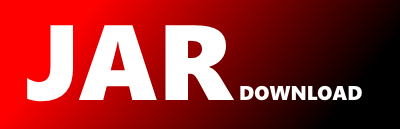
odata.msgraph.client.beta.complex.ManagedDeviceMobileAppConfigurationSettingState Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.enums.ComplianceStatus;
/**
* “Managed Device Mobile App Configuration Setting State for a given device.”
*/@JsonPropertyOrder({
"@odata.type",
"currentValue",
"errorCode",
"errorDescription",
"instanceDisplayName",
"setting",
"settingInstanceId",
"settingName",
"sources",
"state",
"userEmail",
"userId",
"userName",
"userPrincipalName"})
@JsonInclude(Include.NON_NULL)
public class ManagedDeviceMobileAppConfigurationSettingState implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("currentValue")
protected String currentValue;
@JsonProperty("errorCode")
protected Long errorCode;
@JsonProperty("errorDescription")
protected String errorDescription;
@JsonProperty("instanceDisplayName")
protected String instanceDisplayName;
@JsonProperty("setting")
protected String setting;
@JsonProperty("settingInstanceId")
protected String settingInstanceId;
@JsonProperty("settingName")
protected String settingName;
@JsonProperty("sources")
protected List sources;
@JsonProperty("sources@nextLink")
protected String sourcesNextLink;
@JsonProperty("state")
protected ComplianceStatus state;
@JsonProperty("userEmail")
protected String userEmail;
@JsonProperty("userId")
protected String userId;
@JsonProperty("userName")
protected String userName;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
protected ManagedDeviceMobileAppConfigurationSettingState() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.managedDeviceMobileAppConfigurationSettingState";
}
/**
* “Current value of setting on device”
*
* @return property currentValue
*/
@Property(name="currentValue")
@JsonIgnore
public Optional getCurrentValue() {
return Optional.ofNullable(currentValue);
}
/**
* Returns an immutable copy of {@code this} with just the {@code currentValue}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Current value of setting on device”
*
* @param currentValue
* new value of {@code currentValue} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code currentValue} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withCurrentValue(String currentValue) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.currentValue = currentValue;
return _x;
}
/**
* “Error code for the setting”
*
* @return property errorCode
*/
@Property(name="errorCode")
@JsonIgnore
public Optional getErrorCode() {
return Optional.ofNullable(errorCode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code errorCode} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Error code for the setting”
*
* @param errorCode
* new value of {@code errorCode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code errorCode} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withErrorCode(Long errorCode) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.errorCode = errorCode;
return _x;
}
/**
* “Error description”
*
* @return property errorDescription
*/
@Property(name="errorDescription")
@JsonIgnore
public Optional getErrorDescription() {
return Optional.ofNullable(errorDescription);
}
/**
* Returns an immutable copy of {@code this} with just the {@code errorDescription}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Error description”
*
* @param errorDescription
* new value of {@code errorDescription} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code errorDescription} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withErrorDescription(String errorDescription) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.errorDescription = errorDescription;
return _x;
}
/**
* “Name of setting instance that is being reported.”
*
* @return property instanceDisplayName
*/
@Property(name="instanceDisplayName")
@JsonIgnore
public Optional getInstanceDisplayName() {
return Optional.ofNullable(instanceDisplayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* instanceDisplayName} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Name of setting instance that is being reported.”
*
* @param instanceDisplayName
* new value of {@code instanceDisplayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code instanceDisplayName} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withInstanceDisplayName(String instanceDisplayName) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.instanceDisplayName = instanceDisplayName;
return _x;
}
/**
* “The setting that is being reported”
*
* @return property setting
*/
@Property(name="setting")
@JsonIgnore
public Optional getSetting() {
return Optional.ofNullable(setting);
}
/**
* Returns an immutable copy of {@code this} with just the {@code setting} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The setting that is being reported”
*
* @param setting
* new value of {@code setting} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code setting} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withSetting(String setting) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.setting = setting;
return _x;
}
/**
* “SettingInstanceId”
*
* @return property settingInstanceId
*/
@Property(name="settingInstanceId")
@JsonIgnore
public Optional getSettingInstanceId() {
return Optional.ofNullable(settingInstanceId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code settingInstanceId
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “SettingInstanceId”
*
* @param settingInstanceId
* new value of {@code settingInstanceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingInstanceId} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withSettingInstanceId(String settingInstanceId) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.settingInstanceId = settingInstanceId;
return _x;
}
/**
* “Localized/user friendly setting name that is being reported”
*
* @return property settingName
*/
@Property(name="settingName")
@JsonIgnore
public Optional getSettingName() {
return Optional.ofNullable(settingName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code settingName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Localized/user friendly setting name that is being reported”
*
* @param settingName
* new value of {@code settingName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingName} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withSettingName(String settingName) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.settingName = settingName;
return _x;
}
/**
* “Contributing policies”
*
* @return property sources
*/
@Property(name="sources")
@JsonIgnore
public CollectionPage getSources() {
return new CollectionPage(contextPath, SettingSource.class, this.sources, Optional.ofNullable(sourcesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* “Contributing policies”
*
* @param options
* specify connect and read timeouts
* @return property sources
*/
@Property(name="sources")
@JsonIgnore
public CollectionPage getSources(HttpRequestOptions options) {
return new CollectionPage(contextPath, SettingSource.class, this.sources, Optional.ofNullable(sourcesNextLink), Collections.emptyList(), options);
}
/**
* “The compliance state of the setting”
*
* @return property state
*/
@Property(name="state")
@JsonIgnore
public Optional getState() {
return Optional.ofNullable(state);
}
/**
* Returns an immutable copy of {@code this} with just the {@code state} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The compliance state of the setting”
*
* @param state
* new value of {@code state} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code state} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withState(ComplianceStatus state) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.state = state;
return _x;
}
/**
* “UserEmail”
*
* @return property userEmail
*/
@Property(name="userEmail")
@JsonIgnore
public Optional getUserEmail() {
return Optional.ofNullable(userEmail);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userEmail} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “UserEmail”
*
* @param userEmail
* new value of {@code userEmail} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userEmail} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withUserEmail(String userEmail) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.userEmail = userEmail;
return _x;
}
/**
* “UserId”
*
* @return property userId
*/
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “UserId”
*
* @param userId
* new value of {@code userId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userId} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withUserId(String userId) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.userId = userId;
return _x;
}
/**
* “UserName”
*
* @return property userName
*/
@Property(name="userName")
@JsonIgnore
public Optional getUserName() {
return Optional.ofNullable(userName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “UserName”
*
* @param userName
* new value of {@code userName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userName} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withUserName(String userName) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.userName = userName;
return _x;
}
/**
* “UserPrincipalName.”
*
* @return property userPrincipalName
*/
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userPrincipalName
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “UserPrincipalName.”
*
* @param userPrincipalName
* new value of {@code userPrincipalName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userPrincipalName} field changed
*/
public ManagedDeviceMobileAppConfigurationSettingState withUserPrincipalName(String userPrincipalName) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfigurationSettingState");
_x.userPrincipalName = userPrincipalName;
return _x;
}
public ManagedDeviceMobileAppConfigurationSettingState withUnmappedField(String name, String value) {
ManagedDeviceMobileAppConfigurationSettingState _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String currentValue;
private Long errorCode;
private String errorDescription;
private String instanceDisplayName;
private String setting;
private String settingInstanceId;
private String settingName;
private List sources;
private String sourcesNextLink;
private ComplianceStatus state;
private String userEmail;
private String userId;
private String userName;
private String userPrincipalName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Current value of setting on device”
*
* @param currentValue
* value of {@code currentValue} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder currentValue(String currentValue) {
this.currentValue = currentValue;
this.changedFields = changedFields.add("currentValue");
return this;
}
/**
* “Error code for the setting”
*
* @param errorCode
* value of {@code errorCode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder errorCode(Long errorCode) {
this.errorCode = errorCode;
this.changedFields = changedFields.add("errorCode");
return this;
}
/**
* “Error description”
*
* @param errorDescription
* value of {@code errorDescription} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder errorDescription(String errorDescription) {
this.errorDescription = errorDescription;
this.changedFields = changedFields.add("errorDescription");
return this;
}
/**
* “Name of setting instance that is being reported.”
*
* @param instanceDisplayName
* value of {@code instanceDisplayName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder instanceDisplayName(String instanceDisplayName) {
this.instanceDisplayName = instanceDisplayName;
this.changedFields = changedFields.add("instanceDisplayName");
return this;
}
/**
* “The setting that is being reported”
*
* @param setting
* value of {@code setting} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder setting(String setting) {
this.setting = setting;
this.changedFields = changedFields.add("setting");
return this;
}
/**
* “SettingInstanceId”
*
* @param settingInstanceId
* value of {@code settingInstanceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingInstanceId(String settingInstanceId) {
this.settingInstanceId = settingInstanceId;
this.changedFields = changedFields.add("settingInstanceId");
return this;
}
/**
* “Localized/user friendly setting name that is being reported”
*
* @param settingName
* value of {@code settingName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingName(String settingName) {
this.settingName = settingName;
this.changedFields = changedFields.add("settingName");
return this;
}
/**
* “Contributing policies”
*
* @param sources
* value of {@code sources} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder sources(List sources) {
this.sources = sources;
this.changedFields = changedFields.add("sources");
return this;
}
/**
* “Contributing policies”
*
* @param sources
* value of {@code sources} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder sources(SettingSource... sources) {
return sources(Arrays.asList(sources));
}
/**
* “Contributing policies”
*
* @param sourcesNextLink
* value of {@code sources@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder sourcesNextLink(String sourcesNextLink) {
this.sourcesNextLink = sourcesNextLink;
this.changedFields = changedFields.add("sources");
return this;
}
/**
* “The compliance state of the setting”
*
* @param state
* value of {@code state} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder state(ComplianceStatus state) {
this.state = state;
this.changedFields = changedFields.add("state");
return this;
}
/**
* “UserEmail”
*
* @param userEmail
* value of {@code userEmail} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userEmail(String userEmail) {
this.userEmail = userEmail;
this.changedFields = changedFields.add("userEmail");
return this;
}
/**
* “UserId”
*
* @param userId
* value of {@code userId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userId(String userId) {
this.userId = userId;
this.changedFields = changedFields.add("userId");
return this;
}
/**
* “UserName”
*
* @param userName
* value of {@code userName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userName(String userName) {
this.userName = userName;
this.changedFields = changedFields.add("userName");
return this;
}
/**
* “UserPrincipalName.”
*
* @param userPrincipalName
* value of {@code userPrincipalName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public ManagedDeviceMobileAppConfigurationSettingState build() {
ManagedDeviceMobileAppConfigurationSettingState _x = new ManagedDeviceMobileAppConfigurationSettingState();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.managedDeviceMobileAppConfigurationSettingState";
_x.currentValue = currentValue;
_x.errorCode = errorCode;
_x.errorDescription = errorDescription;
_x.instanceDisplayName = instanceDisplayName;
_x.setting = setting;
_x.settingInstanceId = settingInstanceId;
_x.settingName = settingName;
_x.sources = sources;
_x.sourcesNextLink = sourcesNextLink;
_x.state = state;
_x.userEmail = userEmail;
_x.userId = userId;
_x.userName = userName;
_x.userPrincipalName = userPrincipalName;
return _x;
}
}
private ManagedDeviceMobileAppConfigurationSettingState _copy() {
ManagedDeviceMobileAppConfigurationSettingState _x = new ManagedDeviceMobileAppConfigurationSettingState();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.currentValue = currentValue;
_x.errorCode = errorCode;
_x.errorDescription = errorDescription;
_x.instanceDisplayName = instanceDisplayName;
_x.setting = setting;
_x.settingInstanceId = settingInstanceId;
_x.settingName = settingName;
_x.sources = sources;
_x.state = state;
_x.userEmail = userEmail;
_x.userId = userId;
_x.userName = userName;
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedDeviceMobileAppConfigurationSettingState[");
b.append("currentValue=");
b.append(this.currentValue);
b.append(", ");
b.append("errorCode=");
b.append(this.errorCode);
b.append(", ");
b.append("errorDescription=");
b.append(this.errorDescription);
b.append(", ");
b.append("instanceDisplayName=");
b.append(this.instanceDisplayName);
b.append(", ");
b.append("setting=");
b.append(this.setting);
b.append(", ");
b.append("settingInstanceId=");
b.append(this.settingInstanceId);
b.append(", ");
b.append("settingName=");
b.append(this.settingName);
b.append(", ");
b.append("sources=");
b.append(this.sources);
b.append(", ");
b.append("state=");
b.append(this.state);
b.append(", ");
b.append("userEmail=");
b.append(this.userEmail);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append(", ");
b.append("userName=");
b.append(this.userName);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}