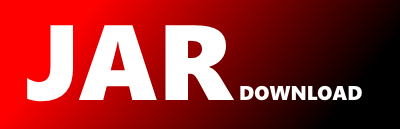
odata.msgraph.client.beta.complex.MacOSKerberosSingleSignOnExtension Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
/**
* “Represents a Kerberos-type Single Sign-On extension profile for macOS devices.”
*/@JsonPropertyOrder({
"@odata.type",
"activeDirectorySiteCode",
"blockActiveDirectorySiteAutoDiscovery",
"blockAutomaticLogin",
"cacheName",
"credentialBundleIdAccessControlList",
"domainRealms",
"domains",
"isDefaultRealm",
"passwordBlockModification",
"passwordChangeUrl",
"passwordEnableLocalSync",
"passwordExpirationDays",
"passwordExpirationNotificationDays",
"passwordMinimumAgeDays",
"passwordMinimumLength",
"passwordPreviousPasswordBlockCount",
"passwordRequireActiveDirectoryComplexity",
"passwordRequirementsDescription",
"realm",
"requireUserPresence",
"userPrincipalName"})
@JsonInclude(Include.NON_NULL)
public class MacOSKerberosSingleSignOnExtension extends MacOSSingleSignOnExtension implements ODataType {
@JsonProperty("activeDirectorySiteCode")
protected String activeDirectorySiteCode;
@JsonProperty("blockActiveDirectorySiteAutoDiscovery")
protected Boolean blockActiveDirectorySiteAutoDiscovery;
@JsonProperty("blockAutomaticLogin")
protected Boolean blockAutomaticLogin;
@JsonProperty("cacheName")
protected String cacheName;
@JsonProperty("credentialBundleIdAccessControlList")
protected List credentialBundleIdAccessControlList;
@JsonProperty("credentialBundleIdAccessControlList@nextLink")
protected String credentialBundleIdAccessControlListNextLink;
@JsonProperty("domainRealms")
protected List domainRealms;
@JsonProperty("domainRealms@nextLink")
protected String domainRealmsNextLink;
@JsonProperty("domains")
protected List domains;
@JsonProperty("domains@nextLink")
protected String domainsNextLink;
@JsonProperty("isDefaultRealm")
protected Boolean isDefaultRealm;
@JsonProperty("passwordBlockModification")
protected Boolean passwordBlockModification;
@JsonProperty("passwordChangeUrl")
protected String passwordChangeUrl;
@JsonProperty("passwordEnableLocalSync")
protected Boolean passwordEnableLocalSync;
@JsonProperty("passwordExpirationDays")
protected Integer passwordExpirationDays;
@JsonProperty("passwordExpirationNotificationDays")
protected Integer passwordExpirationNotificationDays;
@JsonProperty("passwordMinimumAgeDays")
protected Integer passwordMinimumAgeDays;
@JsonProperty("passwordMinimumLength")
protected Integer passwordMinimumLength;
@JsonProperty("passwordPreviousPasswordBlockCount")
protected Integer passwordPreviousPasswordBlockCount;
@JsonProperty("passwordRequireActiveDirectoryComplexity")
protected Boolean passwordRequireActiveDirectoryComplexity;
@JsonProperty("passwordRequirementsDescription")
protected String passwordRequirementsDescription;
@JsonProperty("realm")
protected String realm;
@JsonProperty("requireUserPresence")
protected Boolean requireUserPresence;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
protected MacOSKerberosSingleSignOnExtension() {
super();
}
@Override
public String odataTypeName() {
return "microsoft.graph.macOSKerberosSingleSignOnExtension";
}
/**
* “Gets or sets the Active Directory site.”
*
* @return property activeDirectorySiteCode
*/
@Property(name="activeDirectorySiteCode")
@JsonIgnore
public Optional getActiveDirectorySiteCode() {
return Optional.ofNullable(activeDirectorySiteCode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* activeDirectorySiteCode} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Gets or sets the Active Directory site.”
*
* @param activeDirectorySiteCode
* new value of {@code activeDirectorySiteCode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code activeDirectorySiteCode} field changed
*/
public MacOSKerberosSingleSignOnExtension withActiveDirectorySiteCode(String activeDirectorySiteCode) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.activeDirectorySiteCode = activeDirectorySiteCode;
return _x;
}
/**
* “Enables or disables whether the Kerberos extension can automatically determine
* its site name.”
*
* @return property blockActiveDirectorySiteAutoDiscovery
*/
@Property(name="blockActiveDirectorySiteAutoDiscovery")
@JsonIgnore
public Optional getBlockActiveDirectorySiteAutoDiscovery() {
return Optional.ofNullable(blockActiveDirectorySiteAutoDiscovery);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockActiveDirectorySiteAutoDiscovery} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Enables or disables whether the Kerberos extension can automatically determine
* its site name.”
*
* @param blockActiveDirectorySiteAutoDiscovery
* new value of {@code blockActiveDirectorySiteAutoDiscovery} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockActiveDirectorySiteAutoDiscovery} field changed
*/
public MacOSKerberosSingleSignOnExtension withBlockActiveDirectorySiteAutoDiscovery(Boolean blockActiveDirectorySiteAutoDiscovery) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.blockActiveDirectorySiteAutoDiscovery = blockActiveDirectorySiteAutoDiscovery;
return _x;
}
/**
* “Enables or disables Keychain usage.”
*
* @return property blockAutomaticLogin
*/
@Property(name="blockAutomaticLogin")
@JsonIgnore
public Optional getBlockAutomaticLogin() {
return Optional.ofNullable(blockAutomaticLogin);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockAutomaticLogin} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Enables or disables Keychain usage.”
*
* @param blockAutomaticLogin
* new value of {@code blockAutomaticLogin} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockAutomaticLogin} field changed
*/
public MacOSKerberosSingleSignOnExtension withBlockAutomaticLogin(Boolean blockAutomaticLogin) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.blockAutomaticLogin = blockAutomaticLogin;
return _x;
}
/**
* “Gets or sets the Generic Security Services name of the Kerberos cache to use for
* this profile.”
*
* @return property cacheName
*/
@Property(name="cacheName")
@JsonIgnore
public Optional getCacheName() {
return Optional.ofNullable(cacheName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code cacheName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Gets or sets the Generic Security Services name of the Kerberos cache to use for
* this profile.”
*
* @param cacheName
* new value of {@code cacheName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cacheName} field changed
*/
public MacOSKerberosSingleSignOnExtension withCacheName(String cacheName) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.cacheName = cacheName;
return _x;
}
/**
* “Gets or sets a list of app Bundle IDs allowed to access the Kerberos Ticket
* Granting Ticket.”
*
* @return property credentialBundleIdAccessControlList
*/
@Property(name="credentialBundleIdAccessControlList")
@JsonIgnore
public CollectionPage getCredentialBundleIdAccessControlList() {
return new CollectionPage(contextPath, String.class, this.credentialBundleIdAccessControlList, Optional.ofNullable(credentialBundleIdAccessControlListNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* “Gets or sets a list of app Bundle IDs allowed to access the Kerberos Ticket
* Granting Ticket.”
*
* @param options
* specify connect and read timeouts
* @return property credentialBundleIdAccessControlList
*/
@Property(name="credentialBundleIdAccessControlList")
@JsonIgnore
public CollectionPage getCredentialBundleIdAccessControlList(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.credentialBundleIdAccessControlList, Optional.ofNullable(credentialBundleIdAccessControlListNextLink), Collections.emptyList(), options);
}
/**
* “Gets or sets a list of realms for custom domain-realm mapping. Realms are case
* sensitive.”
*
* @return property domainRealms
*/
@Property(name="domainRealms")
@JsonIgnore
public CollectionPage getDomainRealms() {
return new CollectionPage(contextPath, String.class, this.domainRealms, Optional.ofNullable(domainRealmsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* “Gets or sets a list of realms for custom domain-realm mapping. Realms are case
* sensitive.”
*
* @param options
* specify connect and read timeouts
* @return property domainRealms
*/
@Property(name="domainRealms")
@JsonIgnore
public CollectionPage getDomainRealms(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.domainRealms, Optional.ofNullable(domainRealmsNextLink), Collections.emptyList(), options);
}
/**
* “Gets or sets a list of hosts or domain names for which the app extension
* performs SSO.”
*
* @return property domains
*/
@Property(name="domains")
@JsonIgnore
public CollectionPage getDomains() {
return new CollectionPage(contextPath, String.class, this.domains, Optional.ofNullable(domainsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* “Gets or sets a list of hosts or domain names for which the app extension
* performs SSO.”
*
* @param options
* specify connect and read timeouts
* @return property domains
*/
@Property(name="domains")
@JsonIgnore
public CollectionPage getDomains(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.domains, Optional.ofNullable(domainsNextLink), Collections.emptyList(), options);
}
/**
* “When true, this profile's realm will be selected as the default. Necessary if
* multiple Kerberos-type profiles are configured.”
*
* @return property isDefaultRealm
*/
@Property(name="isDefaultRealm")
@JsonIgnore
public Optional getIsDefaultRealm() {
return Optional.ofNullable(isDefaultRealm);
}
/**
* Returns an immutable copy of {@code this} with just the {@code isDefaultRealm}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “When true, this profile's realm will be selected as the default. Necessary if
* multiple Kerberos-type profiles are configured.”
*
* @param isDefaultRealm
* new value of {@code isDefaultRealm} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isDefaultRealm} field changed
*/
public MacOSKerberosSingleSignOnExtension withIsDefaultRealm(Boolean isDefaultRealm) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.isDefaultRealm = isDefaultRealm;
return _x;
}
/**
* “Enables or disables password changes.”
*
* @return property passwordBlockModification
*/
@Property(name="passwordBlockModification")
@JsonIgnore
public Optional getPasswordBlockModification() {
return Optional.ofNullable(passwordBlockModification);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockModification} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enables or disables password changes.”
*
* @param passwordBlockModification
* new value of {@code passwordBlockModification} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockModification} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordBlockModification(Boolean passwordBlockModification) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordBlockModification = passwordBlockModification;
return _x;
}
/**
* “Gets or sets the URL that the user will be sent to when they initiate a password
* change.”
*
* @return property passwordChangeUrl
*/
@Property(name="passwordChangeUrl")
@JsonIgnore
public Optional getPasswordChangeUrl() {
return Optional.ofNullable(passwordChangeUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code passwordChangeUrl
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Gets or sets the URL that the user will be sent to when they initiate a password
* change.”
*
* @param passwordChangeUrl
* new value of {@code passwordChangeUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordChangeUrl} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordChangeUrl(String passwordChangeUrl) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordChangeUrl = passwordChangeUrl;
return _x;
}
/**
* “Enables or disables password syncing. This won't affect users logged in with a
* mobile account on macOS.”
*
* @return property passwordEnableLocalSync
*/
@Property(name="passwordEnableLocalSync")
@JsonIgnore
public Optional getPasswordEnableLocalSync() {
return Optional.ofNullable(passwordEnableLocalSync);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordEnableLocalSync} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enables or disables password syncing. This won't affect users logged in with a
* mobile account on macOS.”
*
* @param passwordEnableLocalSync
* new value of {@code passwordEnableLocalSync} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordEnableLocalSync} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordEnableLocalSync(Boolean passwordEnableLocalSync) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordEnableLocalSync = passwordEnableLocalSync;
return _x;
}
/**
* “Overrides the default password expiration in days. For most domains, this value
* is calculated automatically.”
*
* @return property passwordExpirationDays
*/
@Property(name="passwordExpirationDays")
@JsonIgnore
public Optional getPasswordExpirationDays() {
return Optional.ofNullable(passwordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Overrides the default password expiration in days. For most domains, this value
* is calculated automatically.”
*
* @param passwordExpirationDays
* new value of {@code passwordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationDays} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordExpirationDays(Integer passwordExpirationDays) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordExpirationDays = passwordExpirationDays;
return _x;
}
/**
* “Gets or sets the number of days until the user is notified that their password
* will expire (default is 15).”
*
* @return property passwordExpirationNotificationDays
*/
@Property(name="passwordExpirationNotificationDays")
@JsonIgnore
public Optional getPasswordExpirationNotificationDays() {
return Optional.ofNullable(passwordExpirationNotificationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationNotificationDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Gets or sets the number of days until the user is notified that their password
* will expire (default is 15).”
*
* @param passwordExpirationNotificationDays
* new value of {@code passwordExpirationNotificationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationNotificationDays} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordExpirationNotificationDays(Integer passwordExpirationNotificationDays) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordExpirationNotificationDays = passwordExpirationNotificationDays;
return _x;
}
/**
* “Gets or sets the minimum number of days until a user can change their password
* again.”
*
* @return property passwordMinimumAgeDays
*/
@Property(name="passwordMinimumAgeDays")
@JsonIgnore
public Optional getPasswordMinimumAgeDays() {
return Optional.ofNullable(passwordMinimumAgeDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumAgeDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Gets or sets the minimum number of days until a user can change their password
* again.”
*
* @param passwordMinimumAgeDays
* new value of {@code passwordMinimumAgeDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumAgeDays} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordMinimumAgeDays(Integer passwordMinimumAgeDays) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordMinimumAgeDays = passwordMinimumAgeDays;
return _x;
}
/**
* “Gets or sets the minimum length of a password.”
*
* @return property passwordMinimumLength
*/
@Property(name="passwordMinimumLength")
@JsonIgnore
public Optional getPasswordMinimumLength() {
return Optional.ofNullable(passwordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumLength} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Gets or sets the minimum length of a password.”
*
* @param passwordMinimumLength
* new value of {@code passwordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumLength} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordMinimumLength(Integer passwordMinimumLength) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordMinimumLength = passwordMinimumLength;
return _x;
}
/**
* “Gets or sets the number of previous passwords to block.”
*
* @return property passwordPreviousPasswordBlockCount
*/
@Property(name="passwordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getPasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(passwordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordPreviousPasswordBlockCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Gets or sets the number of previous passwords to block.”
*
* @param passwordPreviousPasswordBlockCount
* new value of {@code passwordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordPreviousPasswordBlockCount} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
return _x;
}
/**
* “Enables or disables whether passwords must meet Active Directory's complexity
* requirements.”
*
* @return property passwordRequireActiveDirectoryComplexity
*/
@Property(name="passwordRequireActiveDirectoryComplexity")
@JsonIgnore
public Optional getPasswordRequireActiveDirectoryComplexity() {
return Optional.ofNullable(passwordRequireActiveDirectoryComplexity);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequireActiveDirectoryComplexity} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Enables or disables whether passwords must meet Active Directory's complexity
* requirements.”
*
* @param passwordRequireActiveDirectoryComplexity
* new value of {@code passwordRequireActiveDirectoryComplexity} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequireActiveDirectoryComplexity} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordRequireActiveDirectoryComplexity(Boolean passwordRequireActiveDirectoryComplexity) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordRequireActiveDirectoryComplexity = passwordRequireActiveDirectoryComplexity;
return _x;
}
/**
* “Gets or sets a description of the password complexity requirements.”
*
* @return property passwordRequirementsDescription
*/
@Property(name="passwordRequirementsDescription")
@JsonIgnore
public Optional getPasswordRequirementsDescription() {
return Optional.ofNullable(passwordRequirementsDescription);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequirementsDescription} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Gets or sets a description of the password complexity requirements.”
*
* @param passwordRequirementsDescription
* new value of {@code passwordRequirementsDescription} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequirementsDescription} field changed
*/
public MacOSKerberosSingleSignOnExtension withPasswordRequirementsDescription(String passwordRequirementsDescription) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.passwordRequirementsDescription = passwordRequirementsDescription;
return _x;
}
/**
* “Gets or sets the case-sensitive realm name for this profile.”
*
* @return property realm
*/
@Property(name="realm")
@JsonIgnore
public Optional getRealm() {
return Optional.ofNullable(realm);
}
/**
* Returns an immutable copy of {@code this} with just the {@code realm} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Gets or sets the case-sensitive realm name for this profile.”
*
* @param realm
* new value of {@code realm} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code realm} field changed
*/
public MacOSKerberosSingleSignOnExtension withRealm(String realm) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.realm = realm;
return _x;
}
/**
* “Gets or sets whether to require authentication via Touch ID, Face ID, or a
* passcode to access the keychain entry.”
*
* @return property requireUserPresence
*/
@Property(name="requireUserPresence")
@JsonIgnore
public Optional getRequireUserPresence() {
return Optional.ofNullable(requireUserPresence);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* requireUserPresence} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Gets or sets whether to require authentication via Touch ID, Face ID, or a
* passcode to access the keychain entry.”
*
* @param requireUserPresence
* new value of {@code requireUserPresence} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code requireUserPresence} field changed
*/
public MacOSKerberosSingleSignOnExtension withRequireUserPresence(Boolean requireUserPresence) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.requireUserPresence = requireUserPresence;
return _x;
}
/**
* “Gets or sets the principle user name to use for this profile. The realm name
* does not need to be included.”
*
* @return property userPrincipalName
*/
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userPrincipalName
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Gets or sets the principle user name to use for this profile. The realm name
* does not need to be included.”
*
* @param userPrincipalName
* new value of {@code userPrincipalName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userPrincipalName} field changed
*/
public MacOSKerberosSingleSignOnExtension withUserPrincipalName(String userPrincipalName) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSKerberosSingleSignOnExtension");
_x.userPrincipalName = userPrincipalName;
return _x;
}
public MacOSKerberosSingleSignOnExtension withUnmappedField(String name, String value) {
MacOSKerberosSingleSignOnExtension _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMacOSKerberosSingleSignOnExtension() {
return new Builder();
}
public static final class Builder {
private String activeDirectorySiteCode;
private Boolean blockActiveDirectorySiteAutoDiscovery;
private Boolean blockAutomaticLogin;
private String cacheName;
private List credentialBundleIdAccessControlList;
private String credentialBundleIdAccessControlListNextLink;
private List domainRealms;
private String domainRealmsNextLink;
private List domains;
private String domainsNextLink;
private Boolean isDefaultRealm;
private Boolean passwordBlockModification;
private String passwordChangeUrl;
private Boolean passwordEnableLocalSync;
private Integer passwordExpirationDays;
private Integer passwordExpirationNotificationDays;
private Integer passwordMinimumAgeDays;
private Integer passwordMinimumLength;
private Integer passwordPreviousPasswordBlockCount;
private Boolean passwordRequireActiveDirectoryComplexity;
private String passwordRequirementsDescription;
private String realm;
private Boolean requireUserPresence;
private String userPrincipalName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Gets or sets the Active Directory site.”
*
* @param activeDirectorySiteCode
* value of {@code activeDirectorySiteCode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder activeDirectorySiteCode(String activeDirectorySiteCode) {
this.activeDirectorySiteCode = activeDirectorySiteCode;
this.changedFields = changedFields.add("activeDirectorySiteCode");
return this;
}
/**
* “Enables or disables whether the Kerberos extension can automatically determine
* its site name.”
*
* @param blockActiveDirectorySiteAutoDiscovery
* value of {@code blockActiveDirectorySiteAutoDiscovery} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockActiveDirectorySiteAutoDiscovery(Boolean blockActiveDirectorySiteAutoDiscovery) {
this.blockActiveDirectorySiteAutoDiscovery = blockActiveDirectorySiteAutoDiscovery;
this.changedFields = changedFields.add("blockActiveDirectorySiteAutoDiscovery");
return this;
}
/**
* “Enables or disables Keychain usage.”
*
* @param blockAutomaticLogin
* value of {@code blockAutomaticLogin} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockAutomaticLogin(Boolean blockAutomaticLogin) {
this.blockAutomaticLogin = blockAutomaticLogin;
this.changedFields = changedFields.add("blockAutomaticLogin");
return this;
}
/**
* “Gets or sets the Generic Security Services name of the Kerberos cache to use for
* this profile.”
*
* @param cacheName
* value of {@code cacheName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cacheName(String cacheName) {
this.cacheName = cacheName;
this.changedFields = changedFields.add("cacheName");
return this;
}
/**
* “Gets or sets a list of app Bundle IDs allowed to access the Kerberos Ticket
* Granting Ticket.”
*
* @param credentialBundleIdAccessControlList
* value of {@code credentialBundleIdAccessControlList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder credentialBundleIdAccessControlList(List credentialBundleIdAccessControlList) {
this.credentialBundleIdAccessControlList = credentialBundleIdAccessControlList;
this.changedFields = changedFields.add("credentialBundleIdAccessControlList");
return this;
}
/**
* “Gets or sets a list of app Bundle IDs allowed to access the Kerberos Ticket
* Granting Ticket.”
*
* @param credentialBundleIdAccessControlList
* value of {@code credentialBundleIdAccessControlList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder credentialBundleIdAccessControlList(String... credentialBundleIdAccessControlList) {
return credentialBundleIdAccessControlList(Arrays.asList(credentialBundleIdAccessControlList));
}
/**
* “Gets or sets a list of app Bundle IDs allowed to access the Kerberos Ticket
* Granting Ticket.”
*
* @param credentialBundleIdAccessControlListNextLink
* value of {@code credentialBundleIdAccessControlList@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder credentialBundleIdAccessControlListNextLink(String credentialBundleIdAccessControlListNextLink) {
this.credentialBundleIdAccessControlListNextLink = credentialBundleIdAccessControlListNextLink;
this.changedFields = changedFields.add("credentialBundleIdAccessControlList");
return this;
}
/**
* “Gets or sets a list of realms for custom domain-realm mapping. Realms are case
* sensitive.”
*
* @param domainRealms
* value of {@code domainRealms} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domainRealms(List domainRealms) {
this.domainRealms = domainRealms;
this.changedFields = changedFields.add("domainRealms");
return this;
}
/**
* “Gets or sets a list of realms for custom domain-realm mapping. Realms are case
* sensitive.”
*
* @param domainRealms
* value of {@code domainRealms} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domainRealms(String... domainRealms) {
return domainRealms(Arrays.asList(domainRealms));
}
/**
* “Gets or sets a list of realms for custom domain-realm mapping. Realms are case
* sensitive.”
*
* @param domainRealmsNextLink
* value of {@code domainRealms@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domainRealmsNextLink(String domainRealmsNextLink) {
this.domainRealmsNextLink = domainRealmsNextLink;
this.changedFields = changedFields.add("domainRealms");
return this;
}
/**
* “Gets or sets a list of hosts or domain names for which the app extension
* performs SSO.”
*
* @param domains
* value of {@code domains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domains(List domains) {
this.domains = domains;
this.changedFields = changedFields.add("domains");
return this;
}
/**
* “Gets or sets a list of hosts or domain names for which the app extension
* performs SSO.”
*
* @param domains
* value of {@code domains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domains(String... domains) {
return domains(Arrays.asList(domains));
}
/**
* “Gets or sets a list of hosts or domain names for which the app extension
* performs SSO.”
*
* @param domainsNextLink
* value of {@code domains@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder domainsNextLink(String domainsNextLink) {
this.domainsNextLink = domainsNextLink;
this.changedFields = changedFields.add("domains");
return this;
}
/**
* “When true, this profile's realm will be selected as the default. Necessary if
* multiple Kerberos-type profiles are configured.”
*
* @param isDefaultRealm
* value of {@code isDefaultRealm} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder isDefaultRealm(Boolean isDefaultRealm) {
this.isDefaultRealm = isDefaultRealm;
this.changedFields = changedFields.add("isDefaultRealm");
return this;
}
/**
* “Enables or disables password changes.”
*
* @param passwordBlockModification
* value of {@code passwordBlockModification} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockModification(Boolean passwordBlockModification) {
this.passwordBlockModification = passwordBlockModification;
this.changedFields = changedFields.add("passwordBlockModification");
return this;
}
/**
* “Gets or sets the URL that the user will be sent to when they initiate a password
* change.”
*
* @param passwordChangeUrl
* value of {@code passwordChangeUrl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordChangeUrl(String passwordChangeUrl) {
this.passwordChangeUrl = passwordChangeUrl;
this.changedFields = changedFields.add("passwordChangeUrl");
return this;
}
/**
* “Enables or disables password syncing. This won't affect users logged in with a
* mobile account on macOS.”
*
* @param passwordEnableLocalSync
* value of {@code passwordEnableLocalSync} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordEnableLocalSync(Boolean passwordEnableLocalSync) {
this.passwordEnableLocalSync = passwordEnableLocalSync;
this.changedFields = changedFields.add("passwordEnableLocalSync");
return this;
}
/**
* “Overrides the default password expiration in days. For most domains, this value
* is calculated automatically.”
*
* @param passwordExpirationDays
* value of {@code passwordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
this.changedFields = changedFields.add("passwordExpirationDays");
return this;
}
/**
* “Gets or sets the number of days until the user is notified that their password
* will expire (default is 15).”
*
* @param passwordExpirationNotificationDays
* value of {@code passwordExpirationNotificationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationNotificationDays(Integer passwordExpirationNotificationDays) {
this.passwordExpirationNotificationDays = passwordExpirationNotificationDays;
this.changedFields = changedFields.add("passwordExpirationNotificationDays");
return this;
}
/**
* “Gets or sets the minimum number of days until a user can change their password
* again.”
*
* @param passwordMinimumAgeDays
* value of {@code passwordMinimumAgeDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumAgeDays(Integer passwordMinimumAgeDays) {
this.passwordMinimumAgeDays = passwordMinimumAgeDays;
this.changedFields = changedFields.add("passwordMinimumAgeDays");
return this;
}
/**
* “Gets or sets the minimum length of a password.”
*
* @param passwordMinimumLength
* value of {@code passwordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumLength(Integer passwordMinimumLength) {
this.passwordMinimumLength = passwordMinimumLength;
this.changedFields = changedFields.add("passwordMinimumLength");
return this;
}
/**
* “Gets or sets the number of previous passwords to block.”
*
* @param passwordPreviousPasswordBlockCount
* value of {@code passwordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
this.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
return this;
}
/**
* “Enables or disables whether passwords must meet Active Directory's complexity
* requirements.”
*
* @param passwordRequireActiveDirectoryComplexity
* value of {@code passwordRequireActiveDirectoryComplexity} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequireActiveDirectoryComplexity(Boolean passwordRequireActiveDirectoryComplexity) {
this.passwordRequireActiveDirectoryComplexity = passwordRequireActiveDirectoryComplexity;
this.changedFields = changedFields.add("passwordRequireActiveDirectoryComplexity");
return this;
}
/**
* “Gets or sets a description of the password complexity requirements.”
*
* @param passwordRequirementsDescription
* value of {@code passwordRequirementsDescription} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequirementsDescription(String passwordRequirementsDescription) {
this.passwordRequirementsDescription = passwordRequirementsDescription;
this.changedFields = changedFields.add("passwordRequirementsDescription");
return this;
}
/**
* “Gets or sets the case-sensitive realm name for this profile.”
*
* @param realm
* value of {@code realm} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder realm(String realm) {
this.realm = realm;
this.changedFields = changedFields.add("realm");
return this;
}
/**
* “Gets or sets whether to require authentication via Touch ID, Face ID, or a
* passcode to access the keychain entry.”
*
* @param requireUserPresence
* value of {@code requireUserPresence} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder requireUserPresence(Boolean requireUserPresence) {
this.requireUserPresence = requireUserPresence;
this.changedFields = changedFields.add("requireUserPresence");
return this;
}
/**
* “Gets or sets the principle user name to use for this profile. The realm name
* does not need to be included.”
*
* @param userPrincipalName
* value of {@code userPrincipalName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public MacOSKerberosSingleSignOnExtension build() {
MacOSKerberosSingleSignOnExtension _x = new MacOSKerberosSingleSignOnExtension();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSKerberosSingleSignOnExtension";
_x.activeDirectorySiteCode = activeDirectorySiteCode;
_x.blockActiveDirectorySiteAutoDiscovery = blockActiveDirectorySiteAutoDiscovery;
_x.blockAutomaticLogin = blockAutomaticLogin;
_x.cacheName = cacheName;
_x.credentialBundleIdAccessControlList = credentialBundleIdAccessControlList;
_x.credentialBundleIdAccessControlListNextLink = credentialBundleIdAccessControlListNextLink;
_x.domainRealms = domainRealms;
_x.domainRealmsNextLink = domainRealmsNextLink;
_x.domains = domains;
_x.domainsNextLink = domainsNextLink;
_x.isDefaultRealm = isDefaultRealm;
_x.passwordBlockModification = passwordBlockModification;
_x.passwordChangeUrl = passwordChangeUrl;
_x.passwordEnableLocalSync = passwordEnableLocalSync;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordExpirationNotificationDays = passwordExpirationNotificationDays;
_x.passwordMinimumAgeDays = passwordMinimumAgeDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequireActiveDirectoryComplexity = passwordRequireActiveDirectoryComplexity;
_x.passwordRequirementsDescription = passwordRequirementsDescription;
_x.realm = realm;
_x.requireUserPresence = requireUserPresence;
_x.userPrincipalName = userPrincipalName;
return _x;
}
}
private MacOSKerberosSingleSignOnExtension _copy() {
MacOSKerberosSingleSignOnExtension _x = new MacOSKerberosSingleSignOnExtension();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.activeDirectorySiteCode = activeDirectorySiteCode;
_x.blockActiveDirectorySiteAutoDiscovery = blockActiveDirectorySiteAutoDiscovery;
_x.blockAutomaticLogin = blockAutomaticLogin;
_x.cacheName = cacheName;
_x.credentialBundleIdAccessControlList = credentialBundleIdAccessControlList;
_x.domainRealms = domainRealms;
_x.domains = domains;
_x.isDefaultRealm = isDefaultRealm;
_x.passwordBlockModification = passwordBlockModification;
_x.passwordChangeUrl = passwordChangeUrl;
_x.passwordEnableLocalSync = passwordEnableLocalSync;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordExpirationNotificationDays = passwordExpirationNotificationDays;
_x.passwordMinimumAgeDays = passwordMinimumAgeDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequireActiveDirectoryComplexity = passwordRequireActiveDirectoryComplexity;
_x.passwordRequirementsDescription = passwordRequirementsDescription;
_x.realm = realm;
_x.requireUserPresence = requireUserPresence;
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSKerberosSingleSignOnExtension[");
b.append("activeDirectorySiteCode=");
b.append(this.activeDirectorySiteCode);
b.append(", ");
b.append("blockActiveDirectorySiteAutoDiscovery=");
b.append(this.blockActiveDirectorySiteAutoDiscovery);
b.append(", ");
b.append("blockAutomaticLogin=");
b.append(this.blockAutomaticLogin);
b.append(", ");
b.append("cacheName=");
b.append(this.cacheName);
b.append(", ");
b.append("credentialBundleIdAccessControlList=");
b.append(this.credentialBundleIdAccessControlList);
b.append(", ");
b.append("domainRealms=");
b.append(this.domainRealms);
b.append(", ");
b.append("domains=");
b.append(this.domains);
b.append(", ");
b.append("isDefaultRealm=");
b.append(this.isDefaultRealm);
b.append(", ");
b.append("passwordBlockModification=");
b.append(this.passwordBlockModification);
b.append(", ");
b.append("passwordChangeUrl=");
b.append(this.passwordChangeUrl);
b.append(", ");
b.append("passwordEnableLocalSync=");
b.append(this.passwordEnableLocalSync);
b.append(", ");
b.append("passwordExpirationDays=");
b.append(this.passwordExpirationDays);
b.append(", ");
b.append("passwordExpirationNotificationDays=");
b.append(this.passwordExpirationNotificationDays);
b.append(", ");
b.append("passwordMinimumAgeDays=");
b.append(this.passwordMinimumAgeDays);
b.append(", ");
b.append("passwordMinimumLength=");
b.append(this.passwordMinimumLength);
b.append(", ");
b.append("passwordPreviousPasswordBlockCount=");
b.append(this.passwordPreviousPasswordBlockCount);
b.append(", ");
b.append("passwordRequireActiveDirectoryComplexity=");
b.append(this.passwordRequireActiveDirectoryComplexity);
b.append(", ");
b.append("passwordRequirementsDescription=");
b.append(this.passwordRequirementsDescription);
b.append(", ");
b.append("realm=");
b.append(this.realm);
b.append(", ");
b.append("requireUserPresence=");
b.append(this.requireUserPresence);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}