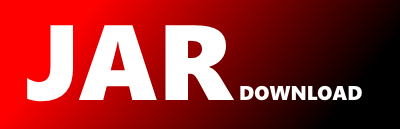
odata.msgraph.client.beta.complex.CopyNotebookModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.beta.enums.OnenoteUserRole;
@JsonPropertyOrder({
"@odata.type",
"createdBy",
"createdByIdentity",
"createdTime",
"id",
"isDefault",
"isShared",
"lastModifiedBy",
"lastModifiedByIdentity",
"lastModifiedTime",
"links",
"name",
"sectionGroupsUrl",
"sectionsUrl",
"self",
"userRole"})
@JsonInclude(Include.NON_NULL)
public class CopyNotebookModel implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("createdBy")
protected String createdBy;
@JsonProperty("createdByIdentity")
protected IdentitySet createdByIdentity;
@JsonProperty("createdTime")
protected OffsetDateTime createdTime;
@JsonProperty("id")
protected String id;
@JsonProperty("isDefault")
protected Boolean isDefault;
@JsonProperty("isShared")
protected Boolean isShared;
@JsonProperty("lastModifiedBy")
protected String lastModifiedBy;
@JsonProperty("lastModifiedByIdentity")
protected IdentitySet lastModifiedByIdentity;
@JsonProperty("lastModifiedTime")
protected OffsetDateTime lastModifiedTime;
@JsonProperty("links")
protected NotebookLinks links;
@JsonProperty("name")
protected String name;
@JsonProperty("sectionGroupsUrl")
protected String sectionGroupsUrl;
@JsonProperty("sectionsUrl")
protected String sectionsUrl;
@JsonProperty("self")
protected String self;
@JsonProperty("userRole")
protected OnenoteUserRole userRole;
protected CopyNotebookModel() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.CopyNotebookModel";
}
@Property(name="createdBy")
@JsonIgnore
public Optional getCreatedBy() {
return Optional.ofNullable(createdBy);
}
public CopyNotebookModel withCreatedBy(String createdBy) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.createdBy = createdBy;
return _x;
}
@Property(name="createdByIdentity")
@JsonIgnore
public Optional getCreatedByIdentity() {
return Optional.ofNullable(createdByIdentity);
}
public CopyNotebookModel withCreatedByIdentity(IdentitySet createdByIdentity) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.createdByIdentity = createdByIdentity;
return _x;
}
@Property(name="createdTime")
@JsonIgnore
public Optional getCreatedTime() {
return Optional.ofNullable(createdTime);
}
public CopyNotebookModel withCreatedTime(OffsetDateTime createdTime) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.createdTime = createdTime;
return _x;
}
@Property(name="id")
@JsonIgnore
public Optional getId() {
return Optional.ofNullable(id);
}
public CopyNotebookModel withId(String id) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.id = id;
return _x;
}
@Property(name="isDefault")
@JsonIgnore
public Optional getIsDefault() {
return Optional.ofNullable(isDefault);
}
public CopyNotebookModel withIsDefault(Boolean isDefault) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.isDefault = isDefault;
return _x;
}
@Property(name="isShared")
@JsonIgnore
public Optional getIsShared() {
return Optional.ofNullable(isShared);
}
public CopyNotebookModel withIsShared(Boolean isShared) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.isShared = isShared;
return _x;
}
@Property(name="lastModifiedBy")
@JsonIgnore
public Optional getLastModifiedBy() {
return Optional.ofNullable(lastModifiedBy);
}
public CopyNotebookModel withLastModifiedBy(String lastModifiedBy) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.lastModifiedBy = lastModifiedBy;
return _x;
}
@Property(name="lastModifiedByIdentity")
@JsonIgnore
public Optional getLastModifiedByIdentity() {
return Optional.ofNullable(lastModifiedByIdentity);
}
public CopyNotebookModel withLastModifiedByIdentity(IdentitySet lastModifiedByIdentity) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.lastModifiedByIdentity = lastModifiedByIdentity;
return _x;
}
@Property(name="lastModifiedTime")
@JsonIgnore
public Optional getLastModifiedTime() {
return Optional.ofNullable(lastModifiedTime);
}
public CopyNotebookModel withLastModifiedTime(OffsetDateTime lastModifiedTime) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.lastModifiedTime = lastModifiedTime;
return _x;
}
@Property(name="links")
@JsonIgnore
public Optional getLinks() {
return Optional.ofNullable(links);
}
public CopyNotebookModel withLinks(NotebookLinks links) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.links = links;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public CopyNotebookModel withName(String name) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.name = name;
return _x;
}
@Property(name="sectionGroupsUrl")
@JsonIgnore
public Optional getSectionGroupsUrl() {
return Optional.ofNullable(sectionGroupsUrl);
}
public CopyNotebookModel withSectionGroupsUrl(String sectionGroupsUrl) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.sectionGroupsUrl = sectionGroupsUrl;
return _x;
}
@Property(name="sectionsUrl")
@JsonIgnore
public Optional getSectionsUrl() {
return Optional.ofNullable(sectionsUrl);
}
public CopyNotebookModel withSectionsUrl(String sectionsUrl) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.sectionsUrl = sectionsUrl;
return _x;
}
@Property(name="self")
@JsonIgnore
public Optional getSelf() {
return Optional.ofNullable(self);
}
public CopyNotebookModel withSelf(String self) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.self = self;
return _x;
}
@Property(name="userRole")
@JsonIgnore
public Optional getUserRole() {
return Optional.ofNullable(userRole);
}
public CopyNotebookModel withUserRole(OnenoteUserRole userRole) {
CopyNotebookModel _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.CopyNotebookModel");
_x.userRole = userRole;
return _x;
}
public CopyNotebookModel withUnmappedField(String name, String value) {
CopyNotebookModel _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String createdBy;
private IdentitySet createdByIdentity;
private OffsetDateTime createdTime;
private String id;
private Boolean isDefault;
private Boolean isShared;
private String lastModifiedBy;
private IdentitySet lastModifiedByIdentity;
private OffsetDateTime lastModifiedTime;
private NotebookLinks links;
private String name;
private String sectionGroupsUrl;
private String sectionsUrl;
private String self;
private OnenoteUserRole userRole;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder createdBy(String createdBy) {
this.createdBy = createdBy;
this.changedFields = changedFields.add("createdBy");
return this;
}
public Builder createdByIdentity(IdentitySet createdByIdentity) {
this.createdByIdentity = createdByIdentity;
this.changedFields = changedFields.add("createdByIdentity");
return this;
}
public Builder createdTime(OffsetDateTime createdTime) {
this.createdTime = createdTime;
this.changedFields = changedFields.add("createdTime");
return this;
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder isDefault(Boolean isDefault) {
this.isDefault = isDefault;
this.changedFields = changedFields.add("isDefault");
return this;
}
public Builder isShared(Boolean isShared) {
this.isShared = isShared;
this.changedFields = changedFields.add("isShared");
return this;
}
public Builder lastModifiedBy(String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
this.changedFields = changedFields.add("lastModifiedBy");
return this;
}
public Builder lastModifiedByIdentity(IdentitySet lastModifiedByIdentity) {
this.lastModifiedByIdentity = lastModifiedByIdentity;
this.changedFields = changedFields.add("lastModifiedByIdentity");
return this;
}
public Builder lastModifiedTime(OffsetDateTime lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
this.changedFields = changedFields.add("lastModifiedTime");
return this;
}
public Builder links(NotebookLinks links) {
this.links = links;
this.changedFields = changedFields.add("links");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder sectionGroupsUrl(String sectionGroupsUrl) {
this.sectionGroupsUrl = sectionGroupsUrl;
this.changedFields = changedFields.add("sectionGroupsUrl");
return this;
}
public Builder sectionsUrl(String sectionsUrl) {
this.sectionsUrl = sectionsUrl;
this.changedFields = changedFields.add("sectionsUrl");
return this;
}
public Builder self(String self) {
this.self = self;
this.changedFields = changedFields.add("self");
return this;
}
public Builder userRole(OnenoteUserRole userRole) {
this.userRole = userRole;
this.changedFields = changedFields.add("userRole");
return this;
}
public CopyNotebookModel build() {
CopyNotebookModel _x = new CopyNotebookModel();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.CopyNotebookModel";
_x.createdBy = createdBy;
_x.createdByIdentity = createdByIdentity;
_x.createdTime = createdTime;
_x.id = id;
_x.isDefault = isDefault;
_x.isShared = isShared;
_x.lastModifiedBy = lastModifiedBy;
_x.lastModifiedByIdentity = lastModifiedByIdentity;
_x.lastModifiedTime = lastModifiedTime;
_x.links = links;
_x.name = name;
_x.sectionGroupsUrl = sectionGroupsUrl;
_x.sectionsUrl = sectionsUrl;
_x.self = self;
_x.userRole = userRole;
return _x;
}
}
private CopyNotebookModel _copy() {
CopyNotebookModel _x = new CopyNotebookModel();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.createdBy = createdBy;
_x.createdByIdentity = createdByIdentity;
_x.createdTime = createdTime;
_x.id = id;
_x.isDefault = isDefault;
_x.isShared = isShared;
_x.lastModifiedBy = lastModifiedBy;
_x.lastModifiedByIdentity = lastModifiedByIdentity;
_x.lastModifiedTime = lastModifiedTime;
_x.links = links;
_x.name = name;
_x.sectionGroupsUrl = sectionGroupsUrl;
_x.sectionsUrl = sectionsUrl;
_x.self = self;
_x.userRole = userRole;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("CopyNotebookModel[");
b.append("createdBy=");
b.append(this.createdBy);
b.append(", ");
b.append("createdByIdentity=");
b.append(this.createdByIdentity);
b.append(", ");
b.append("createdTime=");
b.append(this.createdTime);
b.append(", ");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("isDefault=");
b.append(this.isDefault);
b.append(", ");
b.append("isShared=");
b.append(this.isShared);
b.append(", ");
b.append("lastModifiedBy=");
b.append(this.lastModifiedBy);
b.append(", ");
b.append("lastModifiedByIdentity=");
b.append(this.lastModifiedByIdentity);
b.append(", ");
b.append("lastModifiedTime=");
b.append(this.lastModifiedTime);
b.append(", ");
b.append("links=");
b.append(this.links);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("sectionGroupsUrl=");
b.append(this.sectionGroupsUrl);
b.append(", ");
b.append("sectionsUrl=");
b.append(this.sectionsUrl);
b.append(", ");
b.append("self=");
b.append(this.self);
b.append(", ");
b.append("userRole=");
b.append(this.userRole);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy