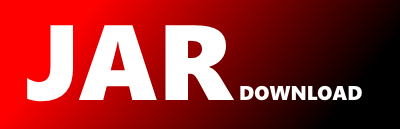
odata.msgraph.client.beta.complex.WindowsMinimumOperatingSystem Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “The minimum operating system required for a Windows mobile app.”
*/@JsonPropertyOrder({
"@odata.type",
"v10_0",
"v10_1607",
"v10_1703",
"v10_1709",
"v10_1803",
"v10_1809",
"v10_1903",
"v10_1909",
"v10_2004",
"v10_21H1",
"v10_2H20",
"v8_0",
"v8_1"})
@JsonInclude(Include.NON_NULL)
public class WindowsMinimumOperatingSystem implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("v10_0")
protected Boolean v10_0;
@JsonProperty("v10_1607")
protected Boolean v10_1607;
@JsonProperty("v10_1703")
protected Boolean v10_1703;
@JsonProperty("v10_1709")
protected Boolean v10_1709;
@JsonProperty("v10_1803")
protected Boolean v10_1803;
@JsonProperty("v10_1809")
protected Boolean v10_1809;
@JsonProperty("v10_1903")
protected Boolean v10_1903;
@JsonProperty("v10_1909")
protected Boolean v10_1909;
@JsonProperty("v10_2004")
protected Boolean v10_2004;
@JsonProperty("v10_21H1")
protected Boolean v10_21H1;
@JsonProperty("v10_2H20")
protected Boolean v10_2H20;
@JsonProperty("v8_0")
protected Boolean v8_0;
@JsonProperty("v8_1")
protected Boolean v8_1;
protected WindowsMinimumOperatingSystem() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.windowsMinimumOperatingSystem";
}
/**
* “Windows version 10.0 or later.”
*
* @return property v10_0
*/
@Property(name="v10_0")
@JsonIgnore
public Optional getV10_0() {
return Optional.ofNullable(v10_0);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_0} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows version 10.0 or later.”
*
* @param v10_0
* new value of {@code v10_0} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_0} field changed
*/
public WindowsMinimumOperatingSystem withV10_0(Boolean v10_0) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_0 = v10_0;
return _x;
}
/**
* “Windows 10 1607 or later.”
*
* @return property v10_1607
*/
@Property(name="v10_1607")
@JsonIgnore
public Optional getV10_1607() {
return Optional.ofNullable(v10_1607);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1607} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1607 or later.”
*
* @param v10_1607
* new value of {@code v10_1607} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1607} field changed
*/
public WindowsMinimumOperatingSystem withV10_1607(Boolean v10_1607) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1607 = v10_1607;
return _x;
}
/**
* “Windows 10 1703 or later.”
*
* @return property v10_1703
*/
@Property(name="v10_1703")
@JsonIgnore
public Optional getV10_1703() {
return Optional.ofNullable(v10_1703);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1703} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1703 or later.”
*
* @param v10_1703
* new value of {@code v10_1703} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1703} field changed
*/
public WindowsMinimumOperatingSystem withV10_1703(Boolean v10_1703) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1703 = v10_1703;
return _x;
}
/**
* “Windows 10 1709 or later.”
*
* @return property v10_1709
*/
@Property(name="v10_1709")
@JsonIgnore
public Optional getV10_1709() {
return Optional.ofNullable(v10_1709);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1709} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1709 or later.”
*
* @param v10_1709
* new value of {@code v10_1709} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1709} field changed
*/
public WindowsMinimumOperatingSystem withV10_1709(Boolean v10_1709) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1709 = v10_1709;
return _x;
}
/**
* “Windows 10 1803 or later.”
*
* @return property v10_1803
*/
@Property(name="v10_1803")
@JsonIgnore
public Optional getV10_1803() {
return Optional.ofNullable(v10_1803);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1803} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1803 or later.”
*
* @param v10_1803
* new value of {@code v10_1803} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1803} field changed
*/
public WindowsMinimumOperatingSystem withV10_1803(Boolean v10_1803) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1803 = v10_1803;
return _x;
}
/**
* “Windows 10 1809 or later.”
*
* @return property v10_1809
*/
@Property(name="v10_1809")
@JsonIgnore
public Optional getV10_1809() {
return Optional.ofNullable(v10_1809);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1809} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1809 or later.”
*
* @param v10_1809
* new value of {@code v10_1809} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1809} field changed
*/
public WindowsMinimumOperatingSystem withV10_1809(Boolean v10_1809) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1809 = v10_1809;
return _x;
}
/**
* “Windows 10 1903 or later.”
*
* @return property v10_1903
*/
@Property(name="v10_1903")
@JsonIgnore
public Optional getV10_1903() {
return Optional.ofNullable(v10_1903);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1903} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1903 or later.”
*
* @param v10_1903
* new value of {@code v10_1903} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1903} field changed
*/
public WindowsMinimumOperatingSystem withV10_1903(Boolean v10_1903) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1903 = v10_1903;
return _x;
}
/**
* “Windows 10 1909 or later.”
*
* @return property v10_1909
*/
@Property(name="v10_1909")
@JsonIgnore
public Optional getV10_1909() {
return Optional.ofNullable(v10_1909);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_1909} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 1909 or later.”
*
* @param v10_1909
* new value of {@code v10_1909} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_1909} field changed
*/
public WindowsMinimumOperatingSystem withV10_1909(Boolean v10_1909) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_1909 = v10_1909;
return _x;
}
/**
* “Windows 10 2004 or later.”
*
* @return property v10_2004
*/
@Property(name="v10_2004")
@JsonIgnore
public Optional getV10_2004() {
return Optional.ofNullable(v10_2004);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_2004} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 2004 or later.”
*
* @param v10_2004
* new value of {@code v10_2004} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_2004} field changed
*/
public WindowsMinimumOperatingSystem withV10_2004(Boolean v10_2004) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_2004 = v10_2004;
return _x;
}
/**
* “Windows 10 21H1 or later.”
*
* @return property v10_21H1
*/
@Property(name="v10_21H1")
@JsonIgnore
public Optional getV10_21H1() {
return Optional.ofNullable(v10_21H1);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_21H1} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 21H1 or later.”
*
* @param v10_21H1
* new value of {@code v10_21H1} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_21H1} field changed
*/
public WindowsMinimumOperatingSystem withV10_21H1(Boolean v10_21H1) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_21H1 = v10_21H1;
return _x;
}
/**
* “Windows 10 2H20 or later.”
*
* @return property v10_2H20
*/
@Property(name="v10_2H20")
@JsonIgnore
public Optional getV10_2H20() {
return Optional.ofNullable(v10_2H20);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_2H20} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows 10 2H20 or later.”
*
* @param v10_2H20
* new value of {@code v10_2H20} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_2H20} field changed
*/
public WindowsMinimumOperatingSystem withV10_2H20(Boolean v10_2H20) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v10_2H20 = v10_2H20;
return _x;
}
/**
* “Windows version 8.0 or later.”
*
* @return property v8_0
*/
@Property(name="v8_0")
@JsonIgnore
public Optional getV8_0() {
return Optional.ofNullable(v8_0);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v8_0} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows version 8.0 or later.”
*
* @param v8_0
* new value of {@code v8_0} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v8_0} field changed
*/
public WindowsMinimumOperatingSystem withV8_0(Boolean v8_0) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v8_0 = v8_0;
return _x;
}
/**
* “Windows version 8.1 or later.”
*
* @return property v8_1
*/
@Property(name="v8_1")
@JsonIgnore
public Optional getV8_1() {
return Optional.ofNullable(v8_1);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v8_1} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Windows version 8.1 or later.”
*
* @param v8_1
* new value of {@code v8_1} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v8_1} field changed
*/
public WindowsMinimumOperatingSystem withV8_1(Boolean v8_1) {
WindowsMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsMinimumOperatingSystem");
_x.v8_1 = v8_1;
return _x;
}
public WindowsMinimumOperatingSystem withUnmappedField(String name, String value) {
WindowsMinimumOperatingSystem _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Boolean v10_0;
private Boolean v10_1607;
private Boolean v10_1703;
private Boolean v10_1709;
private Boolean v10_1803;
private Boolean v10_1809;
private Boolean v10_1903;
private Boolean v10_1909;
private Boolean v10_2004;
private Boolean v10_21H1;
private Boolean v10_2H20;
private Boolean v8_0;
private Boolean v8_1;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Windows version 10.0 or later.”
*
* @param v10_0
* value of {@code v10_0} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_0(Boolean v10_0) {
this.v10_0 = v10_0;
this.changedFields = changedFields.add("v10_0");
return this;
}
/**
* “Windows 10 1607 or later.”
*
* @param v10_1607
* value of {@code v10_1607} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1607(Boolean v10_1607) {
this.v10_1607 = v10_1607;
this.changedFields = changedFields.add("v10_1607");
return this;
}
/**
* “Windows 10 1703 or later.”
*
* @param v10_1703
* value of {@code v10_1703} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1703(Boolean v10_1703) {
this.v10_1703 = v10_1703;
this.changedFields = changedFields.add("v10_1703");
return this;
}
/**
* “Windows 10 1709 or later.”
*
* @param v10_1709
* value of {@code v10_1709} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1709(Boolean v10_1709) {
this.v10_1709 = v10_1709;
this.changedFields = changedFields.add("v10_1709");
return this;
}
/**
* “Windows 10 1803 or later.”
*
* @param v10_1803
* value of {@code v10_1803} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1803(Boolean v10_1803) {
this.v10_1803 = v10_1803;
this.changedFields = changedFields.add("v10_1803");
return this;
}
/**
* “Windows 10 1809 or later.”
*
* @param v10_1809
* value of {@code v10_1809} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1809(Boolean v10_1809) {
this.v10_1809 = v10_1809;
this.changedFields = changedFields.add("v10_1809");
return this;
}
/**
* “Windows 10 1903 or later.”
*
* @param v10_1903
* value of {@code v10_1903} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1903(Boolean v10_1903) {
this.v10_1903 = v10_1903;
this.changedFields = changedFields.add("v10_1903");
return this;
}
/**
* “Windows 10 1909 or later.”
*
* @param v10_1909
* value of {@code v10_1909} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_1909(Boolean v10_1909) {
this.v10_1909 = v10_1909;
this.changedFields = changedFields.add("v10_1909");
return this;
}
/**
* “Windows 10 2004 or later.”
*
* @param v10_2004
* value of {@code v10_2004} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_2004(Boolean v10_2004) {
this.v10_2004 = v10_2004;
this.changedFields = changedFields.add("v10_2004");
return this;
}
/**
* “Windows 10 21H1 or later.”
*
* @param v10_21H1
* value of {@code v10_21H1} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_21H1(Boolean v10_21H1) {
this.v10_21H1 = v10_21H1;
this.changedFields = changedFields.add("v10_21H1");
return this;
}
/**
* “Windows 10 2H20 or later.”
*
* @param v10_2H20
* value of {@code v10_2H20} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_2H20(Boolean v10_2H20) {
this.v10_2H20 = v10_2H20;
this.changedFields = changedFields.add("v10_2H20");
return this;
}
/**
* “Windows version 8.0 or later.”
*
* @param v8_0
* value of {@code v8_0} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v8_0(Boolean v8_0) {
this.v8_0 = v8_0;
this.changedFields = changedFields.add("v8_0");
return this;
}
/**
* “Windows version 8.1 or later.”
*
* @param v8_1
* value of {@code v8_1} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v8_1(Boolean v8_1) {
this.v8_1 = v8_1;
this.changedFields = changedFields.add("v8_1");
return this;
}
public WindowsMinimumOperatingSystem build() {
WindowsMinimumOperatingSystem _x = new WindowsMinimumOperatingSystem();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsMinimumOperatingSystem";
_x.v10_0 = v10_0;
_x.v10_1607 = v10_1607;
_x.v10_1703 = v10_1703;
_x.v10_1709 = v10_1709;
_x.v10_1803 = v10_1803;
_x.v10_1809 = v10_1809;
_x.v10_1903 = v10_1903;
_x.v10_1909 = v10_1909;
_x.v10_2004 = v10_2004;
_x.v10_21H1 = v10_21H1;
_x.v10_2H20 = v10_2H20;
_x.v8_0 = v8_0;
_x.v8_1 = v8_1;
return _x;
}
}
private WindowsMinimumOperatingSystem _copy() {
WindowsMinimumOperatingSystem _x = new WindowsMinimumOperatingSystem();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.v10_0 = v10_0;
_x.v10_1607 = v10_1607;
_x.v10_1703 = v10_1703;
_x.v10_1709 = v10_1709;
_x.v10_1803 = v10_1803;
_x.v10_1809 = v10_1809;
_x.v10_1903 = v10_1903;
_x.v10_1909 = v10_1909;
_x.v10_2004 = v10_2004;
_x.v10_21H1 = v10_21H1;
_x.v10_2H20 = v10_2H20;
_x.v8_0 = v8_0;
_x.v8_1 = v8_1;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsMinimumOperatingSystem[");
b.append("v10_0=");
b.append(this.v10_0);
b.append(", ");
b.append("v10_1607=");
b.append(this.v10_1607);
b.append(", ");
b.append("v10_1703=");
b.append(this.v10_1703);
b.append(", ");
b.append("v10_1709=");
b.append(this.v10_1709);
b.append(", ");
b.append("v10_1803=");
b.append(this.v10_1803);
b.append(", ");
b.append("v10_1809=");
b.append(this.v10_1809);
b.append(", ");
b.append("v10_1903=");
b.append(this.v10_1903);
b.append(", ");
b.append("v10_1909=");
b.append(this.v10_1909);
b.append(", ");
b.append("v10_2004=");
b.append(this.v10_2004);
b.append(", ");
b.append("v10_21H1=");
b.append(this.v10_21H1);
b.append(", ");
b.append("v10_2H20=");
b.append(this.v10_2H20);
b.append(", ");
b.append("v8_0=");
b.append(this.v8_0);
b.append(", ");
b.append("v8_1=");
b.append(this.v8_1);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}