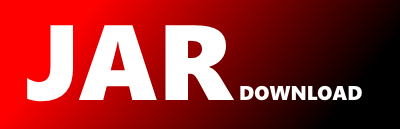
odata.msgraph.client.beta.complex.ConditionalAccessConditionSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.enums.ConditionalAccessClientApp;
import odata.msgraph.client.beta.enums.RiskLevel;
@JsonPropertyOrder({
"@odata.type",
"applications",
"clientApplications",
"clientAppTypes",
"devices",
"deviceStates",
"locations",
"platforms",
"signInRiskLevels",
"userRiskLevels",
"users"})
@JsonInclude(Include.NON_NULL)
public class ConditionalAccessConditionSet implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("applications")
protected ConditionalAccessApplications applications;
@JsonProperty("clientApplications")
protected ConditionalAccessClientApplications clientApplications;
@JsonProperty("clientAppTypes")
protected List clientAppTypes;
@JsonProperty("clientAppTypes@nextLink")
protected String clientAppTypesNextLink;
@JsonProperty("devices")
protected ConditionalAccessDevices devices;
@JsonProperty("deviceStates")
protected ConditionalAccessDeviceStates deviceStates;
@JsonProperty("locations")
protected ConditionalAccessLocations locations;
@JsonProperty("platforms")
protected ConditionalAccessPlatforms platforms;
@JsonProperty("signInRiskLevels")
protected List signInRiskLevels;
@JsonProperty("signInRiskLevels@nextLink")
protected String signInRiskLevelsNextLink;
@JsonProperty("userRiskLevels")
protected List userRiskLevels;
@JsonProperty("userRiskLevels@nextLink")
protected String userRiskLevelsNextLink;
@JsonProperty("users")
protected ConditionalAccessUsers users;
protected ConditionalAccessConditionSet() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.conditionalAccessConditionSet";
}
@Property(name="applications")
@JsonIgnore
public Optional getApplications() {
return Optional.ofNullable(applications);
}
public ConditionalAccessConditionSet withApplications(ConditionalAccessApplications applications) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.applications = applications;
return _x;
}
@Property(name="clientApplications")
@JsonIgnore
public Optional getClientApplications() {
return Optional.ofNullable(clientApplications);
}
public ConditionalAccessConditionSet withClientApplications(ConditionalAccessClientApplications clientApplications) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.clientApplications = clientApplications;
return _x;
}
@Property(name="clientAppTypes")
@JsonIgnore
public CollectionPage getClientAppTypes() {
return new CollectionPage(contextPath, ConditionalAccessClientApp.class, this.clientAppTypes, Optional.ofNullable(clientAppTypesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="clientAppTypes")
@JsonIgnore
public CollectionPage getClientAppTypes(HttpRequestOptions options) {
return new CollectionPage(contextPath, ConditionalAccessClientApp.class, this.clientAppTypes, Optional.ofNullable(clientAppTypesNextLink), Collections.emptyList(), options);
}
@Property(name="devices")
@JsonIgnore
public Optional getDevices() {
return Optional.ofNullable(devices);
}
public ConditionalAccessConditionSet withDevices(ConditionalAccessDevices devices) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.devices = devices;
return _x;
}
@Property(name="deviceStates")
@JsonIgnore
public Optional getDeviceStates() {
return Optional.ofNullable(deviceStates);
}
public ConditionalAccessConditionSet withDeviceStates(ConditionalAccessDeviceStates deviceStates) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.deviceStates = deviceStates;
return _x;
}
@Property(name="locations")
@JsonIgnore
public Optional getLocations() {
return Optional.ofNullable(locations);
}
public ConditionalAccessConditionSet withLocations(ConditionalAccessLocations locations) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.locations = locations;
return _x;
}
@Property(name="platforms")
@JsonIgnore
public Optional getPlatforms() {
return Optional.ofNullable(platforms);
}
public ConditionalAccessConditionSet withPlatforms(ConditionalAccessPlatforms platforms) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.platforms = platforms;
return _x;
}
@Property(name="signInRiskLevels")
@JsonIgnore
public CollectionPage getSignInRiskLevels() {
return new CollectionPage(contextPath, RiskLevel.class, this.signInRiskLevels, Optional.ofNullable(signInRiskLevelsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="signInRiskLevels")
@JsonIgnore
public CollectionPage getSignInRiskLevels(HttpRequestOptions options) {
return new CollectionPage(contextPath, RiskLevel.class, this.signInRiskLevels, Optional.ofNullable(signInRiskLevelsNextLink), Collections.emptyList(), options);
}
@Property(name="userRiskLevels")
@JsonIgnore
public CollectionPage getUserRiskLevels() {
return new CollectionPage(contextPath, RiskLevel.class, this.userRiskLevels, Optional.ofNullable(userRiskLevelsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="userRiskLevels")
@JsonIgnore
public CollectionPage getUserRiskLevels(HttpRequestOptions options) {
return new CollectionPage(contextPath, RiskLevel.class, this.userRiskLevels, Optional.ofNullable(userRiskLevelsNextLink), Collections.emptyList(), options);
}
@Property(name="users")
@JsonIgnore
public Optional getUsers() {
return Optional.ofNullable(users);
}
public ConditionalAccessConditionSet withUsers(ConditionalAccessUsers users) {
ConditionalAccessConditionSet _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.conditionalAccessConditionSet");
_x.users = users;
return _x;
}
public ConditionalAccessConditionSet withUnmappedField(String name, String value) {
ConditionalAccessConditionSet _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private ConditionalAccessApplications applications;
private ConditionalAccessClientApplications clientApplications;
private List clientAppTypes;
private String clientAppTypesNextLink;
private ConditionalAccessDevices devices;
private ConditionalAccessDeviceStates deviceStates;
private ConditionalAccessLocations locations;
private ConditionalAccessPlatforms platforms;
private List signInRiskLevels;
private String signInRiskLevelsNextLink;
private List userRiskLevels;
private String userRiskLevelsNextLink;
private ConditionalAccessUsers users;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder applications(ConditionalAccessApplications applications) {
this.applications = applications;
this.changedFields = changedFields.add("applications");
return this;
}
public Builder clientApplications(ConditionalAccessClientApplications clientApplications) {
this.clientApplications = clientApplications;
this.changedFields = changedFields.add("clientApplications");
return this;
}
public Builder clientAppTypes(List clientAppTypes) {
this.clientAppTypes = clientAppTypes;
this.changedFields = changedFields.add("clientAppTypes");
return this;
}
public Builder clientAppTypes(ConditionalAccessClientApp... clientAppTypes) {
return clientAppTypes(Arrays.asList(clientAppTypes));
}
public Builder clientAppTypesNextLink(String clientAppTypesNextLink) {
this.clientAppTypesNextLink = clientAppTypesNextLink;
this.changedFields = changedFields.add("clientAppTypes");
return this;
}
public Builder devices(ConditionalAccessDevices devices) {
this.devices = devices;
this.changedFields = changedFields.add("devices");
return this;
}
public Builder deviceStates(ConditionalAccessDeviceStates deviceStates) {
this.deviceStates = deviceStates;
this.changedFields = changedFields.add("deviceStates");
return this;
}
public Builder locations(ConditionalAccessLocations locations) {
this.locations = locations;
this.changedFields = changedFields.add("locations");
return this;
}
public Builder platforms(ConditionalAccessPlatforms platforms) {
this.platforms = platforms;
this.changedFields = changedFields.add("platforms");
return this;
}
public Builder signInRiskLevels(List signInRiskLevels) {
this.signInRiskLevels = signInRiskLevels;
this.changedFields = changedFields.add("signInRiskLevels");
return this;
}
public Builder signInRiskLevels(RiskLevel... signInRiskLevels) {
return signInRiskLevels(Arrays.asList(signInRiskLevels));
}
public Builder signInRiskLevelsNextLink(String signInRiskLevelsNextLink) {
this.signInRiskLevelsNextLink = signInRiskLevelsNextLink;
this.changedFields = changedFields.add("signInRiskLevels");
return this;
}
public Builder userRiskLevels(List userRiskLevels) {
this.userRiskLevels = userRiskLevels;
this.changedFields = changedFields.add("userRiskLevels");
return this;
}
public Builder userRiskLevels(RiskLevel... userRiskLevels) {
return userRiskLevels(Arrays.asList(userRiskLevels));
}
public Builder userRiskLevelsNextLink(String userRiskLevelsNextLink) {
this.userRiskLevelsNextLink = userRiskLevelsNextLink;
this.changedFields = changedFields.add("userRiskLevels");
return this;
}
public Builder users(ConditionalAccessUsers users) {
this.users = users;
this.changedFields = changedFields.add("users");
return this;
}
public ConditionalAccessConditionSet build() {
ConditionalAccessConditionSet _x = new ConditionalAccessConditionSet();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.conditionalAccessConditionSet";
_x.applications = applications;
_x.clientApplications = clientApplications;
_x.clientAppTypes = clientAppTypes;
_x.clientAppTypesNextLink = clientAppTypesNextLink;
_x.devices = devices;
_x.deviceStates = deviceStates;
_x.locations = locations;
_x.platforms = platforms;
_x.signInRiskLevels = signInRiskLevels;
_x.signInRiskLevelsNextLink = signInRiskLevelsNextLink;
_x.userRiskLevels = userRiskLevels;
_x.userRiskLevelsNextLink = userRiskLevelsNextLink;
_x.users = users;
return _x;
}
}
private ConditionalAccessConditionSet _copy() {
ConditionalAccessConditionSet _x = new ConditionalAccessConditionSet();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.applications = applications;
_x.clientApplications = clientApplications;
_x.clientAppTypes = clientAppTypes;
_x.devices = devices;
_x.deviceStates = deviceStates;
_x.locations = locations;
_x.platforms = platforms;
_x.signInRiskLevels = signInRiskLevels;
_x.userRiskLevels = userRiskLevels;
_x.users = users;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ConditionalAccessConditionSet[");
b.append("applications=");
b.append(this.applications);
b.append(", ");
b.append("clientApplications=");
b.append(this.clientApplications);
b.append(", ");
b.append("clientAppTypes=");
b.append(this.clientAppTypes);
b.append(", ");
b.append("devices=");
b.append(this.devices);
b.append(", ");
b.append("deviceStates=");
b.append(this.deviceStates);
b.append(", ");
b.append("locations=");
b.append(this.locations);
b.append(", ");
b.append("platforms=");
b.append(this.platforms);
b.append(", ");
b.append("signInRiskLevels=");
b.append(this.signInRiskLevels);
b.append(", ");
b.append("userRiskLevels=");
b.append(this.userRiskLevels);
b.append(", ");
b.append("users=");
b.append(this.users);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy