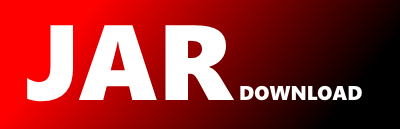
odata.msgraph.client.beta.complex.WindowsPackageInformation Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.beta.enums.WindowsArchitecture;
/**
* “Contains properties for the package information for a Windows line of business
* app.”
*/@JsonPropertyOrder({
"@odata.type",
"applicableArchitecture",
"displayName",
"identityName",
"identityPublisher",
"identityResourceIdentifier",
"identityVersion",
"minimumSupportedOperatingSystem"})
@JsonInclude(Include.NON_NULL)
public class WindowsPackageInformation implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("applicableArchitecture")
protected WindowsArchitecture applicableArchitecture;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("identityName")
protected String identityName;
@JsonProperty("identityPublisher")
protected String identityPublisher;
@JsonProperty("identityResourceIdentifier")
protected String identityResourceIdentifier;
@JsonProperty("identityVersion")
protected String identityVersion;
@JsonProperty("minimumSupportedOperatingSystem")
protected WindowsMinimumOperatingSystem minimumSupportedOperatingSystem;
protected WindowsPackageInformation() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.windowsPackageInformation";
}
/**
* “The Windows architecture for which this app can run on.”
*
* @return property applicableArchitecture
*/
@Property(name="applicableArchitecture")
@JsonIgnore
public Optional getApplicableArchitecture() {
return Optional.ofNullable(applicableArchitecture);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* applicableArchitecture} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The Windows architecture for which this app can run on.”
*
* @param applicableArchitecture
* new value of {@code applicableArchitecture} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code applicableArchitecture} field changed
*/
public WindowsPackageInformation withApplicableArchitecture(WindowsArchitecture applicableArchitecture) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.applicableArchitecture = applicableArchitecture;
return _x;
}
/**
* “The Display Name.”
*
* @return property displayName
*/
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code displayName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Display Name.”
*
* @param displayName
* new value of {@code displayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code displayName} field changed
*/
public WindowsPackageInformation withDisplayName(String displayName) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.displayName = displayName;
return _x;
}
/**
* “The Identity Name.”
*
* @return property identityName
*/
@Property(name="identityName")
@JsonIgnore
public Optional getIdentityName() {
return Optional.ofNullable(identityName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code identityName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Identity Name.”
*
* @param identityName
* new value of {@code identityName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityName} field changed
*/
public WindowsPackageInformation withIdentityName(String identityName) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.identityName = identityName;
return _x;
}
/**
* “The Identity Publisher.”
*
* @return property identityPublisher
*/
@Property(name="identityPublisher")
@JsonIgnore
public Optional getIdentityPublisher() {
return Optional.ofNullable(identityPublisher);
}
/**
* Returns an immutable copy of {@code this} with just the {@code identityPublisher
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Identity Publisher.”
*
* @param identityPublisher
* new value of {@code identityPublisher} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityPublisher} field changed
*/
public WindowsPackageInformation withIdentityPublisher(String identityPublisher) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.identityPublisher = identityPublisher;
return _x;
}
/**
* “The Identity Resource Identifier.”
*
* @return property identityResourceIdentifier
*/
@Property(name="identityResourceIdentifier")
@JsonIgnore
public Optional getIdentityResourceIdentifier() {
return Optional.ofNullable(identityResourceIdentifier);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* identityResourceIdentifier} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The Identity Resource Identifier.”
*
* @param identityResourceIdentifier
* new value of {@code identityResourceIdentifier} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityResourceIdentifier} field changed
*/
public WindowsPackageInformation withIdentityResourceIdentifier(String identityResourceIdentifier) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.identityResourceIdentifier = identityResourceIdentifier;
return _x;
}
/**
* “The Identity Version.”
*
* @return property identityVersion
*/
@Property(name="identityVersion")
@JsonIgnore
public Optional getIdentityVersion() {
return Optional.ofNullable(identityVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code identityVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Identity Version.”
*
* @param identityVersion
* new value of {@code identityVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityVersion} field changed
*/
public WindowsPackageInformation withIdentityVersion(String identityVersion) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.identityVersion = identityVersion;
return _x;
}
/**
* “The value for the minimum applicable operating system.”
*
* @return property minimumSupportedOperatingSystem
*/
@Property(name="minimumSupportedOperatingSystem")
@JsonIgnore
public Optional getMinimumSupportedOperatingSystem() {
return Optional.ofNullable(minimumSupportedOperatingSystem);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumSupportedOperatingSystem} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The value for the minimum applicable operating system.”
*
* @param minimumSupportedOperatingSystem
* new value of {@code minimumSupportedOperatingSystem} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumSupportedOperatingSystem} field changed
*/
public WindowsPackageInformation withMinimumSupportedOperatingSystem(WindowsMinimumOperatingSystem minimumSupportedOperatingSystem) {
WindowsPackageInformation _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPackageInformation");
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
public WindowsPackageInformation withUnmappedField(String name, String value) {
WindowsPackageInformation _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private WindowsArchitecture applicableArchitecture;
private String displayName;
private String identityName;
private String identityPublisher;
private String identityResourceIdentifier;
private String identityVersion;
private WindowsMinimumOperatingSystem minimumSupportedOperatingSystem;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “The Windows architecture for which this app can run on.”
*
* @param applicableArchitecture
* value of {@code applicableArchitecture} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder applicableArchitecture(WindowsArchitecture applicableArchitecture) {
this.applicableArchitecture = applicableArchitecture;
this.changedFields = changedFields.add("applicableArchitecture");
return this;
}
/**
* “The Display Name.”
*
* @param displayName
* value of {@code displayName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
/**
* “The Identity Name.”
*
* @param identityName
* value of {@code identityName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityName(String identityName) {
this.identityName = identityName;
this.changedFields = changedFields.add("identityName");
return this;
}
/**
* “The Identity Publisher.”
*
* @param identityPublisher
* value of {@code identityPublisher} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityPublisher(String identityPublisher) {
this.identityPublisher = identityPublisher;
this.changedFields = changedFields.add("identityPublisher");
return this;
}
/**
* “The Identity Resource Identifier.”
*
* @param identityResourceIdentifier
* value of {@code identityResourceIdentifier} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityResourceIdentifier(String identityResourceIdentifier) {
this.identityResourceIdentifier = identityResourceIdentifier;
this.changedFields = changedFields.add("identityResourceIdentifier");
return this;
}
/**
* “The Identity Version.”
*
* @param identityVersion
* value of {@code identityVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityVersion(String identityVersion) {
this.identityVersion = identityVersion;
this.changedFields = changedFields.add("identityVersion");
return this;
}
/**
* “The value for the minimum applicable operating system.”
*
* @param minimumSupportedOperatingSystem
* value of {@code minimumSupportedOperatingSystem} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder minimumSupportedOperatingSystem(WindowsMinimumOperatingSystem minimumSupportedOperatingSystem) {
this.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
this.changedFields = changedFields.add("minimumSupportedOperatingSystem");
return this;
}
public WindowsPackageInformation build() {
WindowsPackageInformation _x = new WindowsPackageInformation();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsPackageInformation";
_x.applicableArchitecture = applicableArchitecture;
_x.displayName = displayName;
_x.identityName = identityName;
_x.identityPublisher = identityPublisher;
_x.identityResourceIdentifier = identityResourceIdentifier;
_x.identityVersion = identityVersion;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
}
private WindowsPackageInformation _copy() {
WindowsPackageInformation _x = new WindowsPackageInformation();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.applicableArchitecture = applicableArchitecture;
_x.displayName = displayName;
_x.identityName = identityName;
_x.identityPublisher = identityPublisher;
_x.identityResourceIdentifier = identityResourceIdentifier;
_x.identityVersion = identityVersion;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsPackageInformation[");
b.append("applicableArchitecture=");
b.append(this.applicableArchitecture);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("identityName=");
b.append(this.identityName);
b.append(", ");
b.append("identityPublisher=");
b.append(this.identityPublisher);
b.append(", ");
b.append("identityResourceIdentifier=");
b.append(this.identityResourceIdentifier);
b.append(", ");
b.append("identityVersion=");
b.append(this.identityVersion);
b.append(", ");
b.append("minimumSupportedOperatingSystem=");
b.append(this.minimumSupportedOperatingSystem);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}