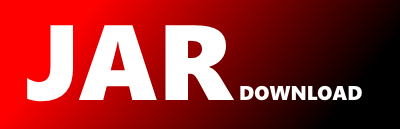
odata.msgraph.client.beta.complex.Win32LobAppRegistryRequirement Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.beta.enums.Win32LobAppDetectionOperator;
import odata.msgraph.client.beta.enums.Win32LobAppRegistryDetectionType;
/**
* “Contains registry properties to detect a Win32 App”
*/@JsonPropertyOrder({
"@odata.type",
"check32BitOn64System",
"detectionType",
"keyPath",
"valueName"})
@JsonInclude(Include.NON_NULL)
public class Win32LobAppRegistryRequirement extends Win32LobAppRequirement implements ODataType {
@JsonProperty("check32BitOn64System")
protected Boolean check32BitOn64System;
@JsonProperty("detectionType")
protected Win32LobAppRegistryDetectionType detectionType;
@JsonProperty("keyPath")
protected String keyPath;
@JsonProperty("valueName")
protected String valueName;
protected Win32LobAppRegistryRequirement() {
super();
}
@Override
public String odataTypeName() {
return "microsoft.graph.win32LobAppRegistryRequirement";
}
/**
* “A value indicating whether this registry path is for checking 32-bit app on 64-
* bit system”
*
* @return property check32BitOn64System
*/
@Property(name="check32BitOn64System")
@JsonIgnore
public Optional getCheck32BitOn64System() {
return Optional.ofNullable(check32BitOn64System);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* check32BitOn64System} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “A value indicating whether this registry path is for checking 32-bit app on 64-
* bit system”
*
* @param check32BitOn64System
* new value of {@code check32BitOn64System} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code check32BitOn64System} field changed
*/
public Win32LobAppRegistryRequirement withCheck32BitOn64System(Boolean check32BitOn64System) {
Win32LobAppRegistryRequirement _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.win32LobAppRegistryRequirement");
_x.check32BitOn64System = check32BitOn64System;
return _x;
}
/**
* “The registry data detection type”
*
* @return property detectionType
*/
@Property(name="detectionType")
@JsonIgnore
public Optional getDetectionType() {
return Optional.ofNullable(detectionType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code detectionType}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The registry data detection type”
*
* @param detectionType
* new value of {@code detectionType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code detectionType} field changed
*/
public Win32LobAppRegistryRequirement withDetectionType(Win32LobAppRegistryDetectionType detectionType) {
Win32LobAppRegistryRequirement _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.win32LobAppRegistryRequirement");
_x.detectionType = detectionType;
return _x;
}
/**
* “The registry key path to detect Win32 Line of Business (LoB) app”
*
* @return property keyPath
*/
@Property(name="keyPath")
@JsonIgnore
public Optional getKeyPath() {
return Optional.ofNullable(keyPath);
}
/**
* Returns an immutable copy of {@code this} with just the {@code keyPath} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The registry key path to detect Win32 Line of Business (LoB) app”
*
* @param keyPath
* new value of {@code keyPath} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code keyPath} field changed
*/
public Win32LobAppRegistryRequirement withKeyPath(String keyPath) {
Win32LobAppRegistryRequirement _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.win32LobAppRegistryRequirement");
_x.keyPath = keyPath;
return _x;
}
/**
* “The registry value name”
*
* @return property valueName
*/
@Property(name="valueName")
@JsonIgnore
public Optional getValueName() {
return Optional.ofNullable(valueName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code valueName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The registry value name”
*
* @param valueName
* new value of {@code valueName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code valueName} field changed
*/
public Win32LobAppRegistryRequirement withValueName(String valueName) {
Win32LobAppRegistryRequirement _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.win32LobAppRegistryRequirement");
_x.valueName = valueName;
return _x;
}
public Win32LobAppRegistryRequirement withUnmappedField(String name, String value) {
Win32LobAppRegistryRequirement _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWin32LobAppRegistryRequirement() {
return new Builder();
}
public static final class Builder {
private String detectionValue;
private Win32LobAppDetectionOperator operator;
private Boolean check32BitOn64System;
private Win32LobAppRegistryDetectionType detectionType;
private String keyPath;
private String valueName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder detectionValue(String detectionValue) {
this.detectionValue = detectionValue;
this.changedFields = changedFields.add("detectionValue");
return this;
}
public Builder operator(Win32LobAppDetectionOperator operator) {
this.operator = operator;
this.changedFields = changedFields.add("operator");
return this;
}
/**
* “A value indicating whether this registry path is for checking 32-bit app on 64-
* bit system”
*
* @param check32BitOn64System
* value of {@code check32BitOn64System} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder check32BitOn64System(Boolean check32BitOn64System) {
this.check32BitOn64System = check32BitOn64System;
this.changedFields = changedFields.add("check32BitOn64System");
return this;
}
/**
* “The registry data detection type”
*
* @param detectionType
* value of {@code detectionType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder detectionType(Win32LobAppRegistryDetectionType detectionType) {
this.detectionType = detectionType;
this.changedFields = changedFields.add("detectionType");
return this;
}
/**
* “The registry key path to detect Win32 Line of Business (LoB) app”
*
* @param keyPath
* value of {@code keyPath} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder keyPath(String keyPath) {
this.keyPath = keyPath;
this.changedFields = changedFields.add("keyPath");
return this;
}
/**
* “The registry value name”
*
* @param valueName
* value of {@code valueName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder valueName(String valueName) {
this.valueName = valueName;
this.changedFields = changedFields.add("valueName");
return this;
}
public Win32LobAppRegistryRequirement build() {
Win32LobAppRegistryRequirement _x = new Win32LobAppRegistryRequirement();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.win32LobAppRegistryRequirement";
_x.detectionValue = detectionValue;
_x.operator = operator;
_x.check32BitOn64System = check32BitOn64System;
_x.detectionType = detectionType;
_x.keyPath = keyPath;
_x.valueName = valueName;
return _x;
}
}
private Win32LobAppRegistryRequirement _copy() {
Win32LobAppRegistryRequirement _x = new Win32LobAppRegistryRequirement();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.detectionValue = detectionValue;
_x.operator = operator;
_x.check32BitOn64System = check32BitOn64System;
_x.detectionType = detectionType;
_x.keyPath = keyPath;
_x.valueName = valueName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Win32LobAppRegistryRequirement[");
b.append("detectionValue=");
b.append(this.detectionValue);
b.append(", ");
b.append("operator=");
b.append(this.operator);
b.append(", ");
b.append("check32BitOn64System=");
b.append(this.check32BitOn64System);
b.append(", ");
b.append("detectionType=");
b.append(this.detectionType);
b.append(", ");
b.append("keyPath=");
b.append(this.keyPath);
b.append(", ");
b.append("valueName=");
b.append(this.valueName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}