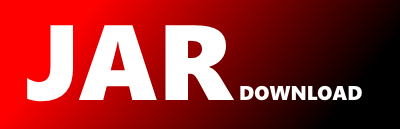
microsoft.dynamics.crm.entity.Template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.complex.BooleanManagedProperty;
import microsoft.dynamics.crm.entity.collection.request.ActivitymimeattachmentCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.AsyncoperationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.BulkdeletefailureCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.EmailCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.OrganizationCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.ProcesssessionCollectionRequest;
import microsoft.dynamics.crm.entity.collection.request.SyncerrorCollectionRequest;
import microsoft.dynamics.crm.entity.request.BusinessunitRequest;
import microsoft.dynamics.crm.entity.request.PrincipalRequest;
import microsoft.dynamics.crm.entity.request.SystemuserRequest;
import microsoft.dynamics.crm.entity.request.TeamRequest;
@JsonPropertyOrder({
"@odata.type",
"openrate",
"replyrate",
"subjectpresentationxml",
"generationtypecode",
"_createdby_value",
"mimetype",
"modifiedon",
"ismanaged",
"safehtml",
"_owningbusinessunit_value",
"_ownerid_value",
"description",
"templateidunique",
"entityimage_url",
"body",
"solutionid",
"_modifiedby_value",
"_owningteam_value",
"templatetypecode",
"ispersonal",
"usedcount",
"componentstate",
"entityimageid",
"entityimage_timestamp",
"title",
"importsequencenumber",
"_owninguser_value",
"overwritetime",
"_modifiedonbehalfby_value",
"isrecommended",
"introducedversion",
"createdon",
"templateid",
"replycount",
"presentationxml",
"iscustomizable",
"subject",
"entityimage",
"subjectsafehtml",
"languagecode",
"versionnumber",
"opencount",
"_createdonbehalfby_value"})
@JsonInclude(Include.NON_NULL)
public class Template extends Crmbaseentity implements ODataEntityType {
@Override
public String odataTypeName() {
return "Microsoft.Dynamics.CRM.template";
}
@JsonProperty("openrate")
protected Integer openrate;
@JsonProperty("replyrate")
protected Integer replyrate;
@JsonProperty("subjectpresentationxml")
protected String subjectpresentationxml;
@JsonProperty("generationtypecode")
protected Integer generationtypecode;
@JsonProperty("_createdby_value")
protected UUID _createdby_value;
@JsonProperty("mimetype")
protected String mimetype;
@JsonProperty("modifiedon")
protected OffsetDateTime modifiedon;
@JsonProperty("ismanaged")
protected Boolean ismanaged;
@JsonProperty("safehtml")
protected String safehtml;
@JsonProperty("_owningbusinessunit_value")
protected UUID _owningbusinessunit_value;
@JsonProperty("_ownerid_value")
protected UUID _ownerid_value;
@JsonProperty("description")
protected String description;
@JsonProperty("templateidunique")
protected UUID templateidunique;
@JsonProperty("entityimage_url")
protected String entityimage_url;
@JsonProperty("body")
protected String body;
@JsonProperty("solutionid")
protected UUID solutionid;
@JsonProperty("_modifiedby_value")
protected UUID _modifiedby_value;
@JsonProperty("_owningteam_value")
protected UUID _owningteam_value;
@JsonProperty("templatetypecode")
protected String templatetypecode;
@JsonProperty("ispersonal")
protected Boolean ispersonal;
@JsonProperty("usedcount")
protected Integer usedcount;
@JsonProperty("componentstate")
protected Integer componentstate;
@JsonProperty("entityimageid")
protected UUID entityimageid;
@JsonProperty("entityimage_timestamp")
protected Long entityimage_timestamp;
@JsonProperty("title")
protected String title;
@JsonProperty("importsequencenumber")
protected Integer importsequencenumber;
@JsonProperty("_owninguser_value")
protected UUID _owninguser_value;
@JsonProperty("overwritetime")
protected OffsetDateTime overwritetime;
@JsonProperty("_modifiedonbehalfby_value")
protected UUID _modifiedonbehalfby_value;
@JsonProperty("isrecommended")
protected Boolean isrecommended;
@JsonProperty("introducedversion")
protected String introducedversion;
@JsonProperty("createdon")
protected OffsetDateTime createdon;
@JsonProperty("templateid")
protected UUID templateid;
@JsonProperty("replycount")
protected Integer replycount;
@JsonProperty("presentationxml")
protected String presentationxml;
@JsonProperty("iscustomizable")
protected BooleanManagedProperty iscustomizable;
@JsonProperty("subject")
protected String subject;
@JsonProperty("entityimage")
protected byte[] entityimage;
@JsonProperty("subjectsafehtml")
protected String subjectsafehtml;
@JsonProperty("languagecode")
protected Integer languagecode;
@JsonProperty("versionnumber")
protected Long versionnumber;
@JsonProperty("opencount")
protected Integer opencount;
@JsonProperty("_createdonbehalfby_value")
protected UUID _createdonbehalfby_value;
protected Template() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderTemplate() {
return new Builder();
}
public static final class Builder {
private Integer openrate;
private Integer replyrate;
private String subjectpresentationxml;
private Integer generationtypecode;
private UUID _createdby_value;
private String mimetype;
private OffsetDateTime modifiedon;
private Boolean ismanaged;
private String safehtml;
private UUID _owningbusinessunit_value;
private UUID _ownerid_value;
private String description;
private UUID templateidunique;
private String entityimage_url;
private String body;
private UUID solutionid;
private UUID _modifiedby_value;
private UUID _owningteam_value;
private String templatetypecode;
private Boolean ispersonal;
private Integer usedcount;
private Integer componentstate;
private UUID entityimageid;
private Long entityimage_timestamp;
private String title;
private Integer importsequencenumber;
private UUID _owninguser_value;
private OffsetDateTime overwritetime;
private UUID _modifiedonbehalfby_value;
private Boolean isrecommended;
private String introducedversion;
private OffsetDateTime createdon;
private UUID templateid;
private Integer replycount;
private String presentationxml;
private BooleanManagedProperty iscustomizable;
private String subject;
private byte[] entityimage;
private String subjectsafehtml;
private Integer languagecode;
private Long versionnumber;
private Integer opencount;
private UUID _createdonbehalfby_value;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder openrate(Integer openrate) {
this.openrate = openrate;
this.changedFields = changedFields.add("openrate");
return this;
}
public Builder replyrate(Integer replyrate) {
this.replyrate = replyrate;
this.changedFields = changedFields.add("replyrate");
return this;
}
public Builder subjectpresentationxml(String subjectpresentationxml) {
this.subjectpresentationxml = subjectpresentationxml;
this.changedFields = changedFields.add("subjectpresentationxml");
return this;
}
public Builder generationtypecode(Integer generationtypecode) {
this.generationtypecode = generationtypecode;
this.changedFields = changedFields.add("generationtypecode");
return this;
}
public Builder _createdby_value(UUID _createdby_value) {
this._createdby_value = _createdby_value;
this.changedFields = changedFields.add("_createdby_value");
return this;
}
public Builder mimetype(String mimetype) {
this.mimetype = mimetype;
this.changedFields = changedFields.add("mimetype");
return this;
}
public Builder modifiedon(OffsetDateTime modifiedon) {
this.modifiedon = modifiedon;
this.changedFields = changedFields.add("modifiedon");
return this;
}
public Builder ismanaged(Boolean ismanaged) {
this.ismanaged = ismanaged;
this.changedFields = changedFields.add("ismanaged");
return this;
}
public Builder safehtml(String safehtml) {
this.safehtml = safehtml;
this.changedFields = changedFields.add("safehtml");
return this;
}
public Builder _owningbusinessunit_value(UUID _owningbusinessunit_value) {
this._owningbusinessunit_value = _owningbusinessunit_value;
this.changedFields = changedFields.add("_owningbusinessunit_value");
return this;
}
public Builder _ownerid_value(UUID _ownerid_value) {
this._ownerid_value = _ownerid_value;
this.changedFields = changedFields.add("_ownerid_value");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder templateidunique(UUID templateidunique) {
this.templateidunique = templateidunique;
this.changedFields = changedFields.add("templateidunique");
return this;
}
public Builder entityimage_url(String entityimage_url) {
this.entityimage_url = entityimage_url;
this.changedFields = changedFields.add("entityimage_url");
return this;
}
public Builder body(String body) {
this.body = body;
this.changedFields = changedFields.add("body");
return this;
}
public Builder solutionid(UUID solutionid) {
this.solutionid = solutionid;
this.changedFields = changedFields.add("solutionid");
return this;
}
public Builder _modifiedby_value(UUID _modifiedby_value) {
this._modifiedby_value = _modifiedby_value;
this.changedFields = changedFields.add("_modifiedby_value");
return this;
}
public Builder _owningteam_value(UUID _owningteam_value) {
this._owningteam_value = _owningteam_value;
this.changedFields = changedFields.add("_owningteam_value");
return this;
}
public Builder templatetypecode(String templatetypecode) {
this.templatetypecode = templatetypecode;
this.changedFields = changedFields.add("templatetypecode");
return this;
}
public Builder ispersonal(Boolean ispersonal) {
this.ispersonal = ispersonal;
this.changedFields = changedFields.add("ispersonal");
return this;
}
public Builder usedcount(Integer usedcount) {
this.usedcount = usedcount;
this.changedFields = changedFields.add("usedcount");
return this;
}
public Builder componentstate(Integer componentstate) {
this.componentstate = componentstate;
this.changedFields = changedFields.add("componentstate");
return this;
}
public Builder entityimageid(UUID entityimageid) {
this.entityimageid = entityimageid;
this.changedFields = changedFields.add("entityimageid");
return this;
}
public Builder entityimage_timestamp(Long entityimage_timestamp) {
this.entityimage_timestamp = entityimage_timestamp;
this.changedFields = changedFields.add("entityimage_timestamp");
return this;
}
public Builder title(String title) {
this.title = title;
this.changedFields = changedFields.add("title");
return this;
}
public Builder importsequencenumber(Integer importsequencenumber) {
this.importsequencenumber = importsequencenumber;
this.changedFields = changedFields.add("importsequencenumber");
return this;
}
public Builder _owninguser_value(UUID _owninguser_value) {
this._owninguser_value = _owninguser_value;
this.changedFields = changedFields.add("_owninguser_value");
return this;
}
public Builder overwritetime(OffsetDateTime overwritetime) {
this.overwritetime = overwritetime;
this.changedFields = changedFields.add("overwritetime");
return this;
}
public Builder _modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
this._modifiedonbehalfby_value = _modifiedonbehalfby_value;
this.changedFields = changedFields.add("_modifiedonbehalfby_value");
return this;
}
public Builder isrecommended(Boolean isrecommended) {
this.isrecommended = isrecommended;
this.changedFields = changedFields.add("isrecommended");
return this;
}
public Builder introducedversion(String introducedversion) {
this.introducedversion = introducedversion;
this.changedFields = changedFields.add("introducedversion");
return this;
}
public Builder createdon(OffsetDateTime createdon) {
this.createdon = createdon;
this.changedFields = changedFields.add("createdon");
return this;
}
public Builder templateid(UUID templateid) {
this.templateid = templateid;
this.changedFields = changedFields.add("templateid");
return this;
}
public Builder replycount(Integer replycount) {
this.replycount = replycount;
this.changedFields = changedFields.add("replycount");
return this;
}
public Builder presentationxml(String presentationxml) {
this.presentationxml = presentationxml;
this.changedFields = changedFields.add("presentationxml");
return this;
}
public Builder iscustomizable(BooleanManagedProperty iscustomizable) {
this.iscustomizable = iscustomizable;
this.changedFields = changedFields.add("iscustomizable");
return this;
}
public Builder subject(String subject) {
this.subject = subject;
this.changedFields = changedFields.add("subject");
return this;
}
public Builder entityimage(byte[] entityimage) {
this.entityimage = entityimage;
this.changedFields = changedFields.add("entityimage");
return this;
}
public Builder subjectsafehtml(String subjectsafehtml) {
this.subjectsafehtml = subjectsafehtml;
this.changedFields = changedFields.add("subjectsafehtml");
return this;
}
public Builder languagecode(Integer languagecode) {
this.languagecode = languagecode;
this.changedFields = changedFields.add("languagecode");
return this;
}
public Builder versionnumber(Long versionnumber) {
this.versionnumber = versionnumber;
this.changedFields = changedFields.add("versionnumber");
return this;
}
public Builder opencount(Integer opencount) {
this.opencount = opencount;
this.changedFields = changedFields.add("opencount");
return this;
}
public Builder _createdonbehalfby_value(UUID _createdonbehalfby_value) {
this._createdonbehalfby_value = _createdonbehalfby_value;
this.changedFields = changedFields.add("_createdonbehalfby_value");
return this;
}
public Template build() {
Template _x = new Template();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "Microsoft.Dynamics.CRM.template";
_x.openrate = openrate;
_x.replyrate = replyrate;
_x.subjectpresentationxml = subjectpresentationxml;
_x.generationtypecode = generationtypecode;
_x._createdby_value = _createdby_value;
_x.mimetype = mimetype;
_x.modifiedon = modifiedon;
_x.ismanaged = ismanaged;
_x.safehtml = safehtml;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._ownerid_value = _ownerid_value;
_x.description = description;
_x.templateidunique = templateidunique;
_x.entityimage_url = entityimage_url;
_x.body = body;
_x.solutionid = solutionid;
_x._modifiedby_value = _modifiedby_value;
_x._owningteam_value = _owningteam_value;
_x.templatetypecode = templatetypecode;
_x.ispersonal = ispersonal;
_x.usedcount = usedcount;
_x.componentstate = componentstate;
_x.entityimageid = entityimageid;
_x.entityimage_timestamp = entityimage_timestamp;
_x.title = title;
_x.importsequencenumber = importsequencenumber;
_x._owninguser_value = _owninguser_value;
_x.overwritetime = overwritetime;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.isrecommended = isrecommended;
_x.introducedversion = introducedversion;
_x.createdon = createdon;
_x.templateid = templateid;
_x.replycount = replycount;
_x.presentationxml = presentationxml;
_x.iscustomizable = iscustomizable;
_x.subject = subject;
_x.entityimage = entityimage;
_x.subjectsafehtml = subjectsafehtml;
_x.languagecode = languagecode;
_x.versionnumber = versionnumber;
_x.opencount = opencount;
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && templateid != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(templateid, UUID.class));
}
}
@Property(name="openrate")
@JsonIgnore
public Optional getOpenrate() {
return Optional.ofNullable(openrate);
}
public Template withOpenrate(Integer openrate) {
Template _x = _copy();
_x.changedFields = changedFields.add("openrate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.openrate = openrate;
return _x;
}
@Property(name="replyrate")
@JsonIgnore
public Optional getReplyrate() {
return Optional.ofNullable(replyrate);
}
public Template withReplyrate(Integer replyrate) {
Template _x = _copy();
_x.changedFields = changedFields.add("replyrate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.replyrate = replyrate;
return _x;
}
@Property(name="subjectpresentationxml")
@JsonIgnore
public Optional getSubjectpresentationxml() {
return Optional.ofNullable(subjectpresentationxml);
}
public Template withSubjectpresentationxml(String subjectpresentationxml) {
Template _x = _copy();
_x.changedFields = changedFields.add("subjectpresentationxml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.subjectpresentationxml = subjectpresentationxml;
return _x;
}
@Property(name="generationtypecode")
@JsonIgnore
public Optional getGenerationtypecode() {
return Optional.ofNullable(generationtypecode);
}
public Template withGenerationtypecode(Integer generationtypecode) {
Template _x = _copy();
_x.changedFields = changedFields.add("generationtypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.generationtypecode = generationtypecode;
return _x;
}
@Property(name="_createdby_value")
@JsonIgnore
public Optional get_createdby_value() {
return Optional.ofNullable(_createdby_value);
}
public Template with_createdby_value(UUID _createdby_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_createdby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._createdby_value = _createdby_value;
return _x;
}
@Property(name="mimetype")
@JsonIgnore
public Optional getMimetype() {
return Optional.ofNullable(mimetype);
}
public Template withMimetype(String mimetype) {
Template _x = _copy();
_x.changedFields = changedFields.add("mimetype");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.mimetype = mimetype;
return _x;
}
@Property(name="modifiedon")
@JsonIgnore
public Optional getModifiedon() {
return Optional.ofNullable(modifiedon);
}
public Template withModifiedon(OffsetDateTime modifiedon) {
Template _x = _copy();
_x.changedFields = changedFields.add("modifiedon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.modifiedon = modifiedon;
return _x;
}
@Property(name="ismanaged")
@JsonIgnore
public Optional getIsmanaged() {
return Optional.ofNullable(ismanaged);
}
public Template withIsmanaged(Boolean ismanaged) {
Template _x = _copy();
_x.changedFields = changedFields.add("ismanaged");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.ismanaged = ismanaged;
return _x;
}
@Property(name="safehtml")
@JsonIgnore
public Optional getSafehtml() {
return Optional.ofNullable(safehtml);
}
public Template withSafehtml(String safehtml) {
Template _x = _copy();
_x.changedFields = changedFields.add("safehtml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.safehtml = safehtml;
return _x;
}
@Property(name="_owningbusinessunit_value")
@JsonIgnore
public Optional get_owningbusinessunit_value() {
return Optional.ofNullable(_owningbusinessunit_value);
}
public Template with_owningbusinessunit_value(UUID _owningbusinessunit_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_owningbusinessunit_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._owningbusinessunit_value = _owningbusinessunit_value;
return _x;
}
@Property(name="_ownerid_value")
@JsonIgnore
public Optional get_ownerid_value() {
return Optional.ofNullable(_ownerid_value);
}
public Template with_ownerid_value(UUID _ownerid_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_ownerid_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._ownerid_value = _ownerid_value;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Template withDescription(String description) {
Template _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.description = description;
return _x;
}
@Property(name="templateidunique")
@JsonIgnore
public Optional getTemplateidunique() {
return Optional.ofNullable(templateidunique);
}
public Template withTemplateidunique(UUID templateidunique) {
Template _x = _copy();
_x.changedFields = changedFields.add("templateidunique");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.templateidunique = templateidunique;
return _x;
}
@Property(name="entityimage_url")
@JsonIgnore
public Optional getEntityimage_url() {
return Optional.ofNullable(entityimage_url);
}
public Template withEntityimage_url(String entityimage_url) {
Template _x = _copy();
_x.changedFields = changedFields.add("entityimage_url");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.entityimage_url = entityimage_url;
return _x;
}
@Property(name="body")
@JsonIgnore
public Optional getBody() {
return Optional.ofNullable(body);
}
public Template withBody(String body) {
Template _x = _copy();
_x.changedFields = changedFields.add("body");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.body = body;
return _x;
}
@Property(name="solutionid")
@JsonIgnore
public Optional getSolutionid() {
return Optional.ofNullable(solutionid);
}
public Template withSolutionid(UUID solutionid) {
Template _x = _copy();
_x.changedFields = changedFields.add("solutionid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.solutionid = solutionid;
return _x;
}
@Property(name="_modifiedby_value")
@JsonIgnore
public Optional get_modifiedby_value() {
return Optional.ofNullable(_modifiedby_value);
}
public Template with_modifiedby_value(UUID _modifiedby_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_modifiedby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._modifiedby_value = _modifiedby_value;
return _x;
}
@Property(name="_owningteam_value")
@JsonIgnore
public Optional get_owningteam_value() {
return Optional.ofNullable(_owningteam_value);
}
public Template with_owningteam_value(UUID _owningteam_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_owningteam_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._owningteam_value = _owningteam_value;
return _x;
}
@Property(name="templatetypecode")
@JsonIgnore
public Optional getTemplatetypecode() {
return Optional.ofNullable(templatetypecode);
}
public Template withTemplatetypecode(String templatetypecode) {
Template _x = _copy();
_x.changedFields = changedFields.add("templatetypecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.templatetypecode = templatetypecode;
return _x;
}
@Property(name="ispersonal")
@JsonIgnore
public Optional getIspersonal() {
return Optional.ofNullable(ispersonal);
}
public Template withIspersonal(Boolean ispersonal) {
Template _x = _copy();
_x.changedFields = changedFields.add("ispersonal");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.ispersonal = ispersonal;
return _x;
}
@Property(name="usedcount")
@JsonIgnore
public Optional getUsedcount() {
return Optional.ofNullable(usedcount);
}
public Template withUsedcount(Integer usedcount) {
Template _x = _copy();
_x.changedFields = changedFields.add("usedcount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.usedcount = usedcount;
return _x;
}
@Property(name="componentstate")
@JsonIgnore
public Optional getComponentstate() {
return Optional.ofNullable(componentstate);
}
public Template withComponentstate(Integer componentstate) {
Template _x = _copy();
_x.changedFields = changedFields.add("componentstate");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.componentstate = componentstate;
return _x;
}
@Property(name="entityimageid")
@JsonIgnore
public Optional getEntityimageid() {
return Optional.ofNullable(entityimageid);
}
public Template withEntityimageid(UUID entityimageid) {
Template _x = _copy();
_x.changedFields = changedFields.add("entityimageid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.entityimageid = entityimageid;
return _x;
}
@Property(name="entityimage_timestamp")
@JsonIgnore
public Optional getEntityimage_timestamp() {
return Optional.ofNullable(entityimage_timestamp);
}
public Template withEntityimage_timestamp(Long entityimage_timestamp) {
Template _x = _copy();
_x.changedFields = changedFields.add("entityimage_timestamp");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.entityimage_timestamp = entityimage_timestamp;
return _x;
}
@Property(name="title")
@JsonIgnore
public Optional getTitle() {
return Optional.ofNullable(title);
}
public Template withTitle(String title) {
Template _x = _copy();
_x.changedFields = changedFields.add("title");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.title = title;
return _x;
}
@Property(name="importsequencenumber")
@JsonIgnore
public Optional getImportsequencenumber() {
return Optional.ofNullable(importsequencenumber);
}
public Template withImportsequencenumber(Integer importsequencenumber) {
Template _x = _copy();
_x.changedFields = changedFields.add("importsequencenumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.importsequencenumber = importsequencenumber;
return _x;
}
@Property(name="_owninguser_value")
@JsonIgnore
public Optional get_owninguser_value() {
return Optional.ofNullable(_owninguser_value);
}
public Template with_owninguser_value(UUID _owninguser_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_owninguser_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._owninguser_value = _owninguser_value;
return _x;
}
@Property(name="overwritetime")
@JsonIgnore
public Optional getOverwritetime() {
return Optional.ofNullable(overwritetime);
}
public Template withOverwritetime(OffsetDateTime overwritetime) {
Template _x = _copy();
_x.changedFields = changedFields.add("overwritetime");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.overwritetime = overwritetime;
return _x;
}
@Property(name="_modifiedonbehalfby_value")
@JsonIgnore
public Optional get_modifiedonbehalfby_value() {
return Optional.ofNullable(_modifiedonbehalfby_value);
}
public Template with_modifiedonbehalfby_value(UUID _modifiedonbehalfby_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_modifiedonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
return _x;
}
@Property(name="isrecommended")
@JsonIgnore
public Optional getIsrecommended() {
return Optional.ofNullable(isrecommended);
}
public Template withIsrecommended(Boolean isrecommended) {
Template _x = _copy();
_x.changedFields = changedFields.add("isrecommended");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.isrecommended = isrecommended;
return _x;
}
@Property(name="introducedversion")
@JsonIgnore
public Optional getIntroducedversion() {
return Optional.ofNullable(introducedversion);
}
public Template withIntroducedversion(String introducedversion) {
Template _x = _copy();
_x.changedFields = changedFields.add("introducedversion");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.introducedversion = introducedversion;
return _x;
}
@Property(name="createdon")
@JsonIgnore
public Optional getCreatedon() {
return Optional.ofNullable(createdon);
}
public Template withCreatedon(OffsetDateTime createdon) {
Template _x = _copy();
_x.changedFields = changedFields.add("createdon");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.createdon = createdon;
return _x;
}
@Property(name="templateid")
@JsonIgnore
public Optional getTemplateid() {
return Optional.ofNullable(templateid);
}
public Template withTemplateid(UUID templateid) {
Template _x = _copy();
_x.changedFields = changedFields.add("templateid");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.templateid = templateid;
return _x;
}
@Property(name="replycount")
@JsonIgnore
public Optional getReplycount() {
return Optional.ofNullable(replycount);
}
public Template withReplycount(Integer replycount) {
Template _x = _copy();
_x.changedFields = changedFields.add("replycount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.replycount = replycount;
return _x;
}
@Property(name="presentationxml")
@JsonIgnore
public Optional getPresentationxml() {
return Optional.ofNullable(presentationxml);
}
public Template withPresentationxml(String presentationxml) {
Template _x = _copy();
_x.changedFields = changedFields.add("presentationxml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.presentationxml = presentationxml;
return _x;
}
@Property(name="iscustomizable")
@JsonIgnore
public Optional getIscustomizable() {
return Optional.ofNullable(iscustomizable);
}
public Template withIscustomizable(BooleanManagedProperty iscustomizable) {
Template _x = _copy();
_x.changedFields = changedFields.add("iscustomizable");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.iscustomizable = iscustomizable;
return _x;
}
@Property(name="subject")
@JsonIgnore
public Optional getSubject() {
return Optional.ofNullable(subject);
}
public Template withSubject(String subject) {
Template _x = _copy();
_x.changedFields = changedFields.add("subject");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.subject = subject;
return _x;
}
@Property(name="entityimage")
@JsonIgnore
public Optional getEntityimage() {
return Optional.ofNullable(entityimage);
}
public Template withEntityimage(byte[] entityimage) {
Template _x = _copy();
_x.changedFields = changedFields.add("entityimage");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.entityimage = entityimage;
return _x;
}
@Property(name="subjectsafehtml")
@JsonIgnore
public Optional getSubjectsafehtml() {
return Optional.ofNullable(subjectsafehtml);
}
public Template withSubjectsafehtml(String subjectsafehtml) {
Template _x = _copy();
_x.changedFields = changedFields.add("subjectsafehtml");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.subjectsafehtml = subjectsafehtml;
return _x;
}
@Property(name="languagecode")
@JsonIgnore
public Optional getLanguagecode() {
return Optional.ofNullable(languagecode);
}
public Template withLanguagecode(Integer languagecode) {
Template _x = _copy();
_x.changedFields = changedFields.add("languagecode");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.languagecode = languagecode;
return _x;
}
@Property(name="versionnumber")
@JsonIgnore
public Optional getVersionnumber() {
return Optional.ofNullable(versionnumber);
}
public Template withVersionnumber(Long versionnumber) {
Template _x = _copy();
_x.changedFields = changedFields.add("versionnumber");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.versionnumber = versionnumber;
return _x;
}
@Property(name="opencount")
@JsonIgnore
public Optional getOpencount() {
return Optional.ofNullable(opencount);
}
public Template withOpencount(Integer opencount) {
Template _x = _copy();
_x.changedFields = changedFields.add("opencount");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x.opencount = opencount;
return _x;
}
@Property(name="_createdonbehalfby_value")
@JsonIgnore
public Optional get_createdonbehalfby_value() {
return Optional.ofNullable(_createdonbehalfby_value);
}
public Template with_createdonbehalfby_value(UUID _createdonbehalfby_value) {
Template _x = _copy();
_x.changedFields = changedFields.add("_createdonbehalfby_value");
_x.odataType = Util.nvl(odataType, "Microsoft.Dynamics.CRM.template");
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
public Template withUnmappedField(String name, Object value) {
Template _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="createdby")
@JsonIgnore
public SystemuserRequest getCreatedby() {
return new SystemuserRequest(contextPath.addSegment("createdby"), RequestHelper.getValue(unmappedFields, "createdby"));
}
@NavigationProperty(name="owningbusinessunit")
@JsonIgnore
public BusinessunitRequest getOwningbusinessunit() {
return new BusinessunitRequest(contextPath.addSegment("owningbusinessunit"), RequestHelper.getValue(unmappedFields, "owningbusinessunit"));
}
@NavigationProperty(name="modifiedby")
@JsonIgnore
public SystemuserRequest getModifiedby() {
return new SystemuserRequest(contextPath.addSegment("modifiedby"), RequestHelper.getValue(unmappedFields, "modifiedby"));
}
@NavigationProperty(name="Template_AsyncOperations")
@JsonIgnore
public AsyncoperationCollectionRequest getTemplate_AsyncOperations() {
return new AsyncoperationCollectionRequest(
contextPath.addSegment("Template_AsyncOperations"), RequestHelper.getValue(unmappedFields, "Template_AsyncOperations"));
}
@NavigationProperty(name="Template_BulkDeleteFailures")
@JsonIgnore
public BulkdeletefailureCollectionRequest getTemplate_BulkDeleteFailures() {
return new BulkdeletefailureCollectionRequest(
contextPath.addSegment("Template_BulkDeleteFailures"), RequestHelper.getValue(unmappedFields, "Template_BulkDeleteFailures"));
}
@NavigationProperty(name="createdonbehalfby")
@JsonIgnore
public SystemuserRequest getCreatedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("createdonbehalfby"), RequestHelper.getValue(unmappedFields, "createdonbehalfby"));
}
@NavigationProperty(name="owningteam")
@JsonIgnore
public TeamRequest getOwningteam() {
return new TeamRequest(contextPath.addSegment("owningteam"), RequestHelper.getValue(unmappedFields, "owningteam"));
}
@NavigationProperty(name="template_activity_mime_attachments")
@JsonIgnore
public ActivitymimeattachmentCollectionRequest getTemplate_activity_mime_attachments() {
return new ActivitymimeattachmentCollectionRequest(
contextPath.addSegment("template_activity_mime_attachments"), RequestHelper.getValue(unmappedFields, "template_activity_mime_attachments"));
}
@NavigationProperty(name="Template_ProcessSessions")
@JsonIgnore
public ProcesssessionCollectionRequest getTemplate_ProcessSessions() {
return new ProcesssessionCollectionRequest(
contextPath.addSegment("Template_ProcessSessions"), RequestHelper.getValue(unmappedFields, "Template_ProcessSessions"));
}
@NavigationProperty(name="ownerid")
@JsonIgnore
public PrincipalRequest getOwnerid() {
return new PrincipalRequest(contextPath.addSegment("ownerid"), RequestHelper.getValue(unmappedFields, "ownerid"));
}
@NavigationProperty(name="Template_SyncErrors")
@JsonIgnore
public SyncerrorCollectionRequest getTemplate_SyncErrors() {
return new SyncerrorCollectionRequest(
contextPath.addSegment("Template_SyncErrors"), RequestHelper.getValue(unmappedFields, "Template_SyncErrors"));
}
@NavigationProperty(name="owninguser")
@JsonIgnore
public SystemuserRequest getOwninguser() {
return new SystemuserRequest(contextPath.addSegment("owninguser"), RequestHelper.getValue(unmappedFields, "owninguser"));
}
@NavigationProperty(name="Template_Organization")
@JsonIgnore
public OrganizationCollectionRequest getTemplate_Organization() {
return new OrganizationCollectionRequest(
contextPath.addSegment("Template_Organization"), RequestHelper.getValue(unmappedFields, "Template_Organization"));
}
@NavigationProperty(name="modifiedonbehalfby")
@JsonIgnore
public SystemuserRequest getModifiedonbehalfby() {
return new SystemuserRequest(contextPath.addSegment("modifiedonbehalfby"), RequestHelper.getValue(unmappedFields, "modifiedonbehalfby"));
}
@NavigationProperty(name="Email_EmailTemplate")
@JsonIgnore
public EmailCollectionRequest getEmail_EmailTemplate() {
return new EmailCollectionRequest(
contextPath.addSegment("Email_EmailTemplate"), RequestHelper.getValue(unmappedFields, "Email_EmailTemplate"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Template patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Template _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Template put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Template _x = _copy();
_x.changedFields = null;
return _x;
}
private Template _copy() {
Template _x = new Template();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.openrate = openrate;
_x.replyrate = replyrate;
_x.subjectpresentationxml = subjectpresentationxml;
_x.generationtypecode = generationtypecode;
_x._createdby_value = _createdby_value;
_x.mimetype = mimetype;
_x.modifiedon = modifiedon;
_x.ismanaged = ismanaged;
_x.safehtml = safehtml;
_x._owningbusinessunit_value = _owningbusinessunit_value;
_x._ownerid_value = _ownerid_value;
_x.description = description;
_x.templateidunique = templateidunique;
_x.entityimage_url = entityimage_url;
_x.body = body;
_x.solutionid = solutionid;
_x._modifiedby_value = _modifiedby_value;
_x._owningteam_value = _owningteam_value;
_x.templatetypecode = templatetypecode;
_x.ispersonal = ispersonal;
_x.usedcount = usedcount;
_x.componentstate = componentstate;
_x.entityimageid = entityimageid;
_x.entityimage_timestamp = entityimage_timestamp;
_x.title = title;
_x.importsequencenumber = importsequencenumber;
_x._owninguser_value = _owninguser_value;
_x.overwritetime = overwritetime;
_x._modifiedonbehalfby_value = _modifiedonbehalfby_value;
_x.isrecommended = isrecommended;
_x.introducedversion = introducedversion;
_x.createdon = createdon;
_x.templateid = templateid;
_x.replycount = replycount;
_x.presentationxml = presentationxml;
_x.iscustomizable = iscustomizable;
_x.subject = subject;
_x.entityimage = entityimage;
_x.subjectsafehtml = subjectsafehtml;
_x.languagecode = languagecode;
_x.versionnumber = versionnumber;
_x.opencount = opencount;
_x._createdonbehalfby_value = _createdonbehalfby_value;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Template[");
b.append("openrate=");
b.append(this.openrate);
b.append(", ");
b.append("replyrate=");
b.append(this.replyrate);
b.append(", ");
b.append("subjectpresentationxml=");
b.append(this.subjectpresentationxml);
b.append(", ");
b.append("generationtypecode=");
b.append(this.generationtypecode);
b.append(", ");
b.append("_createdby_value=");
b.append(this._createdby_value);
b.append(", ");
b.append("mimetype=");
b.append(this.mimetype);
b.append(", ");
b.append("modifiedon=");
b.append(this.modifiedon);
b.append(", ");
b.append("ismanaged=");
b.append(this.ismanaged);
b.append(", ");
b.append("safehtml=");
b.append(this.safehtml);
b.append(", ");
b.append("_owningbusinessunit_value=");
b.append(this._owningbusinessunit_value);
b.append(", ");
b.append("_ownerid_value=");
b.append(this._ownerid_value);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("templateidunique=");
b.append(this.templateidunique);
b.append(", ");
b.append("entityimage_url=");
b.append(this.entityimage_url);
b.append(", ");
b.append("body=");
b.append(this.body);
b.append(", ");
b.append("solutionid=");
b.append(this.solutionid);
b.append(", ");
b.append("_modifiedby_value=");
b.append(this._modifiedby_value);
b.append(", ");
b.append("_owningteam_value=");
b.append(this._owningteam_value);
b.append(", ");
b.append("templatetypecode=");
b.append(this.templatetypecode);
b.append(", ");
b.append("ispersonal=");
b.append(this.ispersonal);
b.append(", ");
b.append("usedcount=");
b.append(this.usedcount);
b.append(", ");
b.append("componentstate=");
b.append(this.componentstate);
b.append(", ");
b.append("entityimageid=");
b.append(this.entityimageid);
b.append(", ");
b.append("entityimage_timestamp=");
b.append(this.entityimage_timestamp);
b.append(", ");
b.append("title=");
b.append(this.title);
b.append(", ");
b.append("importsequencenumber=");
b.append(this.importsequencenumber);
b.append(", ");
b.append("_owninguser_value=");
b.append(this._owninguser_value);
b.append(", ");
b.append("overwritetime=");
b.append(this.overwritetime);
b.append(", ");
b.append("_modifiedonbehalfby_value=");
b.append(this._modifiedonbehalfby_value);
b.append(", ");
b.append("isrecommended=");
b.append(this.isrecommended);
b.append(", ");
b.append("introducedversion=");
b.append(this.introducedversion);
b.append(", ");
b.append("createdon=");
b.append(this.createdon);
b.append(", ");
b.append("templateid=");
b.append(this.templateid);
b.append(", ");
b.append("replycount=");
b.append(this.replycount);
b.append(", ");
b.append("presentationxml=");
b.append(this.presentationxml);
b.append(", ");
b.append("iscustomizable=");
b.append(this.iscustomizable);
b.append(", ");
b.append("subject=");
b.append(this.subject);
b.append(", ");
b.append("entityimage=");
b.append(this.entityimage);
b.append(", ");
b.append("subjectsafehtml=");
b.append(this.subjectsafehtml);
b.append(", ");
b.append("languagecode=");
b.append(this.languagecode);
b.append(", ");
b.append("versionnumber=");
b.append(this.versionnumber);
b.append(", ");
b.append("opencount=");
b.append(this.opencount);
b.append(", ");
b.append("_createdonbehalfby_value=");
b.append(this._createdonbehalfby_value);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy