b.append(this.yomifullname);
b.append(", ");
b.append("address2_addresstypecode=");
b.append(this.address2_addresstypecode);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("accessmode=");
b.append(this.accessmode);
b.append(", ");
b.append("yammeremailaddress=");
b.append(this.yammeremailaddress);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("exchangerate=");
b.append(this.exchangerate);
b.append(", ");
b.append("jobtitle=");
b.append(this.jobtitle);
b.append(", ");
b.append("address2_postofficebox=");
b.append(this.address2_postofficebox);
b.append(", ");
b.append("caltype=");
b.append(this.caltype);
b.append(", ");
b.append("address2_addressid=");
b.append(this.address2_addressid);
b.append(", ");
b.append("processid=");
b.append(this.processid);
b.append(", ");
b.append("applicationiduri=");
b.append(this.applicationiduri);
b.append(", ");
b.append("address2_utcoffset=");
b.append(this.address2_utcoffset);
b.append(", ");
b.append("islicensed=");
b.append(this.islicensed);
b.append(", ");
b.append("address1_telephone2=");
b.append(this.address1_telephone2);
b.append(", ");
b.append("address1_country=");
b.append(this.address1_country);
b.append(", ");
b.append("domainname=");
b.append(this.domainname);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy