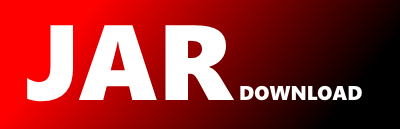
odata.msgraph.client.beta.complex.ConditionalAccessUsers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"excludeGroups",
"excludeRoles",
"excludeUsers",
"includeGroups",
"includeRoles",
"includeUsers"})
@JsonInclude(Include.NON_NULL)
public class ConditionalAccessUsers implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("excludeGroups")
protected List excludeGroups;
@JsonProperty("excludeGroups@nextLink")
protected String excludeGroupsNextLink;
@JsonProperty("excludeRoles")
protected List excludeRoles;
@JsonProperty("excludeRoles@nextLink")
protected String excludeRolesNextLink;
@JsonProperty("excludeUsers")
protected List excludeUsers;
@JsonProperty("excludeUsers@nextLink")
protected String excludeUsersNextLink;
@JsonProperty("includeGroups")
protected List includeGroups;
@JsonProperty("includeGroups@nextLink")
protected String includeGroupsNextLink;
@JsonProperty("includeRoles")
protected List includeRoles;
@JsonProperty("includeRoles@nextLink")
protected String includeRolesNextLink;
@JsonProperty("includeUsers")
protected List includeUsers;
@JsonProperty("includeUsers@nextLink")
protected String includeUsersNextLink;
protected ConditionalAccessUsers() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.conditionalAccessUsers";
}
@Property(name="excludeGroups")
@JsonIgnore
public CollectionPage getExcludeGroups() {
return new CollectionPage(contextPath, String.class, this.excludeGroups, Optional.ofNullable(excludeGroupsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="excludeGroups")
@JsonIgnore
public CollectionPage getExcludeGroups(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.excludeGroups, Optional.ofNullable(excludeGroupsNextLink), Collections.emptyList(), options);
}
@Property(name="excludeRoles")
@JsonIgnore
public CollectionPage getExcludeRoles() {
return new CollectionPage(contextPath, String.class, this.excludeRoles, Optional.ofNullable(excludeRolesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="excludeRoles")
@JsonIgnore
public CollectionPage getExcludeRoles(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.excludeRoles, Optional.ofNullable(excludeRolesNextLink), Collections.emptyList(), options);
}
@Property(name="excludeUsers")
@JsonIgnore
public CollectionPage getExcludeUsers() {
return new CollectionPage(contextPath, String.class, this.excludeUsers, Optional.ofNullable(excludeUsersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="excludeUsers")
@JsonIgnore
public CollectionPage getExcludeUsers(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.excludeUsers, Optional.ofNullable(excludeUsersNextLink), Collections.emptyList(), options);
}
@Property(name="includeGroups")
@JsonIgnore
public CollectionPage getIncludeGroups() {
return new CollectionPage(contextPath, String.class, this.includeGroups, Optional.ofNullable(includeGroupsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="includeGroups")
@JsonIgnore
public CollectionPage getIncludeGroups(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.includeGroups, Optional.ofNullable(includeGroupsNextLink), Collections.emptyList(), options);
}
@Property(name="includeRoles")
@JsonIgnore
public CollectionPage getIncludeRoles() {
return new CollectionPage(contextPath, String.class, this.includeRoles, Optional.ofNullable(includeRolesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="includeRoles")
@JsonIgnore
public CollectionPage getIncludeRoles(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.includeRoles, Optional.ofNullable(includeRolesNextLink), Collections.emptyList(), options);
}
@Property(name="includeUsers")
@JsonIgnore
public CollectionPage getIncludeUsers() {
return new CollectionPage(contextPath, String.class, this.includeUsers, Optional.ofNullable(includeUsersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="includeUsers")
@JsonIgnore
public CollectionPage getIncludeUsers(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.includeUsers, Optional.ofNullable(includeUsersNextLink), Collections.emptyList(), options);
}
public ConditionalAccessUsers withUnmappedField(String name, String value) {
ConditionalAccessUsers _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private List excludeGroups;
private String excludeGroupsNextLink;
private List excludeRoles;
private String excludeRolesNextLink;
private List excludeUsers;
private String excludeUsersNextLink;
private List includeGroups;
private String includeGroupsNextLink;
private List includeRoles;
private String includeRolesNextLink;
private List includeUsers;
private String includeUsersNextLink;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder excludeGroups(List excludeGroups) {
this.excludeGroups = excludeGroups;
this.changedFields = changedFields.add("excludeGroups");
return this;
}
public Builder excludeGroups(String... excludeGroups) {
return excludeGroups(Arrays.asList(excludeGroups));
}
public Builder excludeGroupsNextLink(String excludeGroupsNextLink) {
this.excludeGroupsNextLink = excludeGroupsNextLink;
this.changedFields = changedFields.add("excludeGroups");
return this;
}
public Builder excludeRoles(List excludeRoles) {
this.excludeRoles = excludeRoles;
this.changedFields = changedFields.add("excludeRoles");
return this;
}
public Builder excludeRoles(String... excludeRoles) {
return excludeRoles(Arrays.asList(excludeRoles));
}
public Builder excludeRolesNextLink(String excludeRolesNextLink) {
this.excludeRolesNextLink = excludeRolesNextLink;
this.changedFields = changedFields.add("excludeRoles");
return this;
}
public Builder excludeUsers(List excludeUsers) {
this.excludeUsers = excludeUsers;
this.changedFields = changedFields.add("excludeUsers");
return this;
}
public Builder excludeUsers(String... excludeUsers) {
return excludeUsers(Arrays.asList(excludeUsers));
}
public Builder excludeUsersNextLink(String excludeUsersNextLink) {
this.excludeUsersNextLink = excludeUsersNextLink;
this.changedFields = changedFields.add("excludeUsers");
return this;
}
public Builder includeGroups(List includeGroups) {
this.includeGroups = includeGroups;
this.changedFields = changedFields.add("includeGroups");
return this;
}
public Builder includeGroups(String... includeGroups) {
return includeGroups(Arrays.asList(includeGroups));
}
public Builder includeGroupsNextLink(String includeGroupsNextLink) {
this.includeGroupsNextLink = includeGroupsNextLink;
this.changedFields = changedFields.add("includeGroups");
return this;
}
public Builder includeRoles(List includeRoles) {
this.includeRoles = includeRoles;
this.changedFields = changedFields.add("includeRoles");
return this;
}
public Builder includeRoles(String... includeRoles) {
return includeRoles(Arrays.asList(includeRoles));
}
public Builder includeRolesNextLink(String includeRolesNextLink) {
this.includeRolesNextLink = includeRolesNextLink;
this.changedFields = changedFields.add("includeRoles");
return this;
}
public Builder includeUsers(List includeUsers) {
this.includeUsers = includeUsers;
this.changedFields = changedFields.add("includeUsers");
return this;
}
public Builder includeUsers(String... includeUsers) {
return includeUsers(Arrays.asList(includeUsers));
}
public Builder includeUsersNextLink(String includeUsersNextLink) {
this.includeUsersNextLink = includeUsersNextLink;
this.changedFields = changedFields.add("includeUsers");
return this;
}
public ConditionalAccessUsers build() {
ConditionalAccessUsers _x = new ConditionalAccessUsers();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.conditionalAccessUsers";
_x.excludeGroups = excludeGroups;
_x.excludeGroupsNextLink = excludeGroupsNextLink;
_x.excludeRoles = excludeRoles;
_x.excludeRolesNextLink = excludeRolesNextLink;
_x.excludeUsers = excludeUsers;
_x.excludeUsersNextLink = excludeUsersNextLink;
_x.includeGroups = includeGroups;
_x.includeGroupsNextLink = includeGroupsNextLink;
_x.includeRoles = includeRoles;
_x.includeRolesNextLink = includeRolesNextLink;
_x.includeUsers = includeUsers;
_x.includeUsersNextLink = includeUsersNextLink;
return _x;
}
}
private ConditionalAccessUsers _copy() {
ConditionalAccessUsers _x = new ConditionalAccessUsers();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.excludeGroups = excludeGroups;
_x.excludeRoles = excludeRoles;
_x.excludeUsers = excludeUsers;
_x.includeGroups = includeGroups;
_x.includeRoles = includeRoles;
_x.includeUsers = includeUsers;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ConditionalAccessUsers[");
b.append("excludeGroups=");
b.append(this.excludeGroups);
b.append(", ");
b.append("excludeRoles=");
b.append(this.excludeRoles);
b.append(", ");
b.append("excludeUsers=");
b.append(this.excludeUsers);
b.append(", ");
b.append("includeGroups=");
b.append(this.includeGroups);
b.append(", ");
b.append("includeRoles=");
b.append(this.includeRoles);
b.append(", ");
b.append("includeUsers=");
b.append(this.includeUsers);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy