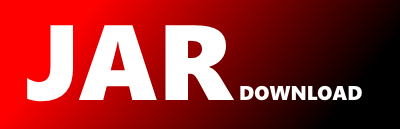
microsoft.dynamics.crm.entity.set.Organizations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.OrganizationCollectionRequest;
public final class Organizations extends OrganizationCollectionRequest {
public Organizations(ContextPath contextPath) {
super(contextPath, Optional.empty());
}
public Templates acknowledgementtemplateid() {
return new Templates(contextPath.addSegment("acknowledgementtemplateid"));
}
public Transactioncurrencies basecurrencyid() {
return new Transactioncurrencies(contextPath.addSegment("basecurrencyid"));
}
public Calendars businessclosurecalendarid_calendar() {
return new Calendars(contextPath.addSegment("businessclosurecalendarid_calendar"));
}
public Systemusers createdby() {
return new Systemusers(contextPath.addSegment("createdby"));
}
public Systemusers createdonbehalfby() {
return new Systemusers(contextPath.addSegment("createdonbehalfby"));
}
public Customcontrols customcontrol_organization() {
return new Customcontrols(contextPath.addSegment("customcontrol_organization"));
}
public Customcontroldefaultconfigs customcontroldefaultconfig_organization() {
return new Customcontroldefaultconfigs(contextPath.addSegment("customcontroldefaultconfig_organization"));
}
public Customcontrolresources customcontrolresource_organization() {
return new Customcontrolresources(contextPath.addSegment("customcontrolresource_organization"));
}
public Emailserverprofiles defaultemailserverprofileid() {
return new Emailserverprofiles(contextPath.addSegment("defaultemailserverprofileid"));
}
public Mobileofflineprofiles defaultmobileofflineprofileid() {
return new Mobileofflineprofiles(contextPath.addSegment("defaultmobileofflineprofileid"));
}
public Languagelocale languagelocale_organization() {
return new Languagelocale(contextPath.addSegment("languagelocale_organization"));
}
public Dataperformances lk_dataperformance_organizationid() {
return new Dataperformances(contextPath.addSegment("lk_dataperformance_organizationid"));
}
public Documenttemplates lk_documenttemplatebase_organization() {
return new Documenttemplates(contextPath.addSegment("lk_documenttemplatebase_organization"));
}
public Fieldsecurityprofiles lk_fieldsecurityprofile_organizationid() {
return new Fieldsecurityprofiles(contextPath.addSegment("lk_fieldsecurityprofile_organizationid"));
}
public Principalobjectattributeaccessset lk_principalobjectattributeaccess_organizationid() {
return new Principalobjectattributeaccessset(contextPath.addSegment("lk_principalobjectattributeaccess_organizationid"));
}
public Mobileofflineprofiles mobileOfflineProfile_organization() {
return new Mobileofflineprofiles(contextPath.addSegment("MobileOfflineProfile_organization"));
}
public Mobileofflineprofileitems mobileOfflineProfileItem_organization() {
return new Mobileofflineprofileitems(contextPath.addSegment("MobileOfflineProfileItem_organization"));
}
public Mobileofflineprofileitemassociations mobileOfflineProfileItemAssociation_organization() {
return new Mobileofflineprofileitemassociations(contextPath.addSegment("MobileOfflineProfileItemAssociation_organization"));
}
public Systemusers modifiedby() {
return new Systemusers(contextPath.addSegment("modifiedby"));
}
public Systemusers modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("modifiedonbehalfby"));
}
public Aciviewmappers organization_aciviewmapper() {
return new Aciviewmappers(contextPath.addSegment("organization_aciviewmapper"));
}
public Appconfigs organization_appconfig() {
return new Appconfigs(contextPath.addSegment("organization_appconfig"));
}
public Appconfiginstances organization_appconfiginstance() {
return new Appconfiginstances(contextPath.addSegment("organization_appconfiginstance"));
}
public Appconfigmasters organization_appconfigmaster() {
return new Appconfigmasters(contextPath.addSegment("organization_appconfigmaster"));
}
public Appmodules organization_appmodule() {
return new Appmodules(contextPath.addSegment("organization_appmodule"));
}
public Asyncoperations organization_AsyncOperations() {
return new Asyncoperations(contextPath.addSegment("Organization_AsyncOperations"));
}
public Bulkdeletefailures organization_BulkDeleteFailures() {
return new Bulkdeletefailures(contextPath.addSegment("Organization_BulkDeleteFailures"));
}
public Businessunitnewsarticles organization_business_unit_news_articles() {
return new Businessunitnewsarticles(contextPath.addSegment("organization_business_unit_news_articles"));
}
public Businessunits organization_business_units() {
return new Businessunits(contextPath.addSegment("organization_business_units"));
}
public Calendars organization_calendars() {
return new Calendars(contextPath.addSegment("organization_calendars"));
}
public Complexcontrols organization_complexcontrols() {
return new Complexcontrols(contextPath.addSegment("organization_complexcontrols"));
}
public Connectionroles organization_connection_roles() {
return new Connectionroles(contextPath.addSegment("organization_connection_roles"));
}
public Displaystrings organization_custom_displaystrings() {
return new Displaystrings(contextPath.addSegment("organization_custom_displaystrings"));
}
public Datalakeworkspaces organization_datalakeworkspace() {
return new Datalakeworkspaces(contextPath.addSegment("organization_datalakeworkspace"));
}
public Datalakeworkspacepermissions organization_datalakeworkspacepermission() {
return new Datalakeworkspacepermissions(contextPath.addSegment("organization_datalakeworkspacepermission"));
}
public Emailserverprofiles organization_emailserverprofile() {
return new Emailserverprofiles(contextPath.addSegment("organization_emailserverprofile"));
}
public Entityanalyticsconfigs organization_entityanalyticsconfig() {
return new Entityanalyticsconfigs(contextPath.addSegment("organization_entityanalyticsconfig"));
}
public Expiredprocesses organization_expiredprocess() {
return new Expiredprocesses(contextPath.addSegment("organization_expiredprocess"));
}
public Importjobs organization_importjob() {
return new Importjobs(contextPath.addSegment("organization_importjob"));
}
public Kbarticletemplates organization_kb_article_templates() {
return new Kbarticletemplates(contextPath.addSegment("organization_kb_article_templates"));
}
public Kbarticles organization_kb_articles() {
return new Kbarticles(contextPath.addSegment("organization_kb_articles"));
}
public Knowledgebaserecords organization_KnowledgeBaseRecord() {
return new Knowledgebaserecords(contextPath.addSegment("organization_KnowledgeBaseRecord"));
}
public Mailboxes organization_mailbox() {
return new Mailboxes(contextPath.addSegment("organization_mailbox"));
}
public Mailboxtrackingfolders organization_MailboxTrackingFolder() {
return new Mailboxtrackingfolders(contextPath.addSegment("Organization_MailboxTrackingFolder"));
}
public Metrics organization_metric() {
return new Metrics(contextPath.addSegment("organization_metric"));
}
public Msdyn_helppages organization_msdyn_helppage() {
return new Msdyn_helppages(contextPath.addSegment("organization_msdyn_helppage"));
}
public Msdyn_solutionhealthrulesets organization_msdyn_solutionhealthruleset() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("organization_msdyn_solutionhealthruleset"));
}
public Navigationsettings organization_navigationsetting() {
return new Navigationsettings(contextPath.addSegment("organization_navigationsetting"));
}
public Newprocesses organization_newprocess() {
return new Newprocesses(contextPath.addSegment("organization_newprocess"));
}
public Officegraphdocuments organization_officegraphdocument() {
return new Officegraphdocuments(contextPath.addSegment("organization_officegraphdocument"));
}
public Pluginassemblies organization_pluginassembly() {
return new Pluginassemblies(contextPath.addSegment("organization_pluginassembly"));
}
public Plugintypes organization_plugintype() {
return new Plugintypes(contextPath.addSegment("organization_plugintype"));
}
public Plugintypestatistics organization_plugintypestatistic() {
return new Plugintypestatistics(contextPath.addSegment("organization_plugintypestatistic"));
}
public Positions organization_position() {
return new Positions(contextPath.addSegment("organization_position"));
}
public Posts organization_post() {
return new Posts(contextPath.addSegment("organization_post"));
}
public Postcomments organization_PostComment() {
return new Postcomments(contextPath.addSegment("organization_PostComment"));
}
public Postlikes organization_postlike() {
return new Postlikes(contextPath.addSegment("organization_postlike"));
}
public Publishers organization_publisher() {
return new Publishers(contextPath.addSegment("organization_publisher"));
}
public Queueitems organization_queueitems() {
return new Queueitems(contextPath.addSegment("organization_queueitems"));
}
public Queues organization_queues() {
return new Queues(contextPath.addSegment("organization_queues"));
}
public Recommendeddocuments organization_recommendeddocument() {
return new Recommendeddocuments(contextPath.addSegment("organization_recommendeddocument"));
}
public Roles organization_roles() {
return new Roles(contextPath.addSegment("organization_roles"));
}
public Savedqueries organization_saved_queries() {
return new Savedqueries(contextPath.addSegment("organization_saved_queries"));
}
public Savedqueryvisualizations organization_saved_query_visualizations() {
return new Savedqueryvisualizations(contextPath.addSegment("organization_saved_query_visualizations"));
}
public Sdkmessages organization_sdkmessage() {
return new Sdkmessages(contextPath.addSegment("organization_sdkmessage"));
}
public Sdkmessagefilters organization_sdkmessagefilter() {
return new Sdkmessagefilters(contextPath.addSegment("organization_sdkmessagefilter"));
}
public Sdkmessageprocessingsteps organization_sdkmessageprocessingstep() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("organization_sdkmessageprocessingstep"));
}
public Sdkmessageprocessingstepimages organization_sdkmessageprocessingstepimage() {
return new Sdkmessageprocessingstepimages(contextPath.addSegment("organization_sdkmessageprocessingstepimage"));
}
public Sdkmessageprocessingstepsecureconfigs organization_sdkmessageprocessingstepsecureconfig() {
return new Sdkmessageprocessingstepsecureconfigs(contextPath.addSegment("organization_sdkmessageprocessingstepsecureconfig"));
}
public Serviceendpoints organization_serviceendpoint() {
return new Serviceendpoints(contextPath.addSegment("organization_serviceendpoint"));
}
public Similarityrules organization_similarityrule() {
return new Similarityrules(contextPath.addSegment("organization_similarityrule"));
}
public Sitemaps organization_sitemap() {
return new Sitemaps(contextPath.addSegment("organization_sitemap"));
}
public Solutions organization_solution() {
return new Solutions(contextPath.addSegment("organization_solution"));
}
public Solutioncomponentattributeconfigurations organization_solutioncomponentattributeconfiguration() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("organization_solutioncomponentattributeconfiguration"));
}
public Solutioncomponentconfigurations organization_solutioncomponentconfiguration() {
return new Solutioncomponentconfigurations(contextPath.addSegment("organization_solutioncomponentconfiguration"));
}
public Solutioncomponentrelationshipconfigurations organization_solutioncomponentrelationshipconfiguration() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("organization_solutioncomponentrelationshipconfiguration"));
}
public Subjects organization_subjects() {
return new Subjects(contextPath.addSegment("organization_subjects"));
}
public Syncerrors organization_SyncErrors() {
return new Syncerrors(contextPath.addSegment("Organization_SyncErrors"));
}
public Systemusers organization_system_users() {
return new Systemusers(contextPath.addSegment("organization_system_users"));
}
public Systemforms organization_systemforms() {
return new Systemforms(contextPath.addSegment("organization_systemforms"));
}
public Teams organization_teams() {
return new Teams(contextPath.addSegment("organization_teams"));
}
public Territories organization_territories() {
return new Territories(contextPath.addSegment("organization_territories"));
}
public Themes organization_theme() {
return new Themes(contextPath.addSegment("organization_theme"));
}
public Tracelogs organization_tracelog() {
return new Tracelogs(contextPath.addSegment("organization_tracelog"));
}
public Transactioncurrencies organization_transactioncurrencies() {
return new Transactioncurrencies(contextPath.addSegment("organization_transactioncurrencies"));
}
public Translationprocesses organization_translationprocess() {
return new Translationprocesses(contextPath.addSegment("organization_translationprocess"));
}
public Usermappings organization_UserMapping() {
return new Usermappings(contextPath.addSegment("organization_UserMapping"));
}
public Webwizards organization_webwizard() {
return new Webwizards(contextPath.addSegment("organization_webwizard"));
}
public Webresourceset webresource_organization() {
return new Webresourceset(contextPath.addSegment("webresource_organization"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy