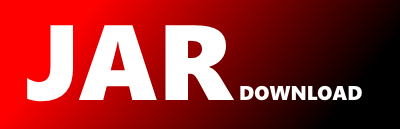
odata.msgraph.client.beta.complex.DeviceExchangeAccessStateSummary Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “Device Exchange Access State summary”
*/@JsonPropertyOrder({
"@odata.type",
"allowedDeviceCount",
"blockedDeviceCount",
"quarantinedDeviceCount",
"unavailableDeviceCount",
"unknownDeviceCount"})
@JsonInclude(Include.NON_NULL)
public class DeviceExchangeAccessStateSummary implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("allowedDeviceCount")
protected Integer allowedDeviceCount;
@JsonProperty("blockedDeviceCount")
protected Integer blockedDeviceCount;
@JsonProperty("quarantinedDeviceCount")
protected Integer quarantinedDeviceCount;
@JsonProperty("unavailableDeviceCount")
protected Integer unavailableDeviceCount;
@JsonProperty("unknownDeviceCount")
protected Integer unknownDeviceCount;
protected DeviceExchangeAccessStateSummary() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.deviceExchangeAccessStateSummary";
}
/**
* “Total count of devices with Exchange Access State: Allowed.”
*
* @return property allowedDeviceCount
*/
@Property(name="allowedDeviceCount")
@JsonIgnore
public Optional getAllowedDeviceCount() {
return Optional.ofNullable(allowedDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedDeviceCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Total count of devices with Exchange Access State: Allowed.”
*
* @param allowedDeviceCount
* new value of {@code allowedDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedDeviceCount} field changed
*/
public DeviceExchangeAccessStateSummary withAllowedDeviceCount(Integer allowedDeviceCount) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceExchangeAccessStateSummary");
_x.allowedDeviceCount = allowedDeviceCount;
return _x;
}
/**
* “Total count of devices with Exchange Access State: Blocked.”
*
* @return property blockedDeviceCount
*/
@Property(name="blockedDeviceCount")
@JsonIgnore
public Optional getBlockedDeviceCount() {
return Optional.ofNullable(blockedDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockedDeviceCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Total count of devices with Exchange Access State: Blocked.”
*
* @param blockedDeviceCount
* new value of {@code blockedDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockedDeviceCount} field changed
*/
public DeviceExchangeAccessStateSummary withBlockedDeviceCount(Integer blockedDeviceCount) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceExchangeAccessStateSummary");
_x.blockedDeviceCount = blockedDeviceCount;
return _x;
}
/**
* “Total count of devices with Exchange Access State: Quarantined.”
*
* @return property quarantinedDeviceCount
*/
@Property(name="quarantinedDeviceCount")
@JsonIgnore
public Optional getQuarantinedDeviceCount() {
return Optional.ofNullable(quarantinedDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* quarantinedDeviceCount} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Total count of devices with Exchange Access State: Quarantined.”
*
* @param quarantinedDeviceCount
* new value of {@code quarantinedDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code quarantinedDeviceCount} field changed
*/
public DeviceExchangeAccessStateSummary withQuarantinedDeviceCount(Integer quarantinedDeviceCount) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceExchangeAccessStateSummary");
_x.quarantinedDeviceCount = quarantinedDeviceCount;
return _x;
}
/**
* “Total count of devices for which no Exchange Access State could be found.”
*
* @return property unavailableDeviceCount
*/
@Property(name="unavailableDeviceCount")
@JsonIgnore
public Optional getUnavailableDeviceCount() {
return Optional.ofNullable(unavailableDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* unavailableDeviceCount} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Total count of devices for which no Exchange Access State could be found.”
*
* @param unavailableDeviceCount
* new value of {@code unavailableDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code unavailableDeviceCount} field changed
*/
public DeviceExchangeAccessStateSummary withUnavailableDeviceCount(Integer unavailableDeviceCount) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceExchangeAccessStateSummary");
_x.unavailableDeviceCount = unavailableDeviceCount;
return _x;
}
/**
* “Total count of devices with Exchange Access State: Unknown.”
*
* @return property unknownDeviceCount
*/
@Property(name="unknownDeviceCount")
@JsonIgnore
public Optional getUnknownDeviceCount() {
return Optional.ofNullable(unknownDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* unknownDeviceCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Total count of devices with Exchange Access State: Unknown.”
*
* @param unknownDeviceCount
* new value of {@code unknownDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code unknownDeviceCount} field changed
*/
public DeviceExchangeAccessStateSummary withUnknownDeviceCount(Integer unknownDeviceCount) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceExchangeAccessStateSummary");
_x.unknownDeviceCount = unknownDeviceCount;
return _x;
}
public DeviceExchangeAccessStateSummary withUnmappedField(String name, String value) {
DeviceExchangeAccessStateSummary _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer allowedDeviceCount;
private Integer blockedDeviceCount;
private Integer quarantinedDeviceCount;
private Integer unavailableDeviceCount;
private Integer unknownDeviceCount;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Total count of devices with Exchange Access State: Allowed.”
*
* @param allowedDeviceCount
* value of {@code allowedDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder allowedDeviceCount(Integer allowedDeviceCount) {
this.allowedDeviceCount = allowedDeviceCount;
this.changedFields = changedFields.add("allowedDeviceCount");
return this;
}
/**
* “Total count of devices with Exchange Access State: Blocked.”
*
* @param blockedDeviceCount
* value of {@code blockedDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockedDeviceCount(Integer blockedDeviceCount) {
this.blockedDeviceCount = blockedDeviceCount;
this.changedFields = changedFields.add("blockedDeviceCount");
return this;
}
/**
* “Total count of devices with Exchange Access State: Quarantined.”
*
* @param quarantinedDeviceCount
* value of {@code quarantinedDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder quarantinedDeviceCount(Integer quarantinedDeviceCount) {
this.quarantinedDeviceCount = quarantinedDeviceCount;
this.changedFields = changedFields.add("quarantinedDeviceCount");
return this;
}
/**
* “Total count of devices for which no Exchange Access State could be found.”
*
* @param unavailableDeviceCount
* value of {@code unavailableDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder unavailableDeviceCount(Integer unavailableDeviceCount) {
this.unavailableDeviceCount = unavailableDeviceCount;
this.changedFields = changedFields.add("unavailableDeviceCount");
return this;
}
/**
* “Total count of devices with Exchange Access State: Unknown.”
*
* @param unknownDeviceCount
* value of {@code unknownDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder unknownDeviceCount(Integer unknownDeviceCount) {
this.unknownDeviceCount = unknownDeviceCount;
this.changedFields = changedFields.add("unknownDeviceCount");
return this;
}
public DeviceExchangeAccessStateSummary build() {
DeviceExchangeAccessStateSummary _x = new DeviceExchangeAccessStateSummary();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.deviceExchangeAccessStateSummary";
_x.allowedDeviceCount = allowedDeviceCount;
_x.blockedDeviceCount = blockedDeviceCount;
_x.quarantinedDeviceCount = quarantinedDeviceCount;
_x.unavailableDeviceCount = unavailableDeviceCount;
_x.unknownDeviceCount = unknownDeviceCount;
return _x;
}
}
private DeviceExchangeAccessStateSummary _copy() {
DeviceExchangeAccessStateSummary _x = new DeviceExchangeAccessStateSummary();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.allowedDeviceCount = allowedDeviceCount;
_x.blockedDeviceCount = blockedDeviceCount;
_x.quarantinedDeviceCount = quarantinedDeviceCount;
_x.unavailableDeviceCount = unavailableDeviceCount;
_x.unknownDeviceCount = unknownDeviceCount;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DeviceExchangeAccessStateSummary[");
b.append("allowedDeviceCount=");
b.append(this.allowedDeviceCount);
b.append(", ");
b.append("blockedDeviceCount=");
b.append(this.blockedDeviceCount);
b.append(", ");
b.append("quarantinedDeviceCount=");
b.append(this.quarantinedDeviceCount);
b.append(", ");
b.append("unavailableDeviceCount=");
b.append(this.unavailableDeviceCount);
b.append(", ");
b.append("unknownDeviceCount=");
b.append(this.unknownDeviceCount);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}