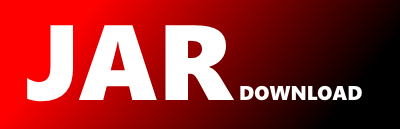
microsoft.dynamics.crm.entity.set.Systemusers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-microsoft-dynamics Show documentation
Show all versions of odata-client-microsoft-dynamics Show documentation
Java client as template for Microsoft Dynamics organisation endpoints
The newest version!
package microsoft.dynamics.crm.entity.set;
import com.github.davidmoten.odata.client.ContextPath;
import java.util.Optional;
import microsoft.dynamics.crm.entity.collection.request.SystemuserCollectionRequest;
public final class Systemusers extends SystemuserCollectionRequest {
public Systemusers(ContextPath contextPath) {
super(contextPath, Optional.empty());
}
public Annotations annotation_owning_user() {
return new Annotations(contextPath.addSegment("annotation_owning_user"));
}
public Appmodulecomponents appmodulecomponent_createdby() {
return new Appmodulecomponents(contextPath.addSegment("appmodulecomponent_createdby"));
}
public Appmodulecomponents appmodulecomponent_modifiedby() {
return new Appmodulecomponents(contextPath.addSegment("appmodulecomponent_modifiedby"));
}
public Businessunits businessunitid() {
return new Businessunits(contextPath.addSegment("businessunitid"));
}
public Calendars calendarid() {
return new Calendars(contextPath.addSegment("calendarid"));
}
public Contacts contact_owning_user() {
return new Contacts(contextPath.addSegment("contact_owning_user"));
}
public Systemusers createdby() {
return new Systemusers(contextPath.addSegment("createdby"));
}
public Connections createdby_connection() {
return new Connections(contextPath.addSegment("createdby_connection"));
}
public Connectionroles createdby_connection_role() {
return new Connectionroles(contextPath.addSegment("createdby_connection_role"));
}
public Pluginassemblies createdby_pluginassembly() {
return new Pluginassemblies(contextPath.addSegment("createdby_pluginassembly"));
}
public Plugintracelogs createdby_plugintracelog() {
return new Plugintracelogs(contextPath.addSegment("createdby_plugintracelog"));
}
public Plugintypes createdby_plugintype() {
return new Plugintypes(contextPath.addSegment("createdby_plugintype"));
}
public Plugintypestatistics createdby_plugintypestatistic() {
return new Plugintypestatistics(contextPath.addSegment("createdby_plugintypestatistic"));
}
public Sdkmessages createdby_sdkmessage() {
return new Sdkmessages(contextPath.addSegment("createdby_sdkmessage"));
}
public Sdkmessagefilters createdby_sdkmessagefilter() {
return new Sdkmessagefilters(contextPath.addSegment("createdby_sdkmessagefilter"));
}
public Sdkmessageprocessingsteps createdby_sdkmessageprocessingstep() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("createdby_sdkmessageprocessingstep"));
}
public Sdkmessageprocessingstepimages createdby_sdkmessageprocessingstepimage() {
return new Sdkmessageprocessingstepimages(contextPath.addSegment("createdby_sdkmessageprocessingstepimage"));
}
public Sdkmessageprocessingstepsecureconfigs createdby_sdkmessageprocessingstepsecureconfig() {
return new Sdkmessageprocessingstepsecureconfigs(contextPath.addSegment("createdby_sdkmessageprocessingstepsecureconfig"));
}
public Serviceendpoints createdby_serviceendpoint() {
return new Serviceendpoints(contextPath.addSegment("createdby_serviceendpoint"));
}
public Systemusers createdonbehalfby() {
return new Systemusers(contextPath.addSegment("createdonbehalfby"));
}
public Mailboxes defaultmailbox() {
return new Mailboxes(contextPath.addSegment("defaultmailbox"));
}
public Sdkmessageprocessingsteps impersonatinguserid_sdkmessageprocessingstep() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("impersonatinguserid_sdkmessageprocessingstep"));
}
public Importfiles importFile_SystemUser() {
return new Importfiles(contextPath.addSegment("ImportFile_SystemUser"));
}
public Knowledgearticles knowledgearticle_primaryauthorid() {
return new Knowledgearticles(contextPath.addSegment("knowledgearticle_primaryauthorid"));
}
public Accounts lk_accountbase_createdby() {
return new Accounts(contextPath.addSegment("lk_accountbase_createdby"));
}
public Accounts lk_accountbase_createdonbehalfby() {
return new Accounts(contextPath.addSegment("lk_accountbase_createdonbehalfby"));
}
public Accounts lk_accountbase_modifiedby() {
return new Accounts(contextPath.addSegment("lk_accountbase_modifiedby"));
}
public Accounts lk_accountbase_modifiedonbehalfby() {
return new Accounts(contextPath.addSegment("lk_accountbase_modifiedonbehalfby"));
}
public Aciviewmappers lk_ACIViewMapper_createdby() {
return new Aciviewmappers(contextPath.addSegment("lk_ACIViewMapper_createdby"));
}
public Aciviewmappers lk_ACIViewMapper_createdonbehalfby() {
return new Aciviewmappers(contextPath.addSegment("lk_ACIViewMapper_createdonbehalfby"));
}
public Aciviewmappers lk_ACIViewMapper_modifiedby() {
return new Aciviewmappers(contextPath.addSegment("lk_ACIViewMapper_modifiedby"));
}
public Aciviewmappers lk_ACIViewMapper_modifiedonbehalfby() {
return new Aciviewmappers(contextPath.addSegment("lk_ACIViewMapper_modifiedonbehalfby"));
}
public Actioncards lk_actioncardbase_createdby() {
return new Actioncards(contextPath.addSegment("lk_actioncardbase_createdby"));
}
public Actioncards lk_actioncardbase_createdonbehalfby() {
return new Actioncards(contextPath.addSegment("lk_actioncardbase_createdonbehalfby"));
}
public Actioncards lk_actioncardbase_modifiedby() {
return new Actioncards(contextPath.addSegment("lk_actioncardbase_modifiedby"));
}
public Actioncards lk_actioncardbase_modifiedonbehalfby() {
return new Actioncards(contextPath.addSegment("lk_actioncardbase_modifiedonbehalfby"));
}
public Activitypointers lk_activitypointer_createdby() {
return new Activitypointers(contextPath.addSegment("lk_activitypointer_createdby"));
}
public Activitypointers lk_activitypointer_createdonbehalfby() {
return new Activitypointers(contextPath.addSegment("lk_activitypointer_createdonbehalfby"));
}
public Activitypointers lk_activitypointer_modifiedby() {
return new Activitypointers(contextPath.addSegment("lk_activitypointer_modifiedby"));
}
public Activitypointers lk_activitypointer_modifiedonbehalfby() {
return new Activitypointers(contextPath.addSegment("lk_activitypointer_modifiedonbehalfby"));
}
public Annotations lk_annotationbase_createdby() {
return new Annotations(contextPath.addSegment("lk_annotationbase_createdby"));
}
public Annotations lk_annotationbase_createdonbehalfby() {
return new Annotations(contextPath.addSegment("lk_annotationbase_createdonbehalfby"));
}
public Annotations lk_annotationbase_modifiedby() {
return new Annotations(contextPath.addSegment("lk_annotationbase_modifiedby"));
}
public Annotations lk_annotationbase_modifiedonbehalfby() {
return new Annotations(contextPath.addSegment("lk_annotationbase_modifiedonbehalfby"));
}
public Annualfiscalcalendars lk_annualfiscalcalendar_createdby() {
return new Annualfiscalcalendars(contextPath.addSegment("lk_annualfiscalcalendar_createdby"));
}
public Annualfiscalcalendars lk_annualfiscalcalendar_createdonbehalfby() {
return new Annualfiscalcalendars(contextPath.addSegment("lk_annualfiscalcalendar_createdonbehalfby"));
}
public Annualfiscalcalendars lk_annualfiscalcalendar_modifiedby() {
return new Annualfiscalcalendars(contextPath.addSegment("lk_annualfiscalcalendar_modifiedby"));
}
public Annualfiscalcalendars lk_annualfiscalcalendar_modifiedonbehalfby() {
return new Annualfiscalcalendars(contextPath.addSegment("lk_annualfiscalcalendar_modifiedonbehalfby"));
}
public Annualfiscalcalendars lk_annualfiscalcalendar_salespersonid() {
return new Annualfiscalcalendars(contextPath.addSegment("lk_annualfiscalcalendar_salespersonid"));
}
public Applicationusers lk_applicationuser_createdby() {
return new Applicationusers(contextPath.addSegment("lk_applicationuser_createdby"));
}
public Applicationusers lk_applicationuser_createdonbehalfby() {
return new Applicationusers(contextPath.addSegment("lk_applicationuser_createdonbehalfby"));
}
public Applicationusers lk_applicationuser_modifiedby() {
return new Applicationusers(contextPath.addSegment("lk_applicationuser_modifiedby"));
}
public Applicationusers lk_applicationuser_modifiedonbehalfby() {
return new Applicationusers(contextPath.addSegment("lk_applicationuser_modifiedonbehalfby"));
}
public Appmodulecomponents lk_appmodulecomponent_createdonbehalfby() {
return new Appmodulecomponents(contextPath.addSegment("lk_appmodulecomponent_createdonbehalfby"));
}
public Appmodulecomponents lk_appmodulecomponent_modifiedonbehalfby() {
return new Appmodulecomponents(contextPath.addSegment("lk_appmodulecomponent_modifiedonbehalfby"));
}
public Appointments lk_appointment_createdby() {
return new Appointments(contextPath.addSegment("lk_appointment_createdby"));
}
public Appointments lk_appointment_createdonbehalfby() {
return new Appointments(contextPath.addSegment("lk_appointment_createdonbehalfby"));
}
public Appointments lk_appointment_modifiedby() {
return new Appointments(contextPath.addSegment("lk_appointment_modifiedby"));
}
public Appointments lk_appointment_modifiedonbehalfby() {
return new Appointments(contextPath.addSegment("lk_appointment_modifiedonbehalfby"));
}
public Asyncoperations lk_asyncoperation_createdby() {
return new Asyncoperations(contextPath.addSegment("lk_asyncoperation_createdby"));
}
public Asyncoperations lk_asyncoperation_createdonbehalfby() {
return new Asyncoperations(contextPath.addSegment("lk_asyncoperation_createdonbehalfby"));
}
public Asyncoperations lk_asyncoperation_modifiedby() {
return new Asyncoperations(contextPath.addSegment("lk_asyncoperation_modifiedby"));
}
public Asyncoperations lk_asyncoperation_modifiedonbehalfby() {
return new Asyncoperations(contextPath.addSegment("lk_asyncoperation_modifiedonbehalfby"));
}
public Audits lk_audit_callinguserid() {
return new Audits(contextPath.addSegment("lk_audit_callinguserid"));
}
public Audits lk_audit_userid() {
return new Audits(contextPath.addSegment("lk_audit_userid"));
}
public Bulkdeleteoperations lk_bulkdeleteoperation_createdonbehalfby() {
return new Bulkdeleteoperations(contextPath.addSegment("lk_bulkdeleteoperation_createdonbehalfby"));
}
public Bulkdeleteoperations lk_bulkdeleteoperation_modifiedonbehalfby() {
return new Bulkdeleteoperations(contextPath.addSegment("lk_bulkdeleteoperation_modifiedonbehalfby"));
}
public Bulkdeleteoperations lk_bulkdeleteoperationbase_createdby() {
return new Bulkdeleteoperations(contextPath.addSegment("lk_bulkdeleteoperationbase_createdby"));
}
public Bulkdeleteoperations lk_bulkdeleteoperationbase_modifiedby() {
return new Bulkdeleteoperations(contextPath.addSegment("lk_bulkdeleteoperationbase_modifiedby"));
}
public Businessunits lk_businessunit_createdonbehalfby() {
return new Businessunits(contextPath.addSegment("lk_businessunit_createdonbehalfby"));
}
public Businessunits lk_businessunit_modifiedonbehalfby() {
return new Businessunits(contextPath.addSegment("lk_businessunit_modifiedonbehalfby"));
}
public Businessunits lk_businessunitbase_createdby() {
return new Businessunits(contextPath.addSegment("lk_businessunitbase_createdby"));
}
public Businessunits lk_businessunitbase_modifiedby() {
return new Businessunits(contextPath.addSegment("lk_businessunitbase_modifiedby"));
}
public Businessunitnewsarticles lk_businessunitnewsarticle_createdonbehalfby() {
return new Businessunitnewsarticles(contextPath.addSegment("lk_businessunitnewsarticle_createdonbehalfby"));
}
public Businessunitnewsarticles lk_businessunitnewsarticle_modifiedonbehalfby() {
return new Businessunitnewsarticles(contextPath.addSegment("lk_businessunitnewsarticle_modifiedonbehalfby"));
}
public Businessunitnewsarticles lk_businessunitnewsarticlebase_createdby() {
return new Businessunitnewsarticles(contextPath.addSegment("lk_businessunitnewsarticlebase_createdby"));
}
public Businessunitnewsarticles lk_businessunitnewsarticlebase_modifiedby() {
return new Businessunitnewsarticles(contextPath.addSegment("lk_businessunitnewsarticlebase_modifiedby"));
}
public Calendars lk_calendar_createdby() {
return new Calendars(contextPath.addSegment("lk_calendar_createdby"));
}
public Calendars lk_calendar_createdonbehalfby() {
return new Calendars(contextPath.addSegment("lk_calendar_createdonbehalfby"));
}
public Calendars lk_calendar_modifiedby() {
return new Calendars(contextPath.addSegment("lk_calendar_modifiedby"));
}
public Calendars lk_calendar_modifiedonbehalfby() {
return new Calendars(contextPath.addSegment("lk_calendar_modifiedonbehalfby"));
}
public Calendarrules lk_calendarrule_createdby() {
return new Calendarrules(contextPath.addSegment("lk_calendarrule_createdby"));
}
public Calendarrules lk_calendarrule_createdonbehalfby() {
return new Calendarrules(contextPath.addSegment("lk_calendarrule_createdonbehalfby"));
}
public Calendarrules lk_calendarrule_modifiedby() {
return new Calendarrules(contextPath.addSegment("lk_calendarrule_modifiedby"));
}
public Calendarrules lk_calendarrule_modifiedonbehalfby() {
return new Calendarrules(contextPath.addSegment("lk_calendarrule_modifiedonbehalfby"));
}
public Categories lk_category_createdby() {
return new Categories(contextPath.addSegment("lk_category_createdby"));
}
public Categories lk_category_createdonbehalfby() {
return new Categories(contextPath.addSegment("lk_category_createdonbehalfby"));
}
public Categories lk_category_modifiedby() {
return new Categories(contextPath.addSegment("lk_category_modifiedby"));
}
public Categories lk_category_modifiedonbehalfby() {
return new Categories(contextPath.addSegment("lk_category_modifiedonbehalfby"));
}
public Columnmappings lk_columnmapping_createdby() {
return new Columnmappings(contextPath.addSegment("lk_columnmapping_createdby"));
}
public Columnmappings lk_columnmapping_createdonbehalfby() {
return new Columnmappings(contextPath.addSegment("lk_columnmapping_createdonbehalfby"));
}
public Columnmappings lk_columnmapping_modifiedby() {
return new Columnmappings(contextPath.addSegment("lk_columnmapping_modifiedby"));
}
public Columnmappings lk_columnmapping_modifiedonbehalfby() {
return new Columnmappings(contextPath.addSegment("lk_columnmapping_modifiedonbehalfby"));
}
public Connections lk_connectionbase_createdonbehalfby() {
return new Connections(contextPath.addSegment("lk_connectionbase_createdonbehalfby"));
}
public Connections lk_connectionbase_modifiedonbehalfby() {
return new Connections(contextPath.addSegment("lk_connectionbase_modifiedonbehalfby"));
}
public Connectionreferences lk_connectionreference_createdby() {
return new Connectionreferences(contextPath.addSegment("lk_connectionreference_createdby"));
}
public Connectionreferences lk_connectionreference_createdonbehalfby() {
return new Connectionreferences(contextPath.addSegment("lk_connectionreference_createdonbehalfby"));
}
public Connectionreferences lk_connectionreference_modifiedby() {
return new Connectionreferences(contextPath.addSegment("lk_connectionreference_modifiedby"));
}
public Connectionreferences lk_connectionreference_modifiedonbehalfby() {
return new Connectionreferences(contextPath.addSegment("lk_connectionreference_modifiedonbehalfby"));
}
public Connectionroles lk_connectionrolebase_createdonbehalfby() {
return new Connectionroles(contextPath.addSegment("lk_connectionrolebase_createdonbehalfby"));
}
public Connectionroles lk_connectionrolebase_modifiedonbehalfby() {
return new Connectionroles(contextPath.addSegment("lk_connectionrolebase_modifiedonbehalfby"));
}
public Connectors lk_connector_createdby() {
return new Connectors(contextPath.addSegment("lk_connector_createdby"));
}
public Connectors lk_connector_createdonbehalfby() {
return new Connectors(contextPath.addSegment("lk_connector_createdonbehalfby"));
}
public Connectors lk_connector_modifiedby() {
return new Connectors(contextPath.addSegment("lk_connector_modifiedby"));
}
public Connectors lk_connector_modifiedonbehalfby() {
return new Connectors(contextPath.addSegment("lk_connector_modifiedonbehalfby"));
}
public Contacts lk_contact_createdonbehalfby() {
return new Contacts(contextPath.addSegment("lk_contact_createdonbehalfby"));
}
public Contacts lk_contact_modifiedonbehalfby() {
return new Contacts(contextPath.addSegment("lk_contact_modifiedonbehalfby"));
}
public Contacts lk_contactbase_createdby() {
return new Contacts(contextPath.addSegment("lk_contactbase_createdby"));
}
public Contacts lk_contactbase_modifiedby() {
return new Contacts(contextPath.addSegment("lk_contactbase_modifiedby"));
}
public Customcontrols lk_customcontrol_createdby() {
return new Customcontrols(contextPath.addSegment("lk_customcontrol_createdby"));
}
public Customcontrols lk_customcontrol_createdonbehalfby() {
return new Customcontrols(contextPath.addSegment("lk_customcontrol_createdonbehalfby"));
}
public Customcontrols lk_customcontrol_modifiedby() {
return new Customcontrols(contextPath.addSegment("lk_customcontrol_modifiedby"));
}
public Customcontrols lk_customcontrol_modifiedonbehalfby() {
return new Customcontrols(contextPath.addSegment("lk_customcontrol_modifiedonbehalfby"));
}
public Customcontroldefaultconfigs lk_customcontroldefaultconfig_createdby() {
return new Customcontroldefaultconfigs(contextPath.addSegment("lk_customcontroldefaultconfig_createdby"));
}
public Customcontroldefaultconfigs lk_customcontroldefaultconfig_createdonbehalfby() {
return new Customcontroldefaultconfigs(contextPath.addSegment("lk_customcontroldefaultconfig_createdonbehalfby"));
}
public Customcontroldefaultconfigs lk_customcontroldefaultconfig_modifiedby() {
return new Customcontroldefaultconfigs(contextPath.addSegment("lk_customcontroldefaultconfig_modifiedby"));
}
public Customcontroldefaultconfigs lk_customcontroldefaultconfig_modifiedonbehalfby() {
return new Customcontroldefaultconfigs(contextPath.addSegment("lk_customcontroldefaultconfig_modifiedonbehalfby"));
}
public Customcontrolresources lk_customcontrolresource_createdby() {
return new Customcontrolresources(contextPath.addSegment("lk_customcontrolresource_createdby"));
}
public Customcontrolresources lk_customcontrolresource_createdonbehalfby() {
return new Customcontrolresources(contextPath.addSegment("lk_customcontrolresource_createdonbehalfby"));
}
public Customcontrolresources lk_customcontrolresource_modifiedby() {
return new Customcontrolresources(contextPath.addSegment("lk_customcontrolresource_modifiedby"));
}
public Customcontrolresources lk_customcontrolresource_modifiedonbehalfby() {
return new Customcontrolresources(contextPath.addSegment("lk_customcontrolresource_modifiedonbehalfby"));
}
public Customeraddresses lk_customeraddress_createdonbehalfby() {
return new Customeraddresses(contextPath.addSegment("lk_customeraddress_createdonbehalfby"));
}
public Customeraddresses lk_customeraddress_modifiedonbehalfby() {
return new Customeraddresses(contextPath.addSegment("lk_customeraddress_modifiedonbehalfby"));
}
public Customeraddresses lk_customeraddressbase_createdby() {
return new Customeraddresses(contextPath.addSegment("lk_customeraddressbase_createdby"));
}
public Customeraddresses lk_customeraddressbase_modifiedby() {
return new Customeraddresses(contextPath.addSegment("lk_customeraddressbase_modifiedby"));
}
public Datalakeworkspaces lk_datalakeworkspace_createdby() {
return new Datalakeworkspaces(contextPath.addSegment("lk_datalakeworkspace_createdby"));
}
public Datalakeworkspaces lk_datalakeworkspace_createdonbehalfby() {
return new Datalakeworkspaces(contextPath.addSegment("lk_datalakeworkspace_createdonbehalfby"));
}
public Datalakeworkspaces lk_datalakeworkspace_modifiedby() {
return new Datalakeworkspaces(contextPath.addSegment("lk_datalakeworkspace_modifiedby"));
}
public Datalakeworkspaces lk_datalakeworkspace_modifiedonbehalfby() {
return new Datalakeworkspaces(contextPath.addSegment("lk_datalakeworkspace_modifiedonbehalfby"));
}
public Datalakeworkspacepermissions lk_datalakeworkspacepermission_createdby() {
return new Datalakeworkspacepermissions(contextPath.addSegment("lk_datalakeworkspacepermission_createdby"));
}
public Datalakeworkspacepermissions lk_datalakeworkspacepermission_createdonbehalfby() {
return new Datalakeworkspacepermissions(contextPath.addSegment("lk_datalakeworkspacepermission_createdonbehalfby"));
}
public Datalakeworkspacepermissions lk_datalakeworkspacepermission_modifiedby() {
return new Datalakeworkspacepermissions(contextPath.addSegment("lk_datalakeworkspacepermission_modifiedby"));
}
public Datalakeworkspacepermissions lk_datalakeworkspacepermission_modifiedonbehalfby() {
return new Datalakeworkspacepermissions(contextPath.addSegment("lk_datalakeworkspacepermission_modifiedonbehalfby"));
}
public Displaystrings lk_DisplayStringbase_createdby() {
return new Displaystrings(contextPath.addSegment("lk_DisplayStringbase_createdby"));
}
public Displaystrings lk_DisplayStringbase_createdonbehalfby() {
return new Displaystrings(contextPath.addSegment("lk_DisplayStringbase_createdonbehalfby"));
}
public Displaystrings lk_DisplayStringbase_modifiedby() {
return new Displaystrings(contextPath.addSegment("lk_DisplayStringbase_modifiedby"));
}
public Displaystrings lk_DisplayStringbase_modifiedonbehalfby() {
return new Displaystrings(contextPath.addSegment("lk_DisplayStringbase_modifiedonbehalfby"));
}
public Documenttemplates lk_documenttemplatebase_createdby() {
return new Documenttemplates(contextPath.addSegment("lk_documenttemplatebase_createdby"));
}
public Documenttemplates lk_documenttemplatebase_createdonbehalfby() {
return new Documenttemplates(contextPath.addSegment("lk_documenttemplatebase_createdonbehalfby"));
}
public Documenttemplates lk_documenttemplatebase_modifiedby() {
return new Documenttemplates(contextPath.addSegment("lk_documenttemplatebase_modifiedby"));
}
public Documenttemplates lk_documenttemplatebase_modifiedonbehalfby() {
return new Documenttemplates(contextPath.addSegment("lk_documenttemplatebase_modifiedonbehalfby"));
}
public Duplicaterules lk_duplicaterule_createdonbehalfby() {
return new Duplicaterules(contextPath.addSegment("lk_duplicaterule_createdonbehalfby"));
}
public Duplicaterules lk_duplicaterule_modifiedonbehalfby() {
return new Duplicaterules(contextPath.addSegment("lk_duplicaterule_modifiedonbehalfby"));
}
public Duplicaterules lk_duplicaterulebase_createdby() {
return new Duplicaterules(contextPath.addSegment("lk_duplicaterulebase_createdby"));
}
public Duplicaterules lk_duplicaterulebase_modifiedby() {
return new Duplicaterules(contextPath.addSegment("lk_duplicaterulebase_modifiedby"));
}
public Duplicateruleconditions lk_duplicaterulecondition_createdonbehalfby() {
return new Duplicateruleconditions(contextPath.addSegment("lk_duplicaterulecondition_createdonbehalfby"));
}
public Duplicateruleconditions lk_duplicaterulecondition_modifiedonbehalfby() {
return new Duplicateruleconditions(contextPath.addSegment("lk_duplicaterulecondition_modifiedonbehalfby"));
}
public Duplicateruleconditions lk_duplicateruleconditionbase_createdby() {
return new Duplicateruleconditions(contextPath.addSegment("lk_duplicateruleconditionbase_createdby"));
}
public Duplicateruleconditions lk_duplicateruleconditionbase_modifiedby() {
return new Duplicateruleconditions(contextPath.addSegment("lk_duplicateruleconditionbase_modifiedby"));
}
public Emails lk_email_createdby() {
return new Emails(contextPath.addSegment("lk_email_createdby"));
}
public Emails lk_email_createdonbehalfby() {
return new Emails(contextPath.addSegment("lk_email_createdonbehalfby"));
}
public Emails lk_email_modifiedby() {
return new Emails(contextPath.addSegment("lk_email_modifiedby"));
}
public Emails lk_email_modifiedonbehalfby() {
return new Emails(contextPath.addSegment("lk_email_modifiedonbehalfby"));
}
public Emailserverprofiles lk_emailserverprofile_createdby() {
return new Emailserverprofiles(contextPath.addSegment("lk_emailserverprofile_createdby"));
}
public Emailserverprofiles lk_emailserverprofile_createdonbehalfby() {
return new Emailserverprofiles(contextPath.addSegment("lk_emailserverprofile_createdonbehalfby"));
}
public Emailserverprofiles lk_emailserverprofile_modifiedby() {
return new Emailserverprofiles(contextPath.addSegment("lk_emailserverprofile_modifiedby"));
}
public Emailserverprofiles lk_emailserverprofile_modifiedonbehalfby() {
return new Emailserverprofiles(contextPath.addSegment("lk_emailserverprofile_modifiedonbehalfby"));
}
public Environmentvariabledefinitions lk_environmentvariabledefinition_createdby() {
return new Environmentvariabledefinitions(contextPath.addSegment("lk_environmentvariabledefinition_createdby"));
}
public Environmentvariabledefinitions lk_environmentvariabledefinition_createdonbehalfby() {
return new Environmentvariabledefinitions(contextPath.addSegment("lk_environmentvariabledefinition_createdonbehalfby"));
}
public Environmentvariabledefinitions lk_environmentvariabledefinition_modifiedby() {
return new Environmentvariabledefinitions(contextPath.addSegment("lk_environmentvariabledefinition_modifiedby"));
}
public Environmentvariabledefinitions lk_environmentvariabledefinition_modifiedonbehalfby() {
return new Environmentvariabledefinitions(contextPath.addSegment("lk_environmentvariabledefinition_modifiedonbehalfby"));
}
public Environmentvariablevalues lk_environmentvariablevalue_createdby() {
return new Environmentvariablevalues(contextPath.addSegment("lk_environmentvariablevalue_createdby"));
}
public Environmentvariablevalues lk_environmentvariablevalue_createdonbehalfby() {
return new Environmentvariablevalues(contextPath.addSegment("lk_environmentvariablevalue_createdonbehalfby"));
}
public Environmentvariablevalues lk_environmentvariablevalue_modifiedby() {
return new Environmentvariablevalues(contextPath.addSegment("lk_environmentvariablevalue_modifiedby"));
}
public Environmentvariablevalues lk_environmentvariablevalue_modifiedonbehalfby() {
return new Environmentvariablevalues(contextPath.addSegment("lk_environmentvariablevalue_modifiedonbehalfby"));
}
public Expiredprocesses lk_expiredprocess_createdby() {
return new Expiredprocesses(contextPath.addSegment("lk_expiredprocess_createdby"));
}
public Expiredprocesses lk_expiredprocess_createdonbehalfby() {
return new Expiredprocesses(contextPath.addSegment("lk_expiredprocess_createdonbehalfby"));
}
public Expiredprocesses lk_expiredprocess_modifiedby() {
return new Expiredprocesses(contextPath.addSegment("lk_expiredprocess_modifiedby"));
}
public Expiredprocesses lk_expiredprocess_modifiedonbehalfby() {
return new Expiredprocesses(contextPath.addSegment("lk_expiredprocess_modifiedonbehalfby"));
}
public Exportsolutionuploads lk_exportsolutionupload_createdby() {
return new Exportsolutionuploads(contextPath.addSegment("lk_exportsolutionupload_createdby"));
}
public Exportsolutionuploads lk_exportsolutionupload_createdonbehalfby() {
return new Exportsolutionuploads(contextPath.addSegment("lk_exportsolutionupload_createdonbehalfby"));
}
public Exportsolutionuploads lk_exportsolutionupload_modifiedby() {
return new Exportsolutionuploads(contextPath.addSegment("lk_exportsolutionupload_modifiedby"));
}
public Exportsolutionuploads lk_exportsolutionupload_modifiedonbehalfby() {
return new Exportsolutionuploads(contextPath.addSegment("lk_exportsolutionupload_modifiedonbehalfby"));
}
public Faxes lk_fax_createdby() {
return new Faxes(contextPath.addSegment("lk_fax_createdby"));
}
public Faxes lk_fax_createdonbehalfby() {
return new Faxes(contextPath.addSegment("lk_fax_createdonbehalfby"));
}
public Faxes lk_fax_modifiedby() {
return new Faxes(contextPath.addSegment("lk_fax_modifiedby"));
}
public Faxes lk_fax_modifiedonbehalfby() {
return new Faxes(contextPath.addSegment("lk_fax_modifiedonbehalfby"));
}
public Feedback lk_feedback_closedby() {
return new Feedback(contextPath.addSegment("lk_feedback_closedby"));
}
public Feedback lk_feedback_createdby() {
return new Feedback(contextPath.addSegment("lk_feedback_createdby"));
}
public Feedback lk_feedback_createdonbehalfby() {
return new Feedback(contextPath.addSegment("lk_feedback_createdonbehalfby"));
}
public Feedback lk_feedback_modifiedby() {
return new Feedback(contextPath.addSegment("lk_feedback_modifiedby"));
}
public Feedback lk_feedback_modifiedonbehalfby() {
return new Feedback(contextPath.addSegment("lk_feedback_modifiedonbehalfby"));
}
public Fieldsecurityprofiles lk_fieldsecurityprofile_createdby() {
return new Fieldsecurityprofiles(contextPath.addSegment("lk_fieldsecurityprofile_createdby"));
}
public Fieldsecurityprofiles lk_fieldsecurityprofile_createdonbehalfby() {
return new Fieldsecurityprofiles(contextPath.addSegment("lk_fieldsecurityprofile_createdonbehalfby"));
}
public Fieldsecurityprofiles lk_fieldsecurityprofile_modifiedby() {
return new Fieldsecurityprofiles(contextPath.addSegment("lk_fieldsecurityprofile_modifiedby"));
}
public Fieldsecurityprofiles lk_fieldsecurityprofile_modifiedonbehalfby() {
return new Fieldsecurityprofiles(contextPath.addSegment("lk_fieldsecurityprofile_modifiedonbehalfby"));
}
public Fixedmonthlyfiscalcalendars lk_fixedmonthlyfiscalcalendar_createdby() {
return new Fixedmonthlyfiscalcalendars(contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_createdby"));
}
public Fixedmonthlyfiscalcalendars lk_fixedmonthlyfiscalcalendar_createdonbehalfby() {
return new Fixedmonthlyfiscalcalendars(contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_createdonbehalfby"));
}
public Fixedmonthlyfiscalcalendars lk_fixedmonthlyfiscalcalendar_modifiedby() {
return new Fixedmonthlyfiscalcalendars(contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_modifiedby"));
}
public Fixedmonthlyfiscalcalendars lk_fixedmonthlyfiscalcalendar_modifiedonbehalfby() {
return new Fixedmonthlyfiscalcalendars(contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_modifiedonbehalfby"));
}
public Fixedmonthlyfiscalcalendars lk_fixedmonthlyfiscalcalendar_salespersonid() {
return new Fixedmonthlyfiscalcalendars(contextPath.addSegment("lk_fixedmonthlyfiscalcalendar_salespersonid"));
}
public Flowsessions lk_flowsession_createdby() {
return new Flowsessions(contextPath.addSegment("lk_flowsession_createdby"));
}
public Flowsessions lk_flowsession_createdonbehalfby() {
return new Flowsessions(contextPath.addSegment("lk_flowsession_createdonbehalfby"));
}
public Flowsessions lk_flowsession_modifiedby() {
return new Flowsessions(contextPath.addSegment("lk_flowsession_modifiedby"));
}
public Flowsessions lk_flowsession_modifiedonbehalfby() {
return new Flowsessions(contextPath.addSegment("lk_flowsession_modifiedonbehalfby"));
}
public Ggw_crews lk_ggw_crew_createdby() {
return new Ggw_crews(contextPath.addSegment("lk_ggw_crew_createdby"));
}
public Ggw_crews lk_ggw_crew_createdonbehalfby() {
return new Ggw_crews(contextPath.addSegment("lk_ggw_crew_createdonbehalfby"));
}
public Ggw_crews lk_ggw_crew_modifiedby() {
return new Ggw_crews(contextPath.addSegment("lk_ggw_crew_modifiedby"));
}
public Ggw_crews lk_ggw_crew_modifiedonbehalfby() {
return new Ggw_crews(contextPath.addSegment("lk_ggw_crew_modifiedonbehalfby"));
}
public Ggw_events lk_ggw_event_createdby() {
return new Ggw_events(contextPath.addSegment("lk_ggw_event_createdby"));
}
public Ggw_events lk_ggw_event_createdonbehalfby() {
return new Ggw_events(contextPath.addSegment("lk_ggw_event_createdonbehalfby"));
}
public Ggw_events lk_ggw_event_modifiedby() {
return new Ggw_events(contextPath.addSegment("lk_ggw_event_modifiedby"));
}
public Ggw_events lk_ggw_event_modifiedonbehalfby() {
return new Ggw_events(contextPath.addSegment("lk_ggw_event_modifiedonbehalfby"));
}
public Ggw_team_applications lk_ggw_team_application_createdby() {
return new Ggw_team_applications(contextPath.addSegment("lk_ggw_team_application_createdby"));
}
public Ggw_team_applications lk_ggw_team_application_createdonbehalfby() {
return new Ggw_team_applications(contextPath.addSegment("lk_ggw_team_application_createdonbehalfby"));
}
public Ggw_team_applications lk_ggw_team_application_modifiedby() {
return new Ggw_team_applications(contextPath.addSegment("lk_ggw_team_application_modifiedby"));
}
public Ggw_team_applications lk_ggw_team_application_modifiedonbehalfby() {
return new Ggw_team_applications(contextPath.addSegment("lk_ggw_team_application_modifiedonbehalfby"));
}
public Ggw_teams lk_ggw_team_createdby() {
return new Ggw_teams(contextPath.addSegment("lk_ggw_team_createdby"));
}
public Ggw_teams lk_ggw_team_createdonbehalfby() {
return new Ggw_teams(contextPath.addSegment("lk_ggw_team_createdonbehalfby"));
}
public Ggw_teams lk_ggw_team_modifiedby() {
return new Ggw_teams(contextPath.addSegment("lk_ggw_team_modifiedby"));
}
public Ggw_teams lk_ggw_team_modifiedonbehalfby() {
return new Ggw_teams(contextPath.addSegment("lk_ggw_team_modifiedonbehalfby"));
}
public Goals lk_goal_createdby() {
return new Goals(contextPath.addSegment("lk_goal_createdby"));
}
public Goals lk_goal_createdonbehalfby() {
return new Goals(contextPath.addSegment("lk_goal_createdonbehalfby"));
}
public Goals lk_goal_modifiedby() {
return new Goals(contextPath.addSegment("lk_goal_modifiedby"));
}
public Goals lk_goal_modifiedonbehalfby() {
return new Goals(contextPath.addSegment("lk_goal_modifiedonbehalfby"));
}
public Goalrollupqueries lk_goalrollupquery_createdby() {
return new Goalrollupqueries(contextPath.addSegment("lk_goalrollupquery_createdby"));
}
public Goalrollupqueries lk_goalrollupquery_createdonbehalfby() {
return new Goalrollupqueries(contextPath.addSegment("lk_goalrollupquery_createdonbehalfby"));
}
public Goalrollupqueries lk_goalrollupquery_modifiedby() {
return new Goalrollupqueries(contextPath.addSegment("lk_goalrollupquery_modifiedby"));
}
public Goalrollupqueries lk_goalrollupquery_modifiedonbehalfby() {
return new Goalrollupqueries(contextPath.addSegment("lk_goalrollupquery_modifiedonbehalfby"));
}
public Imports lk_import_createdonbehalfby() {
return new Imports(contextPath.addSegment("lk_import_createdonbehalfby"));
}
public Imports lk_import_modifiedonbehalfby() {
return new Imports(contextPath.addSegment("lk_import_modifiedonbehalfby"));
}
public Imports lk_importbase_createdby() {
return new Imports(contextPath.addSegment("lk_importbase_createdby"));
}
public Imports lk_importbase_modifiedby() {
return new Imports(contextPath.addSegment("lk_importbase_modifiedby"));
}
public Importdataset lk_importdata_createdonbehalfby() {
return new Importdataset(contextPath.addSegment("lk_importdata_createdonbehalfby"));
}
public Importdataset lk_importdata_modifiedonbehalfby() {
return new Importdataset(contextPath.addSegment("lk_importdata_modifiedonbehalfby"));
}
public Importdataset lk_importdatabase_createdby() {
return new Importdataset(contextPath.addSegment("lk_importdatabase_createdby"));
}
public Importdataset lk_importdatabase_modifiedby() {
return new Importdataset(contextPath.addSegment("lk_importdatabase_modifiedby"));
}
public Importentitymappings lk_importentitymapping_createdby() {
return new Importentitymappings(contextPath.addSegment("lk_importentitymapping_createdby"));
}
public Importentitymappings lk_importentitymapping_createdonbehalfby() {
return new Importentitymappings(contextPath.addSegment("lk_importentitymapping_createdonbehalfby"));
}
public Importentitymappings lk_importentitymapping_modifiedby() {
return new Importentitymappings(contextPath.addSegment("lk_importentitymapping_modifiedby"));
}
public Importentitymappings lk_importentitymapping_modifiedonbehalfby() {
return new Importentitymappings(contextPath.addSegment("lk_importentitymapping_modifiedonbehalfby"));
}
public Importfiles lk_importfilebase_createdby() {
return new Importfiles(contextPath.addSegment("lk_importfilebase_createdby"));
}
public Importfiles lk_importfilebase_createdonbehalfby() {
return new Importfiles(contextPath.addSegment("lk_importfilebase_createdonbehalfby"));
}
public Importfiles lk_importfilebase_modifiedby() {
return new Importfiles(contextPath.addSegment("lk_importfilebase_modifiedby"));
}
public Importfiles lk_importfilebase_modifiedonbehalfby() {
return new Importfiles(contextPath.addSegment("lk_importfilebase_modifiedonbehalfby"));
}
public Importjobs lk_importjobbase_createdby() {
return new Importjobs(contextPath.addSegment("lk_importjobbase_createdby"));
}
public Importjobs lk_importjobbase_createdonbehalfby() {
return new Importjobs(contextPath.addSegment("lk_importjobbase_createdonbehalfby"));
}
public Importjobs lk_importjobbase_modifiedby() {
return new Importjobs(contextPath.addSegment("lk_importjobbase_modifiedby"));
}
public Importjobs lk_importjobbase_modifiedonbehalfby() {
return new Importjobs(contextPath.addSegment("lk_importjobbase_modifiedonbehalfby"));
}
public Importlogs lk_importlog_createdonbehalfby() {
return new Importlogs(contextPath.addSegment("lk_importlog_createdonbehalfby"));
}
public Importlogs lk_importlog_modifiedonbehalfby() {
return new Importlogs(contextPath.addSegment("lk_importlog_modifiedonbehalfby"));
}
public Importlogs lk_importlogbase_createdby() {
return new Importlogs(contextPath.addSegment("lk_importlogbase_createdby"));
}
public Importlogs lk_importlogbase_modifiedby() {
return new Importlogs(contextPath.addSegment("lk_importlogbase_modifiedby"));
}
public Importmaps lk_importmap_createdonbehalfby() {
return new Importmaps(contextPath.addSegment("lk_importmap_createdonbehalfby"));
}
public Importmaps lk_importmap_modifiedonbehalfby() {
return new Importmaps(contextPath.addSegment("lk_importmap_modifiedonbehalfby"));
}
public Importmaps lk_importmapbase_createdby() {
return new Importmaps(contextPath.addSegment("lk_importmapbase_createdby"));
}
public Importmaps lk_importmapbase_modifiedby() {
return new Importmaps(contextPath.addSegment("lk_importmapbase_modifiedby"));
}
public Kbarticles lk_kbarticle_createdonbehalfby() {
return new Kbarticles(contextPath.addSegment("lk_kbarticle_createdonbehalfby"));
}
public Kbarticles lk_kbarticle_modifiedonbehalfby() {
return new Kbarticles(contextPath.addSegment("lk_kbarticle_modifiedonbehalfby"));
}
public Kbarticles lk_kbarticlebase_createdby() {
return new Kbarticles(contextPath.addSegment("lk_kbarticlebase_createdby"));
}
public Kbarticles lk_kbarticlebase_modifiedby() {
return new Kbarticles(contextPath.addSegment("lk_kbarticlebase_modifiedby"));
}
public Kbarticlecomments lk_kbarticlecomment_createdonbehalfby() {
return new Kbarticlecomments(contextPath.addSegment("lk_kbarticlecomment_createdonbehalfby"));
}
public Kbarticlecomments lk_kbarticlecomment_modifiedonbehalfby() {
return new Kbarticlecomments(contextPath.addSegment("lk_kbarticlecomment_modifiedonbehalfby"));
}
public Kbarticlecomments lk_kbarticlecommentbase_createdby() {
return new Kbarticlecomments(contextPath.addSegment("lk_kbarticlecommentbase_createdby"));
}
public Kbarticlecomments lk_kbarticlecommentbase_modifiedby() {
return new Kbarticlecomments(contextPath.addSegment("lk_kbarticlecommentbase_modifiedby"));
}
public Kbarticletemplates lk_kbarticletemplate_createdonbehalfby() {
return new Kbarticletemplates(contextPath.addSegment("lk_kbarticletemplate_createdonbehalfby"));
}
public Kbarticletemplates lk_kbarticletemplate_modifiedonbehalfby() {
return new Kbarticletemplates(contextPath.addSegment("lk_kbarticletemplate_modifiedonbehalfby"));
}
public Kbarticletemplates lk_kbarticletemplatebase_createdby() {
return new Kbarticletemplates(contextPath.addSegment("lk_kbarticletemplatebase_createdby"));
}
public Kbarticletemplates lk_kbarticletemplatebase_modifiedby() {
return new Kbarticletemplates(contextPath.addSegment("lk_kbarticletemplatebase_modifiedby"));
}
public Knowledgearticles lk_knowledgearticle_createdby() {
return new Knowledgearticles(contextPath.addSegment("lk_knowledgearticle_createdby"));
}
public Knowledgearticles lk_knowledgearticle_createdonbehalfby() {
return new Knowledgearticles(contextPath.addSegment("lk_knowledgearticle_createdonbehalfby"));
}
public Knowledgearticles lk_knowledgearticle_modifiedby() {
return new Knowledgearticles(contextPath.addSegment("lk_knowledgearticle_modifiedby"));
}
public Knowledgearticles lk_knowledgearticle_modifiedonbehalfby() {
return new Knowledgearticles(contextPath.addSegment("lk_knowledgearticle_modifiedonbehalfby"));
}
public Knowledgearticleviews lk_knowledgearticleviews_createdby() {
return new Knowledgearticleviews(contextPath.addSegment("lk_knowledgearticleviews_createdby"));
}
public Knowledgearticleviews lk_knowledgearticleviews_createdonbehalfby() {
return new Knowledgearticleviews(contextPath.addSegment("lk_knowledgearticleviews_createdonbehalfby"));
}
public Knowledgearticleviews lk_knowledgearticleviews_modifiedby() {
return new Knowledgearticleviews(contextPath.addSegment("lk_knowledgearticleviews_modifiedby"));
}
public Knowledgearticleviews lk_knowledgearticleviews_modifiedonbehalfby() {
return new Knowledgearticleviews(contextPath.addSegment("lk_knowledgearticleviews_modifiedonbehalfby"));
}
public Knowledgebaserecords lk_KnowledgeBaseRecord_createdby() {
return new Knowledgebaserecords(contextPath.addSegment("lk_KnowledgeBaseRecord_createdby"));
}
public Knowledgebaserecords lk_KnowledgeBaseRecord_createdonbehalfby() {
return new Knowledgebaserecords(contextPath.addSegment("lk_KnowledgeBaseRecord_createdonbehalfby"));
}
public Knowledgebaserecords lk_KnowledgeBaseRecord_modifiedby() {
return new Knowledgebaserecords(contextPath.addSegment("lk_KnowledgeBaseRecord_modifiedby"));
}
public Knowledgebaserecords lk_KnowledgeBaseRecord_modifiedonbehalfby() {
return new Knowledgebaserecords(contextPath.addSegment("lk_KnowledgeBaseRecord_modifiedonbehalfby"));
}
public Letters lk_letter_createdby() {
return new Letters(contextPath.addSegment("lk_letter_createdby"));
}
public Letters lk_letter_createdonbehalfby() {
return new Letters(contextPath.addSegment("lk_letter_createdonbehalfby"));
}
public Letters lk_letter_modifiedby() {
return new Letters(contextPath.addSegment("lk_letter_modifiedby"));
}
public Letters lk_letter_modifiedonbehalfby() {
return new Letters(contextPath.addSegment("lk_letter_modifiedonbehalfby"));
}
public Lookupmappings lk_lookupmapping_createdby() {
return new Lookupmappings(contextPath.addSegment("lk_lookupmapping_createdby"));
}
public Lookupmappings lk_lookupmapping_createdonbehalfby() {
return new Lookupmappings(contextPath.addSegment("lk_lookupmapping_createdonbehalfby"));
}
public Lookupmappings lk_lookupmapping_modifiedby() {
return new Lookupmappings(contextPath.addSegment("lk_lookupmapping_modifiedby"));
}
public Lookupmappings lk_lookupmapping_modifiedonbehalfby() {
return new Lookupmappings(contextPath.addSegment("lk_lookupmapping_modifiedonbehalfby"));
}
public Mailboxes lk_mailbox_createdby() {
return new Mailboxes(contextPath.addSegment("lk_mailbox_createdby"));
}
public Mailboxes lk_mailbox_createdonbehalfby() {
return new Mailboxes(contextPath.addSegment("lk_mailbox_createdonbehalfby"));
}
public Mailboxes lk_mailbox_modifiedby() {
return new Mailboxes(contextPath.addSegment("lk_mailbox_modifiedby"));
}
public Mailboxes lk_mailbox_modifiedonbehalfby() {
return new Mailboxes(contextPath.addSegment("lk_mailbox_modifiedonbehalfby"));
}
public Mailboxtrackingfolders lk_mailboxtrackingfolder_createdby() {
return new Mailboxtrackingfolders(contextPath.addSegment("lk_mailboxtrackingfolder_createdby"));
}
public Mailboxtrackingfolders lk_mailboxtrackingfolder_createdonbehalfby() {
return new Mailboxtrackingfolders(contextPath.addSegment("lk_mailboxtrackingfolder_createdonbehalfby"));
}
public Mailboxtrackingfolders lk_mailboxtrackingfolder_modifiedby() {
return new Mailboxtrackingfolders(contextPath.addSegment("lk_mailboxtrackingfolder_modifiedby"));
}
public Mailboxtrackingfolders lk_mailboxtrackingfolder_modifiedonbehalfby() {
return new Mailboxtrackingfolders(contextPath.addSegment("lk_mailboxtrackingfolder_modifiedonbehalfby"));
}
public Mailmergetemplates lk_mailmergetemplate_createdonbehalfby() {
return new Mailmergetemplates(contextPath.addSegment("lk_mailmergetemplate_createdonbehalfby"));
}
public Mailmergetemplates lk_mailmergetemplate_modifiedonbehalfby() {
return new Mailmergetemplates(contextPath.addSegment("lk_mailmergetemplate_modifiedonbehalfby"));
}
public Mailmergetemplates lk_mailmergetemplatebase_createdby() {
return new Mailmergetemplates(contextPath.addSegment("lk_mailmergetemplatebase_createdby"));
}
public Mailmergetemplates lk_mailmergetemplatebase_modifiedby() {
return new Mailmergetemplates(contextPath.addSegment("lk_mailmergetemplatebase_modifiedby"));
}
public Metrics lk_metric_createdby() {
return new Metrics(contextPath.addSegment("lk_metric_createdby"));
}
public Metrics lk_metric_createdonbehalfby() {
return new Metrics(contextPath.addSegment("lk_metric_createdonbehalfby"));
}
public Metrics lk_metric_modifiedby() {
return new Metrics(contextPath.addSegment("lk_metric_modifiedby"));
}
public Metrics lk_metric_modifiedonbehalfby() {
return new Metrics(contextPath.addSegment("lk_metric_modifiedonbehalfby"));
}
public Mobileofflineprofiles lk_MobileOfflineProfile_createdby() {
return new Mobileofflineprofiles(contextPath.addSegment("lk_MobileOfflineProfile_createdby"));
}
public Mobileofflineprofiles lk_MobileOfflineProfile_createdonbehalfby() {
return new Mobileofflineprofiles(contextPath.addSegment("lk_MobileOfflineProfile_createdonbehalfby"));
}
public Mobileofflineprofiles lk_MobileOfflineProfile_modifiedby() {
return new Mobileofflineprofiles(contextPath.addSegment("lk_MobileOfflineProfile_modifiedby"));
}
public Mobileofflineprofiles lk_MobileOfflineProfile_modifiedonbehalfby() {
return new Mobileofflineprofiles(contextPath.addSegment("lk_MobileOfflineProfile_modifiedonbehalfby"));
}
public Mobileofflineprofileitems lk_MobileOfflineProfileItem_createdby() {
return new Mobileofflineprofileitems(contextPath.addSegment("lk_MobileOfflineProfileItem_createdby"));
}
public Mobileofflineprofileitems lk_mobileofflineprofileitem_createdonbehalfby() {
return new Mobileofflineprofileitems(contextPath.addSegment("lk_mobileofflineprofileitem_createdonbehalfby"));
}
public Mobileofflineprofileitems lk_mobileofflineprofileitem_modifiedby() {
return new Mobileofflineprofileitems(contextPath.addSegment("lk_mobileofflineprofileitem_modifiedby"));
}
public Mobileofflineprofileitems lk_mobileofflineprofileitem_modifiedonbehalfby() {
return new Mobileofflineprofileitems(contextPath.addSegment("lk_mobileofflineprofileitem_modifiedonbehalfby"));
}
public Mobileofflineprofileitemassociations lk_mobileofflineprofileitemassocaition_modifiedby() {
return new Mobileofflineprofileitemassociations(contextPath.addSegment("lk_mobileofflineprofileitemassocaition_modifiedby"));
}
public Mobileofflineprofileitemassociations lk_mobileofflineprofileitemassocaition_modifiedonbehalfby() {
return new Mobileofflineprofileitemassociations(contextPath.addSegment("lk_mobileofflineprofileitemassocaition_modifiedonbehalfby"));
}
public Mobileofflineprofileitemassociations lk_MobileOfflineProfileItemAssociation_createdby() {
return new Mobileofflineprofileitemassociations(contextPath.addSegment("lk_MobileOfflineProfileItemAssociation_createdby"));
}
public Mobileofflineprofileitemassociations lk_mobileofflineprofileitemassociation_createdonbehalfby() {
return new Mobileofflineprofileitemassociations(contextPath.addSegment("lk_mobileofflineprofileitemassociation_createdonbehalfby"));
}
public Monthlyfiscalcalendars lk_monthlyfiscalcalendar_createdby() {
return new Monthlyfiscalcalendars(contextPath.addSegment("lk_monthlyfiscalcalendar_createdby"));
}
public Monthlyfiscalcalendars lk_monthlyfiscalcalendar_createdonbehalfby() {
return new Monthlyfiscalcalendars(contextPath.addSegment("lk_monthlyfiscalcalendar_createdonbehalfby"));
}
public Monthlyfiscalcalendars lk_monthlyfiscalcalendar_modifiedby() {
return new Monthlyfiscalcalendars(contextPath.addSegment("lk_monthlyfiscalcalendar_modifiedby"));
}
public Monthlyfiscalcalendars lk_monthlyfiscalcalendar_modifiedonbehalfby() {
return new Monthlyfiscalcalendars(contextPath.addSegment("lk_monthlyfiscalcalendar_modifiedonbehalfby"));
}
public Monthlyfiscalcalendars lk_monthlyfiscalcalendar_salespersonid() {
return new Monthlyfiscalcalendars(contextPath.addSegment("lk_monthlyfiscalcalendar_salespersonid"));
}
public Msdyn_aibdatasets lk_msdyn_aibdataset_createdby() {
return new Msdyn_aibdatasets(contextPath.addSegment("lk_msdyn_aibdataset_createdby"));
}
public Msdyn_aibdatasets lk_msdyn_aibdataset_createdonbehalfby() {
return new Msdyn_aibdatasets(contextPath.addSegment("lk_msdyn_aibdataset_createdonbehalfby"));
}
public Msdyn_aibdatasets lk_msdyn_aibdataset_modifiedby() {
return new Msdyn_aibdatasets(contextPath.addSegment("lk_msdyn_aibdataset_modifiedby"));
}
public Msdyn_aibdatasets lk_msdyn_aibdataset_modifiedonbehalfby() {
return new Msdyn_aibdatasets(contextPath.addSegment("lk_msdyn_aibdataset_modifiedonbehalfby"));
}
public Msdyn_aibdatasetfiles lk_msdyn_aibdatasetfile_createdby() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("lk_msdyn_aibdatasetfile_createdby"));
}
public Msdyn_aibdatasetfiles lk_msdyn_aibdatasetfile_createdonbehalfby() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("lk_msdyn_aibdatasetfile_createdonbehalfby"));
}
public Msdyn_aibdatasetfiles lk_msdyn_aibdatasetfile_modifiedby() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("lk_msdyn_aibdatasetfile_modifiedby"));
}
public Msdyn_aibdatasetfiles lk_msdyn_aibdatasetfile_modifiedonbehalfby() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("lk_msdyn_aibdatasetfile_modifiedonbehalfby"));
}
public Msdyn_aibdatasetrecords lk_msdyn_aibdatasetrecord_createdby() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("lk_msdyn_aibdatasetrecord_createdby"));
}
public Msdyn_aibdatasetrecords lk_msdyn_aibdatasetrecord_createdonbehalfby() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("lk_msdyn_aibdatasetrecord_createdonbehalfby"));
}
public Msdyn_aibdatasetrecords lk_msdyn_aibdatasetrecord_modifiedby() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("lk_msdyn_aibdatasetrecord_modifiedby"));
}
public Msdyn_aibdatasetrecords lk_msdyn_aibdatasetrecord_modifiedonbehalfby() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("lk_msdyn_aibdatasetrecord_modifiedonbehalfby"));
}
public Msdyn_aibdatasetscontainers lk_msdyn_aibdatasetscontainer_createdby() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("lk_msdyn_aibdatasetscontainer_createdby"));
}
public Msdyn_aibdatasetscontainers lk_msdyn_aibdatasetscontainer_createdonbehalfby() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("lk_msdyn_aibdatasetscontainer_createdonbehalfby"));
}
public Msdyn_aibdatasetscontainers lk_msdyn_aibdatasetscontainer_modifiedby() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("lk_msdyn_aibdatasetscontainer_modifiedby"));
}
public Msdyn_aibdatasetscontainers lk_msdyn_aibdatasetscontainer_modifiedonbehalfby() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("lk_msdyn_aibdatasetscontainer_modifiedonbehalfby"));
}
public Msdyn_aibfiles lk_msdyn_aibfile_createdby() {
return new Msdyn_aibfiles(contextPath.addSegment("lk_msdyn_aibfile_createdby"));
}
public Msdyn_aibfiles lk_msdyn_aibfile_createdonbehalfby() {
return new Msdyn_aibfiles(contextPath.addSegment("lk_msdyn_aibfile_createdonbehalfby"));
}
public Msdyn_aibfiles lk_msdyn_aibfile_modifiedby() {
return new Msdyn_aibfiles(contextPath.addSegment("lk_msdyn_aibfile_modifiedby"));
}
public Msdyn_aibfiles lk_msdyn_aibfile_modifiedonbehalfby() {
return new Msdyn_aibfiles(contextPath.addSegment("lk_msdyn_aibfile_modifiedonbehalfby"));
}
public Msdyn_aibfileattacheddatas lk_msdyn_aibfileattacheddata_createdby() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("lk_msdyn_aibfileattacheddata_createdby"));
}
public Msdyn_aibfileattacheddatas lk_msdyn_aibfileattacheddata_createdonbehalfby() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("lk_msdyn_aibfileattacheddata_createdonbehalfby"));
}
public Msdyn_aibfileattacheddatas lk_msdyn_aibfileattacheddata_modifiedby() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("lk_msdyn_aibfileattacheddata_modifiedby"));
}
public Msdyn_aibfileattacheddatas lk_msdyn_aibfileattacheddata_modifiedonbehalfby() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("lk_msdyn_aibfileattacheddata_modifiedonbehalfby"));
}
public Msdyn_aiconfigurations lk_msdyn_aiconfiguration_createdby() {
return new Msdyn_aiconfigurations(contextPath.addSegment("lk_msdyn_aiconfiguration_createdby"));
}
public Msdyn_aiconfigurations lk_msdyn_aiconfiguration_createdonbehalfby() {
return new Msdyn_aiconfigurations(contextPath.addSegment("lk_msdyn_aiconfiguration_createdonbehalfby"));
}
public Msdyn_aiconfigurations lk_msdyn_aiconfiguration_modifiedby() {
return new Msdyn_aiconfigurations(contextPath.addSegment("lk_msdyn_aiconfiguration_modifiedby"));
}
public Msdyn_aiconfigurations lk_msdyn_aiconfiguration_modifiedonbehalfby() {
return new Msdyn_aiconfigurations(contextPath.addSegment("lk_msdyn_aiconfiguration_modifiedonbehalfby"));
}
public Msdyn_aifptrainingdocuments lk_msdyn_aifptrainingdocument_createdby() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("lk_msdyn_aifptrainingdocument_createdby"));
}
public Msdyn_aifptrainingdocuments lk_msdyn_aifptrainingdocument_createdonbehalfby() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("lk_msdyn_aifptrainingdocument_createdonbehalfby"));
}
public Msdyn_aifptrainingdocuments lk_msdyn_aifptrainingdocument_modifiedby() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("lk_msdyn_aifptrainingdocument_modifiedby"));
}
public Msdyn_aifptrainingdocuments lk_msdyn_aifptrainingdocument_modifiedonbehalfby() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("lk_msdyn_aifptrainingdocument_modifiedonbehalfby"));
}
public Msdyn_aimodels lk_msdyn_aimodel_createdby() {
return new Msdyn_aimodels(contextPath.addSegment("lk_msdyn_aimodel_createdby"));
}
public Msdyn_aimodels lk_msdyn_aimodel_createdonbehalfby() {
return new Msdyn_aimodels(contextPath.addSegment("lk_msdyn_aimodel_createdonbehalfby"));
}
public Msdyn_aimodels lk_msdyn_aimodel_modifiedby() {
return new Msdyn_aimodels(contextPath.addSegment("lk_msdyn_aimodel_modifiedby"));
}
public Msdyn_aimodels lk_msdyn_aimodel_modifiedonbehalfby() {
return new Msdyn_aimodels(contextPath.addSegment("lk_msdyn_aimodel_modifiedonbehalfby"));
}
public Msdyn_aiodimages lk_msdyn_aiodimage_createdby() {
return new Msdyn_aiodimages(contextPath.addSegment("lk_msdyn_aiodimage_createdby"));
}
public Msdyn_aiodimages lk_msdyn_aiodimage_createdonbehalfby() {
return new Msdyn_aiodimages(contextPath.addSegment("lk_msdyn_aiodimage_createdonbehalfby"));
}
public Msdyn_aiodimages lk_msdyn_aiodimage_modifiedby() {
return new Msdyn_aiodimages(contextPath.addSegment("lk_msdyn_aiodimage_modifiedby"));
}
public Msdyn_aiodimages lk_msdyn_aiodimage_modifiedonbehalfby() {
return new Msdyn_aiodimages(contextPath.addSegment("lk_msdyn_aiodimage_modifiedonbehalfby"));
}
public Msdyn_aiodlabels lk_msdyn_aiodlabel_createdby() {
return new Msdyn_aiodlabels(contextPath.addSegment("lk_msdyn_aiodlabel_createdby"));
}
public Msdyn_aiodlabels lk_msdyn_aiodlabel_createdonbehalfby() {
return new Msdyn_aiodlabels(contextPath.addSegment("lk_msdyn_aiodlabel_createdonbehalfby"));
}
public Msdyn_aiodlabels lk_msdyn_aiodlabel_modifiedby() {
return new Msdyn_aiodlabels(contextPath.addSegment("lk_msdyn_aiodlabel_modifiedby"));
}
public Msdyn_aiodlabels lk_msdyn_aiodlabel_modifiedonbehalfby() {
return new Msdyn_aiodlabels(contextPath.addSegment("lk_msdyn_aiodlabel_modifiedonbehalfby"));
}
public Msdyn_aiodtrainingboundingboxes lk_msdyn_aiodtrainingboundingbox_createdby() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_createdby"));
}
public Msdyn_aiodtrainingboundingboxes lk_msdyn_aiodtrainingboundingbox_createdonbehalfby() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_createdonbehalfby"));
}
public Msdyn_aiodtrainingboundingboxes lk_msdyn_aiodtrainingboundingbox_modifiedby() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_modifiedby"));
}
public Msdyn_aiodtrainingboundingboxes lk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("lk_msdyn_aiodtrainingboundingbox_modifiedonbehalfby"));
}
public Msdyn_aiodtrainingimages lk_msdyn_aiodtrainingimage_createdby() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("lk_msdyn_aiodtrainingimage_createdby"));
}
public Msdyn_aiodtrainingimages lk_msdyn_aiodtrainingimage_createdonbehalfby() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("lk_msdyn_aiodtrainingimage_createdonbehalfby"));
}
public Msdyn_aiodtrainingimages lk_msdyn_aiodtrainingimage_modifiedby() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("lk_msdyn_aiodtrainingimage_modifiedby"));
}
public Msdyn_aiodtrainingimages lk_msdyn_aiodtrainingimage_modifiedonbehalfby() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("lk_msdyn_aiodtrainingimage_modifiedonbehalfby"));
}
public Msdyn_aitemplates lk_msdyn_aitemplate_createdby() {
return new Msdyn_aitemplates(contextPath.addSegment("lk_msdyn_aitemplate_createdby"));
}
public Msdyn_aitemplates lk_msdyn_aitemplate_createdonbehalfby() {
return new Msdyn_aitemplates(contextPath.addSegment("lk_msdyn_aitemplate_createdonbehalfby"));
}
public Msdyn_aitemplates lk_msdyn_aitemplate_modifiedby() {
return new Msdyn_aitemplates(contextPath.addSegment("lk_msdyn_aitemplate_modifiedby"));
}
public Msdyn_aitemplates lk_msdyn_aitemplate_modifiedonbehalfby() {
return new Msdyn_aitemplates(contextPath.addSegment("lk_msdyn_aitemplate_modifiedonbehalfby"));
}
public Msdyn_analysiscomponents lk_msdyn_analysiscomponent_createdby() {
return new Msdyn_analysiscomponents(contextPath.addSegment("lk_msdyn_analysiscomponent_createdby"));
}
public Msdyn_analysiscomponents lk_msdyn_analysiscomponent_createdonbehalfby() {
return new Msdyn_analysiscomponents(contextPath.addSegment("lk_msdyn_analysiscomponent_createdonbehalfby"));
}
public Msdyn_analysiscomponents lk_msdyn_analysiscomponent_modifiedby() {
return new Msdyn_analysiscomponents(contextPath.addSegment("lk_msdyn_analysiscomponent_modifiedby"));
}
public Msdyn_analysiscomponents lk_msdyn_analysiscomponent_modifiedonbehalfby() {
return new Msdyn_analysiscomponents(contextPath.addSegment("lk_msdyn_analysiscomponent_modifiedonbehalfby"));
}
public Msdyn_analysisjobs lk_msdyn_analysisjob_createdby() {
return new Msdyn_analysisjobs(contextPath.addSegment("lk_msdyn_analysisjob_createdby"));
}
public Msdyn_analysisjobs lk_msdyn_analysisjob_createdonbehalfby() {
return new Msdyn_analysisjobs(contextPath.addSegment("lk_msdyn_analysisjob_createdonbehalfby"));
}
public Msdyn_analysisjobs lk_msdyn_analysisjob_modifiedby() {
return new Msdyn_analysisjobs(contextPath.addSegment("lk_msdyn_analysisjob_modifiedby"));
}
public Msdyn_analysisjobs lk_msdyn_analysisjob_modifiedonbehalfby() {
return new Msdyn_analysisjobs(contextPath.addSegment("lk_msdyn_analysisjob_modifiedonbehalfby"));
}
public Msdyn_analysisresults lk_msdyn_analysisresult_createdby() {
return new Msdyn_analysisresults(contextPath.addSegment("lk_msdyn_analysisresult_createdby"));
}
public Msdyn_analysisresults lk_msdyn_analysisresult_createdonbehalfby() {
return new Msdyn_analysisresults(contextPath.addSegment("lk_msdyn_analysisresult_createdonbehalfby"));
}
public Msdyn_analysisresults lk_msdyn_analysisresult_modifiedby() {
return new Msdyn_analysisresults(contextPath.addSegment("lk_msdyn_analysisresult_modifiedby"));
}
public Msdyn_analysisresults lk_msdyn_analysisresult_modifiedonbehalfby() {
return new Msdyn_analysisresults(contextPath.addSegment("lk_msdyn_analysisresult_modifiedonbehalfby"));
}
public Msdyn_analysisresultdetails lk_msdyn_analysisresultdetail_createdby() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("lk_msdyn_analysisresultdetail_createdby"));
}
public Msdyn_analysisresultdetails lk_msdyn_analysisresultdetail_createdonbehalfby() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("lk_msdyn_analysisresultdetail_createdonbehalfby"));
}
public Msdyn_analysisresultdetails lk_msdyn_analysisresultdetail_modifiedby() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("lk_msdyn_analysisresultdetail_modifiedby"));
}
public Msdyn_analysisresultdetails lk_msdyn_analysisresultdetail_modifiedonbehalfby() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("lk_msdyn_analysisresultdetail_modifiedonbehalfby"));
}
public Msdyn_dataflows lk_msdyn_dataflow_createdby() {
return new Msdyn_dataflows(contextPath.addSegment("lk_msdyn_dataflow_createdby"));
}
public Msdyn_dataflows lk_msdyn_dataflow_createdonbehalfby() {
return new Msdyn_dataflows(contextPath.addSegment("lk_msdyn_dataflow_createdonbehalfby"));
}
public Msdyn_dataflows lk_msdyn_dataflow_modifiedby() {
return new Msdyn_dataflows(contextPath.addSegment("lk_msdyn_dataflow_modifiedby"));
}
public Msdyn_dataflows lk_msdyn_dataflow_modifiedonbehalfby() {
return new Msdyn_dataflows(contextPath.addSegment("lk_msdyn_dataflow_modifiedonbehalfby"));
}
public Msdyn_helppages lk_msdyn_helppage_createdby() {
return new Msdyn_helppages(contextPath.addSegment("lk_msdyn_helppage_createdby"));
}
public Msdyn_helppages lk_msdyn_helppage_createdonbehalfby() {
return new Msdyn_helppages(contextPath.addSegment("lk_msdyn_helppage_createdonbehalfby"));
}
public Msdyn_helppages lk_msdyn_helppage_modifiedby() {
return new Msdyn_helppages(contextPath.addSegment("lk_msdyn_helppage_modifiedby"));
}
public Msdyn_helppages lk_msdyn_helppage_modifiedonbehalfby() {
return new Msdyn_helppages(contextPath.addSegment("lk_msdyn_helppage_modifiedonbehalfby"));
}
public Msdyn_knowledgearticleimages lk_msdyn_knowledgearticleimage_createdby() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("lk_msdyn_knowledgearticleimage_createdby"));
}
public Msdyn_knowledgearticleimages lk_msdyn_knowledgearticleimage_createdonbehalfby() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("lk_msdyn_knowledgearticleimage_createdonbehalfby"));
}
public Msdyn_knowledgearticleimages lk_msdyn_knowledgearticleimage_modifiedby() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("lk_msdyn_knowledgearticleimage_modifiedby"));
}
public Msdyn_knowledgearticleimages lk_msdyn_knowledgearticleimage_modifiedonbehalfby() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("lk_msdyn_knowledgearticleimage_modifiedonbehalfby"));
}
public Msdyn_knowledgearticletemplates lk_msdyn_knowledgearticletemplate_createdby() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("lk_msdyn_knowledgearticletemplate_createdby"));
}
public Msdyn_knowledgearticletemplates lk_msdyn_knowledgearticletemplate_createdonbehalfby() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("lk_msdyn_knowledgearticletemplate_createdonbehalfby"));
}
public Msdyn_knowledgearticletemplates lk_msdyn_knowledgearticletemplate_modifiedby() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("lk_msdyn_knowledgearticletemplate_modifiedby"));
}
public Msdyn_knowledgearticletemplates lk_msdyn_knowledgearticletemplate_modifiedonbehalfby() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("lk_msdyn_knowledgearticletemplate_modifiedonbehalfby"));
}
public Msdyn_richtextfiles lk_msdyn_richtextfile_createdby() {
return new Msdyn_richtextfiles(contextPath.addSegment("lk_msdyn_richtextfile_createdby"));
}
public Msdyn_richtextfiles lk_msdyn_richtextfile_createdonbehalfby() {
return new Msdyn_richtextfiles(contextPath.addSegment("lk_msdyn_richtextfile_createdonbehalfby"));
}
public Msdyn_richtextfiles lk_msdyn_richtextfile_modifiedby() {
return new Msdyn_richtextfiles(contextPath.addSegment("lk_msdyn_richtextfile_modifiedby"));
}
public Msdyn_richtextfiles lk_msdyn_richtextfile_modifiedonbehalfby() {
return new Msdyn_richtextfiles(contextPath.addSegment("lk_msdyn_richtextfile_modifiedonbehalfby"));
}
public Msdyn_serviceconfigurations lk_msdyn_serviceconfiguration_createdby() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("lk_msdyn_serviceconfiguration_createdby"));
}
public Msdyn_serviceconfigurations lk_msdyn_serviceconfiguration_createdonbehalfby() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("lk_msdyn_serviceconfiguration_createdonbehalfby"));
}
public Msdyn_serviceconfigurations lk_msdyn_serviceconfiguration_modifiedby() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("lk_msdyn_serviceconfiguration_modifiedby"));
}
public Msdyn_serviceconfigurations lk_msdyn_serviceconfiguration_modifiedonbehalfby() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("lk_msdyn_serviceconfiguration_modifiedonbehalfby"));
}
public Msdyn_slakpis lk_msdyn_slakpi_createdby() {
return new Msdyn_slakpis(contextPath.addSegment("lk_msdyn_slakpi_createdby"));
}
public Msdyn_slakpis lk_msdyn_slakpi_createdonbehalfby() {
return new Msdyn_slakpis(contextPath.addSegment("lk_msdyn_slakpi_createdonbehalfby"));
}
public Msdyn_slakpis lk_msdyn_slakpi_modifiedby() {
return new Msdyn_slakpis(contextPath.addSegment("lk_msdyn_slakpi_modifiedby"));
}
public Msdyn_slakpis lk_msdyn_slakpi_modifiedonbehalfby() {
return new Msdyn_slakpis(contextPath.addSegment("lk_msdyn_slakpi_modifiedonbehalfby"));
}
public Msdyn_solutionhealthrules lk_msdyn_solutionhealthrule_createdby() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("lk_msdyn_solutionhealthrule_createdby"));
}
public Msdyn_solutionhealthrules lk_msdyn_solutionhealthrule_createdonbehalfby() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("lk_msdyn_solutionhealthrule_createdonbehalfby"));
}
public Msdyn_solutionhealthrules lk_msdyn_solutionhealthrule_modifiedby() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("lk_msdyn_solutionhealthrule_modifiedby"));
}
public Msdyn_solutionhealthrules lk_msdyn_solutionhealthrule_modifiedonbehalfby() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("lk_msdyn_solutionhealthrule_modifiedonbehalfby"));
}
public Msdyn_solutionhealthrulearguments lk_msdyn_solutionhealthruleargument_createdby() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("lk_msdyn_solutionhealthruleargument_createdby"));
}
public Msdyn_solutionhealthrulearguments lk_msdyn_solutionhealthruleargument_createdonbehalfby() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("lk_msdyn_solutionhealthruleargument_createdonbehalfby"));
}
public Msdyn_solutionhealthrulearguments lk_msdyn_solutionhealthruleargument_modifiedby() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("lk_msdyn_solutionhealthruleargument_modifiedby"));
}
public Msdyn_solutionhealthrulearguments lk_msdyn_solutionhealthruleargument_modifiedonbehalfby() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("lk_msdyn_solutionhealthruleargument_modifiedonbehalfby"));
}
public Msdyn_solutionhealthrulesets lk_msdyn_solutionhealthruleset_createdby() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("lk_msdyn_solutionhealthruleset_createdby"));
}
public Msdyn_solutionhealthrulesets lk_msdyn_solutionhealthruleset_createdonbehalfby() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("lk_msdyn_solutionhealthruleset_createdonbehalfby"));
}
public Msdyn_solutionhealthrulesets lk_msdyn_solutionhealthruleset_modifiedby() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("lk_msdyn_solutionhealthruleset_modifiedby"));
}
public Msdyn_solutionhealthrulesets lk_msdyn_solutionhealthruleset_modifiedonbehalfby() {
return new Msdyn_solutionhealthrulesets(contextPath.addSegment("lk_msdyn_solutionhealthruleset_modifiedonbehalfby"));
}
public Interactionforemails lk_new_interactionforemail_createdby() {
return new Interactionforemails(contextPath.addSegment("lk_new_interactionforemail_createdby"));
}
public Interactionforemails lk_new_interactionforemail_createdonbehalfby() {
return new Interactionforemails(contextPath.addSegment("lk_new_interactionforemail_createdonbehalfby"));
}
public Interactionforemails lk_new_interactionforemail_modifiedby() {
return new Interactionforemails(contextPath.addSegment("lk_new_interactionforemail_modifiedby"));
}
public Interactionforemails lk_new_interactionforemail_modifiedonbehalfby() {
return new Interactionforemails(contextPath.addSegment("lk_new_interactionforemail_modifiedonbehalfby"));
}
public Newprocesses lk_newprocess_createdby() {
return new Newprocesses(contextPath.addSegment("lk_newprocess_createdby"));
}
public Newprocesses lk_newprocess_createdonbehalfby() {
return new Newprocesses(contextPath.addSegment("lk_newprocess_createdonbehalfby"));
}
public Newprocesses lk_newprocess_modifiedby() {
return new Newprocesses(contextPath.addSegment("lk_newprocess_modifiedby"));
}
public Newprocesses lk_newprocess_modifiedonbehalfby() {
return new Newprocesses(contextPath.addSegment("lk_newprocess_modifiedonbehalfby"));
}
public Officegraphdocuments lk_officegraphdocument_createdonbehalfby() {
return new Officegraphdocuments(contextPath.addSegment("lk_officegraphdocument_createdonbehalfby"));
}
public Officegraphdocuments lk_officegraphdocument_modifiedonbehalfby() {
return new Officegraphdocuments(contextPath.addSegment("lk_officegraphdocument_modifiedonbehalfby"));
}
public Organizations lk_organization_createdonbehalfby() {
return new Organizations(contextPath.addSegment("lk_organization_createdonbehalfby"));
}
public Organizations lk_organization_modifiedonbehalfby() {
return new Organizations(contextPath.addSegment("lk_organization_modifiedonbehalfby"));
}
public Organizations lk_organizationbase_createdby() {
return new Organizations(contextPath.addSegment("lk_organizationbase_createdby"));
}
public Organizations lk_organizationbase_modifiedby() {
return new Organizations(contextPath.addSegment("lk_organizationbase_modifiedby"));
}
public Ownermappings lk_ownermapping_createdby() {
return new Ownermappings(contextPath.addSegment("lk_ownermapping_createdby"));
}
public Ownermappings lk_ownermapping_createdonbehalfby() {
return new Ownermappings(contextPath.addSegment("lk_ownermapping_createdonbehalfby"));
}
public Ownermappings lk_ownermapping_modifiedby() {
return new Ownermappings(contextPath.addSegment("lk_ownermapping_modifiedby"));
}
public Ownermappings lk_ownermapping_modifiedonbehalfby() {
return new Ownermappings(contextPath.addSegment("lk_ownermapping_modifiedonbehalfby"));
}
public Personaldocumenttemplates lk_personaldocumenttemplatebase_createdby() {
return new Personaldocumenttemplates(contextPath.addSegment("lk_personaldocumenttemplatebase_createdby"));
}
public Personaldocumenttemplates lk_personaldocumenttemplatebase_createdonbehalfby() {
return new Personaldocumenttemplates(contextPath.addSegment("lk_personaldocumenttemplatebase_createdonbehalfby"));
}
public Personaldocumenttemplates lk_personaldocumenttemplatebase_modifiedby() {
return new Personaldocumenttemplates(contextPath.addSegment("lk_personaldocumenttemplatebase_modifiedby"));
}
public Personaldocumenttemplates lk_personaldocumenttemplatebase_modifiedonbehalfby() {
return new Personaldocumenttemplates(contextPath.addSegment("lk_personaldocumenttemplatebase_modifiedonbehalfby"));
}
public Phonecalls lk_phonecall_createdby() {
return new Phonecalls(contextPath.addSegment("lk_phonecall_createdby"));
}
public Phonecalls lk_phonecall_createdonbehalfby() {
return new Phonecalls(contextPath.addSegment("lk_phonecall_createdonbehalfby"));
}
public Phonecalls lk_phonecall_modifiedby() {
return new Phonecalls(contextPath.addSegment("lk_phonecall_modifiedby"));
}
public Phonecalls lk_phonecall_modifiedonbehalfby() {
return new Phonecalls(contextPath.addSegment("lk_phonecall_modifiedonbehalfby"));
}
public Picklistmappings lk_picklistmapping_createdby() {
return new Picklistmappings(contextPath.addSegment("lk_picklistmapping_createdby"));
}
public Picklistmappings lk_picklistmapping_createdonbehalfby() {
return new Picklistmappings(contextPath.addSegment("lk_picklistmapping_createdonbehalfby"));
}
public Picklistmappings lk_picklistmapping_modifiedby() {
return new Picklistmappings(contextPath.addSegment("lk_picklistmapping_modifiedby"));
}
public Picklistmappings lk_picklistmapping_modifiedonbehalfby() {
return new Picklistmappings(contextPath.addSegment("lk_picklistmapping_modifiedonbehalfby"));
}
public Pluginassemblies lk_pluginassembly_createdonbehalfby() {
return new Pluginassemblies(contextPath.addSegment("lk_pluginassembly_createdonbehalfby"));
}
public Pluginassemblies lk_pluginassembly_modifiedonbehalfby() {
return new Pluginassemblies(contextPath.addSegment("lk_pluginassembly_modifiedonbehalfby"));
}
public Plugintracelogs lk_plugintracelogbase_createdonbehalfby() {
return new Plugintracelogs(contextPath.addSegment("lk_plugintracelogbase_createdonbehalfby"));
}
public Plugintypes lk_plugintype_createdonbehalfby() {
return new Plugintypes(contextPath.addSegment("lk_plugintype_createdonbehalfby"));
}
public Plugintypes lk_plugintype_modifiedonbehalfby() {
return new Plugintypes(contextPath.addSegment("lk_plugintype_modifiedonbehalfby"));
}
public Plugintypestatistics lk_plugintypestatisticbase_createdonbehalfby() {
return new Plugintypestatistics(contextPath.addSegment("lk_plugintypestatisticbase_createdonbehalfby"));
}
public Plugintypestatistics lk_plugintypestatisticbase_modifiedonbehalfby() {
return new Plugintypestatistics(contextPath.addSegment("lk_plugintypestatisticbase_modifiedonbehalfby"));
}
public Positions lk_position_createdby() {
return new Positions(contextPath.addSegment("lk_position_createdby"));
}
public Positions lk_position_createdonbehalfby() {
return new Positions(contextPath.addSegment("lk_position_createdonbehalfby"));
}
public Positions lk_position_modifiedby() {
return new Positions(contextPath.addSegment("lk_position_modifiedby"));
}
public Positions lk_position_modifiedonbehalfby() {
return new Positions(contextPath.addSegment("lk_position_modifiedonbehalfby"));
}
public Posts lk_post_createdby() {
return new Posts(contextPath.addSegment("lk_post_createdby"));
}
public Posts lk_post_createdonbehalfby() {
return new Posts(contextPath.addSegment("lk_post_createdonbehalfby"));
}
public Posts lk_post_modifiedby() {
return new Posts(contextPath.addSegment("lk_post_modifiedby"));
}
public Posts lk_post_modifiedonbehalfby() {
return new Posts(contextPath.addSegment("lk_post_modifiedonbehalfby"));
}
public Postcomments lk_postcomment_createdby() {
return new Postcomments(contextPath.addSegment("lk_postcomment_createdby"));
}
public Postcomments lk_postcomment_createdonbehalfby() {
return new Postcomments(contextPath.addSegment("lk_postcomment_createdonbehalfby"));
}
public Postfollows lk_PostFollow_createdby() {
return new Postfollows(contextPath.addSegment("lk_PostFollow_createdby"));
}
public Postfollows lk_postfollow_createdonbehalfby() {
return new Postfollows(contextPath.addSegment("lk_postfollow_createdonbehalfby"));
}
public Postlikes lk_postlike_createdby() {
return new Postlikes(contextPath.addSegment("lk_postlike_createdby"));
}
public Postlikes lk_postlike_createdonbehalfby() {
return new Postlikes(contextPath.addSegment("lk_postlike_createdonbehalfby"));
}
public Processsessions lk_processsession_canceledby() {
return new Processsessions(contextPath.addSegment("lk_processsession_canceledby"));
}
public Processsessions lk_processsession_completedby() {
return new Processsessions(contextPath.addSegment("lk_processsession_completedby"));
}
public Processsessions lk_processsession_createdby() {
return new Processsessions(contextPath.addSegment("lk_processsession_createdby"));
}
public Processsessions lk_processsession_executedby() {
return new Processsessions(contextPath.addSegment("lk_processsession_executedby"));
}
public Processsessions lk_processsession_modifiedby() {
return new Processsessions(contextPath.addSegment("lk_processsession_modifiedby"));
}
public Processsessions lk_processsession_startedby() {
return new Processsessions(contextPath.addSegment("lk_processsession_startedby"));
}
public Processsessions lk_processsessionbase_createdonbehalfby() {
return new Processsessions(contextPath.addSegment("lk_processsessionbase_createdonbehalfby"));
}
public Processsessions lk_processsessionbase_modifiedonbehalfby() {
return new Processsessions(contextPath.addSegment("lk_processsessionbase_modifiedonbehalfby"));
}
public Processstageparameters lk_processstageparameter_createdby() {
return new Processstageparameters(contextPath.addSegment("lk_processstageparameter_createdby"));
}
public Processstageparameters lk_processstageparameter_createdonbehalfby() {
return new Processstageparameters(contextPath.addSegment("lk_processstageparameter_createdonbehalfby"));
}
public Processstageparameters lk_processstageparameter_modifiedby() {
return new Processstageparameters(contextPath.addSegment("lk_processstageparameter_modifiedby"));
}
public Processstageparameters lk_processstageparameter_modifiedonbehalfby() {
return new Processstageparameters(contextPath.addSegment("lk_processstageparameter_modifiedonbehalfby"));
}
public Processtriggers lk_processtriggerbase_createdby() {
return new Processtriggers(contextPath.addSegment("lk_processtriggerbase_createdby"));
}
public Processtriggers lk_processtriggerbase_createdonbehalfby() {
return new Processtriggers(contextPath.addSegment("lk_processtriggerbase_createdonbehalfby"));
}
public Processtriggers lk_processtriggerbase_modifiedby() {
return new Processtriggers(contextPath.addSegment("lk_processtriggerbase_modifiedby"));
}
public Processtriggers lk_processtriggerbase_modifiedonbehalfby() {
return new Processtriggers(contextPath.addSegment("lk_processtriggerbase_modifiedonbehalfby"));
}
public Publishers lk_publisher_createdby() {
return new Publishers(contextPath.addSegment("lk_publisher_createdby"));
}
public Publishers lk_publisher_modifiedby() {
return new Publishers(contextPath.addSegment("lk_publisher_modifiedby"));
}
public Publisheraddresses lk_publisheraddressbase_createdby() {
return new Publisheraddresses(contextPath.addSegment("lk_publisheraddressbase_createdby"));
}
public Publisheraddresses lk_publisheraddressbase_createdonbehalfby() {
return new Publisheraddresses(contextPath.addSegment("lk_publisheraddressbase_createdonbehalfby"));
}
public Publisheraddresses lk_publisheraddressbase_modifiedby() {
return new Publisheraddresses(contextPath.addSegment("lk_publisheraddressbase_modifiedby"));
}
public Publisheraddresses lk_publisheraddressbase_modifiedonbehalfby() {
return new Publisheraddresses(contextPath.addSegment("lk_publisheraddressbase_modifiedonbehalfby"));
}
public Publishers lk_publisherbase_createdonbehalfby() {
return new Publishers(contextPath.addSegment("lk_publisherbase_createdonbehalfby"));
}
public Publishers lk_publisherbase_modifiedonbehalfby() {
return new Publishers(contextPath.addSegment("lk_publisherbase_modifiedonbehalfby"));
}
public Quarterlyfiscalcalendars lk_quarterlyfiscalcalendar_createdby() {
return new Quarterlyfiscalcalendars(contextPath.addSegment("lk_quarterlyfiscalcalendar_createdby"));
}
public Quarterlyfiscalcalendars lk_quarterlyfiscalcalendar_createdonbehalfby() {
return new Quarterlyfiscalcalendars(contextPath.addSegment("lk_quarterlyfiscalcalendar_createdonbehalfby"));
}
public Quarterlyfiscalcalendars lk_quarterlyfiscalcalendar_modifiedby() {
return new Quarterlyfiscalcalendars(contextPath.addSegment("lk_quarterlyfiscalcalendar_modifiedby"));
}
public Quarterlyfiscalcalendars lk_quarterlyfiscalcalendar_modifiedonbehalfby() {
return new Quarterlyfiscalcalendars(contextPath.addSegment("lk_quarterlyfiscalcalendar_modifiedonbehalfby"));
}
public Quarterlyfiscalcalendars lk_quarterlyfiscalcalendar_salespersonid() {
return new Quarterlyfiscalcalendars(contextPath.addSegment("lk_quarterlyfiscalcalendar_salespersonid"));
}
public Queues lk_queue_createdonbehalfby() {
return new Queues(contextPath.addSegment("lk_queue_createdonbehalfby"));
}
public Queues lk_queue_modifiedonbehalfby() {
return new Queues(contextPath.addSegment("lk_queue_modifiedonbehalfby"));
}
public Queues lk_queuebase_createdby() {
return new Queues(contextPath.addSegment("lk_queuebase_createdby"));
}
public Queues lk_queuebase_modifiedby() {
return new Queues(contextPath.addSegment("lk_queuebase_modifiedby"));
}
public Queueitems lk_queueitem_createdonbehalfby() {
return new Queueitems(contextPath.addSegment("lk_queueitem_createdonbehalfby"));
}
public Queueitems lk_queueitem_modifiedonbehalfby() {
return new Queueitems(contextPath.addSegment("lk_queueitem_modifiedonbehalfby"));
}
public Queueitems lk_queueitembase_createdby() {
return new Queueitems(contextPath.addSegment("lk_queueitembase_createdby"));
}
public Queueitems lk_queueitembase_modifiedby() {
return new Queueitems(contextPath.addSegment("lk_queueitembase_modifiedby"));
}
public Queueitems lk_queueitembase_workerid() {
return new Queueitems(contextPath.addSegment("lk_queueitembase_workerid"));
}
public Recommendeddocuments lk_recommendeddocument_createdby() {
return new Recommendeddocuments(contextPath.addSegment("lk_recommendeddocument_createdby"));
}
public Recommendeddocuments lk_recommendeddocument_createdonbehalfby() {
return new Recommendeddocuments(contextPath.addSegment("lk_recommendeddocument_createdonbehalfby"));
}
public Recommendeddocuments lk_recommendeddocument_modifiedby() {
return new Recommendeddocuments(contextPath.addSegment("lk_recommendeddocument_modifiedby"));
}
public Recommendeddocuments lk_recommendeddocument_modifiedonbehalfby() {
return new Recommendeddocuments(contextPath.addSegment("lk_recommendeddocument_modifiedonbehalfby"));
}
public Recurrencerules lk_recurrencerule_createdby() {
return new Recurrencerules(contextPath.addSegment("lk_recurrencerule_createdby"));
}
public Recurrencerules lk_recurrencerule_modifiedby() {
return new Recurrencerules(contextPath.addSegment("lk_recurrencerule_modifiedby"));
}
public Recurrencerules lk_recurrencerulebase_createdonbehalfby() {
return new Recurrencerules(contextPath.addSegment("lk_recurrencerulebase_createdonbehalfby"));
}
public Recurrencerules lk_recurrencerulebase_modifiedonbehalfby() {
return new Recurrencerules(contextPath.addSegment("lk_recurrencerulebase_modifiedonbehalfby"));
}
public Recurringappointmentmasters lk_recurringappointmentmaster_createdby() {
return new Recurringappointmentmasters(contextPath.addSegment("lk_recurringappointmentmaster_createdby"));
}
public Recurringappointmentmasters lk_recurringappointmentmaster_createdonbehalfby() {
return new Recurringappointmentmasters(contextPath.addSegment("lk_recurringappointmentmaster_createdonbehalfby"));
}
public Recurringappointmentmasters lk_recurringappointmentmaster_modifiedby() {
return new Recurringappointmentmasters(contextPath.addSegment("lk_recurringappointmentmaster_modifiedby"));
}
public Recurringappointmentmasters lk_recurringappointmentmaster_modifiedonbehalfby() {
return new Recurringappointmentmasters(contextPath.addSegment("lk_recurringappointmentmaster_modifiedonbehalfby"));
}
public Reports lk_report_createdonbehalfby() {
return new Reports(contextPath.addSegment("lk_report_createdonbehalfby"));
}
public Reports lk_report_modifiedonbehalfby() {
return new Reports(contextPath.addSegment("lk_report_modifiedonbehalfby"));
}
public Reports lk_reportbase_createdby() {
return new Reports(contextPath.addSegment("lk_reportbase_createdby"));
}
public Reports lk_reportbase_modifiedby() {
return new Reports(contextPath.addSegment("lk_reportbase_modifiedby"));
}
public Reportcategories lk_reportcategory_createdonbehalfby() {
return new Reportcategories(contextPath.addSegment("lk_reportcategory_createdonbehalfby"));
}
public Reportcategories lk_reportcategory_modifiedonbehalfby() {
return new Reportcategories(contextPath.addSegment("lk_reportcategory_modifiedonbehalfby"));
}
public Reportcategories lk_reportcategorybase_createdby() {
return new Reportcategories(contextPath.addSegment("lk_reportcategorybase_createdby"));
}
public Reportcategories lk_reportcategorybase_modifiedby() {
return new Reportcategories(contextPath.addSegment("lk_reportcategorybase_modifiedby"));
}
public Roles lk_role_createdonbehalfby() {
return new Roles(contextPath.addSegment("lk_role_createdonbehalfby"));
}
public Roles lk_role_modifiedonbehalfby() {
return new Roles(contextPath.addSegment("lk_role_modifiedonbehalfby"));
}
public Roles lk_rolebase_createdby() {
return new Roles(contextPath.addSegment("lk_rolebase_createdby"));
}
public Roles lk_rolebase_modifiedby() {
return new Roles(contextPath.addSegment("lk_rolebase_modifiedby"));
}
public Rollupfields lk_rollupfield_createdby() {
return new Rollupfields(contextPath.addSegment("lk_rollupfield_createdby"));
}
public Rollupfields lk_rollupfield_createdonbehalfby() {
return new Rollupfields(contextPath.addSegment("lk_rollupfield_createdonbehalfby"));
}
public Rollupfields lk_rollupfield_modifiedby() {
return new Rollupfields(contextPath.addSegment("lk_rollupfield_modifiedby"));
}
public Rollupfields lk_rollupfield_modifiedonbehalfby() {
return new Rollupfields(contextPath.addSegment("lk_rollupfield_modifiedonbehalfby"));
}
public Savedqueries lk_savedquery_createdonbehalfby() {
return new Savedqueries(contextPath.addSegment("lk_savedquery_createdonbehalfby"));
}
public Savedqueries lk_savedquery_modifiedonbehalfby() {
return new Savedqueries(contextPath.addSegment("lk_savedquery_modifiedonbehalfby"));
}
public Savedqueries lk_savedquerybase_createdby() {
return new Savedqueries(contextPath.addSegment("lk_savedquerybase_createdby"));
}
public Savedqueries lk_savedquerybase_modifiedby() {
return new Savedqueries(contextPath.addSegment("lk_savedquerybase_modifiedby"));
}
public Savedqueryvisualizations lk_savedqueryvisualizationbase_createdby() {
return new Savedqueryvisualizations(contextPath.addSegment("lk_savedqueryvisualizationbase_createdby"));
}
public Savedqueryvisualizations lk_savedqueryvisualizationbase_createdonbehalfby() {
return new Savedqueryvisualizations(contextPath.addSegment("lk_savedqueryvisualizationbase_createdonbehalfby"));
}
public Savedqueryvisualizations lk_savedqueryvisualizationbase_modifiedby() {
return new Savedqueryvisualizations(contextPath.addSegment("lk_savedqueryvisualizationbase_modifiedby"));
}
public Savedqueryvisualizations lk_savedqueryvisualizationbase_modifiedonbehalfby() {
return new Savedqueryvisualizations(contextPath.addSegment("lk_savedqueryvisualizationbase_modifiedonbehalfby"));
}
public Sdkmessages lk_sdkmessage_createdonbehalfby() {
return new Sdkmessages(contextPath.addSegment("lk_sdkmessage_createdonbehalfby"));
}
public Sdkmessages lk_sdkmessage_modifiedonbehalfby() {
return new Sdkmessages(contextPath.addSegment("lk_sdkmessage_modifiedonbehalfby"));
}
public Sdkmessagefilters lk_sdkmessagefilter_createdonbehalfby() {
return new Sdkmessagefilters(contextPath.addSegment("lk_sdkmessagefilter_createdonbehalfby"));
}
public Sdkmessagefilters lk_sdkmessagefilter_modifiedonbehalfby() {
return new Sdkmessagefilters(contextPath.addSegment("lk_sdkmessagefilter_modifiedonbehalfby"));
}
public Sdkmessageprocessingsteps lk_sdkmessageprocessingstep_createdonbehalfby() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("lk_sdkmessageprocessingstep_createdonbehalfby"));
}
public Sdkmessageprocessingsteps lk_sdkmessageprocessingstep_modifiedonbehalfby() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("lk_sdkmessageprocessingstep_modifiedonbehalfby"));
}
public Sdkmessageprocessingstepimages lk_sdkmessageprocessingstepimage_createdonbehalfby() {
return new Sdkmessageprocessingstepimages(contextPath.addSegment("lk_sdkmessageprocessingstepimage_createdonbehalfby"));
}
public Sdkmessageprocessingstepimages lk_sdkmessageprocessingstepimage_modifiedonbehalfby() {
return new Sdkmessageprocessingstepimages(contextPath.addSegment("lk_sdkmessageprocessingstepimage_modifiedonbehalfby"));
}
public Sdkmessageprocessingstepsecureconfigs lk_sdkmessageprocessingstepsecureconfig_createdonbehalfby() {
return new Sdkmessageprocessingstepsecureconfigs(contextPath.addSegment("lk_sdkmessageprocessingstepsecureconfig_createdonbehalfby"));
}
public Sdkmessageprocessingstepsecureconfigs lk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby() {
return new Sdkmessageprocessingstepsecureconfigs(contextPath.addSegment("lk_sdkmessageprocessingstepsecureconfig_modifiedonbehalfby"));
}
public Semiannualfiscalcalendars lk_semiannualfiscalcalendar_createdby() {
return new Semiannualfiscalcalendars(contextPath.addSegment("lk_semiannualfiscalcalendar_createdby"));
}
public Semiannualfiscalcalendars lk_semiannualfiscalcalendar_createdonbehalfby() {
return new Semiannualfiscalcalendars(contextPath.addSegment("lk_semiannualfiscalcalendar_createdonbehalfby"));
}
public Semiannualfiscalcalendars lk_semiannualfiscalcalendar_modifiedby() {
return new Semiannualfiscalcalendars(contextPath.addSegment("lk_semiannualfiscalcalendar_modifiedby"));
}
public Semiannualfiscalcalendars lk_semiannualfiscalcalendar_modifiedonbehalfby() {
return new Semiannualfiscalcalendars(contextPath.addSegment("lk_semiannualfiscalcalendar_modifiedonbehalfby"));
}
public Semiannualfiscalcalendars lk_semiannualfiscalcalendar_salespersonid() {
return new Semiannualfiscalcalendars(contextPath.addSegment("lk_semiannualfiscalcalendar_salespersonid"));
}
public Serviceendpoints lk_serviceendpointbase_createdonbehalfby() {
return new Serviceendpoints(contextPath.addSegment("lk_serviceendpointbase_createdonbehalfby"));
}
public Serviceendpoints lk_serviceendpointbase_modifiedonbehalfby() {
return new Serviceendpoints(contextPath.addSegment("lk_serviceendpointbase_modifiedonbehalfby"));
}
public Serviceplans lk_serviceplan_createdby() {
return new Serviceplans(contextPath.addSegment("lk_serviceplan_createdby"));
}
public Serviceplans lk_serviceplan_createdonbehalfby() {
return new Serviceplans(contextPath.addSegment("lk_serviceplan_createdonbehalfby"));
}
public Serviceplans lk_serviceplan_modifiedby() {
return new Serviceplans(contextPath.addSegment("lk_serviceplan_modifiedby"));
}
public Serviceplans lk_serviceplan_modifiedonbehalfby() {
return new Serviceplans(contextPath.addSegment("lk_serviceplan_modifiedonbehalfby"));
}
public Sharepointdocumentlocations lk_sharepointdocumentlocationbase_createdby() {
return new Sharepointdocumentlocations(contextPath.addSegment("lk_sharepointdocumentlocationbase_createdby"));
}
public Sharepointdocumentlocations lk_sharepointdocumentlocationbase_createdonbehalfby() {
return new Sharepointdocumentlocations(contextPath.addSegment("lk_sharepointdocumentlocationbase_createdonbehalfby"));
}
public Sharepointdocumentlocations lk_sharepointdocumentlocationbase_modifiedby() {
return new Sharepointdocumentlocations(contextPath.addSegment("lk_sharepointdocumentlocationbase_modifiedby"));
}
public Sharepointdocumentlocations lk_sharepointdocumentlocationbase_modifiedonbehalfby() {
return new Sharepointdocumentlocations(contextPath.addSegment("lk_sharepointdocumentlocationbase_modifiedonbehalfby"));
}
public Sharepointsites lk_sharepointsitebase_createdby() {
return new Sharepointsites(contextPath.addSegment("lk_sharepointsitebase_createdby"));
}
public Sharepointsites lk_sharepointsitebase_createdonbehalfby() {
return new Sharepointsites(contextPath.addSegment("lk_sharepointsitebase_createdonbehalfby"));
}
public Sharepointsites lk_sharepointsitebase_modifiedby() {
return new Sharepointsites(contextPath.addSegment("lk_sharepointsitebase_modifiedby"));
}
public Sharepointsites lk_sharepointsitebase_modifiedonbehalfby() {
return new Sharepointsites(contextPath.addSegment("lk_sharepointsitebase_modifiedonbehalfby"));
}
public Similarityrules lk_similarityrule_createdonbehalfby() {
return new Similarityrules(contextPath.addSegment("lk_similarityrule_createdonbehalfby"));
}
public Similarityrules lk_similarityrule_modifiedonbehalfby() {
return new Similarityrules(contextPath.addSegment("lk_similarityrule_modifiedonbehalfby"));
}
public Slas lk_slabase_createdby() {
return new Slas(contextPath.addSegment("lk_slabase_createdby"));
}
public Slas lk_slabase_createdonbehalfby() {
return new Slas(contextPath.addSegment("lk_slabase_createdonbehalfby"));
}
public Slas lk_slabase_modifiedby() {
return new Slas(contextPath.addSegment("lk_slabase_modifiedby"));
}
public Slas lk_slabase_modifiedonbehalfby() {
return new Slas(contextPath.addSegment("lk_slabase_modifiedonbehalfby"));
}
public Slaitems lk_slaitembase_createdby() {
return new Slaitems(contextPath.addSegment("lk_slaitembase_createdby"));
}
public Slaitems lk_slaitembase_createdonbehalfby() {
return new Slaitems(contextPath.addSegment("lk_slaitembase_createdonbehalfby"));
}
public Slaitems lk_slaitembase_modifiedby() {
return new Slaitems(contextPath.addSegment("lk_slaitembase_modifiedby"));
}
public Slaitems lk_slaitembase_modifiedonbehalfby() {
return new Slaitems(contextPath.addSegment("lk_slaitembase_modifiedonbehalfby"));
}
public Slakpiinstances lk_slakpiinstancebase_createdby() {
return new Slakpiinstances(contextPath.addSegment("lk_slakpiinstancebase_createdby"));
}
public Slakpiinstances lk_slakpiinstancebase_createdonbehalfby() {
return new Slakpiinstances(contextPath.addSegment("lk_slakpiinstancebase_createdonbehalfby"));
}
public Slakpiinstances lk_slakpiinstancebase_modifiedby() {
return new Slakpiinstances(contextPath.addSegment("lk_slakpiinstancebase_modifiedby"));
}
public Slakpiinstances lk_slakpiinstancebase_modifiedonbehalfby() {
return new Slakpiinstances(contextPath.addSegment("lk_slakpiinstancebase_modifiedonbehalfby"));
}
public Socialactivities lk_socialactivity_createdby() {
return new Socialactivities(contextPath.addSegment("lk_socialactivity_createdby"));
}
public Socialactivities lk_socialactivity_modifiedby() {
return new Socialactivities(contextPath.addSegment("lk_socialactivity_modifiedby"));
}
public Socialactivities lk_socialactivitybase_createdonbehalfby() {
return new Socialactivities(contextPath.addSegment("lk_socialactivitybase_createdonbehalfby"));
}
public Socialactivities lk_socialactivitybase_modifiedonbehalfby() {
return new Socialactivities(contextPath.addSegment("lk_socialactivitybase_modifiedonbehalfby"));
}
public Socialprofiles lk_SocialProfile_createdonbehalfby() {
return new Socialprofiles(contextPath.addSegment("lk_SocialProfile_createdonbehalfby"));
}
public Socialprofiles lk_SocialProfile_modifiedonbehalfby() {
return new Socialprofiles(contextPath.addSegment("lk_SocialProfile_modifiedonbehalfby"));
}
public Solutions lk_solution_createdby() {
return new Solutions(contextPath.addSegment("lk_solution_createdby"));
}
public Solutions lk_solution_modifiedby() {
return new Solutions(contextPath.addSegment("lk_solution_modifiedby"));
}
public Solutions lk_solutionbase_createdonbehalfby() {
return new Solutions(contextPath.addSegment("lk_solutionbase_createdonbehalfby"));
}
public Solutions lk_solutionbase_modifiedonbehalfby() {
return new Solutions(contextPath.addSegment("lk_solutionbase_modifiedonbehalfby"));
}
public Solutioncomponentattributeconfigurations lk_solutioncomponentattributeconfiguration_createdby() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("lk_solutioncomponentattributeconfiguration_createdby"));
}
public Solutioncomponentattributeconfigurations lk_solutioncomponentattributeconfiguration_createdonbehalfby() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("lk_solutioncomponentattributeconfiguration_createdonbehalfby"));
}
public Solutioncomponentattributeconfigurations lk_solutioncomponentattributeconfiguration_modifiedby() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("lk_solutioncomponentattributeconfiguration_modifiedby"));
}
public Solutioncomponentattributeconfigurations lk_solutioncomponentattributeconfiguration_modifiedonbehalfby() {
return new Solutioncomponentattributeconfigurations(contextPath.addSegment("lk_solutioncomponentattributeconfiguration_modifiedonbehalfby"));
}
public Solutioncomponents lk_solutioncomponentbase_createdonbehalfby() {
return new Solutioncomponents(contextPath.addSegment("lk_solutioncomponentbase_createdonbehalfby"));
}
public Solutioncomponents lk_solutioncomponentbase_modifiedonbehalfby() {
return new Solutioncomponents(contextPath.addSegment("lk_solutioncomponentbase_modifiedonbehalfby"));
}
public Solutioncomponentconfigurations lk_solutioncomponentconfiguration_createdby() {
return new Solutioncomponentconfigurations(contextPath.addSegment("lk_solutioncomponentconfiguration_createdby"));
}
public Solutioncomponentconfigurations lk_solutioncomponentconfiguration_createdonbehalfby() {
return new Solutioncomponentconfigurations(contextPath.addSegment("lk_solutioncomponentconfiguration_createdonbehalfby"));
}
public Solutioncomponentconfigurations lk_solutioncomponentconfiguration_modifiedby() {
return new Solutioncomponentconfigurations(contextPath.addSegment("lk_solutioncomponentconfiguration_modifiedby"));
}
public Solutioncomponentconfigurations lk_solutioncomponentconfiguration_modifiedonbehalfby() {
return new Solutioncomponentconfigurations(contextPath.addSegment("lk_solutioncomponentconfiguration_modifiedonbehalfby"));
}
public Solutioncomponentrelationshipconfigurations lk_solutioncomponentrelationshipconfiguration_createdby() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_createdby"));
}
public Solutioncomponentrelationshipconfigurations lk_solutioncomponentrelationshipconfiguration_createdonbehalfby() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_createdonbehalfby"));
}
public Solutioncomponentrelationshipconfigurations lk_solutioncomponentrelationshipconfiguration_modifiedby() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_modifiedby"));
}
public Solutioncomponentrelationshipconfigurations lk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby() {
return new Solutioncomponentrelationshipconfigurations(contextPath.addSegment("lk_solutioncomponentrelationshipconfiguration_modifiedonbehalfby"));
}
public Stagesolutionuploads lk_stagesolutionupload_createdby() {
return new Stagesolutionuploads(contextPath.addSegment("lk_stagesolutionupload_createdby"));
}
public Stagesolutionuploads lk_stagesolutionupload_createdonbehalfby() {
return new Stagesolutionuploads(contextPath.addSegment("lk_stagesolutionupload_createdonbehalfby"));
}
public Stagesolutionuploads lk_stagesolutionupload_modifiedby() {
return new Stagesolutionuploads(contextPath.addSegment("lk_stagesolutionupload_modifiedby"));
}
public Stagesolutionuploads lk_stagesolutionupload_modifiedonbehalfby() {
return new Stagesolutionuploads(contextPath.addSegment("lk_stagesolutionupload_modifiedonbehalfby"));
}
public Subjects lk_subject_createdonbehalfby() {
return new Subjects(contextPath.addSegment("lk_subject_createdonbehalfby"));
}
public Subjects lk_subject_modifiedonbehalfby() {
return new Subjects(contextPath.addSegment("lk_subject_modifiedonbehalfby"));
}
public Subjects lk_subjectbase_createdby() {
return new Subjects(contextPath.addSegment("lk_subjectbase_createdby"));
}
public Subjects lk_subjectbase_modifiedby() {
return new Subjects(contextPath.addSegment("lk_subjectbase_modifiedby"));
}
public Syncerrors lk_syncerrorbase_createdby() {
return new Syncerrors(contextPath.addSegment("lk_syncerrorbase_createdby"));
}
public Syncerrors lk_syncerrorbase_createdonbehalfby() {
return new Syncerrors(contextPath.addSegment("lk_syncerrorbase_createdonbehalfby"));
}
public Syncerrors lk_syncerrorbase_modifiedby() {
return new Syncerrors(contextPath.addSegment("lk_syncerrorbase_modifiedby"));
}
public Syncerrors lk_syncerrorbase_modifiedonbehalfby() {
return new Syncerrors(contextPath.addSegment("lk_syncerrorbase_modifiedonbehalfby"));
}
public Systemusers lk_systemuser_createdonbehalfby() {
return new Systemusers(contextPath.addSegment("lk_systemuser_createdonbehalfby"));
}
public Systemusers lk_systemuser_modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("lk_systemuser_modifiedonbehalfby"));
}
public Systemusers lk_systemuserbase_createdby() {
return new Systemusers(contextPath.addSegment("lk_systemuserbase_createdby"));
}
public Systemusers lk_systemuserbase_modifiedby() {
return new Systemusers(contextPath.addSegment("lk_systemuserbase_modifiedby"));
}
public Tasks lk_task_createdby() {
return new Tasks(contextPath.addSegment("lk_task_createdby"));
}
public Tasks lk_task_createdonbehalfby() {
return new Tasks(contextPath.addSegment("lk_task_createdonbehalfby"));
}
public Tasks lk_task_modifiedby() {
return new Tasks(contextPath.addSegment("lk_task_modifiedby"));
}
public Tasks lk_task_modifiedonbehalfby() {
return new Tasks(contextPath.addSegment("lk_task_modifiedonbehalfby"));
}
public Teams lk_team_createdonbehalfby() {
return new Teams(contextPath.addSegment("lk_team_createdonbehalfby"));
}
public Teams lk_team_modifiedonbehalfby() {
return new Teams(contextPath.addSegment("lk_team_modifiedonbehalfby"));
}
public Teams lk_teambase_administratorid() {
return new Teams(contextPath.addSegment("lk_teambase_administratorid"));
}
public Teams lk_teambase_createdby() {
return new Teams(contextPath.addSegment("lk_teambase_createdby"));
}
public Teams lk_teambase_modifiedby() {
return new Teams(contextPath.addSegment("lk_teambase_modifiedby"));
}
public Teamtemplates lk_teamtemplate_createdby() {
return new Teamtemplates(contextPath.addSegment("lk_teamtemplate_createdby"));
}
public Teamtemplates lk_teamtemplate_createdonbehalfby() {
return new Teamtemplates(contextPath.addSegment("lk_teamtemplate_createdonbehalfby"));
}
public Teamtemplates lk_teamtemplate_modifiedby() {
return new Teamtemplates(contextPath.addSegment("lk_teamtemplate_modifiedby"));
}
public Teamtemplates lk_teamtemplate_modifiedonbehalfby() {
return new Teamtemplates(contextPath.addSegment("lk_teamtemplate_modifiedonbehalfby"));
}
public Templates lk_templatebase_createdby() {
return new Templates(contextPath.addSegment("lk_templatebase_createdby"));
}
public Templates lk_templatebase_createdonbehalfby() {
return new Templates(contextPath.addSegment("lk_templatebase_createdonbehalfby"));
}
public Templates lk_templatebase_modifiedby() {
return new Templates(contextPath.addSegment("lk_templatebase_modifiedby"));
}
public Templates lk_templatebase_modifiedonbehalfby() {
return new Templates(contextPath.addSegment("lk_templatebase_modifiedonbehalfby"));
}
public Territories lk_territorybase_createdby() {
return new Territories(contextPath.addSegment("lk_territorybase_createdby"));
}
public Territories lk_territorybase_createdonbehalfby() {
return new Territories(contextPath.addSegment("lk_territorybase_createdonbehalfby"));
}
public Territories lk_territorybase_modifiedby() {
return new Territories(contextPath.addSegment("lk_territorybase_modifiedby"));
}
public Territories lk_territorybase_modifiedonbehalfby() {
return new Territories(contextPath.addSegment("lk_territorybase_modifiedonbehalfby"));
}
public Themes lk_theme_createdby() {
return new Themes(contextPath.addSegment("lk_theme_createdby"));
}
public Themes lk_theme_createdonbehalfby() {
return new Themes(contextPath.addSegment("lk_theme_createdonbehalfby"));
}
public Themes lk_theme_modifiedby() {
return new Themes(contextPath.addSegment("lk_theme_modifiedby"));
}
public Themes lk_theme_modifiedonbehalfby() {
return new Themes(contextPath.addSegment("lk_theme_modifiedonbehalfby"));
}
public Timezonedefinitions lk_timezonedefinition_createdby() {
return new Timezonedefinitions(contextPath.addSegment("lk_timezonedefinition_createdby"));
}
public Timezonedefinitions lk_timezonedefinition_createdonbehalfby() {
return new Timezonedefinitions(contextPath.addSegment("lk_timezonedefinition_createdonbehalfby"));
}
public Timezonedefinitions lk_timezonedefinition_modifiedby() {
return new Timezonedefinitions(contextPath.addSegment("lk_timezonedefinition_modifiedby"));
}
public Timezonedefinitions lk_timezonedefinition_modifiedonbehalfby() {
return new Timezonedefinitions(contextPath.addSegment("lk_timezonedefinition_modifiedonbehalfby"));
}
public Timezonelocalizednames lk_timezonelocalizedname_createdby() {
return new Timezonelocalizednames(contextPath.addSegment("lk_timezonelocalizedname_createdby"));
}
public Timezonelocalizednames lk_timezonelocalizedname_createdonbehalfby() {
return new Timezonelocalizednames(contextPath.addSegment("lk_timezonelocalizedname_createdonbehalfby"));
}
public Timezonelocalizednames lk_timezonelocalizedname_modifiedby() {
return new Timezonelocalizednames(contextPath.addSegment("lk_timezonelocalizedname_modifiedby"));
}
public Timezonelocalizednames lk_timezonelocalizedname_modifiedonbehalfby() {
return new Timezonelocalizednames(contextPath.addSegment("lk_timezonelocalizedname_modifiedonbehalfby"));
}
public Timezonerules lk_timezonerule_createdby() {
return new Timezonerules(contextPath.addSegment("lk_timezonerule_createdby"));
}
public Timezonerules lk_timezonerule_createdonbehalfby() {
return new Timezonerules(contextPath.addSegment("lk_timezonerule_createdonbehalfby"));
}
public Timezonerules lk_timezonerule_modifiedby() {
return new Timezonerules(contextPath.addSegment("lk_timezonerule_modifiedby"));
}
public Timezonerules lk_timezonerule_modifiedonbehalfby() {
return new Timezonerules(contextPath.addSegment("lk_timezonerule_modifiedonbehalfby"));
}
public Tracelogs lk_tracelog_createdby() {
return new Tracelogs(contextPath.addSegment("lk_tracelog_createdby"));
}
public Tracelogs lk_tracelog_createdonbehalfby() {
return new Tracelogs(contextPath.addSegment("lk_tracelog_createdonbehalfby"));
}
public Tracelogs lk_tracelog_modifiedby() {
return new Tracelogs(contextPath.addSegment("lk_tracelog_modifiedby"));
}
public Tracelogs lk_tracelog_modifiedonbehalfby() {
return new Tracelogs(contextPath.addSegment("lk_tracelog_modifiedonbehalfby"));
}
public Transactioncurrencies lk_transactioncurrency_createdonbehalfby() {
return new Transactioncurrencies(contextPath.addSegment("lk_transactioncurrency_createdonbehalfby"));
}
public Transactioncurrencies lk_transactioncurrency_modifiedonbehalfby() {
return new Transactioncurrencies(contextPath.addSegment("lk_transactioncurrency_modifiedonbehalfby"));
}
public Transactioncurrencies lk_transactioncurrencybase_createdby() {
return new Transactioncurrencies(contextPath.addSegment("lk_transactioncurrencybase_createdby"));
}
public Transactioncurrencies lk_transactioncurrencybase_modifiedby() {
return new Transactioncurrencies(contextPath.addSegment("lk_transactioncurrencybase_modifiedby"));
}
public Transformationmappings lk_transformationmapping_createdby() {
return new Transformationmappings(contextPath.addSegment("lk_transformationmapping_createdby"));
}
public Transformationmappings lk_transformationmapping_createdonbehalfby() {
return new Transformationmappings(contextPath.addSegment("lk_transformationmapping_createdonbehalfby"));
}
public Transformationmappings lk_transformationmapping_modifiedby() {
return new Transformationmappings(contextPath.addSegment("lk_transformationmapping_modifiedby"));
}
public Transformationmappings lk_transformationmapping_modifiedonbehalfby() {
return new Transformationmappings(contextPath.addSegment("lk_transformationmapping_modifiedonbehalfby"));
}
public Transformationparametermappings lk_transformationparametermapping_createdby() {
return new Transformationparametermappings(contextPath.addSegment("lk_transformationparametermapping_createdby"));
}
public Transformationparametermappings lk_transformationparametermapping_createdonbehalfby() {
return new Transformationparametermappings(contextPath.addSegment("lk_transformationparametermapping_createdonbehalfby"));
}
public Transformationparametermappings lk_transformationparametermapping_modifiedby() {
return new Transformationparametermappings(contextPath.addSegment("lk_transformationparametermapping_modifiedby"));
}
public Transformationparametermappings lk_transformationparametermapping_modifiedonbehalfby() {
return new Transformationparametermappings(contextPath.addSegment("lk_transformationparametermapping_modifiedonbehalfby"));
}
public Translationprocesses lk_translationprocess_createdby() {
return new Translationprocesses(contextPath.addSegment("lk_translationprocess_createdby"));
}
public Translationprocesses lk_translationprocess_createdonbehalfby() {
return new Translationprocesses(contextPath.addSegment("lk_translationprocess_createdonbehalfby"));
}
public Translationprocesses lk_translationprocess_modifiedby() {
return new Translationprocesses(contextPath.addSegment("lk_translationprocess_modifiedby"));
}
public Translationprocesses lk_translationprocess_modifiedonbehalfby() {
return new Translationprocesses(contextPath.addSegment("lk_translationprocess_modifiedonbehalfby"));
}
public Userforms lk_userform_createdby() {
return new Userforms(contextPath.addSegment("lk_userform_createdby"));
}
public Userforms lk_userform_modifiedby() {
return new Userforms(contextPath.addSegment("lk_userform_modifiedby"));
}
public Userforms lk_userformbase_createdonbehalfby() {
return new Userforms(contextPath.addSegment("lk_userformbase_createdonbehalfby"));
}
public Userforms lk_userformbase_modifiedonbehalfby() {
return new Userforms(contextPath.addSegment("lk_userformbase_modifiedonbehalfby"));
}
public Usermappings lk_usermapping_createdby() {
return new Usermappings(contextPath.addSegment("lk_usermapping_createdby"));
}
public Usermappings lk_usermapping_createdonbehalfby() {
return new Usermappings(contextPath.addSegment("lk_usermapping_createdonbehalfby"));
}
public Usermappings lk_usermapping_modifiedby() {
return new Usermappings(contextPath.addSegment("lk_usermapping_modifiedby"));
}
public Usermappings lk_usermapping_modifiedonbehalfby() {
return new Usermappings(contextPath.addSegment("lk_usermapping_modifiedonbehalfby"));
}
public Userqueries lk_userquery_createdby() {
return new Userqueries(contextPath.addSegment("lk_userquery_createdby"));
}
public Userqueries lk_userquery_createdonbehalfby() {
return new Userqueries(contextPath.addSegment("lk_userquery_createdonbehalfby"));
}
public Userqueries lk_userquery_modifiedby() {
return new Userqueries(contextPath.addSegment("lk_userquery_modifiedby"));
}
public Userqueries lk_userquery_modifiedonbehalfby() {
return new Userqueries(contextPath.addSegment("lk_userquery_modifiedonbehalfby"));
}
public Userqueryvisualizations lk_userqueryvisualization_createdby() {
return new Userqueryvisualizations(contextPath.addSegment("lk_userqueryvisualization_createdby"));
}
public Userqueryvisualizations lk_userqueryvisualization_modifiedby() {
return new Userqueryvisualizations(contextPath.addSegment("lk_userqueryvisualization_modifiedby"));
}
public Userqueryvisualizations lk_userqueryvisualizationbase_createdonbehalfby() {
return new Userqueryvisualizations(contextPath.addSegment("lk_userqueryvisualizationbase_createdonbehalfby"));
}
public Userqueryvisualizations lk_userqueryvisualizationbase_modifiedonbehalfby() {
return new Userqueryvisualizations(contextPath.addSegment("lk_userqueryvisualizationbase_modifiedonbehalfby"));
}
public Usersettingscollection lk_usersettings_createdonbehalfby() {
return new Usersettingscollection(contextPath.addSegment("lk_usersettings_createdonbehalfby"));
}
public Usersettingscollection lk_usersettings_modifiedonbehalfby() {
return new Usersettingscollection(contextPath.addSegment("lk_usersettings_modifiedonbehalfby"));
}
public Usersettingscollection lk_usersettingsbase_createdby() {
return new Usersettingscollection(contextPath.addSegment("lk_usersettingsbase_createdby"));
}
public Usersettingscollection lk_usersettingsbase_modifiedby() {
return new Usersettingscollection(contextPath.addSegment("lk_usersettingsbase_modifiedby"));
}
public Webresourceset lk_webresourcebase_createdonbehalfby() {
return new Webresourceset(contextPath.addSegment("lk_webresourcebase_createdonbehalfby"));
}
public Webresourceset lk_webresourcebase_modifiedonbehalfby() {
return new Webresourceset(contextPath.addSegment("lk_webresourcebase_modifiedonbehalfby"));
}
public Webwizards lk_webwizard_createdby() {
return new Webwizards(contextPath.addSegment("lk_webwizard_createdby"));
}
public Webwizards lk_webwizard_createdonbehalfby() {
return new Webwizards(contextPath.addSegment("lk_webwizard_createdonbehalfby"));
}
public Webwizards lk_webwizard_modifiedby() {
return new Webwizards(contextPath.addSegment("lk_webwizard_modifiedby"));
}
public Webwizards lk_webwizard_modifiedonbehalfby() {
return new Webwizards(contextPath.addSegment("lk_webwizard_modifiedonbehalfby"));
}
public Workflowbinaries lk_workflowbinary_createdby() {
return new Workflowbinaries(contextPath.addSegment("lk_workflowbinary_createdby"));
}
public Workflowbinaries lk_workflowbinary_createdonbehalfby() {
return new Workflowbinaries(contextPath.addSegment("lk_workflowbinary_createdonbehalfby"));
}
public Workflowbinaries lk_workflowbinary_modifiedby() {
return new Workflowbinaries(contextPath.addSegment("lk_workflowbinary_modifiedby"));
}
public Workflowbinaries lk_workflowbinary_modifiedonbehalfby() {
return new Workflowbinaries(contextPath.addSegment("lk_workflowbinary_modifiedonbehalfby"));
}
public Workflowlogs lk_workflowlog_createdby() {
return new Workflowlogs(contextPath.addSegment("lk_workflowlog_createdby"));
}
public Workflowlogs lk_workflowlog_createdonbehalfby() {
return new Workflowlogs(contextPath.addSegment("lk_workflowlog_createdonbehalfby"));
}
public Workflowlogs lk_workflowlog_modifiedby() {
return new Workflowlogs(contextPath.addSegment("lk_workflowlog_modifiedby"));
}
public Workflowlogs lk_workflowlog_modifiedonbehalfby() {
return new Workflowlogs(contextPath.addSegment("lk_workflowlog_modifiedonbehalfby"));
}
public Mailboxes mailbox_regarding_systemuser() {
return new Mailboxes(contextPath.addSegment("mailbox_regarding_systemuser"));
}
public Mobileofflineprofiles mobileofflineprofileid() {
return new Mobileofflineprofiles(contextPath.addSegment("mobileofflineprofileid"));
}
public Systemusers modifiedby() {
return new Systemusers(contextPath.addSegment("modifiedby"));
}
public Connections modifiedby_connection() {
return new Connections(contextPath.addSegment("modifiedby_connection"));
}
public Connectionroles modifiedby_connection_role() {
return new Connectionroles(contextPath.addSegment("modifiedby_connection_role"));
}
public Pluginassemblies modifiedby_pluginassembly() {
return new Pluginassemblies(contextPath.addSegment("modifiedby_pluginassembly"));
}
public Plugintypes modifiedby_plugintype() {
return new Plugintypes(contextPath.addSegment("modifiedby_plugintype"));
}
public Plugintypestatistics modifiedby_plugintypestatistic() {
return new Plugintypestatistics(contextPath.addSegment("modifiedby_plugintypestatistic"));
}
public Sdkmessages modifiedby_sdkmessage() {
return new Sdkmessages(contextPath.addSegment("modifiedby_sdkmessage"));
}
public Sdkmessagefilters modifiedby_sdkmessagefilter() {
return new Sdkmessagefilters(contextPath.addSegment("modifiedby_sdkmessagefilter"));
}
public Sdkmessageprocessingsteps modifiedby_sdkmessageprocessingstep() {
return new Sdkmessageprocessingsteps(contextPath.addSegment("modifiedby_sdkmessageprocessingstep"));
}
public Sdkmessageprocessingstepimages modifiedby_sdkmessageprocessingstepimage() {
return new Sdkmessageprocessingstepimages(contextPath.addSegment("modifiedby_sdkmessageprocessingstepimage"));
}
public Sdkmessageprocessingstepsecureconfigs modifiedby_sdkmessageprocessingstepsecureconfig() {
return new Sdkmessageprocessingstepsecureconfigs(contextPath.addSegment("modifiedby_sdkmessageprocessingstepsecureconfig"));
}
public Serviceendpoints modifiedby_serviceendpoint() {
return new Serviceendpoints(contextPath.addSegment("modifiedby_serviceendpoint"));
}
public Systemusers modifiedonbehalfby() {
return new Systemusers(contextPath.addSegment("modifiedonbehalfby"));
}
public Organizations organizationid_organization() {
return new Organizations(contextPath.addSegment("organizationid_organization"));
}
public Ownermappings ownerMapping_SystemUser() {
return new Ownermappings(contextPath.addSegment("OwnerMapping_SystemUser"));
}
public Systemusers parentsystemuserid() {
return new Systemusers(contextPath.addSegment("parentsystemuserid"));
}
public Positions positionid() {
return new Positions(contextPath.addSegment("positionid"));
}
public Queues queue_primary_user() {
return new Queues(contextPath.addSegment("queue_primary_user"));
}
public Queues queueid() {
return new Queues(contextPath.addSegment("queueid"));
}
public Queues queuemembership_association() {
return new Queues(contextPath.addSegment("queuemembership_association"));
}
public Socialprofiles socialProfile_owning_user() {
return new Socialprofiles(contextPath.addSegment("socialProfile_owning_user"));
}
public Processstages stageid_processstage() {
return new Processstages(contextPath.addSegment("stageid_processstage"));
}
public Accounts system_user_accounts() {
return new Accounts(contextPath.addSegment("system_user_accounts"));
}
public Activityparties system_user_activity_parties() {
return new Activityparties(contextPath.addSegment("system_user_activity_parties"));
}
public Asyncoperations system_user_asyncoperation() {
return new Asyncoperations(contextPath.addSegment("system_user_asyncoperation"));
}
public Contacts system_user_contacts() {
return new Contacts(contextPath.addSegment("system_user_contacts"));
}
public Templates system_user_email_templates() {
return new Templates(contextPath.addSegment("system_user_email_templates"));
}
public Territories system_user_territories() {
return new Territories(contextPath.addSegment("system_user_territories"));
}
public Workflows system_user_workflow() {
return new Workflows(contextPath.addSegment("system_user_workflow"));
}
public Appconfigs systemuser_appconfig_createdby() {
return new Appconfigs(contextPath.addSegment("systemuser_appconfig_createdby"));
}
public Appconfigs systemuser_appconfig_createdonbehalfby() {
return new Appconfigs(contextPath.addSegment("systemuser_appconfig_createdonbehalfby"));
}
public Appconfigs systemuser_appconfig_modifiedby() {
return new Appconfigs(contextPath.addSegment("systemuser_appconfig_modifiedby"));
}
public Appconfigs systemuser_appconfig_modifiedonbehalfby() {
return new Appconfigs(contextPath.addSegment("systemuser_appconfig_modifiedonbehalfby"));
}
public Appconfiginstances systemuser_appconfiginstance_createdby() {
return new Appconfiginstances(contextPath.addSegment("systemuser_appconfiginstance_createdby"));
}
public Appconfiginstances systemuser_appconfiginstance_createdonbehalfby() {
return new Appconfiginstances(contextPath.addSegment("systemuser_appconfiginstance_createdonbehalfby"));
}
public Appconfiginstances systemuser_appconfiginstance_modifiedby() {
return new Appconfiginstances(contextPath.addSegment("systemuser_appconfiginstance_modifiedby"));
}
public Appconfiginstances systemuser_appconfiginstance_modifiedonbehalfby() {
return new Appconfiginstances(contextPath.addSegment("systemuser_appconfiginstance_modifiedonbehalfby"));
}
public Appconfigmasters systemuser_appconfigmaster_createdby() {
return new Appconfigmasters(contextPath.addSegment("systemuser_appconfigmaster_createdby"));
}
public Appconfigmasters systemuser_appconfigmaster_createdonbehalfby() {
return new Appconfigmasters(contextPath.addSegment("systemuser_appconfigmaster_createdonbehalfby"));
}
public Appconfigmasters systemuser_appconfigmaster_modifiedby() {
return new Appconfigmasters(contextPath.addSegment("systemuser_appconfigmaster_modifiedby"));
}
public Appconfigmasters systemuser_appconfigmaster_modifiedonbehalfby() {
return new Appconfigmasters(contextPath.addSegment("systemuser_appconfigmaster_modifiedonbehalfby"));
}
public Appmodules systemuser_appmodule_createdby() {
return new Appmodules(contextPath.addSegment("systemuser_appmodule_createdby"));
}
public Appmodules systemuser_appmodule_createdonbehalfby() {
return new Appmodules(contextPath.addSegment("systemuser_appmodule_createdonbehalfby"));
}
public Appmodules systemuser_appmodule_modifiedby() {
return new Appmodules(contextPath.addSegment("systemuser_appmodule_modifiedby"));
}
public Appmodules systemuser_appmodule_modifiedonbehalfby() {
return new Appmodules(contextPath.addSegment("systemuser_appmodule_modifiedonbehalfby"));
}
public Asyncoperations systemUser_AsyncOperations() {
return new Asyncoperations(contextPath.addSegment("SystemUser_AsyncOperations"));
}
public Bulkdeletefailures systemUser_BulkDeleteFailures() {
return new Bulkdeletefailures(contextPath.addSegment("SystemUser_BulkDeleteFailures"));
}
public Callbackregistrations systemuser_callbackregistration_createdby() {
return new Callbackregistrations(contextPath.addSegment("systemuser_callbackregistration_createdby"));
}
public Callbackregistrations systemuser_callbackregistration_createdonbehalfby() {
return new Callbackregistrations(contextPath.addSegment("systemuser_callbackregistration_createdonbehalfby"));
}
public Callbackregistrations systemuser_callbackregistration_modifiedby() {
return new Callbackregistrations(contextPath.addSegment("systemuser_callbackregistration_modifiedby"));
}
public Callbackregistrations systemuser_callbackregistration_modifiedonbehalfby() {
return new Callbackregistrations(contextPath.addSegment("systemuser_callbackregistration_modifiedonbehalfby"));
}
public Connections systemuser_connections1() {
return new Connections(contextPath.addSegment("systemuser_connections1"));
}
public Connections systemuser_connections2() {
return new Connections(contextPath.addSegment("systemuser_connections2"));
}
public Duplicaterecords systemUser_DuplicateBaseRecord() {
return new Duplicaterecords(contextPath.addSegment("SystemUser_DuplicateBaseRecord"));
}
public Duplicaterecords systemUser_DuplicateMatchingRecord() {
return new Duplicaterecords(contextPath.addSegment("SystemUser_DuplicateMatchingRecord"));
}
public Duplicaterules systemUser_DuplicateRules() {
return new Duplicaterules(contextPath.addSegment("SystemUser_DuplicateRules"));
}
public Emails systemUser_Email_EmailSender() {
return new Emails(contextPath.addSegment("SystemUser_Email_EmailSender"));
}
public Importdataset systemUser_ImportData() {
return new Importdataset(contextPath.addSegment("SystemUser_ImportData"));
}
public Importfiles systemUser_ImportFiles() {
return new Importfiles(contextPath.addSegment("SystemUser_ImportFiles"));
}
public Importlogs systemUser_ImportLogs() {
return new Importlogs(contextPath.addSegment("SystemUser_ImportLogs"));
}
public Importmaps systemUser_ImportMaps() {
return new Importmaps(contextPath.addSegment("SystemUser_ImportMaps"));
}
public Imports systemUser_Imports() {
return new Imports(contextPath.addSegment("SystemUser_Imports"));
}
public Navigationsettings systemuser_navigationsetting_createdby() {
return new Navigationsettings(contextPath.addSegment("systemuser_navigationsetting_createdby"));
}
public Navigationsettings systemuser_navigationsetting_createdonbehalfby() {
return new Navigationsettings(contextPath.addSegment("systemuser_navigationsetting_createdonbehalfby"));
}
public Navigationsettings systemuser_navigationsetting_modifiedby() {
return new Navigationsettings(contextPath.addSegment("systemuser_navigationsetting_modifiedby"));
}
public Navigationsettings systemuser_navigationsetting_modifiedonbehalfby() {
return new Navigationsettings(contextPath.addSegment("systemuser_navigationsetting_modifiedonbehalfby"));
}
public Postfollows systemuser_PostFollows() {
return new Postfollows(contextPath.addSegment("systemuser_PostFollows"));
}
public Postregardings systemuser_PostRegardings() {
return new Postregardings(contextPath.addSegment("systemuser_PostRegardings"));
}
public Principalobjectattributeaccessset systemuser_principalobjectattributeaccess() {
return new Principalobjectattributeaccessset(contextPath.addSegment("systemuser_principalobjectattributeaccess"));
}
public Principalobjectattributeaccessset systemuser_principalobjectattributeaccess_principalid() {
return new Principalobjectattributeaccessset(contextPath.addSegment("systemuser_principalobjectattributeaccess_principalid"));
}
public Processsessions systemUser_ProcessSessions() {
return new Processsessions(contextPath.addSegment("SystemUser_ProcessSessions"));
}
public Sitemaps systemuser_SiteMap_createdby() {
return new Sitemaps(contextPath.addSegment("systemuser_SiteMap_createdby"));
}
public Sitemaps systemuser_SiteMap_createdonbehalfby() {
return new Sitemaps(contextPath.addSegment("systemuser_SiteMap_createdonbehalfby"));
}
public Sitemaps systemuser_SiteMap_modifiedby() {
return new Sitemaps(contextPath.addSegment("systemuser_SiteMap_modifiedby"));
}
public Sitemaps systemuser_SiteMap_modifiedonbehalfby() {
return new Sitemaps(contextPath.addSegment("systemuser_SiteMap_modifiedonbehalfby"));
}
public Syncerrors systemUser_SyncError() {
return new Syncerrors(contextPath.addSegment("SystemUser_SyncError"));
}
public Syncerrors systemUser_SyncErrors() {
return new Syncerrors(contextPath.addSegment("SystemUser_SyncErrors"));
}
public Fieldsecurityprofiles systemuserprofiles_association() {
return new Fieldsecurityprofiles(contextPath.addSegment("systemuserprofiles_association"));
}
public Roles systemuserroles_association() {
return new Roles(contextPath.addSegment("systemuserroles_association"));
}
public Teams teammembership_association() {
return new Teams(contextPath.addSegment("teammembership_association"));
}
public Territories territoryid() {
return new Territories(contextPath.addSegment("territoryid"));
}
public Transactioncurrencies transactioncurrencyid() {
return new Transactioncurrencies(contextPath.addSegment("transactioncurrencyid"));
}
public Accounts user_accounts() {
return new Accounts(contextPath.addSegment("user_accounts"));
}
public Activitypointers user_activity() {
return new Activitypointers(contextPath.addSegment("user_activity"));
}
public Appointments user_appointment() {
return new Appointments(contextPath.addSegment("user_appointment"));
}
public Connectionreferences user_connectionreference() {
return new Connectionreferences(contextPath.addSegment("user_connectionreference"));
}
public Connectors user_connector() {
return new Connectors(contextPath.addSegment("user_connector"));
}
public Emails user_email() {
return new Emails(contextPath.addSegment("user_email"));
}
public Environmentvariabledefinitions user_environmentvariabledefinition() {
return new Environmentvariabledefinitions(contextPath.addSegment("user_environmentvariabledefinition"));
}
public Environmentvariablevalues user_environmentvariablevalue() {
return new Environmentvariablevalues(contextPath.addSegment("user_environmentvariablevalue"));
}
public Exchangesyncidmappings user_exchangesyncidmapping() {
return new Exchangesyncidmappings(contextPath.addSegment("user_exchangesyncidmapping"));
}
public Exportsolutionuploads user_exportsolutionupload() {
return new Exportsolutionuploads(contextPath.addSegment("user_exportsolutionupload"));
}
public Faxes user_fax() {
return new Faxes(contextPath.addSegment("user_fax"));
}
public Flowsessions user_flowsession() {
return new Flowsessions(contextPath.addSegment("user_flowsession"));
}
public Ggw_crews user_ggw_crew() {
return new Ggw_crews(contextPath.addSegment("user_ggw_crew"));
}
public Ggw_events user_ggw_event() {
return new Ggw_events(contextPath.addSegment("user_ggw_event"));
}
public Ggw_teams user_ggw_team() {
return new Ggw_teams(contextPath.addSegment("user_ggw_team"));
}
public Ggw_team_applications user_ggw_team_application() {
return new Ggw_team_applications(contextPath.addSegment("user_ggw_team_application"));
}
public Goals user_goal() {
return new Goals(contextPath.addSegment("user_goal"));
}
public Goals user_goal_goalowner() {
return new Goals(contextPath.addSegment("user_goal_goalowner"));
}
public Knowledgearticles user_knowledgearticle() {
return new Knowledgearticles(contextPath.addSegment("user_knowledgearticle"));
}
public Letters user_letter() {
return new Letters(contextPath.addSegment("user_letter"));
}
public Mailboxes user_mailbox() {
return new Mailboxes(contextPath.addSegment("user_mailbox"));
}
public Msdyn_aibdatasets user_msdyn_aibdataset() {
return new Msdyn_aibdatasets(contextPath.addSegment("user_msdyn_aibdataset"));
}
public Msdyn_aibdatasetfiles user_msdyn_aibdatasetfile() {
return new Msdyn_aibdatasetfiles(contextPath.addSegment("user_msdyn_aibdatasetfile"));
}
public Msdyn_aibdatasetrecords user_msdyn_aibdatasetrecord() {
return new Msdyn_aibdatasetrecords(contextPath.addSegment("user_msdyn_aibdatasetrecord"));
}
public Msdyn_aibdatasetscontainers user_msdyn_aibdatasetscontainer() {
return new Msdyn_aibdatasetscontainers(contextPath.addSegment("user_msdyn_aibdatasetscontainer"));
}
public Msdyn_aibfiles user_msdyn_aibfile() {
return new Msdyn_aibfiles(contextPath.addSegment("user_msdyn_aibfile"));
}
public Msdyn_aibfileattacheddatas user_msdyn_aibfileattacheddata() {
return new Msdyn_aibfileattacheddatas(contextPath.addSegment("user_msdyn_aibfileattacheddata"));
}
public Msdyn_aiconfigurations user_msdyn_aiconfiguration() {
return new Msdyn_aiconfigurations(contextPath.addSegment("user_msdyn_aiconfiguration"));
}
public Msdyn_aifptrainingdocuments user_msdyn_aifptrainingdocument() {
return new Msdyn_aifptrainingdocuments(contextPath.addSegment("user_msdyn_aifptrainingdocument"));
}
public Msdyn_aimodels user_msdyn_aimodel() {
return new Msdyn_aimodels(contextPath.addSegment("user_msdyn_aimodel"));
}
public Msdyn_aiodimages user_msdyn_aiodimage() {
return new Msdyn_aiodimages(contextPath.addSegment("user_msdyn_aiodimage"));
}
public Msdyn_aiodlabels user_msdyn_aiodlabel() {
return new Msdyn_aiodlabels(contextPath.addSegment("user_msdyn_aiodlabel"));
}
public Msdyn_aiodtrainingboundingboxes user_msdyn_aiodtrainingboundingbox() {
return new Msdyn_aiodtrainingboundingboxes(contextPath.addSegment("user_msdyn_aiodtrainingboundingbox"));
}
public Msdyn_aiodtrainingimages user_msdyn_aiodtrainingimage() {
return new Msdyn_aiodtrainingimages(contextPath.addSegment("user_msdyn_aiodtrainingimage"));
}
public Msdyn_aitemplates user_msdyn_aitemplate() {
return new Msdyn_aitemplates(contextPath.addSegment("user_msdyn_aitemplate"));
}
public Msdyn_analysiscomponents user_msdyn_analysiscomponent() {
return new Msdyn_analysiscomponents(contextPath.addSegment("user_msdyn_analysiscomponent"));
}
public Msdyn_analysisjobs user_msdyn_analysisjob() {
return new Msdyn_analysisjobs(contextPath.addSegment("user_msdyn_analysisjob"));
}
public Msdyn_analysisresults user_msdyn_analysisresult() {
return new Msdyn_analysisresults(contextPath.addSegment("user_msdyn_analysisresult"));
}
public Msdyn_analysisresultdetails user_msdyn_analysisresultdetail() {
return new Msdyn_analysisresultdetails(contextPath.addSegment("user_msdyn_analysisresultdetail"));
}
public Msdyn_dataflows user_msdyn_dataflow() {
return new Msdyn_dataflows(contextPath.addSegment("user_msdyn_dataflow"));
}
public Msdyn_knowledgearticleimages user_msdyn_knowledgearticleimage() {
return new Msdyn_knowledgearticleimages(contextPath.addSegment("user_msdyn_knowledgearticleimage"));
}
public Msdyn_knowledgearticletemplates user_msdyn_knowledgearticletemplate() {
return new Msdyn_knowledgearticletemplates(contextPath.addSegment("user_msdyn_knowledgearticletemplate"));
}
public Msdyn_richtextfiles user_msdyn_richtextfile() {
return new Msdyn_richtextfiles(contextPath.addSegment("user_msdyn_richtextfile"));
}
public Msdyn_serviceconfigurations user_msdyn_serviceconfiguration() {
return new Msdyn_serviceconfigurations(contextPath.addSegment("user_msdyn_serviceconfiguration"));
}
public Msdyn_slakpis user_msdyn_slakpi() {
return new Msdyn_slakpis(contextPath.addSegment("user_msdyn_slakpi"));
}
public Msdyn_solutionhealthrules user_msdyn_solutionhealthrule() {
return new Msdyn_solutionhealthrules(contextPath.addSegment("user_msdyn_solutionhealthrule"));
}
public Msdyn_solutionhealthrulearguments user_msdyn_solutionhealthruleargument() {
return new Msdyn_solutionhealthrulearguments(contextPath.addSegment("user_msdyn_solutionhealthruleargument"));
}
public Interactionforemails user_new_interactionforemail() {
return new Interactionforemails(contextPath.addSegment("user_new_interactionforemail"));
}
public Postfollows user_owner_postfollows() {
return new Postfollows(contextPath.addSegment("user_owner_postfollows"));
}
public Systemusers user_parent_user() {
return new Systemusers(contextPath.addSegment("user_parent_user"));
}
public Phonecalls user_phonecall() {
return new Phonecalls(contextPath.addSegment("user_phonecall"));
}
public Processstageparameters user_processstageparameter() {
return new Processstageparameters(contextPath.addSegment("user_processstageparameter"));
}
public Recurringappointmentmasters user_recurringappointmentmaster() {
return new Recurringappointmentmasters(contextPath.addSegment("user_recurringappointmentmaster"));
}
public Usersettingscollection user_settings() {
return new Usersettingscollection(contextPath.addSegment("user_settings"));
}
public Sharepointdocumentlocations user_sharepointdocumentlocation() {
return new Sharepointdocumentlocations(contextPath.addSegment("user_sharepointdocumentlocation"));
}
public Sharepointsites user_sharepointsite() {
return new Sharepointsites(contextPath.addSegment("user_sharepointsite"));
}
public Slas user_slabase() {
return new Slas(contextPath.addSegment("user_slabase"));
}
public Socialactivities user_socialactivity() {
return new Socialactivities(contextPath.addSegment("user_socialactivity"));
}
public Stagesolutionuploads user_stagesolutionupload() {
return new Stagesolutionuploads(contextPath.addSegment("user_stagesolutionupload"));
}
public Tasks user_task() {
return new Tasks(contextPath.addSegment("user_task"));
}
public Userforms user_userform() {
return new Userforms(contextPath.addSegment("user_userform"));
}
public Userqueries user_userquery() {
return new Userqueries(contextPath.addSegment("user_userquery"));
}
public Userqueryvisualizations user_userqueryvisualizations() {
return new Userqueryvisualizations(contextPath.addSegment("user_userqueryvisualizations"));
}
public Workflowbinaries user_workflowbinary() {
return new Workflowbinaries(contextPath.addSegment("user_workflowbinary"));
}
public Webresourceset webresource_createdby() {
return new Webresourceset(contextPath.addSegment("webresource_createdby"));
}
public Webresourceset webresource_modifiedby() {
return new Webresourceset(contextPath.addSegment("webresource_modifiedby"));
}
public Workflows workflow_createdby() {
return new Workflows(contextPath.addSegment("workflow_createdby"));
}
public Workflows workflow_createdonbehalfby() {
return new Workflows(contextPath.addSegment("workflow_createdonbehalfby"));
}
public Workflows workflow_modifiedby() {
return new Workflows(contextPath.addSegment("workflow_modifiedby"));
}
public Workflows workflow_modifiedonbehalfby() {
return new Workflows(contextPath.addSegment("workflow_modifiedonbehalfby"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy