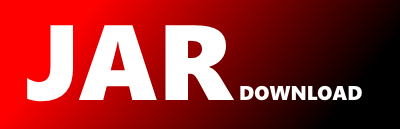
microsoft.dynamics.crm.entity.collection.request.GoalCollectionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity.collection.request;
import com.github.davidmoten.odata.client.CollectionPageEntityRequest;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.NameValue;
import java.lang.Object;
import java.util.Optional;
import java.util.UUID;
import microsoft.dynamics.crm.entity.Goal;
import microsoft.dynamics.crm.entity.request.AnnotationRequest;
import microsoft.dynamics.crm.entity.request.AsyncoperationRequest;
import microsoft.dynamics.crm.entity.request.ConnectionRequest;
import microsoft.dynamics.crm.entity.request.DuplicaterecordRequest;
import microsoft.dynamics.crm.entity.request.GoalRequest;
import microsoft.dynamics.crm.entity.request.PrincipalobjectattributeaccessRequest;
import microsoft.dynamics.crm.entity.request.ProcesssessionRequest;
import microsoft.dynamics.crm.entity.request.SyncerrorRequest;
public class GoalCollectionRequest extends CollectionPageEntityRequest{
protected ContextPath contextPath;
public GoalCollectionRequest(ContextPath contextPath, Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy