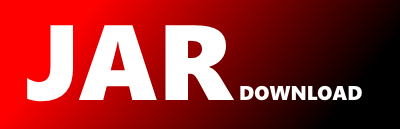
odata.msgraph.client.beta.complex.ComanagementEligibleDevicesSummary Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"comanagedCount",
"eligibleButNotAzureAdJoinedCount",
"eligibleCount",
"ineligibleCount",
"needsOsUpdateCount"})
@JsonInclude(Include.NON_NULL)
public class ComanagementEligibleDevicesSummary implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("comanagedCount")
protected Integer comanagedCount;
@JsonProperty("eligibleButNotAzureAdJoinedCount")
protected Integer eligibleButNotAzureAdJoinedCount;
@JsonProperty("eligibleCount")
protected Integer eligibleCount;
@JsonProperty("ineligibleCount")
protected Integer ineligibleCount;
@JsonProperty("needsOsUpdateCount")
protected Integer needsOsUpdateCount;
protected ComanagementEligibleDevicesSummary() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.comanagementEligibleDevicesSummary";
}
/**
* “Count of devices already Co-Managed”
*
* @return property comanagedCount
*/
@Property(name="comanagedCount")
@JsonIgnore
public Optional getComanagedCount() {
return Optional.ofNullable(comanagedCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code comanagedCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Count of devices already Co-Managed”
*
* @param comanagedCount
* new value of {@code comanagedCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code comanagedCount} field changed
*/
public ComanagementEligibleDevicesSummary withComanagedCount(Integer comanagedCount) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.comanagementEligibleDevicesSummary");
_x.comanagedCount = comanagedCount;
return _x;
}
/**
* “Count of devices eligible for Co-Management but not yet joined to Azure Active
* Directory”
*
* @return property eligibleButNotAzureAdJoinedCount
*/
@Property(name="eligibleButNotAzureAdJoinedCount")
@JsonIgnore
public Optional getEligibleButNotAzureAdJoinedCount() {
return Optional.ofNullable(eligibleButNotAzureAdJoinedCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* eligibleButNotAzureAdJoinedCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Count of devices eligible for Co-Management but not yet joined to Azure Active
* Directory”
*
* @param eligibleButNotAzureAdJoinedCount
* new value of {@code eligibleButNotAzureAdJoinedCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code eligibleButNotAzureAdJoinedCount} field changed
*/
public ComanagementEligibleDevicesSummary withEligibleButNotAzureAdJoinedCount(Integer eligibleButNotAzureAdJoinedCount) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.comanagementEligibleDevicesSummary");
_x.eligibleButNotAzureAdJoinedCount = eligibleButNotAzureAdJoinedCount;
return _x;
}
/**
* “Count of devices fully eligible for Co-Management”
*
* @return property eligibleCount
*/
@Property(name="eligibleCount")
@JsonIgnore
public Optional getEligibleCount() {
return Optional.ofNullable(eligibleCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code eligibleCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Count of devices fully eligible for Co-Management”
*
* @param eligibleCount
* new value of {@code eligibleCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code eligibleCount} field changed
*/
public ComanagementEligibleDevicesSummary withEligibleCount(Integer eligibleCount) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.comanagementEligibleDevicesSummary");
_x.eligibleCount = eligibleCount;
return _x;
}
/**
* “Count of devices ineligible for Co-Management”
*
* @return property ineligibleCount
*/
@Property(name="ineligibleCount")
@JsonIgnore
public Optional getIneligibleCount() {
return Optional.ofNullable(ineligibleCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code ineligibleCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Count of devices ineligible for Co-Management”
*
* @param ineligibleCount
* new value of {@code ineligibleCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code ineligibleCount} field changed
*/
public ComanagementEligibleDevicesSummary withIneligibleCount(Integer ineligibleCount) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.comanagementEligibleDevicesSummary");
_x.ineligibleCount = ineligibleCount;
return _x;
}
/**
* “Count of devices that will be eligible for Co-Management after an OS update”
*
* @return property needsOsUpdateCount
*/
@Property(name="needsOsUpdateCount")
@JsonIgnore
public Optional getNeedsOsUpdateCount() {
return Optional.ofNullable(needsOsUpdateCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* needsOsUpdateCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Count of devices that will be eligible for Co-Management after an OS update”
*
* @param needsOsUpdateCount
* new value of {@code needsOsUpdateCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code needsOsUpdateCount} field changed
*/
public ComanagementEligibleDevicesSummary withNeedsOsUpdateCount(Integer needsOsUpdateCount) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.comanagementEligibleDevicesSummary");
_x.needsOsUpdateCount = needsOsUpdateCount;
return _x;
}
public ComanagementEligibleDevicesSummary withUnmappedField(String name, String value) {
ComanagementEligibleDevicesSummary _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer comanagedCount;
private Integer eligibleButNotAzureAdJoinedCount;
private Integer eligibleCount;
private Integer ineligibleCount;
private Integer needsOsUpdateCount;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Count of devices already Co-Managed”
*
* @param comanagedCount
* value of {@code comanagedCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder comanagedCount(Integer comanagedCount) {
this.comanagedCount = comanagedCount;
this.changedFields = changedFields.add("comanagedCount");
return this;
}
/**
* “Count of devices eligible for Co-Management but not yet joined to Azure Active
* Directory”
*
* @param eligibleButNotAzureAdJoinedCount
* value of {@code eligibleButNotAzureAdJoinedCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder eligibleButNotAzureAdJoinedCount(Integer eligibleButNotAzureAdJoinedCount) {
this.eligibleButNotAzureAdJoinedCount = eligibleButNotAzureAdJoinedCount;
this.changedFields = changedFields.add("eligibleButNotAzureAdJoinedCount");
return this;
}
/**
* “Count of devices fully eligible for Co-Management”
*
* @param eligibleCount
* value of {@code eligibleCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder eligibleCount(Integer eligibleCount) {
this.eligibleCount = eligibleCount;
this.changedFields = changedFields.add("eligibleCount");
return this;
}
/**
* “Count of devices ineligible for Co-Management”
*
* @param ineligibleCount
* value of {@code ineligibleCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder ineligibleCount(Integer ineligibleCount) {
this.ineligibleCount = ineligibleCount;
this.changedFields = changedFields.add("ineligibleCount");
return this;
}
/**
* “Count of devices that will be eligible for Co-Management after an OS update”
*
* @param needsOsUpdateCount
* value of {@code needsOsUpdateCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder needsOsUpdateCount(Integer needsOsUpdateCount) {
this.needsOsUpdateCount = needsOsUpdateCount;
this.changedFields = changedFields.add("needsOsUpdateCount");
return this;
}
public ComanagementEligibleDevicesSummary build() {
ComanagementEligibleDevicesSummary _x = new ComanagementEligibleDevicesSummary();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.comanagementEligibleDevicesSummary";
_x.comanagedCount = comanagedCount;
_x.eligibleButNotAzureAdJoinedCount = eligibleButNotAzureAdJoinedCount;
_x.eligibleCount = eligibleCount;
_x.ineligibleCount = ineligibleCount;
_x.needsOsUpdateCount = needsOsUpdateCount;
return _x;
}
}
private ComanagementEligibleDevicesSummary _copy() {
ComanagementEligibleDevicesSummary _x = new ComanagementEligibleDevicesSummary();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.comanagedCount = comanagedCount;
_x.eligibleButNotAzureAdJoinedCount = eligibleButNotAzureAdJoinedCount;
_x.eligibleCount = eligibleCount;
_x.ineligibleCount = ineligibleCount;
_x.needsOsUpdateCount = needsOsUpdateCount;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ComanagementEligibleDevicesSummary[");
b.append("comanagedCount=");
b.append(this.comanagedCount);
b.append(", ");
b.append("eligibleButNotAzureAdJoinedCount=");
b.append(this.eligibleButNotAzureAdJoinedCount);
b.append(", ");
b.append("eligibleCount=");
b.append(this.eligibleCount);
b.append(", ");
b.append("ineligibleCount=");
b.append(this.ineligibleCount);
b.append(", ");
b.append("needsOsUpdateCount=");
b.append(this.needsOsUpdateCount);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}